[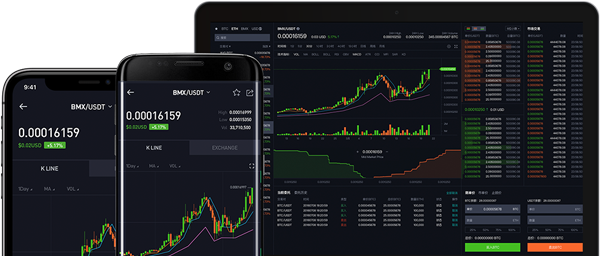](https://bitmart.com)
BitMart-Python-SDK-API
=========================
[](https://pypi.python.org/pypi/bitmart-python-sdk-api)
[](https://www.python.org/downloads/)
[](https://opensource.org/licenses/MIT)
[](https://t.me/bitmart_api)
[BitMart Exchange official](https://bitmart.com) Python client for the BitMart Cloud API.
Feature
=========================
- Provides exchange quick trading API
- Easier withdrawal
- Efficiency, higher speeds, and lower latencies
- Priority in development and maintenance
- Dedicated and responsive technical support
- Supported APIs:
- `/spot/*`
- `/contract/*`
- `/account/*`
- Supported websockets:
- Spot WebSocket Market Stream
- Spot User Data Stream
- futures User Data Stream
- futures WebSocket Market Stream
- Test cases and examples
Installation
=========================
```bash
pip install bitmart-python-sdk-api
```
Documentation
=========================
[API Documentation](https://developer-pro.bitmart.com/en/spot/#change-log)
Example
=========================
#### Spot Public API Example
```python
from bitmart.api_spot import APISpot
if __name__ == '__main__':
spotAPI = APISpot(timeout=(2, 10))
# Get a list of all cryptocurrencies on the platform
response = spotAPI.get_currencies()
print("response:", response[0])
# Querying aggregated tickers of a particular trading pair
response = spotAPI.get_v3_ticker(symbol='BTC_USDT')
print("response:", response[0])
# Get the quotations of all trading pairs
response = spotAPI.get_v3_tickers()
print("response:", response[0])
# Get full depth of trading pairs
response = spotAPI.get_v3_depth(symbol='BTC_USDT')
print("response:", response[0])
# Get the latest trade records of the specified trading pair
response = spotAPI.get_v3_trades(symbol='BTC_USDT', limit=10)
print("response:", response[0])
```
#### Spot Trade API Example
```python
from bitmart.api_spot import APISpot
from bitmart.lib import cloud_exceptions
if __name__ == '__main__':
api_key = "Your API KEY"
secret_key = "Your Secret KEY"
memo = "Your Memo"
try:
spotAPI = APISpot(api_key, secret_key, memo, timeout=(3, 10))
response = spotAPI.post_submit_order(
symbol='BTC_USDT',
side='sell',
type='limit',
size='10000',
price='1000000'
)
except cloud_exceptions.APIException as apiException:
print("Error[HTTP<>200]:", apiException.response)
except Exception as exception:
print("Error[Exception]:", exception)
else:
if response[0]['code'] == 1000:
print('Call Success:', response[0])
else:
print('Call Failed:', response[0]['message'])
```
Please find [examples/spot](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/spot) folder to check for more endpoints.
