# AstraPy
A pythonic client for [DataStax Astra DB](https://astra.datastax.com).
_This README targets AstraPy version **1.0.0+**, which introduces a whole new API.
Click [here](https://github.com/datastax/astrapy/blob/cd3f5ce8146093e10a095709c0f5c3f8e3f2c7da/README.md) for the pre-existing API (fully compatible with newer versions)._
## Quickstart
Install with `pip install astrapy`.
Get the *API Endpoint* and the *Token* to your Astra DB instance at [astra.datastax.com](https://astra.datastax.com).
Try the following code after replacing the connection parameters:
```python
import astrapy
ASTRA_DB_APPLICATION_TOKEN = "AstraCS:..."
ASTRA_DB_API_ENDPOINT = "https://01234567-....apps.astra.datastax.com"
my_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)
my_database = my_client.get_database(ASTRA_DB_API_ENDPOINT)
my_collection = my_database.create_collection(
"dreams",
dimension=3,
metric=astrapy.constants.VectorMetric.COSINE,
)
my_collection.insert_one({"summary": "I was flying", "$vector": [-0.4, 0.7, 0]})
my_collection.insert_many(
[
{
"_id": astrapy.ids.UUID("018e65c9-e33d-749b-9386-e848739582f0"),
"summary": "A dinner on the Moon",
"$vector": [0.2, -0.3, -0.5],
},
{
"summary": "Riding the waves",
"tags": ["sport"],
"$vector": [0, 0.2, 1],
},
{
"summary": "Friendly aliens in town",
"tags": ["scifi"],
"$vector": [-0.3, 0, 0.8],
},
{
"summary": "Meeting Beethoven at the dentist",
"$vector": [0.2, 0.6, 0],
},
],
)
my_collection.update_one(
{"tags": "sport"},
{"$set": {"summary": "Surfers' paradise"}},
)
cursor = my_collection.find(
{},
sort={"$vector": [0, 0.2, 0.4]},
limit=2,
include_similarity=True,
)
for result in cursor:
print(f"{result['summary']}: {result['$similarity']}")
# This would print:
# Surfers' paradise: 0.98238194
# Friendly aliens in town: 0.91873914
```
Next steps:
- More info and usage patterns are given in the docstrings of classes and methods
- [Data API reference](https://docs.datastax.com/en/astra/astra-db-vector/api-reference/overview.html)
- [AstraPy reference](https://docs.datastax.com/en/astra/astra-db-vector/api-reference/dataapiclient.html)
- Package on [PyPI](https://pypi.org/project/astrapy/)
## AstraPy's API
### Abstraction diagram
AstraPy's abstractions for working at the data and admin layers are structured
as depicted by this diagram:
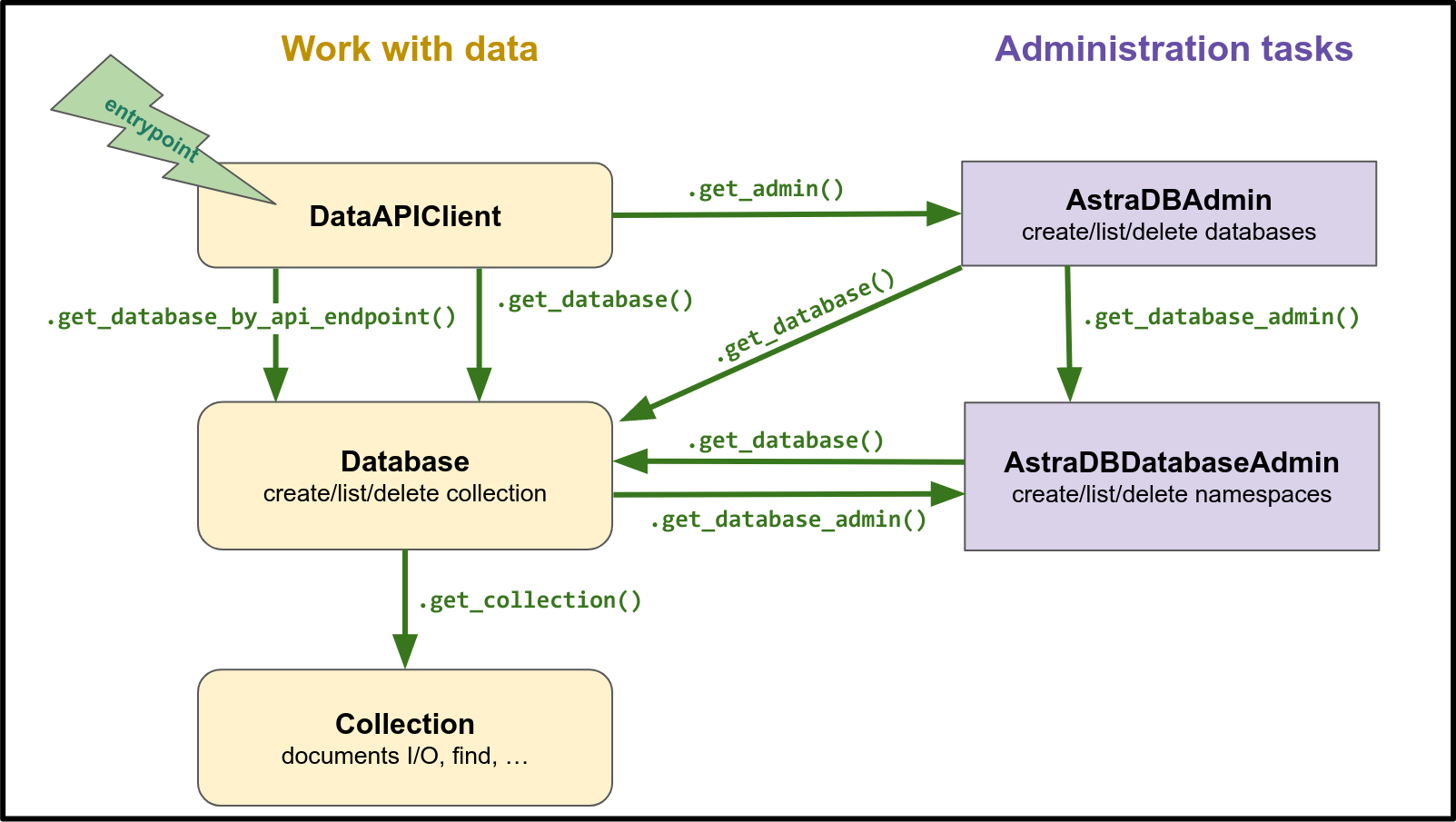
Here's a small admin-oriented example:
```python
import astrapy
ASTRA_DB_APPLICATION_TOKEN = "AstraCS:..."
