A typed async client for the [taginfo] API, a system for finding and aggregating
information about [OpenStreetMap]'s [tags], and making it browsable and searchable.
This library makes use of [aiohttp] for requests, and [Pydantic] for parsing
and validating the responses.
[taginfo]: https://taginfo.openstreetmap.org
[OpenStreetMap]: https://www.openstreetmap.org
[tags]: https://wiki.openstreetmap.org/wiki/Tags
[aiohttp]: https://docs.aiohttp.org/
[Pydantic]: https://pydantic.dev/
#### Contents
- [Rationale](#rationale)
- [Usage](#usage)
- [Endpoints](#endpoints)
#### See also
- An overview of modules, classes and functions can be found in the [API reference](https://www.timwie.dev/aio-taginfo/)
- The version history is available in [RELEASES.md](https://github.com/timwie/aio-taginfo/blob/main/RELEASES.md)
- The [taginfo website](https://taginfo.openstreetmap.org/),
its [API documentation](https://taginfo.openstreetmap.org/taginfo/apidoc),
its [OSM Wiki page](https://wiki.openstreetmap.org/wiki/Taginfo),
and its [repository](https://github.com/taginfo/taginfo)
<br>
## Rationale
A tag consists of two items, a key and a value. For instance,
[`highway=residential`](https://wiki.openstreetmap.org/wiki/Tag:highway%3Dresidential)
is a tag with a key of [`highway`](https://wiki.openstreetmap.org/wiki/Key:highway)
and a value of `residential`, which should be used on a
[way](https://wiki.openstreetmap.org/wiki/Way) to indicate a road along which people live.
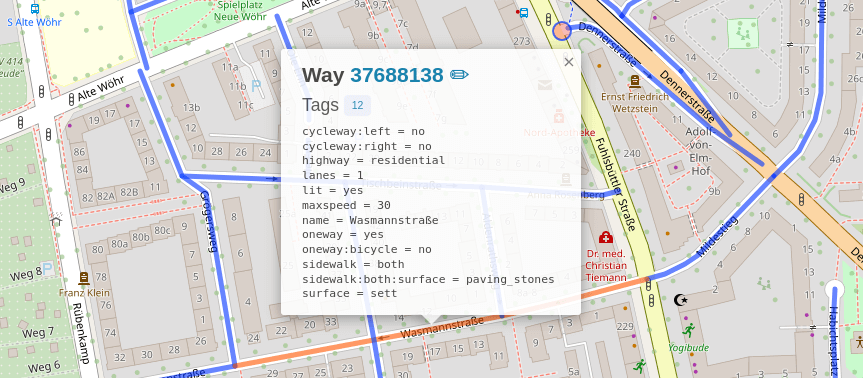
Now, why is there need for the taginfo API?
> OpenStreetMap uses tags to add meaning to geographic objects. There is no fixed
> list of those tags. New tags can be invented and used as needed. Everybody can
> come up with a new tag and add it to new or existing objects. This makes
> OpenStreetMap enormously flexible, but sometimes also a bit hard to work with.
>
> Whether you are contributing to OSM or using the OSM data, there are always
> questions like: What tags do people use for feature X? What tags can I use for
> feature Y so that it appears properly on the map? Is the tag Z described on the
> wiki actually in use and where?
>
> Taginfo helps you by showing statistics about which tags are actually in the
> database, how many people use those tags, where they are used and so on. It also
> gets information about tags from the wiki and from other places. Taginfo tries
> to bring together all information about tags to help you understand how they are
> used and what they mean.
<br>
## Usage
Before using this library, please be aware of the general rules when using the taginfo API:
> Taginfo has an API that lets you access the contents of its databases in several
> ways. The API is used internally for the web user interface and can also be used
> by anybody who wants to integrate taginfo data into their websites or
> applications.
> The API is intended for the use of the OpenStreetMap community. Do not use it
> for other services. If you are not sure, ask on the mailing list (see below).
>
> Always use a sensible User-agent header with enough information that we can
> contact you if there is a problem.
>
> The server running the taginfo API does not have unlimited resources. Please use
> the API responsibly. Do not create huge amounts of requests to get the whole
> database or large chunks of it, instead use the [database downloads] provided.
> If you are using the API and you find it is slow, you are probably overusing it.
>
> If you are using the taginfo API it is recommended that you join the
> [taginfo-dev mailing list]. Updates to the API will be announced there and this
> is also the right place for your questions.
