# Appwrite Middleware and Authentication for Django



[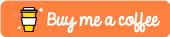](https://www.buymeacoffee.com/khashashin)
A Django package to authenticate users with Appwrite using middleware and/or Django Rest Framework (DRF) authentication classes.
## Installation
To install `django_appwrite`, simply run:
```bash
$ pip install django-appwrite
```
## Usage
### Middleware
Add `django_appwrite.middleware.AppwriteMiddleware` to your `MIDDLEWARE` list in your Django project's `settings.py` file. It should be placed after the `AuthenticationMiddleware`:
```python
MIDDLEWARE = [
...,
'django.contrib.auth.middleware.AuthenticationMiddleware',
'django_appwrite.middleware.AppwriteMiddleware',
...
]
```
### Django Rest Framework Authentication
If you're using Django Rest Framework, you can use the `AppwriteAuthentication` class.
Add `django_appwrite.authentication.AppwriteAuthentication` to the `DEFAULT_AUTHENTICATION_CLASSES` in your `settings.py`:
```python
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': (
'django_appwrite.authentication.AppwriteAuthentication',
...
),
...
}
```
## Configuration
Configure the Appwrite client settings in your Django settings file:
```python
APPWRITE = {
'PROJECT_ENDPOINT': 'https://example.com/v1',
'PROJECT_ID': 'PROJECT_ID',
}
```
| Setting | Default | Description |
|--------------------|--------------------|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|
| `PROJECT_ENDPOINT` | | The endpoint of your Appwrite project. You can find this in the Appwrite console under Settings > General. |
| `PROJECT_ID` | | The ID of your Appwrite project. You can find this in the Appwrite console under Settings > General. |
| `AUTH_HEADER` | HTTP_AUTHORIZATION | The header name that will be used to get the JWT from the authorization header. The value of this header should be `Bearer [JWT]` |
| `VERIFY_EMAIL` | False | If set to `True`, the middleware will check if the user's email address has been verified in Appwrite before authenticating the user. If the email address has not been verified, the user will not be authenticated. |
| `VERIFY_PHONE` | False | If set to `True`, the middleware will check if the user's phone number has been verified in Appwrite before authenticating the user. If the phone number has not been verified, the user will not be authenticated. |
| `PREFIX_EMAIL` | | The prefix to use for the email address when checking if the user's email address has been verified. This is useful if you are integrating django_appwrite to existing projects that already have users. |
## How it works
1. **Middleware:** This middleware class will authorize the user by checking the JWT in the Authorization header. The JWT is obtained from the Authorization header and is then sent to the Appwrite API to verify the JWT. If the JWT is valid, the user will be authenticated.
2. **Authentication Class:** When used with Django Rest Framework, the authentication class provides an additional way to authenticate users via Appwrite.
Please note that this middleware is intended to be used in conjunction with the Appwrite client-side SDK to authorize users on the frontend, and does not provide any APIs for user authentication on its own.
## Example
This is an example of how you can configure your frontend to use this middleware. Note that this example uses the [axios](https://axios-http.com/) library.
```javascript
// axios interceptor to add jwt to the request header
import axios from 'axios';
import { Client, Account } from 'appwrite';
const client = new Client()
.setEndpoint('https://example.com/v1')
.setProject('PROJECT_ID')
const account = new Account(client);
axios.interceptors.request.use(async (config) => {
// get jwt from your auth service/provider
const { jwt } = await yourAuthService();
config.headers = {
...config.headers,
Authorization: `Bearer ${jwt}`
}
return config;
});
```
## Important Note on CSRF Protection
When using the `AppwriteMiddleware`, be aware that Django's built-in CSRF protection will expect a CSRF token for any mutating HTTP methods (like POST, PUT, DELETE, etc.). If you're using Appwrite tokens for authentication with this middleware, you might encounter CSRF validation issues, since the CSRF token might not be present or validated.
