# Django Form Generator
django form generator will help you create forms very easily.
you can use it any where in your html files.
there is an API-manager tool for you to call some APIs on loading form or after submit.
---
## Installation
---
- install it via pip:
```bash
pip install django-form-generator
```
- add (`'django_form_generator'`, `'django_htmx'`, `'crispy_forms'`, `'crispy_bootstrap5'`, `'captcha'`, `'tempus_dominus', 'rest_framework', 'drf_recaptcha'`) to your `INSTALLED_APPS`:
```python
INSTALLED_APPS = [
...
'django_htmx',
'crispy_forms',
'crispy_bootstrap5',
'captcha',
'tempus_dominus',
'rest_framework',
'drf_recaptcha',
'django_form_generator',
]
```
- do a `migrate`:
```bash
python manage.py migrate
```
- do a `collectstatic`:
```bash
python manage.py collectstatic
```
- finally include django_form_generator urls to your `urls.py`
```python
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
...
path('form-generator/', include(('django_form_generator.urls', 'django_form_generator'), namespace='django_form_generator')),
...
]
```
---
## Requirements
---
* Python >= 3.10
* Django >= 4.0
---
## Form Usage
---
- ### regular html usage:
```html
<!doctype html>
<html lang="en">
<head>
...
</head>
<body>
{% load django_form_generator %}
{% render_form 1 %} {# it will render the form that has id of 1 #}
</body>
</html>
```
- ### RichText fields:
***To use this tags on a Richtext field like `CKeditor` or `tinymce`.***
>Note: In some richtext packages some elements (specialy jinja elements) will be removed from the field
there are some tweaks to solve this problems on the packages documentations.
- #### Admin panel:
*CK-Editor example*
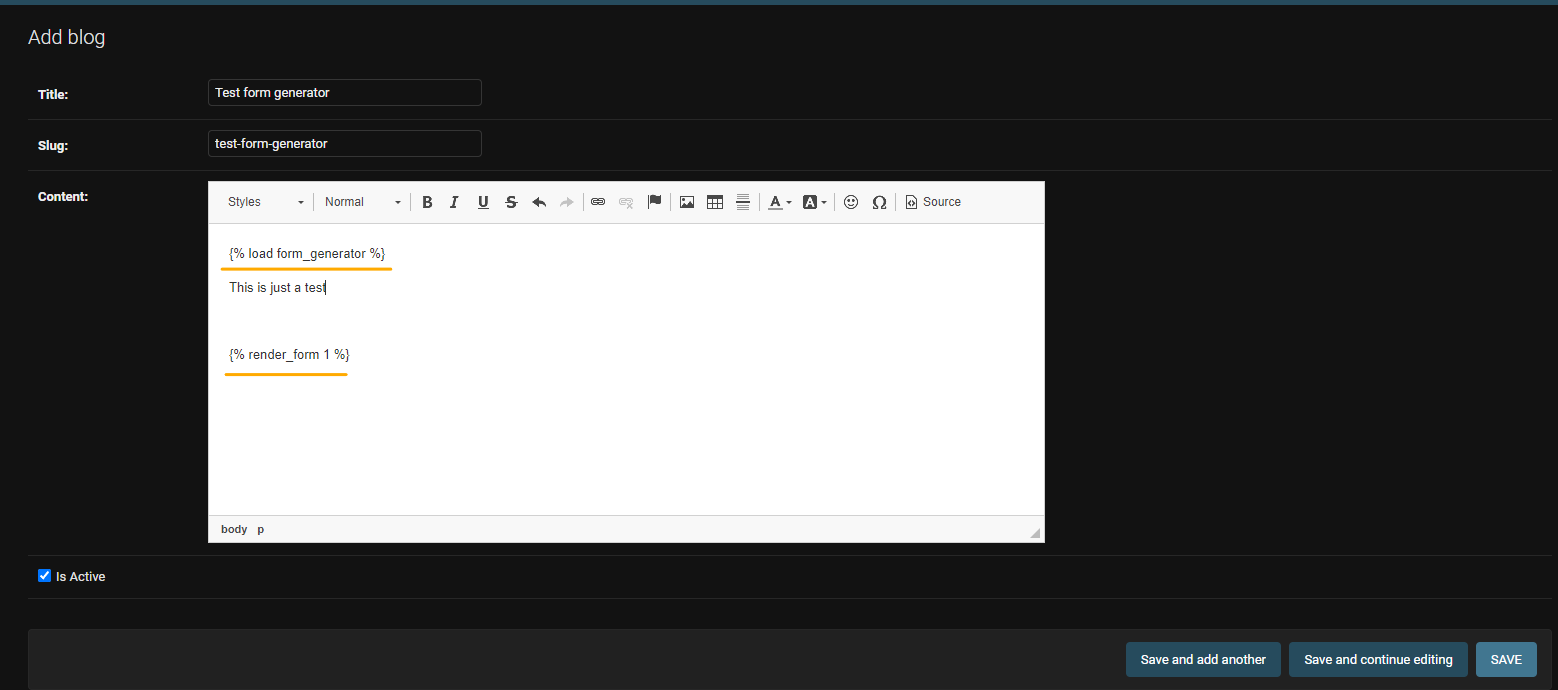
- ```./app_name/templates/index.html ```
```html
<!doctype html>
<html lang="en">
<head>
...
