Name | brain-loop-search JSON |
Version |
0.1.7
JSON |
| download |
home_page | |
Summary | Screen loops among brain structures(or any entities comprising a graph). |
upload_time | 2023-07-27 11:10:15 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.10 |
license | MIT License |
keywords |
neuron-morphology
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# Brain Loop Search
Tools for screening significant loop structures in a graph, typically a
brain structure graph with physiological or anatomical edge data.
## Installation
```shell
$ pip install brain-loop-search
```
## Usage
### Packing a bigger graph for regions of interest
Packing vertices:
```python
import brain_loop_search as bls
vertices = [322, 329, 981, 337, 453, 8, 1070] # ccf brain id
# ccf ontology
ontology = bls.brain_utils.CCFv3Ontology()
vp = bls.packing.VertexPacker(vertices, ontology)
# filtering by level
vp.filter_by_level(fro=1, to=2)
```
Packing a graph:
```python
import brain_loop_search as bls
import pandas as pd
import numpy as np
vertices = [322, 329, 981, 337, 453, 1070, 345, 353, 361] # ccf brain id
# adjacent matrix
adj_mat = pd.DataFrame(np.array([
[0, 1, 1, 0, 0, 0, 1, 1, 0],
[0, 0, 1, 1, 1, 1, 0, 1, 0],
[0, 0, 0, 0, 1, 1, 1, 1, 1],
[0, 0, 1, 0, 0, 1, 0, 1, 0],
[1, 0, 1, 0, 0, 1, 0, 1, 0],
[0, 1, 1, 1, 1, 1, 0, 1, 0],
[0, 0, 0, 0, 0, 1, 0, 0, 1],
[1, 0, 0, 0, 0, 1, 1, 1, 0],
[1, 1, 1, 0, 0, 1, 0, 1, 1]
]), index=vertices, columns=vertices)
# ccf ontology
ontology = bls.brain_utils.CCFv3Ontology()
# packing
new_rows = [322]
new_cols = [337, 329, 353]
graph_packer = bls.packing.GraphPacker(adj_mat, ontology)
new_mat = graph_packer.pack(new_rows, new_cols, def_val=0, superior_as_complement=True, aggr_func=np.sum)
```
### Shortest Path Loop Search
Screen simple loops from the graph.
```python
import brain_loop_search as bls
import pandas as pd
edges = pd.DataFrame({
"a": [1, 2, 3, 4, 5],
"b": [4, -1, -1, 1, -1],
"c": [5, 6, 7, 8, 9],
"d": [-1, 2, 3, 1, 2],
"e": [-1, -1, -1, -1, -1]
}, index=["a", "b", "c", "d", "e"])
g = bls.search.ShortestPathLoopSearch()
g.add_subgraph(edges)
# search by single shortest path with a reverse edge
loops = g.pair_complement(axis_pool=['a', 'b', 'c'])
# search by chaining 3 of the shortest paths found
loops, sssp = g.chain_screen(n_axis=3)
```
### Max Flow Loop Search
Generate a new graph of potentially integrated loops.
