Name | api-analytics JSON |
Version |
1.2.6
JSON |
| download |
home_page | https://github.com/tom-draper/api-analytics |
Summary | Monitoring and analytics for Python API frameworks. |
upload_time | 2025-03-14 23:10:52 |
maintainer | None |
docs_url | None |
author | Tom Draper |
requires_python | >=3.6 |
license | MIT License Copyright (c) 2023 Tom Draper Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
analytics
api
dashboard
django
fastapi
flask
tornado
middleware
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# API Analytics
A free and lightweight API analytics solution, complete with a dashboard.
## Getting Started
### 1. Generate an API key
Head to [apianalytics.dev/generate](https://apianalytics.dev/generate) to generate your unique API key with a single click. This key is used to monitor your specific API and should be stored privately. It's also required in order to access your API analytics dashboard and data.
### 2. Add middleware to your API
Add our lightweight middleware to your API. Almost all processing is handled by our servers so there is minimal impact on the performance of your API.
#### FastAPI
[](https://pypi.org/project/api-analytics)
```bash
pip install api-analytics[fastapi]
```
```py
import uvicorn
from fastapi import FastAPI
from api_analytics.fastapi import Analytics
app = FastAPI()
app.add_middleware(Analytics, api_key=<API-KEY>) # Add middleware
@app.get('/')
async def root():
return {'message': 'Hello World!'}
if __name__ == "__main__":
uvicorn.run("app:app", reload=True)
```
#### Flask
[](https://pypi.org/project/api-analytics)
```bash
pip install api-analytics[flask]
```
```py
from flask import Flask
from api_analytics.flask import add_middleware
app = Flask(__name__)
add_middleware(app, <API-KEY>) # Add middleware
@app.get('/')
def root():
return {'message': 'Hello World!'}
if __name__ == "__main__":
app.run()
```
#### Django
[](https://pypi.org/project/api-analytics)
```bash
pip install api-analytics[django]
```
Assign your API key to `ANALYTICS_API_KEY` in `settings.py` and add the Analytics middleware to the top of your middleware stack.
```py
ANALYTICS_API_KEY = <API-KEY>
MIDDLEWARE = [
'api_analytics.django.Analytics', # Add middleware
...
]
```
#### Tornado
[](https://pypi.org/project/api-analytics)
```bash
pip install api-analytics[tornado]
```
Modify your handler to inherit from `Analytics`. Create a `__init__()` method, passing along the application and response along with your unique API key.
```py
import asyncio
from tornado.web import Application
from api_analytics.tornado import Analytics
# Inherit from the Analytics middleware class
class MainHandler(Analytics):
def __init__(self, app, res):
super().__init__(app, res, <API-KEY>) # Provide api key
def get(self):
self.write({'message': 'Hello World!'})
def make_app():
return Application([
(r"/", MainHandler),
])
if __name__ == "__main__":
app = make_app()
app.listen(8080)
IOLoop.instance().start()
```
### 3. View your analytics
Your API will now log and store incoming request data on all routes. Your logged data can be viewed using two methods:
