<div align="center">
<br>
<h1> Android-Notifiy </h1>
<p> A Python library for effortlessly creating and managing Android notifications in Kivy android apps.</p>
<p>Supports various styles and ensures seamless integration and customization.</p>
<!-- <br> -->
<!-- <img src="https://raw.githubusercontent.com/Fector101/android_notify/main/docs/imgs/democollage.jpg"> -->
</div>
## Features
- Also Compatible with Android 8.0+.
- Supports including images in notifications.
- All Notifications can take Functions (version 1.50+) [functions section](#functions).
- Advanced Notification Handling [section](#advanced-features).
- Changing default app notification icon with PNG,
- Support for multiple notification styles:
- [Simple](#basic-usage)
- [Progress](#progress-bar-notification)
- [Big Picture](#notification-with-an-image-big-picture-style)
- [Inbox](#inbox-notification-style)
- [Large Icon](#notification-with-an-image-large-icon-style)
- [Buttons](#notification-with-buttons)
- [Big Text](#big-text-notification)
- persistenting notification [section](#methods)
This module automatically handles:
- Permission requests for notifications
- Customizable notification channels.
- Opening app on notification click
## Installation
This package is available on PyPI and can be installed via pip:
```bash
pip install android-notify
```
## **Dependencies**
**Prerequisites:**
- Kivy
In your **`buildozer.spec`** file, ensure you include the following:
```ini
# Add pyjnius so ensure it's packaged with the build
requirements = python3, kivy, pyjnius, android-notify
# Add permission for notifications
android.permissions = POST_NOTIFICATIONS
# Required dependencies (write exactly as shown, no quotation marks)
android.gradle_dependencies = androidx.core:core:1.6.0, androidx.core:core-ktx:1.15.0
android.enable_androidx = True
android.api = 35
```
---
## Basic Usage
```python
from android_notify import Notification
# Create a simple notification
notification = Notification(
title="Hello",
message="This is a basic notification."
)
notification.send()
```
**Sample Image:**
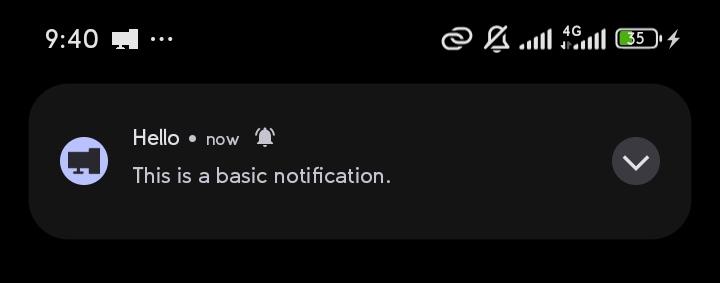
## Notification Styles
The library supports multiple notification styles:
1. `simple` - Basic notification with title and message
2. `progress` - Shows a progress bar
3. `big_picture` - Notification with a large image
4. `large_icon` - Notification with a custom icon
5. `both_imgs` - Combines big picture and large icon
6. `inbox` - List-style notification
7. `big_text` - Expandable notification with long text
8. `custom` - For custom notification styles
### Style Examples
#### Progress Bar notification
```python
from kivy.clock import Clock
progress = 0
notification = Notification(
title="Downloading...", message="0% downloaded",
style= "progress",
progress_current_value=0,progress_max_value=100
)
notification.send()
def update_progress(dt):
global progress
progress = min(progress + 10, 100)
notification.updateProgressBar(progress, f"{progress}% downloaded")
return progress < 100 # Stops when reaching 100%
Clock.schedule_interval(update_progress, 3)
```
**Sample Image:**
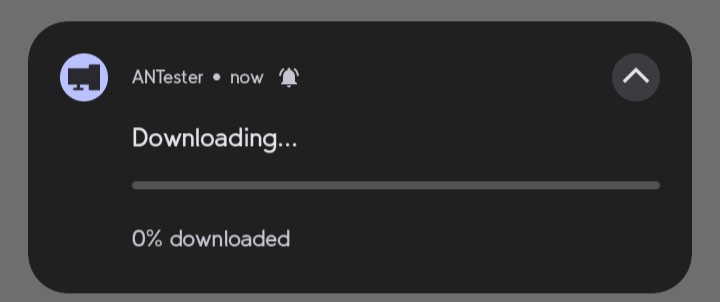
#### Images
##### Notification with an Image (Big Picture Style)
> [!NOTE]
> Online Images should start with `http://` or `https://`
