# Construct Editor
This package provides a GUI (based on wxPython) for 'construct', which is a powerful declarative and symmetrical parser and builder for binary data. It can either be used standalone or embedded as a widget in another application.
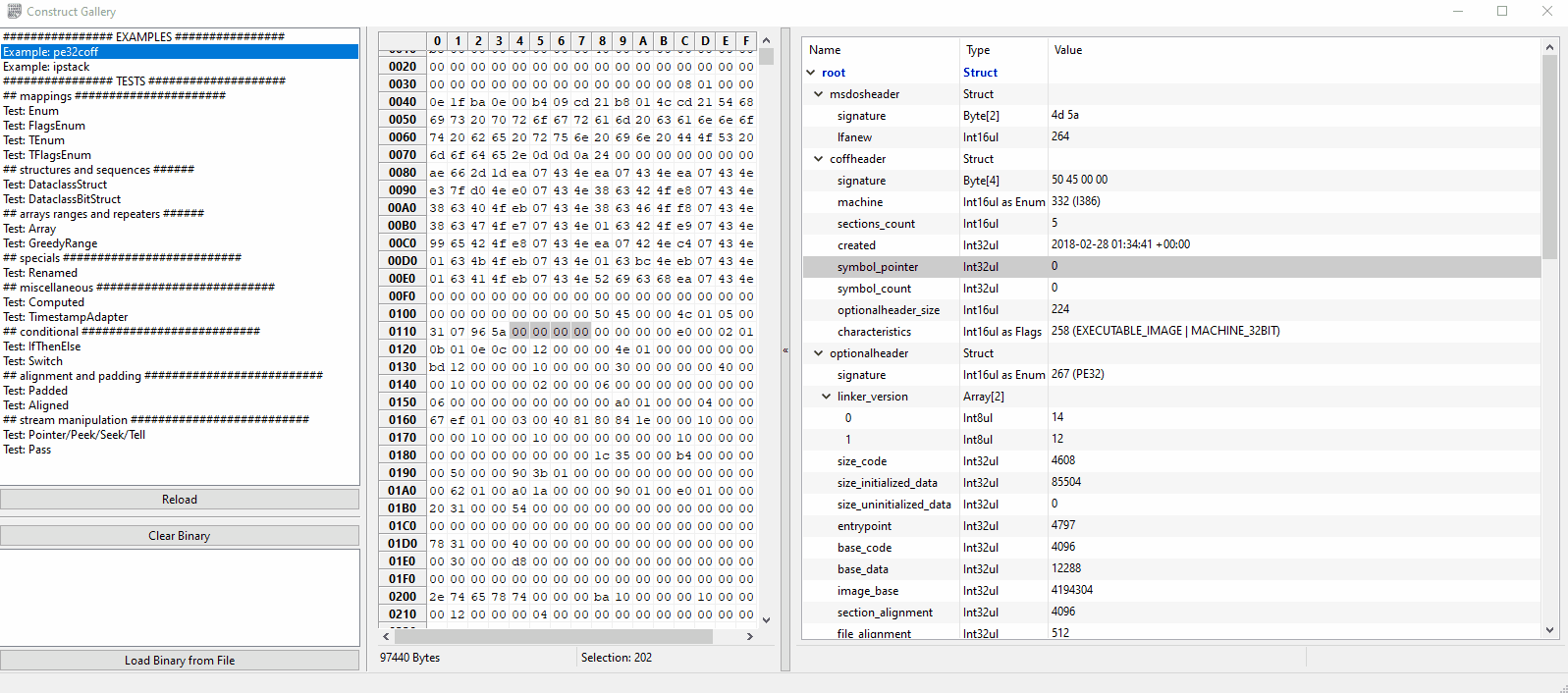
Features:
- show documentation as tooltip
- different editors for:
- Integer values
- Enum values
- FlagsEnum values
- DateTime values
- undo/redo in HexEditor and in ConstructEditor
- extensible for custom constructs
## Installation
The preferred way to installation is via PyPI:
```
pip install construct-editor
```
## Getting started (Standalone)
To start the standalone version, just execute the following in the command line:
```
construct-editor
```
## Getting started (as Widgets)
This is a simple example
```python
import wx
import construct as cs
from construct_editor.wx_widgets import WxConstructHexEditor
constr = cs.Struct(
"a" / cs.Int16sb,
"b" / cs.Int16sb,
)
b = bytes([0x12, 0x34, 0x56, 0x78])
app = wx.App(False)
frame = wx.Frame(None, title="Construct Hex Editor", size=(1000, 200))
editor_panel = WxConstructHexEditor(frame, construct=constr, binary=b)
editor_panel.construct_editor.expand_all()
frame.Show(True)
app.MainLoop()
```
This snipped generates a GUI like this:

## Widgets
### ConstructHexEditor
This is the main widget ot this library. It offers a look at the raw binary data and also at the parsed structure.
