# DiscordLevelingCard
A library with Rank cards for your discord bot.
<h3 style="color:yellow;"> now create your own custom rank cards!</h3>
<br>
[](https://pepy.tech/project/discordlevelingcard)
# card preview
`card1`
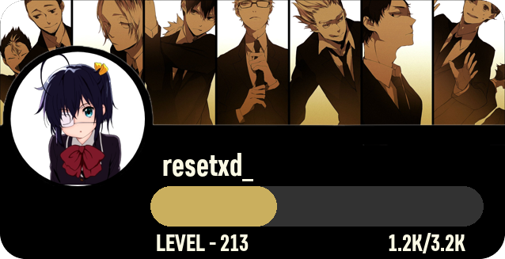
`card2`
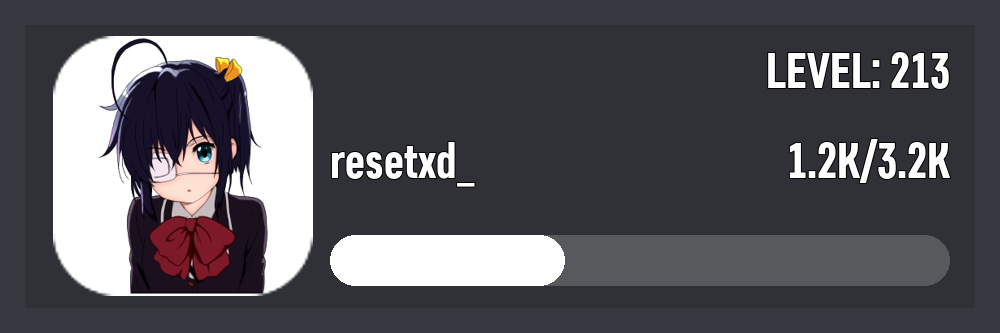
`card3` *same as card2 but with background*
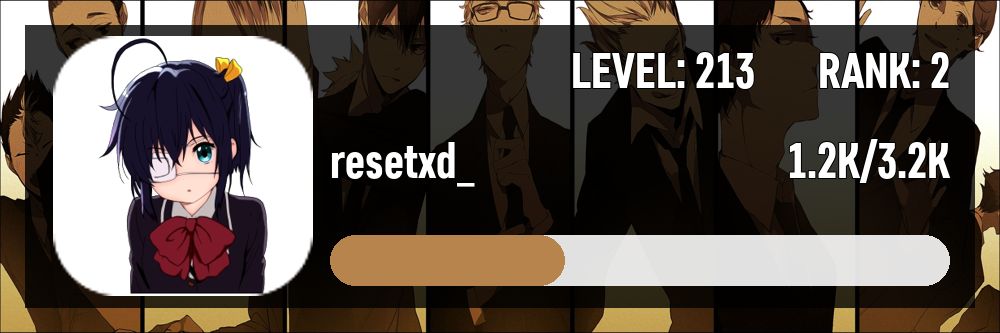
<br>
# installation
`for pypi version`
```sh
pip install discordlevelingcard
```
`for github developement version`
```sh
pip install git+https://github.com/krishsharma0413/DiscordLevelingCard
```
# How To Use
If you don't provide `path` then the method will return `bytes` which can directly be used in discord.py/disnake/pycord/nextcord 's `File class`.
<br>
# Example
`since no path was provided, it returns bytes which can directly be used in discord.py and its fork's File class.`
```py
from disnake.ext import commands
from DiscordLevelingCard import RankCard, Settings
import disnake
client = commands.Bot()
# define background, bar_color, text_color at one place
card_settings = Settings(
background="url or path to background image",
text_color="white",
bar_color="#000000"
)
@client.slash_command(name="rank")
async def user_rank_card(ctx, user:disnake.Member):
await ctx.response.defer()
a = RankCard(
settings=card_settings,
avatar=user.display_avatar.url,
level=1,
current_exp=1,
max_exp=1,
username="cool username"
)
image = await a.card1()
await ctx.edit_original_message(file=disnake.File(image, filename="rank.png")) # providing filename is very important
```
<br>
## Documentation
<details>
<summary> <span style="color:yellow">RankCard</span> class</summary>
<br>
`__init__` method
```py
RankCard(
settings: Settings,
avatar:str,
level:int,
current_exp:int,
max_exp:int,
username:str,
rank: Optional[int] = None
)
```
- `settings` - Settings class from DiscordLevelingCard.
- `avatar` - avatar image url.
- `level` - level of the user.
- `current_exp` - current exp of the user.
- `max_exp` - max exp of the user.
- `username` - username of the user.
- `rank` - rank of the user. (optional)
## methods
- `card1`
- `card2`
- `card3`
</details>
<details>
<summary> <span style="color:yellow">Sandbox</span> class</summary>
<br>
`__init__` method
```py
RankCard(
settings: Settings,
avatar:str,
level:int,
current_exp:int,
max_exp:int,
username:str,
cacheing:bool = True,
rank: Optional[int] = None
)
```
- `settings` - Settings class from DiscordLevelingCard.
- `avatar` - avatar image url.
- `level` - level of the user.
- `current_exp` - current exp of the user.
- `max_exp` - max exp of the user.
- `username` - username of the user.
