# Cyclical
[](https://github.com/jojoee/cyclical/actions/workflows/continuous-integration.yml)
[](https://github.com/jojoee/cyclical/actions/workflows/continuous-delivery.yml)
[](https://pypi.python.org/pypi/cyclical/)
[](https://opensource.org/licenses/MIT)
[](https://codecov.io/gh/jojoee/cyclical)
Encode item list into "cyclical"
## Installation
```
pip install cyclical
# or
git clone https://github.com/jojoee/cyclical
cd cyclical
python setup.py install
```
## Usage
```python
from cyclical import cyclical
n_rows = 1000
n_hrs = 24
hrs = [item % n_hrs for item in list(range(0, n_rows, 1))]
encoded_hrs = cyclical.encode(hrs, n_hrs)
print(encoded_hrs)
"""
([0.0, 0.25881904510252074, 0.49999999999999994, 0.7071067811865476, 0.8660254037844386,
0.9659258262890682, 1.0, 0.9659258262890683, 0.8660254037844387, 0.7071067811865476,
0.5000000000000003, 0.258819045102521, 1.2246467991473532e-16, -0.25881904510252035,
-0.4999999999999997, ...
"""
```
## Real use case
TLTR: normalize cyclical data (e.g. month number [0-11], hour number [0, 23]) by mapping them into sin and cos of 1-radius-circle
2 years ago while I was doing the “ocean current prediction model”. From the background knowledge of its nature which the ocean current has a strong relation with wind speed and wind speed also based on the season. So, I try to give the model “month number” which starts with 0 and ends with 11.
With the deep learning model, I have to normalize data into [0, 1] which 1 refers to the maximum magnitude. There have many ways to normalize data such as min/max, mean/std, and other normalization but it can’t apply to this “month number” data.
“Month number” has a cyclical characteristic, so month-number-11 can’t be compared with month-number-0 as it showed, Thus I have to represent “month number” with other normalization method instead which is “cyclical” in this module.
```python
import pandas as pd
from cyclical import cyclical
import math
import matplotlib.pyplot as plt
%matplotlib inline
n_rows = 1000
n_hrs = 24
hrs = [item % n_hrs for item in list(range(0, n_rows, 1))]
encoded_hrs = cyclical.encode(hrs, n_hrs)
# print(encoded_hrs)
n_months = 12
months = [item % n_months for item in list(range(0, n_rows, 1))]
encoded_months = cyclical.encode(months, n_months)
# datframe
df = pd.DataFrame({
# hr
'hr_sin': encoded_hrs[0],
'hr_cos': encoded_hrs[1],
# month
'month_sin': encoded_months[0],
'month_cos': encoded_months[1],
})
display(df)
# plot
n_samples = math.floor(n_rows * 0.1)
df.sample(n_samples).plot.scatter('hr_sin', 'hr_cos').set_aspect('equal')
plt.show()
# plot
df.sample(n_samples).plot.scatter('month_sin', 'month_cos').set_aspect('equal')
plt.show()
```
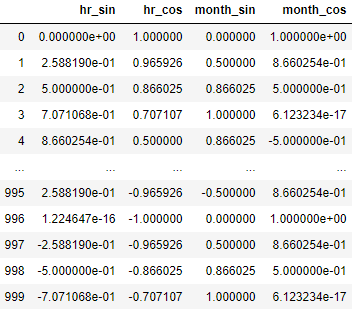
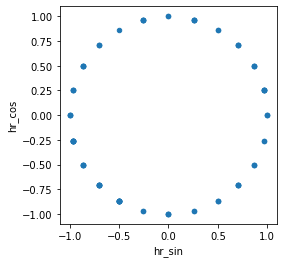
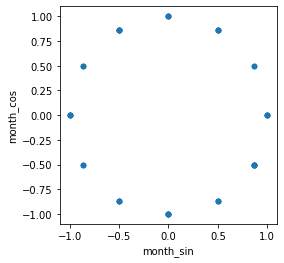
## Reference
- [Encoding cyclical continuous features - 24-hour time](https://ianlondon.github.io/blog/encoding-cyclical-features-24hour-time/)
Raw data
{
"_id": null,
"home_page": "https://github.com/jojoee/cyclical",
"name": "cyclical",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "cyclical,cyclic,normalization,normalize",
"author": "Nathachai Thongniran",
"author_email": "inid3a@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/b3/ce/abd48b33762a2f0d9245bdd1df70f7e36ca76deb9970860683a1638b5cc6/cyclical-1.0.2.tar.gz",
"platform": null,
"description": "# Cyclical\n\n[](https://github.com/jojoee/cyclical/actions/workflows/continuous-integration.yml)\n[](https://github.com/jojoee/cyclical/actions/workflows/continuous-delivery.yml)\n\n[](https://pypi.python.org/pypi/cyclical/)\n[](https://opensource.org/licenses/MIT)\n[](https://codecov.io/gh/jojoee/cyclical)\n\nEncode item list into \"cyclical\"\n\n## Installation\n\n```\npip install cyclical\n\n# or\ngit clone https://github.com/jojoee/cyclical\ncd cyclical\npython setup.py install\n```\n\n## Usage\n\n```python\nfrom cyclical import cyclical\n\nn_rows = 1000\nn_hrs = 24\nhrs = [item % n_hrs for item in list(range(0, n_rows, 1))]\nencoded_hrs = cyclical.encode(hrs, n_hrs)\nprint(encoded_hrs)\n\n\"\"\"\n([0.0, 0.25881904510252074, 0.49999999999999994, 0.7071067811865476, 0.8660254037844386,\n0.9659258262890682, 1.0, 0.9659258262890683, 0.8660254037844387, 0.7071067811865476,\n0.5000000000000003, 0.258819045102521, 1.2246467991473532e-16, -0.25881904510252035,\n-0.4999999999999997, ...