1. Through visualizations and statistics on the dashboard
2. Accessed directly via the data API
You can use the same API key across multiple APIs, but all of your data will appear in the same dashboard. We recommend generating a new API key for each additional API server you want analytics for.
#### Dashboard
Head to [apianalytics.dev/dashboard](https://apianalytics.dev/dashboard) and paste in your API key to access your dashboard.
Demo: [apianalytics.dev/dashboard/demo](https://apianalytics.dev/dashboard/demo)
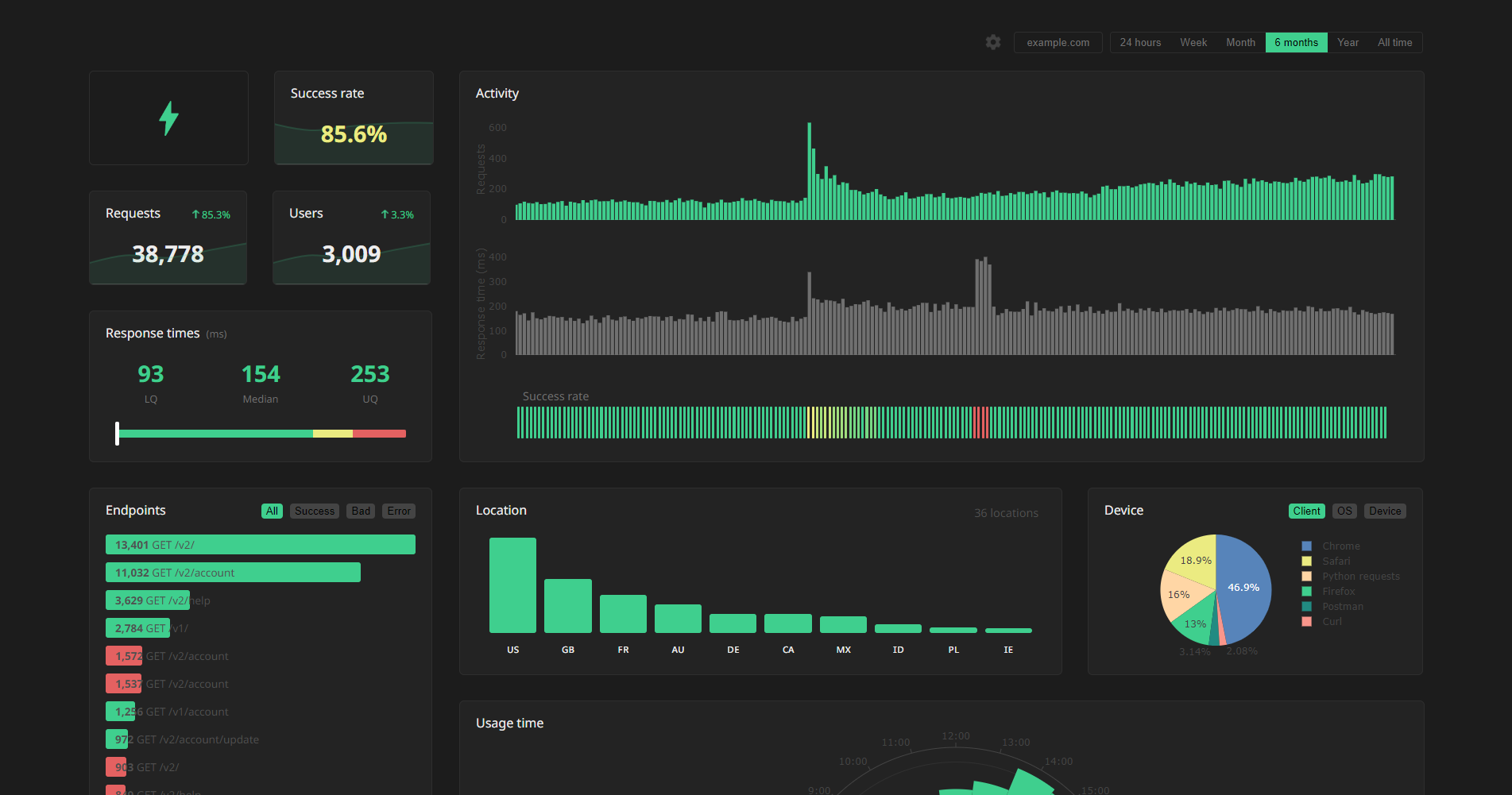
#### Data API
Logged data for all requests can be accessed via our REST API. Simply send a GET request to `https://apianalytics-server.com/api/data` with your API key set as `X-AUTH-TOKEN` in the headers.
##### Python
```py
import requests
headers = {
"X-AUTH-TOKEN": <API-KEY>
}
response = requests.get("https://apianalytics-server.com/api/data", headers=headers)
print(response.json())
```
##### Node.js
```js
fetch("https://apianalytics-server.com/api/data", {
headers: { "X-AUTH-TOKEN": <API-KEY> },
})
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
});
```
##### cURL
```bash
curl --header "X-AUTH-TOKEN: <API-KEY>" https://apianalytics-server.com/api/data
```
##### Parameters
You can filter your data by providing URL parameters in your request.
- `page` - the page number, with a max page size of 50,000 (defaults to 1)
- `date` - the exact day the requests occurred on (`YYYY-MM-DD`)
- `dateFrom` - a lower bound of a date range the requests occurred in (`YYYY-MM-DD`)
- `dateTo` - a upper bound of a date range the requests occurred in (`YYYY-MM-DD`)
- `hostname` - the hostname of your service
- `ipAddress` - the IP address of the client
- `status` - the status code of the response
- `location` - a two-character location code of the client
- `user_id` - a custom user identifier (only relevant if a `get_user_id` mapper function has been set)
Example:
```bash
curl --header "X-AUTH-TOKEN: <API-KEY>" https://apianalytics-server.com/api/data?page=3&dateFrom=2022-01-01&hostname=apianalytics.dev&status=200&user_id=b56cbd92-1168-4d7b-8d94-0418da207908
```
## Customisation
Custom mapping functions can be assigned to override the default behaviour and define how values are extracted from each incoming request to better suit your specific API.
### FastAPI
```py
from fastapi import FastAPI
from api_analytics.fastapi import Analytics, Config
config = Config()
config.get_ip_address = lambda request: request.headers.get('X-Forwarded-For', request.client.host)
config.get_user_agent = lambda request: request.headers.get('User-Agent', '')
app = FastAPI()
app.add_middleware(Analytics, api_key=<API-KEY>, config=config) # Add middleware
```
### Flask
```py
from flask import Flask
from api_analytics.flask import add_middleware, Config
app = Flask(__name__)
config = Config()
config.get_ip_address = lambda request: request.headers['X-Forwarded-For']
config.get_user_agent = lambda request: request.headers['User-Agent']
add_middleware(app, <API-KEY>, config) # Add middleware
```
### Django
Assign your config to `ANALYTICS_CONFIG` in `settings.py`.