- `rank` - rank of the user. (optional)
- `cacheing` - if set to `True` then it will cache the image and will not regenerate it again. (default is `True`)
## methods
- `custom_card1`
</details>
<details>
<summary> <span style="color:yellow">Settings</span> class</summary>
<br>
`__init__` method
```py
Settings(
background: Union[PathLike, BufferedIOBase, str],
bar_color: Optional[str] = 'white',
text_color: Optional[str] = 'white',
background_color: Optional[str]= "#36393f"
)
```
- `background` - background image url or file-object in `rb` mode.
- `4:1` aspect ratio recommended.
- `bar_color` - color of the bar [example: "white" or "#000000"]
- `text_color` - color of the text [example: "white" or "#000000"]
- `background_color` - color of the background [example: "white" or "#000000"]
</details>
<details>
<summary> <span style="color:yellow">card1</span> method</summary>
```py
RankCard.card1(resize: int = 100)
```
## attribute
- `resize` : resize the final image. (default is 100, treat it as a percentage.)
## returns
- `bytes` which can directly be used within `discord.File` class.
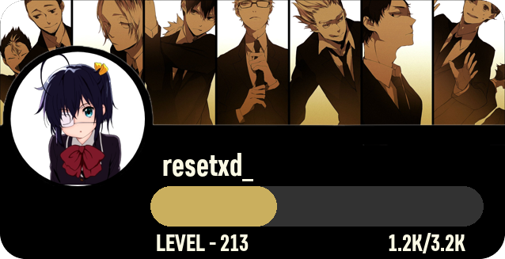
<br>
</details>
<details>
<summary> <span style="color:yellow">card2</span> method</summary>
```py
RankCard.card2(resize: int = 100)
```
## attribute
- `resize` : resize the final image. (default is 100, treat it as a percentage.)
## returns
- `bytes` which can directly be used within `discord.File` class.
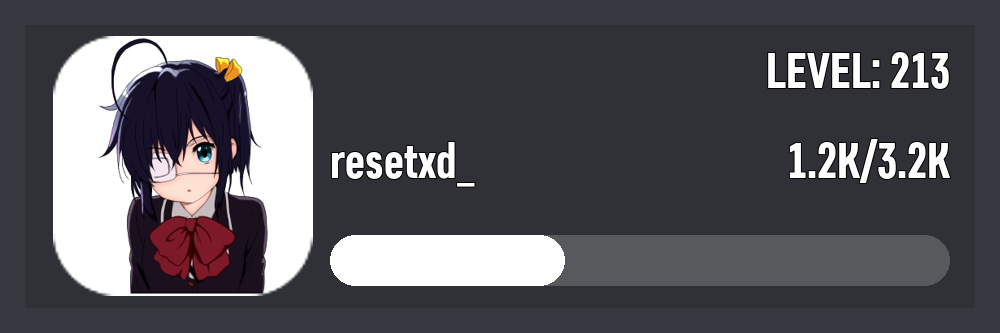
<br>
</details>
<details>
<summary> <span style="color:yellow">card3</span> method</summary>
```py
RankCard.card3(resize: int = 100)
```
## attribute
- `resize` : resize the final image. (default is 100, treat it as a percentage.)
## returns
- `bytes` which can directly be used within `discord.File` class.
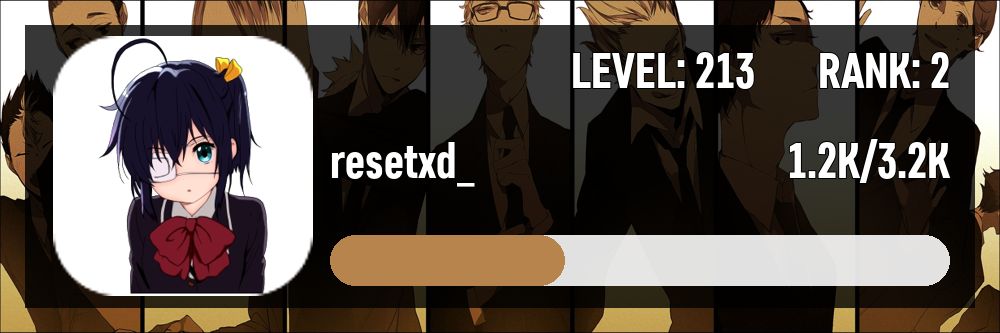
<br>
</details>
<details>
<summary> <span style="color:yellow">custom_card1</span> method</summary>
```py
Sandbox.custom_card1(card_colour:str = "black", resize: int = 100)
```
## attribute
- `resize` : resize the final image. (default is 100, treat it as a percentage.)
- `card_colour` : color of the card. (default is black)
## returns
- `bytes` which can directly be used within `discord.File` class.