#### Spot WebSocket Public Channel Example
```python
import logging
import time
from bitmart.lib.cloud_consts import SPOT_PUBLIC_WS_URL
from bitmart.lib.cloud_utils import config_logging
from bitmart.websocket.spot_socket_client import SpotSocketClient
def message_handler(message):
logging.info(f"message_handler: {message}")
if __name__ == '__main__':
config_logging(logging, logging.INFO)
my_client = SpotSocketClient(stream_url=SPOT_PUBLIC_WS_URL,
on_message=message_handler)
# Subscribe to a single symbol stream
my_client.subscribe(args="spot/ticker:BMX_USDT")
# Subscribe to multiple symbol streams
my_client.subscribe(args=["spot/ticker:BMX_USDT", "spot/ticker:BTC_USDT"])
# Send the original subscription message
my_client.send({"op": "subscribe", "args": ["spot/ticker:ETH_USDT"]})
time.sleep(5)
# Unsubscribe
my_client.unsubscribe(args="spot/ticker:BMX_USDT")
my_client.send({"op": "unsubscribe", "args": ["spot/ticker:BMX_USDT"]})
```
#### Spot WebSocket Private Channel Example
```python
import logging
from bitmart.lib.cloud_consts import SPOT_PRIVATE_WS_URL
from bitmart.lib.cloud_utils import config_logging
from bitmart.websocket.spot_socket_client import SpotSocketClient
def message_handler(message):
logging.info(f"message_handler: {message}")
if __name__ == '__main__':
config_logging(logging, logging.INFO)
my_client = SpotSocketClient(stream_url=SPOT_PRIVATE_WS_URL,
on_message=message_handler,
api_key="your_api_key",
api_secret_key="your_secret_key",
api_memo="your_api_memo")
# Login
my_client.login()
# Subscribe to a single symbol stream
my_client.subscribe(args="spot/user/balance:BALANCE_UPDATE")
# Stop
# my_client.stop()
```
Please find [examples/websocket/spot/websocket_stream](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/websocket/spot/websocket_stream) folder to check for more endpoints.
#### Futures Public API Example
```python
from bitmart.api_contract import APIContract
if __name__ == '__main__':
contractAPI = APIContract(timeout=(2, 10))
# query contract details
response = contractAPI.get_details(contract_symbol='ETHUSDT')
print("response:", response[0])
# Get full depth of trading pairs.
response = contractAPI.get_depth(contract_symbol='ETHUSDT')
print("response:", response[0])
# Querying the open interest and open interest value data of the specified contract
response = contractAPI.get_open_interest(contract_symbol='ETHUSDT')
print("response:", response[0])
# Applicable for checking the current funding rate of a specified contract
response = contractAPI.get_funding_rate(contract_symbol='ETHUSDT')
print("response:", response[0])
# querying K-line data
response = contractAPI.get_kline(contract_symbol='ETHUSDT', step=5, start_time=1662518172, end_time=1662518172)
print("response:", response[0])
```
#### Futures Trade API Example
```python
from bitmart.api_contract import APIContract
from bitmart.lib import cloud_exceptions
if __name__ == '__main__':
api_key = "Your API KEY"
secret_key = "Your Secret KEY"
memo = "Your Memo"
contractAPI = APIContract(api_key, secret_key, memo, timeout=(3, 10))
try:
response = contractAPI.post_submit_order(contract_symbol='BTCUSDT',
client_order_id="BM1234",
side=4,
mode=1,
type='limit',
leverage='1',
open_type='isolated',
size=10,
price='20000')
except cloud_exceptions.APIException as apiException:
print("Error[HTTP<>200]:", apiException.response)
except Exception as exception:
print("Error[Exception]:", exception)
else:
if response[0]['code'] == 1000:
print('Call Success:', response[0])
else:
print('Call Failed:', response[0]['message'])
```
Please find [examples/futures](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/futures) folder to check for more endpoints.
#### Futures WebSocket Public Channel Example
```python
import logging
import time
from bitmart.lib.cloud_consts import FUTURES_PUBLIC_WS_URL
from bitmart.lib.cloud_utils import config_logging
from bitmart.websocket.futures_socket_client import FuturesSocketClient
def message_handler(message):
logging.info(f"message_handler: {message}")
if __name__ == '__main__':
config_logging(logging, logging.INFO)
my_client = FuturesSocketClient(stream_url=FUTURES_PUBLIC_WS_URL,
on_message=message_handler)
# Example 1:
# Subscribe to a single symbol stream
my_client.subscribe(args="futures/ticker")
time.sleep(2)
# Unsubscribe
my_client.unsubscribe(args="futures/ticker")
time.sleep(5)
# Example 2:
# Send the original subscription message
my_client.send({"action": "subscribe", "args": ["futures/ticker"]})
time.sleep(2)
# Unsubscribe
my_client.send({"action": "unsubscribe", "args": ["futures/ticker"]})
# Stop
# my_client.stop()
```
#### Futures WebSocket Private Channel Example
```python
import logging
from bitmart.lib.cloud_consts import FUTURES_PRIVATE_WS_URL
from bitmart.lib.cloud_utils import config_logging
from bitmart.websocket.futures_socket_client import FuturesSocketClient
def message_handler(message):
logging.info(f"message_handler: {message}")
if __name__ == '__main__':
config_logging(logging, logging.INFO)
my_client = FuturesSocketClient(stream_url=FUTURES_PRIVATE_WS_URL,
on_message=message_handler,
api_key="your_api_key",
api_secret_key="your_secret_key",
api_memo="your_api_memo")
# Login
my_client.login()
# Subscribe to a single symbol stream
my_client.subscribe(args="futures/asset:USDT")
# Subscribe to multiple symbol streams
my_client.subscribe(args=["futures/asset:BTC", "futures/asset:ETH"])
# Stop
# my_client.stop()
```
Please find [examples/websocket/futures/websocket_stream](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/websocket/futures/websocket_stream) folder to check for more endpoints.