</head>
<body>
{% load django_form_generator %}
{{ object.rich_text_field|eval_data }}
</body>
</html>
```
---
## API manager Usage
---
**There is also an API manager for the forms**
- ### Admin panel:
- **example:**
>Note: `first_name`, `last_name` and `job` are the name of the fields that are defined in a form which this api is assigned to.
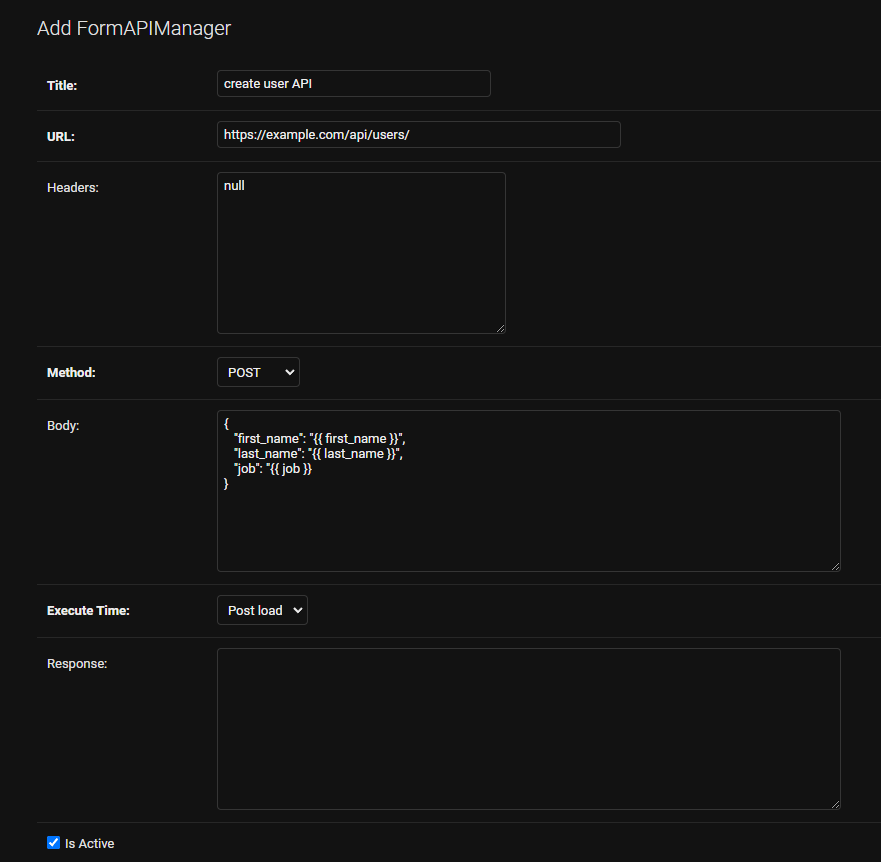
---
>***Note: assigend apis will be called automatically.
the ones with execution_time of `PRE` will be called when form renders.
and the ones with execution_time of `POST` will be called after form submition.***
>***Note: in the response field you can write HTML tags
>it will be rendrend as `safe`***.
