Name | ctk-listbox-typed JSON |
Version |
1.5.0
JSON |
| download |
home_page | None |
Summary | Customtkinter Listbox widget (with typing) |
upload_time | 2024-10-24 13:34:42 |
maintainer | None |
docs_url | None |
author | Akash Bora |
requires_python | >=3.12 |
license | None |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# CTkListbox
This is a **listbox widget** for customtkinter, works just like the tkinter listbox.
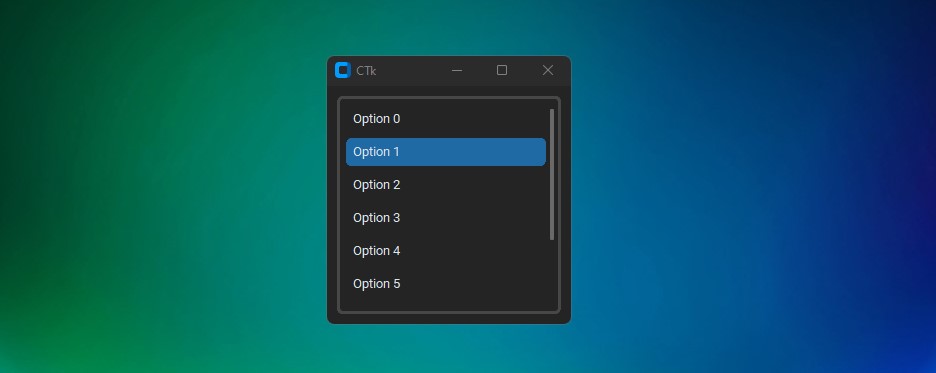
## Installation
> NOTE: This is a fork of the original CTkListbox library by Akascape, which fixes some bugs (that would otherwise cause the widget to be hardly usable), adds typing and a few other features.
```
pip install ctk_listbox
```
### [<img alt="GitHub repo size" src="https://img.shields.io/github/repo-size/Akascape/CTkListbox?&color=white&label=Download%20Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge" width="400">](https://github.com/Akascape/CTkListbox/archive/refs/heads/main.zip)
## Usage
```python
import customtkinter
from CTkListbox import *
def show_value(selected_option):
print(selected_option)
root = customtkinter.CTk()
listbox = CTkListbox(root, command=show_value)
listbox.pack(fill="both", expand=True, padx=10, pady=10)
listbox.insert(0, "Option 0")
listbox.insert(1, "Option 1")
listbox.insert(2, "Option 2")
listbox.insert(3, "Option 3")
listbox.insert(4, "Option 4")
listbox.insert(5, "Option 5")
listbox.insert(6, "Option 6")
listbox.insert(7, "Option 7")
listbox.insert("END", "Option 8")
root.mainloop()
```
## Arguments
| Parameter | Description |
|-----------| ------------|
| **master** | parent widget |
| width | **optional**, set width of the listbox |
| height | **optional**, set height of the listbox |
| fg_color | foreground color of the listbox |
| border_color | border color of the listbox frame |
| border_width | width of the border frame |
| text_color | set the color of the option text |
| hover_color | set hover color of the options |
| button_color | set color of unselected buttons |
| highlight_color | set the selected color of the option |
| font | set font of the option text |
| command | calls a command when a option is selected |
| multiple_selection | select multiple options in the listbox, `default=False`|
| listvariable | use a tkinter variable to change the listbox content |
| *other_parameters | _all other parameters of ctk_scrollable frame can be passed_ |
## Methods
- **.insert(index, option)**
add new option to the listbox
- **.get()**
get the selected option(s)
- **.delete(index)**
delete any option from the listbox. `.delete("all")` deletes all options
- **.size()**
get the size of the listbox
- **.activate(index)**
activate any option
- **.deactivate(index)**
deactivate any option
- **.curselection()**
returns indexes of selected options
- **.configure()**
change some parameters for the listbox.