```python
import brain_loop_search as bls
import pandas as pd
edges = pd.DataFrame({
"a": [1, 2, 3, 4, 5],
"b": [4, -1, -1, 1, -1],
"c": [5, 6, 7, 8, 9],
"d": [-1, 2, 3, 1, 2],
"e": [-1, -1, -1, -1, -1]
}, index=["a", "b", "c", "d", "e"])
g = bls.search.ShortestPathLoopSearch()
g.add_subgraph(edges)
# find a single max flow with a reverse edge (like a magnet field)
new_g = g.magnet_flow(s='b', t='a')
# find cycled max flows and merge them into a new graph
new_g = g.merged_cycle_flow(axes=['b', 'c', 'a'])
```
### Visualization
Visualize a single loop
```python
import brain_loop_search as bls
# a loop is a list of list, with the head and tail of the sublist as axes
# here are some random picked brain regions
loop = [[950, 974, 417], [417, 993], [993, 234, 289, 950]]
bls.brain_utils.draw_single_loop(loop, 'test.png')
```
Figure:
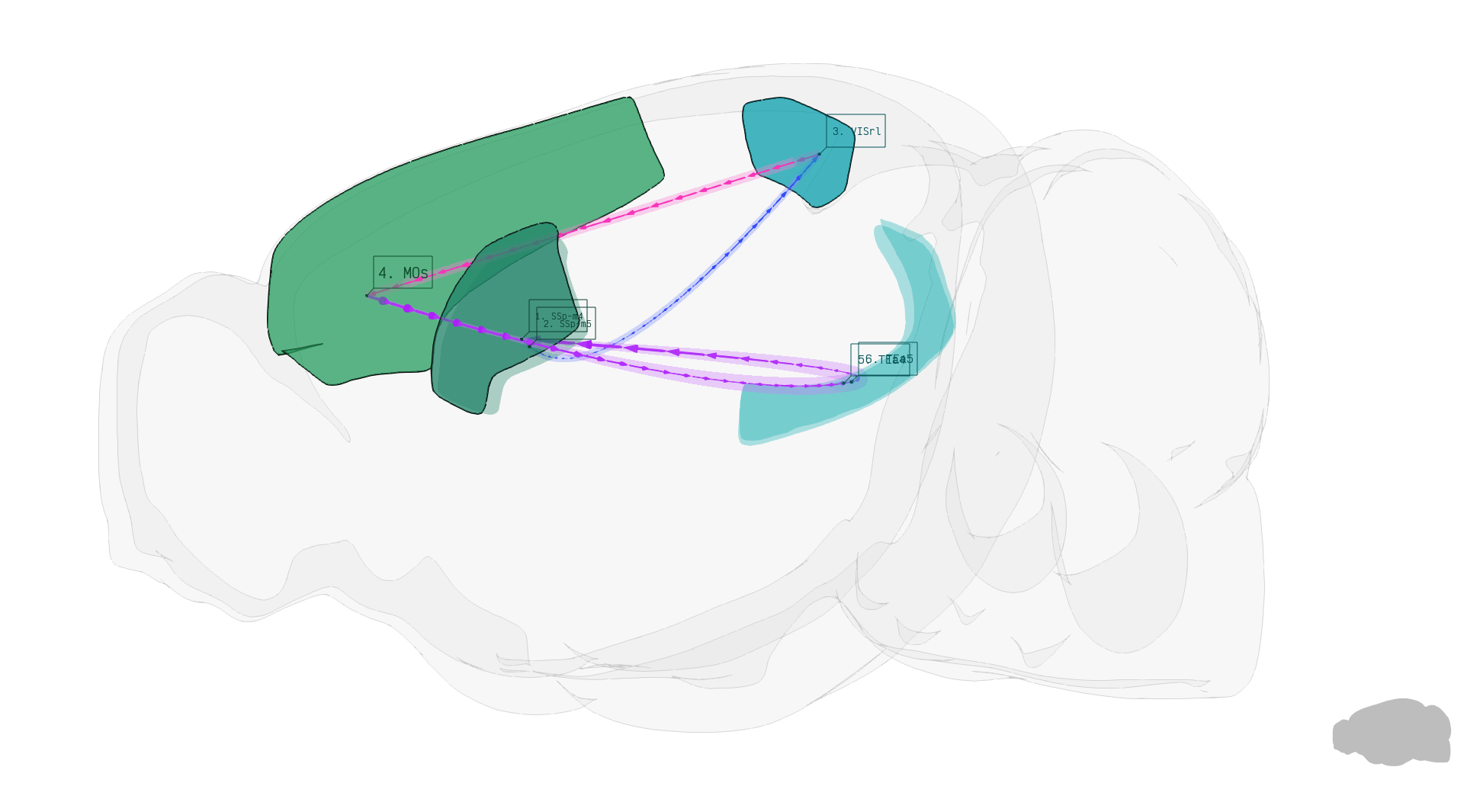
Visualize a graph
```python
import numpy as np
import pandas as pd
import brain_loop_search as bls
vertices = [322, 329, 981, 337, 453, 1070, 345, 353, 361]
adj_mat = pd.DataFrame(np.array([
[0, 2, 1, 0, 0, 0, 1, 8, 0],
[0, 0, 3, 1, 5, 1, 0, 5, 0],
[0, 0, 0, 0, 1, 3, 2, 1, 2],
[0, 0, 6, 0, 0, 1, 0, 4, 0],
[1, 0, 1, 0, 0, 1, 0, 4, 0],
[0, 1, 7, 1, 4, 1, 0, 1, 0],
[0, 0, 0, 0, 0, 1, 0, 0, 1],
[1, 0, 0, 0, 0, 2, 2, 1, 0],
[1, 2, 2, 0, 0, 1, 0, 1, 1]
]), index=vertices, columns=vertices)
g = bls.search.GraphMaintainer()
