# [alright](#)
<samp>
Python wrapper for WhatsApp web made with selenium inspired by [PyWhatsApp](https://github.com/shauryauppal/PyWhatsapp)
[](https://pepy.tech/project/alright)
[](https://pepy.tech/project/alright)
[](https://pepy.tech/project/alright)
[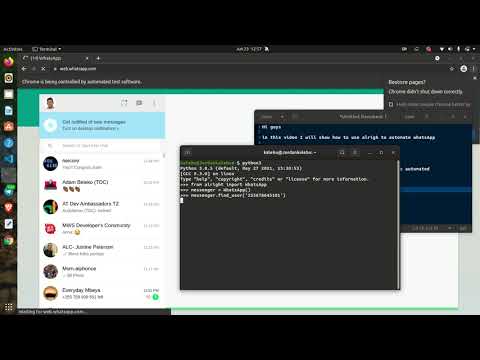](https://www.youtube.com/watch?v=yitQTt-NukM)
## Why alright ?
I was looking for a way to control and automate WhatsApp web with Python, I came across some very nice libaries and wrapper implementations including;
- [pywhatkit](https://pypi.org/project/pywhatkit/)
- [pywhatsapp](https://github.com/tax/pywhatsapp)
- [PyWhatsapp](https://github.com/shauryauppal/PyWhatsapp)
- [WebWhatsapp-Wrapper](https://github.com/mukulhase/WebWhatsapp-Wrapper)
- and many others
So I tried [**pywhatkit**](https://pypi.org/project/pywhatkit/), a really cool one well crafted to be used by others but its implementations require you to open a new browser tab and scan QR code everytime you send a message no matter if its to the same person, which was deal breaker for using it.
Then I tried [**pywhatsapp**](https://github.com/tax/pywhatsapp) which is based on [yowsup](https://github.com/tgalal/yowsup) and thus requiring you to do some registration with yowsup before using it of which after bit of googling I got scared of having my number blocked, so I went for the next option
I then went for [**WebWhatsapp-Wrapper**](https://github.com/mukulhase/WebWhatsapp-Wrapper), it has some good documentation and recent commits so I had hopes that it would work. But unfortunately it didn't for me, and after having a couple of errors I abandoned it to look for the next alternative.
Which is [**PyWhatsapp**](https://github.com/shauryauppal/PyWhatsapp) by [shauryauppal](https://github.com/shauryauppal/). This was more of CLI tool than a wrapper which suprisingly worked well and it's approach allows you to dynamically send whatsapp message to unsaved contacts without rescanning QR-code everytime.
So what I did is more of a refactoring of the implementation of that tool to be more of wrapper to easily allow people to run different scripts on top of it instead of just using it as a tool. I then thought of sharing the codebase to people who might have struggled to do this as I did.
## Getting started
You need to do a little bit of work to get [**alright**](https://github.com/Kalebu/alright) running, but don't worry I got you, everything will work well if you just carefully follow through the documentation.
### Installation
We need to have alright installed on our machine to start using it which can either be done directly from **GitHub** or using **pip**.
#### Installing directly
You first need to clone or download the repo to your local directory and then move into the project directory as shown in the example and then run the command below;
```bash
git clone https://github.com/Kalebu/alright
cd alright
alright > python setup.py install
....
```
#### Installing from pip
```bash
pip install alright --upgrade
```
### Setting up Selenium
Underneath alright is **Selenium** which is what does all the automation work by directly controlling the browser, so you need to have a selenium driver on your machine for **alright** to work. But luckily alright uses [webdriver-manager](https://pypi.org/project/webdriver-manager/), which does this automatically. You just need to install a browser. By default alright uses [Google Chrome](https://www.google.com/chrome/).
## What you can do with alright?
- [Send Messages](#sending-messages)
- [Send Messages1](#sending-messages1)
- [Send Images](#sending-images)
- [Send Videos](#sending-videos)
- [Send Documents](#sending-documents)
- [Get first chat](#get-first-chat)
- [Search chat by name](#search-chat-by-name)
- [logout](#logout)
*When you're running your program made with **alright**, you can only have one controlled browser window at a time. Running a new window while another window is live will raise an error. So make sure to close the controlled window before running another one*
### Unsaved contact vs saved contacts
Alright allows you to send the messages and media to both saved and unsaved contacts as explained earlier. But there is a tiny distinction on how you do that, you will observe this clearly as you use the package.
