# discordwebhook
[](https://pypi.org/project/discordwebhook/)
[](https://opensource.org/licenses/MIT)
[](https://codecov.io/gh/10mohi6/discord-webhook-python)
[](https://travis-ci.com/10mohi6/discord-webhook-python)
[](https://pypi.org/project/discordwebhook/)
[](https://pepy.tech/project/discordwebhook)
discordwebhook is a python library for discord webhook with discord rest api on Python 3.6 and above.
## Installation
$ pip install discordwebhook
## Usage
### basic
```python
from discordwebhook import Discord
discord = Discord(url="<your webhook url>")
discord.post(content="Hello, world.")
```

### basic, username and avatar_url
```python
from discordwebhook import Discord
discord = Discord(url="<your webhook url>")
discord.post(
content="Hello, world.",
username="10mohi6",
avatar_url="https://avatars2.githubusercontent.com/u/38859131?s=460&v=4"
)
```
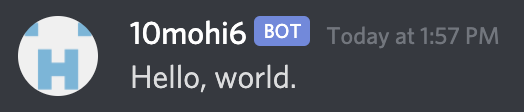
### basic embed
```python
from discordwebhook import Discord
discord = Discord(url="<your webhook url>")
discord.post(
embeds=[{"title": "Embed Title", "description": "Embed description"}],
)
```
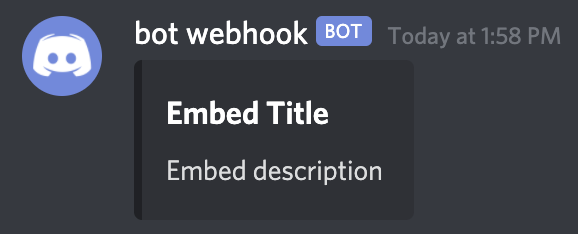
### advanced embed
```python
from discordwebhook import Discord
discord = Discord(url="<your webhook url>")
discord.post(
embeds=[
{
"author": {
"name": "Embed Name",
