
# Installation
Install latest via pip:
`pip install discord-menu`
# What is discord-menu?
discord-menu is a flexible python framework for creating interactive menus out of Discord Embeds. Users can click specified emojis and the embed responds according to preset instructions.
Its primary features are:
1. **Declaritive UI Syntax** - React/SwiftUI like definition of Views.
1. **Stateless compute** - Menus do not require maintaining a session and no data needs to be stored on the bot's server.
1. **Event driven** - No polling needed and interactions respond immediately to input.
1. **Scalable state** - Message state is managed directly in the Embed, leveraging Discord's storage scalability.
1. **Flexibility** - Arbitrary code can be executed on emoji clicks, allowing for complex features like pagination, child menus, or user authorizaton!
# How to use
Example code demonstrated with Red-DiscordBot. Raw discord.py should also work with slight modification of imports.
# Supported convenience menus
1. **SimpleTextMenu** - Use this if you just want to display some text.
1. **SimpleTabbedTextMenu** - This is useful if you a few different panes of text content that a user would select between.
1. **ClosableMenu** - Write a custom view (more than just text) with a close button.
1. **TabbedMenu** - Write custom views, and tab between them using dedicated emojis. Closable by default.
1. **ScrollableMenu** - Write custom views, and scroll between them using left and right arrows. Closable by default.
## SimpleTextMenu
```python
import discord
from redbot.core import commands
from discordmenu.menu.listener.menu_listener import MenuListener
from discordmenu.menu.listener.menu_map import MenuMap, MenuMapEntry
from discordmenu.menu.simple_text_menu import SimpleTextMenu, SimpleTextViewState
menu_map = MenuMap()
menu_map[SimpleTextMenu.MENU_TYPE] = MenuMapEntry(SimpleTextMenu, EmbedTransitions)
class TestCog(commands.Cog):
def __init__(self, bot):
self.listener = MenuListener(bot, menu_map)
@commands.Cog.listener('on_raw_reaction_add')
@commands.Cog.listener('on_raw_reaction_remove')
async def on_raw_reaction_update(self, payload: discord.RawReactionActionEvent):
await self.listener.on_raw_reaction_update(payload)
async def simplemenu(self, ctx):
await SimpleTextMenu.menu().create(ctx, SimpleTextViewState("Hello World!"))
```
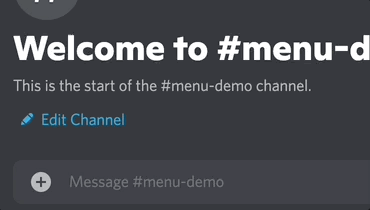
[Code](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py#L38)
## SimpleTabbedTextMenu
```python
import discord
from redbot.core import commands
from discordmenu.menu.listener.menu_listener import MenuListener
from discordmenu.menu.listener.menu_map import MenuMap, MenuMapEntry
from discordmenu.menu.simple_tabbed_text_menu import SimpleTabbedTextMenu, SimpleTabbedTextMenuTransitions,
SimpleTabbedTextViewState
menu_map = MenuMap()
menu_map[SimpleTabbedTextMenu.MENU_TYPE] = MenuMapEntry(SimpleTabbedTextMenu, SimpleTabbedTextMenuTransitions)
class TestCog(commands.Cog):
def __init__(self, bot):
self.listener = MenuListener(bot, menu_map)
@commands.Cog.listener('on_raw_reaction_add')
@commands.Cog.listener('on_raw_reaction_remove')
async def on_raw_reaction_update(self, payload: discord.RawReactionActionEvent):
await self.listener.on_raw_reaction_update(payload)
async def simpletabbedtextmenu(self, ctx):
vs = SimpleTabbedTextViewState("Initial message.", ["Message 1", "Message 2", "Message 3"])
await SimpleTabbedTextMenu.menu().create(ctx, vs)
```
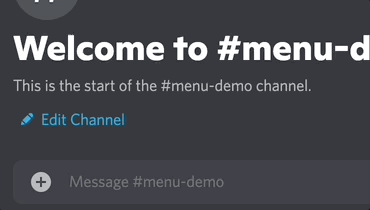
[Code](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py#L43)
## Sample code for other menus
For example code for `ClosableMenu`, `TabbedMenu`, or `ScrollableMenu`, refer to the [test file](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py).