g.add_subgraph(adj_mat)
bls.brain_utils.draw_brain_graph(g.graph, 'test2.png', thr=3)
```
Figure:
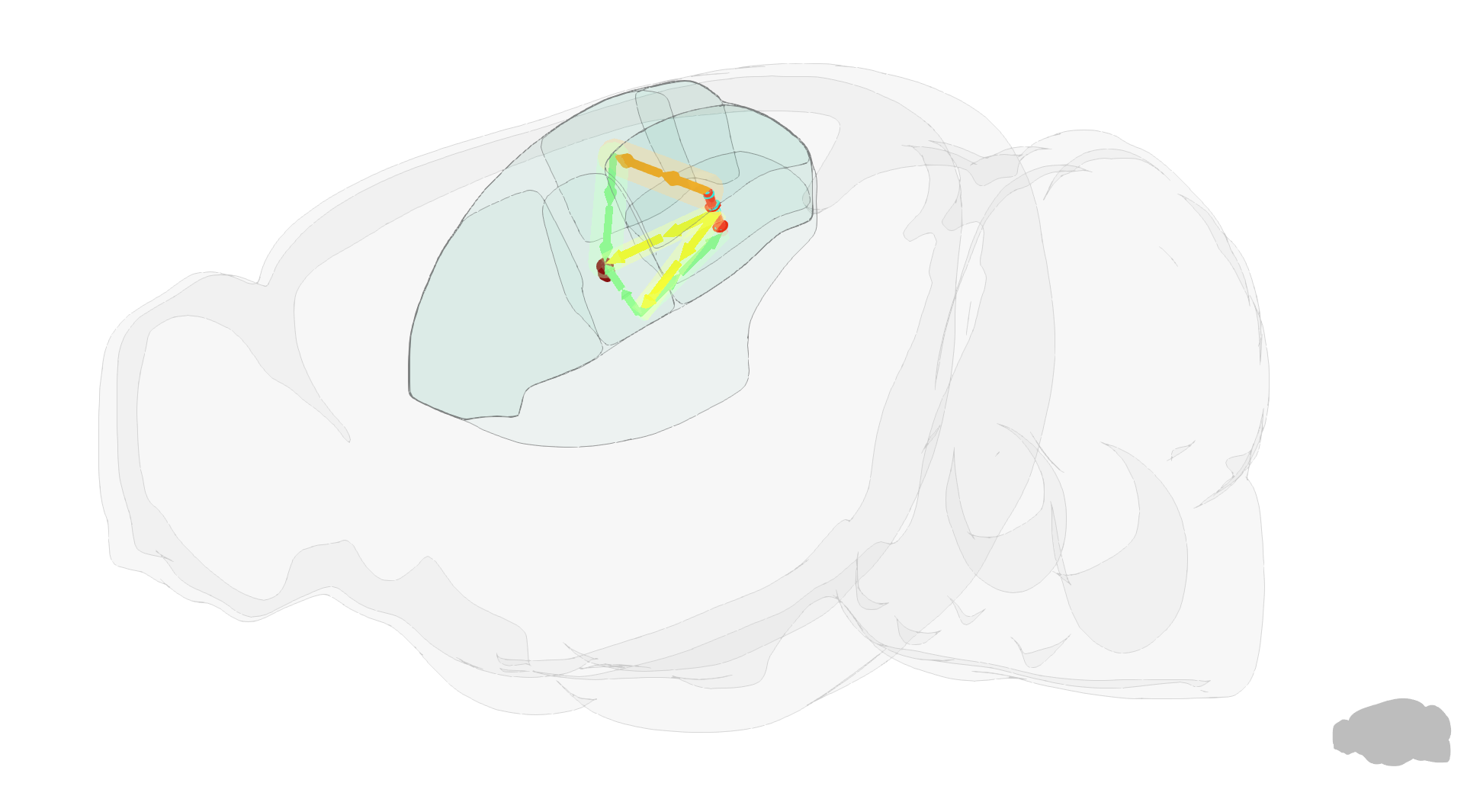
## Useful Links
Github project: https://github.com/SEU-ALLEN-codebase/brain-loop-search
Documentation: https://SEU-ALLEN-codebase.github.io/brain-loop-search
Raw data
{
"_id": null,
"home_page": "",
"name": "brain-loop-search",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": "",
"keywords": "neuron-morphology",
"author": "",
"author_email": "Zuohan Zhao <zzhmark@126.com>",
"download_url": "https://files.pythonhosted.org/packages/a2/79/c789b8cd67622030966f0c8eef6a0744750932aff4f96f6839daae3aafdc/brain-loop-search-0.1.7.tar.gz",
"platform": null,
"description": "# Brain Loop Search\r\nTools for screening significant loop structures in a graph, typically a\r\nbrain structure graph with physiological or anatomical edge data.\r\n\r\n## Installation\r\n\r\n```shell\r\n$ pip install brain-loop-search\r\n```\r\n\r\n## Usage\r\n\r\n### Packing a bigger graph for regions of interest\r\nPacking vertices:\r\n```python\r\nimport brain_loop_search as bls\r\n\r\nvertices = [322, 329, 981, 337, 453, 8, 1070] # ccf brain id\r\n\r\n# ccf ontology\r\nontology = bls.brain_utils.CCFv3Ontology()\r\n\r\nvp = bls.packing.VertexPacker(vertices, ontology)\r\n# filtering by level\r\nvp.filter_by_level(fro=1, to=2)\r\n```\r\nPacking a