>***Note: You have access to `request` globaly in api manager***
***Also you have access to the response of the api. so you can use `Jinja` syntax to access data.
You can use this feature on the (`url`, `body`, `response`) fields of `APIManager` model***
- ### Creating API
- **example:**
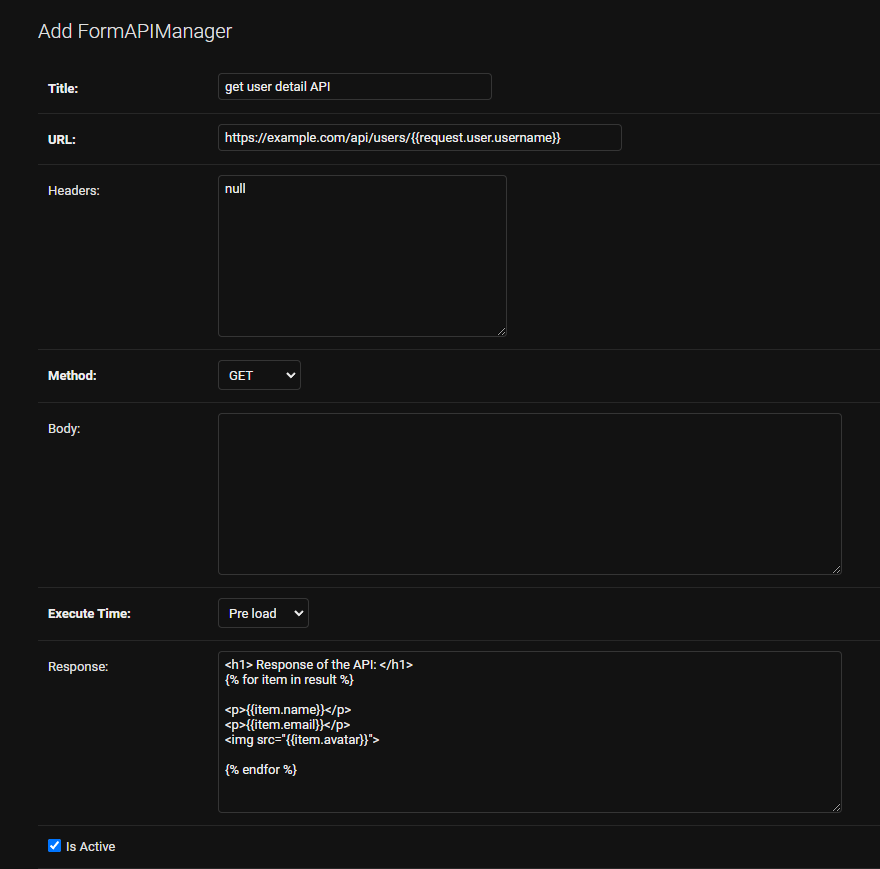
>Note: To show the response use the template tag of `{% render_pre_api form_id api_id %}` inside your html file that the `form` is there.
- ### Response
example:
- output of the api:
```json
{
"result": [
{
"first_name": "john",
"last_name": "doe",
"avatar": "https://www.example.com/img/john-doe.png",
},
{
"first_name": "mary",
"last_name": "doe",
"avatar": "https://www.example.com/img/mary-doe.png",
}
]
}
```
- in the response field you can do:
```html
<h1> Response of the API: </h1>
{% for item in result %}
<p>{{item.first_name}}</p>
<p>{{item.last_name}}</p>
<img src="{{item.avatar}}">
{% endfor %}
```
- ### html usage:
```html
<!doctype html>
<html lang="en">
<head>
...
</head>
<body>
{% load django_form_generator %}
{% render_pre_api 1 %} {# it will render all pre apis that are assigned to a form with id of 1 #}
{% render_pre_api 1 6 %} {# it will render the pre api with id of 6 which is assigned to a form with id of 1 #}
{% render_post_api 1 %} {# it will render all pre apis that are assigned to a form with id of 1 #}
{% render_post_api 1 7 %} {# it will render the post api with id of 7 which is assigned to a form with id of 1 #}
</body>
</html>
```
---
## Extra
---
- ### API Manager:
you can access the whole submited data by adding `{{ form_data }}` to your api `body`.
- ### Form Style:
to have multiple styles the html of `template_name_p` (attribute of django.forms.Form) will be replace by our html file. (`{{form.as_p}}`)
there are 3 styles(or actualy styel of rendering fields) for the forms:
1. In-line Style[^1].
2. In-order Style[^2].
3. Dynamic Style[^3].
[^1]: In every line two fields will be rendered.
[^2]: In every line one field will be rendered.
[^3]: this style will render fields respect to the field position (`inline`, `inorder`, `break`)
>Note: If you want to render two fields `inline` and one field `inorder` you should use `break` for the second field
- example:
- field1 inline
- field2 break
- field3 inorder
>Note: You can add your custom styles by create new TextChoices object and add it to `settings.py`
- example:
1. *myapp/const.py*
```python
from django.db.models import TextChoices
class CustomFormGeneratorStyle(TextChoices):
MY_STYLE = "myapp/templates/my_style.html", 'My Custom Style'
...
