# automathon
<p align="center">
<a href="https://rohaquinlop.github.io/automathon/"><img src="docs/img/logo-vector.svg" alt="automathon"></a>
</p>
<p align="center">
<em>A Python library for simulating and visualizing finite automata.</em>
</p>
<p align="center">
<a href="https://github.com/rohaquinlop/automathon" target="_blank">
<img src="https://github.com/rohaquinlop/automathon/actions/workflows/main.yml/badge.svg?branch=main" alt="Test">
</a>
<a href="https://sonarcloud.io/summary/new_code?id=rohaquinlop_automathon" target="_blank">
<img src="https://sonarcloud.io/api/project_badges/measure?project=rohaquinlop_automathon&metric=alert_status" alt="Quality Gate">
</a>
<a href="https://pypi.org/project/automathon" target="_blank">
<img src="https://img.shields.io/pypi/v/automathon?color=%2334D058&label=pypi%20package" alt="Package version">
</a>
</p>
---
**Documentation**: <a href="https://rohaquinlop.github.io/automathon/" target="_blank">https://rohaquinlop.github.io/automathon/</a>
**Source Code**: <a href="https://github.com/rohaquinlop/automathon" target="_blank">https://github.com/rohaquinlop/automathon</a>
**PyPI**: <a href="https://pypi.org/project/automathon/" target="_blank">https://pypi.org/project/automathon/</a>
---
## Requirements
- Python >= 3.10
- You also need to install Graphviz on your computer ([download page](https://www.graphviz.org/download/), [installation procedure for Windows](https://forum.graphviz.org/t/new-simplified-installation-procedure-on-windows/224), [archived versions](https://www2.graphviz.org/Archive/stable/)).Make sure that the directory containing the **dot** executable is on your systems’ path.
## Installation
```bash
pip install automathon
```
### Upgrade
```bash
pip install automathon --upgrade
```
## Example
Here is are some examples about what you can do with **automathon**, you can
check the documentation about [Deterministic Finite Automata](dfa.md) and
[Non-Deterministic Finite Automata](nfa.md) to know more about the functions and
methods that are available.
### DFA - Deterministic Finite Automata
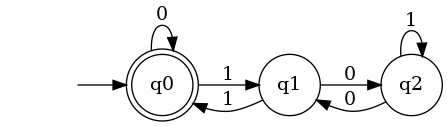
This image was created using **automathon**.
Let's create the previous automata using the library:
```python
from automathon import DFA
q = {'q0', 'q1', 'q2'}
sigma = {'0', '1'}
delta = { 'q0' : {'0' : 'q0', '1' : 'q1'},
'q1' : {'0' : 'q2', '1' : 'q0'},
'q2' : {'0' : 'q1', '1' : 'q2'}
}
initial_state = 'q0'
f = {'q0'}
automata = DFA(q, sigma, delta, initial_state, f)
```
#### Verify if the automata is valid
```python
automata.is_valid() # True
```
In this case, the automata is valid but if it wasn't, the library would raise an
exception with the error message.
#### Errors
Errors that the library can raise are:
- **SigmaError**:
- The automata contain an initial state, or a final state that's not defined in Q.
- The automata contain a delta transition that's not defined in Q nor Sigma.
- **InputError**:
- The automata is trying to consume a letter that's not defined in sigma.
#### Verify if the automata accept a given string
```python
automata.accept("001001") # True
automata.accept("00100") # False
```
#### Get the automata's complement
```python
not_automata = automata.complement()
not_automata.accept("00100") #True
```
Note that this function returns a new automata, it doesn't modify the original
one.
#### Visualize the automata
For both, [DFA](dfa.md) and [NFA](nfa.md), the view method enables to visualize the automaton, receives as parameter a String as the file name for the png and svg files.
More information about the graphviz attributes [here](https://www.graphviz.org/doc/info/attrs.html).
