# asciichart
[](https://npmjs.com/package/asciichart) [](https://pypi.python.org/pypi/asciichartpy) [](https://travis-ci.org/kroitor/asciichart) [](https://coveralls.io/github/kroitor/asciichart?branch=master) [](https://github.com/kroitor/asciichart/blob/master/LICENSE.txt)
Console ASCII line charts in pure Javascript (for NodeJS and browsers) with no dependencies. This code is absolutely free for any usage, you just do whatever the fuck you want.
<img width="789" alt="Console ASCII Line charts in pure Javascript (for NodeJS and browsers)" src="https://cloud.githubusercontent.com/assets/1294454/22818709/9f14e1c2-ef7f-11e6-978f-34b5b595fb63.png">
## Usage
### NodeJS
```sh
npm install asciichart
```
```javascript
var asciichart = require ('asciichart')
var s0 = new Array (120)
for (var i = 0; i < s0.length; i++)
s0[i] = 15 * Math.sin (i * ((Math.PI * 4) / s0.length))
console.log (asciichart.plot (s0))
```
### Browsers
```html
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<meta charset="UTF-8">
<title>asciichart</title>
<script src="asciichart.js"></script>
<script type="text/javascript">
var s0 = new Array (120)
for (var i = 0; i < s0.length; i++)
s0[i] = 15 * Math.sin (i * ((Math.PI * 4) / s0.length))
console.log (asciichart.plot (s0))
</script>
</head>
<body>
</body>
</html>
```
### Options
The width of the chart will always equal the length of data series. The height and range are determined automatically.
```javascript
var s0 = new Array (120)
for (var i = 0; i < s0.length; i++)
s0[i] = 15 * Math.sin (i * ((Math.PI * 4) / s0.length))
console.log (asciichart.plot (s0))
```
<img width="788" alt="Console ASCII Line charts in pure Javascript (for NodeJS and browsers)" src="https://cloud.githubusercontent.com/assets/1294454/22818807/313cd636-ef80-11e6-9d1a-7a90abdb38c8.png">
The output can be configured by passing a second parameter to the `plot (series, config)` function. The following options are supported:
```javascript
var config = {
offset: 3, // axis offset from the left (min 2)
padding: ' ', // padding string for label formatting (can be overrided)
height: 10, // any height you want
// the label format function applies default padding
format: function (x, i) { return (padding + x.toFixed (2)).slice (-padding.length) }
}
```
### Scale To Desired Height
<img width="791" alt="Console ASCII Line charts in pure Javascript (for NodeJS and browsers)" src="https://cloud.githubusercontent.com/assets/1294454/22818711/9f166128-ef7f-11e6-9748-b23b151974ed.png">
```javascript
var s = []
for (var i = 0; i < 120; i++)
s[i] = 15 * Math.cos (i * ((Math.PI * 8) / 120)) // values range from -15 to +15
console.log (asciichart.plot (s, { height: 6 })) // this rescales the graph to ±3 lines
```
<img width="787" alt="Console ASCII Line charts in pure Javascript (for NodeJS and browsers)" src="https://cloud.githubusercontent.com/assets/1294454/22825525/dd295294-ef9e-11e6-93d1-0beb80b93133.png">
### Auto-range
```javascript
var s2 = new Array (120)
s2[0] = Math.round (Math.random () * 15)
for (i = 1; i < s2.length; i++)
s2[i] = s2[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))
console.log (asciichart.plot (s2))
```
<img width="788" alt="Console ASCII Line charts in pure Javascript (for NodeJS and browsers)" src="https://cloud.githubusercontent.com/assets/1294454/22818710/9f157a74-ef7f-11e6-893a-f7494b5abef1.png">
### Multiple Series
```javascript
var s2 = new Array (120)
s2[0] = Math.round (Math.random () * 15)