> And request for permission, `android.permissions = INTERNET`
> No additionally permissions needed for images in App folder
```python
# Image notification
notification = Notification(
title='Picture Alert!',
message='This notification includes an image.',
style="big_picture",
big_picture_path="assets/imgs/photo.png"
)
notification.send()
```
**Sample Image:**
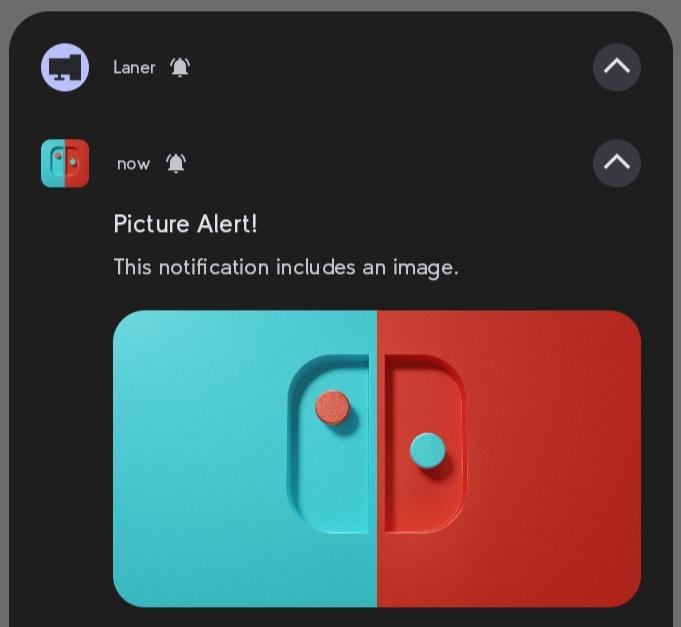
##### Notification with an Image (Large Icon Style)
```python
notification = Notification(
title="FabianDev_",
message="A twitter about some programming stuff",
style="large_icon",
large_icon_path="assets/imgs/profile.png"
)
```
**Sample Image:**
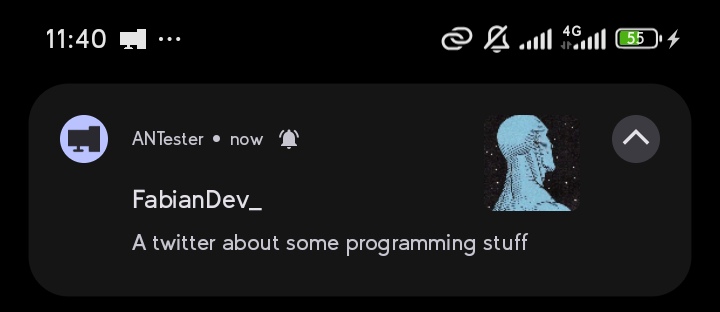
#### Inbox Notification Style
```python
# Send a notification with inbox style
notification = Notification(
title='Inbox Notification',
message='Line 1\nLine 2\nLine 3',
style='inbox'
)
notification.send()
```
**Sample Image:**
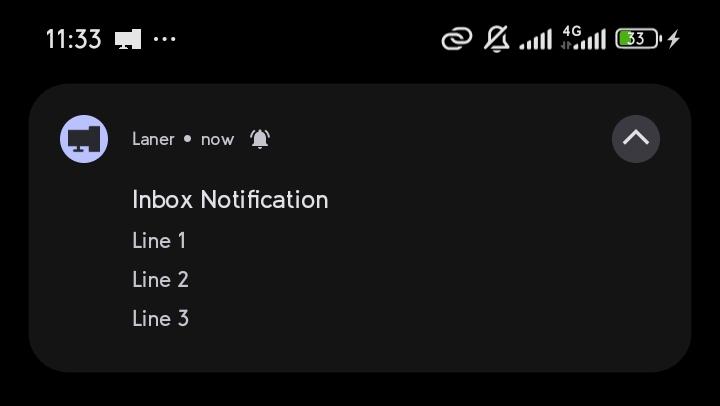
#### Notification with Buttons
Here's a sample of how to add buttons below, To Remove Buttons Simply Call the `removeButtons` method on the Notification Instance
```python
notification = Notification(title="Jane Dough", message="How to use android-notify #coding #purepython")
def playVideo():
print('Playing Video')
def turnOffNoti():
print('Please Turn OFf Noti')
def watchLater():
print('Add to Watch Later')
notification.addButton(text="Play",on_release=playVideo)
notification.addButton(text="Turn Off",on_release=turnOffNoti)
notification.addButton(text="Watch Later",on_release=watchLater)
notification.send()
```
**Sample Image:**
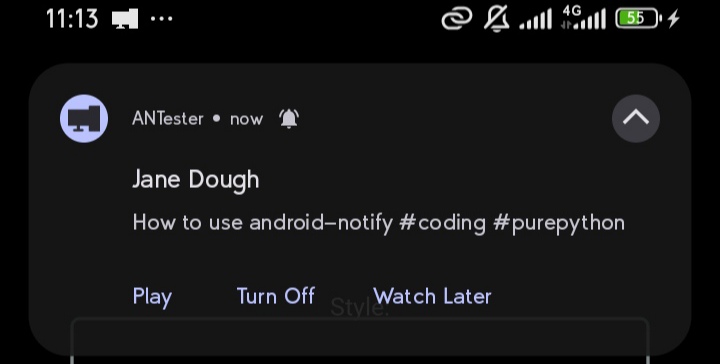
#### Big text notification
When using `big_text` style `message` acts as sub-title, Then when notification drop down button is pressed `body` is revealed
```python
notification = Notification(
title="Article",
message="Histroy of Loerm Ipsuim",
body="Lorem Ipsum is simply dummy text of the printing and ...",
style="big_text"
)
```

## Advanced Features
### Updating Notifications
```python
notification = Notification(title="Initial Title")
notification.send()
# Update title
notification.updateTitle("New Title")
# Update message
notification.updateMessage("New Message")
```
### Progress Bar Management
```python
notification = Notification(
title="Download..",
style="progress"
)
# send notification
notification.send()
# Update progress
notification.updateProgressBar(30, "30% downloaded")
# Remove progress bar
# show_on_update to notification briefly after removed progressbar
notification.removeProgressBar("Download Complete",show_on_update=True)
```
### Adding Style even when already sent
This is how you add a new style to notification, If already sent or not
```python
from android_notify import NotificationStyles
notification = Notification(
title="Download..",
style="progress"
)
notification.send()
notification.updateTitle("Download Completed")
notification.removeProgressBar()
# Add New Style
notification.large_icon_path="users/imgs/profile1234.png"
notification.addNotificationStyle(NotificationStyles.LARGE_ICON,already_sent=True)
```
### Channel Management
Notifications are organized into channels. You can customize the channel name and ID:
- Custom Channel Name's Gives User ability to turn on/off specific notifications