[database downloads]: https://taginfo.openstreetmap.org/download
[taginfo-dev mailing list]: https://lists.openstreetmap.org/listinfo/taginfo-dev
The data available through taginfo is licenced under [ODbL],
the same license as the OpenStreetMap data.
> OpenStreetMap[®] is open data, licensed under the
> [Open Data Commons Open Database License] (ODbL)
> by the [OpenStreetMap Foundation] (OSMF).
>
> You are free to copy, distribute, transmit and adapt our data, as long as you
> credit OpenStreetMap and its contributors. If you alter or build upon our data,
> you may distribute the result only under the same licence. The full [legal code]
> explains your rights and responsibilities.
[ODbL]: https://www.openstreetmap.org/copyright/en
[®]: https://www.openstreetmap.org/copyright/en#trademarks
[Open Data Commons Open Database License]: https://opendatacommons.org/licenses/odbl/
[OpenStreetMap Foundation]: https://osmfoundation.org/
[legal code]: https://opendatacommons.org/licenses/odbl/1.0/
### Example
Here is an example of an API request using this library:
```python
from aio_taginfo import key_overview
# either use a temporary session…
response: Response[KeyOverview] = await key_overview(key="amenity")
# …or provide your own
headers = {"User-Agent": "your contact info"}
async with aiohttp.ClientSession(headers=headers) as session:
response: Response[KeyOverview] = await key_overview(key="amenity", session=session)
```
Most endpoints will return a [`Response[T]`](https://www.timwie.dev/aio-taginfo/aio_taginfo/api/v4.html#Response),
or `Response[list[T]]` for those returning multiple or paginated items.
<br>
## Endpoints
This library is in early development and most endpoints are still missing.
| | Endpoint | Schema |
|--:|--------------------------------------|--------------------------------|
| ✅ | `/api/4/key/chronology` | `Response[list[T]](page=None)` |
| ✅ | `/api/4/key/combinations` | `Response[list[T]]` |
| ✅ | `/api/4/key/distribution/nodes` | `PngResponse` |
| ✅ | `/api/4/key/distribution/ways` | `PngResponse` |
| ✅ | `/api/4/key/overview` | `Response[T]` |
| ✅ | `/api/4/key/prevalent_values` | `Response[list[T]](page=None)` |
| ✅ | `/api/4/key/projects` | `Response[list[T]]` |
| ✅ | `/api/4/key/similar` | `Response[list[T]]` |
| ✅ | `/api/4/key/stats` | `Response[list[T]](page=None)` |
| | `/api/4/key/values` | `Response[list[T]]` |
| | `/api/4/key/wiki_pages` | `Response[list[T]](page=None)` |
| | `/api/4/keys/all` | `Response[list[T]]` |
| | `/api/4/keys/similar` | `Response[list[T]]` |
| | `/api/4/keys/wiki_pages` | `Response[list[T]]` |
| | `/api/4/keys/without_wiki_page` | `Response[list[T]]` |
| | `/api/4/project/icon` | `PngResponse` |
| | `/api/4/project/tags` | `Response[list[T]]` |
| | `/api/4/projects/all` | `Response[list[T]]` |
| | `/api/4/projects/keys` | `Response[list[T]]` |
| | `/api/4/projects/tags` | `Response[list[T]]` |
| ✅ | `/api/4/relation/projects` | `Response[list[T]]` |
| | `/api/4/relation/roles` | `Response[list[T]]` |
| | `/api/4/relation/stats` | `Response[list[T]](page=None)` |
| | `/api/4/relation/wiki_pages` | `Response[list[T]](page=None)` |
| | `/api/4/relations/all` | `Response[list[T]]` |
| | `/api/4/search/by_key_and_value` | `Response[list[T]]` |
| | `/api/4/search/by_keyword` | `Response[list[T]]` |
| | `/api/4/search/by_role` | `Response[list[T]]` |
| | `/api/4/search/by_value` | `Response[list[T]]` |
| ✅ | `/api/4/site/config/geodistribution` | `T` |
| | `/api/4/site/info` | `T` |
| | `/api/4/site/sources` | `T` |
| | `/api/4/tag/chronology` | `Response[list[T]](page=None)` |
| | `/api/4/tag/combinations` | `Response[list[T]]` |
| | `/api/4/tag/distribution/nodes` | `PngResponse` |
| | `/api/4/tag/distribution/ways` | `PngResponse` |