The first step before sending anything to the user is first to locate the user and then you can start sending the information, thats where the main difference lies between saved and unsaved contacts.
#### Saved contacts
To saved contact use method *find_by_username()* to locate a saved user. You can also use the same method to locate WhatsApp groups. The parameter can either be;
- saved username
- mobile number
- group name
Here an Example on how to do that
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.find_by_username('saved-name or number or group')
```
#### Unsaved contacts
In sending message to unsaved whatsapp contacts use *find_user()* method to locate the user and the parameter can only be users number with country code with (+) omitted as shown below;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.find_user('255-74848xxxx')
```
Now Let's dive in on how we can get started on sending messages and medias
### Sending Messages
>Use this if you don't have WhatsApp desktop installed
To send a message with alright, you first need to target a specific user by using *find_user()* method (include the **country code** in your number without the '+' symbol) and then after that you can start sending messages to the target user using *send_message()* method as shown in the example below;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.find_user('2557xxxxxz')
>>> messages = ['Morning my love', 'I wish you a good night!']
>>> for message in messages:
messenger.send_message(message)
```
### Send Direct Message [NEW]
> Recommended
This is newly added method that makes it a bit simpler to send a direct message without having to do the **find_user** or **find_by_username**, It works well even if you have or not have WhatsApp installed on your machine. It assumes the number is a saved contact by default.
```python
>>> messenger.send_direct_message(mobile, message, saved=True)
```
It does receive the following parameters;
1. **mobile[str]** - The mobile number of the user you want to send the message to
2. **message[str]** - The message you want to send
3. **saved[bool]** - If you want to send to a saved contact or not, default is True
Here is an example on how to use it;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> >>> messenger.send_direct_message('25573652xxx', 'Hello')
2022-08-14 17:27:57,264 - root -- [INFO] >> Message sent successfuly to
2022-08-14 17:27:57,264 - root -- [INFO] >> send_message() finished running!
>>> messenger.send_direct_message('25573652xxx', 'Who is This ?', False)
2022-08-14 17:28:30,953 - root -- [INFO] >> Message sent successfuly to 255736524388
2022-08-14 17:28:30,953 - root -- [INFO] >> send_message() finished running!
```
### Sending Messages1
This Send Message does NOT find the user first like in the above Send Message, AND it does work even if you have the Desktop WhatsApp app installed.
Include the **country code** in your number withour '+' symbol as shown in the example below;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messages = ['Morning my love', 'I wish you a good night!']
>>> mobNum = 27792346512
>>> for message in messages:
messenger.send_message1(mobNum, msg)
```
#### Multiple numbers
Here how to send a message to multiple users, Let's say we want to wish merry-x mass to all our contacts, our code is going to look like this;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> numbers = ['2557xxxxxx', '2557xxxxxx', '....']
>>> for number in numbers:
messenger.find_user(number)
messenger.send_message("I wish you a Merry X-mass and Happy new year ")
```
*You have to include the **country code** in your number for this library to work but don't include the (+) symbol*
> If you're sending media either picture, file, or video each of them have an optional parameter called <message> which is usually the caption to accompany the media.
### Sending Images
Sending Images is nothing new, its just the fact you have to include a path to your image and the message to accompany the image instead of just the raw string characters and also you have use *send_picture()*, Here an example;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.find_user('mobile')
>>> messenger.send_picture('path-to-image-without-caption')
>>> messenger.send_picture('path-to-image',"Text to accompany image")
```
### Sending Videos
Similarly, to send videos just use the *send_video()* method;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.find_user('mobile')
>>> messenger.send_video('path-to-video')
```
>
### Sending Documents
To send documents such as docx, pdf, audio etc, you can use the *send_file()* method to do that;
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.find_user('mobile')
>>> messenger.send_file('path-to-file')
```
### Check if a chat has unread messages or not
This method checks if a chat, which name is passed as a *query* parameter, has got unread messages or not.
