# Dekimashita

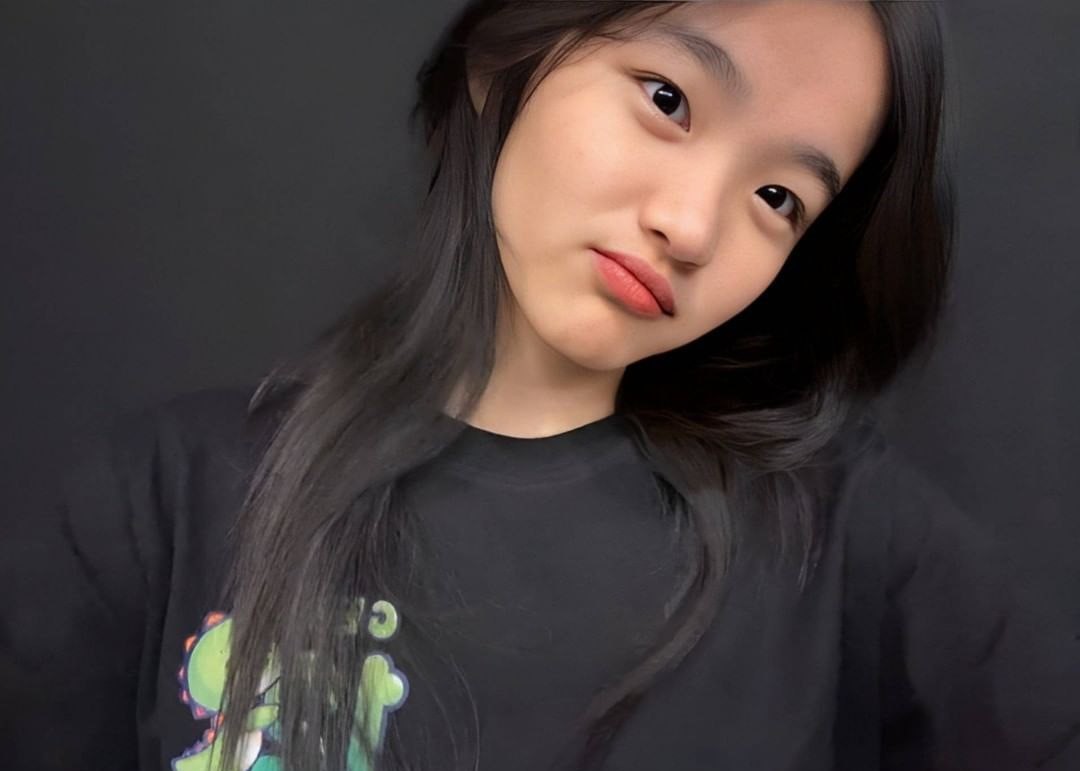
**a library containing a collection of utility functions designed to filter and process text data based on certain criteria. These functions are useful for various text processing tasks, such as removing unwanted characters, extracting specific information, or cleaning input data.**
## Features ✨
- **Alphabetic Filtering**: Easily filter out non-alphabetic characters from text data.
- **Numeric Filtering**: Quickly remove non-numeric characters from text strings.
- **Alphanumeric Filtering**: Filter text to retain only alphanumeric characters, excluding special symbols.
- **Customization**: Ability to customize the filtering criteria based on specific requirements.
- **TextCleaning**: Cleanse input text from unwanted characters to prepare it for further processing or analysis.
- **Normalization**: Standardize text data by removing irregular characters or symbols
## Requirement ⚙️
- [Python](https://www.python.org/) v3.11.6+
## Installation 🛠️
```sh
pip install dekimashita
```
## How To Usage 🤔
#### 1. Dekimashita.vdict(data, [chars])
Filter dictionary values recursively, ignoring specified characters.
Args:
data (dict or list): Data (dictionary or list containing dictionaries) to filter.
chars (list): List of characters to filter.
Returns:
dict or list: Filtered data.
