Name | dask-ee JSON |
Version |
0.0.3
JSON |
| download |
home_page | None |
Summary | Google Earth Engine FeatureCollections via Dask DataFrames. |
upload_time | 2024-06-25 15:48:20 |
maintainer | None |
docs_url | None |
author | Aaron Zuspan |
requires_python | >=3.8 |
license | Apache-2.0 |
keywords |
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# dask-ee
_Google Earth Engine Feature Collections via Dask DataFrames._
[](https://github.com/alxmrs/dask-ee/actions/workflows/ci-build.yml)
[](https://pypi.python.org/pypi/dask-ee)
[](https://pepy.tech/project/dask-ee)
## How to use
Install with pip:
```shell
pip install dask-ee
```
Then, authenticate Earth Engine:
```shell
earthengine authenticate
```
In your Python environment, you may now import the library:
```python
import ee
import dask_ee
```
You'll need to initialize Earth Engine before working with data:
```python
ee.Initialize()
```
From here, you can read Earth Engine FeatureCollections like they are DataFrames:
```python
df = dask_ee.read_ee("WRI/GPPD/power_plants")
df.head()
```
These work like Pandas DataFrames, but they are lazily evaluated via [Dask](https://dask.org/).
Feel free to do any analysis you wish. For example:
```python
# Thanks @aazuspan, https://www.aazuspan.dev/blog/dask_featurecollection
(
df[df.comm_year.gt(1940) & df.country.eq("USA") & df.fuel1.isin(["Coal", "Wind"])]
.astype({"comm_year": int})
.drop(columns=["geo"])
.groupby(["comm_year", "fuel1"])
.agg({"capacitymw": "sum"})
.reset_index()
.sort_values(by=["comm_year"])
.compute(scheduler="threads")
.pivot_table(index="comm_year", columns="fuel1", values="capacitymw", fill_value=0)
.plot()
)
```
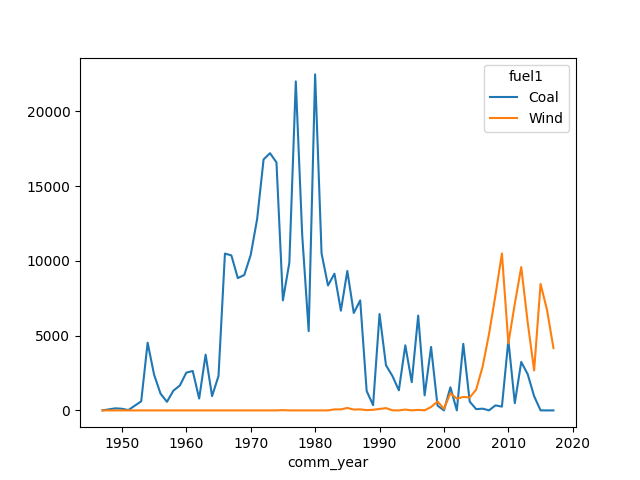
There are a few other useful things you can do.
For one, you may pass in a pre-processed `ee.FeatureCollection`. This allows full utilization
of the Earth Engine API.
```python
fc = (
ee.FeatureCollection("WRI/GPPD/power_plants")
.filter(ee.Filter.gt("comm_year", 1940))
.filter(ee.Filter.eq("country", "USA"))
)
df = dask_ee.read_ee(fc)
```
In addition, you may change the `chunksize`, which controls how many rows are included in each
Dask partition.
```python
df = dask_ee.read_ee("WRI/GPPD/power_plants", chunksize=7_000)
df.head()
```
## Contributing
Contributions are welcome. A good way to start is to check out open [issues](https://github.com/alxmrs/dask-ee/issues)
or file a new one. We're happy to review pull requests, too.
Before writing code, please install the development dependencies (after cloning the repo):
```shell
pip install -e ".[dev]"
```
## License
```
Copyright 2024 Alexander S Merose
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
https://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
```
Some sources are re-distributed from Google LLC via https://github.com/google/Xee (also Apache-2.0 License) with and
without modification. These files are subject to the original copyright; they include the original license header
comment as well as a note to indicate modifications (when appropriate).