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.check_if_given_chat_has_unread_messages(query="Chat 123")
```
### Get first chat
This method fetches the first chat in the list on the left of the web app - since they are not ordered in an expected way, a fair workaround is applied. One can also ignore (or not ignore) pinned chats (placed at the top of the list) by passing the parameter *ignore_pinned* to do that - default value is `ignore_pinned=True`.
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.get_first_chat()
```
### Search chat by name
This method searches the opened chats by a partial name provided as a `query` parameter, returning the first match. Case sensitivity is treated and does not impact the search.
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.search_chat_by_name(query="Friend")
```
### Get last message received in a given chat
This method searches for the last message received in a given chat, received as a `query` parameter, returning the sender, text and time. Groups, numbers and contacts cases are treated, as well as possible non-received messages, video/images/stickers and others.
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.get_last_message_received(query="Friend")
```
### Retrieve all chat names with unread messages
This method searches for all chats with unread messages, possibly receiving parameters to `limit` the search to a `top` number of chats or not, returning a list of chat names.
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.fetch_all_unread_chats(limit=True, top=30)
```
#### DISCLAIMER: Apparently, `fetch_all_unread_chats` functionallity works on most updated browser versions (for example, `Chrome Version 102.0.5005.115 (Official Build) (x86_64)`). If it fails with you, please consider updating your browser while we work on an alternatives for non-updated broswers.
### logout from whatsapp
You can sign out of an account that is currently saved
```python
>>> from alright import WhatsApp
>>> messenger = WhatsApp()
>>> messenger.logout()
```
Well! thats all for now from the package, to request new feature make an issue.
## Contributions
**alright** is an open-source package under **MIT** license, so contributions are warmly welcome whether that be a code , docs or typo just fork it.
When contributing to code please make an issue for that before making your changes so that we can have a discussion before implementation.
## Issues
If you're facing any issue or difficulty with the usage of the package just raise one so that we can fix it as soon as possible.
**Please, be as much comprehensive as possible!** Use as many screenshots and detailed description sets as possible; this will save us some time that we'd dedicate on asking you for "a more detailed descriptiton", and it'll make your request be solved faster.
## Give it a star
Was this useful to you ? Then give it a star so that more people can manke use of this.
## Credits
All the credits to:
- [kalebu](https://github.com/kalebu)
- [Eurico Nicacio](https://github.com/euriconicacio)
- [Victor Daniel](https://github.com/vadolasi)
- [Cornelius Mostert](https://github.com/theCJMan)
- [shauryauppal](https://github.com/shauryauppal/)
- and all the contributors
</samp>
Raw data
{
"_id": null,
"home_page": "https://github.com/Kalebu/alright",
"name": "alright",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "alright,python-whatsapp,PyWhatsApp,pywhatsapp,python-whatsapp-wrapper",
"author": "Jordan Kalebu",
"author_email": "isaackeinstein@gmail.com",
"download_url": "https://github.com/Kalebu/alright/archive/refs/tags/v1.7.tar.gz",
"platform": null,
"description": "# [alright](#)\n\n<samp>\n\nPython wrapper for WhatsApp web made with selenium inspired by [PyWhatsApp](https://github.com/shauryauppal/PyWhatsapp)\n\n[](https://pepy.tech/project/alright)\n[](https://pepy.tech/project/alright)\n[](https://pepy.tech/project/alright)\n\n[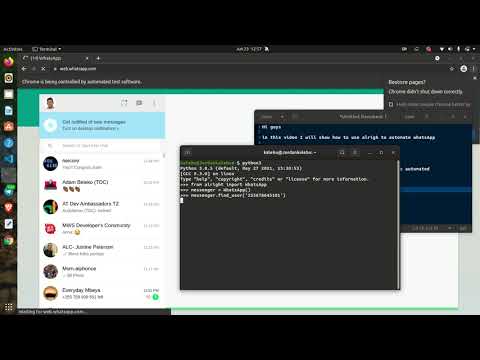](https://www.youtube.com/watch?v=yitQTt-NukM)\n\n## Why alright ?\n\nI was looking for a way to control and automate WhatsApp web with Python, I came across some very nice libaries and wrapper implementations including;\n\n- [pywhatkit](https://pypi.org/project/pywhatkit/)\n- [pywhatsapp](https://github.com/tax/pywhatsapp)\n- [PyWhatsapp](https://github.com/shauryauppal/PyWhatsapp)\n- [WebWhatsapp-Wrapper](https://github.com/mukulhase/WebWhatsapp-Wrapper)\n- and many others\n\nSo I tried [**pywhatkit**](https://pypi.org/project/pywhatkit/), a really cool one well crafted to be used by others but its implementations require you to open a new browser tab and scan QR code everytime you send a message no matter if its to the same person, which was deal breaker for using it.