### ⚠️ Sample ⚠️
```python
data = {
"university": {
"name": "Example University",
"location": "City XYZ",
"courses": [
{
"course_id": "CS101",
"title": "Introduction \n to \n Computer \n\n Science",
"lecturer": {
"name": "Dr. Alan\n Smith",
"email": "alan.smith@example.com",
"office": {
"building": "Engineering Tower",
"room_number": "123"
}
},
"students": {
"name": "John Doe",
"student_id": "123456",
"email": "john.doe@example.com",
"grades": {
"assignments": [
{
"assignment_id": "001",
"score": 95,
"comments": "Great job on the assignment!\nKeep up the good work."
},
{
"assignment_id": "002",
"score": 85,
"comments": "Your\n effort is commendable.\r\r However,\nthere is room"
}
],
"final_exam": {
"score": 88,
"comments": "Solid \nperformance overall.\n\rYour understanding of the subject"
}
}
}
}
]
}
}
```
<br>
#### without Dekimashita filter
```python
import json
data = # data_sample
with open("data.json", "w") as json_file:
json.dump(data, json_file, indent=4)
```
#### If you have a very complex dictionary and you write without using the Dekimashita filter you will get results like this
<br>
<div style="text-align: center;">
<img src="https://raw.githubusercontent.com/ryyos/ryyos/main/images/Dekimashita/nopng.png">
</div>
<br>
#### with Dekimashita filter
```python
import json
from dekimashita import Dekimashita
data = # data_sample
clear = Dekimashita.vdict(data, ['\n', '\r'])
with open("data.json", "w") as json_file:
json.dump(clear, json_file, indent=4)
```
#### By using the Dekimashita filter you get a clean dictionary like this
<br>
<div style="text-align: center;">
<img src="https://raw.githubusercontent.com/ryyos/ryyos/main/images/Dekimashita/yeee.png">
</div>
<br>
#### 2. Dekimashita.vspace(text)
Remove extra spaces from text.
Args:
text (str): Input text.
Returns:
str: Text with extra spaces removed.
### sample
```python
from dekimashita import Dekimashita
text = 'moon beautiful isn"t it'
clear = Dekimashita.vspace(text)
print('without Dekimashita filter: '+ text)
print('with Dekimashita filter: ' + clear)
```
```
# output
without Dekimashita filter: moon beautiful isn"t it
with Dekimashita filter: moon beautiful isn"t it
```
#### 3. Dekimashita.valpha(text)
Remove non-alphabetic characters (except a-z, A-Z) from text.
Args:
text (str): Input text.
Returns:
str: Filtered text containing only alphabetic characters.
### sample
```python
from dekimashita import Dekimashita
text = 'mo&on b)(*&^%$e!au!t@#$i*f!ul is!!$#n"t i)(*&^t'
clear = Dekimashita.valpha(text)
print('without Dekimashita filter: '+ text)
print('with Dekimashita filter: ' + clear)
```
```
# output
without Dekimashita filter: mo&on b)(*&^%$e!au!t@#$i*f!ul is!!$#n"t i)(*&^t
with Dekimashita filter: moon beautiful isnt it
```
#### 4. Dekimashita.vnum(text)
Remove non-numeric characters from text.
Args:
text (str): Input text.
Returns:
str: Filtered text containing only numeric characters.
### sample
```python
from dekimashita import Dekimashita
text = ' mo30on be7aut20iful i05sn"t it'
clear = Dekimashita.vnum(text)
print('without Dekimashita filter: '+ text)
print('with Dekimashita filter: ' + clear)
```
```
# output
without Dekimashita filter: mo30on be7aut20iful i05sn"t it
with Dekimashita filter: 3072005
```
#### 5. Dekimashita.vtext(text)
Remove non-alphanumeric characters (except a-z, A-Z, 0-9) from text.
Double spaces are replaced with a single space.
Args:
text (str): Input text.
Returns:
str: Filtered text containing only alphanumeric characters.