my_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)
my_astra_admin = my_client.get_admin()
database_list = list(my_astra_admin.list_databases())
db_info = database_list[0].info
print(db_info.name, db_info.id, db_info.region)
my_database_admin = my_astra_admin.get_database_admin(db_info.id)
my_database_admin.list_namespaces()
my_database_admin.create_namespace("my_dreamspace")
```
### Exceptions
The package comes with its own set of exceptions, arranged in this hierarchy:
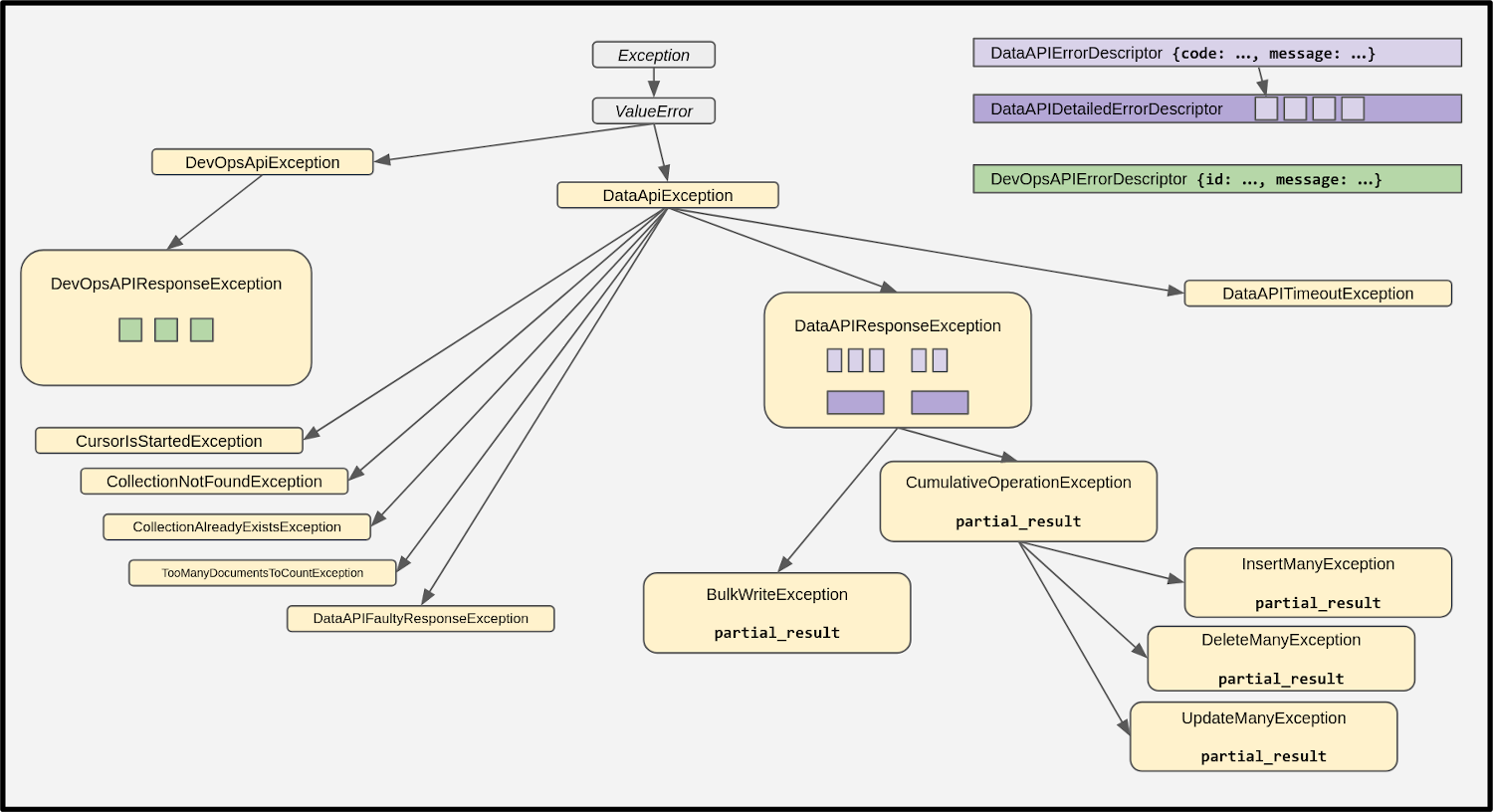
For more information, and code examples, check out the docstrings and consult
the API reference linked above.
### Working with dates
Date and datetime objects, i.e. instances of the standard library
`datetime.datetime` and `datetime.date` classes, can be used anywhere in documents:
```python
import datetime
import astrapy
ASTRA_DB_APPLICATION_TOKEN = "AstraCS:..."