graph:\r\n```python\r\nimport brain_loop_search as bls\r\nimport pandas as pd\r\nimport numpy as np\r\n\r\nvertices = [322, 329, 981, 337, 453, 1070, 345, 353, 361] # ccf brain id\r\n\r\n# adjacent matrix\r\nadj_mat = pd.DataFrame(np.array([\r\n [0, 1, 1, 0, 0, 0, 1, 1, 0],\r\n [0, 0, 1, 1, 1, 1, 0, 1, 0],\r\n [0, 0, 0, 0, 1, 1, 1, 1, 1],\r\n [0, 0, 1, 0, 0, 1, 0, 1, 0],\r\n [1, 0, 1, 0, 0, 1, 0, 1, 0],\r\n [0, 1, 1, 1, 1, 1, 0, 1, 0],\r\n [0, 0, 0, 0, 0, 1, 0, 0, 1],\r\n [1, 0, 0, 0, 0, 1, 1, 1, 0],\r\n [1, 1, 1, 0, 0, 1, 0, 1, 1]\r\n ]), index=vertices, columns=vertices)\r\n\r\n# ccf ontology\r\nontology = bls.brain_utils.CCFv3Ontology()\r\n\r\n# packing\r\nnew_rows = [322]\r\nnew_cols = [337, 329, 353]\r\ngraph_packer = bls.packing.GraphPacker(adj_mat, ontology)\r\nnew_mat = graph_packer.pack(new_rows, new_cols, def_val=0, superior_as_complement=True, aggr_func=np.sum)\r\n```\r\n### Shortest Path Loop Search\r\n\r\nScreen simple loops from the graph.\r\n\r\n```python\r\nimport brain_loop_search as bls\r\nimport pandas as pd\r\n\r\nedges = pd.DataFrame({\r\n \"a\": [1, 2, 3, 4, 5],\r\n \"b\": [4, -1, -1, 1, -1],\r\n \"c\": [5, 6, 7, 8, 9],\r\n \"d\": [-1, 2, 3, 1, 2],\r\n \"e\": [-1, -1, -1, -1, -1]\r\n }, index=[\"a\", \"b\", \"c\", \"d\", \"e\"])\r\n\r\ng = bls.search.ShortestPathLoopSearch()\r\ng.add_subgraph(edges)\r\n\r\n# search by single shortest path with a reverse