### sample
```python
from dekimashita import Dekimashita
text = 'moon \t\t bea^%$#@utiful isn"t it 30705'
clear = Dekimashita.vtext(text)
print('without Dekimashita filter: '+ text)
print('with Dekimashita filter: ' + clear)
```
```
# output
without Dekimashita filter: moon bea^%$#@utiful isn"t it 30705
with Dekimashita filter: moon beautiful isnt it 30705
```
#### 6. Dekimashita.vdir(text, separator)
"""
Remove non-alphanumeric characters (except a-z, A-Z, 0-9) from text.
Convert all letters to lowercase. Replace spaces with a specified separator.
Double separators are replaced with a single separator.
Args:
text (str): Input text.
separator (str): Separator to replace spaces (default is '_').
Returns:
str: Filtered and normalized text.
"""
### sample
```python
from dekimashita import Dekimashita
text = 'Moon Beautiful Isn"t It'
clear = Dekimashita.vdir(text)
print('without Dekimashita filter: '+ text)
print('with Dekimashita filter: ' + clear)
```
```
# output
without Dekimashita filter: Moon Beautiful Isn"t It
with Dekimashita filter: moon_beautiful_isnt_it
```
#### 7. Dekimashita.vpath(text, separator)
"""
Remove non-alphanumeric characters (except a-z, A-Z, 0-9) from text.
Convert all letters to lowercase. Replace spaces with a specified separator.
Double separators are replaced with a single separator.
Args:
text (str): Input text.
separator (str): Separator to replace spaces (default is '_').
Returns:
str: Filtered and normalized text.
"""
### sample
```python
from dekimashita import Dekimashita
text = 'data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]'
clear = Dekimashita.vpath(text)
print('without Dekimashita filter: '+ text)
print('with Dekimashita filter: ' + clear)
```
```
# output
without Dekimashita filter: data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]
with Dekimashita filter: data/data_statistic/baru/kategori/sub_kategori/format_type
```
#### 8. Dekimashita.v3path(text, separator)
"""
Remove non-alphanumeric characters (except a-z, A-Z, 0-9, '/', '.', ':', '-') from text.
Convert all letters to lowercase. Replace spaces with a specified separator.
Double separators are replaced with a single separator.
Args:
text (str): Input text.
separator (str): Separator to replace spaces (default is '_').
Returns:
str: Filtered and normalized text.
"""
### sample
```python
from dekimashita import Dekimashita
text = 's3://ini-path-s3-hehe/data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]'
clear = Dekimashita.v3path(text)
print('without Dekimashita filter: '+ text)
print('with Dekimashita filter: ' + clear)
```
```
# output
without Dekimashita filter: s3://ini-path-s3-hehe/data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]
with Dekimashita filter: s3://ini-path-s3-hehe/data/data_statistic/baru/kategori/sub_kategori/format_type
```
## 🚀Structure
```
│ LICENSE
│ README.md
│ setup.py
│
└───dekimashita
dekimashita.py
__init__.py
```
## Author
👤 **Rio Dwi Saputra**
- Twitter: [@ryyo_cs](https://twitter.com/ryyo_cs)