\n\nThen I tried [**pywhatsapp**](https://github.com/tax/pywhatsapp) which is based on [yowsup](https://github.com/tgalal/yowsup) and thus requiring you to do some registration with yowsup before using it of which after bit of googling I got scared of having my number blocked, so I went for the next option\n\nI then went for [**WebWhatsapp-Wrapper**](https://github.com/mukulhase/WebWhatsapp-Wrapper), it has some good documentation and recent commits so I had hopes that it would work. But unfortunately it didn't for me, and after having a couple of errors I abandoned it to look for the next alternative.\n\nWhich is [**PyWhatsapp**](https://github.com/shauryauppal/PyWhatsapp) by [shauryauppal](https://github.com/shauryauppal/). This was more of CLI tool than a wrapper which suprisingly worked well and it's approach allows you to dynamically send whatsapp message to unsaved contacts without rescanning QR-code everytime.\n\nSo what I did is more of a refactoring of the implementation of that tool to be more of wrapper to easily allow people to run different scripts on top of it instead of just using it as a tool. I then thought of sharing the codebase to people who might have struggled to do this as I did.\n\n## Getting started\n\nYou need to do a little bit of work to get [**alright**](https://github.com/Kalebu/alright) running, but don't worry I got you, everything will work well if you just carefully follow through the documentation.\n\n### Installation\n\nWe need to have alright installed on our machine to start using it which can either be done directly from **GitHub** or using **pip**.\n\n#### Installing directly\n\nYou first need to clone or download the repo to your local directory and then move into the project directory as shown in the example and then run the command below;\n\n```bash\ngit clone https://github.com/Kalebu/alright\ncd alright\nalright > python setup.py install \n....\n```\n\n#### Installing from pip\n\n```bash\npip install alright --upgrade\n```\n\n### Setting up Selenium\n\nUnderneath alright is **Selenium** which is what does all the automation work by directly controlling the browser, so you need to have a selenium driver on your machine for **alright** to work. But luckily alright uses [webdriver-manager](https://pypi.org/project/webdriver-manager/), which does this automatically. You just need to install a browser. By default alright uses [Google Chrome](https://www.google.com/chrome/).\n\n## What you can do with alright?\n\n- [Send Messages](#sending-messages)\n- [Send Messages1](#sending-messages1)\n- [Send Images](#sending-images)\n- [Send Videos](#sending-videos)\n- [Send Documents](#sending-documents)\n- [Get first chat](#get-first-chat)\n- [Search chat by name](#search-chat-by-name)\n- [logout](#logout)\n\n*When you're running your program made with **alright**, you can only have one controlled browser window at a time. Running a new window while another window is live will raise an error. So make sure to close the controlled window before running another one*\n\n### Unsaved contact vs saved contacts\n\nAlright allows you to send the messages and media to both saved and unsaved contacts as explained earlier. But there is a tiny distinction on how you do that, you will observe this clearly as you use the package.\n\nThe first step before sending anything to the user is first to locate the user and then you can start sending the information, thats where the main difference lies between saved and unsaved contacts.