## examples
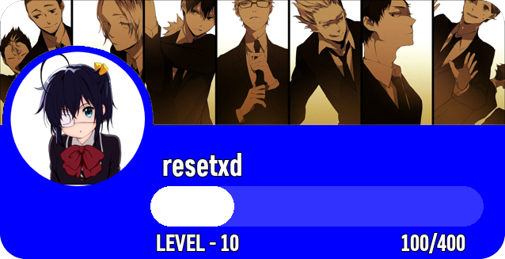
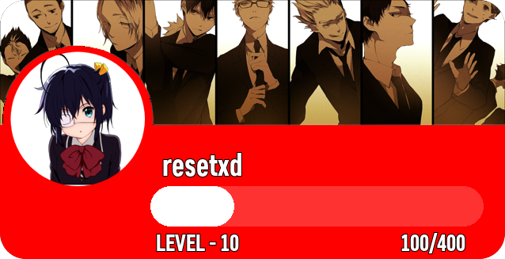
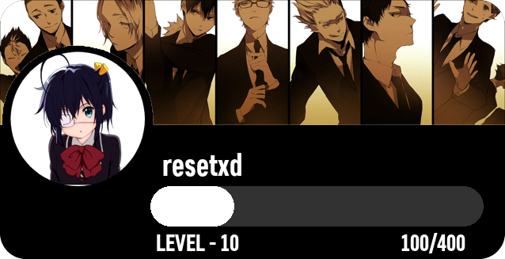
<br>
</details>
<details>
<summary> <span style="color:yellow">custom_canvas</span> method</summary>
```py
Sandbox.custom_canvas(
resize:int = 100,
senstivity:int = 200,
card_colour: str = "black",
border_width: int = 25,
border_height: int = 25,
avatar_frame: str = "curvedborder",
avatar_size: int = 260,
avatar_position: tuple = (53, 36),
text_font: str = "levelfont.otf",
username_position: tuple = (330,130),
username_font_size: int = 50,
level_position: tuple = (500,40),
level_font_size: int = 50,
exp_position: tuple = (775,130),
exp_font_size: int = 50,
)
```
## attribute
- `has_background` : if set to `True` then it will add a background to the image. (default is `True`)
- `background_colour` : color of the background. (default is `black`)
- `canvas_size` : size of the canvas. (default is `(1000, 333)`)
- `resize` : resize the final image. (default is 100, treat it as a percentage.)
- `overlay` : A list of overlays to be placed on the background. (Default is `[[(1000-50, 333-50),(25, 25), "black", 200]]`.)
- `avatar_frame` : `circle` `square` `curvedborder` `hexagon` or path to a self created mask. (Default is `curvedborder`.)
- `text_font` : Default is `levelfont.otf` or path to a custom otf or ttf file type font.
- `avatar_size` : size of the avatar. (default is `260`)
- `avatar_position` : position of the avatar. (default is `(53, 36)`)
- `username_position` : position of the username. (default is `(330,130)`)
- `username_font_size` : font size of the username. (default is `50`)
- `level_position` : position of the level. (default is `(500,40)`)
- `level_font_size` : font size of the level. (default is `50`)
- `exp_position` : position of the exp. (default is `(775,130)`)
- `exp_font_size` : font size of the exp. (default is `50`)
- `exp_bar_width` : width of the exp bar. (default is `619`)
- `exp_bar_height` : height of the exp bar. (default is `50`)
- `exp_bar_background_colour` : color of the exp bar background. (default is `white`)
- `exp_bar_position` : position of the exp bar. (default is `(330, 235)`)
- `exp_bar_curve` : curve of the exp bar. (default is `30`)
- `extra_text` : A list of extra text to be placed on the image. (Default is `None`.)
- `exp_bar` : The calculated exp of the user. (Default is `None`.)
## returns
- `bytes` which can directly be used within `discord.File` class.
## examples
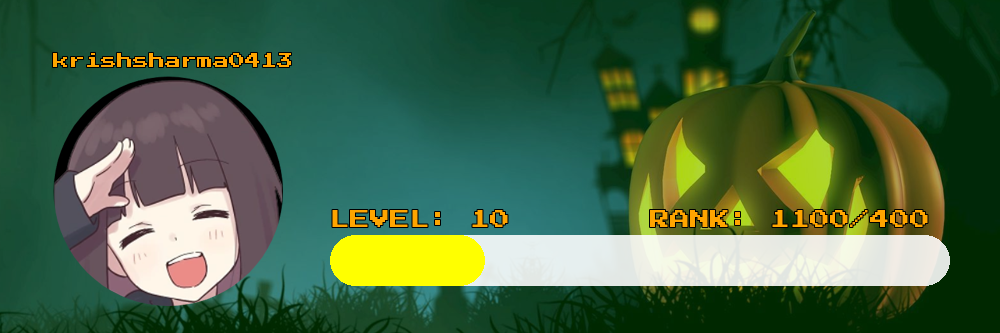
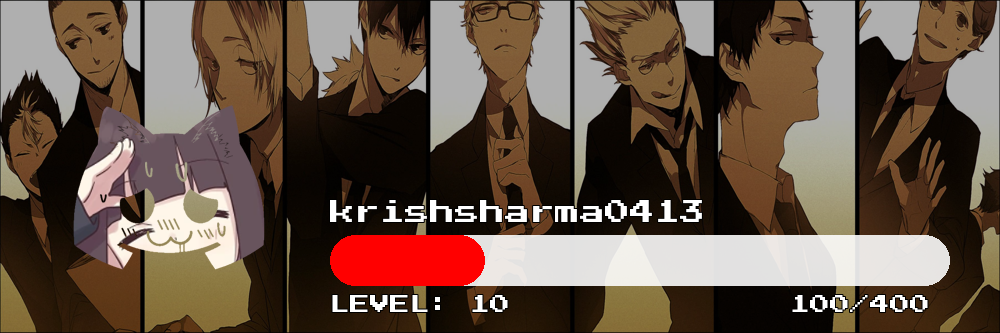
An Example that i really loved was this one, here is the code for it as well. (you might have to tweak a lot to make it work for you though. )
```py
from DiscordLevelingCard import Sandbox, Settings
import asyncio
from PIL import Image
