Github page: https://github.com/0x1618/CSS-Selector-Minifier
# CSS-Selector-Minifier
CSS-Selector-Minifier is a Python package that minifies CSS class and id names in CSS, HTML and JavaScript files. It's a custom made project and it may not meet your requirements. I decided to share my code with you because I also couldn't find a working minifer selector.
## Installation
You can install the package using pip:
```
pip install css-selector-minifier
```
# Usage
## ** Important note **
**You have to prepare your code to make my code work**.
CSS-Selector-Minifier uses a regex pattern to find your CSS selectors, and that regex pattern needs an unique prefix.
By default, CSS-Selector-Minifier uses the prefix `'-s-'` for the beginning of the CSS selector and `'-e-'` for the end.
## Example for preparation
If you want to use `default` prefix:
CSS that **won't work**
```css
.main {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
#child {
background: grey;
font-size: 21px;
opacity: 0.51;
}
.bettermain, .betterchild {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
.child .main {
color: grey;
}
```
CSS that **will work**
```css
.-s-main-e- {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
#-s-child-e- {
background: grey;
font-size: 21px;
opacity: 0.51;
}
.-s-bettermain-e-, .-s-betterchild-e- {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
.-s-child-e- .-s-main-e- {
color: grey;
}
```
## CSS-Selector-Minifier arguments
- `css`: a list of paths to CSS files. (optional)
- `html`: a list of paths to HTML files. (optional)
- `js`: a list of paths to JavaScript files. (optional)
- `start_prefix`: a string to prefix the start of the class or id to be minified (default: -s-).
- `end_prefix`: a string to prefix the end of the class or id to be minified (default: -e-).
- `min_letters`: an integer representing the minimum length of the minified class or id name (default: 1).
## CSS-Selector-Minifier methods
- `Get_All_CSS_Selectors()`: returns a set of all CSS selectors in the specified CSS files.
- `Generate_Minifed_Selectors()`: returns a generator object containing smart generated minified CSS selectors.
- `Generate_Map_For_CSS_Selectors()`: returns a dictionary mapping original CSS selectors to their minified counterparts.
- `Replace_CSS_Selectors_With_Minifed(backup=True)`: replaces all CSS selectors in the specified CSS, HTML, and JavaScript files with their minified counterparts. If backup is set to True, a backup copy of each file will be created with a .bak extension.
- `Minify(backup=True)`: perform minification. If backup is set to True, a backup copy of each file will be created with a .bak extension.
# Example
Before CSS-Selector-Minifier
style.css
```css
.-s-main-e- {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
#-s-child-e- {
background: grey;
font-size: 21px;
opacity: 0.51;
}
.-s-bettermain-e-, .-s-betterchild-e- {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
.-s-child-e- .-s-main-e- {
color: grey;
}
```
index.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Test</title>
</head>
<body>
<div class="-s-main-e-">
<p id="-s-child-e-">Lorem ipsum. child</p>
<form id="main">
<input>
</form>
</div>
<div class="-s-bettermain-e-">
<p class="-s-betterchild-e-">Lorem ipsum. betterchild</p>
</div>
</body>
</html>
```
main.js
```js
$(document).ready(function() {
$('.-s-child-e-').text('jquery is working')
$('#-s-child-e-').attr('message', 'child')
});
```
After CSS-Selector-Minifier
style.css
```css
.ae {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
#ad {
background: grey;
font-size: 21px;
opacity: 0.51;
}
.ac, .aa {
background: yellow;
font-size: 10px;
opacity: 0.5;
}
.ab .ae {
color: grey;
}
```
index.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Test</title>
</head>
<body>
<div class="ae">
<p id="ab">Lorem ipsum. child</p>
<form id="main">
<input>
</form>
</div>
<div class="ac">
<p class="aa">Lorem ipsum. betterchild</p>
</div>
</body>
</html>
```
main.js
```js
$(document).ready(function() {
$('.ab').text('jquery is working')
$('#ab').attr('message', 'child')
});
```
main.py
```python
from css_selector_minifier import Minify_CSS_Names
m = Minify_CSS_Names(
css=['src/style.css'],
html=['src/index.html'],
js=['src/main.js'],
start_prefix='-s-',
end_prefix='-e-',
min_letters=2
)
m.Minify()
```
src folder after running Minify_CSS_Names with default backup arg in m.Replace_CSS_Selectors_With_Minifed()
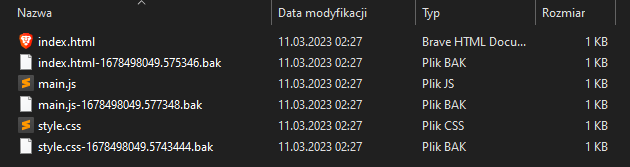
This will replace the CSS selectors in your files with minified selectors.