Extra Options
=========================
### Authentication
How to set API KEY?
```python
from bitmart.api_spot import APISpot
from bitmart.api_contract import APIContract
spotAPI = APISpot(api_key="your api access key", secret_key="your api secret key", memo="your api memo")
contractAPI = APIContract(api_key="your api access key", secret_key="your api secret key", memo="your api memo")
```
### Timeout
Set HTTP `connection timeout` and `read timeout`.
```python
from bitmart.api_spot import APISpot
from bitmart.api_contract import APIContract
spotAPI = APISpot(timeout=(2, 10))
contractAPI = APIContract(timeout=(2, 10))
```
### Logging
If you want to `debug` the data requested by the API and the corresponding data returned by the API,
you can set it like this:
```python
import logging
from bitmart.api_spot import APISpot
from bitmart.lib.cloud_utils import config_logging
config_logging(logging, logging.DEBUG)
logger = logging.getLogger(__name__)
spotAPI = APISpot(logger=logger)
```
### Domain
How to set API domain name? The domain name parameter is optional,
the default domain name is `https://api-cloud.bitmart.com`.
```python
from bitmart.api_spot import APISpot
from bitmart.api_contract import APIContract
spotAPI = APISpot(url='https://api-cloud.bitmart.com')
contractAPI = APIContract(url='https://api-cloud.bitmart.com')
```
### Custom request headers
You can add your own request header information here, but please do not fill in `X-BM-KEY, X-BM-SIGN, X-BM-TIMESTAMP`
```python
from bitmart.api_spot import APISpot
spotAPI = APISpot(headers={'Your-Custom-Header': 'xxxxxxx'})
```
### Response Metadata
The bitmart API server provides the endpoint rate limit usage in the header of each response.
This information can be obtained from the headers property.
`x-bm-ratelimit-remaining` indicates the number of times the current window has been used,
`x-bm-ratelimit-limit` indicates the maximum number of times the current window can be used,
and `x-bm-ratelimit-reset` indicates the current window time.
##### Example:
```
x-bm-ratelimit-mode: IP
x-bm-ratelimit-remaining: 10
x-bm-ratelimit-limit: 600
x-bm-ratelimit-reset: 60
```
This means that this IP can call the endpoint 600 times within 60 seconds, and has called 10 times so far.