ASTRA_DB_API_ENDPOINT = "https://01234567-....apps.astra.datastax.com"
my_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)
my_database = my_client.get_database(ASTRA_DB_API_ENDPOINT)
my_collection = my_database.dreams
my_collection.insert_one({"when": datetime.datetime.now()})
my_collection.insert_one({"date_of_birth": datetime.date(2000, 1, 1)})
my_collection.update_one(
{"registered_at": datetime.date(1999, 11, 14)},
{"$set": {"message": "happy Sunday!"}},
)
print(
my_collection.find_one(
{"date_of_birth": {"$lt": datetime.date(2001, 1, 1)}},
projection={"_id": False},
)
)
# This would print:
# {'date_of_birth': datetime.datetime(2000, 1, 1, 0, 0)}
```
_**Note**: reads from a collection will always_
_return the `datetime` class regardless of wheter a `date` or a `datetime` was provided_
_in the insertion._
### Working with ObjectIds and UUIDs
Astrapy repackages the ObjectId from `bson` and the UUID class and utilities
from the `uuid` package and its `uuidv6` extension. You can also use them directly.
Even when setting a default ID type for a collection, you still retain the freedom
to use any ID type for any document:
```python
import astrapy
import bson
ASTRA_DB_APPLICATION_TOKEN = "AstraCS:..."
ASTRA_DB_API_ENDPOINT = "https://01234567-....apps.astra.datastax.com"
my_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)
my_database = my_client.get_database(ASTRA_DB_API_ENDPOINT)
my_collection = my_database.create_collection(
"ecommerce",
default_id_type=astrapy.constants.DefaultIdType.UUIDV6,
)
my_collection.insert_one({"_id": astrapy.ids.ObjectId("65fd9b52d7fabba03349d013")})
my_collection.find({
"_id": astrapy.ids.UUID("018e65c9-e33d-749b-9386-e848739582f0"),
})
my_collection.update_one(
{"tag": "in_stock"},
{"$set": {"inventory_id": bson.objectid.ObjectId()}},
upsert=True,
)
my_collection.insert_one({"_id": astrapy.ids.uuid8()})
```
## For contributors
First install poetry with `pip install poetry` and then the project dependencies with `poetry install --with dev`.
Linter, style and typecheck should all pass for a PR:
```bash
poetry run black --check astrapy && poetry run ruff astrapy && poetry run mypy astrapy
poetry run black --check tests && poetry run ruff tests && poetry run mypy tests
```
Features must be thoroughly covered in tests (see `tests/idiomatic/*` for
naming convention and module structure).
### Running tests
"Full regular" testing requires environment variables:
```bash
export ASTRA_DB_APPLICATION_TOKEN="AstraCS:..."
export ASTRA_DB_API_ENDPOINT="https://.......apps.astra.datastax.com"
export ASTRA_DB_KEYSPACE="default_keyspace"
# Optional:
export ASTRA_DB_SECONDARY_KEYSPACE="..."
```
Tests can be started in various ways:
```bash
# test the "idiomatic" layer
poetry run pytest tests/idiomatic
poetry run pytest tests/idiomatic/unit
poetry run pytest tests/idiomatic/integration
# remove logging noise:
poetry run pytest [...] -o log_cli=0
```
The above runs the regular testing (i.e. non-Admin, non-core).
The (idiomatic) Admin part is tested manually by you, on Astra accounts with room
for up to 3 new databases, possibly both on prod and dev, and uses specific env vars,
as can be seen on `tests/idiomatic/integration/test_admin.py`.
Vectorize tests are confined in `tests/vectorize_idiomatic` and are run
separately. A separate set of credentials is required to do the full testing:
refer to `tests/.vectorize.env.template` for the complete listing, including
the secrets that should be added to the database beforehand, through the UI.
Should you be interested in testing the "core" modules, moreover,
this is also something for you to run manually (do that if you touch "core"):
```bash
# test the core modules
poetry run pytest tests/core
# do not drop collections:
TEST_SKIP_COLLECTION_DELETE=1 poetry run pytest [...]
# include astrapy.core.ops testing (tester must clean up after that):
TEST_ASTRADBOPS=1 poetry run pytest [...]
```
## Appendices
### Appendix A: quick reference for imports
Client, data and admin abstractions:
```python
from astrapy import (
DataAPIClient,
Database,
AsyncDatabase,
Collection,
AsyncCollection,
AstraDBAdmin,
AstraDBDatabaseAdmin,
DataAPIDatabaseAdmin,
)
```
Constants for data-related use:
```python
from astrapy.constants import (
ReturnDocument,
SortDocuments,
VectorMetric,
DefaultIdType,
Environment,
)
```
ObjectIds and UUIDs:
```python
from astrapy.ids import (
ObjectId,
uuid1,
uuid3,
uuid4,
uuid5,
uuid6,
uuid7,
uuid8,
UUID,
)
```
Operations (for `bulk_write` collection method):
```python
from astrapy.operations import (
BaseOperation,
InsertOne,
InsertMany,
UpdateOne,
UpdateMany,
ReplaceOne,
DeleteOne,
DeleteMany,
AsyncBaseOperation,
AsyncInsertOne,
AsyncInsertMany,
AsyncUpdateOne,
AsyncUpdateMany,
AsyncReplaceOne,
AsyncDeleteOne,
AsyncDeleteMany,
)
```
Result classes:
```python
from astrapy.results import (
OperationResult,
DeleteResult,
InsertOneResult,
InsertManyResult,
UpdateResult,
BulkWriteResult,
)
```
Exceptions:
```python
from astrapy.exceptions import (
DevOpsAPIException,
DevOpsAPIResponseException,
DevOpsAPIErrorDescriptor,
DataAPIErrorDescriptor,
DataAPIDetailedErrorDescriptor,
DataAPIException,
DataAPITimeoutException,
CursorIsStartedException,
CollectionNotFoundException,
CollectionAlreadyExistsException,
TooManyDocumentsToCountException,
DataAPIFaultyResponseException,
DataAPIResponseException,
CumulativeOperationException,
InsertManyException,
DeleteManyException,
UpdateManyException,
BulkWriteException,
)
```
Info/metadata classes:
```python
from astrapy.info import (
AdminDatabaseInfo,
DatabaseInfo,
CollectionInfo,
CollectionVectorServiceOptions,
CollectionDefaultIDOptions,
CollectionVectorOptions,
CollectionOptions,
CollectionDescriptor,
)
```
Admin-related classes and constants:
```python
from astrapy.admin import (
ParsedAPIEndpoint,
)
```
Cursors:
```python
from astrapy.cursors import (
BaseCursor,
Cursor,
AsyncCursor,
CommandCursor,
AsyncCommandCursor,
)
```
### Appendix B: compatibility with pre-1.0.0 library
If your code uses the pre-1.0.0 astrapy (i.e. `from astrapy.db import Database, Collection` and so on) you are strongly advised to migrate to the current API.