\n\"\"\"\n```\n\n## Real use case\n\nTLTR: normalize cyclical data (e.g. month number [0-11], hour number [0, 23]) by mapping them into sin and cos of 1-radius-circle\n\n2 years ago while I was doing the \u201cocean current prediction model\u201d. From the background knowledge of its nature which the ocean current has a strong relation with wind speed and wind speed also based on the season. So, I try to give the model \u201cmonth number\u201d which starts with 0 and ends with 11.\n\nWith the deep learning model, I have to normalize data into [0, 1] which 1 refers to the maximum magnitude. There have many ways to normalize data such as min/max, mean/std, and other normalization but it can\u2019t apply to this \u201cmonth number\u201d data.\n\n\u201cMonth number\u201d has a cyclical characteristic, so month-number-11 can\u2019t be compared with month-number-0 as it showed, Thus I have to represent \u201cmonth number\u201d with other normalization method instead which is \u201ccyclical\u201d in this module.\n\n```python\nimport pandas as pd\nfrom cyclical import cyclical\nimport math\nimport matplotlib.pyplot as plt\n%matplotlib inline\n\nn_rows = 1000\nn_hrs = 24\nhrs = [item % n_hrs for item in list(range(0, n_rows, 1))]\nencoded_hrs = cyclical.encode(hrs, n_hrs)\n# print(encoded_hrs)\n\nn_months = 12\nmonths = [item % n_months for item in list(range(0, n_rows, 1))]\nencoded_months = cyclical.encode(months, n_months)\n\n# datframe\ndf = pd.DataFrame({\n # hr\n 'hr_sin': encoded_hrs[0],\n 'hr_cos': encoded_hrs[1],\n\n # month\n 'month_sin': encoded_months[0],\n 'month_cos': encoded_months[1],\n})\ndisplay(df)\n\n# plot\nn_samples = math.floor(n_rows * 0.1)\ndf.sample(n_samples).plot.scatter('hr_sin', 'hr_cos').set_aspect('equal')\nplt.show()\n\n# plot\ndf.sample(n_samples).plot.scatter('month_sin', 'month_cos').set_aspect('equal')\nplt.show()\n```\n\n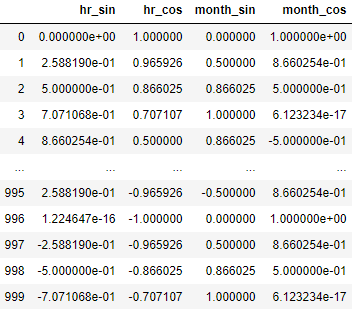\n\n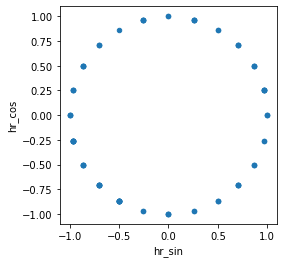\n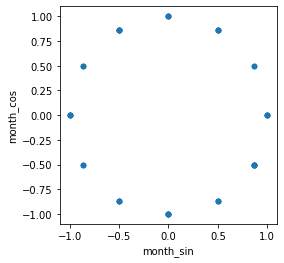\n\n## Reference\n- [Encoding cyclical continuous features - 24-hour time](https://ianlondon.github.io/blog/encoding-cyclical-features-24hour-time/)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Encode item list into cyclical",
"version": "1.0.2",
"project_urls": {
"Homepage": "https://github.com/jojoee/cyclical"
},
"split_keywords": [
"cyclical",
"cyclic",
"normalization",
"normalize"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "cefbce3323c05b54a7a4a96afaf708fa63998950909333fbda8d8245e0468070",
"md5": "21716c6574a5971caec79bda6441d8b8",
"sha256": "45818f66a745ec67d061621c47665f756ff4f45fd0f5ab0866cd4f9166359f20"
},
"downloads": -1,
"filename": "cyclical-1.0.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "21716c6574a5971caec79bda6441d8b8",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 3988,
"upload_time": "2023-08-27T13:45:09",
"upload_time_iso_8601": "2023-08-27T13:45:09.610472Z",
"url": "https://files.pythonhosted.org/packages/ce/fb/ce3323c05b54a7a4a96afaf708fa63998950909333fbda8d8245e0468070/cyclical-1.0.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "b3ceabd48b33762a2f0d9245bdd1df70f7e36ca76deb9970860683a1638b5cc6",
"md5": "1199755ad1287f62bef03a4a1f99902a",
"sha256": "2199267d994866186efc13e4ad1802cfa8ef6eb81cd6f2fa4268f08a5dd3ffd3"
},
"downloads": -1,
"filename": "cyclical-1.0.2.tar.gz",
"has_sig": false,
"md5_digest": "1199755ad1287f62bef03a4a1f99902a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 3896,
"upload_time": "2023-08-27T13:45:10",
"upload_time_iso_8601": "2023-08-27T13:45:10.686968Z",
"url": "https://files.pythonhosted.org/packages/b3/ce/abd48b33762a2f0d9245bdd1df70f7e36ca76deb9970860683a1638b5cc6/cyclical-1.0.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-08-27 13:45:10",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "jojoee",
"github_project": "cyclical",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "cyclical"
}