setting = Settings(
background="./bg.jpg",
bar_color="green",
text_color="white")
async def main():
rank = Sandbox(
username="krishsharma0413",
level=1,
current_exp=10,
max_exp=400,
settings=setting,
avatar=Image.open("./avatarimg.png")
)
result = await rank.custom_canvas(
avatar_frame="square",
avatar_size=233,
avatar_position=(50, 50),
exp_bar_background_colour = "black",
exp_bar_height=50,
exp_bar_width=560,
exp_bar_curve=0,
exp_bar_position=(70, 400),
username_position=(320, 50),
level_position=(320, 225),
exp_position=(70, 330),
canvas_size=(700, 500),
overlay=[[(350, 233),(300, 50), "white", 100],
[(600, 170),(50, 300), "white", 100]],
extra_text=[
["bio-", (320, 110), 25, "white"],
["this can very well be a bio", (320, 140), 25, "white"],
["even mutiple lines!", (320, 170), 25, "white"],
["if we remove bio- even more!", (320, 200), 25, "white"],
]
)
# you don't need this line if you are using this in discord.py
Image.open(result).save("result.png", "PNG")
asyncio.run(main())
```
and this is how it looks :D
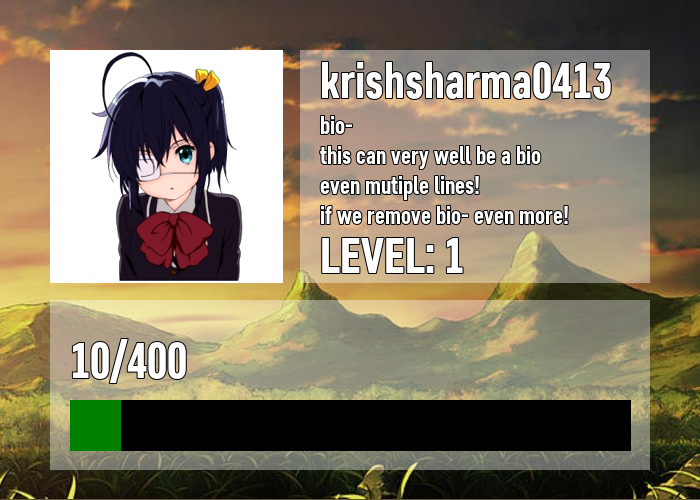
<br>
</details>
<br><br>
if you want to see changelog then click [here](https://github.com/krishsharma0413/DiscordLevelingCard/blob/main/CHANGELOG.md)
<br><br>
please star the <a href="https://github.com/krishsharma0413/DiscordLevelingCard">repository</a> if you like it :D
Raw data
{
"_id": null,
"home_page": "https://github.com/krishsharma0413/DiscordLevelingCard",
"name": "discordlevelingcard",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8,<4",
"maintainer_email": "",
"keywords": "discord,leveling,card,image,discord.py,disnake,nextcord,rank,ranking,level,discord-bot,bot,discord-leveling,level-card,discord-leveling-card,discord-rank-card,sandbox,custom,custom-card",
"author": "Reset",
"author_email": "krishsharma0413@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/13/fa/98758387ff05f8f849d40ff411c038a70348194bad987f6e95ce550171b0/discordlevelingcard-0.6.0.tar.gz",
"platform": null,
"description": "# DiscordLevelingCard\nA library with Rank cards for your discord bot.\n\n<h3 style=\"color:yellow;\"> now create your own custom rank cards!</h3>\n\n<br>\n\n[](https://pepy.tech/project/discordlevelingcard)\n\n# card preview\n\n`card1`\n\n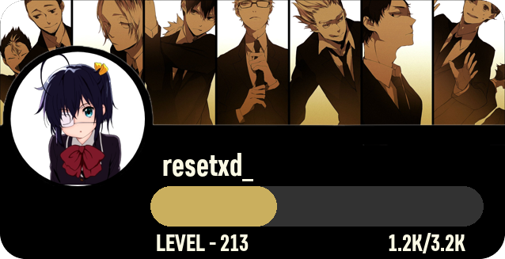\n\n\n`card2`\n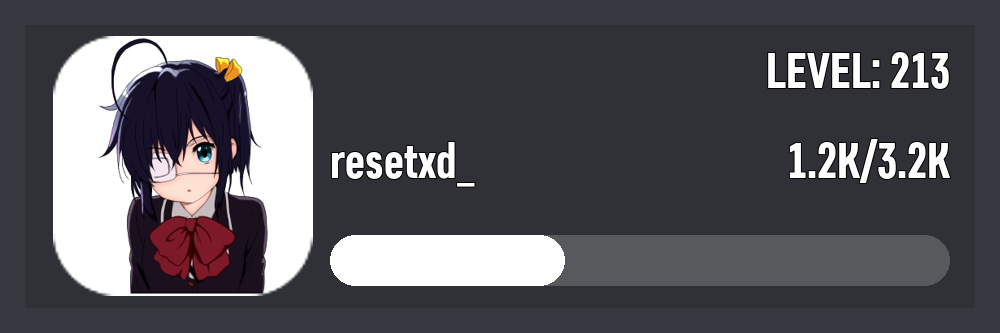\n\n\n`card3` *same as card2 but with background*\n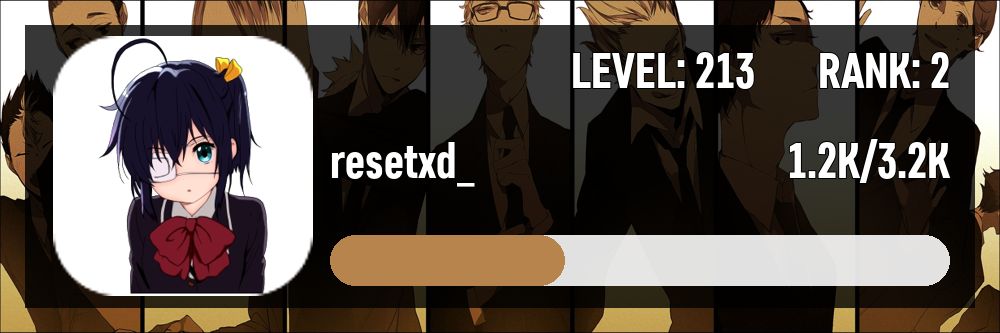\n\n<br>\n\n# installation\n\n`for pypi version`\n```sh\npip install discordlevelingcard\n```\n\n`for github developement version`\n```sh\npip install git+https://github.com/krishsharma0413/DiscordLevelingCard\n```\n\n# How To Use\n\nIf you don't provide `path` then the method will return `bytes` which can directly be used in discord.py/disnake/pycord/nextcord 's `File class`.