Name | arrayviz JSON |
Version |
0.0.1
JSON |
| download |
home_page | None |
Summary | A Python package for visualizing array-based algorithms using Manim |
upload_time | 2024-08-01 18:50:04 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.8 |
license | None |
keywords |
array
visualization
manim
animation
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# ArrayViz
<!-- badges: start -->
| | |
| --- | --- |
| Meta | [](https://opensource.org/licenses/MIT) |
| Testing | [](https://github.com/aanas-sayed/arrayviz/actions/workflows/lint_and_test.yaml) [](https://github.com/aanas-sayed/arrayviz/actions/workflows/build_and_release.yaml) |
<!-- badges: end -->
This package allows users to pass a function and visualize it as blocks and elements using Manim, a powerful mathematical animation engine. It provides an easy way to animate algorithms step-by-step, helping with educational purposes and debugging.
Note
This project is a work in progress. Package has not been released yet but is planned to be. Only limited functionality is implemented as of now.
## Installation
First, install Manim according to the instructions on [Manim's official website](https://docs.manim.community/en/stable/installation.html).
Install the package via PyPI:
```bash
python -m pip install --upgrade arrayviz
```
## Usage
### ArrayBlockVisualizer Class
The `ArrayBlockVisualizer` class is designed to animate the execution of a user-provided function. Users can control when the pointers should update in relation to the array frame updates (before, at the same time, or after).
#### Parameters
- `func`: The user-provided function to animate.
- `func_args`: Arguments to pass to the user-provided function.
- `delay` (float): The delay time between frames in seconds. Default is 1.
- `**kwargs`: Additional keyword arguments for the `Scene` superclass.
### Example
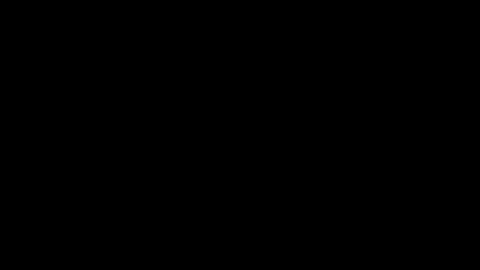
The following example demonstrates how to use the `ArrayBlockVisualizer` class to animate an example merge function for sorted arrays.
**`examples/merge_sorted_arrays.py`**
```python
from arrayviz import ArrayBlockVisualizer
def merge(nums1, m, nums2, n, record_frame):
"""
Merges two sorted arrays nums1 and nums2 into a single sorted array in place.
Args:
nums1 (list): The first sorted array with extra space at the end to hold nums2 elements.
m (int): The number of valid elements in nums1.
nums2 (list): The second sorted array.
n (int): The number of elements in nums2.
record_frame (callable): A callback function to record the state of arrays and pointers.
"""
merged_index = m + n
# Record initial array
record_frame(arrays=[nums1[:], nums2[:]])
while m > 0 and n > 0:
# Record pointers
record_frame(pointers=[{"i": m - 1}, {"j": n - 1}])
if nums1[m - 1] > nums2[n - 1]:
nums1[merged_index - 1] = nums1[m - 1]
m -= 1
else:
nums1[merged_index - 1] = nums2[n - 1]
n -= 1
merged_index -= 1
# Record new array
record_frame(arrays=[nums1[:], nums2[:]])
# Record final pointers
record_frame(pointers=[{"i": n - 1}, {"j": n - 1}])
nums1[:n] = nums2[:n]
# Record final array
record_frame(arrays=[nums1[:], nums2[:]])
# Initial arrays for the merge function
nums1 = [4, 5, 7, 0, 0, 0, 0, 0, 0]
nums2 = [1, 2, 2, 3, 5, 6]
m = 3 # Number of initial valid elements in nums1
n = 6 # Number of elements in nums2
class MergeScene(ArrayBlockVisualizer):
"""
A Manim scene to visualize the merge function using the FunctionAnimation class.
"""
def __init__(self, **kwargs):
"""
Initializes the MergeScene with the merge function and its arguments.