"url": "https://github.com/10mohi6/discord-webhook-python",
"icon_url": "https://picsum.photos/24/24",
},
"title": "Embed Title",
"description": "Embed description",
"fields": [
{"name": "Field Name 1", "value": "Value 1", "inline": True},
{"name": "Field Name 2", "value": "Value 2", "inline": True},
{"name": "Field Name 3", "value": "Field Value 3"},
],
"thumbnail": {"url": "https://picsum.photos/80/60"},
"image": {"url": "https://picsum.photos/400/300"},
"footer": {
"text": "Embed Footer",
"icon_url": "https://picsum.photos/20/20",
},
}
],
)
```
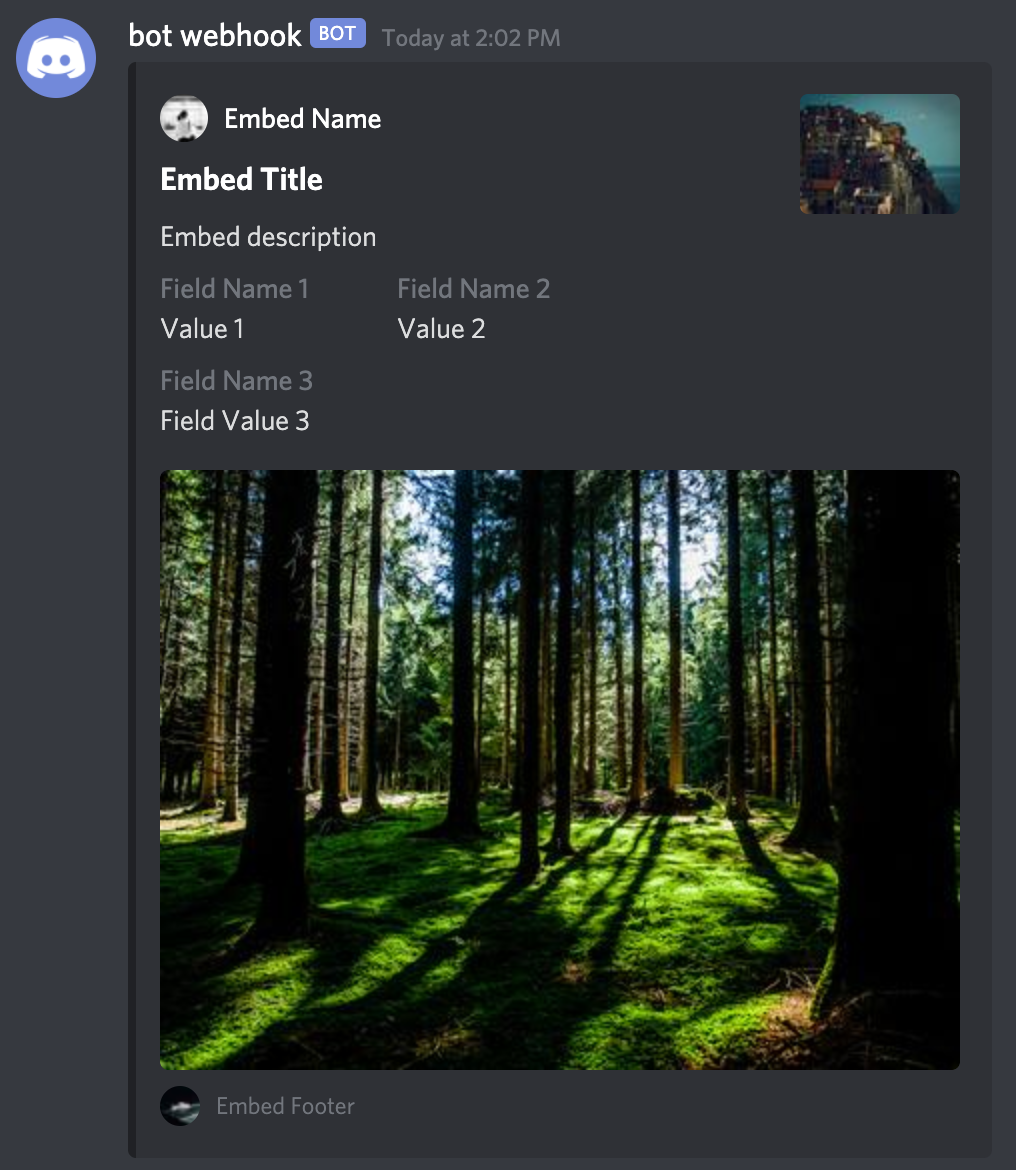
### send file
```python
from discordwebhook import Discord
discord = Discord(url="<your webhook url>")
discord.post(
file={
"file1": open("tests/file1.jpg", "rb"),
"file2": open("tests/file2.jpg", "rb"),
},
)
```
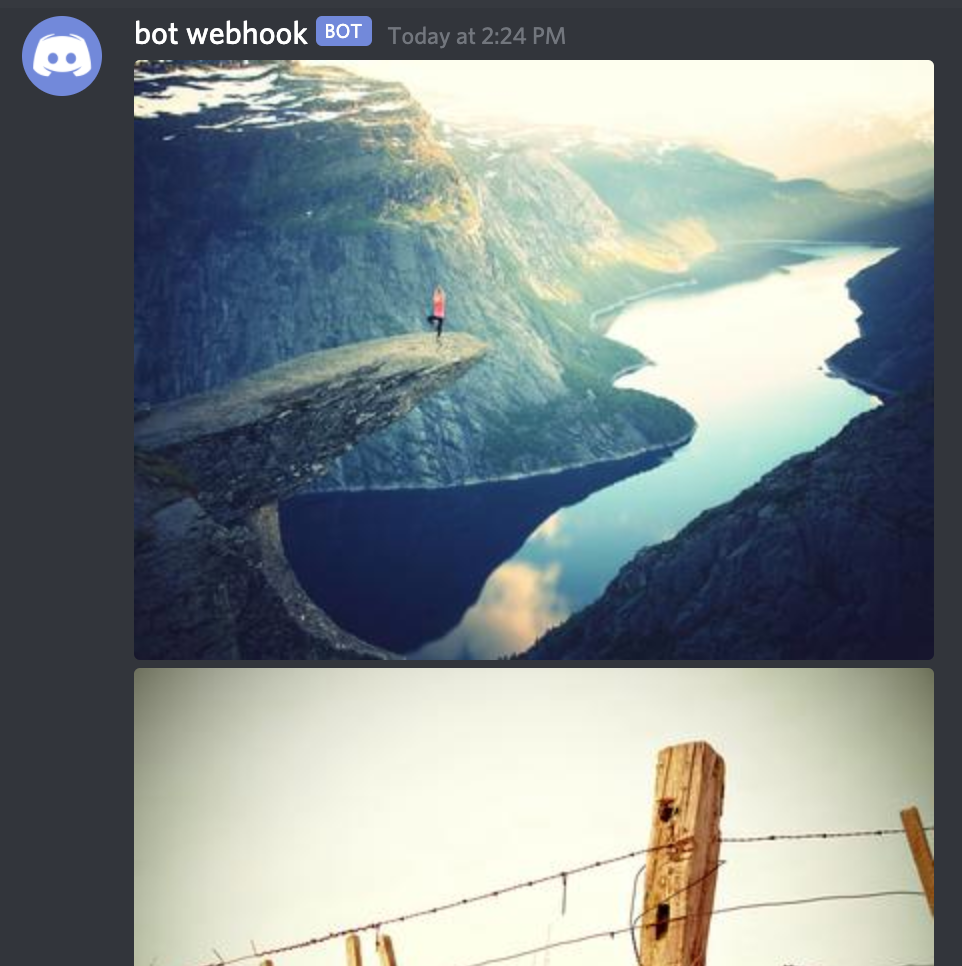
## Getting started
For help getting started with discord webhook, view our online [documentation](https://discordapp.com/developers/docs/resources/webhook).
## Contributing
1. Fork it
2. Create your feature branch (`git checkout -b my-new-feature`)
3. Commit your changes (`git commit -am 'Add some feature'`)
4. Push to the branch (`git push origin my-new-feature`)
5. Create new Pull Request
Raw data
{
"_id": null,
"home_page": "https://github.com/10mohi6/discord-webhook-python",