```
2. *myproject/settings.py*
```python
DJANGO_FORM_GENERATOR = {
"FORM_STYLE_CHOICES": "myapp.const.CustomFormGeneratorStyle"
}
```
- ### URLS:
```python
# to access the form you can call this url:
"""GET: http://127.0.0.1:8000/form-generator/form/1/"""
#or
reverse('django_form_generator:form_detail', kwargs={'pk': 1})
#api:
reverse('django_form_generator:api_form_detail', kwargs={'pk': 1})
# to access the response of the form you can call this url the kwarg_lookup is UUID field
"""GET: http://127.0.0.1:8000/form-generator/form-response/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/"""
#or
reverse('django_form_generator:form_response', kwargs={'unique_id': 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'})
#api:
reverse('django_form_generator:api_form_response', kwargs={'unique_id': 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'})
```
- ### Settings:
**below is the default settings for django_form_generator. you can change them by adding it to your `settings.py`**
```python
DJANGO_FORM_GENERATOR = {
'FORM_RESPONSE_SAVE': 'django_form_generator.models.save_form_response',
'FORM_EVALUATIONS': {'form_data': '{{form_data}}'},
'FORM_GENERATOR_FORM': 'django_form_generator.forms.FormGeneratorForm',
'FORM_RESPONSE_FORM': 'django_form_generator.forms.FormGeneratorResponseForm',
'FORM_STYLE_CHOICES': 'django_form_generator.const.FormStyle',
'FORM_MANAGER': 'django_form_generator.managers.FormManager',
'FORM_GENERATOR_SERIALIZER': 'django_form_generator.api.serializers.FormGeneratorSerializer',
'FORM_RESPONSE_SERIALIZER': 'django_form_generator.api.serializers.FormGeneratorResponseSerializer',
}
```
---
## Attention
---
**To have nice styled fields you can add crispy-form to your `settings.py`**
```python
CRISPY_ALLOWED_TEMPLATE_PACKS = 'bootstrap5'
CRISPY_TEMPLATE_PACK = 'bootstrap5'
```
**To access google recaptcha you should add these to your `settings.py`**
>Note: you can have public & private keys by registring your domain on [Google recaptcha admin console](https://www.google.com/recaptcha/admin/create)
```python
RECAPTCHA_PUBLIC_KEY = 'public_key'
RECAPTCHA_PRIVATE_KEY = 'private_key'
# ===== FOR DRF =========
DRF_RECAPTCHA_SECRET_KEY = "YOUR SECRET KEY"