If you're only using the Appwrite token for authentication, and you're confident about the origin protection measures of your front-end, you might consider exempting certain views from CSRF protection. However, do this with caution and ensure you understand the security implications. Always ensure that your application is protected against Cross-Site Request Forgery attacks.
You can exempt views from CSRF protection using the `@csrf_exempt` decorator or by using the `csrf_exempt` function on specific routes in your `urls.py`. However, these methods can leave your application vulnerable if used carelessly. Always prioritize the security of your application and its users.
## License
The django-appwrite package is released under the MIT License. See the bundled [LICENSE](LICENSE) file for details.
Raw data
{
"_id": null,
"home_page": "https://github.com/khashashin/django-appwrite",
"name": "django-appwrite",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "appwrite auth django",
"author": "Yusuf Khasbulatov",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/5b/d2/6eb593b00bdc30a2aa1f4f637c644dac0034ca449b18bd01f88da213bf59/django-appwrite-1.6.0.tar.gz",
"platform": null,
"description": "# Appwrite Middleware and Authentication for Django\r\n\r\n\r\n\r\n\r\n[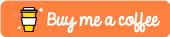](https://www.buymeacoffee.com/khashashin)\r\n\r\nA Django package to authenticate users with Appwrite using middleware and/or Django Rest Framework (DRF) authentication classes.\r\n\r\n## Installation\r\n\r\nTo install `django_appwrite`, simply run:\r\n\r\n```bash\r\n$ pip install django-appwrite\r\n```\r\n\r\n## Usage\r\n### Middleware\r\nAdd `django_appwrite.middleware.AppwriteMiddleware` to your `MIDDLEWARE` list in your Django project's `settings.py` file. It should be placed after the `AuthenticationMiddleware`:\r\n\r\n```python\r\nMIDDLEWARE = [\r\n ...,\r\n 'django.contrib.auth.middleware.AuthenticationMiddleware',\r\n 'django_appwrite.middleware.AppwriteMiddleware',\r\n ...\r\n]\r\n```\r\n### Django Rest Framework Authentication\r\nIf you're using Django Rest Framework, you can use the `AppwriteAuthentication` class.\r\nAdd `django_appwrite.authentication.AppwriteAuthentication` to the `DEFAULT_AUTHENTICATION_CLASSES` in your `settings.py`:\r\n```python\r\nREST_FRAMEWORK = {\r\n 'DEFAULT_AUTHENTICATION_CLASSES': (\r\n 'django_appwrite.authentication.AppwriteAuthentication',\r\n ...\r\n ),\r\n ...\r\n}\r\n```\r\n## Configuration\r\nConfigure the Appwrite client settings in your Django settings file:\r\n```python\r\nAPPWRITE = {\r\n 'PROJECT_ENDPOINT': 'https://example.com/v1',\r\n 'PROJECT_ID': 'PROJECT_ID',\r\n}\r\n```\r\n| Setting | Default | Description |\r\n|--------------------|--------------------|-----------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------|\r\n| `PROJECT_ENDPOINT` | | The endpoint of your Appwrite project. You can find this in the Appwrite console under Settings > General. |\r\n| `PROJECT_ID` | | The ID of your Appwrite project. You can find this in the Appwrite console under Settings > General. |\r\n| `AUTH_HEADER` | HTTP_AUTHORIZATION | The header name that will be used to get the JWT from the authorization header. The value of this header should be `Bearer [JWT]` |\r\n| `VERIFY_EMAIL` | False | If set to `True`, the middleware will check if the user's email address has been verified in Appwrite before authenticating the user. If the email address has not been verified, the user will not be authenticated. |\r\n| `VERIFY_PHONE` | False | If set to `True`, the middleware will check if the user's phone number has been verified in Appwrite before authenticating the user. If the phone number has not been verified, the user will not be authenticated. |\r\n| `PREFIX_EMAIL` | | The prefix to use for the email address when checking if the user's email address has been verified. This is useful if you are integrating django_appwrite to existing projects that already have users. |\r\n\r\n## How it works\r\n1. **Middleware:** This middleware class will authorize the user by checking the JWT in the Authorization header. The JWT is obtained from the Authorization header and is then sent to the Appwrite API to verify the JWT. If the JWT is valid, the user will be authenticated.