# auto_profiler
A realtime timer for profiling a Python function or snippet.
## Features
- Filtering external libraries profiling.
- Filtering very short time functions-> threshold
- Allow depth: you can easily find the time consuming function
- Allow loop or multiple function call
- Allow recursive function call
- Disable it globaly by Profiler.GlobalDisable=True to save time :)
## Installation
Release version:
```bash
$ pip install auto_profiler
```
Development version:
```bash
$ pip install -e git+https://github.com/modaresimr/auto_profiler.git#egg=auto_profiler
```
Install in Jupyter
```bash
$ pip install ipytree
$ jupyter nbextension enable --py --sys-prefix ipytree
```
## Quick start
[Jupyter Notebook](example.ipynb)
### Auto profiling
More commonly, chances are that we want to measure the execution time of an entry function and all its subfunctions. In this case, it's too tedious to do it manually, and we can leverage `Profiler` to inject all the timing points for us automatically:
```python
import time # line number 1
import random
from auto_profiler import Profiler, Tree
def f1():
mysleep(.6+random.random())
def mysleep(t):
time.sleep(t)
def fact(i):
f1()
if(i==1):
return 1
return i*fact(i-1)
@Profiler()
def main():
for i in range(5):
f1()
fact(3)
if __name__ == '__main__':
main()
```
#### Example Output
##### In Jupyter
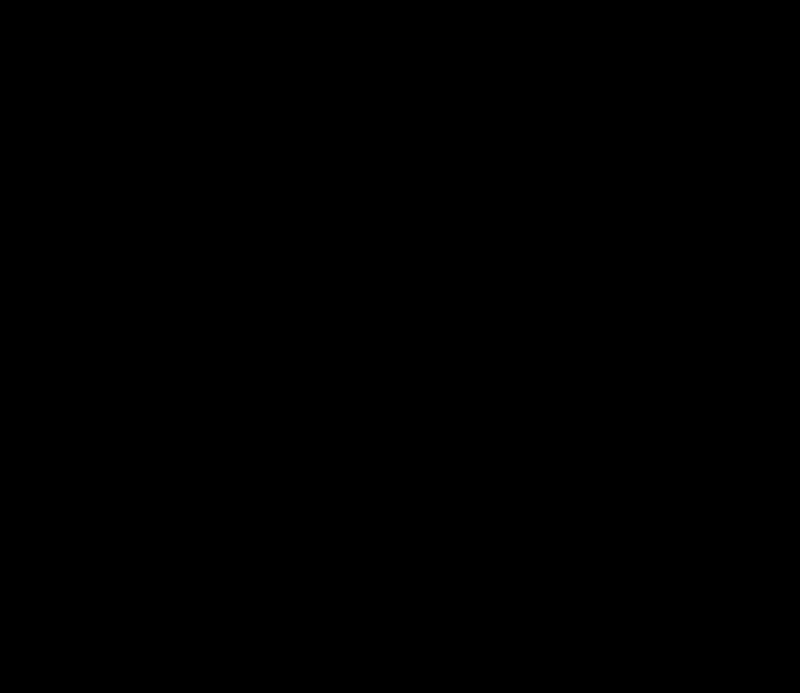
```
Time [Hits * PerHit] Function name [Called from] [function location]
-----------------------------------------------------------------------
8.974s [1 * 8.974] main [auto-profiler/profiler.py:267] [/test/t2.py:30]
├── 5.954s [5 * 1.191] f1 [/test/t2.py:34] [/test/t2.py:14]
│ └── 5.954s [5 * 1.191] mysleep [/test/t2.py:15] [/test/t2.py:17]
│ └── 5.954s [5 * 1.191] <time.sleep>
|
|
| # The rest is for the example recursive function call fact
└── 3.020s [1 * 3.020] fact [/test/t2.py:36] [/test/t2.py:20]
├── 0.849s [1 * 0.849] f1 [/test/t2.py:21] [/test/t2.py:14]
│ └── 0.849s [1 * 0.849] mysleep [/test/t2.py:15] [/test/t2.py:17]
│ └── 0.849s [1 * 0.849] <time.sleep>
└── 2.171s [1 * 2.171] fact [/test/t2.py:24] [/test/t2.py:20]
├── 1.552s [1 * 1.552] f1 [/test/t2.py:21] [/test/t2.py:14]
│ └── 1.552s [1 * 1.552] mysleep [/test/t2.py:15] [/test/t2.py:17]
└── 0.619s [1 * 0.619] fact [/test/t2.py:24] [/test/t2.py:20]
└── 0.619s [1 * 0.619] f1 [/test/t2.py:21] [/test/t2.py:14]
```
### Manual profiling
Sometimes, we only want to measure the execution time of partial snippets or a few functions, then we can inject all timing points into our code manually by leveraging `Timer`:
```python
# manual_example.py
import time
from auto_profiler import Timer, Tree
def main():
t = Timer('sleep1', parent_name='main').start()
time.sleep(1)
t.stop()
t = Timer('sleep2', parent_name='main').start()
time.sleep(1.5)
t.stop()
print(Tree(Timer.root))
if __name__ == '__main__':
main()
```
Run the example code:
```bash
$ python manual_example.py
```
and it will show you the profiling result:
```
2.503s main
├── 1.001s sleep1
└── 1.501s sleep2
```
## advanced setup
```
def show(p):
print('Time [Hits * PerHit] Function name [Called from] [Function Location]\n'+\
'-----------------------------------------------------------------------')
print(Tree(p.root, threshold=0.5))
@Profiler(depth=4, on_disable=show)
def main():
for i in range(5):
f1()
fact(3)
```
## Supported frameworks
While you can do profiling on normal Python code, as a web developer, chances are that you will usually do profiling on web service code.