```
>Note: If you don't want to use google-recaptcha(`django-recaptcah`)
you should add below code to your `settings.py`
```python
SILENCED_SYSTEM_CHECKS = ['captcha.recaptcha_test_key_error']
```
---
## Dependency Packages
---
1. [HTMX](https://htmx.org/docs/)
2. [CRISPY-FORMS (crispy-bootstrap5)](https://github.com/django-crispy-forms/crispy-bootstrap5)
3. [REQUESTS](https://requests.readthedocs.io/en/latest/)
4. [DJANGO-RECAPTCHA](https://pypi.org/project/django-recaptcha/)
5. [DJANGO-TEMPUS-DOMINUS](https://github.com/FlipperPA/django-tempus-dominus)
6. [DJANGO-REST-FRAMEWORK](https://www.django-rest-framework.org/)
---
## License
---
[MIT](https://choosealicense.com/licenses/mit/)
Raw data
{
"_id": null,
"home_page": "https://github.com/m3h-D/Form-Generator/tree/main",
"name": "django-form-generator",
"maintainer": "Mahdi Asadnejad",
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": "",
"keywords": "django form generator form_generator django_form_generator",
"author": "Mahdi Asadnejad",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/8c/5e/fd5e72fd0817f48169bb973c1678fb347899b98f4831aaa68e238638d09f/django_form_generator-0.0.55.tar.gz",
"platform": null,
"description": "\n# Django Form Generator\n\ndjango form generator will help you create forms very easily.\nyou can use it any where in your html files.\n\nthere is an API-manager tool for you to call some APIs on loading form or after submit.\n\n---\n## Installation\n---\n- install it via pip:\n \n ```bash\n pip install django-form-generator\n ```\n\n- add (`'django_form_generator'`, `'django_htmx'`, `'crispy_forms'`, `'crispy_bootstrap5'`, `'captcha'`, `'tempus_dominus', 'rest_framework', 'drf_recaptcha'`) to your `INSTALLED_APPS`:\n ```python\n INSTALLED_APPS = [\n ...\n 'django_htmx',\n 'crispy_forms',\n 'crispy_bootstrap5',\n 'captcha',\n 'tempus_dominus',\n 'rest_framework',\n 'drf_recaptcha',\n 'django_form_generator',\n ]\n ```\n\n- do a `migrate`:\n \n ```bash\n python manage.py migrate\n ```\n\n- do a `collectstatic`:\n \n ```bash\n python manage.py collectstatic\n ```\n\n- finally include django_form_generator urls to your `urls.py`\n ```python\n\n from django.contrib import admin\n from django.urls import path, include\n\n urlpatterns = [\n path('admin/', admin.site.urls),\n ...