"name": "discordwebhook",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6.0",
"maintainer_email": "",
"keywords": "discord webhook api python",
"author": "10mohi6",
"author_email": "10.mohi.6.y@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/15/e1/9a4b4ed4aded1c4dc6e708d8d10dd2a72097f8711f027e4cc76364783d83/discordwebhook-1.0.3.tar.gz",
"platform": "",
"description": "# discordwebhook\n\n[](https://pypi.org/project/discordwebhook/)\n[](https://opensource.org/licenses/MIT)\n[](https://codecov.io/gh/10mohi6/discord-webhook-python)\n[](https://travis-ci.com/10mohi6/discord-webhook-python)\n[](https://pypi.org/project/discordwebhook/)\n[](https://pepy.tech/project/discordwebhook)\n\ndiscordwebhook is a python library for discord webhook with discord rest api on Python 3.6 and above.\n\n\n## Installation\n\n $ pip install discordwebhook\n\n## Usage\n\n### basic\n```python\nfrom discordwebhook import Discord\n\ndiscord = Discord(url=\"<your webhook url>\")\ndiscord.post(content=\"Hello, world.\")\n```\n\n\n### basic, username and avatar_url\n```python\nfrom discordwebhook import Discord\n\ndiscord = Discord(url=\"<your webhook url>\")\ndiscord.post(\n content=\"Hello, world.