That being said, there are no known breakings of backward compatibility:
**legacy code would run with a newest astrapy version just as well.**
Here is a recap of the minor changes that came _to the old API_ with 1.0.0:
- Added support for null tokens (with the effect of no authentication/token header in requests)
- Added Content-Type header to all HTTP requests to the API
- Added methods to `[Async]AstraDBCollection`: `delete_one_filter`,
- Paginated find methods (sync/async) type change from Iterable to Generator
- Bugfix: handling of the mutable caller identity in copy and convert (sync/async) methods
- Default value of `sort` is `None` and not `{}` for `find` (sync/async)
- Introduction of `[Async]AstraDBCollection.chunked_delete_many` method
- Added `projection` parameter to `find_one_and[replace/update]` (sync/async)
- Bugfix: projection was silently ignored in `vector_find_one_and_[replace/update]` (sync/async)
- Added `options` to `update_many` (sync/async)
- `[Async]AstraDBDatabase.chunked_insert_many` does not intercept generic exceptions anymore, only `APIRequestError`
- Bugfix: `AsyncAstraDBCollection.async chunked_insert_many` stops at the first error when `ordered=True`
- Added payload info to `DataAPIException`
- Added `find_one_and_delete` method (sync/async)
- Added `skip_error_check` parameter to `delete_many` (sync/async)
- Timeout support throughout the library
- Added `sort` to `update_one`, `delete_one` and `delete_one_by_predicate` methods (sync/async)
- Full support for UUID v1,3,4,5,6,7,8 and ObjectID at the collection data I/O level
- `AstraDBOps.create_database` raises errors in case of failures
- `AstraDBOps.create_database`, return type corrected
- Fixed behaviour and return type of `AstraDBOps.create_keyspace` and `AstraDBOps.terminate_db`
- Added `AstraDBOps.delete_keyspace` method
- Method `create_collection` of `AstraDB` relaxes checks on passing `dimensions` for vector collections
- AstraDBOps core class acquired async methods: `async_get_databases`, `async_get_database`, `async_create_database`, `async_terminate_database`, `async_create_keyspace`, `async_delete_keyspace`
Keep in mind that the pre-1.0.0 library, now dubbed "core", is what the current 1.0.0 API ("idiomatic") builds on.
Raw data
{
"_id": null,
"home_page": "https://github.com/datastax/astrapy",
"name": "astrapy",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0.0,>=3.8.0",
"maintainer_email": null,
"keywords": "DataStax, Astra",
"author": "Stefano Lottini",
"author_email": "stefano.lottini@datastax.com",
"download_url": "https://files.pythonhosted.org/packages/8e/47/ca6714926ad3d6ce8863592dac95a31d7a057ea31c58940fad28afc98dd3/astrapy-1.3.0.tar.gz",
"platform": null,
"description": "# AstraPy\n\nA pythonic client for [DataStax Astra DB](https://astra.datastax.com).\n\n_This README targets AstraPy version **1.0.0+**, which introduces a whole new API.\nClick [here](https://github.com/datastax/astrapy/blob/cd3f5ce8146093e10a095709c0f5c3f8e3f2c7da/README.md) for the pre-existing API (fully compatible with newer versions)._\n\n\n## Quickstart\n\nInstall with `pip install astrapy`.\n\nGet the *API Endpoint* and the *Token* to your Astra DB instance at [astra.datastax.com](https://astra.datastax.com).\n\nTry the following code after replacing the connection parameters:\n\n```python\nimport astrapy\n\nASTRA_DB_APPLICATION_TOKEN = \"AstraCS:...