Name | colorful-logger JSON |
Version |
0.1.13
JSON |
| download |
home_page | |
Summary | A colorful logger for python3. |
upload_time | 2023-02-21 05:03:05 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3 |
license | MIT License Copyright (c) 2021 thep0y Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
log
logger
logging
colorful
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
> :warning: This README is translated by Google. If there is a grammatical error, please open an issue to correct it!
# python-logger
Colorful logger for python3
## How to use
### Install
```shell
pip install colorful-logger
```
### Usage
#### 1 default logger
You can directly use the default logger, the colored logs will be printed on the terminal, and the default logger level is **warning**.
```python
from colorful_logger import logger
with logger:
logger.debug("default logger")
logger.info("default logger")
logger.warning("default logger")
logger.error("default logger")
```
As you can see, `logger` needs to be executed in the `with` statement, because this package uses `QueueListener` to call log output. You need to call the `start` method before using `logger` to output the log, and you need to call the `stop` after the end of use. I encapsulated these two methods in the `with` statement. In non-special scenarios, there is no need to call the `start` and `stop` methods separately.

#### 2 custom logger
You can also change the log level, save the log to a file, change the logger name, and the log may not be output to the terminal.
```python
from colorful_logger import get_logger, DEBUG
def demo_logger(to_file=False):
file = "test_%d.log"
l1 = get_logger(
"demo",
DEBUG,
add_file_path=False,
disable_line_number_filter=False,
file_path=file % 1 if to_file else None,
)
with l1:
l1.debug("without file path")
l1.info("without file path")
l1.warning("without file path")
l1.error("without file path")
l2 = get_logger(
"demo",
DEBUG,
add_file_path=True,
disable_line_number_filter=False,
file_path=file % 2 if to_file else None,
)
with l2:
l2.debug("with file path")
l2.info("with file path")
l2.warning("with file path")
l2.error("with file path")
l3 = get_logger(
None,
DEBUG,
add_file_path=True,
disable_line_number_filter=True,
file_path=file % 3 if to_file else None,
)
with l3:
l3.debug("without name, and with path")
l3.info("without name, and with path")
l3.warning("without name, and with path")
l3.error("without name, and with path")
l4 = get_logger(
None,
DEBUG,
add_file_path=False,
disable_line_number_filter=True,
file_path=file % 4 if to_file else None,
)
with l4:
l4.debug("without name and path")
l4.info("without name and path")
l4.warning("without name and path")
l4.error("without name and path")
```
There may be unexpected situations when outputting logs outside of the `with` statement, which may not achieve the expected results.
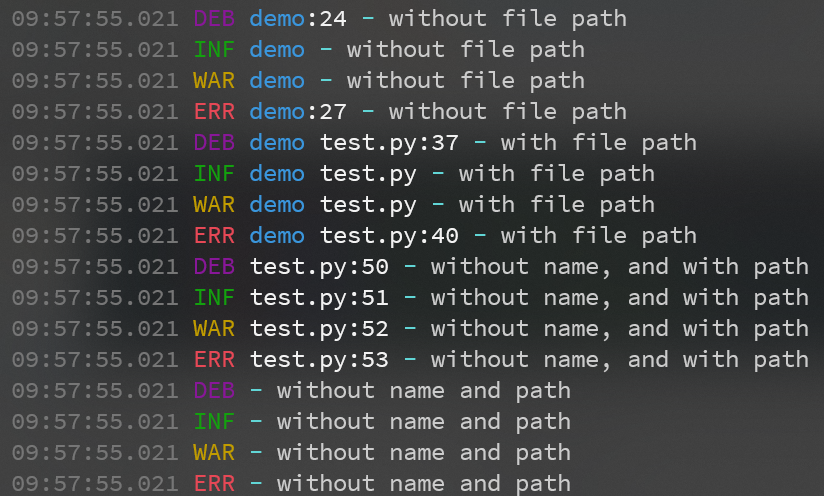
The content of the log file `./test.log` (example, inconsistent with the information in the above figure):
```
[90m10:09:33.146[0m [35mDEB[0m [36mdemo[0m[1m:26[0m [96m-[0m without file path
[90m10:09:33.146[0m [32mINF[0m [36mdemo[0m [96m-[0m without file path
[90m10:09:33.146[0m [33mWAR[0m [36mdemo[0m [96m-[0m without file path
[90m10:09:33.146[0m [91mERR[0m [36mdemo[0m[1m:29[0m [96m-[0m without file path
```
The log output to the file is not a color log by default.
If you need to save the color log in a file, set the `file_colorful` parameter to `True`. In this example, the color log is saved.
The color log file has only one function, which is to view the real-time log in the terminal:
- Unix
```shell
tail -f test.log
# 或
cat test.log
```
- Windows
```powershell
Get-Content -Path test.log
```
#### 3 child logger
After defining a `logger`, I want to use all the parameters of this `logger` except `name` to output the log. At this time, you need to use the `child_logger` method to generate a child logger. The child logger needs to be in the `with` of the parent logger Execute in the statement:
```python
from colorful_logger import get_logger, DEBUG
# parent logger
logger = get_logger(name="sample_logger", level=DEBUG, file_path="./test.log")
with logger:
logger.error("parent error")
l1 = logger.child("l1")
l1.error("l1 error")
l1.fatal("l1 fatal")
```
The child logger is the same except that the name is different from the parent logger, and it will not output the log of the third-party library.