```py
from api_analytics.django import Config
config = Config()
config.get_ip_address = lambda request: request.headers['X-Forwarded-For']
config.get_user_agent = lambda request: request.headers['User-Agent']
ANALYTICS_CONFIG = config
```
### Tornado
```py
from api_analytics.tornado import Analytics, Config
class MainHandler(Analytics):
def __init__(self, app, res):
config = Config()
config.get_ip_address = lambda request: request.headers['X-Forwarded-For']
config.get_user_agent = lambda request: request.headers['User-Agent']
super().__init__(app, res, <API-KEY>, config) # Provide api key
```
## Client ID and Privacy
By default, API Analytics logs and stores the client IP address of all incoming requests made to your API and infers a location (country) from each IP address if possible. The IP address is used as a form of client identification in the dashboard to estimate the number of users accessing your service.
This behaviour can be controlled through a privacy level defined in the configuration of the API middleware. There are three privacy levels to choose from 0 (default) to a maximum of 2. A privacy level of 1 will disable IP address storing, and a value of 2 will also disable location inference.
Privacy Levels:
- `0` - The client IP address is used to infer a location and then stored for user identification. (default)
- `1` - The client IP address is used to infer a location and then discarded.
- `2` - The client IP address is never accessed and location is never inferred.
```py
config = Config()
config.privacy_level = 2 # Disable IP storing and location inference
```
With any of these privacy levels, there is the option to define a custom user ID as a function of a request by providing a mapper function in the API middleware configuration. For example, your service may require an API key sent in the `X-AUTH-TOKEN` header field that can be used to identify a user. In the dashboard, this custom user ID will identify the user in conjunction with the IP address or as an alternative.
```py
config = Config()
config.get_user_id = lambda request: request.headers.get('X-AUTH-TOKEN', '')
```
## Data and Security
All data is stored securely in compliance with The EU General Data Protection Regulation (GDPR).
For any given request to your API, data recorded is limited to:
- Path requested by client
- Client IP address (optional)
- Client operating system
- Client browser
- Request method (GET, POST, PUT, etc.)
- Time of request
- Status code
- Response time
- API hostname
- API framework (FastAPI, Flask, Django, Tornado)
Data collected is only ever used to populate your analytics dashboard. All stored data is pseudo-anonymous, with the API key the only link between you and your logged request data. Should you lose your API key, you will have no method to access your API analytics.