\r\n2. **Authentication Class:** When used with Django Rest Framework, the authentication class provides an additional way to authenticate users via Appwrite.\r\n\r\nPlease note that this middleware is intended to be used in conjunction with the Appwrite client-side SDK to authorize users on the frontend, and does not provide any APIs for user authentication on its own.\r\n\r\n## Example\r\nThis is an example of how you can configure your frontend to use this middleware. Note that this example uses the [axios](https://axios-http.com/) library.\r\n\r\n```javascript\r\n// axios interceptor to add jwt to the request header\r\nimport axios from 'axios';\r\nimport { Client, Account } from 'appwrite';\r\n\r\nconst client = new Client()\r\n .setEndpoint('https://example.com/v1')\r\n .setProject('PROJECT_ID')\r\n \r\nconst account = new Account(client);\r\n\r\naxios.interceptors.request.use(async (config) => {\r\n // get jwt from your auth service/provider\r\n const { jwt } = await yourAuthService();\r\n \r\n config.headers = {\r\n ...config.headers,\r\n Authorization: `Bearer ${jwt}`\r\n }\r\n \r\n return config;\r\n});\r\n```\r\n## Important Note on CSRF Protection\r\nWhen using the `AppwriteMiddleware`, be aware that Django's built-in CSRF protection will expect a CSRF token for any mutating HTTP methods (like POST, PUT, DELETE, etc.). If you're using Appwrite tokens for authentication with this middleware, you might encounter CSRF validation issues, since the CSRF token might not be present or validated.\r\n\r\nIf you're only using the Appwrite token for authentication, and you're confident about the origin protection measures of your front-end, you might consider exempting certain views from CSRF protection. However, do this with caution and ensure you understand the security implications. Always ensure that your application is protected against Cross-Site Request Forgery attacks.\r\n\r\nYou can exempt views from CSRF protection using the `@csrf_exempt` decorator or by using the `csrf_exempt` function on specific routes in your `urls.py`. However, these methods can leave your application vulnerable if used carelessly. Always prioritize the security of your application and its users.\r\n## License\r\nThe django-appwrite package is released under the MIT License. See the bundled [LICENSE](LICENSE) file for details.\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Django Middleware to authenticate users with Appwrite",
"version": "1.6.0",
"project_urls": {
"Documentation": "https://github.com/khashashin/django-appwrite/blob/main/README.md",
"Funding": "https://donate.pypi.org",
"Homepage": "https://github.com/khashashin/django-appwrite",
"Say Thanks!": "https://patreon.com/khashashin",
"Source": "https://github.com/khashashin/django-appwrite",
"Tracker": "https://github.com/khashashin/django-appwrite/issues"
},
"split_keywords": [
"appwrite",
"auth",
"django"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "5bd26eb593b00bdc30a2aa1f4f637c644dac0034ca449b18bd01f88da213bf59",
"md5": "4c83793109d7f72cd2d9b91d49f4b0ab",
"sha256": "fe70571adb0cb07e0ba93fa85ab835b2cd9fab98d132f462acfd1583ec70d91a"
},
"downloads": -1,
"filename": "django-appwrite-1.6.0.tar.gz",
"has_sig": false,
"md5_digest": "4c83793109d7f72cd2d9b91d49f4b0ab",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 9409,
"upload_time": "2023-11-05T21:15:37",
"upload_time_iso_8601": "2023-11-05T21:15:37.965804Z",
"url": "https://files.pythonhosted.org/packages/5b/d2/6eb593b00bdc30a2aa1f4f637c644dac0034ca449b18bd01f88da213bf59/django-appwrite-1.6.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-11-05 21:15:37",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "khashashin",
"github_project": "django-appwrite",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "django-appwrite"
}