The execution of the child logger in the `with` statement of the parent logger does not mean that it must be called directly in the `with` statement. It can be executed in a function in the `with` statement, such as:
```python
# log.py
from colorful_logger import get_logger, DEBUG
logger = get_logger(name="sample_logger", level=DEBUG, file_path="./test.log")
```
```python
# main.py
from log import logger
from other_file import test
with logger:
test()
```
```python
# other_file.py
test_logger = logger.child("test_logger")
def test():
test_logger.error("test error")
```
Raw data
{
"_id": null,
"home_page": "",
"name": "colorful-logger",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3",
"maintainer_email": "",
"keywords": "log,logger,logging,colorful",
"author": "",
"author_email": "thep0y <thepoy@163.com>",
"download_url": "https://files.pythonhosted.org/packages/6f/c0/a3081036a1213bc10a22e1d1e3d1cbca2c996473d72ed53f6c53bf6ede4b/colorful-logger-0.1.13.tar.gz",
"platform": null,
"description": "> :warning: This README is translated by Google. If there is a grammatical error, please open an issue to correct it!\n\n# python-logger\n\nColorful logger for python3\n\n## How to use\n\n### Install\n\n```shell\npip install colorful-logger\n```\n\n### Usage\n\n#### 1 default logger\n\nYou can directly use the default logger, the colored logs will be printed on the terminal, and the default logger level is **warning**.\n\n```python\nfrom colorful_logger import logger\n\nwith logger:\n logger.debug(\"default logger\")\n logger.info(\"default logger\")\n logger.warning(\"default logger\")\n logger.error(\"default logger\")\n```\n\nAs you can see, `logger` needs to be executed in the `with` statement, because this package uses `QueueListener` to call log output. You need to call the `start` method before using `logger` to output the log, and you need to call the `stop` after the end of use. I encapsulated these two methods in the `with` statement. In non-special scenarios, there is no need to call the `start` and `stop` methods separately.\n\n\n\n#### 2 custom logger\n\nYou can also change the log level, save the log to a file, change the logger name, and the log may not be output to the terminal.\n\n```python\nfrom colorful_logger import get_logger, DEBUG\n\n\ndef demo_logger(to_file=False):\n file = \"test_%d.log\"\n\n l1 = get_logger(\n \"demo\",\n DEBUG,\n add_file_path=False,\n disable_line_number_filter=False,\n file_path=file % 1 if to_file else None,\n )\n with l1:\n l1.debug(\"without file path\")\n l1.info(\"without file path\")\n l1.warning(\"without file path\")\n l1.error(\"without file path\")\n\n l2 = get_logger(\n \"demo\",\n DEBUG,\n add_file_path=True,\n disable_line_number_filter=False,\n file_path=file % 2 if to_file else None,\n )\n with l2:\n l2.debug(\"with file path\")\n l2.info(\"with file path\")\n l2.warning(\"with file path\")\n l2.error(\"with file path\")\n\n l3 = get_logger(\n None,\n DEBUG,\n add_file_path=True,\n disable_line_number_filter=True,\n file_path=file % 3 if to_file else None,\n )\n with l3:\n l3.debug(\"without name, and with path\")\n l3.info(\"without name, and with path\")\n l3.warning(\"without name, and with path\")\n l3.error(\"without name, and with path\")\n\n l4 = get_logger(\n None,\n DEBUG,\n add_file_path=False,\n disable_line_number_filter=True,\n file_path=file % 4 if to_file else None,\n )\n with l4:\n l4.debug(\"without name and path\")\n l4.info(\"without name and path\")\n l4.warning(\"without name and path\")\n l4.error(\"without name and path\")\n```\n\nThere may be unexpected situations when outputting logs outside of the `with` statement, which may not achieve the expected results.