It offers a way to modify the raw binary data, which is then automaticly converted to the structed view. It also supports to modify the structed data and build the binary data from it.
### ConstructEditor
This is just the right side of the `ConstructHexEditor`, but can be used also used as standalone widget. It provides:
- Viewing the structure of a construct (without binary data)
- Parsing binary data according to the construct
### HexEditor
Just the left side of the `ConstructHexEditor`, but can be used also used as standalone widget. It provides:
- Viewing Bytes in a Hexadecimal form
- Callbacks when some value changed
- Changeable format via `TableFormat`
Raw data
{
"_id": null,
"home_page": "https://github.com/timrid/construct-editor",
"name": "construct-editor",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "",
"keywords": "gui,wx,wxpython,widget,binary,editorconstruct,kaitai,declarative,data structure,struct,binary,symmetric,parser,builder,parsing,building,pack,unpack,packer,unpacker,bitstring,bytestring,bitstruct",
"author": "Tim Riddermann",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/57/ca/f56e4399d49a32143c070f31b163121f62a0f07a293e5c0ee9f24c22a13a/construct-editor-0.1.5.tar.gz",
"platform": "Windows",
"description": "# Construct Editor\nThis package provides a GUI (based on wxPython) for 'construct', which is a powerful declarative and symmetrical parser and builder for binary data. It can either be used standalone or embedded as a widget in another application.\n\n\n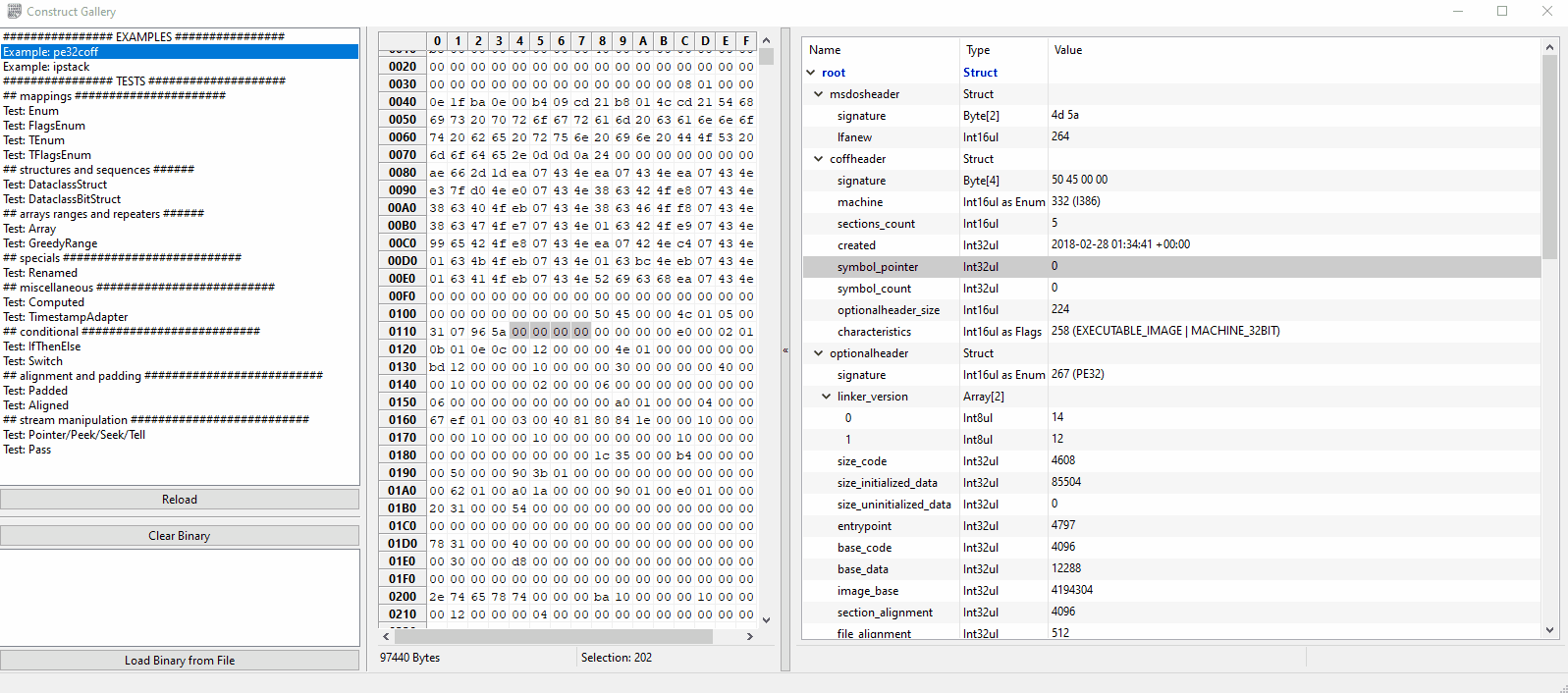\n\n\nFeatures:\n- show documentation as tooltip\n- different editors for:\n - Integer values\n - Enum values\n - FlagsEnum values\n - DateTime values\n- undo/redo in HexEditor and in ConstructEditor\n- extensible for custom constructs\n\n## Installation\nThe preferred way to installation is via PyPI:\n```\npip install construct-editor\n```\n\n## Getting started (Standalone)\nTo start the standalone version, just execute the following in the command line:\n```\nconstruct-editor\n```\n\n## Getting started (as Widgets)\nThis is a simple example \n```python\nimport wx\nimport construct as cs\nfrom construct_editor.wx_widgets import WxConstructHexEditor\n\nconstr = cs.Struct(\n \"a\" / cs.Int16sb,\n \"b\" / cs.Int16sb,\n)\nb = bytes([0x12, 0x34, 0x56, 0x78])\n\napp = wx.App(False)\nframe = wx.Frame(None, title=\"Construct Hex Editor\", size=(1000, 200))\neditor_panel = WxConstructHexEditor(frame, construct=constr, binary=b)\neditor_panel.construct_editor.expand_all()\nframe.Show(True)\napp.MainLoop()\n```\n\nThis snipped generates a GUI like this:\n\n\n\n\n## Widgets\n### ConstructHexEditor\nThis is the main widget ot this library. It offers a look at the raw binary data and also at the parsed structure.