\"\nASTRA_DB_API_ENDPOINT = \"https://01234567-....apps.astra.datastax.com\"\n\nmy_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)\nmy_database = my_client.get_database(ASTRA_DB_API_ENDPOINT)\n\nmy_collection = my_database.create_collection(\n \"dreams\",\n dimension=3,\n metric=astrapy.constants.VectorMetric.COSINE,\n)\n\nmy_collection.insert_one({\"summary\": \"I was flying\", \"$vector\": [-0.4, 0.7, 0]})\n\nmy_collection.insert_many(\n [\n {\n \"_id\": astrapy.ids.UUID(\"018e65c9-e33d-749b-9386-e848739582f0\"),\n \"summary\": \"A dinner on the Moon\",\n \"$vector\": [0.2, -0.3, -0.5],\n },\n {\n \"summary\": \"Riding the waves\",\n \"tags\": [\"sport\"],\n \"$vector\": [0, 0.2, 1],\n },\n {\n \"summary\": \"Friendly aliens in town\",\n \"tags\": [\"scifi\"],\n \"$vector\": [-0.3, 0, 0.8],\n },\n {\n \"summary\": \"Meeting Beethoven at the dentist\",\n \"$vector\": [0.2, 0.6, 0],\n },\n ],\n)\n\nmy_collection.update_one(\n {\"tags\": \"sport\"},\n {\"$set\": {\"summary\": \"Surfers' paradise\"}},\n)\n\ncursor = my_collection.find(\n {},\n sort={\"$vector\": [0, 0.2, 0.4]},\n limit=2,\n include_similarity=True,\n)\n\nfor result in cursor:\n print(f\"{result['summary']}: {result['$similarity']}\")\n\n# This would print:\n# Surfers' paradise: 0.98238194\n# Friendly aliens in town: 0.91873914\n```\n\nNext steps:\n\n- More info and usage patterns are given in the docstrings of classes and methods\n- [Data API reference](https://docs.datastax.com/en/astra/astra-db-vector/api-reference/overview.html)\n- [AstraPy reference](https://docs.datastax.com/en/astra/astra-db-vector/api-reference/dataapiclient.html)\n- Package on [PyPI](https://pypi.org/project/astrapy/)\n\n## AstraPy's API\n\n### Abstraction diagram\n\nAstraPy's abstractions for working at the data and admin layers are structured\nas depicted by this diagram:\n\n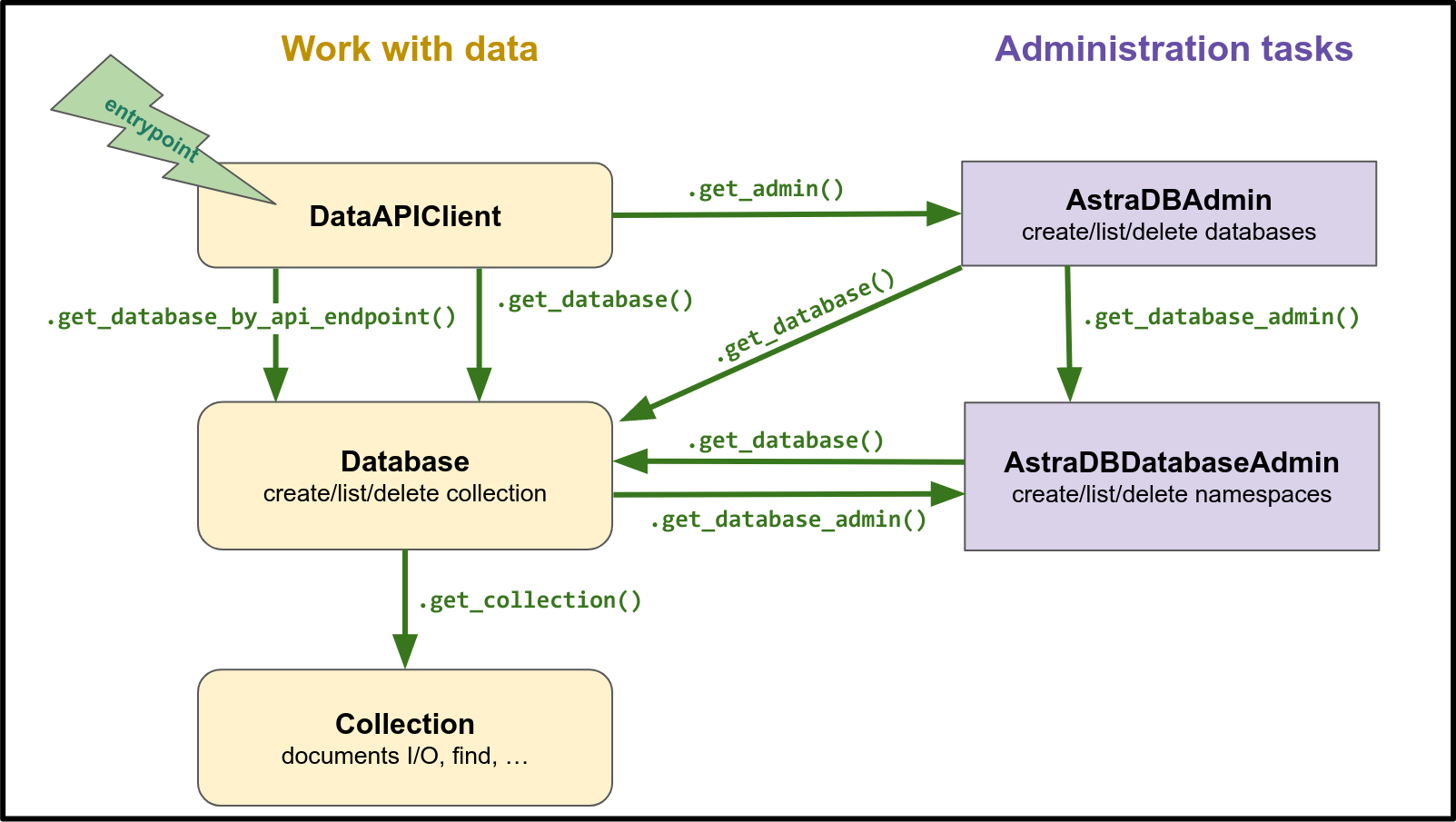\n\nHere's a small admin-oriented example:\n\n```python\nimport astrapy\n\n\nASTRA_DB_APPLICATION_TOKEN = \"AstraCS:...\"\n\nmy_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)\n\nmy_astra_admin = my_client.get_admin()\n\ndatabase_list = list(my_astra_admin.list_databases())\n\ndb_info = database_list[0].info\nprint(db_info.name, db_info.id, db_info.region)\n\nmy_database_admin = my_astra_admin.get_database_admin(db_info.id)\n\nmy_database_admin.list_namespaces()\nmy_database_admin.create_namespace(\"my_dreamspace\")\n```\n\n### Exceptions\n\nThe package comes with its own set of exceptions, arranged in this hierarchy:\n\n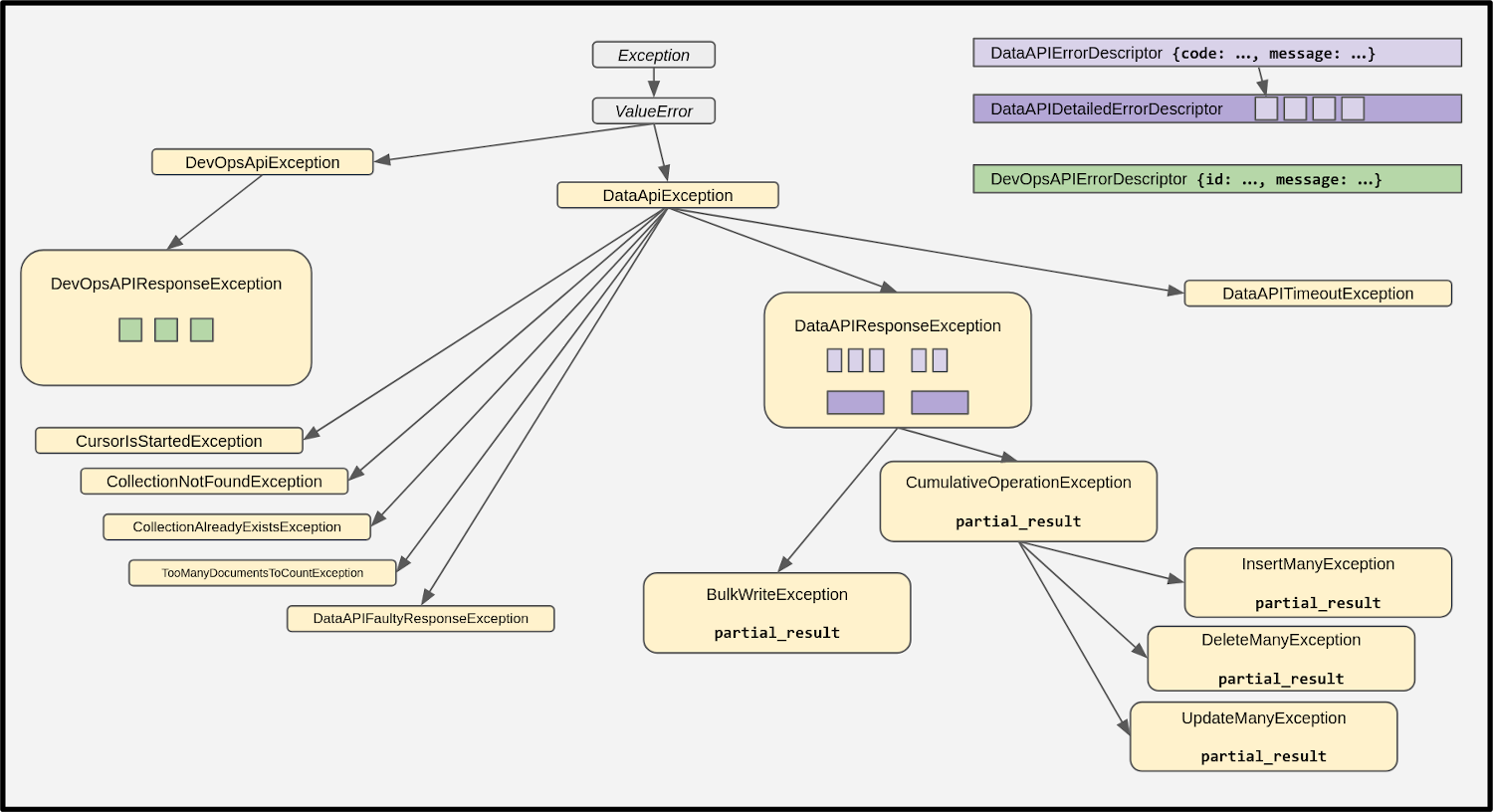\n\nFor more information, and code examples, check out the docstrings and consult\nthe API reference linked above.\n\n### Working with dates\n\nDate and datetime objects, i.e. instances of the standard library\n`datetime.datetime` and `datetime.date` classes, can be used anywhere in documents:\n\n```python\nimport datetime\nimport astrapy\n\n\nASTRA_DB_APPLICATION_TOKEN = \"AstraCS:...\"\nASTRA_DB_API_ENDPOINT = \"https://01234567-....apps.astra.datastax.com\"\n\nmy_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)\nmy_database = my_client.get_database(ASTRA_DB_API_ENDPOINT)\nmy_collection = my_database.dreams\n\nmy_collection.insert_one({\"when\": datetime.datetime.now()})\nmy_collection.insert_one({\"date_of_birth\": datetime.date(2000, 1, 1)})\n\nmy_collection.update_one(\n {\"registered_at\": datetime.date(1999, 11, 14)},\n {\"$set\": {\"message\": \"happy Sunday!