\n\n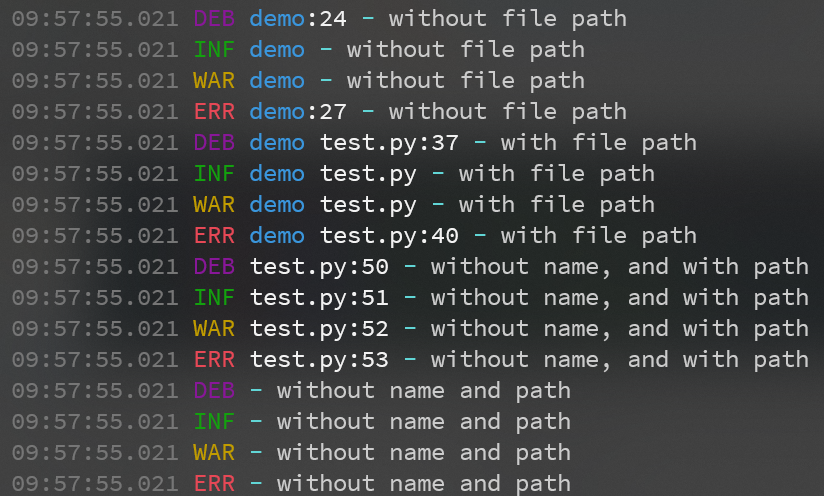\n\nThe content of the log file `./test.log` (example, inconsistent with the information in the above figure):\n\n```\n\u001b[90m10:09:33.146\u001b[0m \u001b[35mDEB\u001b[0m \u001b[36mdemo\u001b[0m\u001b[1m:26\u001b[0m \u001b[96m-\u001b[0m without file path\n\u001b[90m10:09:33.146\u001b[0m \u001b[32mINF\u001b[0m \u001b[36mdemo\u001b[0m \u001b[96m-\u001b[0m without file path\n\u001b[90m10:09:33.146\u001b[0m \u001b[33mWAR\u001b[0m \u001b[36mdemo\u001b[0m \u001b[96m-\u001b[0m without file path\n\u001b[90m10:09:33.146\u001b[0m \u001b[91mERR\u001b[0m \u001b[36mdemo\u001b[0m\u001b[1m:29\u001b[0m \u001b[96m-\u001b[0m without file path\n```\n\nThe log output to the file is not a color log by default.\n\nIf you need to save the color log in a file, set the `file_colorful` parameter to `True`. In this example, the color log is saved.\n\nThe color log file has only one function, which is to view the real-time log in the terminal:\n\n- Unix\n\n```shell\ntail -f test.log\n# \u6216\ncat test.log\n```\n\n- Windows\n\n```powershell\nGet-Content -Path test.log\n```\n\n#### 3 child logger\n\nAfter defining a `logger`, I want to use all the parameters of this `logger` except `name` to output the log. At this time, you need to use the `child_logger` method to generate a child logger. The child logger needs to be in the `with` of the parent logger Execute in the statement:\n\n```python\nfrom colorful_logger import get_logger, DEBUG\n\n# parent logger\nlogger = get_logger(name=\"sample_logger\", level=DEBUG, file_path=\"./test.log\")\n\nwith logger:\n logger.error(\"parent error\")\n l1 = logger.child(\"l1\")\n l1.error(\"l1 error\")\n l1.fatal(\"l1 fatal\")\n```\n\nThe child logger is the same except that the name is different from the parent logger, and it will not output the log of the third-party library.\n\nThe execution of the child logger in the `with` statement of the parent logger does not mean that it must be called directly in the `with` statement. It can be executed in a function in the `with` statement, such as:\n\n```python\n# log.py\nfrom colorful_logger import get_logger, DEBUG\n\nlogger = get_logger(name=\"sample_logger\", level=DEBUG, file_path=\"./test.log\")\n```\n\n```python\n# main.py\nfrom log import logger\nfrom other_file import test\n\nwith logger:\n test()\n```\n\n```python\n# other_file.py\n\ntest_logger = logger.child(\"test_logger\")\n\ndef test():\n test_logger.error(\"test error\")\n```\n\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2021 thep0y Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "A colorful logger for python3.",
"version": "0.1.13",
"project_urls": {
"homepage": "https://github.com/thep0y/python-logger",
"repository": "https://github.com/thep0y/python-logger"
},
"split_keywords": [
"log",
"logger",
"logging",
"colorful"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "9a1999dced161f158a3f0bdf37d8cca82199cb02172e58af34142400c46f048a",
"md5": "6a102db7f0275d3ebabd061802f2b7f3",
"sha256": "3cc2a9cff1f713cfdff3a3c36ffc19ce636b084911ce234f23a76feeda83bf62"
},
"downloads": -1,
"filename": "colorful_logger-0.1.13-py3-none-any.whl",
"has_sig": false,
"md5_digest": "6a102db7f0275d3ebabd061802f2b7f3",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3",
"size": 11026,
"upload_time": "2023-02-21T05:03:04",
"upload_time_iso_8601": "2023-02-21T05:03:04.300028Z",
"url": "https://files.pythonhosted.org/packages/9a/19/99dced161f158a3f0bdf37d8cca82199cb02172e58af34142400c46f048a/colorful_logger-0.1.13-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6fc0a3081036a1213bc10a22e1d1e3d1cbca2c996473d72ed53f6c53bf6ede4b",
"md5": "fd0a087afc9c3b186a5fcc10f505cf3d",
"sha256": "5a34983d496ef65f4b96991c56f34fccda30e966593d05c04b484fa26e9f30d1"
},
"downloads": -1,
"filename": "colorful-logger-0.1.13.tar.gz",
"has_sig": false,
"md5_digest": "fd0a087afc9c3b186a5fcc10f505cf3d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3",
"size": 13273,
"upload_time": "2023-02-21T05:03:05",
"upload_time_iso_8601": "2023-02-21T05:03:05.619456Z",
"url": "https://files.pythonhosted.org/packages/6f/c0/a3081036a1213bc10a22e1d1e3d1cbca2c996473d72ed53f6c53bf6ede4b/colorful-logger-0.1.13.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-02-21 05:03:05",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "thep0y",
"github_project": "python-logger",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "colorful-logger"
}