Name | cv2-enumerate-cameras JSON |
Version |
1.1.18.2
JSON |
| download |
home_page | None |
Summary | Enumerate / List / Find / Detect / Search index for opencv VideoCapture. |
upload_time | 2024-12-26 13:13:47 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.6 |
license | MIT License Copyright (c) 2024 Yu He Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
opencv
cv2
enumerate
camera
video capture
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
<div align="center">
# cv2_enumerate_cameras



Retrieve camera's name, VID, PID, and the corresponding OpenCV index.
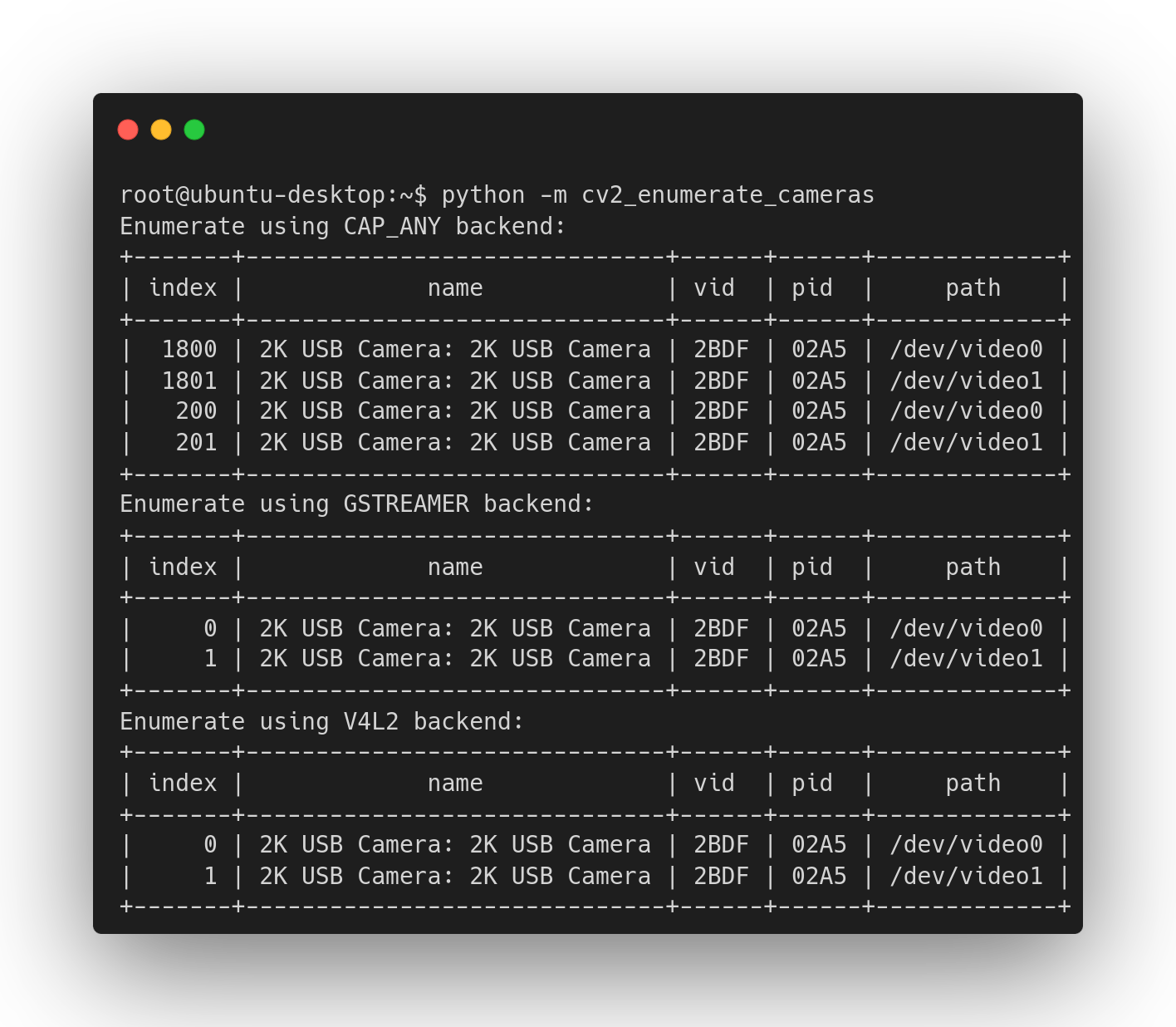
</div>
## Installation
### Install from PyPI
```commandline
pip install cv2_enumerate_cameras
```
### Install from Source
```commandline
pip install git+https://github.com/chinaheyu/cv2_enumerate_cameras.git
```
## Example
### Run as Script
We have provided a simple script that prints out information for all cameras. Open a shell and simply run:
```commandline
python -m cv2_enumerate_cameras
```
### Supported Backends
Since OpenCV supports many different backends, not all of them provide support for camera enumeration. The following code demonstrates how to retrieve the names of the supported backends.