edge\r\nloops = g.pair_complement(axis_pool=['a', 'b', 'c'])\r\n# search by chaining 3 of the shortest paths found\r\nloops, sssp = g.chain_screen(n_axis=3)\r\n```\r\n\r\n### Max Flow Loop Search\r\n\r\nGenerate a new graph of potentially integrated loops.\r\n\r\n```python\r\nimport brain_loop_search as bls\r\nimport pandas as pd\r\n\r\nedges = pd.DataFrame({\r\n \"a\": [1, 2, 3, 4, 5],\r\n \"b\": [4, -1, -1, 1, -1],\r\n \"c\": [5, 6, 7, 8, 9],\r\n \"d\": [-1, 2, 3, 1, 2],\r\n \"e\": [-1, -1, -1, -1, -1]\r\n }, index=[\"a\", \"b\", \"c\", \"d\", \"e\"])\r\n\r\ng = bls.search.ShortestPathLoopSearch()\r\ng.add_subgraph(edges)\r\n\r\n# find a single max flow with a reverse edge (like a magnet field)\r\nnew_g = g.magnet_flow(s='b', t='a')\r\n# find cycled max flows and merge them into a new graph\r\nnew_g = g.merged_cycle_flow(axes=['b', 'c', 'a'])\r\n```\r\n\r\n### Visualization\r\n\r\nVisualize a single loop\r\n\r\n```python\r\nimport brain_loop_search as bls\r\n\r\n# a loop is a list of list, with the head and tail of the sublist as axes\r\n# here are some random picked brain regions\r\nloop = [[950, 974, 417], [417, 993], [993, 234, 289, 950]]\r\nbls.brain_utils.draw_single_loop(loop, 'test.png')\r\n```\r\nFigure:\r\n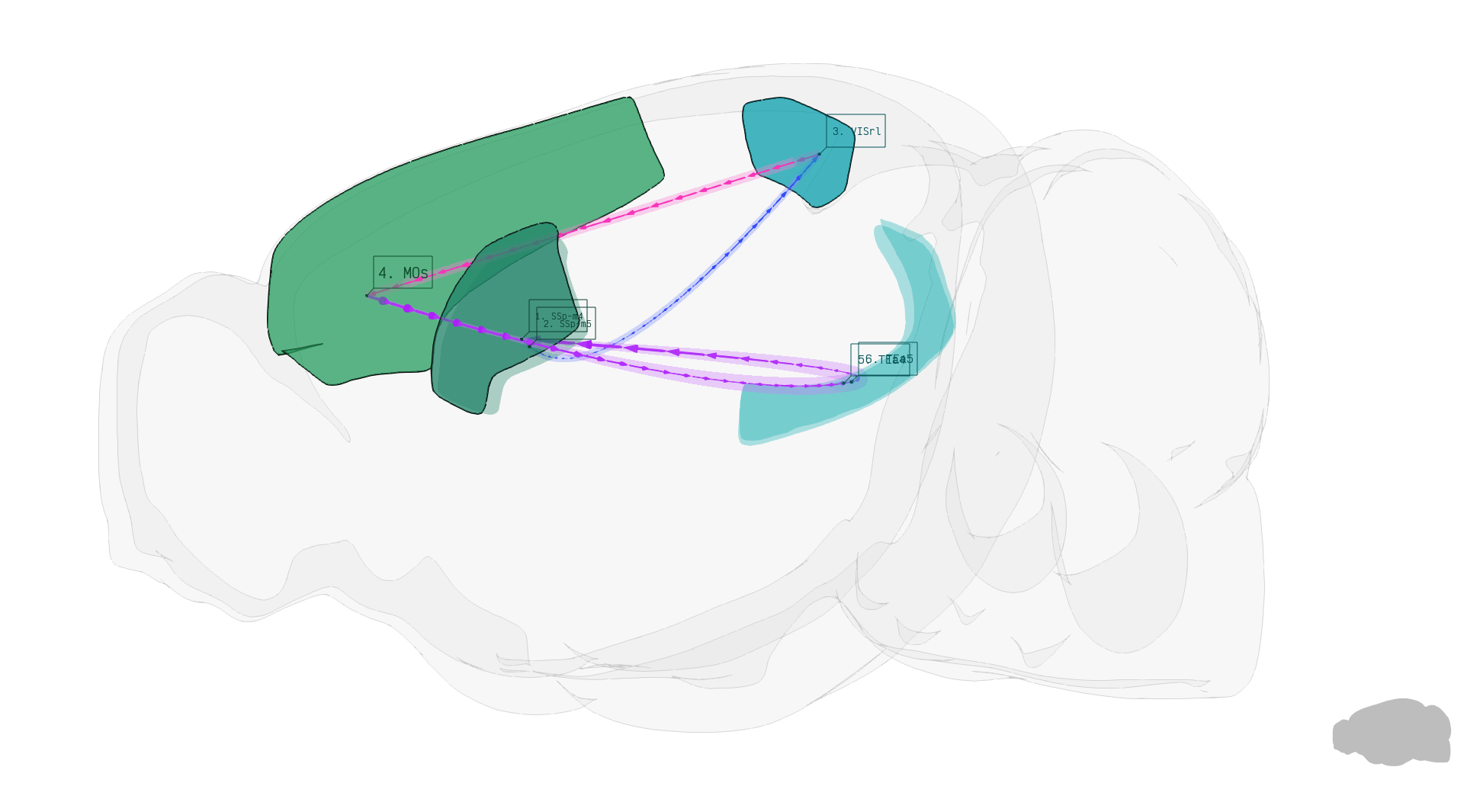\r\n\r\nVisualize a graph\r\n\r\n```python\r\nimport numpy as np\r\nimport pandas as pd\r\nimport brain_loop_search as