for (i = 1; i < s2.length; i++)
s2[i] = s2[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))
var s3 = new Array (120)
s3[0] = Math.round (Math.random () * 15)
for (i = 1; i < s3.length; i++)
s3[i] = s3[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))
console.log (asciichart.plot ([ s2, s3 ]))
```
<img width="788" alt="Console ASCII Line charts in pure Javascript (for NodeJS and browsers)" src="https://user-images.githubusercontent.com/27967284/79398277-5322da80-7f91-11ea-8da8-e47976b76c12.png">
### Colors
```javascript
var arr1 = new Array (120)
arr1[0] = Math.round (Math.random () * 15)
for (i = 1; i < arr1.length; i++)
arr1[i] = arr1[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))
var arr2 = new Array (120)
arr2[0] = Math.round (Math.random () * 15)
for (i = 1; i < arr2.length; i++)
arr2[i] = arr2[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))
var arr3 = new Array (120)
arr3[0] = Math.round (Math.random () * 15)
for (i = 1; i < arr3.length; i++)
arr3[i] = arr3[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))
var arr4 = new Array (120)
arr4[0] = Math.round (Math.random () * 15)
for (i = 1; i < arr4.length; i++)
arr4[i] = arr4[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))
var config = {
colors: [
asciichart.blue,
asciichart.green,
asciichart.default, // default color
undefined, // equivalent to default
]
}
console.log (asciichart.plot([ arr1, arr2, arr3, arr4 ], config))
```
<img width="788" alt="Console ASCII Line charts in pure Javascript (for NodeJS and browsers)" src="https://user-images.githubusercontent.com/27967284/79398700-51a5e200-7f92-11ea-9048-8dbdeeb60830.png">
### See Also
A util by [madnight](https://github.com/madnight) for drawing Bitcoin/Ether/altcoin charts in command-line console: [bitcoin-chart-cli](https://github.com/madnight/bitcoin-chart-cli).

### Ports
Special thx to all who helped port it to other languages, great stuff!
- [Python port](https://pypi.org/project/asciichartpy) included!
- Java: [ASCIIGraph](https://github.com/MitchTalmadge/ASCIIGraph), ported by [MitchTalmadge](https://github.com/MitchTalmadge). If you're a Java-person, check it out!
- Go: [asciigraph](https://github.com/guptarohit/asciigraph), ported by [guptarohit](https://github.com/guptarohit), Go people! )
- Haskell: [asciichart](https://github.com/madnight/asciichart), ported by [madnight](https://github.com/madnight) to Haskell world!
- Ruby: [ascii_chart](https://github.com/zhustec/ascii_chart), ported by [zhustec](https://github.com/zhustec)!
- Elixir: [asciichart](https://github.com/sndnv/asciichart), ported by [sndv](https://github.com/sndnv)!
- Perl: [App::AsciiChart](https://github.com/vti/app-asciichart), ported by [vti](https://github.com/vti)!
- C: [plot](https://github.com/annacrombie/plot), ported by [annacrombie](https://github.com/annacrombie) with a ruby extension!
- R: [asciichartr](https://github.com/blmayer/asciichartr), ported by [blmayer](https://github.com/blmayer)!
- Rust: [rasciigraph](https://github.com/orhanbalci/rasciigraph), ported by [orhanbalci](https://github.com/orhanbalci)!
- PHP: [PHP-colored-ascii-linechart](https://github.com/noximo/PHP-colored-ascii-linechart), ported by [noximo](https://github.com/noximo)!
- C#: [asciichart-sharp](https://github.com/samcarton/asciichart-sharp), ported by [samcarton](https://github.com/samcarton)!
### Future work (coming soon, hopefully)
- levels and points on the graph!
- even better value formatting and auto-scaling!