### Data Deletion
At any time you can delete all stored data associated with your API key by going to [apianalytics.dev/delete](https://apianalytics.dev/delete) and entering your API key.
API keys and their associated logged request data are scheduled to be deleted after 6 months of inactivity.
## Monitoring
Active API monitoring can be set up by heading to [apianalytics.dev/monitoring](https://apianalytics.dev/monitoring) to enter your API key. Our servers will regularly ping chosen API endpoints to monitor uptime and response time.
<!-- Optional email alerts when your endpoints are down can be subscribed to. -->
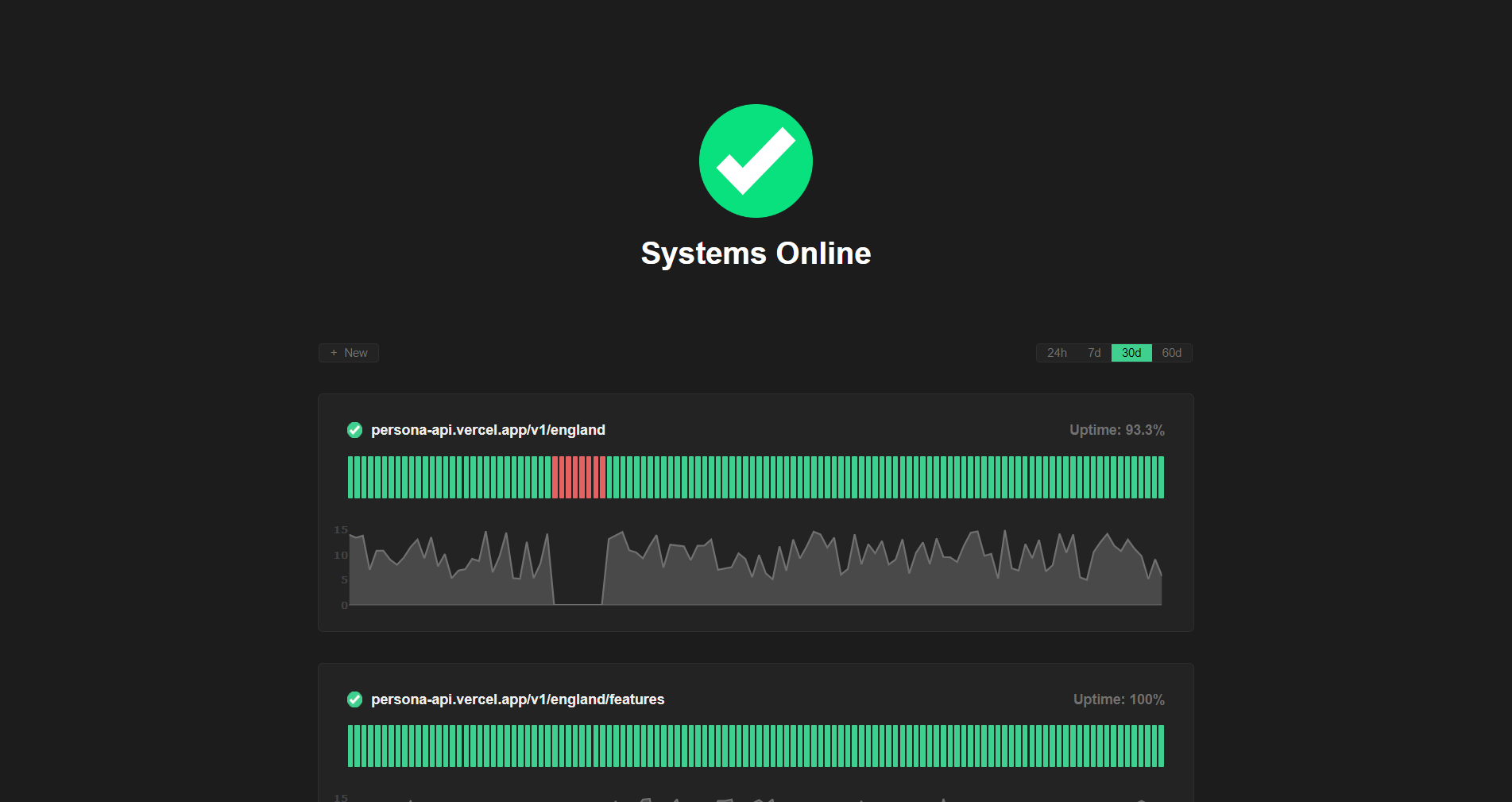
## Contributions
Contributions, issues and feature requests are welcome.
- Fork it (https://github.com/tom-draper/api-analytics)
- Create your feature branch (`git checkout -b my-new-feature`)
- Commit your changes (`git commit -am 'Add some feature'`)
- Push to the branch (`git push origin my-new-feature`)
- Create a new Pull Request
---
If you find value in my work consider supporting me.
Buy Me a Coffee: https://www.buymeacoffee.com/tomdraper<br>
PayPal: https://www.paypal.com/paypalme/tomdraper
Raw data
{
"_id": null,
"home_page": "https://github.com/tom-draper/api-analytics",
"name": "api-analytics",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "analytics, api, dashboard, django, fastapi, flask, tornado, middleware",
"author": "Tom Draper",
"author_email": "Tom Draper <tomjdraper1@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/cd/c2/8982e5c6da1597653a4ad435747975d30fcb5ef2492251a24f84815cbd18/api_analytics-1.2.6.tar.gz",
"platform": null,
"description": "# API Analytics\r\n\r\nA free and lightweight API analytics solution, complete with a dashboard.\r\n\r\n## Getting Started\r\n\r\n### 1. Generate an API key\r\n\r\nHead to [apianalytics.dev/generate](https://apianalytics.dev/generate) to generate your unique API key with a single click. This key is used to monitor your specific API and should be stored privately. It's also required in order to access your API analytics dashboard and data.\r\n\r\n### 2. Add middleware to your API\r\n\r\nAdd our lightweight middleware to your API. Almost all processing is handled by our servers so there is minimal impact on the performance of your API.\r\n\r\n#### FastAPI\r\n\r\n[](https://pypi.org/project/api-analytics)\r\n\r\n```bash\r\npip install api-analytics[fastapi]\r\n```\r\n\r\n```py\r\nimport uvicorn\r\nfrom fastapi import FastAPI\r\nfrom api_analytics.fastapi import Analytics\r\n\r\napp = FastAPI()\r\napp.add_middleware(Analytics, api_key=<API-KEY>) # Add middleware\r\n\r\n@app.get('/')\r\nasync def root():\r\n return {'message': 'Hello World!'}\r\n\r\nif __name__ == \"__main__\":\r\n uvicorn.run(\"app:app\", reload=True)\r\n```\r\n\r\n#### Flask\r\n\r\n[](https://pypi.org/project/api-analytics)\r\n\r\n```bash\r\npip install api-analytics[flask]\r\n```\r\n\r\n```py\r\nfrom flask import Flask\r\nfrom api_analytics.flask import add_middleware\r\n\r\napp = Flask(__name__)\r\nadd_middleware(app, <API-KEY>) # Add middleware\r\n\r\n@app.get('/')\r\ndef root():\r\n return {'message': 'Hello World!'