bls\r\nvertices = [322, 329, 981, 337, 453, 1070, 345, 353, 361]\r\nadj_mat = pd.DataFrame(np.array([\r\n [0, 2, 1, 0, 0, 0, 1, 8, 0],\r\n [0, 0, 3, 1, 5, 1, 0, 5, 0],\r\n [0, 0, 0, 0, 1, 3, 2, 1, 2],\r\n [0, 0, 6, 0, 0, 1, 0, 4, 0],\r\n [1, 0, 1, 0, 0, 1, 0, 4, 0],\r\n [0, 1, 7, 1, 4, 1, 0, 1, 0],\r\n [0, 0, 0, 0, 0, 1, 0, 0, 1],\r\n [1, 0, 0, 0, 0, 2, 2, 1, 0],\r\n [1, 2, 2, 0, 0, 1, 0, 1, 1]\r\n]), index=vertices, columns=vertices)\r\ng = bls.search.GraphMaintainer()\r\ng.add_subgraph(adj_mat)\r\nbls.brain_utils.draw_brain_graph(g.graph, 'test2.png', thr=3)\r\n```\r\n\r\nFigure:\r\n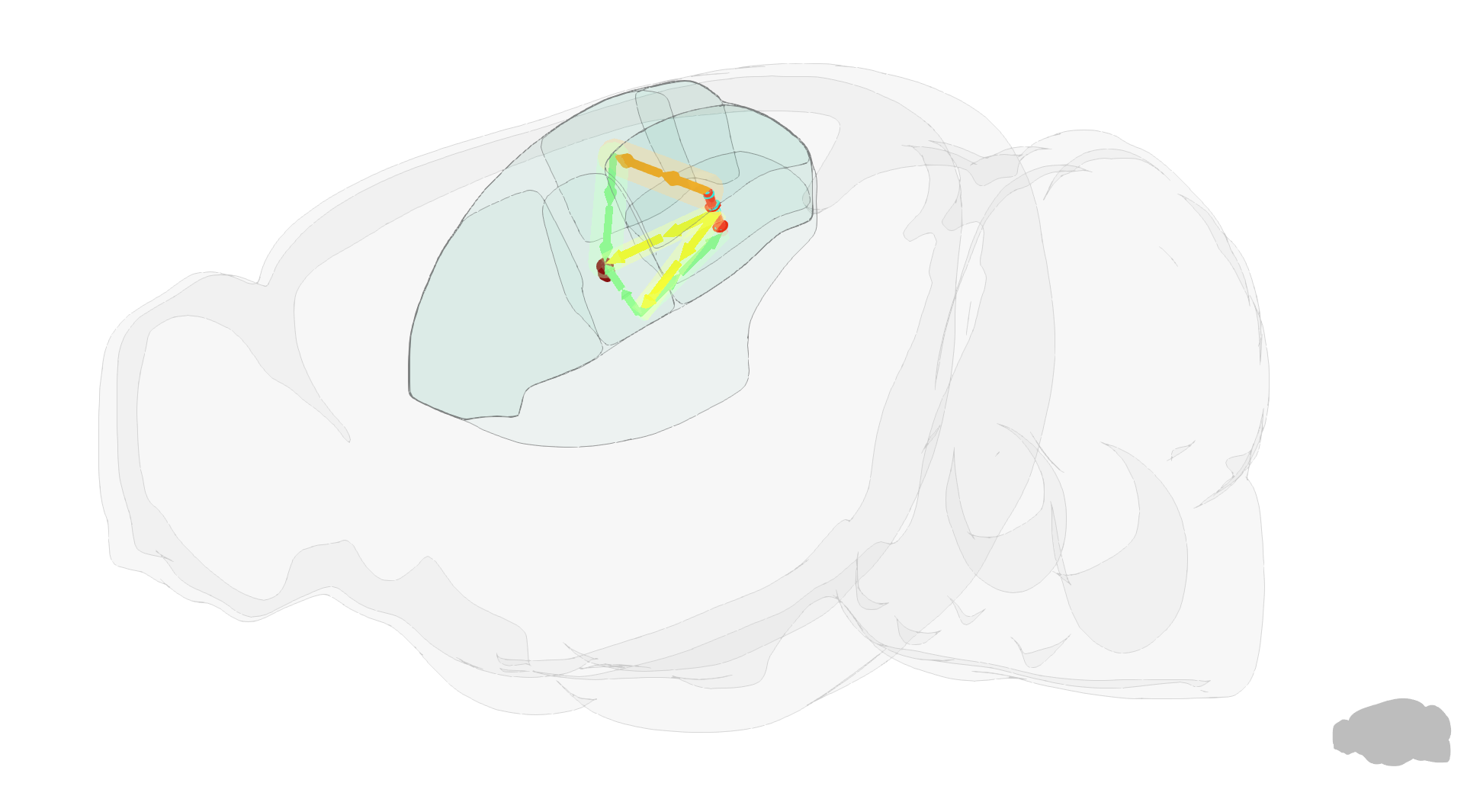\r\n\r\n\r\n## Useful Links\r\n\r\nGithub project: https://github.com/SEU-ALLEN-codebase/brain-loop-search\r\n\r\nDocumentation: https://SEU-ALLEN-codebase.github.io/brain-loop-search\r\n",
"bugtrack_url": null,
"license": "MIT License",
"summary": "Screen loops among brain structures(or any entities comprising a graph).",
"version": "0.1.7",
"project_urls": {
"Documentation": "https://SEU-ALLEN-codebase.github.io/brain-loop-search",
"GitHub Project": "https://github.com/SEU-ALLEN-codebase/brain-loop-search"
},
"split_keywords": [
"neuron-morphology"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6e1815eff5d379441653856c043dc34877f44b6cd87569e63a5b978e95ee67ab",
"md5": "67d7d01e33e9d004904907b26bf47850",
"sha256": "87408b464176cd5b810454a80000a37dc9ae88045b23c1eb7d53058514ba0469"
