# BeauPy
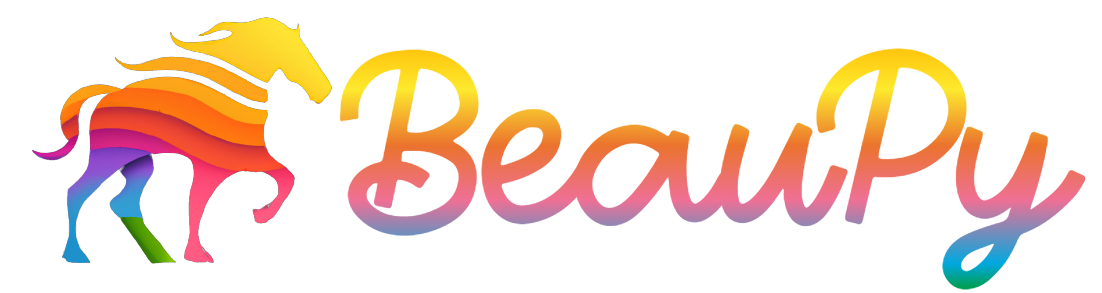
> A Python library of interactive CLI elements you have been looking for
---
[](https://github.com/petereon/beaupy/actions/workflows/python-test.yml)
[](https://github.com/petereon/beaupy/actions/workflows/python-lint.yml)
[](https://codecov.io/gh/petereon/beaupy)
[](https://sonarcloud.io/summary/new_code?id=petereon_beaupy)
[](https://sonarcloud.io/summary/new_code?id=petereon_beaupy)

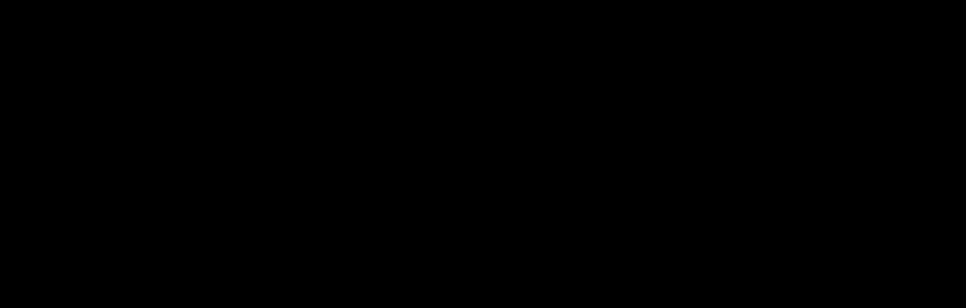
For documentation but more and prettier see [**here**](https://petereon.github.io/beaupy/)
## Acknowledgment
BeauPy stands on the shoulders of giants. It is based on another library with which it shares some of the source code, [`cutie`](https://github.com/kamik423/cutie), developed by [Kamik423](https://github.com/Kamik423). It has begun as a fork but has since diverged into its own thing and as such, detached from the original repository.
## Overview
**BeauPy** implements a number of common interactive elements:
| Function | Functionality |
|:---------------------------------------------------------------------------------|:-------------------------------------------------------------------------------------------|
| [`select`](https://petereon.github.io/beaupy/api/#select) | Prompt to pick a choice from a list |
| [`select_multiple`](https://petereon.github.io/beaupy/api/#select_multiple) | Prompt to select one or multiple choices from a list |
| [`confirm`](https://petereon.github.io/beaupy/api/#confirm) | Prompt with a question and yes/no options |
| [`prompt`](https://petereon.github.io/beaupy/api/#prompt) | Prompt that takes free input with optional validation, type conversion and input hiding |
TUI elements shown in the above gif are the result of the following code:
```python
import time
from beaupy import confirm, prompt, select, select_multiple
from beaupy.spinners import *
from rich.console import Console
console = Console()
# Confirm a dialog
if confirm("Will you take the ring to Mordor?"):
names = [
"Frodo Baggins",
"Samwise Gamgee",
"Legolas",
"Aragorn",
"[red]Sauron[/red]",
]
console.print("Who are you?")