}\r\n\r\nif __name__ == \"__main__\":\r\n app.run()\r\n```\r\n\r\n#### Django\r\n\r\n[](https://pypi.org/project/api-analytics)\r\n\r\n```bash\r\npip install api-analytics[django]\r\n```\r\n\r\nAssign your API key to `ANALYTICS_API_KEY` in `settings.py` and add the Analytics middleware to the top of your middleware stack.\r\n\r\n```py\r\nANALYTICS_API_KEY = <API-KEY>\r\n\r\nMIDDLEWARE = [\r\n 'api_analytics.django.Analytics', # Add middleware\r\n ...\r\n]\r\n```\r\n\r\n#### Tornado\r\n\r\n[](https://pypi.org/project/api-analytics)\r\n\r\n```bash\r\npip install api-analytics[tornado]\r\n```\r\n\r\nModify your handler to inherit from `Analytics`. Create a `__init__()` method, passing along the application and response along with your unique API key.\r\n\r\n```py\r\nimport asyncio\r\nfrom tornado.web import Application\r\nfrom api_analytics.tornado import Analytics\r\n\r\n# Inherit from the Analytics middleware class\r\nclass MainHandler(Analytics):\r\n def __init__(self, app, res):\r\n super().__init__(app, res, <API-KEY>) # Provide api key\r\n \r\n def get(self):\r\n self.write({'message': 'Hello World!'})\r\n\r\ndef make_app():\r\n return Application([\r\n (r\"/\", MainHandler),\r\n ])\r\n\r\nif __name__ == \"__main__\":\r\n app = make_app()\r\n app.listen(8080)\r\n IOLoop.instance().start()\r\n```\r\n\r\n### 3. View your analytics\r\n\r\nYour API will now log and store incoming request data on all routes. Your logged data can be viewed using two methods:\r\n\r\n1. Through visualizations and statistics on the dashboard\r\n2. Accessed directly via the data API\r\n\r\nYou can use the same API key across multiple APIs, but all of your data will appear in the same dashboard. We recommend generating a new API key for each additional API server you want analytics for.\r\n\r\n#### Dashboard\r\n\r\nHead to [apianalytics.dev/dashboard](https://apianalytics.dev/dashboard) and paste in your API key to access your dashboard.\r\n\r\nDemo: [apianalytics.dev/dashboard/demo](https://apianalytics.dev/dashboard/demo)\r\n\r\n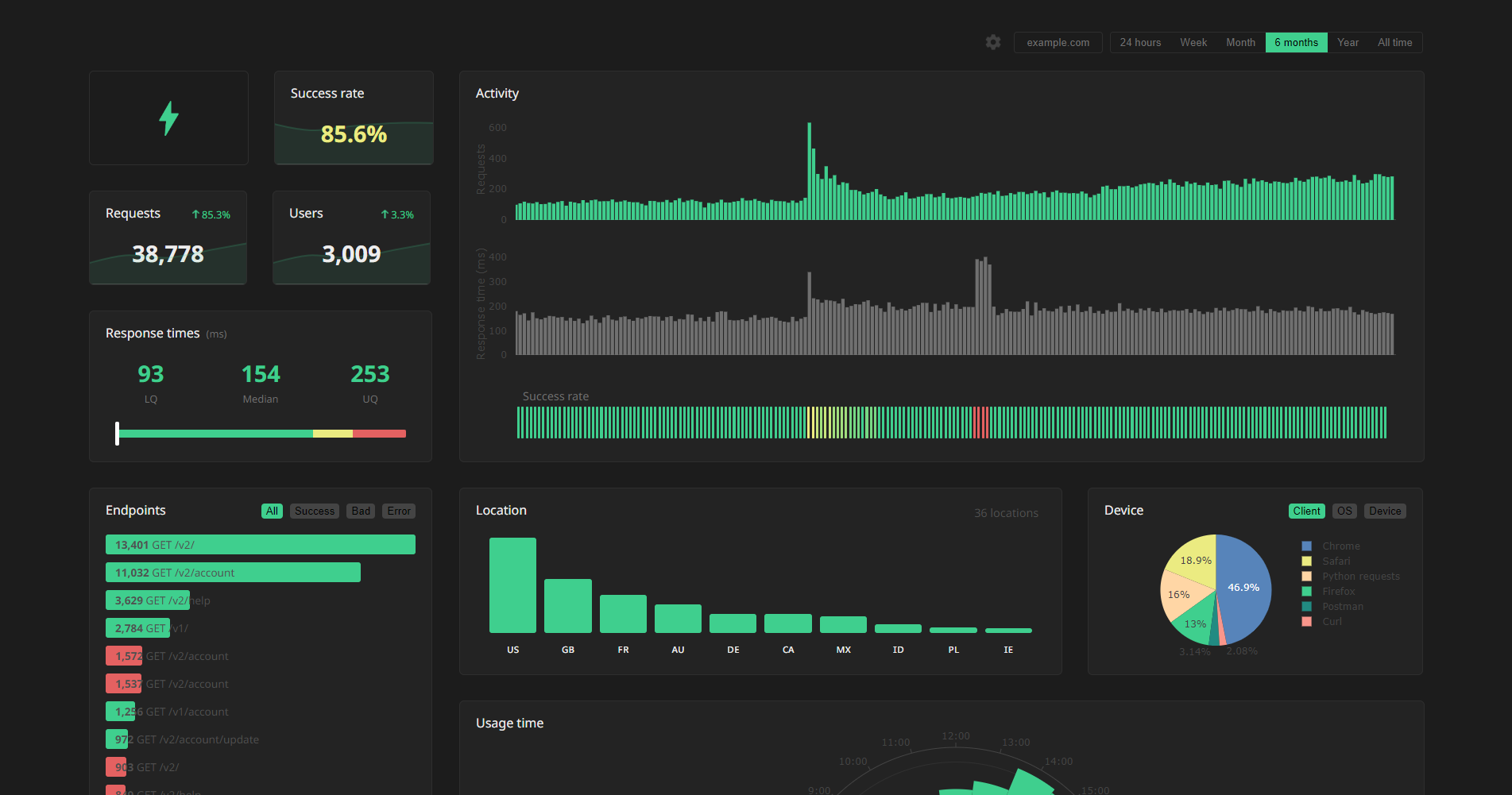\r\n\r\n#### Data API\r\n\r\nLogged data for all requests can be accessed via our REST API. Simply send a GET request to `https://apianalytics-server.com/api/data` with your API key set as `X-AUTH-TOKEN` in the headers.\r\n\r\n##### Python\r\n\r\n```py\r\nimport requests\r\n\r\nheaders = {\r\n \"X-AUTH-TOKEN\": <API-KEY>\r\n}\r\n\r\nresponse = requests.get(\"https://apianalytics-server.com/api/data\", headers=headers)\r\nprint(response.json())\r\n```\r\n\r\n##### Node.js\r\n\r\n```js\r\nfetch(\"https://apianalytics-server.com/api/data\", {\r\n headers: { \"X-AUTH-TOKEN\": <API-KEY> },\r\n})\r\n .then((response) => {\r\n return response.json();\r\n })\r\n .then((data) => {\r\n console.log(data);\r\n });\r\n```\r\n\r\n##### cURL\r\n\r\n```bash\r\ncurl --header \"X-AUTH-TOKEN: <API-KEY>\" https://apianalytics-server.com/api/data\r\n```\r\n\r\n##### Parameters\r\n\r\nYou can filter your data by providing URL parameters in your request.