Args:
**kwargs: Additional keyword arguments for the ArrayBlockVisualizer superclass.
"""
super().__init__(merge, (nums1, m, nums2, n), **kwargs)
# To render this scene, use the following command in your terminal:
# manim -pql examples/merge_sorted_arrays.py MergeScene
```
### Running the Example
To render the `MergeScene`, run the following command in your terminal:
```bash
manim -pql example.py MergeScene
```
- `-pql` stands for play in low quality. You can adjust this based on the desired rendering quality.
## License
This project is licensed under the MIT License - see the LICENSE file for details.
## Contributing
Contributions are welcome! Please open an issue or submit a pull request for any improvements or bug fixes.
## Acknowledgements
[Manim Community](https://github.com/ManimCommunity/manim) for developing the Manim library.
Raw data
{
"_id": null,
"home_page": null,
"name": "arrayviz",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "Aanas Sayed <aanas.sayed@outlook.com>",
"keywords": "array, visualization, manim, animation",
"author": null,
"author_email": "Aanas Sayed <aanas.sayed@outlook.com>",
"download_url": "https://files.pythonhosted.org/packages/5c/86/c07e5ccc4692285e24395c8ac66e1822b5d4e04e471f07c869c557689ae7/arrayviz-0.0.1.tar.gz",
"platform": null,
"description": "# ArrayViz\n\n<!-- badges: start -->\n| | |\n| --- | --- |\n| Meta | [](https://opensource.org/licenses/MIT) |\n| Testing | [](https://github.com/aanas-sayed/arrayviz/actions/workflows/lint_and_test.yaml) [](https://github.com/aanas-sayed/arrayviz/actions/workflows/build_and_release.yaml) |\n\n<!-- badges: end -->\n\nThis package allows users to pass a function and visualize it as blocks and elements using Manim, a powerful mathematical animation engine. It provides an easy way to animate algorithms step-by-step, helping with educational purposes and debugging.\n\nNote\n\nThis project is a work in progress. Package has not been released yet but is planned to be. Only limited functionality is implemented as of now.\n\n## Installation\n\nFirst, install Manim according to the instructions on [Manim's official website](https://docs.manim.community/en/stable/installation.html).\n\nInstall the package via PyPI:\n\n```bash\npython -m pip install --upgrade arrayviz\n```\n\n## Usage\n\n### ArrayBlockVisualizer Class\n\nThe `ArrayBlockVisualizer` class is designed to animate the execution of a user-provided function. Users can control when the pointers should update in relation to the array frame updates (before, at the same time, or after).\n\n#### Parameters\n\n- `func`: The user-provided function to animate.\n- `func_args`: Arguments to pass to the user-provided function.\n- `delay` (float): The delay time between frames in seconds. Default is 1.\n- `**kwargs`: Additional keyword arguments for the `Scene` superclass.\n\n### Example\n\n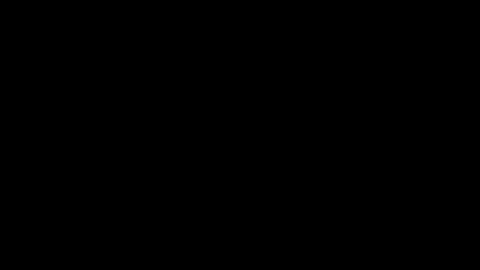\n\nThe following example demonstrates how to use the `ArrayBlockVisualizer` class to animate an example merge function for sorted arrays.\n\n**`examples/merge_sorted_arrays.py`**\n\n```python\nfrom arrayviz import ArrayBlockVisualizer\n\n\ndef merge(nums1, m, nums2, n, record_frame):\n \"\"\"\n Merges two sorted arrays nums1 and nums2 into a single sorted array in place.\n\n Args:\n nums1 (list): The first sorted array with extra space at the end to hold nums2 elements.\n m (int): The number of valid elements in nums1.\n nums2 (list): The second sorted array.\n n (int): The number of elements in nums2.\n record_frame (callable): A callback function to record the state of arrays and pointers.