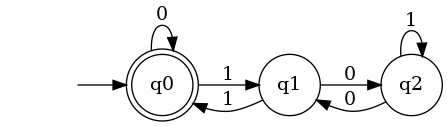
```python
# Default styling
automata.view("DFA Visualization")
# If you want to add custom styling, you can use the following
automata.view(
file_name="DFA Custom Styling",
node_attr={'fontsize': '20'},
edge_attr={'fontsize': '20pt'}
)
```
If you want to explore more about the functions and methods of the DFA class,
you can check the [DFA documentation](dfa.md). And if you want to know more about
the NFA class, you can check the [NFA documentation](nfa.md).
### NFA - Non-Deterministic Finite Automata
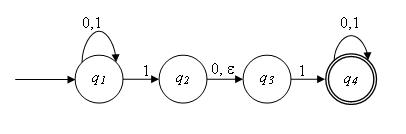
Image taken from: [r9paul.org](http://www.r9paul.org/blog/2008/nondeterministic-finite-state-machine/)
#### Representing the previous automata
```python
from automathon import NFA
## Epsilon Transition is denoted by '' -> Empty string
q = {'q1', 'q2', 'q3', 'q4'}
sigma = {'0', '1'}
delta = {
'q1' : {
'0' : {'q1'},
'1' : {'q1', 'q2'}
},
'q2' : {
'0' : {'q3'},
'' : {'q3'}
},
'q3' : {
'1' : {'q4'},
},
'q4' : {
'0' : {'q4'},
'1' : {'q4'},
},
}
initial_state = 'q1'
f = {'q4'}
automata = NFA(q, sigma, delta, initial_state, f)
```
#### Verify if the automata is valid
```python
automata.is_valid() # True
```
#### Verify if the automata accept a string
```python
automata.accept("0000011") #True
automata.accept("000001") #False
```
#### Get the automata's complement
```python
not_automata = automata.complement()
not_automata.accept("000001") #True
```
#### Visualize the automata
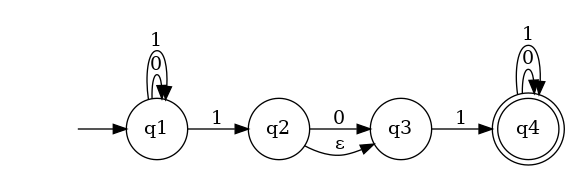
```python
# Default styling
automata.view("NFA Visualization")
# If you want to add custom styling, you can use the following
automata.view(
file_name="NFA Custom Styling",
node_attr={'fontsize': '20'},
edge_attr={'fontsize': '20pt'}
)
```
## License
This project is licensed under the terms of the MIT license.
Raw data
{
"_id": null,
"home_page": "https://github.com/rohaquinlop/automathon",
"name": "automathon",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "python, automata, automata, automathon",
"author": "Robin Hafid Quintero Lopez",
"author_email": "rohaquinlop301@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/03/97/fb50d1a2d093d8766ee42088a73caea70c0c9b90494a107380ccd504804a/automathon-0.0.15.tar.gz",
"platform": null,
"description": "# automathon\n\n<p align=\"center\">\n <a href=\"https://rohaquinlop.github.io/automathon/\"><img src=\"docs/img/logo-vector.svg\" alt=\"automathon\"></a>\n</p>\n\n<p align=\"center\">\n <em>A Python library for simulating and visualizing finite automata.</em>\n</p>\n\n<p align=\"center\">\n <a href=\"https://github.com/rohaquinlop/automathon\" target=\"_blank\">\n <img src=\"https://github.com/rohaquinlop/automathon/actions/workflows/main.yml/badge.svg?branch=main\" alt=\"Test\">\n </a>\n <a href=\"https://sonarcloud.io/summary/new_code?id=rohaquinlop_automathon\" target=\"_blank\">\n <img src=\"https://sonarcloud.io/api/project_badges/measure?project=rohaquinlop_automathon&metric=alert_status\" alt=\"Quality Gate\">\n </a>\n <a href=\"https://pypi.org/project/automathon\" target=\"_blank\">\n <img src=\"https://img.shields.io/pypi/v/automathon?color=%2334D058&label=pypi%20package\" alt=\"Package version\">\n</a>\n</p>\n\n---\n\n**Documentation**: <a href=\"https://rohaquinlop.github.io/automathon/\" target=\"_blank\">https://rohaquinlop.github.io/automathon/</a>\n\n**Source