\"}},\n)\n\nprint(\n my_collection.find_one(\n {\"date_of_birth\": {\"$lt\": datetime.date(2001, 1, 1)}},\n projection={\"_id\": False},\n )\n)\n# This would print:\n# {'date_of_birth': datetime.datetime(2000, 1, 1, 0, 0)}\n```\n\n_**Note**: reads from a collection will always_\n_return the `datetime` class regardless of wheter a `date` or a `datetime` was provided_\n_in the insertion._\n\n### Working with ObjectIds and UUIDs\n\nAstrapy repackages the ObjectId from `bson` and the UUID class and utilities\nfrom the `uuid` package and its `uuidv6` extension. You can also use them directly.\n\nEven when setting a default ID type for a collection, you still retain the freedom\nto use any ID type for any document:\n\n```python\nimport astrapy\nimport bson\n\nASTRA_DB_APPLICATION_TOKEN = \"AstraCS:...\"\nASTRA_DB_API_ENDPOINT = \"https://01234567-....apps.astra.datastax.com\"\n\nmy_client = astrapy.DataAPIClient(ASTRA_DB_APPLICATION_TOKEN)\nmy_database = my_client.get_database(ASTRA_DB_API_ENDPOINT)\n\nmy_collection = my_database.create_collection(\n \"ecommerce\",\n default_id_type=astrapy.constants.DefaultIdType.UUIDV6,\n)\n\nmy_collection.insert_one({\"_id\": astrapy.ids.ObjectId(\"65fd9b52d7fabba03349d013\")})\nmy_collection.find({\n \"_id\": astrapy.ids.UUID(\"018e65c9-e33d-749b-9386-e848739582f0\"),\n})\n\nmy_collection.update_one(\n {\"tag\": \"in_stock\"},\n {\"$set\": {\"inventory_id\": bson.objectid.ObjectId()}},\n upsert=True,\n)\n\nmy_collection.insert_one({\"_id\": astrapy.ids.uuid8()})\n```\n\n## For contributors\n\nFirst install poetry with `pip install poetry` and then the project dependencies with `poetry install --with dev`.\n\nLinter, style and typecheck should all pass for a PR:\n\n```bash\npoetry run black --check astrapy && poetry run ruff astrapy && poetry run mypy astrapy\n\npoetry run black --check tests && poetry run ruff tests && poetry run mypy tests\n```\n\nFeatures must be thoroughly covered in tests (see `tests/idiomatic/*` for\nnaming convention and module structure).\n\n### Running tests\n\n\"Full regular\" testing requires environment variables:\n\n```bash\nexport ASTRA_DB_APPLICATION_TOKEN=\"AstraCS:...\"\nexport ASTRA_DB_API_ENDPOINT=\"https://.......apps.astra.datastax.com\"\n\nexport ASTRA_DB_KEYSPACE=\"default_keyspace\"\n# Optional:\nexport ASTRA_DB_SECONDARY_KEYSPACE=\"...\"\n```\n\nTests can be started in various ways:\n\n```bash\n# test the \"idiomatic\" layer\npoetry run pytest tests/idiomatic\npoetry run pytest tests/idiomatic/unit\npoetry run pytest tests/idiomatic/integration\n\n# remove logging noise:\npoetry run pytest [...] -o log_cli=0\n```\n\nThe above runs the regular testing (i.e. non-Admin, non-core).\nThe (idiomatic) Admin part is tested manually by you, on Astra accounts with room\nfor up to 3 new databases, possibly both on prod and dev, and uses specific env vars,\nas can be seen on `tests/idiomatic/integration/test_admin.py`.\n\nVectorize tests are confined in `tests/vectorize_idiomatic` and are run\nseparately. A separate set of credentials is required to do the full testing:\nrefer to `tests/.vectorize.env.template` for the complete listing, including\nthe secrets that should be added to the database beforehand, through the UI.\n\nShould you be interested in testing the \"core\" modules, moreover,\nthis is also something for you to run manually (do that if you touch \"core\"):\n\n```bash\n# test the core modules\npoetry run pytest tests/core\n\n# do not drop collections:\nTEST_SKIP_COLLECTION_DELETE=1 poetry run pytest [...]