```python
notification = Notification(
title="Download finished",
message="How to Catch a Fish.mp4",
channel_name="Download Notifications", # Will create User-visible name "Download Notifications"
channel_id="downloads_notifications" # Optional: specify custom channel ID
)
```
**Sample Image:**
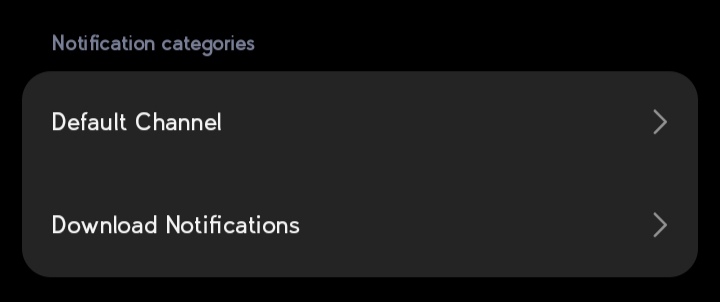
### Silent Notifications
To send a notification without sound or heads-up display:
```python
notification = Notification(title="Silent Update")
notification.send(silent=True)
```
## Functions
```python
from kivymd.app import MDApp
from android_notify import Notification
class Myapp(MDApp):
def on_start(self):
Notification(title="Hello", message="This is a basic notification.",callback=self.doSomething).send()
def doSomething(self):
print("print in Debug Console")
```
### Get Which Notification was used to Open App - identifer (str)
If you just want to get the Exact Notification Clicked to Open App, you can use NotificationHandler to get unique identifer
```python
from kivymd.app import MDApp
from android_notify import Notification, NotificationHandler
class Myapp(MDApp):
def on_start(self):
notify = Notification(title="Change Page", message="Click to change App page.", identifer='change_app_page')
notify.send()
notify1 = Notification(title="Change Colour", message="Click to change App Colour", identifer='change_app_color')
notify1.send()
def on_resume(self):
# Is called everytime app is reopened
notify_identifer = NotificationHandler.getIdentifer()
if notify_identifer == 'change_app_page':
# Code to change Screen
pass
elif notify_identifer == 'change_app_color':
# Code to change Screen Color
pass
```
### Assist
- Avoiding Human Error when using different notification styles
```python
from android_notify import Notification, NotificationStyles
Notification(
title="New Photo",
message="Check out this image",
style=NotificationStyles.BIG_PICTURE,
big_picture_path="assets/imgs/photo.png"
).send()
```
## Development Mode
When developing on non-Android platforms, the library provides debugging output:
```python
# Enable logs (default is True when not on Android)
Notification.logs = True
# Create notification for testing
notification = Notification(title="Test")
notification.send()
# Will print notification properties instead of sending
```
### Methods
### Instance.**init**
args
- app_icon: If not specified defaults to app icon, To Change Default app icon use PNG Or Image Will display as a Black Box
### Instance.send
args
- persistent : To make notification stay after user clicks clear All
- close_on_click : To close notification on click
### Instance.addButton
args
- text : Button Text
### Instance.updateTitle
args
- new_title : String to be set as New notification Title
### Instance.updateMessage
args
- new_message : String to be set as New notification Message
### Instance.updateProgressBar
args
- current_value (str): the value from progressbar current progress
- message (str,optional): defaults to last message
### Instance.removeProgressBar
This Removes Progress bar
args
- message (str, optional): notification message, Defaults to 'last message'.
- show_on_update (bool, optional): To show notification brifely when progressbar removed. Defaults to True.