Code**: <a href=\"https://github.com/rohaquinlop/automathon\" target=\"_blank\">https://github.com/rohaquinlop/automathon</a>\n\n**PyPI**: <a href=\"https://pypi.org/project/automathon/\" target=\"_blank\">https://pypi.org/project/automathon/</a>\n\n---\n\n## Requirements\n\n- Python >= 3.10\n- You also need to install Graphviz on your computer ([download page](https://www.graphviz.org/download/), [installation procedure for Windows](https://forum.graphviz.org/t/new-simplified-installation-procedure-on-windows/224), [archived versions](https://www2.graphviz.org/Archive/stable/)).Make sure that the directory containing the **dot** executable is on your systems\u2019 path.\n\n## Installation\n\n```bash\npip install automathon\n```\n\n### Upgrade\n\n```bash\npip install automathon --upgrade\n```\n\n## Example\n\nHere is are some examples about what you can do with **automathon**, you can\ncheck the documentation about [Deterministic Finite Automata](dfa.md) and\n[Non-Deterministic Finite Automata](nfa.md) to know more about the functions and\nmethods that are available.\n\n### DFA - Deterministic Finite Automata\n\n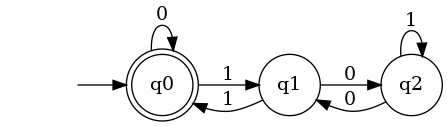\n\nThis image was created using **automathon**.\n\nLet's create the previous automata using the library:\n\n```python\nfrom automathon import DFA\nq = {'q0', 'q1', 'q2'}\nsigma = {'0', '1'}\ndelta = { 'q0' : {'0' : 'q0', '1' : 'q1'},\n 'q1' : {'0' : 'q2', '1' : 'q0'},\n 'q2' : {'0' : 'q1', '1' : 'q2'}\n }\ninitial_state = 'q0'\nf = {'q0'}\n\nautomata = DFA(q, sigma, delta, initial_state, f)\n```\n\n#### Verify if the automata is valid\n\n```python\nautomata.is_valid() # True\n```\n\nIn this case, the automata is valid but if it wasn't, the library would raise an\nexception with the error message.\n\n#### Errors\n\nErrors that the library can raise are:\n\n- **SigmaError**:\n - The automata contain an initial state, or a final state that's not defined in Q.\n - The automata contain a delta transition that's not defined in Q nor Sigma.\n\n- **InputError**:\n - The automata is trying to consume a letter that's not defined in sigma.\n\n#### Verify if the automata accept a given string\n\n```python\nautomata.accept(\"001001\") # True\nautomata.accept(\"00100\") # False\n```\n\n#### Get the automata's complement\n\n```python\nnot_automata = automata.complement()\nnot_automata.accept(\"00100\") #True\n```\n\nNote that this function returns a new automata, it doesn't modify the original\none.\n\n#### Visualize the automata\n\nFor both, [DFA](dfa.md) and [NFA](nfa.md), the view method enables to visualize the automaton, receives as parameter a String as the file name for the png and svg files.\n\nMore information about the graphviz attributes [here](https://www.graphviz.org/doc/info/attrs.html).\n\n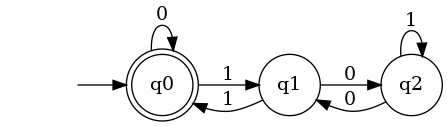\n```python\n# Default styling\nautomata.view(\"DFA Visualization\")\n\n# If you want to add custom styling, you can use the following\n\nautomata.view(\n file_name=\"DFA Custom Styling\",\n node_attr={'fontsize': '20'},\n edge_attr={'fontsize': '20pt'}\n)\n```\n\nIf you want to explore more about the functions and methods of the DFA class,\nyou can check the [DFA documentation](dfa.md). And if you want to know more about\nthe NFA class, you can check the [NFA documentation](nfa.md).