Currently supported web frameworks:
- [Flask](http://flask.pocoo.org/)
## Examples
For profiling web service code (involving web requests), check out [examples](examples).
## License
[MIT](http://opensource.org/licenses/MIT)
Raw data
{
"_id": null,
"home_page": "https://github.com/modaresimr/auto_profiler",
"name": "auto-profiler",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "Profiling,Timer,Python,Auto prfiling,line profiler",
"author": "modaresi mr",
"author_email": "modaresimr+git@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/1d/66/aeed1b46280650e6c380e5b3b5c1e2de795687892f5654690938cd949c93/auto_profiler-2.0.tar.gz",
"platform": null,
"description": "# auto_profiler\n\nA realtime timer for profiling a Python function or snippet.\n\n## Features\n- Filtering external libraries profiling.\n- Filtering very short time functions-> threshold\n- Allow depth: you can easily find the time consuming function\n- Allow loop or multiple function call\n- Allow recursive function call\n- Disable it globaly by Profiler.GlobalDisable=True to save time :)\n## Installation\n\nRelease version:\n\n```bash\n$ pip install auto_profiler\n```\n\nDevelopment version:\n\n```bash\n$ pip install -e git+https://github.com/modaresimr/auto_profiler.git#egg=auto_profiler\n```\n\nInstall in Jupyter\n\n```bash\n$ pip install ipytree\n$ jupyter nbextension enable --py --sys-prefix ipytree\n```\n\n\n\n## Quick start\n\n[Jupyter Notebook](example.ipynb)\n### Auto profiling\nMore commonly, chances are that we want to measure the execution time of an entry function and all its subfunctions. In this case, it's too tedious to do it manually, and we can leverage `Profiler` to inject all the timing points for us automatically:\n\n```python\nimport time # line number 1\nimport random\n\nfrom auto_profiler import Profiler, Tree\n\ndef f1():\n mysleep(.6+random.random())\n\ndef mysleep(t):\n time.sleep(t)\n\ndef fact(i):\n f1()\n if(i==1):\n return 1\n return i*fact(i-1)\n\n\n@Profiler()\ndef main():\n for i in range(5):\n f1()\n\n fact(3)\n\n\nif __name__ == '__main__':\n main()\n\n```\n\n#### Example Output\n##### In Jupyter\n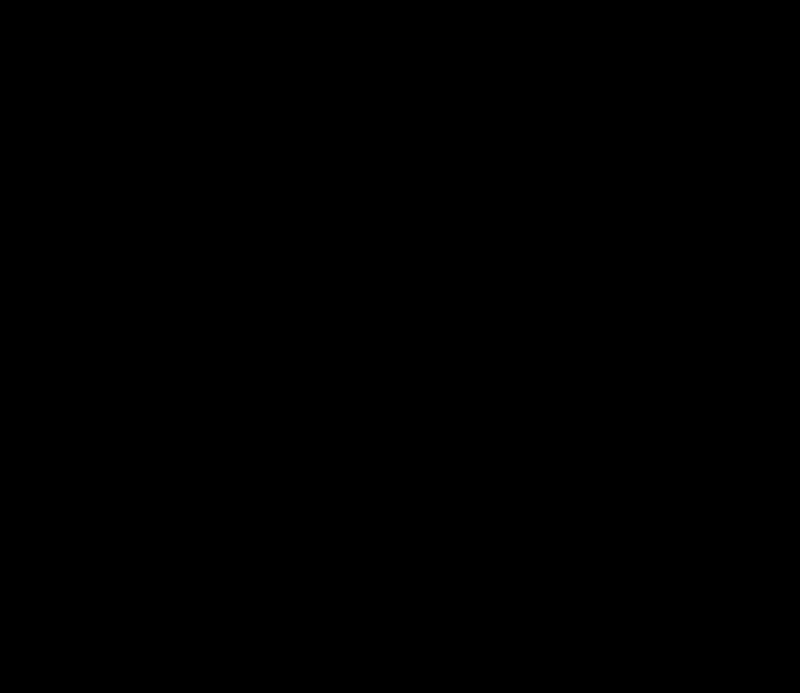\n```\n\nTime [Hits * PerHit] Function name [Called from] [function location]\n-----------------------------------------------------------------------\n8.974s [1 * 8.974] main [auto-profiler/profiler.py:267] [/test/t2.py:30]\n\u251c\u2500\u2500 5.954s [5 * 1.191] f1 [/test/t2.py:34] [/test/t2.py:14]\n\u2502 \u2514\u2500\u2500 5.954s [5 * 1.191] mysleep [/test/t2.py:15] [/test/t2.py:17]\n\u2502 \u2514\u2500\u2500 5.954s [5 * 1.191] <time.sleep>\n|\n|\n| # The rest is for the example recursive function call fact\n\u2514\u2500\u2500 3.020s [1 * 3.020] fact [/test/t2.py:36] [/test/t2.py:20]\n \u251c\u2500\u2500 0.849s [1 * 0.849] f1 [/test/t2.py:21] [/test/t2.py:14]\n \u2502 \u2514\u2500\u2500 0.849s [1 * 0.849] mysleep [/test/t2.py:15] [/test/t2.py:17]\n \u2502 \u2514\u2500\u2500 0.849s [1 * 0.849] <time.sleep>\n \u2514\u2500\u2500 2.171s [1 * 2.171] fact [/test/t2.py:24] [/test/t2.py:20]\n \u251c\u2500\u2500 1.552s [1 * 1.552] f1 [/test/t2.py:21] [/test/t2.py:14]\n \u2502 \u2514\u2500\u2500 1.552s [1 * 1.552] mysleep [/test/t2.py:15] [/test/t2.py:17]\n \u2514\u2500\u2500 0.619s [1 * 