### Instance.addNotificationStyle
This is useful to add style after Notification is sent or Add more styles to Notification
args
- style: choosen style. All options are in the `NotificationStyles` class `['simple','progress','inbox','big_text','large_icon','big_picture','both_imgs]`
- already_sent: specfiy if notification.send() method has already been called, it defaults to false
## Image Requirements
- Online Images should start with `http://` or `https://`
- Local Images must be located within your app's folder
## Error Handling
The library validates arguments and provides helpful error messages:
- Invalid style names will suggest the closest matching style
- Invalid arguments will list all valid options
- Missing image files will list all files in App Directory
## Limitation
1. Only works on Android devices
## Best Practices
1. Always handle permissions appropriately
2. use `NotificationStyles` to set `style`, use `style=NotificationStyles.LARGE_ICON` instead of `style="large_icon"`
3. Use meaningful channel names for organization
4. Keep progress bar updates reasonable (don't update too frequently)
5. Test notifications on different Android versions
6. Consider using silent notifications for frequent updates
## Debugging Tips
1. Enable logs during development: `Notification.logs = True`
2. Check channel creation with Android's notification settings
3. Verify image paths before sending notifications
4. Test different styles to ensure proper display
Remember to check Android's notification documentation for best practices and guidelines regarding notification frequency and content.
## Contribution
Feel free to open issues or submit pull requests for improvements!
## Reporting Issues
Found a bug? Please open an issue on our [GitHub Issues](https://github.com/Fector101/android_notify/issues) page.
## Author
- Fabian - <fector101@yahoo.com>
- GitHub: [Android Notify Repo](https://github.com/Fector101/android_notify)
- Twitter: [FabianDev_](https://twitter.com/intent/user?user_id=1246911115319263233)
For feedback or contributions, feel free to reach out!
---
## ☕ Support the Project
If you find this project helpful, consider buying me a coffee! 😊 Or Giving it a star on 🌟 [GitHub](https://github.com/Fector101/android_notify/) Your support helps maintain and improve the project.
<a href="https://www.buymeacoffee.com/fector101" target="_blank">
<img src="https://cdn.buymeacoffee.com/buttons/v2/default-yellow.png" alt="Buy Me A Coffee" height="60">
</a>
---
## Acknowledgments
- This Project was thoroughly Tested by the [Laner Project](https://github.com/Fector101/Laner/) - A application for Securely Transfering Files Wirelessly between your PC and Phone.
- Thanks to the Kivy and Pyjnius communities.
---
## 🌐 **Links**
- **PyPI:** [android-notify on PyPI](https://pypi.org/project/android-notify/)
- **GitHub:** [Source Code Repository](https://github.com/Fector101/android_notify/)
Raw data
{
"_id": null,
"home_page": "https://github.com/fector101/android-notify",
"name": "android-notify",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "android, notifications, kivy, mobile, post-notifications, pyjnius, android-notifications, kivy-notifications, python-android, mobile-development, push-notifications, mobile-app, kivy-application",
"author": "Fabian",
"author_email": "fector101@yahoo.com",
"download_url": "https://files.pythonhosted.org/packages/d8/da/f47ac217b447f955a9255b0c5f7121d2f67dc2945fa67331fe34d8e20598/android_notify-1.53.1.tar.gz",
"platform": null,