- Github: [@ryyos](https://github.com/ryyos)
- Instagram: [@ryyo.cs](https://www.instagram.com/ryyo.cs/)
- LinkedIn: [@rio-dwi-saputra-23560b287](https://www.linkedin.com/in/rio-dwi-saputra-23560b287/)
<a href="https://www.linkedin.com/in/rio-dwi-saputra-23560b287/">
<img align="left" alt="Ryo's LinkedIn" width="24px" src="https://cdn.jsdelivr.net/npm/simple-icons@v3/icons/linkedin.svg" />
</a>
<a href="https://www.instagram.com/ryyo.cs/">
<img align="left" alt="Ryo's Instagram" width="24px" src="https://cdn.jsdelivr.net/npm/simple-icons@v3/icons/instagram.svg" />
</a>
Raw data
{
"_id": null,
"home_page": null,
"name": "dekimashita",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "filter, dictionary, text, textCleaning, alphabetic",
"author": "ryyos (Rio Dwi Saputra)",
"author_email": "<riodwi12174@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/2c/08/4912259f9990c00a7cdffc4195b4ca386a798d3ff18c3a163b4172464849/dekimashita-0.0.4.tar.gz",
"platform": null,
"description": "\n# Dekimashita\n\n\n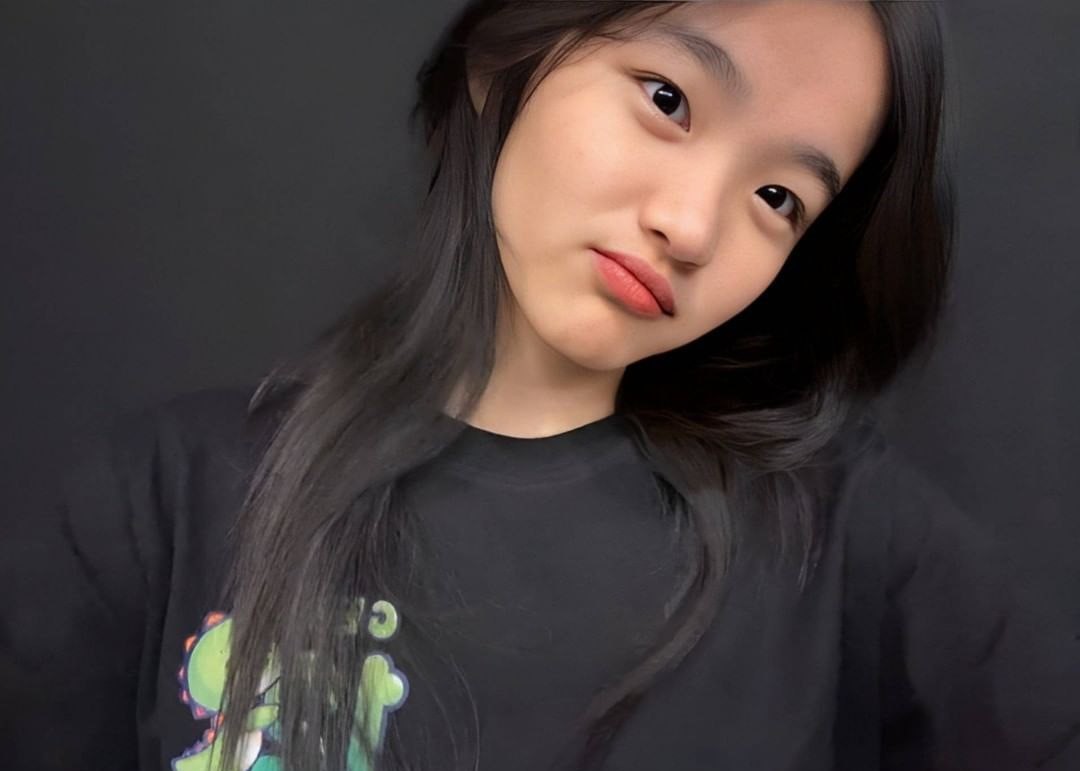\n\n**a library containing a collection of utility functions designed to filter and process text data based on certain criteria. These functions are useful for various text processing tasks, such as removing unwanted characters, extracting specific information, or cleaning input data.**\n\n## Features \u2728\n\n- **Alphabetic Filtering**: Easily filter out non-alphabetic characters from text data.\n- **Numeric Filtering**: Quickly remove non-numeric characters from text strings.\n- **Alphanumeric Filtering**: Filter text to retain only alphanumeric characters, excluding special symbols.\n- **Customization**: Ability to customize the filtering criteria based on specific requirements.\n- **TextCleaning**: Cleanse input text from unwanted characters to prepare it for further processing or analysis.\n- **Normalization**: Standardize text data by removing irregular characters or symbols\n\n## Requirement \u2699\ufe0f\n\n- [Python](https://www.python.org/) v3.11.6+\n\n## Installation \ud83d\udee0\ufe0f\n\n```sh\npip install dekimashita\n```\n\n## How To Usage \ud83e\udd14\n\n#### 1. Dekimashita.vdict(data, [chars])\n\n Filter dictionary values recursively, ignoring specified characters.\n\n Args:\n data (dict or list): Data (dictionary or list containing dictionaries) to filter.\n chars (list): List of characters to filter.\n\n Returns:\n dict or list: Filtered data.\n\n### \u26a0\ufe0f Sample \u26a0\ufe0f\n\n```python\ndata = {\n \"university\": {\n \"name\": \"Example University\",\n \"location\": \"City XYZ\",\n \"courses\": [\n {\n \"course_id\": \"CS101\",\n \"title\": \"Introduction \\n to \\n Computer \\n\\n Science\",\n \"lecturer\": {\n \"name\": \"Dr. Alan\\n Smith\",\n \"email\": \"alan.smith@example.com\",\n \"office\": {\n \"building\": \"Engineering Tower\",\n \"room_number\": \"123\"\n }\n },\n \"students\": {\n \"name\": \"John Doe\",\n \"student_id\": \"123456\",\n \"email\": \"john.doe@example.com\",\n \"grades\": {\n \"assignments\": [\n {\n \"assignment_id\": \"001\",\n \"score\": 95,\n \"comments\": \"Great job on the assignment!