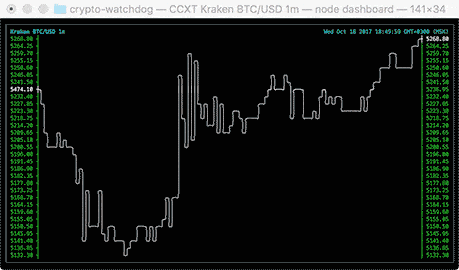
Raw data
{
"_id": null,
"home_page": "https://github.com/kroitor/asciichart",
"name": "asciichartpy",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "asciichart,ascii,chart,charting,charts,console,terminal,draw,box-drawing,box drawing,drawing,stats,line,linear,plot,graph,ascii graphics,graphics,command line,commandline,bash,shell,sh,light",
"author": "Igor Kroitor",
"author_email": "igor.kroitor@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/41/3a/b01436be647f881515ec2f253616bf4a40c1d27d02a69e7f038e27fcdf81/asciichartpy-1.5.25.tar.gz",
"platform": "",
"description": "# asciichart\n\n[](https://npmjs.com/package/asciichart) [](https://pypi.python.org/pypi/asciichartpy) [](https://travis-ci.org/kroitor/asciichart) [](https://coveralls.io/github/kroitor/asciichart?branch=master) [](https://github.com/kroitor/asciichart/blob/master/LICENSE.txt)\n\nConsole ASCII line charts in pure Javascript (for NodeJS and browsers) with no dependencies. This code is absolutely free for any usage, you just do whatever the fuck you want.\n\n<img width=\"789\" alt=\"Console ASCII Line charts in pure Javascript (for NodeJS and browsers)\" src=\"https://cloud.githubusercontent.com/assets/1294454/22818709/9f14e1c2-ef7f-11e6-978f-34b5b595fb63.png\">\n\n## Usage\n\n### NodeJS\n\n```sh\nnpm install asciichart\n```\n\n```javascript\nvar asciichart = require ('asciichart')\nvar s0 = new Array (120)\nfor (var i = 0; i < s0.length; i++)\n s0[i] = 15 * Math.sin (i * ((Math.PI * 4) / s0.length))\nconsole.log (asciichart.plot (s0))\n```\n\n### Browsers\n\n```html\n<!DOCTYPE html>\n<html>\n <head>\n <meta http-equiv=\"Content-Type\" content=\"text/html; charset=utf-8\">\n <meta charset=\"UTF-8\">\n <title>asciichart</title>\n <script src=\"asciichart.js\"></script>\n <script type=\"text/javascript\">\n var s0 = new Array (120)\n for (var i = 0; i < s0.length; i++)\n s0[i] = 15 * Math.sin (i * ((Math.PI * 4) / s0.length))\n console.log (asciichart.plot (s0))\n </script>\n </head>\n <body>\n </body>\n</html>\n```\n\n### Options\n\nThe width of the chart will always equal the length of data series. The height and range are determined automatically.\n\n```javascript\nvar s0 = new Array (120)\nfor (var i = 0; i < s0.length; i++)\n s0[i] = 15 * Math.sin (i * ((Math.PI * 4) / s0.length))\nconsole.log (asciichart.plot (s0))\n```\n\n<img width=\"788\" alt=\"Console ASCII Line charts in pure Javascript (for NodeJS and browsers)\" src=\"https://cloud.githubusercontent.com/assets/1294454/22818807/313cd636-ef80-11e6-9d1a-7a90abdb38c8.png\">\n\nThe output can be configured by passing a second parameter to the `plot (series, config)` function. The following options are supported:\n```javascript\nvar config = {\n\n offset: 3, // axis offset from the left (min 2)\n padding: ' ', // padding string for label formatting (can be overrided)\n height: 10, // any height you want\n\n // the label format function applies default padding\n format: function (x, i) { return (padding + x.toFixed (2)).slice (-padding.length) }\n}\n```\n\n### Scale To Desired Height\n\n<img width=\"791\" alt=\"Console ASCII Line charts in pure Javascript (for NodeJS and browsers)\" src=\"https://cloud.githubusercontent.com/assets/1294454/22818711/9f166128-ef7f-11e6-9748-b23b151974ed.png\">\n\n```javascript\nvar s = []\nfor (var i = 0; i < 120; i++)\n s[i] = 