```python
import logging
from bitmart.api_spot import APISpot
logger = logging.getLogger(__name__)
spotAPI = APISpot()
response = spotAPI.get_currencies()[0]
limit = spotAPI.get_currencies()[1]
logger.info(f"x-bm-ratelimit-remaining={limit['Remaining']},"
f"x-bm-ratelimit-limit={limit['Limit']},"
f"x-bm-ratelimit-reset={limit['Reset']},"
f"x-bm-ratelimit-mode={limit['Mode']}")
```
Raw data
{
"_id": null,
"home_page": "https://github.com/bitmartexchange/bitmart-python-sdk-api",
"name": "bitmart-python-sdk-api",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": null,
"keywords": "BitMart Exchange, BitMart API, BitMart Websocket",
"author": null,
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/33/2a/7c8bc558f2721013310aea1886110efb9608b0d8875fa75aa80e109a3e2c/bitmart-python-sdk-api-2.3.1.tar.gz",
"platform": null,
"description": "[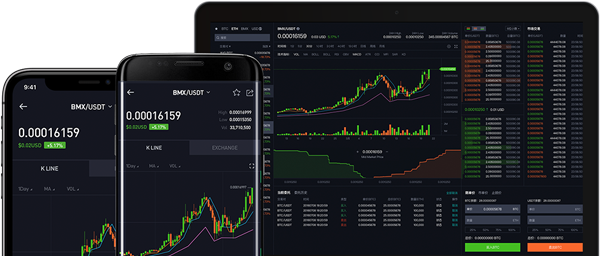](https://bitmart.com)\n\nBitMart-Python-SDK-API\n=========================\n[](https://pypi.python.org/pypi/bitmart-python-sdk-api)\n[](https://www.python.org/downloads/)\n[](https://opensource.org/licenses/MIT)\n[](https://t.me/bitmart_api)\n\n\n[BitMart Exchange official](https://bitmart.com) Python client for the BitMart Cloud API.\n\n\n\nFeature\n=========================\n- Provides exchange quick trading API\n- Easier withdrawal\n- Efficiency, higher speeds, and lower latencies\n- Priority in development and maintenance\n- Dedicated and responsive technical support\n- Supported APIs:\n - `/spot/*`\n - `/contract/*`\n - `/account/*`\n- Supported websockets:\n - Spot WebSocket Market Stream\n - Spot User Data Stream\n - futures User Data Stream\n - futures WebSocket Market Stream\n- Test cases and examples\n\n\n\nInstallation\n=========================\n```bash\npip install bitmart-python-sdk-api\n```\n\nDocumentation\n=========================\n[API Documentation](https://developer-pro.bitmart.com/en/spot/#change-log)\n\n\nExample\n=========================\n\n#### Spot Public API Example\n```python\nfrom bitmart.api_spot import APISpot\n\nif __name__ == '__main__':\n spotAPI = APISpot(timeout=(2, 10))\n\n # Get a list of all cryptocurrencies on the platform\n response = spotAPI.get_currencies()\n print(\"response:\", response[0])\n\n # Querying aggregated tickers of a particular trading pair\n response = spotAPI.get_v3_ticker(symbol='BTC_USDT')\n print(\"response:\", response[0])\n\n # Get the quotations of all trading pairs\n response = spotAPI.get_v3_tickers()\n print(\"response:\", response[0])\n\n # Get full depth of trading pairs\n response = spotAPI.get_v3_depth(symbol='BTC_USDT')\n print(\"response:\", response[0])\n\n # Get the latest trade records of the specified trading pair\n response = spotAPI.get_v3_trades(symbol='BTC_USDT', limit=10)\n print(\"response:\", response[0])\n```\n\n\n#### Spot Trade API Example\n\n```python\nfrom bitmart.api_spot import APISpot\nfrom bitmart.lib import cloud_exceptions\n\nif __name__ == '__main__':\n\n api_key = \"Your API KEY\"\n secret_key = \"Your Secret KEY\"\n memo = \"Your Memo\"\n\n try:\n spotAPI = APISpot(api_key, secret_key, memo, timeout=(3, 10))\n\n response = spotAPI.post_submit_order(\n symbol='BTC_USDT',\n side='sell',\n type='limit',\n size='10000',\n price='1000000'\n )\n\n except cloud_exceptions.APIException as apiException:\n print(\"Error[HTTP<>200]:\", apiException.response)\n except Exception as exception:\n print(\"Error[Exception]:\", exception)\n else:\n if response[0]['code'] == 1000:\n print('Call Success:', response[0])\n else:\n print('Call Failed:', response[0]['message'])\n \n```\n\n\nPlease find [examples/spot](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/spot) folder to check for more endpoints.