Raw data
{
"_id": null,
"home_page": null,
"name": "dask-ee",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": null,
"author": "Aaron Zuspan",
"author_email": "Alexander Merose <al@merose.com>",
"download_url": "https://files.pythonhosted.org/packages/7d/79/9b5d238a4158c339c98613fdd0c6843684ad0bcb314f3858832b80708a76/dask_ee-0.0.3.tar.gz",
"platform": null,
"description": "# dask-ee\n\n_Google Earth Engine Feature Collections via Dask DataFrames._\n\n[](https://github.com/alxmrs/dask-ee/actions/workflows/ci-build.yml)\n[](https://pypi.python.org/pypi/dask-ee)\n[](https://pepy.tech/project/dask-ee)\n\n## How to use\n\nInstall with pip:\n```shell\npip install dask-ee\n```\n\nThen, authenticate Earth Engine:\n```shell\nearthengine authenticate\n```\n\nIn your Python environment, you may now import the library:\n\n```python\nimport ee\nimport dask_ee\n```\n\nYou'll need to initialize Earth Engine before working with data:\n```python\nee.Initialize()\n```\n\nFrom here, you can read Earth Engine FeatureCollections like they are DataFrames:\n```python\ndf = dask_ee.read_ee(\"WRI/GPPD/power_plants\")\ndf.head()\n```\nThese work like Pandas DataFrames, but they are lazily evaluated via [Dask](https://dask.org/).\n\nFeel free to do any analysis you wish. For example:\n```python\n# Thanks @aazuspan, https://www.aazuspan.dev/blog/dask_featurecollection\n(\n df[df.comm_year.gt(1940) & df.country.eq(\"USA\") & df.fuel1.isin([\"Coal\", \"Wind\"])]\n .astype({\"comm_year\": int})\n .drop(columns=[\"geo\"])\n .groupby([\"comm_year\", \"fuel1\"])\n .agg({\"capacitymw\": \"sum\"})\n .reset_index()\n .sort_values(by=[\"comm_year\"])\n .compute(scheduler=\"threads\")\n .pivot_table(index=\"comm_year\", columns=\"fuel1\", values=\"capacitymw\", fill_value=0)\n .plot()\n)\n```\n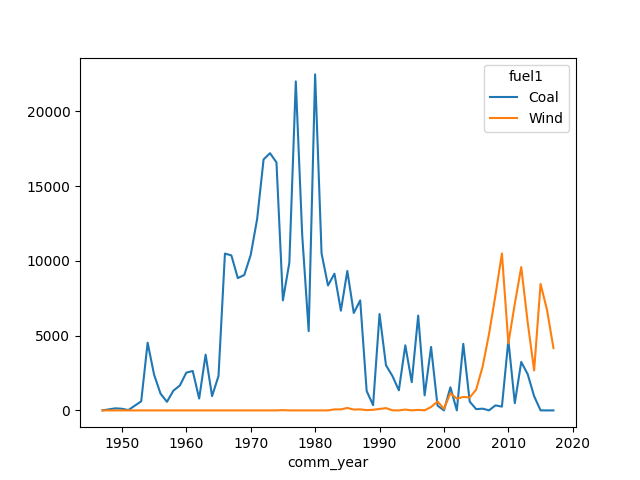\n\nThere are a few other useful things you can do. \n\nFor one, you may pass in a pre-processed `ee.FeatureCollection`. This allows full utilization\nof the Earth Engine API.\n\n```python\nfc = (\n ee.FeatureCollection(\"WRI/GPPD/power_plants\")\n .filter(ee.Filter.gt(\"comm_year\", 1940))\n .filter(ee.Filter.eq(\"country\", \"USA\"))\n)\ndf = dask_ee.read_ee(fc)\n```\n\nIn addition, you may change the `chunksize`, which controls how many rows are included in each\nDask partition.\n```python\ndf = dask_ee.read_ee(\"WRI/GPPD/power_plants\", chunksize=7_000)\ndf.head()\n```\n\n## Contributing\n\nContributions are welcome. A good way to start is to check out open [issues](https://github.com/alxmrs/dask-ee/issues)\nor file a new one. We're happy to review pull requests, too.\n\nBefore writing code, please install the development dependencies (after cloning the repo): \n```shell\npip install -e \".[dev]\"\n```\n\n## License\n```\nCopyright 2024 Alexander S Merose\n\nLicensed under the Apache License, Version 2.0 (the \"License\");\nyou may not use this file except in compliance with the License.\nYou may obtain a copy of the License at\n\n https://www.apache.org/licenses/LICENSE-2.0\n\nUnless required by applicable law or agreed to in writing, software\ndistributed under the License is distributed on an \"AS IS\" BASIS,\nWITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.\nSee the License for the specific language governing permissions and\nlimitations under the License.\n```\n\nSome sources are re-distributed from Google LLC via https://github.com/google/Xee (also Apache-2.0 License) with and\nwithout modification. These files are subject to the original copyright; they include the original license header\ncomment as well as a note to indicate modifications (when appropriate).\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Google Earth Engine FeatureCollections via Dask DataFrames.",
"version": "0.0.3",
"project_urls": {
"Homepage": "https://github.com/alxmrs/dask-ee",
"Issues": "https://github.com/alxmrs/dask-ee/issues"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "9d75397ae952231e00c9aaee7b2be87610829ff8dbed067a6097f6e1d013876c",
"md5": "df1b0280b57174afbee8f742d2a2ba67",
"sha256": "9fb8085bd3f32bbd547c9ae8e1266e3f8d69153674d88ecbe7a256950a00dacd"
},
"downloads": -1,
"filename": "dask_ee-0.0.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "df1b0280b57174afbee8f742d2a2ba67",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 10462,
"upload_time": "2024-06-25T15:48:19",
"upload_time_iso_8601": "2024-06-25T15:48:19.089421Z",
"url": "https://files.pythonhosted.org/packages/9d/75/397ae952231e00c9aaee7b2be87610829ff8dbed067a6097f6e1d013876c/dask_ee-0.0.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7d799b5d238a4158c339c98613fdd0c6843684ad0bcb314f3858832b80708a76",
"md5": "0da0ec158cc46bd6f001b23ce5439c28",
"sha256": "86383caacd58cd20ce85c69357daff47a511396a00017e6b580670fa7751504a"
},
"downloads": -1,
"filename": "dask_ee-0.0.3.tar.gz",
"has_sig": false,
"md5_digest": "0da0ec158cc46bd6f001b23ce5439c28",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 51683,
"upload_time": "2024-06-25T15:48:20",
"upload_time_iso_8601": "2024-06-25T15:48:20.837328Z",
"url": "https://files.pythonhosted.org/packages/7d/79/9b5d238a4158c339c98613fdd0c6843684ad0bcb314f3858832b80708a76/dask_ee-0.0.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-25 15:48:20",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "alxmrs",
"github_project": "dask-ee",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "dask-ee"
}