# 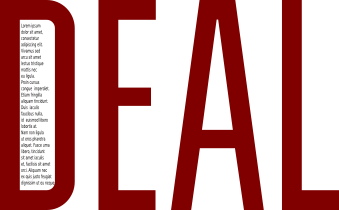
[](https://cloud.drone.io/life4/deal)
[](https://pypi.python.org/pypi/deal)
[](https://pypi.python.org/pypi/deal)
A Python library for [design by contract](https://en.wikipedia.org/wiki/Design_by_contract) (DbC) and checking values, exceptions, and side-effects. In a nutshell, deal empowers you to write bug-free code. By adding a few decorators to your code, you get for free tests, static analysis, formal verification, and much more. Read [intro](https://deal.readthedocs.io/basic/intro.html) to get started.
## Features
* [Classic DbC: precondition, postcondition, invariant.][values]
* [Tracking exceptions and side-effects.][exceptions]
* [Property-based testing.][tests]
* [Static checker.][linter]
* Integration with pytest, flake8, sphinx, and hypothesis.
* Type annotations support.
* [External validators support.][validators]
* [Contracts for importing modules.][module_load]
* [Can be enabled or disabled on production.][runtime]
* [Colorless][colorless]: annotate only what you want. Hence, easy integration into an existing project.
* Colorful: syntax highlighting for every piece of code in every command.
* [Memory leaks detection][leaks]: deal makes sure that pure functions don't leave unexpected objects in the memory.
* DRY: test discovery, error messages generation.
* Partial execution: linter executes contracts to statically check possible values.
* [Formal verification][verification]: prove that your code works for all input (or find out when it doesn't).
* Zero-dependency runtime: there are some dependencies for analysis tools, but nothing of it is required on the production.
* Fast: each code change is benchmarked and profiled.
* Reliable: the library has 100% test coverage, partially verified, and runs on production by multiple companies since 2018.
[values]: https://deal.readthedocs.io/basic/values.html
[exceptions]: https://deal.readthedocs.io/basic/exceptions.html
[tests]: https://deal.readthedocs.io/basic/tests.html
[linter]: https://deal.readthedocs.io/basic/linter.html
[validators]: https://deal.readthedocs.io/details/contracts.html#external-validators
[module_load]: https://deal.readthedocs.io/details/module_load.html
[runtime]: https://deal.readthedocs.io/basic/runtime.html
[colorless]: http://journal.stuffwithstuff.com/2015/02/01/what-color-is-your-function/
[leaks]: https://deal.readthedocs.io/basic/tests.html#memory-leaks
[verification]: https://deal.readthedocs.io/basic/verification.html
## Deal in 30 seconds
```python
# the result is always non-negative
@deal.post(lambda result: result >= 0)
# the function has no side-effects
@deal.pure
def count(items: List[str], item: str) -> int:
return items.count(item)
# generate test function
test_count = deal.cases(count)
```
Now we can:
* Run `python3 -m deal lint` or `flake8` to statically check errors.
* Run `python3 -m deal test` or `pytest` to generate and run tests.
* Just use the function in the project and check errors in runtime.
Read more in the [documentation](https://deal.readthedocs.io/).
## Installation
```bash
python3 -m pip install --user 'deal[all]'
```
## Contributing
Contributions are welcome! A few ideas what you can contribute:
* Add new checks for the linter.
* Improve documentation.
* Add more tests.
* Improve performance.
* Found a bug? Fix it!
* Made an article about deal? Great! Let's add it into the `README.md`.
* Don't have time to code? No worries! Just tell your friends and subscribers about the project. More users -> more contributors -> more cool features.
To run tests locally, all you need is [task](http://taskfile.dev/). Run `task all` to run all code formatters, linters, and tests.