},
"downloads": -1,
"filename": "brain_loop_search-0.1.7-py3-none-any.whl",
"has_sig": false,
"md5_digest": "67d7d01e33e9d004904907b26bf47850",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 33389,
"upload_time": "2023-07-27T11:10:13",
"upload_time_iso_8601": "2023-07-27T11:10:13.290581Z",
"url": "https://files.pythonhosted.org/packages/6e/18/15eff5d379441653856c043dc34877f44b6cd87569e63a5b978e95ee67ab/brain_loop_search-0.1.7-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a279c789b8cd67622030966f0c8eef6a0744750932aff4f96f6839daae3aafdc",
"md5": "3458e0148bacabe0e35df36936d29be8",
"sha256": "f0878cdeaa89f8044aa96128fc66fcccb35928ef9a53d5a10e4a58e2febdbad7"
},
"downloads": -1,
"filename": "brain-loop-search-0.1.7.tar.gz",
"has_sig": false,
"md5_digest": "3458e0148bacabe0e35df36936d29be8",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 321959,
"upload_time": "2023-07-27T11:10:15",
"upload_time_iso_8601": "2023-07-27T11:10:15.221685Z",
"url": "https://files.pythonhosted.org/packages/a2/79/c789b8cd67622030966f0c8eef6a0744750932aff4f96f6839daae3aafdc/brain-loop-search-0.1.7.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-27 11:10:15",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "SEU-ALLEN-codebase",
"github_project": "brain-loop-search",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "brain-loop-search"
}