\n\n\n<br>\n\n\n# Example\n\n`since no path was provided, it returns bytes which can directly be used in discord.py and its fork's File class.`\n\n```py\nfrom disnake.ext import commands\nfrom DiscordLevelingCard import RankCard, Settings\nimport disnake\n\nclient = commands.Bot()\n# define background, bar_color, text_color at one place\ncard_settings = Settings(\n background=\"url or path to background image\",\n text_color=\"white\",\n bar_color=\"#000000\"\n)\n\n@client.slash_command(name=\"rank\")\nasync def user_rank_card(ctx, user:disnake.Member):\n await ctx.response.defer()\n a = RankCard(\n settings=card_settings,\n avatar=user.display_avatar.url,\n level=1,\n current_exp=1,\n max_exp=1,\n username=\"cool username\"\n )\n image = await a.card1()\n await ctx.edit_original_message(file=disnake.File(image, filename=\"rank.png\")) # providing filename is very important\n\n```\n\n<br>\n\n## Documentation\n\n\n<details>\n\n<summary> <span style=\"color:yellow\">RankCard</span> class</summary>\n\n<br>\n\n`__init__` method\n\n```py\nRankCard(\n settings: Settings,\n avatar:str,\n level:int,\n current_exp:int,\n max_exp:int,\n username:str,\n rank: Optional[int] = None\n)\n```\n\n- `settings` - Settings class from DiscordLevelingCard.\n\n- `avatar` - avatar image url.\n\n- `level` - level of the user.\n\n- `current_exp` - current exp of the user.\n\n- `max_exp` - max exp of the user.\n\n- `username` - username of the user.\n\n- `rank` - rank of the user. (optional)\n\n## methods\n\n- `card1`\n- `card2`\n- `card3`\n\n</details>\n\n<details>\n\n<summary> <span style=\"color:yellow\">Sandbox</span> class</summary>\n\n<br>\n\n`__init__` method\n\n```py\nRankCard(\n settings: Settings,\n avatar:str,\n level:int,\n current_exp:int,\n max_exp:int,\n username:str,\n cacheing:bool = True,\n rank: Optional[int] = None\n)\n```\n\n- `settings` - Settings class from DiscordLevelingCard.\n\n- `avatar` - avatar image url.\n\n- `level` - level of the user.\n\n- `current_exp` - current exp of the user.\n\n- `max_exp` - max exp of the user.\n\n- `username` - username of the user.\n\n- `rank` - rank of the user. (optional)\n\n- `cacheing` - if set to `True` then it will cache the image and will not regenerate it again. (default is `True`)\n \n\n## methods\n- `custom_card1`\n \n</details>\n\n\n\n\n<details>\n\n<summary> <span style=\"color:yellow\">Settings</span> class</summary>\n\n<br>\n\n`__init__` method\n\n```py\nSettings(\n background: Union[PathLike, BufferedIOBase, str],\n bar_color: Optional[str] = 'white',\n text_color: Optional[str] = 'white',\n background_color: Optional[str]= \"#36393f\"\n\n)\n```\n\n- `background` - background image url or file-object in `rb` mode.\n - `4:1` aspect ratio recommended.