# Choose one item from a list
name = select(names, cursor="π’§", cursor_style="cyan")
console.print(f"AlΓ‘menΓ«, {name}")
item_options = [
"The One Ring",
"Dagger",
"Po-tae-toes",
"Lightsaber (Wrong franchise! Nevermind, roll with it!)",
]
console.print("What do you bring with you?")
# Choose multiple options from a list
items = select_multiple(item_options, tick_character='π', ticked_indices=[0], maximal_count=3)
potato_count = 0
if "Po-tae-toes" in items:
# Prompt with type conversion and validation
potato_count = prompt('How many potatoes?', target_type=int, validator=lambda count: count > 0)
# Spinner to show while doing some work
spinner = Spinner(DOTS, "Packing things...")
spinner.start()
time.sleep(2)
spinner.stop()
# Get input without showing it being typed
if "friend" == prompt("Speak, [blue bold underline]friend[/blue bold underline], and enter", secure=True).lower():
# Custom spinner animation
spinner_animation = ['ββ', 'ββ', ' ', 'ββ', 'ββ']
spinner = Spinner(spinner_animation, "Opening the Door of Durin...")
spinner.start()
time.sleep(2)
spinner.stop()
else:
spinner_animation = ['ππ βοΈ ', 'π π βοΈ ', 'π π βοΈ ', 'π π βοΈ ', 'π πβοΈ ']
spinner = Spinner(spinner_animation, "Getting attacked by an octopus...")
spinner.start()
time.sleep(2)
spinner.stop()
if 'The One Ring' in items:
console.print("[green]You throw The One Ring to a lava from an eagle![/green]")
else:
console.print("[red]You forgot the ring and brought Middle-Earth to its knees![/red]")
console.print(f"And you brought {potato_count} taters!")
```
For more information refer to [more examples](https://petereon.github.io/beaupy/examples/) or definitive, but much less exciting [API documentation](https://petereon.github.io/beaupy/api/)
## Installation
From PyPI:
```sh
pip install beaupy
```
From source:
```sh
git clone https://github.com/petereon/beaupy.git
poetry build
pip install ./dist/beaupy-{{some-version}}-py3-none-any.whl
```
## Roadmap
This repository has a [associated GitHub project](https://github.com/users/petereon/projects/3/views/1) where work that is currently done can be seen.
## Contributing
If you would like to contribute, please feel free to suggest features or implement them yourself.
Also **please report any issues and bugs you might find!**
### Development
To start development you can clone the repository:
```sh
git clone https://github.com/petereon/beaupy.git
```
Change the directory to the project directory:
```sh
cd ./beaupy/
```
This project uses [`poetry`](https://python-poetry.org/) as a dependency manager. You can install the dependencies using:
```sh
poetry install
```
For testing, this project relies on [`ward`](https://github.com/darrenburns/ward). It is included as a development dependency, so
after installing the dependencies you can simply execute the following:
```sh
poetry run poe test
```
Making sure the code follows quality standards and formatting can be ensured by executing
```sh
poetry run poe lint
```
You can also have the tests and lints run after every saved change by executing a respective watch command
```sh
poetry run poe test:watch
```
or
```sh
poetry run poe lint:watch
```
After you have made your changes, create a pull request towards a master branch of this repository
Looking forward to your pull requests!
## Compatibility
Internal logic of `beaupy` is supported for all the major platforms (Windows, Linux, macOS).
- For user input from console, `beaupy` relies on [petereon/yakh](https://github.com/petereon/yakh), which is tested against all the major platforms and Python versions.
- For printing to console `beaupy` relies on [Textualize/rich](https://github.com/Textualize/rich), which [claims to support](https://github.com/Textualize/rich#compatibility) all the major platforms.
## Known Issues
- Version `2.x.x`: arrow keys reportedly don't always work on Windows. Resolved in `3.x.x`
- Version `3.5.0`: various option display bugs in `select` and `select_multiple`. Resolved in `3.5.4`
## Awesome projects using `beaupy`
- [therealOri/Genter](https://github.com/therealOri/Genter): A strong password generator and built in password manager made with python3!
- [therealOri/byte](https://github.com/therealOri/byte): Steganography Image/Data Injector. For artists or people to inject their own Datamark "Watermark" into their images/art or files!
- [AnirudhG07/ntfyme](https://github.com/AnirudhG07/ntfyme): A notification tool to notify you about status of your long running processes on termination via local-notification, Gmail, Telergram, etc.