"description": "<div align=\"center\">\n <br>\n <h1> Android-Notifiy </h1>\n <p> A Python library for effortlessly creating and managing Android notifications in Kivy android apps.</p>\n <p>Supports various styles and ensures seamless integration and customization.</p>\n <!-- <br> -->\n <!-- <img src=\"https://raw.githubusercontent.com/Fector101/android_notify/main/docs/imgs/democollage.jpg\"> -->\n</div>\n\n## Features\n\n- Also Compatible with Android 8.0+.\n- Supports including images in notifications.\n- All Notifications can take Functions (version 1.50+) [functions section](#functions).\n- Advanced Notification Handling [section](#advanced-features).\n- Changing default app notification icon with PNG,\n- Support for multiple notification styles:\n - [Simple](#basic-usage)\n - [Progress](#progress-bar-notification)\n - [Big Picture](#notification-with-an-image-big-picture-style)\n - [Inbox](#inbox-notification-style)\n - [Large Icon](#notification-with-an-image-large-icon-style)\n - [Buttons](#notification-with-buttons)\n - [Big Text](#big-text-notification)\n\n- persistenting notification [section](#methods)\n\nThis module automatically handles:\n\n- Permission requests for notifications\n- Customizable notification channels.\n- Opening app on notification click\n\n## Installation\n\nThis package is available on PyPI and can be installed via pip:\n\n```bash\npip install android-notify\n```\n\n## **Dependencies**\n\n**Prerequisites:** \n\n- Kivy\n\nIn your **`buildozer.spec`** file, ensure you include the following:\n\n```ini\n# Add pyjnius so ensure it's packaged with the build\nrequirements = python3, kivy, pyjnius, android-notify\n\n# Add permission for notifications\nandroid.permissions = POST_NOTIFICATIONS\n\n# Required dependencies (write exactly as shown, no quotation marks)\nandroid.gradle_dependencies = androidx.core:core:1.6.0, androidx.core:core-ktx:1.15.0\nandroid.enable_androidx = True\nandroid.api = 35\n```\n\n---\n\n## Basic Usage\n\n```python\nfrom android_notify import Notification\n\n# Create a simple notification\nnotification = Notification(\n title=\"Hello\",\n message=\"This is a basic notification.\"\n)\nnotification.send()\n```\n\n**Sample Image:** \n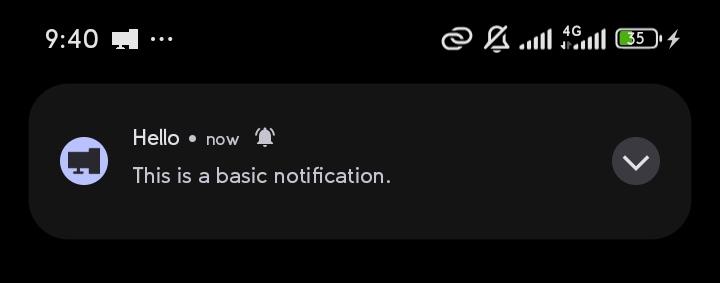\n\n## Notification Styles\n\nThe library supports multiple notification styles:\n\n1. `simple` - Basic notification with title and message\n2. `progress` - Shows a progress bar\n3. `big_picture` - Notification with a large image\n4. `large_icon` - Notification with a custom icon\n5. `both_imgs` - Combines big picture and large icon\n6. `inbox` - List-style notification\n7. `big_text` - Expandable notification with long text\n8. `custom` - For custom notification styles\n\n### Style Examples\n\n#### Progress Bar notification\n\n```python\nfrom kivy.clock import Clock\n\nprogress = 0\n\nnotification = Notification(\n title=\"Downloading...\", message=\"0% downloaded\",\n style= \"progress\",\n progress_current_value=0,progress_max_value=100\n )\nnotification.send()\n\ndef update_progress(dt):\n global progress\n progress = min(progress + 10, 100)\n notification.updateProgressBar(progress, f\"{progress}% downloaded\")\n return progress < 100 # Stops when reaching 100%\n\nClock.schedule_interval(update_progress, 3)\n```\n\n**Sample Image:**\n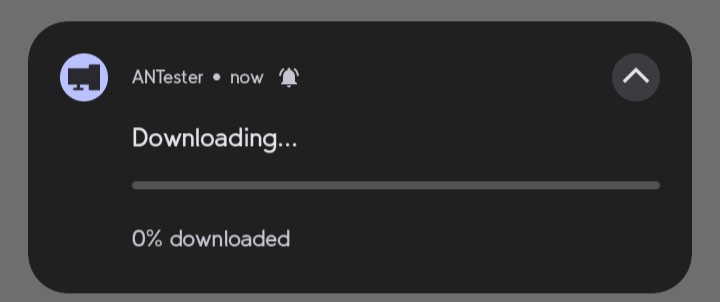\n\n#### Images\n\n##### Notification with an Image (Big Picture Style)\n\n> [!NOTE]\n> Online Images should start with `http://` or `https://` \n> And request for permission, `android.permissions = INTERNET` \n> No additionally permissions needed for images in App folder\n\n```python\n# Image notification\nnotification = Notification(\n title='Picture Alert!',\n message='This notification includes an image.',\n style=\"big_picture\",\n big_picture_path=\"assets/imgs/photo.png\"\n)\nnotification.send()\n\n```\n\n**Sample Image:**\n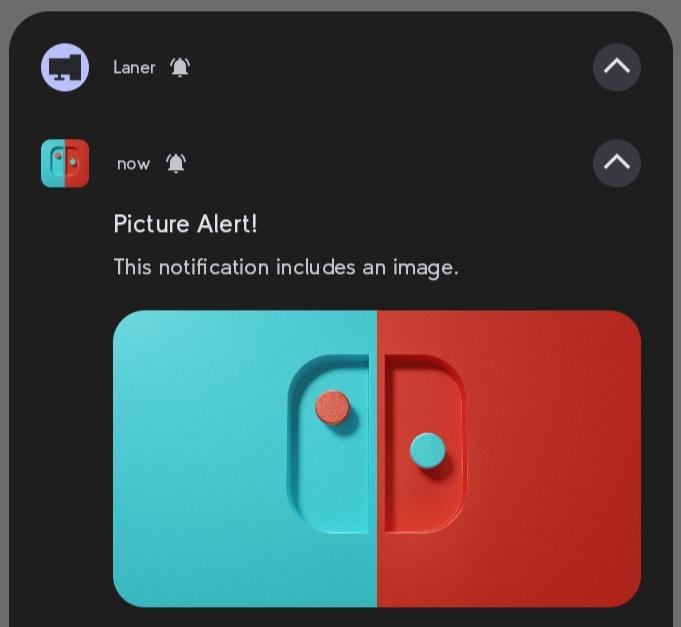\n\n##### Notification with an Image (Large Icon Style)\n\n```python\nnotification = Notification(\n title=\"FabianDev_\",\n message=\"A twitter about some programming stuff\",\n style=\"large_icon\",\n large_icon_path=\"assets/imgs/profile.png\"\n)\n\n```\n\n**Sample Image:** \n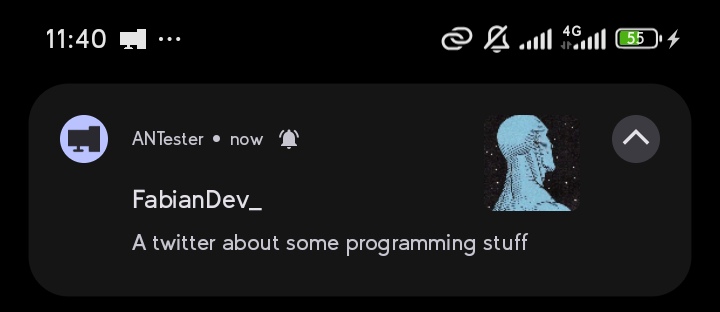\n\n#### Inbox