\\nKeep up the good work.\"\n },\n {\n \"assignment_id\": \"002\",\n \"score\": 85,\n \"comments\": \"Your\\n effort is commendable.\\r\\r However,\\nthere is room\"\n }\n ],\n \"final_exam\": {\n \"score\": 88,\n \"comments\": \"Solid \\nperformance overall.\\n\\rYour understanding of the subject\"\n }\n }\n }\n }\n ]\n }\n}\n```\n\n<br>\n\n#### without Dekimashita filter\n\n```python\nimport json\n\ndata = # data_sample\n\nwith open(\"data.json\", \"w\") as json_file:\n json.dump(data, json_file, indent=4)\n```\n\n#### If you have a very complex dictionary and you write without using the Dekimashita filter you will get results like this\n\n<br>\n<div style=\"text-align: center;\">\n <img src=\"https://raw.githubusercontent.com/ryyos/ryyos/main/images/Dekimashita/nopng.png\"> \n</div>\n<br>\n\n#### with Dekimashita filter\n\n```python\nimport json\nfrom dekimashita import Dekimashita\n\ndata = # data_sample\nclear = Dekimashita.vdict(data, ['\\n', '\\r'])\n\nwith open(\"data.json\", \"w\") as json_file:\n json.dump(clear, json_file, indent=4)\n```\n\n#### By using the Dekimashita filter you get a clean dictionary like this\n\n<br>\n<div style=\"text-align: center;\">\n <img src=\"https://raw.githubusercontent.com/ryyos/ryyos/main/images/Dekimashita/yeee.png\"> \n</div>\n<br>\n\n#### 2. Dekimashita.vspace(text)\n\n Remove extra spaces from text.\n\n Args:\n text (str): Input text.\n\n Returns:\n str: Text with extra spaces removed.\n\n### sample\n\n```python\nfrom dekimashita import Dekimashita\n\ntext = 'moon beautiful isn\"t it'\n\nclear = Dekimashita.vspace(text)\n\nprint('without Dekimashita filter: '+ text)\nprint('with Dekimashita filter: ' + clear)\n```\n\n```\n# output\n\nwithout Dekimashita filter: moon beautiful isn\"t it\nwith Dekimashita filter: moon beautiful isn\"t it\n```\n\n#### 3. Dekimashita.valpha(text)\n\n Remove non-alphabetic characters (except a-z, A-Z) from text.\n\n Args:\n text (str): Input text.\n\n Returns:\n str: Filtered text containing only alphabetic characters.\n\n### sample\n\n```python\nfrom dekimashita import Dekimashita\n\ntext = 'mo&on b)(*&^%$e!au!t@#$i*f!ul is!!$#n\"t i)(*&^t'\n\nclear = Dekimashita.valpha(text)\n\nprint('without Dekimashita filter: '+ text)\nprint('with Dekimashita filter: ' + clear)\n```\n\n```\n# output\n\nwithout Dekimashita filter: mo&on b)(*&^%$e!au!t@#$i*f!ul is!!$#n\"t i)(*&^t\nwith Dekimashita filter: moon beautiful isnt it\n```\n\n#### 4. Dekimashita.vnum(text)\n\n Remove non-numeric characters from text.\n\n Args:\n text (str): Input text.\n\n Returns:\n str: Filtered text containing only numeric characters.\n\n### sample\n\n```python\nfrom dekimashita import Dekimashita\n\ntext = ' mo30on be7aut20iful i05sn\"t it'\n\nclear = Dekimashita.vnum(text)\n\nprint('without Dekimashita filter: '+ text)\nprint('with Dekimashita filter: ' + clear)\n\n```\n\n```\n# output\n\nwithout Dekimashita filter: mo30on be7aut20iful i05sn\"t it\nwith Dekimashita filter: 3072005\n```\n\n#### 5. Dekimashita.vtext(text)\n\n Remove non-alphanumeric characters (except a-z, A-Z, 0-9) from text.