## Advanced usage
If you're looking for ideas on what you can do with this framework, or for info on how to create complex menus, refer to [documentation on advanced usage](https://github.com/TsubakiBotPad/discord-menu/blob/main/docs/advanced-usage.md).
This menu system is originally built for looking up data from the popular puzzle gacha game [Puzzle & Dragons](https://en.wikipedia.org/wiki/Puzzle_%26_Dragons) - feel free to join the [discord server](https://discord.gg/QCRxNtC) for a demo on the complex system that powers data accessbility within the community.
# Running tests + sample code
## Prerequisite: Create a Discord bot
If you don't have one already, follow the instructions to create a bot in Red's official documentation:
[Creating a bot account](https://docs.discord.red/en/stable/bot_application_guide.html#creating-a-bot-account)
Keep the bot `token` that you get from Discord at the end of the instructions handy - you will need it to set up the bot later.
## Installation
1. Create a python 3 venv in the `test` folder. `virtualenv -p python3 <envname>`
1. Activate the venv.
1. `pip install -r requirements.txt`
The above steps install Red-Discord bot framework. You can now follow more detailed [instructions](https://docs.discord.red/en/stable/install_guides/mac.html#setting-up-and-running-red) to startup the bot. Or run these in command line and follow the prompts:
- `redbot-setup`
- `redbot <bot_name>`
## Interact with the bot
The rest of the guide takes place from inside Discord. Replace `^` with your prefix to talk to your bot.
1. Once the bot is launched, set it to the `test` directory as a cog path.
```
^addpath /Users/me/src/discord-menu/test
```
1. Load `testcog`
```
^reload testcog
```
1. Run a test command. Test [code](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py#L37) for simplemenu.
```
^t
```
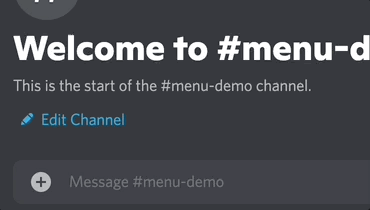
# Contributing
If you encounter a bug or would like to make a feature request, please file a Github issue or submit a pull request.
Also, if you don't understand something in the documentation, you are experiencing problems, or you just need a gentle nudge in the right direction, please don't hesitate to join the [discord server](https://discord.gg/QCRxNtC).
Raw data
{
"_id": null,
"home_page": "https://github.com/TsubakiBotPad/discord-menu",
"name": "discord-menu",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": "",
"keywords": "",
"author": "The Tsubotki Team",
"author_email": "69992611+TsubakiBotPad@users.noreply.github.com",
"download_url": "https://files.pythonhosted.org/packages/e4/8c/53d87a19bcc3e5ede4b6fd24a2ccb36a17c7676812fc945312fabca7e01d/discord-menu-0.16.16.tar.gz",
"platform": null,
"description": "\r\n\r\n# Installation\r\n\r\nInstall latest via pip:\r\n\r\n`pip install discord-menu`\r\n\r\n# What is discord-menu?\r\n\r\ndiscord-menu is a flexible python framework for creating interactive menus out of Discord Embeds. Users can click specified emojis and the embed responds according to preset instructions.\r\n\r\nIts primary features are:\r\n\r\n1. **Declaritive UI Syntax** - React/SwiftUI like definition of Views.\r\n1. **Stateless compute** - Menus do not require maintaining a session and no data needs to be stored on the bot's server.\r\n1. **Event driven** - No polling needed and interactions respond immediately to input.\r\n1. **Scalable state** - Message state is managed directly in the Embed, leveraging Discord's storage scalability.