```python
from cv2.videoio_registry import getBackendName
from cv2_enumerate_cameras import supported_backends
for backend in supported_backends:
print(getBackendName(backend))
```
Currently supported backends on windows:
- Microsoft Media Foundation (CAP_MSMF)
- DirectShow (CAP_DSHOW)
Currently supported backends on linux:
- GStreamer (CAP_GSTREAMER)
- V4L/V4L2 (CAP_V4L2)
### Enumerate Cameras
This is an example showing how to enumerate cameras.
```python
from cv2_enumerate_cameras import enumerate_cameras
for camera_info in enumerate_cameras():
print(f'{camera_info.index}: {camera_info.name}')
```
The `enumerate_cameras(apiPreference: int = CAP_ANY)` function comes with the default parameter `CAP_ANY`, and you will receive output similar to the following:
```
1400: HD Webcam
...
700: HD Webcam
701: OBS Virtual Camera
...
```
These indices may seem strange, since [opencv defaults to using the high digits of index to represent the backend](https://github.com/opencv/opencv/blob/4.x/modules/videoio/src/cap.cpp#L245). For example, 701 indicates the second camera on the DSHOW backend (700).
You can also select a supported backend for enumerating camera devices.
Here's an example of enumerating camera devices via the `CAP_MSMF` backend on windows.
```python
import cv2
from cv2_enumerate_cameras import enumerate_cameras
for camera_info in enumerate_cameras(cv2.CAP_MSMF):
print(f'{camera_info.index}: {camera_info.name}')
```
Output:
```
0: HD Webcam
...
```
Once you have found the target camera, you can create a `cv2.VideoCapture` by its **index** and **backend** properties.
```pycon
cap = cv2.VideoCapture(camera_info.index, camera_info.backend)
```
When using `CAP_ANY` as an option for the `enumerate_cameras` function, the backend parameter can be omitted. However, it is highly recommended to pass it along when creating a `VideoCapture`.
### Camera Info
The `cv2_enumerate_cameras.enumerate_cameras()` function will return a list of `CameraInfo` objects, each containing information about a specific camera.
```pycon
def enumerate_cameras(apiPreference: int = CAP_ANY) -> list[CameraInfo]:
...
```
- `CameraInfo.index`: Camera index for creating `cv2.VideoCapture`ach containing all the information about a camera;
- `CameraInfo.name`: Camera name;
- `CameraInfo.path`: Camera device path;
- `CameraInfo.vid`: Vendor identifier;
- `CameraInfo.pid`: Product identifier;
- `CameraInfo.backend`: Camera backend.
### Find Camera by Vendor and Product Identifier
This example demonstrates how to automatically select a camera based on its VID and PID and create a `VideoCapture` object.
```python
import cv2
from cv2_enumerate_cameras import enumerate_cameras
# define a function to search for a camera
def find_camera(vid, pid, apiPreference=cv2.CAP_ANY):
for i in enumerate_cameras(apiPreference):
if i.vid == vid and i.pid == pid:
return cv2.VideoCapture(i.index, i.backend)
return None
# find the camera with VID 0x04F2 and PID 0xB711
cap = find_camera(0x04F2, 0xB711)
# read and display the camera frame
while True:
result, frame = cap.read()
if not result:
break
cv2.imshow('frame', frame)
if cv2.waitKey(1) == ord('q'):
break
```
## TODO
Currently, support for MacOS is in the planning stage, and pull requests are very welcome.