\r\n\r\n- `page` - the page number, with a max page size of 50,000 (defaults to 1)\r\n- `date` - the exact day the requests occurred on (`YYYY-MM-DD`)\r\n- `dateFrom` - a lower bound of a date range the requests occurred in (`YYYY-MM-DD`)\r\n- `dateTo` - a upper bound of a date range the requests occurred in (`YYYY-MM-DD`)\r\n- `hostname` - the hostname of your service\r\n- `ipAddress` - the IP address of the client\r\n- `status` - the status code of the response\r\n- `location` - a two-character location code of the client\r\n- `user_id` - a custom user identifier (only relevant if a `get_user_id` mapper function has been set)\r\n\r\nExample:\r\n\r\n```bash\r\ncurl --header \"X-AUTH-TOKEN: <API-KEY>\" https://apianalytics-server.com/api/data?page=3&dateFrom=2022-01-01&hostname=apianalytics.dev&status=200&user_id=b56cbd92-1168-4d7b-8d94-0418da207908\r\n```\r\n\r\n## Customisation\r\n\r\nCustom mapping functions can be assigned to override the default behaviour and define how values are extracted from each incoming request to better suit your specific API.\r\n\r\n### FastAPI\r\n\r\n```py\r\nfrom fastapi import FastAPI\r\nfrom api_analytics.fastapi import Analytics, Config\r\n\r\nconfig = Config()\r\nconfig.get_ip_address = lambda request: request.headers.get('X-Forwarded-For', request.client.host)\r\nconfig.get_user_agent = lambda request: request.headers.get('User-Agent', '')\r\n\r\napp = FastAPI()\r\napp.add_middleware(Analytics, api_key=<API-KEY>, config=config) # Add middleware\r\n```\r\n\r\n### Flask\r\n\r\n```py\r\nfrom flask import Flask\r\nfrom api_analytics.flask import add_middleware, Config\r\n\r\napp = Flask(__name__)\r\nconfig = Config()\r\nconfig.get_ip_address = lambda request: request.headers['X-Forwarded-For']\r\nconfig.get_user_agent = lambda request: request.headers['User-Agent']\r\nadd_middleware(app, <API-KEY>, config) # Add middleware\r\n```\r\n\r\n### Django\r\n\r\nAssign your config to `ANALYTICS_CONFIG` in `settings.py`.\r\n\r\n```py\r\nfrom api_analytics.django import Config\r\n\r\nconfig = Config()\r\nconfig.get_ip_address = lambda request: request.headers['X-Forwarded-For']\r\nconfig.get_user_agent = lambda request: request.headers['User-Agent']\r\nANALYTICS_CONFIG = config\r\n```\r\n\r\n### Tornado\r\n\r\n```py\r\nfrom api_analytics.tornado import Analytics, Config\r\n\r\nclass MainHandler(Analytics):\r\n def __init__(self, app, res):\r\n config = Config()\r\n config.get_ip_address = lambda request: request.headers['X-Forwarded-For']\r\n config.get_user_agent = lambda request: request.headers['User-Agent']\r\n super().