Notification Style\n\n```python\n# Send a notification with inbox style\nnotification = Notification(\n title='Inbox Notification',\n message='Line 1\\nLine 2\\nLine 3',\n style='inbox'\n)\nnotification.send()\n\n```\n\n**Sample Image:**\n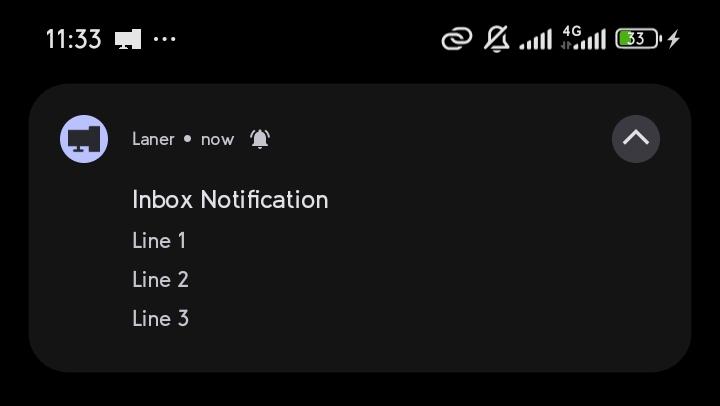\n\n#### Notification with Buttons\n\nHere's a sample of how to add buttons below, To Remove Buttons Simply Call the `removeButtons` method on the Notification Instance\n\n```python\nnotification = Notification(title=\"Jane Dough\", message=\"How to use android-notify #coding #purepython\")\ndef playVideo():\n print('Playing Video')\n\ndef turnOffNoti():\n print('Please Turn OFf Noti')\n\ndef watchLater():\n print('Add to Watch Later')\n\nnotification.addButton(text=\"Play\",on_release=playVideo)\nnotification.addButton(text=\"Turn Off\",on_release=turnOffNoti)\nnotification.addButton(text=\"Watch Later\",on_release=watchLater)\nnotification.send()\n```\n\n**Sample Image:** \n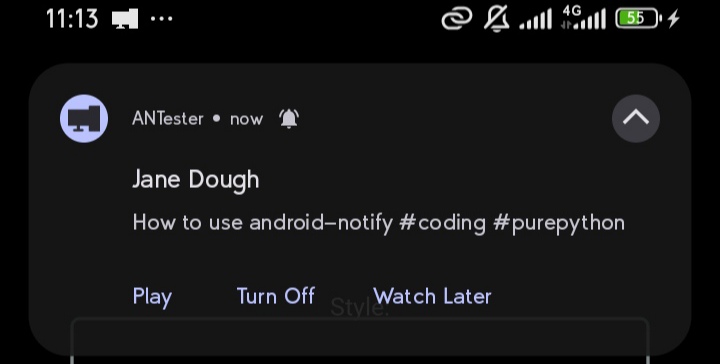\n\n#### Big text notification\n\nWhen using `big_text` style `message` acts as sub-title, Then when notification drop down button is pressed `body` is revealed\n\n```python\nnotification = Notification(\n title=\"Article\",\n message=\"Histroy of Loerm Ipsuim\",\n body=\"Lorem Ipsum is simply dummy text of the printing and ...\",\n style=\"big_text\"\n)\n```\n\n\n\n## Advanced Features\n\n### Updating Notifications\n\n```python\nnotification = Notification(title=\"Initial Title\")\nnotification.send()\n\n# Update title\nnotification.updateTitle(\"New Title\")\n\n# Update message\nnotification.updateMessage(\"New Message\")\n```\n\n### Progress Bar Management\n\n```python\nnotification = Notification(\n title=\"Download..\",\n style=\"progress\"\n)\n# send notification\nnotification.send()\n\n# Update progress\nnotification.updateProgressBar(30, \"30% downloaded\")\n\n# Remove progress bar\n# show_on_update to notification briefly after removed progressbar\nnotification.removeProgressBar(\"Download Complete\",show_on_update=True)\n```\n\n### Adding Style even when already sent\n\nThis is how you add a new style to notification, If already sent or not\n\n```python\nfrom android_notify import NotificationStyles\n\nnotification = Notification(\n title=\"Download..\",\n style=\"progress\"\n)\nnotification.send()\n\nnotification.updateTitle(\"Download Completed\")\nnotification.removeProgressBar()\n\n# Add New Style\nnotification.large_icon_path=\"users/imgs/profile1234.png\"\nnotification.addNotificationStyle(NotificationStyles.LARGE_ICON,already_sent=True)\n\n```\n\n### Channel Management\n\nNotifications are organized into channels. You can customize the channel name and ID:\n\n- Custom Channel Name's Gives User ability to turn on/off specific notifications\n\n```python\nnotification = Notification(\n title=\"Download finished\",\n message=\"How to Catch a Fish.mp4\",\n channel_name=\"Download Notifications\", # Will create User-visible name \"Download Notifications\"\n channel_id=\"downloads_notifications\" # Optional: specify custom channel ID\n)\n```\n\n**Sample Image:** \n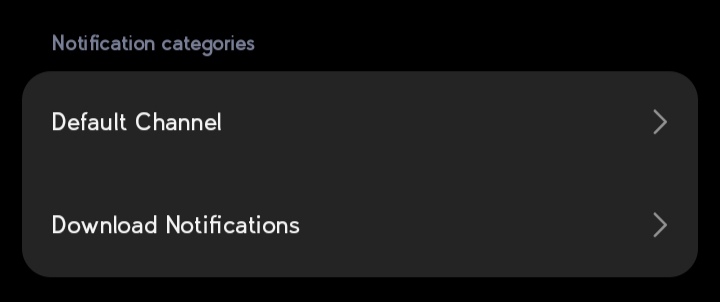\n\n### Silent Notifications\n\nTo send a notification without sound or heads-up display:\n\n```python\nnotification = Notification(title=\"Silent Update\")\nnotification.send(silent=True)\n```\n\n## Functions\n\n```python\nfrom kivymd.app import MDApp\nfrom android_notify import Notification\n\nclass Myapp(MDApp):\n\n def on_start(self):\n Notification(title=\"Hello\", message=\"This is a basic notification.\",callback=self.doSomething).send()\n\n def doSomething(self):\n print(\"print in Debug Console\")\n```\n\n### Get Which Notification was used to Open App - identifer (str)\n\nIf you just want to get the Exact Notification Clicked to Open App, you can use NotificationHandler to get unique identifer\n\n```python\nfrom kivymd.app import MDApp\nfrom android_notify import Notification, NotificationHandler\n\nclass Myapp(MDApp):\n \n def on_start(self):\n \n notify = Notification(title=\"Change Page\", message=\"Click to change App page.