\n \"\"\"\n merged_index = m + n\n\n # Record initial array\n record_frame(arrays=[nums1[:], nums2[:]])\n\n while m > 0 and n > 0:\n # Record pointers\n record_frame(pointers=[{\"i\": m - 1}, {\"j\": n - 1}])\n if nums1[m - 1] > nums2[n - 1]:\n nums1[merged_index - 1] = nums1[m - 1]\n m -= 1\n else:\n nums1[merged_index - 1] = nums2[n - 1]\n n -= 1\n merged_index -= 1\n\n # Record new array\n record_frame(arrays=[nums1[:], nums2[:]])\n\n # Record final pointers\n record_frame(pointers=[{\"i\": n - 1}, {\"j\": n - 1}])\n\n nums1[:n] = nums2[:n]\n\n # Record final array\n record_frame(arrays=[nums1[:], nums2[:]])\n\n\n# Initial arrays for the merge function\nnums1 = [4, 5, 7, 0, 0, 0, 0, 0, 0]\nnums2 = [1, 2, 2, 3, 5, 6]\nm = 3 # Number of initial valid elements in nums1\nn = 6 # Number of elements in nums2\n\n\nclass MergeScene(ArrayBlockVisualizer):\n \"\"\"\n A Manim scene to visualize the merge function using the FunctionAnimation class.\n \"\"\"\n\n def __init__(self, **kwargs):\n \"\"\"\n Initializes the MergeScene with the merge function and its arguments.\n\n Args:\n **kwargs: Additional keyword arguments for the ArrayBlockVisualizer superclass.\n \"\"\"\n super().__init__(merge, (nums1, m, nums2, n), **kwargs)\n\n\n# To render this scene, use the following command in your terminal:\n# manim -pql examples/merge_sorted_arrays.py MergeScene\n```\n\n### Running the Example\n\nTo render the `MergeScene`, run the following command in your terminal:\n\n```bash\nmanim -pql example.py MergeScene\n```\n\n- `-pql` stands for play in low quality. You can adjust this based on the desired rendering quality.\n\n## License\n\nThis project is licensed under the MIT License - see the LICENSE file for details.\n\n## Contributing\n\nContributions are welcome! Please open an issue or submit a pull request for any improvements or bug fixes.\n\n## Acknowledgements\n\n[Manim Community](https://github.com/ManimCommunity/manim) for developing the Manim library.\n",
"bugtrack_url": null,
"license": null,
"summary": "A Python package for visualizing array-based algorithms using Manim",
"version": "0.0.1",
"project_urls": {
"Documentation": "https://github.com/aanas-sayed/arrayviz",
"Homepage": "https://github.com/aanas-sayed/arrayviz",
"Issues": "https://github.com/aanas-sayed/arrayviz/issues",
"Repository": "https://github.com/aanas-sayed/arrayviz.git"
},
"split_keywords": [
"array",
" visualization",
" manim",
" animation"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "3f7e65933b967c7853845031f5389b2fcd51cab0f6f6a28c8bce46addcddb3ed",
"md5": "18abe456556b3e61a20cb6f874e9b5ef",
"sha256": "b627d4f0ecb3840d40546b37fecced406f5ecf5627c3ee092d5487371fdb2cfb"
},
"downloads": -1,
"filename": "arrayviz-0.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "18abe456556b3e61a20cb6f874e9b5ef",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 6620,
"upload_time": "2024-08-01T18:50:02",
"upload_time_iso_8601": "2024-08-01T18:50:02.684870Z",
"url": "https://files.pythonhosted.org/packages/3f/7e/65933b967c7853845031f5389b2fcd51cab0f6f6a28c8bce46addcddb3ed/arrayviz-0.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "5c86c07e5ccc4692285e24395c8ac66e1822b5d4e04e471f07c869c557689ae7",
"md5": "82553aeb2fe2beb00739e69ad1004f17",
"sha256": "f36e8424916cf099e5fd0208068e86988e87eb87c1e7d48e965880950851f12c"
},
"downloads": -1,
"filename": "arrayviz-0.0.1.tar.gz",
"has_sig": false,
"md5_digest": "82553aeb2fe2beb00739e69ad1004f17",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 6513,
"upload_time": "2024-08-01T18:50:04",
"upload_time_iso_8601": "2024-08-01T18:50:04.259941Z",
"url": "https://files.pythonhosted.org/packages/5c/86/c07e5ccc4692285e24395c8ac66e1822b5d4e04e471f07c869c557689ae7/arrayviz-0.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-01 18:50:04",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "aanas-sayed",
"github_project": "arrayviz",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "arrayviz"
}