\n\n- `bar_color` - color of the bar [example: \"white\" or \"#000000\"]\n\n- `text_color` - color of the text [example: \"white\" or \"#000000\"]\n\n- `background_color` - color of the background [example: \"white\" or \"#000000\"]\n\n</details>\n\n\n<details>\n\n<summary> <span style=\"color:yellow\">card1</span> method</summary>\n\n\n```py\nRankCard.card1(resize: int = 100)\n```\n\n## attribute\n- `resize` : resize the final image. (default is 100, treat it as a percentage.)\n\n\n\n## returns \n- `bytes` which can directly be used within `discord.File` class.\n\n\n\n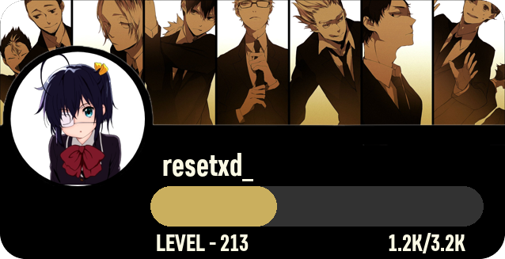\n\n<br>\n\n</details>\n\n\n<details>\n\n<summary> <span style=\"color:yellow\">card2</span> method</summary>\n\n\n```py\nRankCard.card2(resize: int = 100)\n```\n\n## attribute\n- `resize` : resize the final image. (default is 100, treat it as a percentage.)\n\n## returns\n- `bytes` which can directly be used within `discord.File` class.\n\n\n\n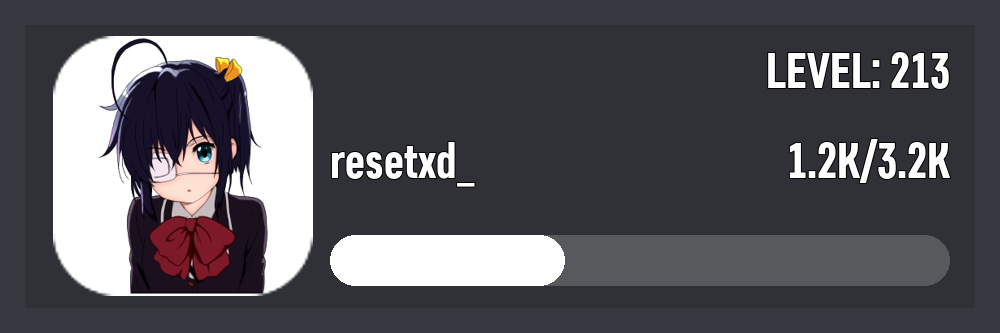\n\n<br>\n\n</details>\n\n\n<details>\n\n<summary> <span style=\"color:yellow\">card3</span> method</summary>\n\n\n```py\nRankCard.card3(resize: int = 100)\n```\n\n## attribute\n- `resize` : resize the final image. (default is 100, treat it as a percentage.)\n\n## returns\n- `bytes` which can directly be used within `discord.File` class.\n\n\n\n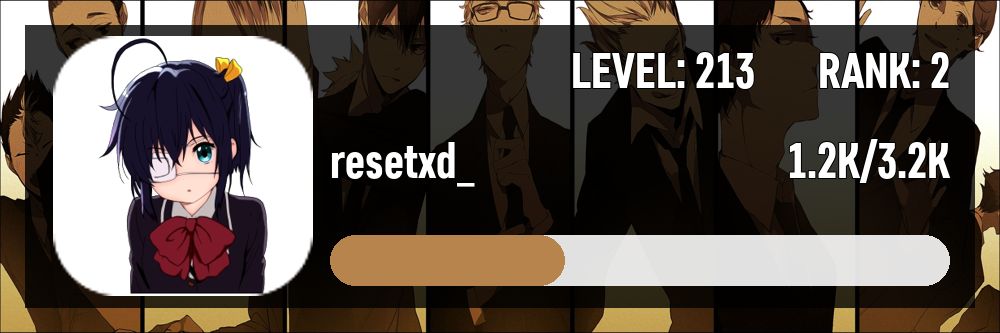\n\n<br>\n\n</details>\n\n<details>\n\n<summary> <span style=\"color:yellow\">custom_card1</span> method</summary>\n\n\n```py\nSandbox.custom_card1(card_colour:str = \"black\", resize: int = 100)\n```\n\n## attribute\n- `resize` : resize the final image. (default is 100, treat it as a percentage.)\n- `card_colour` : color of the card. (default is black)\n\n\n\n## returns \n- `bytes` which can directly be used within `discord.File` class.\n\n\n## examples\n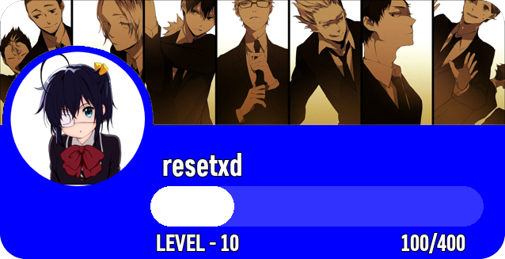\n\n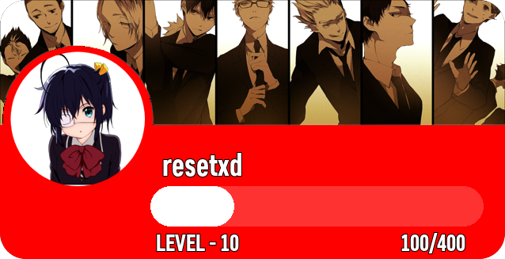\n\n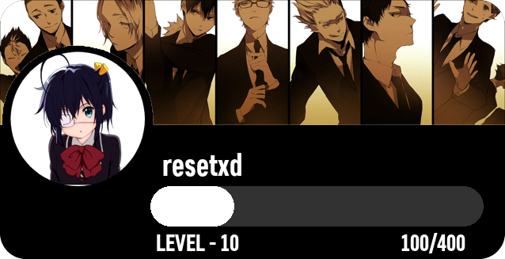\n\n<br>\n\n</details>\n\n<details>\n\n<summary> <span style=\"color:yellow\">custom_canvas</span> method</summary>\n\n\n```py\nSandbox.custom_canvas(\n resize:int = 100,\n\n senstivity:int = 200,\n card_colour: str = \"black\",\n\n border_width: int = 25,\n border_height: int = 25,\n \n avatar_frame: str = \"curvedborder\",\n avatar_size: int = 260,\n avatar_position: tuple = (53, 36),\n \n text_font: str = \"levelfont.otf\",\n\n username_position: tuple = (330,130),\n username_font_size: int = 50,\n\n level_position: tuple = (500,40),\n level_font_size: int = 50,\n\n exp_position: tuple = (775,130),\n exp_font_size: int = 50,\n\n)\n```\n\n## attribute\n - `has_background` : if set to `True` then it will add a background to the image. (default is `True`)\n - `background_colour` : color of the background. (default is `black`)\n - `canvas_size` : size of the canvas. (default is `(1000, 333)`)\n - `resize` : resize the final image. (default is 100, treat it as a percentage.)