0.619] fact [/test/t2.py:24] [/test/t2.py:20]\n \u2514\u2500\u2500 0.619s [1 * 0.619] f1 [/test/t2.py:21] [/test/t2.py:14]\n```\n\n### Manual profiling\n\nSometimes, we only want to measure the execution time of partial snippets or a few functions, then we can inject all timing points into our code manually by leveraging `Timer`:\n\n```python\n\n# manual_example.py\n\nimport time\n\nfrom auto_profiler import Timer, Tree\n\n\ndef main():\n t = Timer('sleep1', parent_name='main').start()\n time.sleep(1)\n t.stop()\n\n t = Timer('sleep2', parent_name='main').start()\n time.sleep(1.5)\n t.stop()\n\n print(Tree(Timer.root))\n\n\nif __name__ == '__main__':\n main()\n```\n\nRun the example code:\n\n```bash\n$ python manual_example.py\n```\n\nand it will show you the profiling result:\n\n```\n2.503s main\n\u251c\u2500\u2500 1.001s sleep1\n\u2514\u2500\u2500 1.501s sleep2\n\n```\n\n## advanced setup\n\n\n```\ndef show(p):\n print('Time [Hits * PerHit] Function name [Called from] [Function Location]\\n'+\\\n '-----------------------------------------------------------------------')\n print(Tree(p.root, threshold=0.5))\n \n@Profiler(depth=4, on_disable=show)\ndef main():\n for i in range(5):\n f1()\n\n fact(3)\n\n```\n\n## Supported frameworks\n\nWhile you can do profiling on normal Python code, as a web developer, chances are that you will usually do profiling on web service code.\n\nCurrently supported web frameworks:\n\n- [Flask](http://flask.pocoo.org/)\n\n\n## Examples\n\nFor profiling web service code (involving web requests), check out [examples](examples).\n\n\n## License\n\n[MIT](http://opensource.org/licenses/MIT)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A realtime timer for profiling a Python function or snippet.",
"version": "2.0",
"split_keywords": [
"profiling",
"timer",
"python",
"auto prfiling",
"line profiler"
],
"urls": [
{
"comment_text": "",
"digests": {
"md5": "c1b956ce158e5b836cc6de165b84f6ea",
"sha256": "131305f8190a7ec476cfaa7a585fa6441cfc240328c2806837409155040e2e6c"
},
"downloads": -1,
"filename": "auto_profiler-2.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "c1b956ce158e5b836cc6de165b84f6ea",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 12707,
"upload_time": "2022-09-15T21:01:28",
"upload_time_iso_8601": "2022-09-15T21:01:28.506063Z",
"url": "https://files.pythonhosted.org/packages/d0/ff/37e6a7b3aa6e547f0e9ff54c73350ba1d46816a4f5f558a52dc0e2d6ab86/auto_profiler-2.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"md5": "b6914e376b9874bd833bc97c68688f3b",
"sha256": "985bf3cb28b3fb130bae94fc4de71f3e9da987eac65ffc9c2bdc9018650d3323"
},
"downloads": -1,
"filename": "auto_profiler-2.0.tar.gz",
"has_sig": false,
"md5_digest": "b6914e376b9874bd833bc97c68688f3b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 11765,
"upload_time": "2022-09-15T21:01:30",
"upload_time_iso_8601": "2022-09-15T21:01:30.033020Z",
"url": "https://files.pythonhosted.org/packages/1d/66/aeed1b46280650e6c380e5b3b5c1e2de795687892f5654690938cd949c93/auto_profiler-2.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2022-09-15 21:01:30",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "modaresimr",
"github_project": "auto_profiler",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "auto-profiler"
}