- [x] Windows Support
- [x] Linux Support
- [ ] MacOS Support
Raw data
{
"_id": null,
"home_page": null,
"name": "cv2-enumerate-cameras",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": null,
"keywords": "opencv, cv2, enumerate, camera, video capture",
"author": null,
"author_email": "Yu He <chinaheyu@outlook.com>",
"download_url": "https://files.pythonhosted.org/packages/37/02/c86273478f9dffbcedceedbdb7add459f07c58950d627ec1a6c62839be1a/cv2_enumerate_cameras-1.1.18.2.tar.gz",
"platform": null,
"description": "<div align=\"center\">\r\n\r\n# cv2_enumerate_cameras\r\n\r\n\r\n\r\n\r\n\r\nRetrieve camera's name, VID, PID, and the corresponding OpenCV index.\r\n\r\n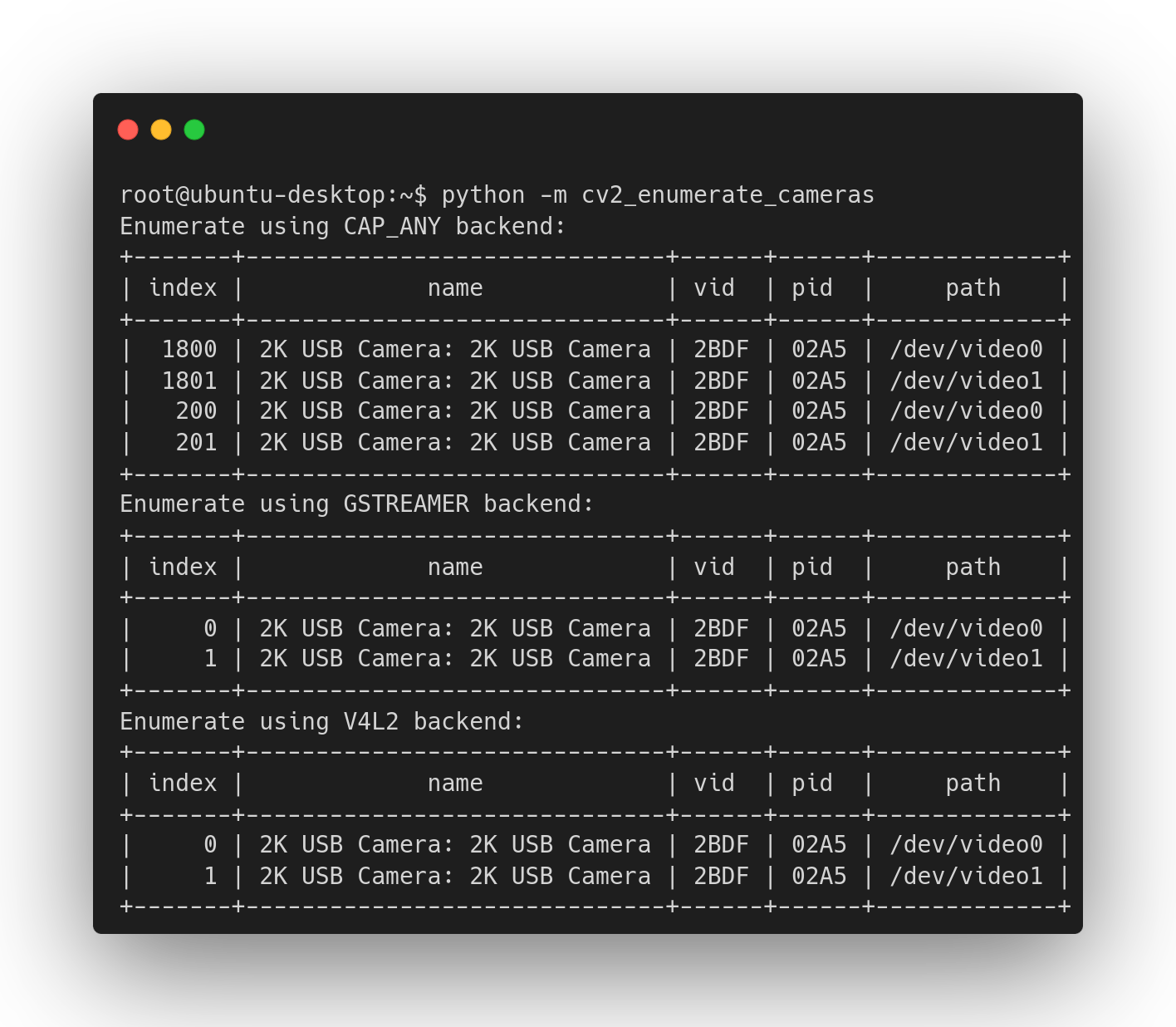\r\n\r\n</div>\r\n\r\n## Installation\r\n\r\n### Install from PyPI\r\n\r\n```commandline\r\npip install cv2_enumerate_cameras\r\n```\r\n\r\n### Install from Source\r\n\r\n```commandline\r\npip install git+https://github.com/chinaheyu/cv2_enumerate_cameras.git\r\n```\r\n\r\n## Example\r\n\r\n### Run as Script\r\n\r\nWe have provided a simple script that prints out information for all cameras. Open a shell and simply run:\r\n\r\n```commandline\r\npython -m cv2_enumerate_cameras\r\n```\r\n\r\n### Supported Backends\r\n\r\nSince OpenCV supports many different backends, not all of them provide support for camera enumeration. The following code demonstrates how to retrieve the names of the supported backends.\r\n\r\n```python\r\nfrom cv2.videoio_registry import getBackendName\r\nfrom cv2_enumerate_cameras import supported_backends\r\n\r\nfor backend in supported_backends:\r\n print(getBackendName(backend))\r\n```\r\n\r\nCurrently supported backends on windows:\r\n\r\n- Microsoft Media Foundation (CAP_MSMF)\r\n- DirectShow (CAP_DSHOW)\r\n\r\nCurrently supported backends on linux:\r\n\r\n- GStreamer (CAP_GSTREAMER)\r\n- V4L/V4L2 (CAP_V4L2)\r\n\r\n### Enumerate Cameras\r\n\r\nThis is an example showing how to enumerate cameras.\r\n\r\n```python\r\nfrom cv2_enumerate_cameras import enumerate_cameras\r\n\r\nfor camera_info in enumerate_cameras():\r\n print(f'{camera_info.index}: {camera_info.name}')\r\n```\r\n\r\nThe `enumerate_cameras(apiPreference: int = CAP_ANY)` function comes with the default parameter `CAP_ANY`, and you will receive output similar to the following:\r\n\r\n```\r\n1400: HD Webcam\r\n...\r\n700: HD Webcam\r\n701: OBS Virtual Camera\r\n...\r\n```\r\n\r\nThese indices may seem strange, since [opencv defaults to using the high digits of index to represent the backend](https://github.com/opencv/opencv/blob/4.x/modules/videoio/src/cap.cpp#L245). For example, 701 indicates the second camera on the DSHOW backend (700).