# AWS Lambda Typing


[](LICENSE)

[](https://pypi.org/project/aws-lambda-typing)
[](https://pypi.org/project/aws-lambda-typing)
A package that provides type hints for AWS Lambda event, context and response
objects. It's a convenient way to get autocomplete and type hints built into
IDEs. Type annotations are not checked at runtime but are only enforced by third
party tools such as type checkers, IDEs, linters, etc.
##### Table of Contents
- [Usage](#usage)
- [Demo](#demo)
- [Types](#types)
- [Context](#context)
- [Events](#events)
- [Responses](#responses)
- [Test](#test)
- [Contributing](#contributing)
- [Issues](#issues)
## Usage
### Example: AWS SQS event
```python
from aws_lambda_typing import context as context_, events
def handler(event: events.SQSEvent, context: context_.Context) -> None:
for record in event['Records']:
print(record['body'])
print(context.get_remaining_time_in_millis())
message: events.sqs.SQSMessage
```
## Demo
### IDE autocomplete
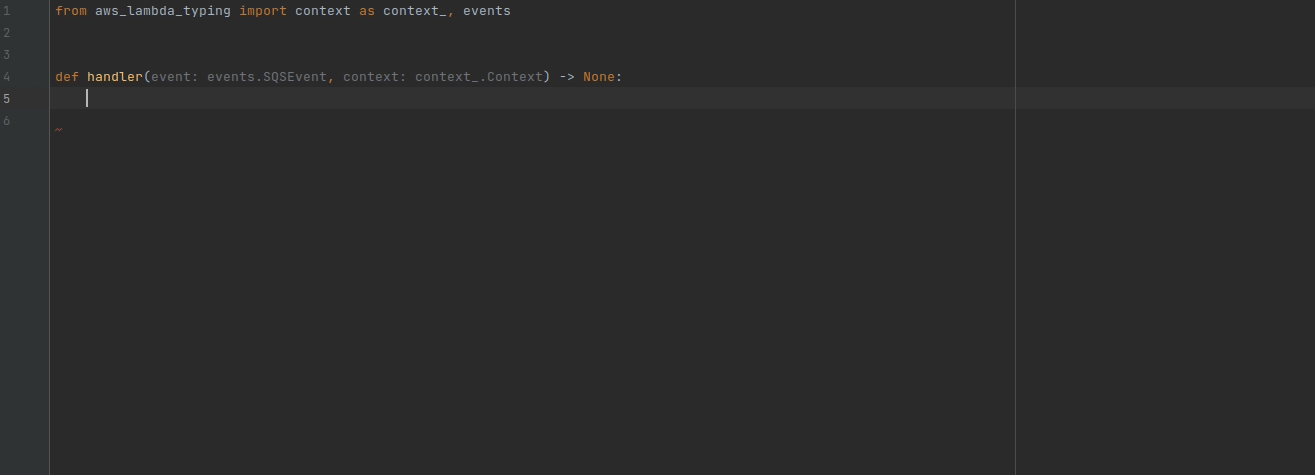
### IDE code reference information
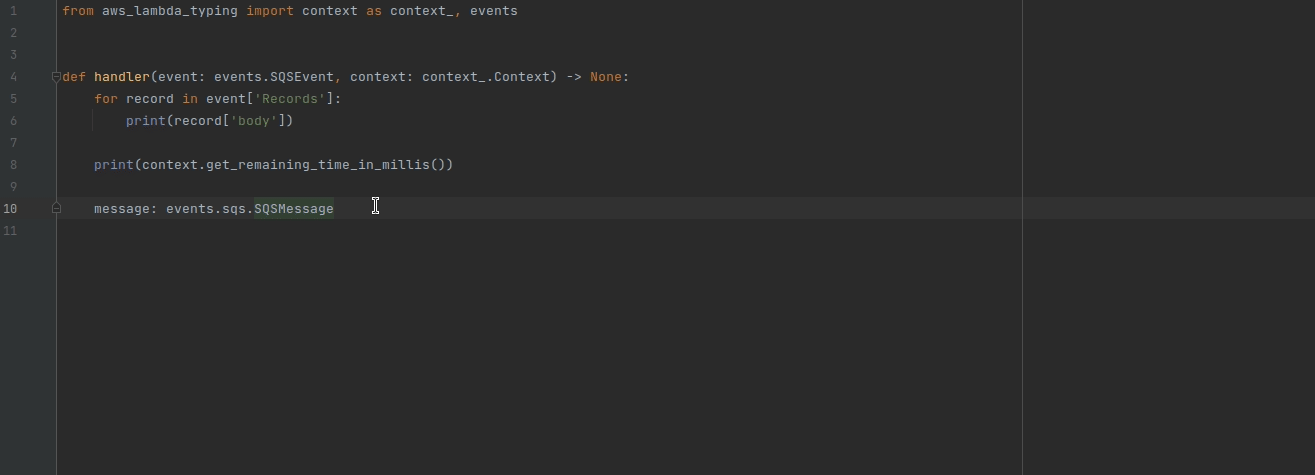
## Types
### Context
- Context
### Events
- ALBEvent
- ApacheKafkaEvent
- APIGatewayRequestAuthorizerEvent
- APIGatewayTokenAuthorizerEvent
- APIGatewayProxyEventV1
- APIGatewayProxyEventV2
- AppSyncResolverEvent
- CloudFormationCustomResourceEvent
- CloudWatchEventsMessageEvent (Deprecated since version 2.10.0: use `EventBridgeEvent` instead.)
- CloudWatchLogsEvent
- CodeCommitMessageEvent
- CodePipelineEvent
- CognitoCustomMessageEvent
- CognitoPostConfirmationEvent
- ConfigEvent
- DynamoDBStreamEvent
- EventBridgeEvent
- EC2ASGCustomTerminationPolicyEvent
- IoTPreProvisioningHookEvent
- KinesisFirehoseEvent
- KinesisStreamEvent
- MQEvent
- MSKEvent
- S3Event
- S3BatchEvent
- SecretsManagerRotationEvent
- SESEvent
- SNSEvent
- SQSEvent
- WebSocketConnectEvent
- WebSocketRouteEvent
### Requests
- SNSPublish