\n\n\n#### Spot WebSocket Public Channel Example\n\n```python\n\nimport logging\nimport time\n\nfrom bitmart.lib.cloud_consts import SPOT_PUBLIC_WS_URL\nfrom bitmart.lib.cloud_utils import config_logging\nfrom bitmart.websocket.spot_socket_client import SpotSocketClient\n\ndef message_handler(message):\n logging.info(f\"message_handler: {message}\")\n \nif __name__ == '__main__':\n config_logging(logging, logging.INFO)\n \n \n my_client = SpotSocketClient(stream_url=SPOT_PUBLIC_WS_URL,\n on_message=message_handler)\n \n # Subscribe to a single symbol stream\n my_client.subscribe(args=\"spot/ticker:BMX_USDT\")\n \n # Subscribe to multiple symbol streams\n my_client.subscribe(args=[\"spot/ticker:BMX_USDT\", \"spot/ticker:BTC_USDT\"])\n \n # Send the original subscription message\n my_client.send({\"op\": \"subscribe\", \"args\": [\"spot/ticker:ETH_USDT\"]})\n \n time.sleep(5)\n \n # Unsubscribe\n my_client.unsubscribe(args=\"spot/ticker:BMX_USDT\")\n \n my_client.send({\"op\": \"unsubscribe\", \"args\": [\"spot/ticker:BMX_USDT\"]})\n\n\n```\n\n\n#### Spot WebSocket Private Channel Example\n\n```python\n\nimport logging\n\nfrom bitmart.lib.cloud_consts import SPOT_PRIVATE_WS_URL\nfrom bitmart.lib.cloud_utils import config_logging\nfrom bitmart.websocket.spot_socket_client import SpotSocketClient\n\ndef message_handler(message):\n logging.info(f\"message_handler: {message}\")\n \nif __name__ == '__main__':\n config_logging(logging, logging.INFO)\n\n my_client = SpotSocketClient(stream_url=SPOT_PRIVATE_WS_URL,\n on_message=message_handler,\n api_key=\"your_api_key\",\n api_secret_key=\"your_secret_key\",\n api_memo=\"your_api_memo\")\n\n # Login\n my_client.login()\n\n # Subscribe to a single symbol stream\n my_client.subscribe(args=\"spot/user/balance:BALANCE_UPDATE\")\n\n # Stop\n # my_client.stop()\n\n```\n\nPlease find [examples/websocket/spot/websocket_stream](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/websocket/spot/websocket_stream) folder to check for more endpoints.\n\n\n#### Futures Public API Example\n```python\nfrom bitmart.api_contract import APIContract\n\nif __name__ == '__main__':\n contractAPI = APIContract(timeout=(2, 10))\n\n # query contract details\n response = contractAPI.get_details(contract_symbol='ETHUSDT')\n print(\"response:\", response[0])\n\n # Get full depth of trading pairs.\n response = contractAPI.get_depth(contract_symbol='ETHUSDT')\n print(\"response:\", response[0])\n\n # Querying the open interest and open interest value data of the specified contract\n response = contractAPI.get_open_interest(contract_symbol='ETHUSDT')\n print(\"response:\", response[0])\n\n # Applicable for checking the current funding rate of a specified contract\n response = contractAPI.get_funding_rate(contract_symbol='ETHUSDT')\n print(\"response:\", response[0])\n\n # querying K-line data\n response = contractAPI.get_kline(contract_symbol='ETHUSDT', step=5, start_time=1662518172, end_time=1662518172)\n print(\"response:\", response[0])\n```\n\n\n#### Futures Trade API Example\n```python\nfrom bitmart.api_contract import APIContract\nfrom bitmart.lib import cloud_exceptions\n\nif __name__ == '__main__':\n\n api_key = \"Your API KEY\"\n secret_key = \"Your Secret KEY\"\n memo = \"Your Memo\"\n\n contractAPI = APIContract(api_key, secret_key, memo, timeout=(3, 10))\n\n try:\n response = contractAPI.post_submit_order(contract_symbol='BTCUSDT',\n client_order_id=\"BM1234\",\n side=4,\n mode=1,\n type='limit',\n leverage='1',\n open_type='isolated',\n size=10,\n price='20000')\n except cloud_exceptions.APIException as apiException:\n print(\"Error[HTTP<>200]:\", apiException.response)\n except Exception as exception:\n print(\"Error[Exception]:\", exception)\n else:\n if response[0]['code'] == 1000:\n print('Call Success:', response[0])\n else:\n print('Call Failed:', response[0]['message'])\n\n```\n\nPlease find [examples/futures](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/futures) folder to check for more endpoints.