\r\n\r\nYou can also select a supported backend for enumerating camera devices.\r\n\r\nHere's an example of enumerating camera devices via the `CAP_MSMF` backend on windows.\r\n\r\n```python\r\nimport cv2\r\nfrom cv2_enumerate_cameras import enumerate_cameras\r\n\r\nfor camera_info in enumerate_cameras(cv2.CAP_MSMF):\r\n print(f'{camera_info.index}: {camera_info.name}')\r\n```\r\n\r\nOutput:\r\n\r\n```\r\n0: HD Webcam\r\n...\r\n```\r\n\r\nOnce you have found the target camera, you can create a `cv2.VideoCapture` by its **index** and **backend** properties.\r\n\r\n```pycon\r\ncap = cv2.VideoCapture(camera_info.index, camera_info.backend)\r\n```\r\n\r\nWhen using `CAP_ANY` as an option for the `enumerate_cameras` function, the backend parameter can be omitted. However, it is highly recommended to pass it along when creating a `VideoCapture`.\r\n\r\n### Camera Info\r\n\r\nThe `cv2_enumerate_cameras.enumerate_cameras()` function will return a list of `CameraInfo` objects, each containing information about a specific camera.\r\n\r\n```pycon\r\ndef enumerate_cameras(apiPreference: int = CAP_ANY) -> list[CameraInfo]:\r\n ...\r\n```\r\n\r\n- `CameraInfo.index`: Camera index for creating `cv2.VideoCapture`ach containing all the information about a camera;\r\n- `CameraInfo.name`: Camera name;\r\n- `CameraInfo.path`: Camera device path;\r\n- `CameraInfo.vid`: Vendor identifier;\r\n- `CameraInfo.pid`: Product identifier;\r\n- `CameraInfo.backend`: Camera backend.\r\n\r\n### Find Camera by Vendor and Product Identifier\r\n\r\nThis example demonstrates how to automatically select a camera based on its VID and PID and create a `VideoCapture` object.\r\n\r\n```python\r\nimport cv2\r\nfrom cv2_enumerate_cameras import enumerate_cameras\r\n\r\n# define a function to search for a camera\r\ndef find_camera(vid, pid, apiPreference=cv2.CAP_ANY):\r\n for i in enumerate_cameras(apiPreference):\r\n if i.vid == vid and i.pid == pid:\r\n return cv2.VideoCapture(i.index, i.backend)\r\n return None\r\n\r\n# find the camera with VID 0x04F2 and PID 0xB711\r\ncap = find_camera(0x04F2, 0xB711)\r\n\r\n# read and display the camera frame\r\nwhile True:\r\n result, frame = cap.read()\r\n if not result:\r\n break\r\n cv2.imshow('frame', frame)\r\n if cv2.waitKey(1) == ord('q'):\r\n break\r\n```\r\n\r\n## TODO\r\n\r\nCurrently, support for MacOS is in the planning stage, and pull requests are very welcome.\r\n\r\n- [x] Windows Support\r\n- [x] Linux Support\r\n- [ ] MacOS Support\r\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2024 Yu He Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. ",
"summary": "Enumerate / List / Find / Detect / Search index for opencv VideoCapture.",