\", identifer='change_app_page')\n notify.send()\n\n notify1 = Notification(title=\"Change Colour\", message=\"Click to change App Colour\", identifer='change_app_color')\n notify1.send()\n\n def on_resume(self):\n # Is called everytime app is reopened\n notify_identifer = NotificationHandler.getIdentifer()\n if notify_identifer == 'change_app_page':\n # Code to change Screen\n pass\n elif notify_identifer == 'change_app_color':\n # Code to change Screen Color\n pass\n```\n\n### Assist\n\n- Avoiding Human Error when using different notification styles\n\n```python\nfrom android_notify import Notification, NotificationStyles\nNotification(\n title=\"New Photo\",\n message=\"Check out this image\",\n style=NotificationStyles.BIG_PICTURE,\n big_picture_path=\"assets/imgs/photo.png\"\n).send()\n```\n\n## Development Mode\n\nWhen developing on non-Android platforms, the library provides debugging output:\n\n```python\n# Enable logs (default is True when not on Android)\nNotification.logs = True\n\n# Create notification for testing\nnotification = Notification(title=\"Test\")\nnotification.send()\n# Will print notification properties instead of sending\n```\n\n### Methods\n\n### Instance.**init**\n\nargs\n\n- app_icon: If not specified defaults to app icon, To Change Default app icon use PNG Or Image Will display as a Black Box\n\n### Instance.send\n\nargs\n\n- persistent : To make notification stay after user clicks clear All\n- close_on_click : To close notification on click\n\n### Instance.addButton\n\nargs\n\n- text : Button Text\n\n### Instance.updateTitle\n\nargs\n\n- new_title : String to be set as New notification Title\n\n### Instance.updateMessage\n\nargs\n\n- new_message : String to be set as New notification Message\n\n### Instance.updateProgressBar\n\nargs\n\n- current_value (str): the value from progressbar current progress\n- message (str,optional): defaults to last message\n\n### Instance.removeProgressBar\n\nThis Removes Progress bar\n\nargs\n\n- message (str, optional): notification message, Defaults to 'last message'.\n- show_on_update (bool, optional): To show notification brifely when progressbar removed. Defaults to True.\n\n### Instance.addNotificationStyle\n\nThis is useful to add style after Notification is sent or Add more styles to Notification\nargs\n\n- style: choosen style. All options are in the `NotificationStyles` class `['simple','progress','inbox','big_text','large_icon','big_picture','both_imgs]`\n- already_sent: specfiy if notification.send() method has already been called, it defaults to false\n\n## Image Requirements\n\n- Online Images should start with `http://` or `https://`\n- Local Images must be located within your app's folder\n\n## Error Handling\n\nThe library validates arguments and provides helpful error messages:\n\n- Invalid style names will suggest the closest matching style\n- Invalid arguments will list all valid options\n- Missing image files will list all files in App Directory\n\n## Limitation\n\n1. Only works on Android devices\n\n## Best Practices\n\n1. Always handle permissions appropriately\n2. use `NotificationStyles` to set `style`, use `style=NotificationStyles.LARGE_ICON` instead of `style=\"large_icon\"`\n3. Use meaningful channel names for organization\n4. Keep progress bar updates reasonable (don't update too frequently)\n5. Test notifications on different Android versions\n6. Consider using silent notifications for frequent updates\n\n## Debugging Tips\n\n1. Enable logs during development: `Notification.logs = True`\n2. Check channel creation with Android's notification settings\n3. Verify image paths before sending notifications\n4. Test different styles to ensure proper display\n\nRemember to check Android's notification documentation for best practices and guidelines regarding notification frequency and content.