## License
The project is licensed under the [MIT License](https://raw.githubusercontent.com/petereon/beaupy/master/LICENSE).
Raw data
{
"_id": null,
"home_page": "https://github.com/petereon/beaupy",
"name": "beaupy",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0.0,>=3.7.8",
"maintainer_email": null,
"keywords": "cli, interactive, console, terminal, interface",
"author": "Peter Vyboch",
"author_email": "pvyboch1@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/83/0d/8f8a9c2e48eb02e2325a11538a22b70d86f22f2a27110502b180375b45e4/beaupy-3.9.2.tar.gz",
"platform": null,
"description": "# BeauPy\n\n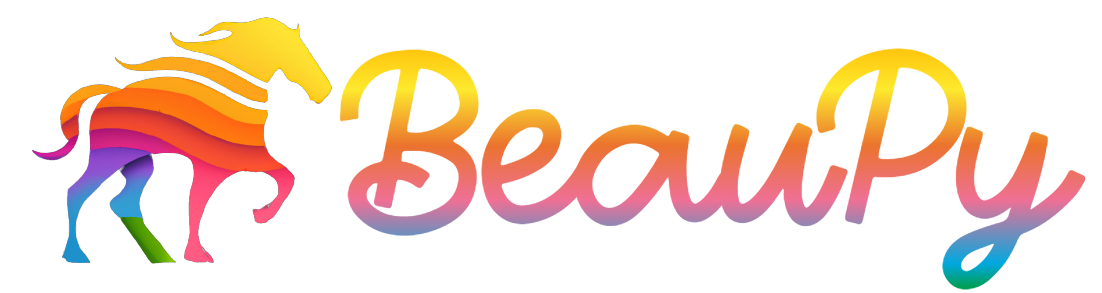\n\n> A Python library of interactive CLI elements you have been looking for\n\n---\n\n[](https://github.com/petereon/beaupy/actions/workflows/python-test.yml)\n[](https://github.com/petereon/beaupy/actions/workflows/python-lint.yml)\n[](https://codecov.io/gh/petereon/beaupy)\n[](https://sonarcloud.io/summary/new_code?id=petereon_beaupy)\n[](https://sonarcloud.io/summary/new_code?id=petereon_beaupy)\n\n\n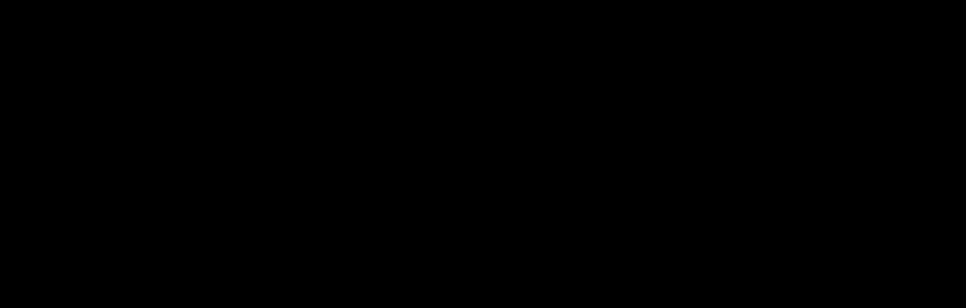\n\nFor documentation but more and prettier see [**here**](https://petereon.github.io/beaupy/)\n\n## Acknowledgment\n\nBeauPy stands on the shoulders of giants. It is based on another library with which it shares some of the source code, [`cutie`](https://github.com/kamik423/cutie), developed by [Kamik423](https://github.com/Kamik423). It has begun as a fork but has since diverged into its own thing and as such, detached from the original repository.\n\n## Overview\n\n**BeauPy** implements a number of common interactive elements:\n\n| Function | Functionality |\n|:---------------------------------------------------------------------------------|:-------------------------------------------------------------------------------------------|\n| [`select`](https://petereon.github.io/beaupy/api/#select) | Prompt to pick a choice from a list |\n| [`select_multiple`](https://petereon.github.io/beaupy/api/#select_multiple) | Prompt to select one or multiple choices from a list |\n| [`confirm`](https://petereon.github.io/beaupy/api/#confirm) | Prompt with a question and yes/no options |\n| [`prompt`](https://petereon.github.io/beaupy/api/#prompt) | Prompt that takes free input with optional validation, type conversion and input hiding |\n\nTUI elements shown in the above gif are the result of the following code:\n\n```python\nimport time\nfrom beaupy import confirm, prompt, select, select_multiple\nfrom beaupy.spinners import *\nfrom rich.console import Console\n\nconsole = Console()\n\n# Confirm a dialog\nif confirm(\"Will you take the ring to Mordor?\"):\n names = [\n \"Frodo Baggins\",\n \"Samwise Gamgee\",\n \"Legolas\",\n \"Aragorn\",\n \"[red]Sauron[/red]\",\n ]\n console.print(\"Who are you?\")\n # Choose one item from a list\n name = select(names, cursor=\"\ud83e\udca7\", cursor_style=\"cyan\")\n console.print(f\"Al\u00e1men\u00eb, {name}\")\n\n\n item_options = [\n \"The One Ring\",\n \"Dagger\",\n \"Po-tae-toes\",\n \"Lightsaber (Wrong franchise! Nevermind, roll with it!)\",\n ]\n console.print(\"What do you bring with you?