\r\n1. **Flexibility** - Arbitrary code can be executed on emoji clicks, allowing for complex features like pagination, child menus, or user authorizaton!\r\n\r\n# How to use\r\n\r\nExample code demonstrated with Red-DiscordBot. Raw discord.py should also work with slight modification of imports.\r\n\r\n# Supported convenience menus\r\n\r\n1. **SimpleTextMenu** - Use this if you just want to display some text.\r\n1. **SimpleTabbedTextMenu** - This is useful if you a few different panes of text content that a user would select between.\r\n1. **ClosableMenu** - Write a custom view (more than just text) with a close button.\r\n1. **TabbedMenu** - Write custom views, and tab between them using dedicated emojis. Closable by default.\r\n1. **ScrollableMenu** - Write custom views, and scroll between them using left and right arrows. Closable by default.\r\n\r\n## SimpleTextMenu\r\n\r\n```python\r\nimport discord\r\nfrom redbot.core import commands\r\n\r\nfrom discordmenu.menu.listener.menu_listener import MenuListener\r\nfrom discordmenu.menu.listener.menu_map import MenuMap, MenuMapEntry\r\nfrom discordmenu.menu.simple_text_menu import SimpleTextMenu, SimpleTextViewState\r\n\r\nmenu_map = MenuMap()\r\nmenu_map[SimpleTextMenu.MENU_TYPE] = MenuMapEntry(SimpleTextMenu, EmbedTransitions)\r\n\r\nclass TestCog(commands.Cog):\r\n def __init__(self, bot):\r\n self.listener = MenuListener(bot, menu_map)\r\n\r\n @commands.Cog.listener('on_raw_reaction_add')\r\n @commands.Cog.listener('on_raw_reaction_remove')\r\n async def on_raw_reaction_update(self, payload: discord.RawReactionActionEvent):\r\n await self.listener.on_raw_reaction_update(payload)\r\n\r\n async def simplemenu(self, ctx):\r\n await SimpleTextMenu.menu().create(ctx, SimpleTextViewState(\"Hello World!\"))\r\n```\r\n\r\n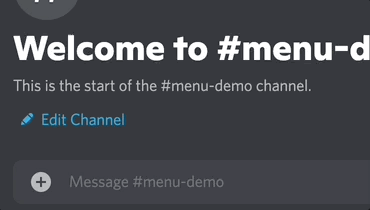\r\n\r\n[Code](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py#L38)\r\n\r\n## SimpleTabbedTextMenu\r\n\r\n```python\r\nimport discord\r\nfrom redbot.core import commands\r\n\r\nfrom discordmenu.menu.listener.menu_listener import MenuListener\r\nfrom discordmenu.menu.listener.menu_map import MenuMap, MenuMapEntry\r\nfrom discordmenu.menu.simple_tabbed_text_menu import SimpleTabbedTextMenu, SimpleTabbedTextMenuTransitions,\r\n SimpleTabbedTextViewState\r\n\r\nmenu_map = MenuMap()\r\nmenu_map[SimpleTabbedTextMenu.MENU_TYPE] = MenuMapEntry(SimpleTabbedTextMenu, SimpleTabbedTextMenuTransitions)\r\n\r\n\r\nclass TestCog(commands.Cog):\r\n def __init__(self, bot):\r\n self.listener = MenuListener(bot, menu_map)\r\n\r\n @commands.Cog.listener('on_raw_reaction_add')\r\n @commands.Cog.listener('on_raw_reaction_remove')\r\n async def on_raw_reaction_update(self, payload: discord.RawReactionActionEvent):\r\n await self.listener.on_raw_reaction_update(payload)\r\n\r\n async def simpletabbedtextmenu(self, ctx):\r\n vs = SimpleTabbedTextViewState(\"Initial message.\", [\"Message 1\", \"Message 2\", \"Message 3\"])\r\n await SimpleTabbedTextMenu.menu().create(ctx, vs)\r\n```\r\n\r\n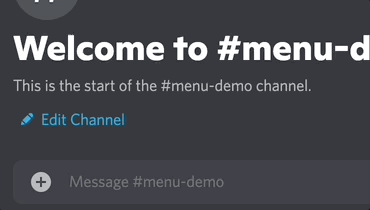\r\n\r\n[Code](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py#L43)\r\n\r\n## Sample code for other menus\r\n\r\nFor example code for `ClosableMenu`, `TabbedMenu`, or `ScrollableMenu`, refer to the [test file](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py).