__init__(app, res, <API-KEY>, config) # Provide api key\r\n```\r\n\r\n## Client ID and Privacy\r\n\r\nBy default, API Analytics logs and stores the client IP address of all incoming requests made to your API and infers a location (country) from each IP address if possible. The IP address is used as a form of client identification in the dashboard to estimate the number of users accessing your service.\r\n\r\nThis behaviour can be controlled through a privacy level defined in the configuration of the API middleware. There are three privacy levels to choose from 0 (default) to a maximum of 2. A privacy level of 1 will disable IP address storing, and a value of 2 will also disable location inference.\r\n\r\nPrivacy Levels:\r\n\r\n- `0` - The client IP address is used to infer a location and then stored for user identification. (default)\r\n- `1` - The client IP address is used to infer a location and then discarded.\r\n- `2` - The client IP address is never accessed and location is never inferred.\r\n\r\n```py\r\nconfig = Config()\r\nconfig.privacy_level = 2 # Disable IP storing and location inference\r\n```\r\n\r\nWith any of these privacy levels, there is the option to define a custom user ID as a function of a request by providing a mapper function in the API middleware configuration. For example, your service may require an API key sent in the `X-AUTH-TOKEN` header field that can be used to identify a user. In the dashboard, this custom user ID will identify the user in conjunction with the IP address or as an alternative.\r\n\r\n```py\r\nconfig = Config()\r\nconfig.get_user_id = lambda request: request.headers.get('X-AUTH-TOKEN', '')\r\n```\r\n\r\n## Data and Security\r\n\r\nAll data is stored securely in compliance with The EU General Data Protection Regulation (GDPR).\r\n\r\nFor any given request to your API, data recorded is limited to:\r\n\r\n- Path requested by client\r\n- Client IP address (optional)\r\n- Client operating system\r\n- Client browser\r\n- Request method (GET, POST, PUT, etc.)\r\n- Time of request\r\n- Status code\r\n- Response time\r\n- API hostname\r\n- API framework (FastAPI, Flask, Django, Tornado)\r\n\r\nData collected is only ever used to populate your analytics dashboard. All stored data is pseudo-anonymous, with the API key the only link between you and your logged request data. Should you lose your API key, you will have no method to access your API analytics.\r\n\r\n### Data Deletion\r\n\r\nAt any time you can delete all stored data associated with your API key by going to [apianalytics.dev/delete](https://apianalytics.dev/delete) and entering your API key.\r\n\r\nAPI keys and their associated logged request data are scheduled to be deleted after 6 months of inactivity.