\n Double spaces are replaced with a single space.\n\n Args:\n text (str): Input text.\n\n Returns:\n str: Filtered text containing only alphanumeric characters.\n\n### sample\n\n```python\nfrom dekimashita import Dekimashita\n\ntext = 'moon \\t\\t bea^%$#@utiful isn\"t it 30705'\n\nclear = Dekimashita.vtext(text)\n\nprint('without Dekimashita filter: '+ text)\nprint('with Dekimashita filter: ' + clear)\n\n```\n\n```\n# output\n\nwithout Dekimashita filter: moon bea^%$#@utiful isn\"t it 30705\nwith Dekimashita filter: moon beautiful isnt it 30705\n```\n\n#### 6. Dekimashita.vdir(text, separator)\n\n \"\"\"\n Remove non-alphanumeric characters (except a-z, A-Z, 0-9) from text.\n Convert all letters to lowercase. Replace spaces with a specified separator.\n Double separators are replaced with a single separator.\n\n Args:\n text (str): Input text.\n separator (str): Separator to replace spaces (default is '_').\n\n Returns:\n str: Filtered and normalized text.\n \"\"\"\n\n### sample\n\n```python\nfrom dekimashita import Dekimashita\n\ntext = 'Moon Beautiful Isn\"t It'\n\nclear = Dekimashita.vdir(text)\n\nprint('without Dekimashita filter: '+ text)\nprint('with Dekimashita filter: ' + clear)\n```\n\n```\n# output\n\nwithout Dekimashita filter: Moon Beautiful Isn\"t It\nwith Dekimashita filter: moon_beautiful_isnt_it\n```\n\n#### 7. Dekimashita.vpath(text, separator)\n\n \"\"\"\n Remove non-alphanumeric characters (except a-z, A-Z, 0-9) from text.\n Convert all letters to lowercase. Replace spaces with a specified separator.\n Double separators are replaced with a single separator.\n\n Args:\n text (str): Input text.\n separator (str): Separator to replace spaces (default is '_').\n\n Returns:\n str: Filtered and normalized text.\n \"\"\"\n\n### sample\n\n```python\nfrom dekimashita import Dekimashita\n\ntext = 'data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]'\n\nclear = Dekimashita.vpath(text)\n\nprint('without Dekimashita filter: '+ text)\nprint('with Dekimashita filter: ' + clear)\n```\n\n```\n# output\n\nwithout Dekimashita filter: data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]\nwith Dekimashita filter: data/data_statistic/baru/kategori/sub_kategori/format_type\n```\n\n#### 8. Dekimashita.v3path(text, separator)\n\n \"\"\"\n Remove non-alphanumeric characters (except a-z, A-Z, 0-9, '/', '.', ':', '-') from text.\n Convert all letters to lowercase. Replace spaces with a specified separator.\n Double separators are replaced with a single separator.\n\n Args:\n text (str): Input text.\n separator (str): Separator to replace spaces (default is '_').\n\n Returns:\n str: Filtered and normalized text.