By default, the package will back up your files before replacing the selectors. You can disable this by passing backup=False to the Replace_CSS_Selectors_With_Minifed method.
# Note
You can use glob for paths to files
```python
from css_selector_minifier import Minify_CSS_Names
import glob
m = Minify_CSS_Names(
css=glob.glob('src/*.css'),
html=glob.glob('src/*.html'),
js=glob.glob('src/*.js'),
start_prefix='-s-',
end_prefix='-e-',
min_letters=2
)
m.Minify()
```
Raw data
{
"_id": null,
"home_page": "",
"name": "css-selector-minifier",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "python,css,html,js,minify,css selectors,selector,selectors,minify css selectors",
"author": "0x1618 aka ctrlshifti (Maksymilian Sawicz)",
"author_email": "<max.sawicz@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/1f/5a/7d2d69b2fe30771631962df3974d7118f7f7f8c071ce35b5210b1142c85c/css_selector_minifier-2.0.tar.gz",
"platform": null,
"description": "Github page: https://github.com/0x1618/CSS-Selector-Minifier \n\n# CSS-Selector-Minifier\n\nCSS-Selector-Minifier is a Python package that minifies CSS class and id names in CSS, HTML and JavaScript files. It's a custom made project and it may not meet your requirements. I decided to share my code with you because I also couldn't find a working minifer selector.\n\n## Installation\n\nYou can install the package using pip:\n\n```\npip install css-selector-minifier\n```\n\n# Usage\n\n## ** Important note **\n\n**You have to prepare your code to make my code work**.\n\nCSS-Selector-Minifier uses a regex pattern to find your CSS selectors, and that regex pattern needs an unique prefix.\n\nBy default, CSS-Selector-Minifier uses the prefix `'-s-'` for the beginning of the CSS selector and `'-e-'` for the end.\n\n## Example for preparation\n\nIf you want to use `default` prefix:\n\nCSS that **won't work**\n```css\n.main {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n#child {\n background: grey;\n font-size: 21px;\n opacity: 0.51;\n}\n\n.bettermain, .betterchild {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n.child .main {\n color: grey;\n}\n```\n\nCSS that **will work**\n```css\n.-s-main-e- {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n#-s-child-e- {\n background: grey;\n font-size: 21px;\n opacity: 0.51;\n}\n\n.-s-bettermain-e-, .-s-betterchild-e- {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n.-s-child-e- .-s-main-e- {\n color: grey;\n}\n```\n\n\n## CSS-Selector-Minifier arguments\n\n- `css`: a list of paths to CSS files. (optional)\n- `html`: a list of paths to HTML files. (optional)\n- `js`: a list of paths to JavaScript files. (optional)\n- `start_prefix`: a string to prefix the start of the class or id to be minified (default: -s-).\n- `end_prefix`: a string to prefix the end of the class or id to be minified (default: -e-).\n- `min_letters`: an integer representing the minimum length of the minified class or id name (default: 1).\n\n## CSS-Selector-Minifier methods\n- `Get_All_CSS_Selectors()`: returns a set of all CSS selectors in the specified CSS files.\n- `Generate_Minifed_Selectors()`: returns a generator object containing smart generated minified CSS selectors.\n- `Generate_Map_For_CSS_Selectors()`: returns a dictionary mapping original CSS selectors to their minified counterparts.\n- `Replace_CSS_Selectors_With_Minifed(backup=True)`: replaces all CSS selectors in the specified CSS, HTML, and JavaScript files with their minified counterparts. If backup is set to True, a backup copy of each file will be created with a .bak extension.\n- `Minify(backup=True)`: perform minification. If backup is set to True, a backup copy of each file will be created with a .bak extension.\n\n# Example\nBefore CSS-Selector-Minifier\n\nstyle.css\n```css\n.-s-main-e- {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n#-s-child-e- {\n background: grey;\n font-size: 21px;\n opacity: 0.51;\n}\n\n.-s-bettermain-e-, .-s-betterchild-e- {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n.-s-child-e- .