15 * Math.cos (i * ((Math.PI * 8) / 120)) // values range from -15 to +15\nconsole.log (asciichart.plot (s, { height: 6 })) // this rescales the graph to \u00b13 lines\n```\n\n<img width=\"787\" alt=\"Console ASCII Line charts in pure Javascript (for NodeJS and browsers)\" src=\"https://cloud.githubusercontent.com/assets/1294454/22825525/dd295294-ef9e-11e6-93d1-0beb80b93133.png\">\n\n### Auto-range\n\n```javascript\nvar s2 = new Array (120)\ns2[0] = Math.round (Math.random () * 15)\nfor (i = 1; i < s2.length; i++)\n s2[i] = s2[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))\nconsole.log (asciichart.plot (s2))\n```\n\n<img width=\"788\" alt=\"Console ASCII Line charts in pure Javascript (for NodeJS and browsers)\" src=\"https://cloud.githubusercontent.com/assets/1294454/22818710/9f157a74-ef7f-11e6-893a-f7494b5abef1.png\">\n\n### Multiple Series\n\n```javascript\nvar s2 = new Array (120)\ns2[0] = Math.round (Math.random () * 15)\nfor (i = 1; i < s2.length; i++)\n s2[i] = s2[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))\n\nvar s3 = new Array (120)\ns3[0] = Math.round (Math.random () * 15)\nfor (i = 1; i < s3.length; i++)\n s3[i] = s3[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))\n\nconsole.log (asciichart.plot ([ s2, s3 ]))\n```\n\n<img width=\"788\" alt=\"Console ASCII Line charts in pure Javascript (for NodeJS and browsers)\" src=\"https://user-images.githubusercontent.com/27967284/79398277-5322da80-7f91-11ea-8da8-e47976b76c12.png\">\n\n### Colors\n\n```javascript\nvar arr1 = new Array (120)\narr1[0] = Math.round (Math.random () * 15)\nfor (i = 1; i < arr1.length; i++)\n arr1[i] = arr1[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))\n\nvar arr2 = new Array (120)\narr2[0] = Math.round (Math.random () * 15)\nfor (i = 1; i < arr2.length; i++)\n arr2[i] = arr2[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))\n\nvar arr3 = new Array (120)\narr3[0] = Math.round (Math.random () * 15)\nfor (i = 1; i < arr3.length; i++)\n arr3[i] = arr3[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))\n\nvar arr4 = new Array (120)\narr4[0] = Math.round (Math.random () * 15)\nfor (i = 1; i < arr4.length; i++)\n arr4[i] = arr4[i - 1] + Math.round (Math.random () * (Math.random () > 0.5 ? 2 : -2))\n\nvar config = {\n colors: [\n asciichart.blue,\n asciichart.green,\n asciichart.default, // default color\n undefined, // equivalent to default\n ]\n}\n\nconsole.log (asciichart.plot([ arr1, arr2, arr3, arr4 ], config))\n```\n\n<img width=\"788\" alt=\"Console ASCII Line charts in pure Javascript (for NodeJS and browsers)\" src=\"https://user-images.githubusercontent.com/27967284/79398700-51a5e200-7f92-11ea-9048-8dbdeeb60830.png\">\n\n### See Also\n\nA util by [madnight](https://github.com/madnight) for drawing Bitcoin/Ether/altcoin charts in command-line console: [bitcoin-chart-cli](https://github.com/madnight/bitcoin-chart-cli).\n\n\n\n### Ports\n\nSpecial thx to all who helped port it to other languages, great stuff!\n\n- [Python port](https://pypi.org/project/asciichartpy) included!\n- Java: [ASCIIGraph](https://github.com/MitchTalmadge/ASCIIGraph),\u00a0ported by [MitchTalmadge](https://github.com/MitchTalmadge). If you're a Java-person, check it out!\n- Go: [asciigraph](https://github.com/guptarohit/asciigraph), ported by [guptarohit](https://github.com/guptarohit), Go people! )\n- Haskell: [asciichart](https://github.com/madnight/asciichart), ported by [madnight](https://github.com/madnight) to Haskell world!