Thank you :heart:
Raw data
{
"_id": null,
"home_page": null,
"name": "deal",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "deal, contracts, pre, post, invariant, decorators, validation, pythonic, functional",
"author": null,
"author_email": "Gram <gram@orsinium.dev>",
"download_url": "https://files.pythonhosted.org/packages/2b/7f/50dc3e77b4bb4ea0164f8e09204b263de3ce7d2f206cd8ee8bc49a9a956b/deal-4.24.4.tar.gz",
"platform": null,
"description": "# 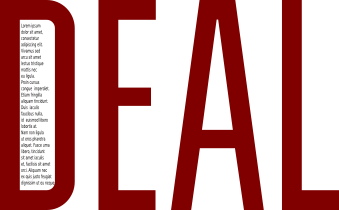\n\n[](https://cloud.drone.io/life4/deal)\n[](https://pypi.python.org/pypi/deal)\n[](https://pypi.python.org/pypi/deal)\n\nA Python library for [design by contract](https://en.wikipedia.org/wiki/Design_by_contract) (DbC) and checking values, exceptions, and side-effects. In a nutshell, deal empowers you to write bug-free code. By adding a few decorators to your code, you get for free tests, static analysis, formal verification, and much more. Read [intro](https://deal.readthedocs.io/basic/intro.html) to get started.\n\n## Features\n\n* [Classic DbC: precondition, postcondition, invariant.][values]\n* [Tracking exceptions and side-effects.][exceptions]\n* [Property-based testing.][tests]\n* [Static checker.][linter]\n* Integration with pytest, flake8, sphinx, and hypothesis.\n* Type annotations support.\n* [External validators support.][validators]\n* [Contracts for importing modules.][module_load]\n* [Can be enabled or disabled on production.][runtime]\n* [Colorless][colorless]: annotate only what you want. Hence, easy integration into an existing project.\n* Colorful: syntax highlighting for every piece of code in every command.\n* [Memory leaks detection][leaks]: deal makes sure that pure functions don't leave unexpected objects in the memory.\n* DRY: test discovery, error messages generation.\n* Partial execution: linter executes contracts to statically check possible values.\n* [Formal verification][verification]: prove that your code works for all input (or find out when it doesn't).\n* Zero-dependency runtime: there are some dependencies for analysis tools, but nothing of it is required on the production.\n* Fast: each code change is benchmarked and profiled.\n* Reliable: the library has 100% test coverage, partially verified, and runs on production by multiple companies since 2018.\n\n[values]: https://deal.readthedocs.io/basic/values.html\n[exceptions]: https://deal.readthedocs.io/basic/exceptions.html\n[tests]: https://deal.readthedocs.io/basic/tests.html\n[linter]: https://deal.readthedocs.io/basic/linter.html\n[validators]: https://deal.readthedocs.io/details/contracts.html#external-validators\n[module_load]: https://deal.readthedocs.io/details/module_load.html\n[runtime]: https://deal.readthedocs.io/basic/runtime.html\n[colorless]: http://journal.stuffwithstuff.com/2015/02/01/what-color-is-your-function/\n[leaks]: https://deal.readthedocs.io/basic/tests.html#memory-leaks\n[verification]: https://deal.readthedocs.io/basic/verification.html\n\n## Deal in 30 seconds\n\n```python\n# the result is always non-negative\n@deal.post(lambda result: result >= 0)\n# the function has no side-effects\n@deal.pure\ndef count(items: List[str], item: str) -> int:\n return items.count(item)\n\n# generate test function\ntest_count = deal.cases(count)\n```\n\nNow we can:\n\n* Run `python3 -m deal lint` or `flake8` to statically check errors.\n* Run `python3 -m deal test` or `pytest` to generate and run tests.\n* Just use the function in the project and check errors in runtime.\n\nRead more in the [documentation](https://deal.readthedocs.io/).\n\n## Installation\n\n```bash\npython3 -m pip install --user 'deal[all]'\n```\n\n## Contributing\n\nContributions are welcome! A few ideas what you can contribute:\n\n* Add new checks for the linter.\n* Improve documentation.\n* Add more tests.\n* Improve performance.\n* Found a bug? Fix it!\n* Made an article about deal? Great! Let's add it into the `README.md`.\n* Don't have time to code? No worries! Just tell your friends and subscribers about the project. More users -> more contributors -> more cool features.\n\nTo run tests locally, all you need is [task](http://taskfile.dev/). Run `task all` to run all code formatters, linters, and tests.\n\nThank you :heart:\n",
"bugtrack_url": null,
"license": null,
"summary": "**Deal** is a Python library for [design by contract][wiki] (DbC) programming.",
"version": "4.24.4",
"project_urls": {
"Documentation": "https://deal.readthedocs.io/",
"Repository": "https://github.com/life4/deal"
},
"split_keywords": [
"deal",
" contracts",
" pre",
" post",
" invariant",
" decorators",
" validation",
" pythonic",
" functional"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "7bcf4c27b6c1afc6320c79c3db756e54f337ba0c36c243a16c2c1ea54d61e8d1",
"md5": "bd2033e6c92b647a628ae7e2c173d6c4",
"sha256": "7fa4139296843fc16e5b8edd49b9a379d829113b1b4bdab0d8a7374cc826e031"
},
"downloads": -1,
"filename": "deal-4.24.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "bd2033e6c92b647a628ae7e2c173d6c4",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 206879,
"upload_time": "2024-03-23T10:26:52",
"upload_time_iso_8601": "2024-03-23T10:26:52.567628Z",
"url": "https://files.pythonhosted.org/packages/7b/cf/4c27b6c1afc6320c79c3db756e54f337ba0c36c243a16c2c1ea54d61e8d1/deal-4.24.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "2b7f50dc3e77b4bb4ea0164f8e09204b263de3ce7d2f206cd8ee8bc49a9a956b",
"md5": "9b8b81dfef33cc1e5a541fee1613bd34",
"sha256": "0470af54234cebc760346f4be1a6fdaeefe0215fc58efd630cd4b2d193e4e82e"
},
"downloads": -1,
"filename": "deal-4.24.4.tar.gz",
"has_sig": false,
"md5_digest": "9b8b81dfef33cc1e5a541fee1613bd34",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 724356,
"upload_time": "2024-03-23T10:26:56",
"upload_time_iso_8601": "2024-03-23T10:26:56.223789Z",
"url": "https://files.pythonhosted.org/packages/2b/7f/50dc3e77b4bb4ea0164f8e09204b263de3ce7d2f206cd8ee8bc49a9a956b/deal-4.24.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-23 10:26:56",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "life4",
"github_project": "deal",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "deal"
}