\n - `overlay` : A list of overlays to be placed on the background. (Default is `[[(1000-50, 333-50),(25, 25), \"black\", 200]]`.)\n - `avatar_frame` : `circle` `square` `curvedborder` `hexagon` or path to a self created mask. (Default is `curvedborder`.)\n - `text_font` : Default is `levelfont.otf` or path to a custom otf or ttf file type font.\n - `avatar_size` : size of the avatar. (default is `260`)\n - `avatar_position` : position of the avatar. (default is `(53, 36)`)\n - `username_position` : position of the username. (default is `(330,130)`)\n - `username_font_size` : font size of the username. (default is `50`)\n - `level_position` : position of the level. (default is `(500,40)`)\n - `level_font_size` : font size of the level. (default is `50`)\n - `exp_position` : position of the exp. (default is `(775,130)`)\n - `exp_font_size` : font size of the exp. (default is `50`)\n - `exp_bar_width` : width of the exp bar. (default is `619`)\n - `exp_bar_height` : height of the exp bar. (default is `50`)\n - `exp_bar_background_colour` : color of the exp bar background. (default is `white`)\n - `exp_bar_position` : position of the exp bar. (default is `(330, 235)`)\n - `exp_bar_curve` : curve of the exp bar. (default is `30`)\n - `extra_text` : A list of extra text to be placed on the image. (Default is `None`.)\n - `exp_bar` : The calculated exp of the user. (Default is `None`.)\n\n\n## returns \n- `bytes` which can directly be used within `discord.File` class.\n\n\n## examples\n\n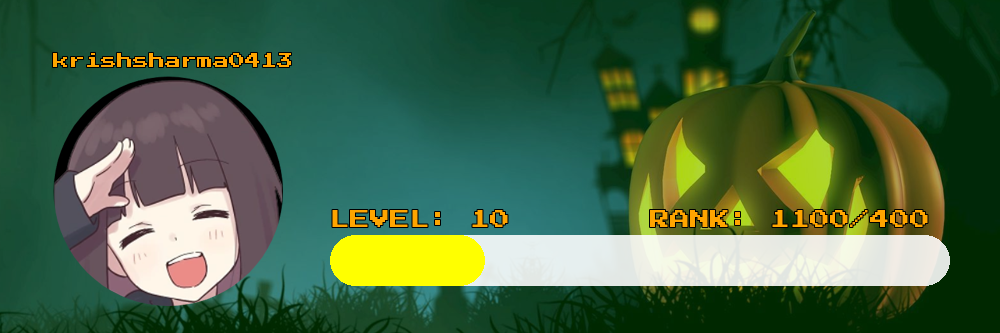\n\n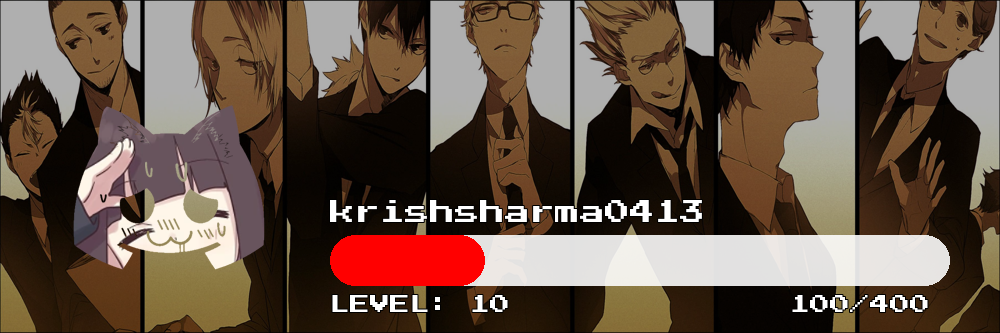\n\n\nAn Example that i really loved was this one, here is the code for it as well. (you might have to tweak a lot to make it work for you though. )\n\n```py\nfrom DiscordLevelingCard import Sandbox, Settings\nimport asyncio\nfrom PIL import Image\n\nsetting = Settings(\n background=\"./bg.jpg\",\n bar_color=\"green\",\n text_color=\"white\")\n\nasync def main():\n rank = Sandbox(\n username=\"krishsharma0413\",\n level=1,\n current_exp=10,\n max_exp=400,\n settings=setting,\n avatar=Image.open(\"./avatarimg.png\")\n )\n result = await rank.custom_canvas(\n avatar_frame=\"square\",\n avatar_size=233,\n avatar_position=(50, 50),\n exp_bar_background_colour = \"black\",\n exp_bar_height=50,\n exp_bar_width=560,\n exp_bar_curve=0,\n exp_bar_position=(70, 400),\n username_position=(320, 50),\n level_position=(320, 225),\n exp_position=(70, 330),\n canvas_size=(700, 500),\n\n overlay=[[(350, 233),(300, 50), \"white\", 100],\n [(600, 170),(50, 300), \"white\", 100]],\n\n extra_text=[\n [\"bio-\", (320, 110), 25, \"white\"],\n [\"this can very well be a bio\", (320, 140), 25, \"white\"],\n [\"even mutiple lines!