- SNSPublishBatch
### Responses
- ALBResponse
- APIGatewayAuthorizerResponse
- APIGatewayProxyResponseV1
- APIGatewayProxyResponseV2
- DynamoDBBatchResponse
- IoTPreProvisioningHookResponse
- KinesisFirehoseTransformationResponse
- S3BatchResponse
### Other
- PolicyDocument
## Contributing
Contributions are welcome! See the [Contributing Guide](https://github.com/MousaZeidBaker/aws-lambda-typing/blob/master/CONTRIBUTING.md).
## Issues
If you encounter any problems, please file an
[issue](https://github.com/MousaZeidBaker/aws-lambda-typing/issues) along with a
detailed description.
Raw data
{
"_id": null,
"home_page": "https://github.com/MousaZeidBaker/aws-lambda-typing",
"name": "aws-lambda-typing",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.6",
"maintainer_email": null,
"keywords": "typing, type hints, aws, lambda, serverless, development",
"author": "Mousa Zeid Baker",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/ba/2a/14e7a3dba738265db6eddfd0c9ec1619c0c14e48fcf70627fc75fca83305/aws-lambda-typing-2.20.0.tar.gz",
"platform": null,
"description": "# AWS Lambda Typing\n\n\n\n[](LICENSE)\n\n[](https://pypi.org/project/aws-lambda-typing)\n[](https://pypi.org/project/aws-lambda-typing)\n\nA package that provides type hints for AWS Lambda event, context and response\nobjects. It's a convenient way to get autocomplete and type hints built into\nIDEs. Type annotations are not checked at runtime but are only enforced by third\nparty tools such as type checkers, IDEs, linters, etc.\n\n##### Table of Contents\n- [Usage](#usage)\n- [Demo](#demo)\n- [Types](#types)\n - [Context](#context)\n - [Events](#events)\n - [Responses](#responses)\n- [Test](#test)\n- [Contributing](#contributing)\n- [Issues](#issues)\n\n## Usage\n### Example: AWS SQS event\n\n```python\nfrom aws_lambda_typing import context as context_, events\n\n\ndef handler(event: events.SQSEvent, context: context_.Context) -> None:\n for record in event['Records']:\n print(record['body'])\n\n print(context.get_remaining_time_in_millis())\n\n message: events.sqs.SQSMessage\n\n```\n\n## Demo\n### IDE autocomplete\n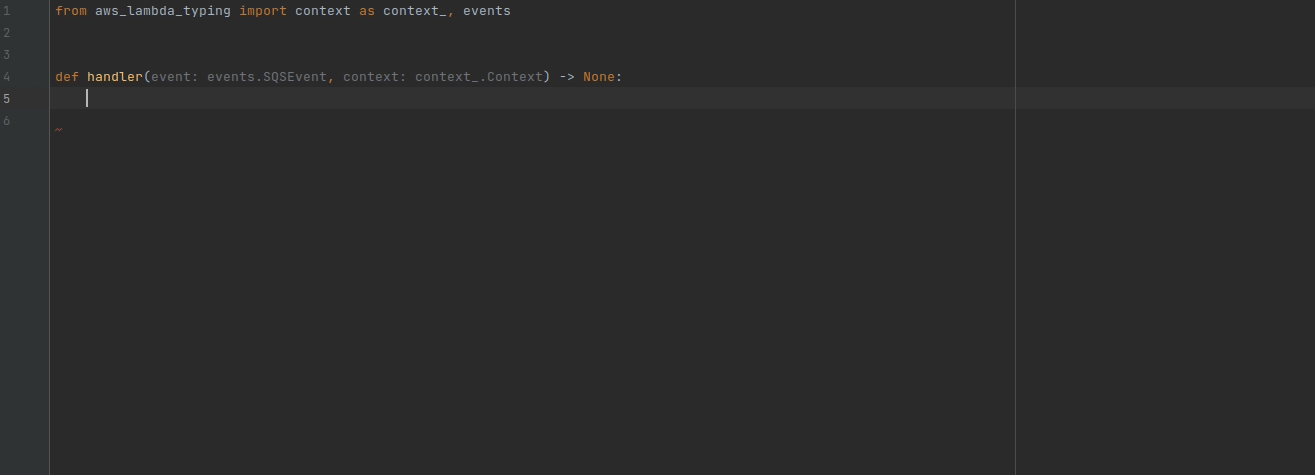\n\n### IDE code reference information\n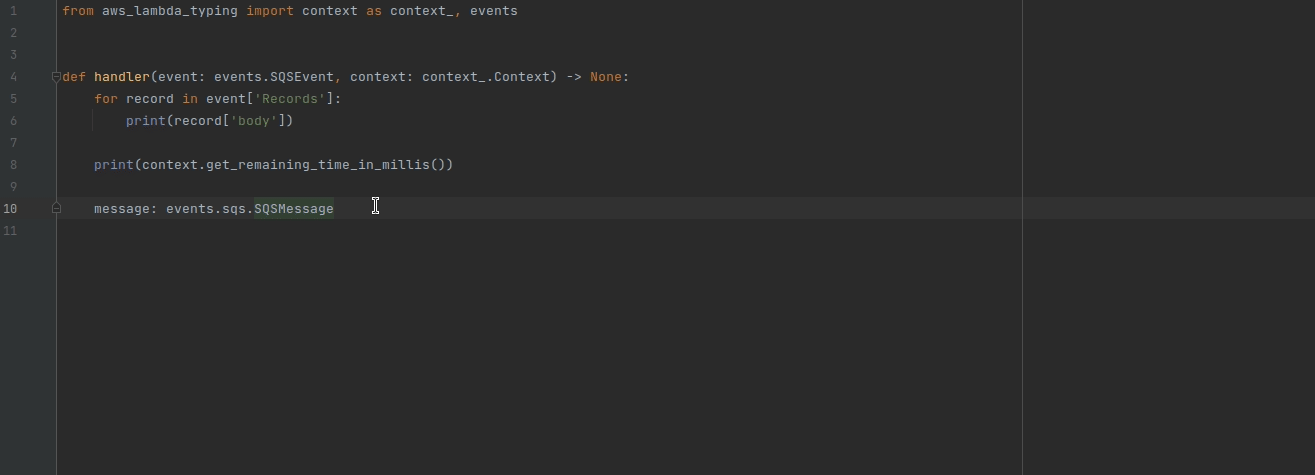\n\n## Types\n### Context\n- Context\n\n### Events\n- ALBEvent\n- ApacheKafkaEvent\n- APIGatewayRequestAuthorizerEvent\n- APIGatewayTokenAuthorizerEvent\n- APIGatewayProxyEventV1\n- APIGatewayProxyEventV2\n- AppSyncResolverEvent\n- CloudFormationCustomResourceEvent\n- CloudWatchEventsMessageEvent (Deprecated since version 2.10.0: use `EventBridgeEvent` instead.)\n- CloudWatchLogsEvent\n- CodeCommitMessageEvent\n- CodePipelineEvent\n- CognitoCustomMessageEvent\n- CognitoPostConfirmationEvent\n- ConfigEvent\n- DynamoDBStreamEvent\n- EventBridgeEvent\n- EC2ASGCustomTerminationPolicyEvent\n- IoTPreProvisioningHookEvent\n- KinesisFirehoseEvent\n- KinesisStreamEvent\n- MQEvent\n- MSKEvent\n- S3Event\n- S3BatchEvent\n- SecretsManagerRotationEvent\n- SESEvent\n- SNSEvent\n- SQSEvent\n- WebSocketConnectEvent\n- WebSocketRouteEvent\n\n### Requests\n- SNSPublish\n- SNSPublishBatch\n\n### Responses\n- ALBResponse\n- APIGatewayAuthorizerResponse\n- APIGatewayProxyResponseV1\n- APIGatewayProxyResponseV2\n- DynamoDBBatchResponse\n- IoTPreProvisioningHookResponse\n- KinesisFirehoseTransformationResponse\n- S3BatchResponse\n\n### Other\n- PolicyDocument\n\n## Contributing\n\nContributions are welcome! See the [Contributing Guide](https://github.com/MousaZeidBaker/aws-lambda-typing/blob/master/CONTRIBUTING.md).\n\n## Issues\n\nIf you encounter any problems, please file an\n[issue](https://github.com/MousaZeidBaker/aws-lambda-typing/issues) along with a\ndetailed description.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A package that provides type hints for AWS Lambda event, context and response objects",