\r\n\r\n## Monitoring\r\n\r\nActive API monitoring can be set up by heading to [apianalytics.dev/monitoring](https://apianalytics.dev/monitoring) to enter your API key. Our servers will regularly ping chosen API endpoints to monitor uptime and response time. \r\n<!-- Optional email alerts when your endpoints are down can be subscribed to. -->\r\n\r\n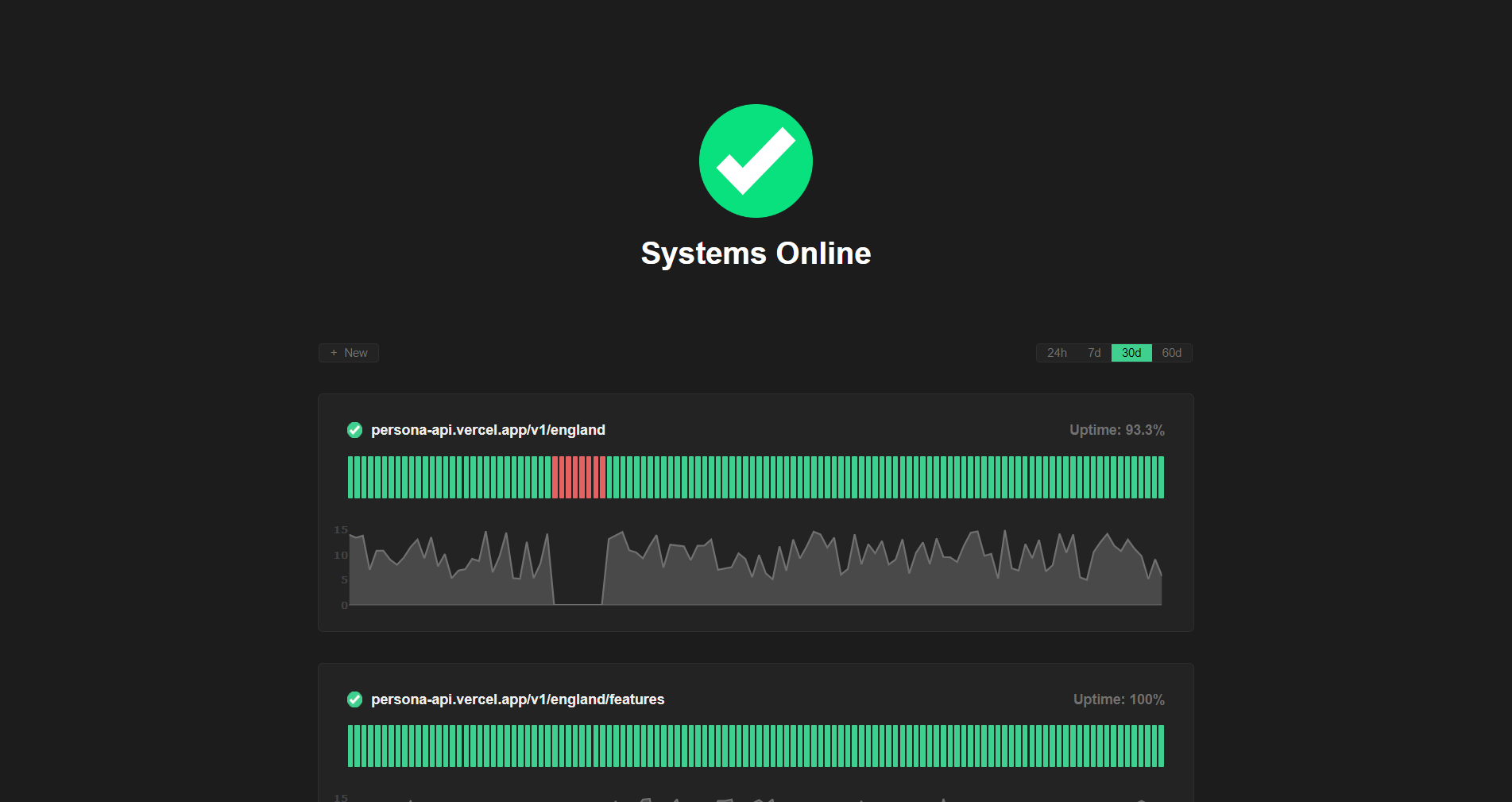\r\n\r\n## Contributions\r\n\r\nContributions, issues and feature requests are welcome.\r\n\r\n- Fork it (https://github.com/tom-draper/api-analytics)\r\n- Create your feature branch (`git checkout -b my-new-feature`)\r\n- Commit your changes (`git commit -am 'Add some feature'`)\r\n- Push to the branch (`git push origin my-new-feature`)\r\n- Create a new Pull Request\r\n\r\n---\r\n\r\nIf you find value in my work consider supporting me.\r\n\r\nBuy Me a Coffee: https://www.buymeacoffee.com/tomdraper<br>\r\nPayPal: https://www.paypal.com/paypalme/tomdraper\r\n\r\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2023 Tom Draper Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Monitoring and analytics for Python API frameworks.",
"version": "1.2.6",
"project_urls": {
"Homepage": "https://github.com/tom-draper/api-analytics",
"repository": "https://github.com/tom-draper/api-analytics"
},
"split_keywords": [
"analytics",
" api",
" dashboard",
" django",
" fastapi",
" flask",
" tornado",
" middleware"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "26f0d3b1509f631a50c6f42376e41611206a9427a9139979109bffc3b578f014",
"md5": "3383dcda60a97b1b05b3d3df69ea9744",
"sha256": "25f2fb7b814cae024e7462bc8993fafafe02528c2d0161d9001ca6458702e61c"
},
"downloads": -1,
"filename": "api_analytics-1.2.6-py3-none-any.whl",
"has_sig": false,
"md5_digest": "3383dcda60a97b1b05b3d3df69ea9744",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 13872,
"upload_time": "2025-03-14T23:10:51",
"upload_time_iso_8601": "2025-03-14T23:10:51.269452Z",
"url": "https://files.pythonhosted.org/packages/26/f0/d3b1509f631a50c6f42376e41611206a9427a9139979109bffc3b578f014/api_analytics-1.2.6-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "cdc28982e5c6da1597653a4ad435747975d30fcb5ef2492251a24f84815cbd18",
"md5": "89a0267b17bb825e21b15466ade88f81",
"sha256": "cf016d91ea933bb17e57727d97013eb03c42ee813dd1ac5186b62bdf5e7ea064"
},
"downloads": -1,
"filename": "api_analytics-1.2.6.tar.gz",
"has_sig": false,
"md5_digest": "89a0267b17bb825e21b15466ade88f81",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 14624,
"upload_time": "2025-03-14T23:10:52",
"upload_time_iso_8601": "2025-03-14T23:10:52.585153Z",
"url": "https://files.pythonhosted.org/packages/cd/c2/8982e5c6da1597653a4ad435747975d30fcb5ef2492251a24f84815cbd18/api_analytics-1.2.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-03-14 23:10:52",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "tom-draper",
"github_project": "api-analytics",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "api-analytics"
}