\", (320, 170), 25, \"white\"],\n [\"if we remove bio- even more!\", (320, 200), 25, \"white\"],\n ]\n\n )\n \n # you don't need this line if you are using this in discord.py\n Image.open(result).save(\"result.png\", \"PNG\")\n\n\nasyncio.run(main())\n```\n\nand this is how it looks :D\n\n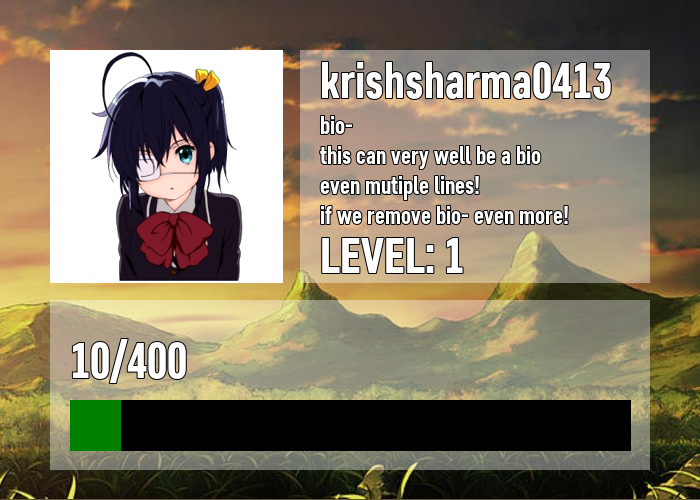\n\n<br>\n\n</details>\n\n\n<br><br>\n\nif you want to see changelog then click [here](https://github.com/krishsharma0413/DiscordLevelingCard/blob/main/CHANGELOG.md)\n\n<br><br>\nplease star the <a href=\"https://github.com/krishsharma0413/DiscordLevelingCard\">repository</a> if you like it :D\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A library with leveling cards for your discord bot.",
"version": "0.6.0",
"project_urls": {
"Homepage": "https://github.com/krishsharma0413/DiscordLevelingCard",
"Repository": "https://github.com/krishsharma0413/DiscordLevelingCard"
},
"split_keywords": [
"discord",
"leveling",
"card",
"image",
"discord.py",
"disnake",
"nextcord",
"rank",
"ranking",
"level",
"discord-bot",
"bot",
"discord-leveling",
"level-card",
"discord-leveling-card",
"discord-rank-card",
"sandbox",
"custom",
"custom-card"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "41009daa68c97a0036276abc6c300c0a52a63cb6c308257291f7cecdf5a8cc54",
"md5": "673fa7589fca1d58598f9ce095e2ef87",
"sha256": "ef61894ecc925b8b58bda1b7450780baac7e5ab944fbc3de9d15c59bce0fa3d0"
},
"downloads": -1,
"filename": "discordlevelingcard-0.6.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "673fa7589fca1d58598f9ce095e2ef87",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8,<4",
"size": 72814,
"upload_time": "2023-06-02T16:33:27",
"upload_time_iso_8601": "2023-06-02T16:33:27.810805Z",
"url": "https://files.pythonhosted.org/packages/41/00/9daa68c97a0036276abc6c300c0a52a63cb6c308257291f7cecdf5a8cc54/discordlevelingcard-0.6.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "13fa98758387ff05f8f849d40ff411c038a70348194bad987f6e95ce550171b0",
"md5": "bf258862d65b538e8e473b94ce3c1f85",
"sha256": "075f2c7b271c6c853e1d14e4c06f6370bd5dde12c4b38baf45eee924404752f0"
},
"downloads": -1,
"filename": "discordlevelingcard-0.6.0.tar.gz",
"has_sig": false,
"md5_digest": "bf258862d65b538e8e473b94ce3c1f85",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8,<4",
"size": 69266,
"upload_time": "2023-06-02T16:33:32",
"upload_time_iso_8601": "2023-06-02T16:33:32.623992Z",
"url": "https://files.pythonhosted.org/packages/13/fa/98758387ff05f8f849d40ff411c038a70348194bad987f6e95ce550171b0/discordlevelingcard-0.6.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-06-02 16:33:32",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "krishsharma0413",
"github_project": "DiscordLevelingCard",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "discordlevelingcard"
}