- **.move_up(index)/.move_down(index)**
Reorder options in the listbox
### Thanks for visiting! Hope it will help :)
Raw data
{
"_id": null,
"home_page": null,
"name": "ctk-listbox-typed",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.12",
"maintainer_email": null,
"keywords": null,
"author": "Akash Bora",
"author_email": "melektron <matteo@elektron.work>",
"download_url": "https://files.pythonhosted.org/packages/a3/15/0a889ad8bcb616fab2fe32a8e6b5fab3f0d81f7e5a547b1a1e58fb19943f/ctk_listbox_typed-1.5.0.tar.gz",
"platform": null,
"description": "# CTkListbox\nThis is a **listbox widget** for customtkinter, works just like the tkinter listbox.\n\n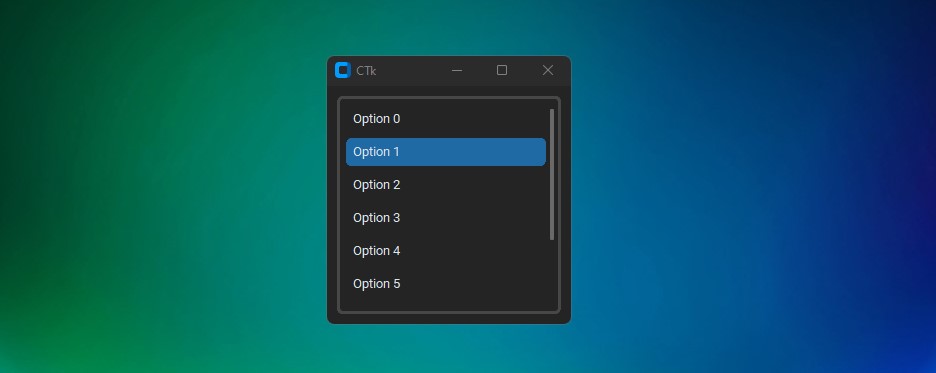\n\n## Installation\n\n> NOTE: This is a fork of the original CTkListbox library by Akascape, which fixes some bugs (that would otherwise cause the widget to be hardly usable), adds typing and a few other features.\n```\npip install ctk_listbox\n```\n### [<img alt=\"GitHub repo size\" src=\"https://img.shields.io/github/repo-size/Akascape/CTkListbox?&color=white&label=Download%20Source%20Code&logo=Python&logoColor=yellow&style=for-the-badge\" width=\"400\">](https://github.com/Akascape/CTkListbox/archive/refs/heads/main.zip)\n\n## Usage\n```python\nimport customtkinter\nfrom CTkListbox import *\n\ndef show_value(selected_option):\n print(selected_option)\n \nroot = customtkinter.CTk()\n\nlistbox = CTkListbox(root, command=show_value)\nlistbox.pack(fill=\"both\", expand=True, padx=10, pady=10)\n\nlistbox.insert(0, \"Option 0\")\nlistbox.insert(1, \"Option 1\")\nlistbox.insert(2, \"Option 2\")\nlistbox.insert(3, \"Option 3\")\nlistbox.insert(4, \"Option 4\")\nlistbox.insert(5, \"Option 5\")\nlistbox.insert(6, \"Option 6\")\nlistbox.insert(7, \"Option 7\")\nlistbox.insert(\"END\", \"Option 8\")\n\nroot.mainloop()\n```\n## Arguments\n| Parameter | Description |\n|-----------| ------------|\n| **master** | parent widget |\n| width | **optional**, set width of the listbox |\n| height | **optional**, set height of the listbox |\n| fg_color | foreground color of the listbox |\n| border_color | border color of the listbox frame |\n| border_width | width of the border frame |\n| text_color | set the color of the option text |\n| hover_color | set hover color of the options |\n| button_color | set color of unselected buttons |\n| highlight_color | set the selected color of the option |\n| font | set font of the option text |\n| command | calls a command when a option is selected |\n| multiple_selection | select multiple options in the listbox, `default=False`|\n| listvariable | use a tkinter variable to change the listbox content |\n| *other_parameters | _all other parameters of ctk_scrollable frame can be passed_ |\n\n## Methods\n- **.insert(index, option)**\n add new option to the listbox\n- **.get()**\n get the selected option(s)\n- **.delete(index)**\n delete any option from the listbox. `.delete(\"all\")` deletes all options\n- **.size()**\n get the size of the listbox\n- **.activate(index)**\n activate any option\n- **.deactivate(index)**\n deactivate any option\n- **.curselection()**\n returns indexes of selected options\n- **.configure()**\n change some parameters for the listbox.\n- **.move_up(index)/.move_down(index)**\n Reorder options in the listbox\n \n### Thanks for visiting! Hope it will help :)\n",
"bugtrack_url": null,
"license": null,
"summary": "Customtkinter Listbox widget (with typing)",
"version": "1.5.0",
"project_urls": {
"Homepage": "https://github.com/melektron/ctk_listbox",
"Issues": "https://github.com/melektron/ctk_listbox/issues"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "1386401fbd81fae8548c059ba81e278a94700d2a70334311b5da44c6824e1e06",
"md5": "e70db97c3182565f2878ce59ba75339d",
"sha256": "0479fdee1cd073515e579273fa05cd7714c293a83a727b7b0dcb1f0ffc85d98c"
},
"downloads": -1,
"filename": "ctk_listbox_typed-1.5.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e70db97c3182565f2878ce59ba75339d",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.12",
"size": 6591,
"upload_time": "2024-10-24T13:34:40",
"upload_time_iso_8601": "2024-10-24T13:34:40.619740Z",
"url": "https://files.pythonhosted.org/packages/13/86/401fbd81fae8548c059ba81e278a94700d2a70334311b5da44c6824e1e06/ctk_listbox_typed-1.5.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "a3150a889ad8bcb616fab2fe32a8e6b5fab3f0d81f7e5a547b1a1e58fb19943f",
"md5": "7c7711cc01188f32efa5f43195e38589",
"sha256": "435e54108302549e6e75f226754bab5701d8550687848075adf75b2cf4a7e15d"
},
"downloads": -1,
"filename": "ctk_listbox_typed-1.5.0.tar.gz",
"has_sig": false,
"md5_digest": "7c7711cc01188f32efa5f43195e38589",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.12",
"size": 7281,
"upload_time": "2024-10-24T13:34:42",
"upload_time_iso_8601": "2024-10-24T13:34:42.055187Z",
"url": "https://files.pythonhosted.org/packages/a3/15/0a889ad8bcb616fab2fe32a8e6b5fab3f0d81f7e5a547b1a1e58fb19943f/ctk_listbox_typed-1.5.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-10-24 13:34:42",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "melektron",
"github_project": "ctk_listbox",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "ctk-listbox-typed"
}