\n- Ruby: [ascii_chart](https://github.com/zhustec/ascii_chart), ported by [zhustec](https://github.com/zhustec)!\n- Elixir: [asciichart](https://github.com/sndnv/asciichart), ported by [sndv](https://github.com/sndnv)!\n- Perl: [App::AsciiChart](https://github.com/vti/app-asciichart), ported by [vti](https://github.com/vti)!\n- C: [plot](https://github.com/annacrombie/plot), ported by [annacrombie](https://github.com/annacrombie) with a ruby extension!\n- R: [asciichartr](https://github.com/blmayer/asciichartr), ported by [blmayer](https://github.com/blmayer)!\n- Rust: [rasciigraph](https://github.com/orhanbalci/rasciigraph), ported by [orhanbalci](https://github.com/orhanbalci)!\n- PHP: [PHP-colored-ascii-linechart](https://github.com/noximo/PHP-colored-ascii-linechart), ported by [noximo](https://github.com/noximo)!\n- C#: [asciichart-sharp](https://github.com/samcarton/asciichart-sharp), ported by [samcarton](https://github.com/samcarton)!\n\n### Future work (coming soon, hopefully)\n\n- levels and points on the graph!\n- even better value formatting and auto-scaling!\n\n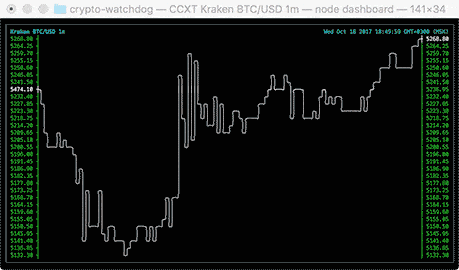\n\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Nice-looking lightweight console ASCII line charts \u256d\u2508\u256f with no dependencies",
"version": "1.5.25",
"project_urls": {
"Homepage": "https://github.com/kroitor/asciichart"
},
"split_keywords": [
"asciichart",
"ascii",
"chart",
"charting",
"charts",
"console",
"terminal",
"draw",
"box-drawing",
"box drawing",
"drawing",
"stats",
"line",
"linear",
"plot",
"graph",
"ascii graphics",
"graphics",
"command line",
"commandline",
"bash",
"shell",
"sh",
"light"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "3fd07b958df957e4827837b590944008f0b28078f552b451f7407b4b3d54f574",
"md5": "3ae24c160eebb23c66f45f5fab8e07a0",
"sha256": "33c417a3c8ef7d0a11b98eb9ea6dd9b2c1b17559e539b207a17d26d4302d0258"
},
"downloads": -1,
"filename": "asciichartpy-1.5.25-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "3ae24c160eebb23c66f45f5fab8e07a0",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": null,
"size": 7228,
"upload_time": "2020-08-17T02:07:16",
"upload_time_iso_8601": "2020-08-17T02:07:16.386957Z",
"url": "https://files.pythonhosted.org/packages/3f/d0/7b958df957e4827837b590944008f0b28078f552b451f7407b4b3d54f574/asciichartpy-1.5.25-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "413ab01436be647f881515ec2f253616bf4a40c1d27d02a69e7f038e27fcdf81",
"md5": "826faf3f92f8c9c2c6ad99e68251a1db",
"sha256": "63a305302b2aad51da288b58226009b7b0313eba7d8e2452d5a21a13fcf44d74"
},
"downloads": -1,
"filename": "asciichartpy-1.5.25.tar.gz",
"has_sig": false,
"md5_digest": "826faf3f92f8c9c2c6ad99e68251a1db",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 8201,
"upload_time": "2020-08-17T02:07:18",
"upload_time_iso_8601": "2020-08-17T02:07:18.292486Z",
"url": "https://files.pythonhosted.org/packages/41/3a/b01436be647f881515ec2f253616bf4a40c1d27d02a69e7f038e27fcdf81/asciichartpy-1.5.25.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2020-08-17 02:07:18",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "kroitor",
"github_project": "asciichart",
"travis_ci": true,
"coveralls": false,
"github_actions": false,
"tox": true,
"lcname": "asciichartpy"
}