\n \"\"\"\n\n### sample\n\n```python\nfrom dekimashita import Dekimashita\n\ntext = 's3://ini-path-s3-hehe/data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]'\n\nclear = Dekimashita.v3path(text)\n\nprint('without Dekimashita filter: '+ text)\nprint('with Dekimashita filter: ' + clear)\n```\n\n```\n# output\n\nwithout Dekimashita filter: s3://ini-path-s3-hehe/data/data_statistic/baru/[kategori]/[sub kategori]/[format_type]\nwith Dekimashita filter: s3://ini-path-s3-hehe/data/data_statistic/baru/kategori/sub_kategori/format_type\n```\n\n## \ud83d\ude80Structure\n\n```\n\u2502 LICENSE\n\u2502 README.md\n\u2502 setup.py\n\u2502\n\u2514\u2500\u2500\u2500dekimashita\n dekimashita.py\n __init__.py\n```\n\n## Author\n\n\ud83d\udc64 **Rio Dwi Saputra**\n\n- Twitter: [@ryyo_cs](https://twitter.com/ryyo_cs)\n- Github: [@ryyos](https://github.com/ryyos)\n- Instagram: [@ryyo.cs](https://www.instagram.com/ryyo.cs/)\n- LinkedIn: [@rio-dwi-saputra-23560b287](https://www.linkedin.com/in/rio-dwi-saputra-23560b287/)\n\n<a href=\"https://www.linkedin.com/in/rio-dwi-saputra-23560b287/\">\n <img align=\"left\" alt=\"Ryo's LinkedIn\" width=\"24px\" src=\"https://cdn.jsdelivr.net/npm/simple-icons@v3/icons/linkedin.svg\" />\n</a>\n<a href=\"https://www.instagram.com/ryyo.cs/\">\n <img align=\"left\" alt=\"Ryo's Instagram\" width=\"24px\" src=\"https://cdn.jsdelivr.net/npm/simple-icons@v3/icons/instagram.svg\" />\n</a>\n",
"bugtrack_url": null,
"license": null,
"summary": "Library designed to process text with various filter criteria",
"version": "0.0.4",
"project_urls": null,
"split_keywords": [
"filter",
" dictionary",
" text",
" textcleaning",
" alphabetic"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "cad028692a921a2976e9d0dca274c8886441fbf9cb81c8d16d59151525f4e6d3",
"md5": "df223dd93dd2d9f241f625022a4064fc",
"sha256": "5b7b47c1ac5ab01a01cf7336dcf973a37353f8f7f828a2f6ec36e7f1c3dad065"
},
"downloads": -1,
"filename": "dekimashita-0.0.4-py3-none-any.whl",
"has_sig": false,
"md5_digest": "df223dd93dd2d9f241f625022a4064fc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 9284,
"upload_time": "2024-08-06T05:35:14",
"upload_time_iso_8601": "2024-08-06T05:35:14.686178Z",
"url": "https://files.pythonhosted.org/packages/ca/d0/28692a921a2976e9d0dca274c8886441fbf9cb81c8d16d59151525f4e6d3/dekimashita-0.0.4-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "2c084912259f9990c00a7cdffc4195b4ca386a798d3ff18c3a163b4172464849",
"md5": "df936195b4e7f4cd06c7fa366b11378b",
"sha256": "3dec8cc2c3416390c57d68ebc1e98066ba7487473a108039ca696927dab8a4e3"
},
"downloads": -1,
"filename": "dekimashita-0.0.4.tar.gz",
"has_sig": false,
"md5_digest": "df936195b4e7f4cd06c7fa366b11378b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 9202,
"upload_time": "2024-08-06T05:35:16",
"upload_time_iso_8601": "2024-08-06T05:35:16.740179Z",
"url": "https://files.pythonhosted.org/packages/2c/08/4912259f9990c00a7cdffc4195b4ca386a798d3ff18c3a163b4172464849/dekimashita-0.0.4.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-06 05:35:16",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "dekimashita"
}