\",\n username=\"10mohi6\",\n avatar_url=\"https://avatars2.githubusercontent.com/u/38859131?s=460&v=4\"\n)\n```\n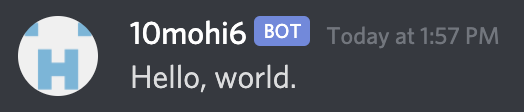\n\n### basic embed\n```python\nfrom discordwebhook import Discord\n\ndiscord = Discord(url=\"<your webhook url>\")\ndiscord.post(\n embeds=[{\"title\": \"Embed Title\", \"description\": \"Embed description\"}],\n)\n```\n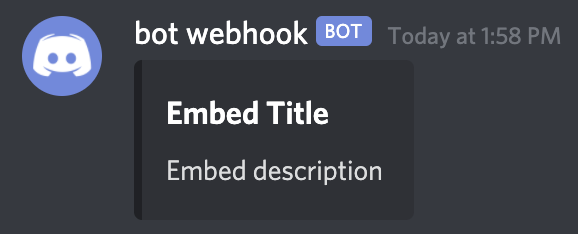\n\n### advanced embed\n```python\nfrom discordwebhook import Discord\n\ndiscord = Discord(url=\"<your webhook url>\")\ndiscord.post(\n embeds=[\n {\n \"author\": {\n \"name\": \"Embed Name\",\n \"url\": \"https://github.com/10mohi6/discord-webhook-python\",\n \"icon_url\": \"https://picsum.photos/24/24\",\n },\n \"title\": \"Embed Title\",\n \"description\": \"Embed description\",\n \"fields\": [\n {\"name\": \"Field Name 1\", \"value\": \"Value 1\", \"inline\": True},\n {\"name\": \"Field Name 2\", \"value\": \"Value 2\", \"inline\": True},\n {\"name\": \"Field Name 3\", \"value\": \"Field Value 3\"},\n ],\n \"thumbnail\": {\"url\": \"https://picsum.photos/80/60\"},\n \"image\": {\"url\": \"https://picsum.photos/400/300\"},\n \"footer\": {\n \"text\": \"Embed Footer\",\n \"icon_url\": \"https://picsum.photos/20/20\",\n },\n }\n ],\n)\n```\n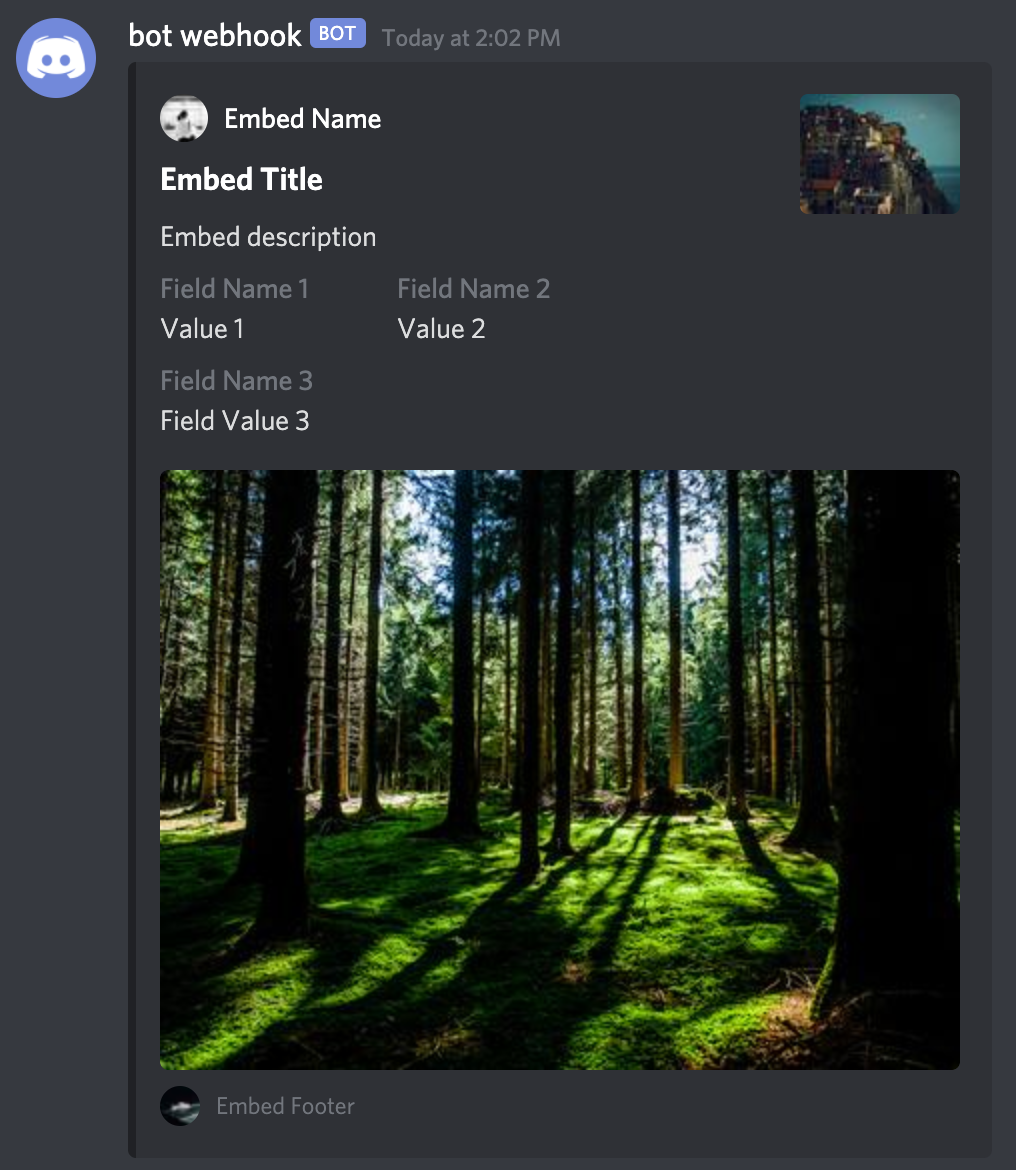\n\n### send file\n```python\nfrom discordwebhook import Discord\n\ndiscord = Discord(url=\"<your webhook url>\")\ndiscord.post(\n file={\n \"file1\": open(\"tests/file1.jpg\", \"rb\"),\n \"file2\": open(\"tests/file2.jpg\", \"rb\"),\n },\n)\n```\n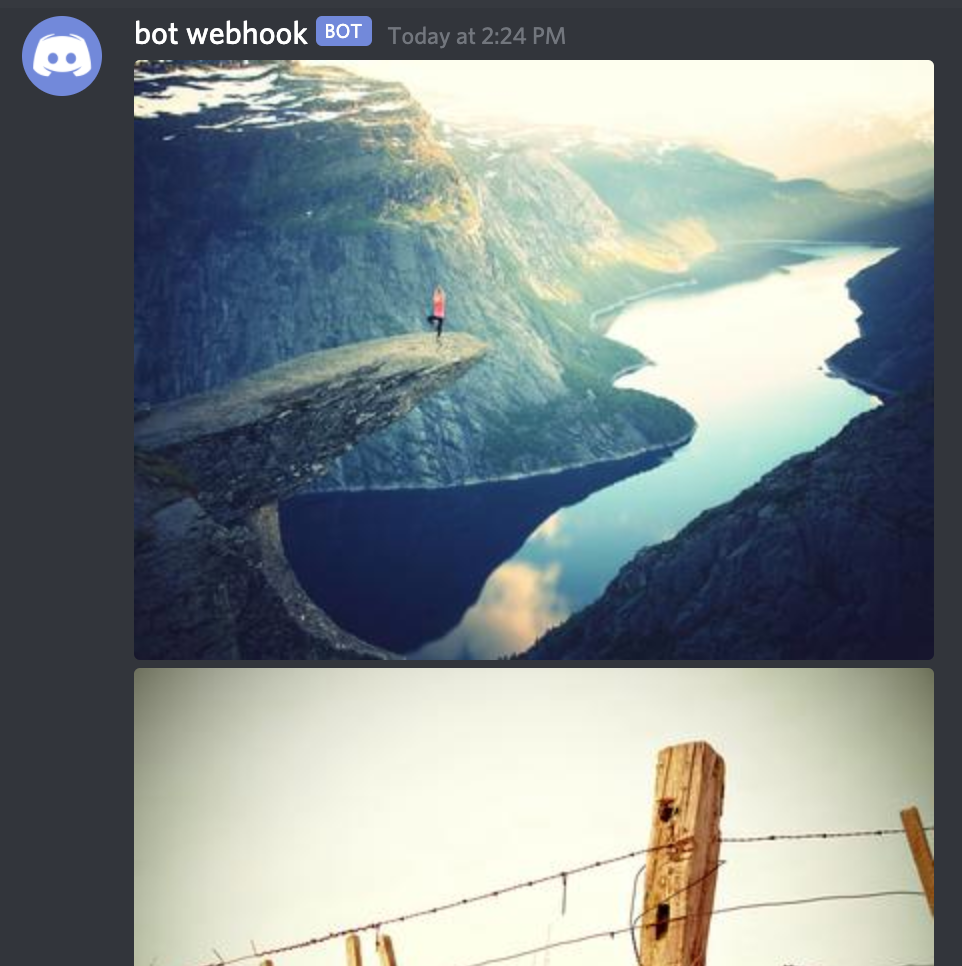\n\n\n## Getting started\n\nFor help getting started with discord webhook, view our online [documentation](https://discordapp.com/developers/docs/resources/webhook).\n\n\n## Contributing\n\n1. Fork it\n2. Create your feature branch (`git checkout -b my-new-feature`)\n3. Commit your changes (`git commit -am 'Add some feature'`)\n4. Push to the branch (`git push origin my-new-feature`)\n5. Create new Pull Request\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "discordwebhlok is a python library for discord webhook with discord rest api on Python 3.6 and above.",
"version": "1.0.3",
"project_urls": {
"Homepage": "https://github.com/10mohi6/discord-webhook-python"
},
"split_keywords": [
"discord",
"webhook",
"api",
"python"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "0055fdf733fc29268d278e6d28daed6e9772d3ba9d01a53f365a568222f6958c",
"md5": "342cf63938dfa0947b753dfa53bc2298",
"sha256": "0dddc400d631c57795cae03939aa37163299d2d7e3361036fa508836326c7ea6"
},
"downloads": -1,
"filename": "discordwebhook-1.0.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "342cf63938dfa0947b753dfa53bc2298",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6.0",
"size": 4013,