\n\n### NFA - Non-Deterministic Finite Automata\n\n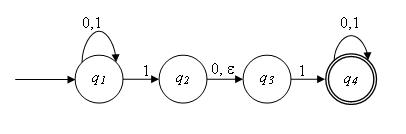\n\nImage taken from: [r9paul.org](http://www.r9paul.org/blog/2008/nondeterministic-finite-state-machine/)\n\n#### Representing the previous automata\n\n```python\nfrom automathon import NFA\n\n## Epsilon Transition is denoted by '' -> Empty string\nq = {'q1', 'q2', 'q3', 'q4'}\nsigma = {'0', '1'}\ndelta = {\n 'q1' : {\n '0' : {'q1'},\n '1' : {'q1', 'q2'}\n },\n 'q2' : {\n '0' : {'q3'},\n '' : {'q3'}\n },\n 'q3' : {\n '1' : {'q4'},\n },\n 'q4' : {\n '0' : {'q4'},\n '1' : {'q4'},\n },\n}\ninitial_state = 'q1'\nf = {'q4'}\n\nautomata = NFA(q, sigma, delta, initial_state, f)\n```\n\n#### Verify if the automata is valid\n\n```python\nautomata.is_valid() # True\n```\n\n#### Verify if the automata accept a string\n\n```python\nautomata.accept(\"0000011\") #True\nautomata.accept(\"000001\") #False\n```\n\n#### Get the automata's complement\n\n```python\nnot_automata = automata.complement()\nnot_automata.accept(\"000001\") #True\n```\n\n#### Visualize the automata\n\n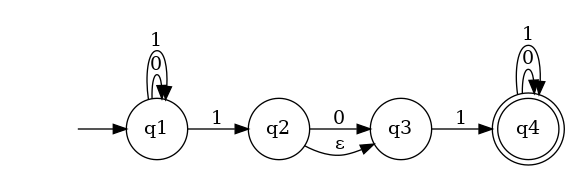\n```python\n# Default styling\nautomata.view(\"NFA Visualization\")\n\n# If you want to add custom styling, you can use the following\n\nautomata.view(\n file_name=\"NFA Custom Styling\",\n node_attr={'fontsize': '20'},\n edge_attr={'fontsize': '20pt'}\n)\n```\n\n## License\n\nThis project is licensed under the terms of the MIT license.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python library for simulating and visualizing finite automata",
"version": "0.0.15",
"project_urls": {
"Download": "https://github.com/rohaquinlop/automathon/archive/refs/tags/v0.0.15.tar.gz",
"Homepage": "https://github.com/rohaquinlop/automathon"
},
"split_keywords": [
"python",
" automata",
" automata",
" automathon"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "cbba3c8bfeb61437c78cf3616cd67670326798fba5709544edd9046c2e099eef",
"md5": "8f742ae2a57e6531b979bb9d1ef39cd5",
"sha256": "93ff6f25a0022cf52633d12cc91938a8c3326bd3580290a4bc81f2378b7036db"
},
"downloads": -1,
"filename": "automathon-0.0.15-py3-none-any.whl",
"has_sig": false,
"md5_digest": "8f742ae2a57e6531b979bb9d1ef39cd5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 13509,
"upload_time": "2024-04-09T06:19:56",
"upload_time_iso_8601": "2024-04-09T06:19:56.087123Z",
"url": "https://files.pythonhosted.org/packages/cb/ba/3c8bfeb61437c78cf3616cd67670326798fba5709544edd9046c2e099eef/automathon-0.0.15-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0397fb50d1a2d093d8766ee42088a73caea70c0c9b90494a107380ccd504804a",
"md5": "c580542fe0e6cc12b159eca9cdf5e098",
"sha256": "81106726b5e44b8bb530b0c76468e027987ef9774010a469e0f8a1f2c2ae2171"
},
"downloads": -1,
"filename": "automathon-0.0.15.tar.gz",
"has_sig": false,
"md5_digest": "c580542fe0e6cc12b159eca9cdf5e098",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 13229,
"upload_time": "2024-04-09T06:19:57",
"upload_time_iso_8601": "2024-04-09T06:19:57.868818Z",
"url": "https://files.pythonhosted.org/packages/03/97/fb50d1a2d093d8766ee42088a73caea70c0c9b90494a107380ccd504804a/automathon-0.0.15.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-09 06:19:57",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "rohaquinlop",
"github_project": "automathon",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "automathon"
}