\n\n\n#### Futures WebSocket Public Channel Example\n\n```python\n\nimport logging\nimport time\n\nfrom bitmart.lib.cloud_consts import FUTURES_PUBLIC_WS_URL\nfrom bitmart.lib.cloud_utils import config_logging\nfrom bitmart.websocket.futures_socket_client import FuturesSocketClient\n\ndef message_handler(message):\n logging.info(f\"message_handler: {message}\")\n \n \n\nif __name__ == '__main__':\n config_logging(logging, logging.INFO)\n \n my_client = FuturesSocketClient(stream_url=FUTURES_PUBLIC_WS_URL,\n on_message=message_handler)\n\n # Example 1:\n # Subscribe to a single symbol stream\n my_client.subscribe(args=\"futures/ticker\")\n\n time.sleep(2)\n\n # Unsubscribe\n my_client.unsubscribe(args=\"futures/ticker\")\n\n time.sleep(5)\n # Example 2:\n # Send the original subscription message\n my_client.send({\"action\": \"subscribe\", \"args\": [\"futures/ticker\"]})\n\n time.sleep(2)\n\n # Unsubscribe\n my_client.send({\"action\": \"unsubscribe\", \"args\": [\"futures/ticker\"]})\n\n # Stop\n # my_client.stop()\n\n\n```\n\n\n#### Futures WebSocket Private Channel Example\n\n```python\n\nimport logging\n\nfrom bitmart.lib.cloud_consts import FUTURES_PRIVATE_WS_URL\nfrom bitmart.lib.cloud_utils import config_logging\nfrom bitmart.websocket.futures_socket_client import FuturesSocketClient\n\ndef message_handler(message):\n logging.info(f\"message_handler: {message}\")\n\n\nif __name__ == '__main__':\n config_logging(logging, logging.INFO)\n \n my_client = FuturesSocketClient(stream_url=FUTURES_PRIVATE_WS_URL,\n on_message=message_handler,\n api_key=\"your_api_key\",\n api_secret_key=\"your_secret_key\",\n api_memo=\"your_api_memo\")\n\n # Login\n my_client.login()\n\n # Subscribe to a single symbol stream\n my_client.subscribe(args=\"futures/asset:USDT\")\n\n # Subscribe to multiple symbol streams\n my_client.subscribe(args=[\"futures/asset:BTC\", \"futures/asset:ETH\"])\n\n # Stop\n # my_client.stop()\n```\n\nPlease find [examples/websocket/futures/websocket_stream](https://github.com/bitmartexchange/bitmart-python-sdk-api/tree/master/examples/websocket/futures/websocket_stream) folder to check for more endpoints.\n\n\nExtra Options\n=========================\n\n### Authentication\nHow to set API KEY?\n\n```python\nfrom bitmart.api_spot import APISpot\nfrom bitmart.api_contract import APIContract\n\nspotAPI = APISpot(api_key=\"your api access key\", secret_key=\"your api secret key\", memo=\"your api memo\")\ncontractAPI = APIContract(api_key=\"your api access key\", secret_key=\"your api secret key\", memo=\"your api memo\")\n```\n\n### Timeout\nSet HTTP `connection timeout` and `read timeout`.\n\n```python\nfrom bitmart.api_spot import APISpot\nfrom bitmart.api_contract import APIContract\nspotAPI = APISpot(timeout=(2, 10))\ncontractAPI = APIContract(timeout=(2, 10))\n```\n\n### Logging\nIf you want to `debug` the data requested by the API and the corresponding data returned by the API,\nyou can set it like this:\n\n```python\nimport logging\nfrom bitmart.api_spot import APISpot\nfrom bitmart.lib.cloud_utils import config_logging\n\nconfig_logging(logging, logging.DEBUG)\nlogger = logging.getLogger(__name__)\n\nspotAPI = APISpot(logger=logger)\n```\n\n\n\n### Domain\nHow to set API domain name? The domain name parameter is optional,\nthe default domain name is `https://api-cloud.bitmart.com`.