\nIt offers a way to modify the raw binary data, which is then automaticly converted to the structed view. It also supports to modify the structed data and build the binary data from it.\n\n\n### ConstructEditor\nThis is just the right side of the `ConstructHexEditor`, but can be used also used as standalone widget. It provides:\n- Viewing the structure of a construct (without binary data)\n- Parsing binary data according to the construct\n\n\n### HexEditor\nJust the left side of the `ConstructHexEditor`, but can be used also used as standalone widget. It provides:\n- Viewing Bytes in a Hexadecimal form\n- Callbacks when some value changed\n- Changeable format via `TableFormat`\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "GUI (based on wxPython) for 'construct', which is a powerful declarative and symmetrical parser and builder for binary data.",
"version": "0.1.5",
"project_urls": {
"Homepage": "https://github.com/timrid/construct-editor"
},
"split_keywords": [
"gui",
"wx",
"wxpython",
"widget",
"binary",
"editorconstruct",
"kaitai",
"declarative",
"data structure",
"struct",
"binary",
"symmetric",
"parser",
"builder",
"parsing",
"building",
"pack",
"unpack",
"packer",
"unpacker",
"bitstring",
"bytestring",
"bitstruct"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "821276673426fd82f23642578e4d2d44299dc8d53746b2427c1b433e28d94cb5",
"md5": "b8120682a5eb9cb344229921e60ce6b5",
"sha256": "deb4acfb79736cae1b423fa44b73a9c6fb4ee8116a51c69e39f366ce0df7b1ae"
},
"downloads": -1,
"filename": "construct_editor-0.1.5-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b8120682a5eb9cb344229921e60ce6b5",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 96129,
"upload_time": "2023-07-24T09:22:54",
"upload_time_iso_8601": "2023-07-24T09:22:54.598215Z",
"url": "https://files.pythonhosted.org/packages/82/12/76673426fd82f23642578e4d2d44299dc8d53746b2427c1b433e28d94cb5/construct_editor-0.1.5-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "57caf56e4399d49a32143c070f31b163121f62a0f07a293e5c0ee9f24c22a13a",
"md5": "af833913b3255584716c1cb320d68e4c",
"sha256": "51f24fc5d5d8c8cf26c8f369474a742553129d54644c43f20d3fd74ca8f6caec"
},
"downloads": -1,
"filename": "construct-editor-0.1.5.tar.gz",
"has_sig": false,
"md5_digest": "af833913b3255584716c1cb320d68e4c",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 77141,
"upload_time": "2023-07-24T09:22:56",
"upload_time_iso_8601": "2023-07-24T09:22:56.261038Z",
"url": "https://files.pythonhosted.org/packages/57/ca/f56e4399d49a32143c070f31b163121f62a0f07a293e5c0ee9f24c22a13a/construct-editor-0.1.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-07-24 09:22:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "timrid",
"github_project": "construct-editor",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "construct-editor"
}