\n\n#### Saved contacts\n\nTo saved contact use method *find_by_username()* to locate a saved user. You can also use the same method to locate WhatsApp groups. The parameter can either be;\n\n- saved username\n- mobile number\n- group name\n\nHere an Example on how to do that\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.find_by_username('saved-name or number or group')\n```\n\n#### Unsaved contacts\n\nIn sending message to unsaved whatsapp contacts use *find_user()* method to locate the user and the parameter can only be users number with country code with (+) omitted as shown below;\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.find_user('255-74848xxxx')\n```\n\nNow Let's dive in on how we can get started on sending messages and medias\n\n### Sending Messages\n\n>Use this if you don't have WhatsApp desktop installed\n\nTo send a message with alright, you first need to target a specific user by using *find_user()* method (include the **country code** in your number without the '+' symbol) and then after that you can start sending messages to the target user using *send_message()* method as shown in the example below;\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.find_user('2557xxxxxz')\n>>> messages = ['Morning my love', 'I wish you a good night!']\n>>> for message in messages: \n messenger.send_message(message) \n```\n\n### Send Direct Message [NEW]\n\n> Recommended\n\nThis is newly added method that makes it a bit simpler to send a direct message without having to do the **find_user** or **find_by_username**, It works well even if you have or not have WhatsApp installed on your machine. It assumes the number is a saved contact by default.\n\n```python\n>>> messenger.send_direct_message(mobile, message, saved=True)\n```\n\nIt does receive the following parameters;\n\n1. **mobile[str]** - The mobile number of the user you want to send the message to\n2. **message[str]** - The message you want to send\n3. **saved[bool]** - If you want to send to a saved contact or not, default is True\n\nHere is an example on how to use it;\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> >>> messenger.send_direct_message('25573652xxx', 'Hello')\n2022-08-14 17:27:57,264 - root -- [INFO] >> Message sent successfuly to \n2022-08-14 17:27:57,264 - root -- [INFO] >> send_message() finished running!\n>>> messenger.send_direct_message('25573652xxx', 'Who is This ?', False)\n2022-08-14 17:28:30,953 - root -- [INFO] >> Message sent successfuly to 255736524388\n2022-08-14 17:28:30,953 - root -- [INFO] >> send_message() finished running!\n```\n\n### Sending Messages1\n\nThis Send Message does NOT find the user first like in the above Send Message, AND it does work even if you have the Desktop WhatsApp app installed.\nInclude the **country code** in your number withour '+' symbol as shown in the example below;\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messages = ['Morning my love', 'I wish you a good night!']\n>>> mobNum = 27792346512\n>>> for message in messages: \n messenger.send_message1(mobNum, msg)\n```\n\n#### Multiple numbers\n\nHere how to send a message to multiple users, Let's say we want to wish merry-x mass to all our contacts, our code is going to look like this;\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> numbers = ['2557xxxxxx', '2557xxxxxx', '....']\n>>> for number in numbers:\n messenger.find_user(number)\n messenger.send_message(\"I wish you a Merry X-mass and Happy new year \")\n```\n\n*You have to include the **country code** in your number for this library to work but don't include the (+) symbol*\n\n\n> If you're sending media either picture, file, or video each of them have an optional parameter called <message> which is usually the caption to accompany the media. \n\n### Sending Images\n\nSending Images is nothing new, its just the fact you have to include a path to your image and the message to accompany the image instead of just the raw string characters and also you have use *send_picture()*, Here an example;\n\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.find_user('mobile')\n>>> messenger.send_picture('path-to-image-without-caption')\n>>> messenger.send_picture('path-to-image',\"Text to accompany image\")\n```\n\n### Sending Videos\n\nSimilarly, to send videos just use the *send_video()* method;\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.find_user('mobile')\n>>> messenger.send_video('path-to-video')\n```\n\n>\n\n### Sending Documents\n\nTo send documents such as docx, pdf, audio etc, you can use the *send_file()* method to do that;\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.find_user('mobile')\n>>> messenger.send_file('path-to-file')\n```\n\n### Check if a chat has unread messages or not\n\nThis method checks if a chat, which name is passed as a *query* parameter, has got unread messages or not.