| | `/api/4/tag/overview` | `Response[T]` |
| ✅ | `/api/4/tag/projects` | `Response[list[T]]` |
| | `/api/4/tag/stats` | `Response[list[T]](page=None)` |
| | `/api/4/tag/wiki_pages` | `Response[list[T]](page=None)` |
| | `/api/4/tags/list` | `Response[list[T]](page=None)` |
| ✅ | `/api/4/tags/popular` | `Response[list[T]]` |
| | `/api/4/unicode/characters` | `Response[list[T]](page=None)` |
| | `/api/4/wiki/languages` | `Response[list[T]](page=None)` |
Raw data
{
"_id": null,
"home_page": "https://github.com/timwie/aio-taginfo",
"name": "aio-taginfo",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.10",
"maintainer_email": null,
"keywords": "geospatial, gis, openstreetmap, osm, taginfo, spatial-analysis, spatial-data",
"author": "Tim Wiechers",
"author_email": "mail@timwie.dev",
"download_url": "https://files.pythonhosted.org/packages/ca/eb/a63c78f43b1887a26832493ac85b3d5365c82541d2b02e959dbf379b4c12/aio_taginfo-0.4.0.tar.gz",
"platform": null,
"description": "A typed async client for the [taginfo] API, a system for finding and aggregating\ninformation about [OpenStreetMap]'s [tags], and making it browsable and searchable.\n\nThis library makes use of [aiohttp] for requests, and [Pydantic] for parsing\nand validating the responses.\n\n[taginfo]: https://taginfo.openstreetmap.org\n[OpenStreetMap]: https://www.openstreetmap.org\n[tags]: https://wiki.openstreetmap.org/wiki/Tags\n[aiohttp]: https://docs.aiohttp.org/\n[Pydantic]: https://pydantic.dev/\n\n#### Contents\n- [Rationale](#rationale)\n- [Usage](#usage)\n- [Endpoints](#endpoints)\n\n#### See also\n- An overview of modules, classes and functions can be found in the [API reference](https://www.timwie.dev/aio-taginfo/)\n- The version history is available in [RELEASES.md](https://github.com/timwie/aio-taginfo/blob/main/RELEASES.md)\n- The [taginfo website](https://taginfo.openstreetmap.org/),\n its [API documentation](https://taginfo.openstreetmap.org/taginfo/apidoc),\n its [OSM Wiki page](https://wiki.openstreetmap.org/wiki/Taginfo),\n and its [repository](https://github.com/taginfo/taginfo)\n\n<br>\n\n## Rationale\nA tag consists of two items, a key and a value. For instance,\n[`highway=residential`](https://wiki.openstreetmap.org/wiki/Tag:highway%3Dresidential)\nis a tag with a key of [`highway`](https://wiki.openstreetmap.org/wiki/Key:highway)\nand a value of `residential`, which should be used on a\n[way](https://wiki.openstreetmap.org/wiki/Way) to indicate a road along which people live.\n\n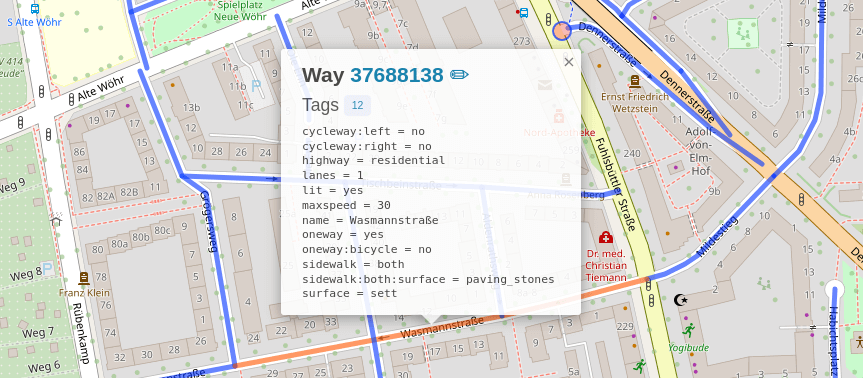\n\nNow, why is there need for the taginfo API?\n> OpenStreetMap uses tags to add meaning to geographic objects. There is no fixed\n> list of those tags. New tags can be invented and used as needed. Everybody can\n> come up with a new tag and add it to new or existing objects. This makes\n> OpenStreetMap enormously flexible, but sometimes also a bit hard to work with.\n> \n> Whether you are contributing to OSM or using the OSM data, there are always\n> questions like: What tags do people use for feature X? What tags can I use for\n> feature Y so that it appears properly on the map? Is the tag Z described on the\n> wiki actually in use and where?\n> \n> Taginfo helps you by showing statistics about which tags are actually in the\n> database, how many people use those tags, where they are used and so on. It also\n> gets information about tags from the wiki and from other places. Taginfo tries\n> to bring together all information about tags to help you understand how they are\n> used and what they mean.\n\n\n<br>\n\n## Usage\nBefore using this library, please be aware of the general rules when using the taginfo API:\n\n> Taginfo has an API that lets you access the contents of its databases in several\n> ways. The API is used internally for the web user interface and can also be used\n> by anybody who wants to integrate taginfo data into their websites or\n> applications.