\r\n\r\n## Advanced usage\r\n\r\nIf you're looking for ideas on what you can do with this framework, or for info on how to create complex menus, refer to [documentation on advanced usage](https://github.com/TsubakiBotPad/discord-menu/blob/main/docs/advanced-usage.md).\r\n\r\nThis menu system is originally built for looking up data from the popular puzzle gacha game [Puzzle & Dragons](https://en.wikipedia.org/wiki/Puzzle_%26_Dragons) - feel free to join the [discord server](https://discord.gg/QCRxNtC) for a demo on the complex system that powers data accessbility within the community.\r\n\r\n# Running tests + sample code\r\n\r\n## Prerequisite: Create a Discord bot\r\n\r\nIf you don't have one already, follow the instructions to create a bot in Red's official documentation:\r\n\r\n[Creating a bot account](https://docs.discord.red/en/stable/bot_application_guide.html#creating-a-bot-account)\r\n\r\nKeep the bot `token` that you get from Discord at the end of the instructions handy - you will need it to set up the bot later.\r\n\r\n## Installation\r\n\r\n1. Create a python 3 venv in the `test` folder. `virtualenv -p python3 <envname>`\r\n1. Activate the venv.\r\n1. `pip install -r requirements.txt`\r\n\r\nThe above steps install Red-Discord bot framework. You can now follow more detailed [instructions](https://docs.discord.red/en/stable/install_guides/mac.html#setting-up-and-running-red) to startup the bot. Or run these in command line and follow the prompts:\r\n\r\n- `redbot-setup`\r\n- `redbot <bot_name>`\r\n\r\n## Interact with the bot\r\n\r\nThe rest of the guide takes place from inside Discord. Replace `^` with your prefix to talk to your bot.\r\n\r\n1. Once the bot is launched, set it to the `test` directory as a cog path.\r\n\r\n```\r\n^addpath /Users/me/src/discord-menu/test\r\n```\r\n\r\n1. Load `testcog`\r\n\r\n```\r\n^reload testcog\r\n```\r\n\r\n1. Run a test command. Test [code](https://github.com/TsubakiBotPad/discord-menu/blob/main/test/testcog/main.py#L37) for simplemenu.\r\n\r\n```\r\n^t\r\n```\r\n\r\n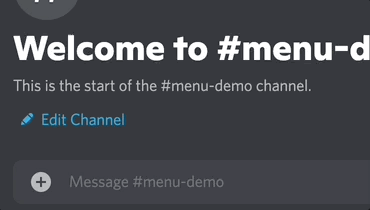\r\n\r\n# Contributing\r\n\r\nIf you encounter a bug or would like to make a feature request, please file a Github issue or submit a pull request.\r\n\r\nAlso, if you don't understand something in the documentation, you are experiencing problems, or you just need a gentle nudge in the right direction, please don't hesitate to join the [discord server](https://discord.gg/QCRxNtC).\r\n",
"bugtrack_url": null,
"license": "Apache-2.0 License",
"summary": "A library to create tabbed menus in Discord messages",
"version": "0.16.16",
"project_urls": {
"Homepage": "https://github.com/TsubakiBotPad/discord-menu"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "e48c53d87a19bcc3e5ede4b6fd24a2ccb36a17c7676812fc945312fabca7e01d",
"md5": "64813983b01fbaadb0e74215e6cffac0",
"sha256": "47554cd937cf5bd9500ae5480980bc1e612e51e2032248653012fccdad199195"
},
"downloads": -1,
"filename": "discord-menu-0.16.16.tar.gz",
"has_sig": false,
"md5_digest": "64813983b01fbaadb0e74215e6cffac0",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 25029,
"upload_time": "2023-05-04T23:35:03",
"upload_time_iso_8601": "2023-05-04T23:35:03.672840Z",
"url": "https://files.pythonhosted.org/packages/e4/8c/53d87a19bcc3e5ede4b6fd24a2ccb36a17c7676812fc945312fabca7e01d/discord-menu-0.16.16.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-04 23:35:03",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "TsubakiBotPad",
"github_project": "discord-menu",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "discord-menu"
}