"version": "2.20.0",
"project_urls": {
"Homepage": "https://github.com/MousaZeidBaker/aws-lambda-typing",
"Repository": "https://github.com/MousaZeidBaker/aws-lambda-typing"
},
"split_keywords": [
"typing",
" type hints",
" aws",
" lambda",
" serverless",
" development"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "553d4031f5950d65e89136d20a18b5ac985d5f087c29c902f285b6e8063619e4",
"md5": "8f41d2826ad3a4dbf4e5384115544dd7",
"sha256": "1d44264cabfeab5ac38e67ddd0c874e677b2cbbae77a42d0519df470e6bbb49b"
},
"downloads": -1,
"filename": "aws_lambda_typing-2.20.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "8f41d2826ad3a4dbf4e5384115544dd7",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.6",
"size": 35296,
"upload_time": "2024-04-02T09:43:05",
"upload_time_iso_8601": "2024-04-02T09:43:05.881939Z",
"url": "https://files.pythonhosted.org/packages/55/3d/4031f5950d65e89136d20a18b5ac985d5f087c29c902f285b6e8063619e4/aws_lambda_typing-2.20.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ba2a14e7a3dba738265db6eddfd0c9ec1619c0c14e48fcf70627fc75fca83305",
"md5": "f51f4bd5786b107efd713ea36ed60fe8",
"sha256": "78b0d8ebab73b3a6b0da98a7969f4e9c4bb497298ec50f3217da8a8dfba17154"
},
"downloads": -1,
"filename": "aws-lambda-typing-2.20.0.tar.gz",
"has_sig": false,
"md5_digest": "f51f4bd5786b107efd713ea36ed60fe8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.6",
"size": 19413,
"upload_time": "2024-04-02T09:43:03",
"upload_time_iso_8601": "2024-04-02T09:43:03.326057Z",
"url": "https://files.pythonhosted.org/packages/ba/2a/14e7a3dba738265db6eddfd0c9ec1619c0c14e48fcf70627fc75fca83305/aws-lambda-typing-2.20.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-02 09:43:03",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "MousaZeidBaker",
"github_project": "aws-lambda-typing",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "aws-lambda-typing"
}