"upload_time": "2021-12-15T15:08:55",
"upload_time_iso_8601": "2021-12-15T15:08:55.810342Z",
"url": "https://files.pythonhosted.org/packages/00/55/fdf733fc29268d278e6d28daed6e9772d3ba9d01a53f365a568222f6958c/discordwebhook-1.0.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "15e19a4b4ed4aded1c4dc6e708d8d10dd2a72097f8711f027e4cc76364783d83",
"md5": "3b0d70210a267c89bee83784d6468e3b",
"sha256": "b2a89cc322e036c36f694f23a02023e79d6916f5b6be127de2733b7b1f93445c"
},
"downloads": -1,
"filename": "discordwebhook-1.0.3.tar.gz",
"has_sig": false,
"md5_digest": "3b0d70210a267c89bee83784d6468e3b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6.0",
"size": 3672,
"upload_time": "2021-12-15T15:08:57",
"upload_time_iso_8601": "2021-12-15T15:08:57.837980Z",
"url": "https://files.pythonhosted.org/packages/15/e1/9a4b4ed4aded1c4dc6e708d8d10dd2a72097f8711f027e4cc76364783d83/discordwebhook-1.0.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2021-12-15 15:08:57",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "10mohi6",
"github_project": "discord-webhook-python",
"travis_ci": true,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "pytest",
"specs": [
[
"==",
"6.2.5"
]
]
},
{
"name": "pytest-cov",
"specs": [
[
"==",
"3.0.0"
]
]
},
{
"name": "requests",
"specs": [
[
"==",
"2.26.0"
]
]
}
],
"lcname": "discordwebhook"
}