\")\n # Choose multiple options from a list\n items = select_multiple(item_options, tick_character='\ud83c\udf92', ticked_indices=[0], maximal_count=3)\n\n potato_count = 0\n if \"Po-tae-toes\" in items:\n # Prompt with type conversion and validation\n potato_count = prompt('How many potatoes?', target_type=int, validator=lambda count: count > 0)\n\n # Spinner to show while doing some work\n spinner = Spinner(DOTS, \"Packing things...\")\n spinner.start()\n\n time.sleep(2)\n\n spinner.stop()\n # Get input without showing it being typed\n if \"friend\" == prompt(\"Speak, [blue bold underline]friend[/blue bold underline], and enter\", secure=True).lower():\n\n # Custom spinner animation\n spinner_animation = ['\u2589\u2589', '\u258c\u2590', ' ', '\u258c\u2590', '\u2589\u2589']\n spinner = Spinner(spinner_animation, \"Opening the Door of Durin...\")\n spinner.start()\n\n time.sleep(2)\n\n spinner.stop()\n else:\n spinner_animation = ['\ud83d\udc19\ud83c\udf0a \u2694\ufe0f ', '\ud83d\udc19 \ud83c\udf0a \u2694\ufe0f ', '\ud83d\udc19 \ud83c\udf0a \u2694\ufe0f ', '\ud83d\udc19 \ud83c\udf0a \u2694\ufe0f ', '\ud83d\udc19 \ud83c\udf0a\u2694\ufe0f ']\n spinner = Spinner(spinner_animation, \"Getting attacked by an octopus...\")\n spinner.start()\n\n time.sleep(2)\n\n spinner.stop()\n\n if 'The One Ring' in items:\n console.print(\"[green]You throw The One Ring to a lava from an eagle![/green]\")\n else:\n console.print(\"[red]You forgot the ring and brought Middle-Earth to its knees![/red]\")\n console.print(f\"And you brought {potato_count} taters!\")\n```\n\nFor more information refer to [more examples](https://petereon.github.io/beaupy/examples/) or definitive, but much less exciting [API documentation](https://petereon.github.io/beaupy/api/)\n\n## Installation\n\nFrom PyPI:\n\n```sh\npip install beaupy\n```\n\nFrom source:\n\n```sh\ngit clone https://github.com/petereon/beaupy.git\npoetry build\npip install ./dist/beaupy-{{some-version}}-py3-none-any.whl\n```\n\n## Roadmap\n\nThis repository has a [associated GitHub project](https://github.com/users/petereon/projects/3/views/1) where work that is currently done can be seen.\n\n## Contributing\n\nIf you would like to contribute, please feel free to suggest features or implement them yourself.\n\nAlso **please report any issues and bugs you might find!**\n\n### Development\n\nTo start development you can clone the repository:\n\n```sh\ngit clone https://github.com/petereon/beaupy.git\n```\n\nChange the directory to the project directory:\n\n```sh\ncd ./beaupy/\n```\n\nThis project uses [`poetry`](https://python-poetry.org/) as a dependency manager. You can install the dependencies using:\n\n```sh\npoetry install\n```\n\nFor testing, this project relies on [`ward`](https://github.com/darrenburns/ward). It is included as a development dependency, so\nafter installing the dependencies you can simply execute the following:\n\n```sh\npoetry run poe test\n```\n\nMaking sure the code follows quality standards and formatting can be ensured by executing\n\n```sh\npoetry run poe lint\n```\n\nYou can also have the tests and lints run after every saved change by executing a respective watch command\n\n```sh\npoetry run poe test:watch\n```\n\nor\n\n```sh\npoetry run poe lint:watch\n```\n\nAfter you have made your changes, create a pull request towards a master branch of this repository\n\nLooking forward to your pull requests!