"version": "1.1.18.2",
"project_urls": {
"Homepage": "https://github.com/chinaheyu/cv2_enumerate_cameras",
"Issues": "https://github.com/chinaheyu/cv2_enumerate_cameras/issues"
},
"split_keywords": [
"opencv",
" cv2",
" enumerate",
" camera",
" video capture"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "099f645f58630aa5fad0598395fd2293dbd6a46512310a0cb591047ab10988e5",
"md5": "489d10a40cec179769a77f00c77bc432",
"sha256": "b002fee99f684b796a3e609de700fc8235657b778d86fd1c55feb1c67a253c85"
},
"downloads": -1,
"filename": "cv2_enumerate_cameras-1.1.18.2-cp32-abi3-win_amd64.whl",
"has_sig": false,
"md5_digest": "489d10a40cec179769a77f00c77bc432",
"packagetype": "bdist_wheel",
"python_version": "cp32",
"requires_python": ">=3.6",
"size": 19861,
"upload_time": "2024-12-26T13:13:43",
"upload_time_iso_8601": "2024-12-26T13:13:43.719938Z",
"url": "https://files.pythonhosted.org/packages/09/9f/645f58630aa5fad0598395fd2293dbd6a46512310a0cb591047ab10988e5/cv2_enumerate_cameras-1.1.18.2-cp32-abi3-win_amd64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "027786696e0617d38d247d9384c5ec8979efb4b3de9715a1ae722335400e49a5",
"md5": "b7187a0f03135c31b991a92ad0b5ec82",
"sha256": "e87f778ad59d20211a5002453557df2e98b8046a89991920d0dd0809df791cfe"
},
"downloads": -1,
"filename": "cv2_enumerate_cameras-1.1.18.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "b7187a0f03135c31b991a92ad0b5ec82",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 10191,
"upload_time": "2024-12-26T13:13:44",
"upload_time_iso_8601": "2024-12-26T13:13:44.975359Z",
"url": "https://files.pythonhosted.org/packages/02/77/86696e0617d38d247d9384c5ec8979efb4b3de9715a1ae722335400e49a5/cv2_enumerate_cameras-1.1.18.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "3702c86273478f9dffbcedceedbdb7add459f07c58950d627ec1a6c62839be1a",
"md5": "6ec6b01b2967c66334fdd84031b2a753",
"sha256": "91ba2970d9c2a80c5671a18408cc5d9403883c2013380eee06a2bc5a705f4614"
},
"downloads": -1,
"filename": "cv2_enumerate_cameras-1.1.18.2.tar.gz",
"has_sig": false,
"md5_digest": "6ec6b01b2967c66334fdd84031b2a753",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 9659,
"upload_time": "2024-12-26T13:13:47",
"upload_time_iso_8601": "2024-12-26T13:13:47.256193Z",
"url": "https://files.pythonhosted.org/packages/37/02/c86273478f9dffbcedceedbdb7add459f07c58950d627ec1a6c62839be1a/cv2_enumerate_cameras-1.1.18.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-26 13:13:47",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "chinaheyu",
"github_project": "cv2_enumerate_cameras",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "cv2-enumerate-cameras"
}