\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.check_if_given_chat_has_unread_messages(query=\"Chat 123\")\n```\n\n### Get first chat\n\nThis method fetches the first chat in the list on the left of the web app - since they are not ordered in an expected way, a fair workaround is applied. One can also ignore (or not ignore) pinned chats (placed at the top of the list) by passing the parameter *ignore_pinned* to do that - default value is `ignore_pinned=True`.\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.get_first_chat()\n```\n\n### Search chat by name\n\nThis method searches the opened chats by a partial name provided as a `query` parameter, returning the first match. Case sensitivity is treated and does not impact the search.\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.search_chat_by_name(query=\"Friend\")\n```\n\n### Get last message received in a given chat\n\nThis method searches for the last message received in a given chat, received as a `query` parameter, returning the sender, text and time. Groups, numbers and contacts cases are treated, as well as possible non-received messages, video/images/stickers and others.\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.get_last_message_received(query=\"Friend\")\n```\n\n### Retrieve all chat names with unread messages\n\nThis method searches for all chats with unread messages, possibly receiving parameters to `limit` the search to a `top` number of chats or not, returning a list of chat names.\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.fetch_all_unread_chats(limit=True, top=30)\n```\n\n#### DISCLAIMER: Apparently, `fetch_all_unread_chats` functionallity works on most updated browser versions (for example, `Chrome Version 102.0.5005.115 (Official Build) (x86_64)`). If it fails with you, please consider updating your browser while we work on an alternatives for non-updated broswers.\n\n### logout from whatsapp\n\nYou can sign out of an account that is currently saved\n\n```python\n>>> from alright import WhatsApp\n>>> messenger = WhatsApp()\n>>> messenger.logout()\n```\n\nWell! thats all for now from the package, to request new feature make an issue.\n\n## Contributions\n\n**alright** is an open-source package under **MIT** license, so contributions are warmly welcome whether that be a code , docs or typo just fork it.\n\nWhen contributing to code please make an issue for that before making your changes so that we can have a discussion before implementation.\n\n## Issues\n\nIf you're facing any issue or difficulty with the usage of the package just raise one so that we can fix it as soon as possible.\n\n**Please, be as much comprehensive as possible!** Use as many screenshots and detailed description sets as possible; this will save us some time that we'd dedicate on asking you for \"a more detailed descriptiton\", and it'll make your request be solved faster.\n\n## Give it a star\n\nWas this useful to you ? Then give it a star so that more people can manke use of this.\n\n## Credits\n\nAll the credits to:\n\n- [kalebu](https://github.com/kalebu)\n- [Eurico Nicacio](https://github.com/euriconicacio)\n- [Victor Daniel](https://github.com/vadolasi)\n- [Cornelius Mostert](https://github.com/theCJMan)\n- [shauryauppal](https://github.com/shauryauppal/)\n- and all the contributors\n\n</samp>\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Python wrapper for WhatsApp web based on selenium",
"version": "2.61",
"project_urls": {
"Download": "https://github.com/Kalebu/alright/archive/refs/tags/v1.7.tar.gz",
"Homepage": "https://github.com/Kalebu/alright"
},
"split_keywords": [
"alright",
"python-whatsapp",
"pywhatsapp",
"pywhatsapp",
"python-whatsapp-wrapper"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "294ad543e3ef21f2fb2b802c4e45f797753156b1a92c1297a327c1125bc00f1c",
"md5": "8ee6d0c935a7ad34acd993cb2805c7ef",
"sha256": "29a9dee8fcad6ae48eee2aa17ceb5ae09da28302a178143ef1f6445043a4d1df"
},
"downloads": -1,
"filename": "alright-2.61-py3-none-any.whl",
"has_sig": false,
"md5_digest": "8ee6d0c935a7ad34acd993cb2805c7ef",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 13833,
"upload_time": "2023-09-16T23:49:02",
"upload_time_iso_8601": "2023-09-16T23:49:02.586624Z",
"url": "https://files.pythonhosted.org/packages/29/4a/d543e3ef21f2fb2b802c4e45f797753156b1a92c1297a327c1125bc00f1c/alright-2.61-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-09-16 23:49:02",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "Kalebu",
"github_project": "alright",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "alright"
}