\n\n## Contribution\n\nFeel free to open issues or submit pull requests for improvements!\n\n## Reporting Issues\n\nFound a bug? Please open an issue on our [GitHub Issues](https://github.com/Fector101/android_notify/issues) page.\n\n## Author\n\n- Fabian - <fector101@yahoo.com>\n- GitHub: [Android Notify Repo](https://github.com/Fector101/android_notify)\n- Twitter: [FabianDev_](https://twitter.com/intent/user?user_id=1246911115319263233)\n\nFor feedback or contributions, feel free to reach out!\n\n---\n\n## \u2615 Support the Project\n\nIf you find this project helpful, consider buying me a coffee! \ud83d\ude0a Or Giving it a star on \ud83c\udf1f [GitHub](https://github.com/Fector101/android_notify/) Your support helps maintain and improve the project.\n\n<a href=\"https://www.buymeacoffee.com/fector101\" target=\"_blank\">\n <img src=\"https://cdn.buymeacoffee.com/buttons/v2/default-yellow.png\" alt=\"Buy Me A Coffee\" height=\"60\">\n</a>\n\n---\n\n## Acknowledgments\n\n- This Project was thoroughly Tested by the [Laner Project](https://github.com/Fector101/Laner/) - A application for Securely Transfering Files Wirelessly between your PC and Phone.\n- Thanks to the Kivy and Pyjnius communities.\n\n---\n\n## \ud83c\udf10 **Links**\n\n- **PyPI:** [android-notify on PyPI](https://pypi.org/project/android-notify/)\n- **GitHub:** [Source Code Repository](https://github.com/Fector101/android_notify/)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python package that simpilfies creating Android notifications in Kivy apps.",
"version": "1.53.1",
"project_urls": {
"Documentation": "https://github.com/fector101/android-notify/",
"Funding": "https://www.buymeacoffee.com/fector101",
"Homepage": "https://github.com/fector101/android-notify",
"Source": "https://github.com/fector101/android-notify",
"Tracker": "https://github.com/fector101/android-notify/issues"
},
"split_keywords": [
"android",
" notifications",
" kivy",
" mobile",
" post-notifications",
" pyjnius",
" android-notifications",
" kivy-notifications",
" python-android",
" mobile-development",
" push-notifications",
" mobile-app",
" kivy-application"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "9c8489fa9ca6ef8ff27ea78e47b42984fc1eb6f5fdb6cf3746d79c40d9750ee7",
"md5": "d7b4e793b863dce0080b9474d18e6642",
"sha256": "3de2546dfcb20f0cbff63d8ba82224685db064fb984ad60885d994d6c8c989bb"
},
"downloads": -1,
"filename": "android_notify-1.53.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "d7b4e793b863dce0080b9474d18e6642",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 17834,
"upload_time": "2025-02-22T17:06:15",
"upload_time_iso_8601": "2025-02-22T17:06:15.454076Z",
"url": "https://files.pythonhosted.org/packages/9c/84/89fa9ca6ef8ff27ea78e47b42984fc1eb6f5fdb6cf3746d79c40d9750ee7/android_notify-1.53.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "d8daf47ac217b447f955a9255b0c5f7121d2f67dc2945fa67331fe34d8e20598",
"md5": "6fcfdae6ad74764a387145190529532b",
"sha256": "02f134006aebee0a32a1d923ec8e4616d6f5ee16374d2eb3e94c371e975db8c5"
},
"downloads": -1,
"filename": "android_notify-1.53.1.tar.gz",
"has_sig": false,
"md5_digest": "6fcfdae6ad74764a387145190529532b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 20650,
"upload_time": "2025-02-22T17:06:23",
"upload_time_iso_8601": "2025-02-22T17:06:23.702004Z",
"url": "https://files.pythonhosted.org/packages/d8/da/f47ac217b447f955a9255b0c5f7121d2f67dc2945fa67331fe34d8e20598/android_notify-1.53.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-02-22 17:06:23",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "fector101",
"github_project": "android-notify",
"github_not_found": true,
"lcname": "android-notify"
}