\n\n# include astrapy.core.ops testing (tester must clean up after that):\nTEST_ASTRADBOPS=1 poetry run pytest [...]\n```\n\n## Appendices\n\n### Appendix A: quick reference for imports\n\nClient, data and admin abstractions:\n\n```python\nfrom astrapy import (\n DataAPIClient,\n Database,\n AsyncDatabase,\n Collection,\n AsyncCollection,\n AstraDBAdmin,\n AstraDBDatabaseAdmin,\n DataAPIDatabaseAdmin,\n)\n```\n\nConstants for data-related use:\n\n```python\nfrom astrapy.constants import (\n ReturnDocument,\n SortDocuments,\n VectorMetric,\n DefaultIdType,\n Environment,\n)\n```\n\nObjectIds and UUIDs:\n\n```python\nfrom astrapy.ids import (\n ObjectId,\n uuid1,\n uuid3,\n uuid4,\n uuid5,\n uuid6,\n uuid7,\n uuid8,\n UUID,\n)\n```\n\nOperations (for `bulk_write` collection method):\n\n```python\nfrom astrapy.operations import (\n BaseOperation,\n InsertOne,\n InsertMany,\n UpdateOne,\n UpdateMany,\n ReplaceOne,\n DeleteOne,\n DeleteMany,\n AsyncBaseOperation,\n AsyncInsertOne,\n AsyncInsertMany,\n AsyncUpdateOne,\n AsyncUpdateMany,\n AsyncReplaceOne,\n AsyncDeleteOne,\n AsyncDeleteMany,\n)\n```\n\nResult classes:\n\n```python\nfrom astrapy.results import (\n OperationResult,\n DeleteResult,\n InsertOneResult,\n InsertManyResult,\n UpdateResult,\n BulkWriteResult,\n)\n```\n\nExceptions:\n\n```python\nfrom astrapy.exceptions import (\n DevOpsAPIException,\n DevOpsAPIResponseException,\n DevOpsAPIErrorDescriptor,\n DataAPIErrorDescriptor,\n DataAPIDetailedErrorDescriptor,\n DataAPIException,\n DataAPITimeoutException,\n CursorIsStartedException,\n CollectionNotFoundException,\n CollectionAlreadyExistsException,\n TooManyDocumentsToCountException,\n DataAPIFaultyResponseException,\n DataAPIResponseException,\n CumulativeOperationException,\n InsertManyException,\n DeleteManyException,\n UpdateManyException,\n BulkWriteException,\n)\n```\n\nInfo/metadata classes:\n\n```python\nfrom astrapy.info import (\n AdminDatabaseInfo,\n DatabaseInfo,\n CollectionInfo,\n CollectionVectorServiceOptions,\n CollectionDefaultIDOptions,\n CollectionVectorOptions,\n CollectionOptions,\n CollectionDescriptor,\n)\n```\n\nAdmin-related classes and constants:\n\n```python\nfrom astrapy.admin import (\n ParsedAPIEndpoint,\n)\n```\n\nCursors:\n\n```python\nfrom astrapy.cursors import (\n BaseCursor,\n Cursor,\n AsyncCursor,\n CommandCursor,\n AsyncCommandCursor,\n)\n```\n\n### Appendix B: compatibility with pre-1.0.0 library\n\nIf your code uses the pre-1.0.0 astrapy (i.e. `from astrapy.db import Database, Collection` and so on) you are strongly advised to migrate to the current API.\n\nThat being said, there are no known breakings of backward compatibility:\n**legacy code would run with a newest astrapy version just as well.**\nHere is a recap of the minor changes that came _to the old API_ with 1.0.0:\n\n- Added support for null tokens (with the effect of no authentication/token header in requests)\n- Added Content-Type header to all HTTP requests to the API\n- Added methods to `[Async]AstraDBCollection`: `delete_one_filter`, \n- Paginated find methods (sync/async) type change from Iterable to Generator\n- Bugfix: handling of the mutable caller identity in copy and convert (sync/async) methods\n- Default value of `sort` is `None` and not `{}` for `find` (sync/async)\n- Introduction of `[Async]AstraDBCollection.chunked_delete_many` method\n- Added `projection` parameter to `find_one_and[replace/update]` (sync/async)\n- Bugfix: projection was silently ignored in `vector_find_one_and_[replace/update]` (sync/async)\n- Added `options` to `update_many` (sync/async)\n- `[Async]AstraDBDatabase.chunked_insert_many` does not intercept generic exceptions anymore, only `APIRequestError`\n- Bugfix: `AsyncAstraDBCollection.async chunked_insert_many` stops at the first error when `ordered=True`\n- Added payload info to `DataAPIException`\n- Added `find_one_and_delete` method (sync/async)\n- Added `skip_error_check` parameter to `delete_many` (sync/async)\n- Timeout support throughout the library\n- Added `sort` to `update_one`, `delete_one` and `delete_one_by_predicate` methods (sync/async)\n- Full support for UUID v1,3,4,5,6,7,8 and ObjectID at the collection data I/O level\n- `AstraDBOps.create_database` raises errors in case of failures\n- `AstraDBOps.create_database`, return type corrected\n- Fixed behaviour and return type of `AstraDBOps.create_keyspace` and `AstraDBOps.terminate_db`\n- Added `AstraDBOps.delete_keyspace` method\n- Method `create_collection` of `AstraDB` relaxes checks on passing `dimensions` for vector collections\n- AstraDBOps core class acquired async methods: `async_get_databases`, `async_get_database`, `async_create_database`, `async_terminate_database`, `async_create_keyspace`, `async_delete_keyspace`\n\nKeep in mind that the pre-1.0.0 library, now dubbed \"core\", is what the current 1.0.0 API (\"idiomatic\") builds on.\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "AstraPy is a Pythonic SDK for DataStax Astra and its Data API",
"version": "1.3.0",
"project_urls": {
"Homepage": "https://github.com/datastax/astrapy",
"Repository": "https://github.com/datastax/astrapy"
},
"split_keywords": [
"datastax",
" astra"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "bed3abc4d7a44c58d603db65b728eab134ab55cb886032c8bd75d1203108a9a0",
"md5": "a0d3afc0ca2b48af1d1aff2379d25a66",
"sha256": "349589b1616e6d7765cbd5c0062f9b8cebcee1a8315057531307ff066994a70d"
},
"downloads": -1,
"filename": "astrapy-1.3.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "a0d3afc0ca2b48af1d1aff2379d25a66",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0.0,>=3.8.0",
"size": 145402,
"upload_time": "2024-06-22T07:10:03",
"upload_time_iso_8601": "2024-06-22T07:10:03.144242Z",
"url": "https://files.pythonhosted.org/packages/be/d3/abc4d7a44c58d603db65b728eab134ab55cb886032c8bd75d1203108a9a0/astrapy-1.3.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8e47ca6714926ad3d6ce8863592dac95a31d7a057ea31c58940fad28afc98dd3",
"md5": "e0751ae4c5792c828ee9868afa5a679d",
"sha256": "7d6fbccb93cf69433182d3e258b12f9e3c6f91b4b109aaa91df161f4434ea1d2"
},
"downloads": -1,
"filename": "astrapy-1.3.0.tar.gz",
"has_sig": false,
"md5_digest": "e0751ae4c5792c828ee9868afa5a679d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0.0,>=3.8.0",
"size": 131656,
"upload_time": "2024-06-22T07:10:06",
"upload_time_iso_8601": "2024-06-22T07:10:06.524154Z",
"url": "https://files.pythonhosted.org/packages/8e/47/ca6714926ad3d6ce8863592dac95a31d7a057ea31c58940fad28afc98dd3/astrapy-1.3.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-22 07:10:06",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "datastax",
"github_project": "astrapy",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "astrapy"
}