-s-main-e- {\n color: grey;\n}\n```\n\nindex.html\n```html\n<!DOCTYPE html>\n<html>\n<head>\n\t<meta charset=\"utf-8\">\n\t<meta name=\"viewport\" content=\"width=device-width, initial-scale=1\">\n\t<title>Test</title>\n</head>\n<body>\n\t<div class=\"-s-main-e-\">\n\t\t<p id=\"-s-child-e-\">Lorem ipsum. child</p>\n\t\t<form id=\"main\">\n\t\t\t<input>\n\t\t</form>\n\t</div>\n\t<div class=\"-s-bettermain-e-\">\n\t\t<p class=\"-s-betterchild-e-\">Lorem ipsum. betterchild</p>\n\t</div>\n</body>\n</html>\n```\n\nmain.js\n```js\n$(document).ready(function() {\n\t$('.-s-child-e-').text('jquery is working')\n\t$('#-s-child-e-').attr('message', 'child')\n});\n```\n\nAfter CSS-Selector-Minifier\n\nstyle.css\n```css\n.ae {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n#ad {\n background: grey;\n font-size: 21px;\n opacity: 0.51;\n}\n\n.ac, .aa {\n background: yellow;\n font-size: 10px;\n opacity: 0.5;\n}\n\n.ab .ae {\n color: grey;\n}\n```\n\nindex.html\n```html\n<!DOCTYPE html>\n<html>\n<head>\n\t<meta charset=\"utf-8\">\n\t<meta name=\"viewport\" content=\"width=device-width, initial-scale=1\">\n\t<title>Test</title>\n</head>\n<body>\n\t<div class=\"ae\">\n\t\t<p id=\"ab\">Lorem ipsum. child</p>\n\t\t<form id=\"main\">\n\t\t\t<input>\n\t\t</form>\n\t</div>\n\t<div class=\"ac\">\n\t\t<p class=\"aa\">Lorem ipsum. betterchild</p>\n\t</div>\n</body>\n</html>\n```\n\nmain.js\n```js\n$(document).ready(function() {\n\t$('.ab').text('jquery is working')\n\t$('#ab').attr('message', 'child')\n});\n```\n\nmain.py\n```python\nfrom css_selector_minifier import Minify_CSS_Names\n\nm = Minify_CSS_Names(\n css=['src/style.css'],\n html=['src/index.html'],\n js=['src/main.js'],\n start_prefix='-s-',\n end_prefix='-e-',\n min_letters=2\n)\n\nm.Minify()\n\n```\n\nsrc folder after running Minify_CSS_Names with default backup arg in m.Replace_CSS_Selectors_With_Minifed()\n\n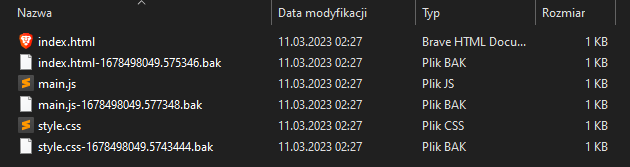\n\nThis will replace the CSS selectors in your files with minified selectors.\n\nBy default, the package will back up your files before replacing the selectors. You can disable this by passing backup=False to the Replace_CSS_Selectors_With_Minifed method.\n\n# Note\n\nYou can use glob for paths to files\n\n```python\nfrom css_selector_minifier import Minify_CSS_Names\nimport glob\n\nm = Minify_CSS_Names(\n css=glob.glob('src/*.css'),\n html=glob.glob('src/*.html'),\n js=glob.glob('src/*.js'),\n start_prefix='-s-',\n end_prefix='-e-',\n min_letters=2\n)\n\nm.Minify()\n```\n",
"bugtrack_url": null,
"license": "",
"summary": "Minify css selectors in css, html, js files.",
"version": "2.0",
"project_urls": null,
"split_keywords": [
"python",
"css",
"html",
"js",
"minify",
"css selectors",
"selector",
"selectors",
"minify css selectors"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "328cd8d7502dfbba4d7db8fd0987164dfceaead6930f5151056ef977eec133dd",
"md5": "73cbc9ea12b74f587190daf4870f573e",
"sha256": "bd6882cb98dec587f1521523b608b8f4715ec54b5bc394ca50825f87bde20db5"
},
"downloads": -1,
"filename": "css_selector_minifier-2.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "73cbc9ea12b74f587190daf4870f573e",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 6164,
"upload_time": "2023-06-26T22:00:27",
"upload_time_iso_8601": "2023-06-26T22:00:27.482440Z",
"url": "https://files.pythonhosted.org/packages/32/8c/d8d7502dfbba4d7db8fd0987164dfceaead6930f5151056ef977eec133dd/css_selector_minifier-2.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1f5a7d2d69b2fe30771631962df3974d7118f7f7f8c071ce35b5210b1142c85c",
"md5": "c930eec1588ed718885dfc7f193d7535",
"sha256": "7c0130ec2fca92e8649d7bb1f3090da7beb46f0080798aec9a88146f8373e9dd"
},
"downloads": -1,
"filename": "css_selector_minifier-2.0.tar.gz",
"has_sig": false,
"md5_digest": "c930eec1588ed718885dfc7f193d7535",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 5814,
"upload_time": "2023-06-26T22:00:29",
"upload_time_iso_8601": "2023-06-26T22:00:29.557868Z",
"url": "https://files.pythonhosted.org/packages/1f/5a/7d2d69b2fe30771631962df3974d7118f7f7f8c071ce35b5210b1142c85c/css_selector_minifier-2.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-06-26 22:00:29",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "css-selector-minifier"
}