\n\n## Compatibility\n\nInternal logic of `beaupy` is supported for all the major platforms (Windows, Linux, macOS).\n\n- For user input from console, `beaupy` relies on [petereon/yakh](https://github.com/petereon/yakh), which is tested against all the major platforms and Python versions.\n- For printing to console `beaupy` relies on [Textualize/rich](https://github.com/Textualize/rich), which [claims to support](https://github.com/Textualize/rich#compatibility) all the major platforms.\n\n## Known Issues\n\n- Version `2.x.x`: arrow keys reportedly don't always work on Windows. Resolved in `3.x.x`\n- Version `3.5.0`: various option display bugs in `select` and `select_multiple`. Resolved in `3.5.4`\n\n## Awesome projects using `beaupy`\n\n- [therealOri/Genter](https://github.com/therealOri/Genter): A strong password generator and built in password manager made with python3!\n- [therealOri/byte](https://github.com/therealOri/byte): Steganography Image/Data Injector. For artists or people to inject their own Datamark \"Watermark\" into their images/art or files!\n- [AnirudhG07/ntfyme](https://github.com/AnirudhG07/ntfyme): A notification tool to notify you about status of your long running processes on termination via local-notification, Gmail, Telergram, etc.\n\n## License\n\nThe project is licensed under the [MIT License](https://raw.githubusercontent.com/petereon/beaupy/master/LICENSE).\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A library of elements for interactive TUIs in Python",
"version": "3.9.2",
"project_urls": {
"Homepage": "https://github.com/petereon/beaupy",
"Repository": "https://github.com/petereon/beaupy"
},
"split_keywords": [
"cli",
" interactive",
" console",
" terminal",
" interface"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "c769e2dc735c38de6524d9c7dad4056e5093adf35a6c4c8478007b2fce5470e0",
"md5": "f3b098d6a7466ef885a9d35789bc2e88",
"sha256": "b1e2d20281c58ac629097aab99464cd6951855e34f408a983dfa103bbd3cc08a"
},
"downloads": -1,
"filename": "beaupy-3.9.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "f3b098d6a7466ef885a9d35789bc2e88",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0.0,>=3.7.8",
"size": 14509,
"upload_time": "2024-08-23T10:59:37",
"upload_time_iso_8601": "2024-08-23T10:59:37.500574Z",
"url": "https://files.pythonhosted.org/packages/c7/69/e2dc735c38de6524d9c7dad4056e5093adf35a6c4c8478007b2fce5470e0/beaupy-3.9.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "830d8f8a9c2e48eb02e2325a11538a22b70d86f22f2a27110502b180375b45e4",
"md5": "354c52dc99e6b48afc8436cd78bd10f4",
"sha256": "b71579ba626d29ceddae96575d2de9a9508a194e1058d2095627116f349ad780"
},
"downloads": -1,
"filename": "beaupy-3.9.2.tar.gz",
"has_sig": false,
"md5_digest": "354c52dc99e6b48afc8436cd78bd10f4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0.0,>=3.7.8",
"size": 16714,
"upload_time": "2024-08-23T10:59:38",
"upload_time_iso_8601": "2024-08-23T10:59:38.463793Z",
"url": "https://files.pythonhosted.org/packages/83/0d/8f8a9c2e48eb02e2325a11538a22b70d86f22f2a27110502b180375b45e4/beaupy-3.9.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-23 10:59:38",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "petereon",
"github_project": "beaupy",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "beaupy"
}