\n\n> The API is intended for the use of the OpenStreetMap community. Do not use it\n> for other services. If you are not sure, ask on the mailing list (see below).\n> \n> Always use a sensible User-agent header with enough information that we can\n> contact you if there is a problem.\n> \n> The server running the taginfo API does not have unlimited resources. Please use\n> the API responsibly. Do not create huge amounts of requests to get the whole\n> database or large chunks of it, instead use the [database downloads] provided.\n> If you are using the API and you find it is slow, you are probably overusing it.\n> \n> If you are using the taginfo API it is recommended that you join the\n> [taginfo-dev mailing list]. Updates to the API will be announced there and this\n> is also the right place for your questions. \n\n[database downloads]: https://taginfo.openstreetmap.org/download\n[taginfo-dev mailing list]: https://lists.openstreetmap.org/listinfo/taginfo-dev\n\nThe data available through taginfo is licenced under [ODbL],\nthe same license as the OpenStreetMap data.\n\n> OpenStreetMap[\u00ae] is open data, licensed under the\n> [Open Data Commons Open Database License] (ODbL)\n> by the [OpenStreetMap Foundation] (OSMF).\n> \n> You are free to copy, distribute, transmit and adapt our data, as long as you\n> credit OpenStreetMap and its contributors. If you alter or build upon our data,\n> you may distribute the result only under the same licence. The full [legal code]\n> explains your rights and responsibilities. \n\n[ODbL]: https://www.openstreetmap.org/copyright/en\n[\u00ae]: https://www.openstreetmap.org/copyright/en#trademarks\n[Open Data Commons Open Database License]: https://opendatacommons.org/licenses/odbl/\n[OpenStreetMap Foundation]: https://osmfoundation.org/\n[legal code]: https://opendatacommons.org/licenses/odbl/1.0/\n\n### Example\nHere is an example of an API request using this library:\n\n```python\nfrom aio_taginfo import key_overview\n\n# either use a temporary session\u2026\nresponse: Response[KeyOverview] = await key_overview(key=\"amenity\")\n\n# \u2026or provide your own\nheaders = {\"User-Agent\": \"your contact info\"}\nasync with aiohttp.ClientSession(headers=headers) as session:\n response: Response[KeyOverview] = await key_overview(key=\"amenity\", session=session)\n```\n\nMost endpoints will return a [`Response[T]`](https://www.timwie.dev/aio-taginfo/aio_taginfo/api/v4.html#Response),\nor `Response[list[T]]` for those returning multiple or paginated items.\n\n<br>\n\n## Endpoints\nThis library is in early development and most endpoints are still missing.\n\n| | Endpoint | Schema |\n|--:|--------------------------------------|--------------------------------|\n| \u2705 | `/api/4/key/chronology` | `Response[list[T]](page=None)` |\n| \u2705 | `/api/4/key/combinations` | `Response[list[T]]` |\n| \u2705 | `/api/4/key/distribution/nodes` | `PngResponse` |\n| \u2705 | `/api/4/key/distribution/ways` | `PngResponse` |\n| \u2705 | `/api/4/key/overview` | `Response[T]` |\n| \u2705 | `/api/4/key/prevalent_values` | `Response[list[T]](page=None)` |\n| \u2705 | `/api/4/key/projects` | `Response[list[T]]` |\n| \u2705 | `/api/4/key/similar` | `Response[list[T]]` |\n| \u2705 | `/api/4/key/stats` | `Response[list[T]](page=None)` |\n| | `/api/4/key/values` | `Response[list[T]]` |\n| | `/api/4/key/wiki_pages` | `Response[list[T]](page=None)` |\n| | `/api/4/keys/all` | `Response[list[T]]` |\n| | `/api/4/keys/similar` | `Response[list[T]]` |\n| | `/api/4/keys/wiki_pages` | `Response[list[T]]` |\n| | `/api/4/keys/without_wiki_page` | `Response[list[T]]` |\n| | `/api/4/project/icon` | `PngResponse` |\n| | `/api/4/project/tags` | `Response[list[T]]` |\n| | `/api/4/projects/all` | `Response[list[T]]` |\n| | `/api/4/projects/keys` | `Response[list[T]]` |\n| | `/api/4/projects/tags` | `Response[list[T]]` |\n| \u2705 | `/api/4/relation/projects` | `Response[list[T]]` |\n| | `/api/4/relation/roles` | `Response[list[T]]` |\n| | `/api/4/relation/stats` | `Response[list[T]](page=None)` |\n| | `/api/4/relation/wiki_pages` | `Response[list[T]](page=None)` |\n| | `/api/4/relations/all` | `Response[list[T]]` |\n| | `/api/4/search/by_key_and_value` | `Response[list[T]]` |\n| | `/api/4/search/by_keyword` | `Response[list[T]]` |\n| | `/api/4/search/by_role` | `Response[list[T]]` |\n| | `/api/4/search/by_value` | `Response[list[T]]` |\n| \u2705 | `/api/4/site/config/geodistribution` | `T` |\n| | `/api/4/site/info` | `T` |\n| | `/api/4/site/sources` | `T` |\n| | `/api/4/tag/chronology` | `Response[list[T]](page=None)` |\n| | `/api/4/tag/combinations` | `Response[list[T]]` |\n| | `/api/4/tag/distribution/nodes` | `PngResponse` |\n| | `/api/4/tag/distribution/ways` | `PngResponse` |\n| | `/api/4/tag/overview` | `Response[T]` |\n| \u2705 | `/api/4/tag/projects` | `Response[list[T]]` |\n| | `/api/4/tag/stats` | `Response[list[T]](page=None)` |\n| | `/api/4/tag/wiki_pages` | `Response[list[T]](page=None)` |\n| | `/api/4/tags/list` | `Response[list[T]](page=None)` |\n| \u2705 | `/api/4/tags/popular` | `Response[list[T]]` |\n| | `/api/4/unicode/characters` | `Response[list[T]](page=None)` |\n| | `/api/4/wiki/languages` | `Response[list[T]](page=None)` |\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Async client for the OpenStreetMap taginfo API",
"version": "0.4.0",
"project_urls": {
"Documentation": "https://www.timwie.dev/aio-taginfo/",
"Homepage": "https://github.com/timwie/aio-taginfo",
"Release Notes": "https://github.com/timwie/aio-taginfo/blob/main/RELEASES.md",
"Repository": "https://github.com/timwie/aio-taginfo",
"Test Coverage": "https://codecov.io/gh/timwie/aio-taginfo"
},
"split_keywords": [
"geospatial",
" gis",
" openstreetmap",
" osm",
" taginfo",
" spatial-analysis",
" spatial-data"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "b78d67918beb720a560e927a0581c11102323d00296de8e6e7e1a6b34a51c26a",
"md5": "19000aeea084e4bf42703e5068c03fc6",
"sha256": "d11229175fb4c3edb3d3b38f49773862cef1615555a34b82f04e400760986c44"
},
"downloads": -1,
"filename": "aio_taginfo-0.4.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "19000aeea084e4bf42703e5068c03fc6",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.10",
"size": 37354,
"upload_time": "2024-07-21T21:44:04",
"upload_time_iso_8601": "2024-07-21T21:44:04.063524Z",
"url": "https://files.pythonhosted.org/packages/b7/8d/67918beb720a560e927a0581c11102323d00296de8e6e7e1a6b34a51c26a/aio_taginfo-0.4.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "caeba63c78f43b1887a26832493ac85b3d5365c82541d2b02e959dbf379b4c12",
"md5": "50241665b9ab17f2720efd75f65ae2d2",
"sha256": "84a7d5ca42fd462ce7eb0a2c3455356ae4160f341973935186b6946aa3ccb604"
},
"downloads": -1,
"filename": "aio_taginfo-0.4.0.tar.gz",
"has_sig": false,
"md5_digest": "50241665b9ab17f2720efd75f65ae2d2",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.10",
"size": 19929,
"upload_time": "2024-07-21T21:44:05",
"upload_time_iso_8601": "2024-07-21T21:44:05.051824Z",
"url": "https://files.pythonhosted.org/packages/ca/eb/a63c78f43b1887a26832493ac85b3d5365c82541d2b02e959dbf379b4c12/aio_taginfo-0.4.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-07-21 21:44:05",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "timwie",
"github_project": "aio-taginfo",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "aio-taginfo"
}