\n\n```python\nfrom bitmart.api_spot import APISpot\nfrom bitmart.api_contract import APIContract\nspotAPI = APISpot(url='https://api-cloud.bitmart.com')\ncontractAPI = APIContract(url='https://api-cloud.bitmart.com')\n```\n\n\n### Custom request headers\nYou can add your own request header information here, but please do not fill in `X-BM-KEY, X-BM-SIGN, X-BM-TIMESTAMP`\n\n```python\nfrom bitmart.api_spot import APISpot\nspotAPI = APISpot(headers={'Your-Custom-Header': 'xxxxxxx'})\n```\n\n\n### Response Metadata\n\nThe bitmart API server provides the endpoint rate limit usage in the header of each response. \nThis information can be obtained from the headers property. \n`x-bm-ratelimit-remaining` indicates the number of times the current window has been used, \n`x-bm-ratelimit-limit` indicates the maximum number of times the current window can be used, \nand `x-bm-ratelimit-reset` indicates the current window time.\n\n\n##### Example:\n\n```\nx-bm-ratelimit-mode: IP\nx-bm-ratelimit-remaining: 10\nx-bm-ratelimit-limit: 600\nx-bm-ratelimit-reset: 60\n```\n\nThis means that this IP can call the endpoint 600 times within 60 seconds, and has called 10 times so far.\n\n\n```python\n\nimport logging\n\nfrom bitmart.api_spot import APISpot\nlogger = logging.getLogger(__name__)\nspotAPI = APISpot()\n\nresponse = spotAPI.get_currencies()[0]\nlimit = spotAPI.get_currencies()[1]\nlogger.info(f\"x-bm-ratelimit-remaining={limit['Remaining']},\"\n f\"x-bm-ratelimit-limit={limit['Limit']},\"\n f\"x-bm-ratelimit-reset={limit['Reset']},\"\n f\"x-bm-ratelimit-mode={limit['Mode']}\")\n\n```\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "BitMart Exchange official(https://bitmart.com) Python client for the BitMart Cloud API",
"version": "2.3.1",
"project_urls": {
"Homepage": "https://github.com/bitmartexchange/bitmart-python-sdk-api"
},
"split_keywords": [
"bitmart exchange",
" bitmart api",
" bitmart websocket"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "bca5d53e99091558e3db503c670923f7d6e080932edde61c98c2ffeaadde12b6",
"md5": "8c23f395e9d4be3eb7edafdcaf2558ef",
"sha256": "ccea8081b1848e1055083d0da82267b91846f49fcf63a1b497f2d8d868dff883"
},
"downloads": -1,
"filename": "bitmart_python_sdk_api-2.3.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "8c23f395e9d4be3eb7edafdcaf2558ef",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.7",
"size": 27803,
"upload_time": "2025-02-07T02:09:21",
"upload_time_iso_8601": "2025-02-07T02:09:21.861182Z",
"url": "https://files.pythonhosted.org/packages/bc/a5/d53e99091558e3db503c670923f7d6e080932edde61c98c2ffeaadde12b6/bitmart_python_sdk_api-2.3.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "332a7c8bc558f2721013310aea1886110efb9608b0d8875fa75aa80e109a3e2c",
"md5": "257a1cf67d606c55e4be5b251b2b15d4",
"sha256": "10cdadb67a219b87401de338a60e1e51a2bd5c5fb698a7a171fdb88211a4d2c1"
},
"downloads": -1,
"filename": "bitmart-python-sdk-api-2.3.1.tar.gz",
"has_sig": false,
"md5_digest": "257a1cf67d606c55e4be5b251b2b15d4",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 24268,
"upload_time": "2025-02-07T02:09:24",
"upload_time_iso_8601": "2025-02-07T02:09:24.294043Z",
"url": "https://files.pythonhosted.org/packages/33/2a/7c8bc558f2721013310aea1886110efb9608b0d8875fa75aa80e109a3e2c/bitmart-python-sdk-api-2.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-02-07 02:09:24",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "bitmartexchange",
"github_project": "bitmart-python-sdk-api",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "bitmart-python-sdk-api"
}