\n path('form-generator/', include(('django_form_generator.urls', 'django_form_generator'), namespace='django_form_generator')),\n ...\n ]\n ```\n \n\n---\n## Requirements\n---\n* Python >= 3.10\n* Django >= 4.0\n\n\n---\n## Form Usage\n---\n\n- ### regular html usage:\n ```html\n <!doctype html>\n <html lang=\"en\">\n <head>\n ...\n </head>\n\n <body>\n {% load django_form_generator %}\n\n {% render_form 1 %} {# it will render the form that has id of 1 #}\n\n </body>\n\n </html>\n ```\n\n\n- ### RichText fields:\n ***To use this tags on a Richtext field like `CKeditor` or `tinymce`.***\n\n >Note: In some richtext packages some elements (specialy jinja elements) will be removed from the field\n there are some tweaks to solve this problems on the packages documentations.\n\n - #### Admin panel:\n *CK-Editor example*\n 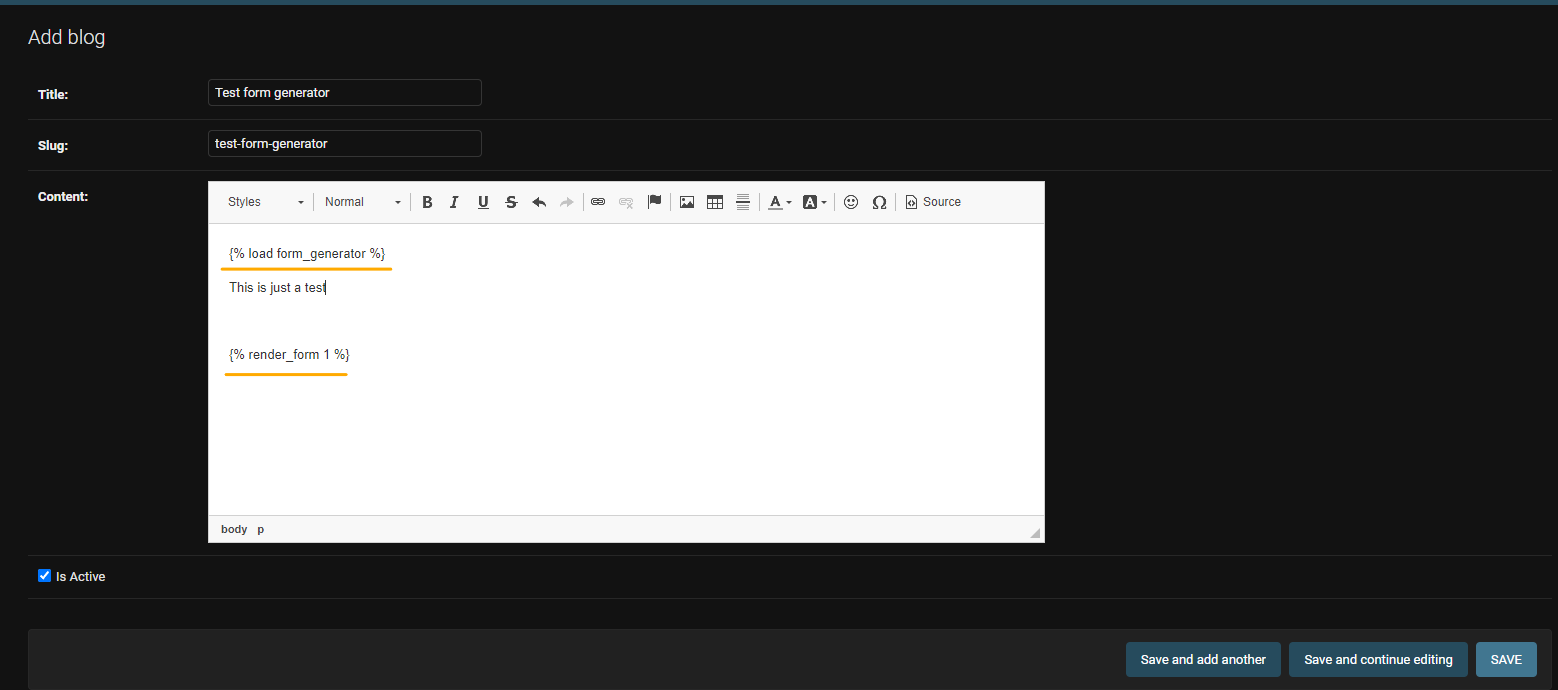\n\n\n - ```./app_name/templates/index.html ```\n\n ```html\n <!doctype html>\n <html lang=\"en\">\n <head>\n ...\n </head>\n\n <body>\n {% load django_form_generator %}\n\n {{ object.rich_text_field|eval_data }}\n\n </body>\n\n </html>\n ```\n\n---\n## API manager Usage\n---\n**There is also an API manager for the forms**\n\n- ### Admin panel:\n\n - **example:**\n >Note: `first_name`, `last_name` and `job` are the name of the fields that are defined in a form which this api is assigned to.\n\n 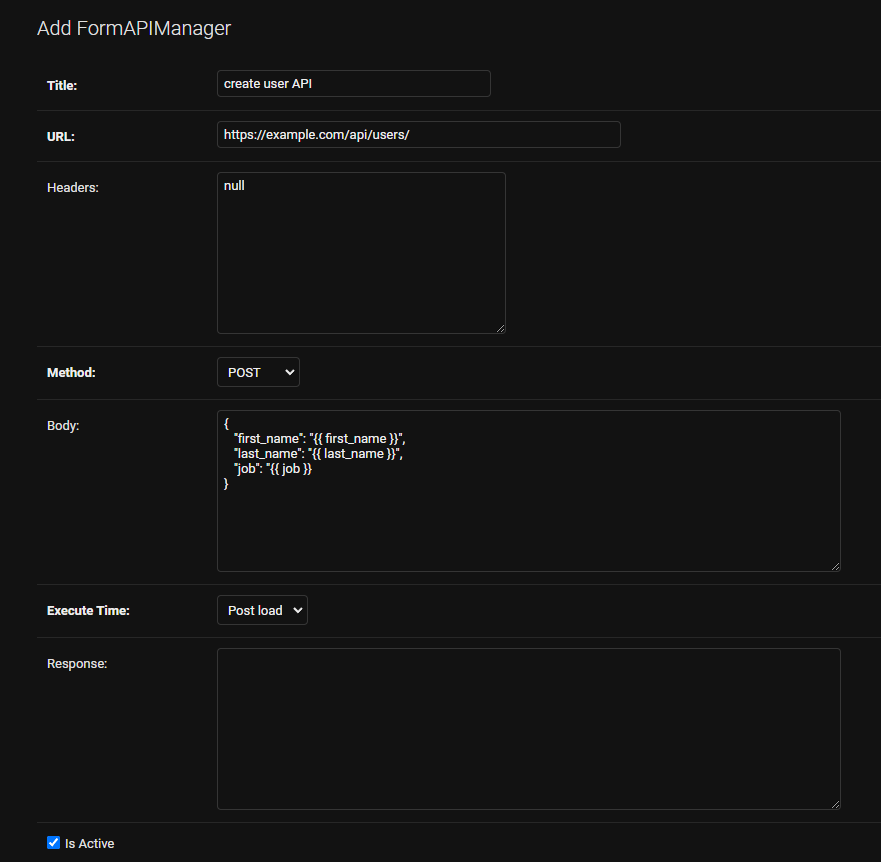\n\n\n---\n\n>***Note: assigend apis will be called automatically.\nthe ones with execution_time of `PRE` will be called when form renders.\nand the ones with execution_time of `POST` will be called after form submition.***\n\n\n>***Note: in the response field you can write HTML tags \n>it will be rendrend as `safe`***.\n\n>***Note: You have access to `request` globaly in api manager***\n\n***Also you have access to the response of the api. so you can use `Jinja` syntax to access data.\nYou can use this feature on the (`url`, `body`, `response`) fields of `APIManager` model***\n\n- ### Creating API\n - **example:**\n \n \n 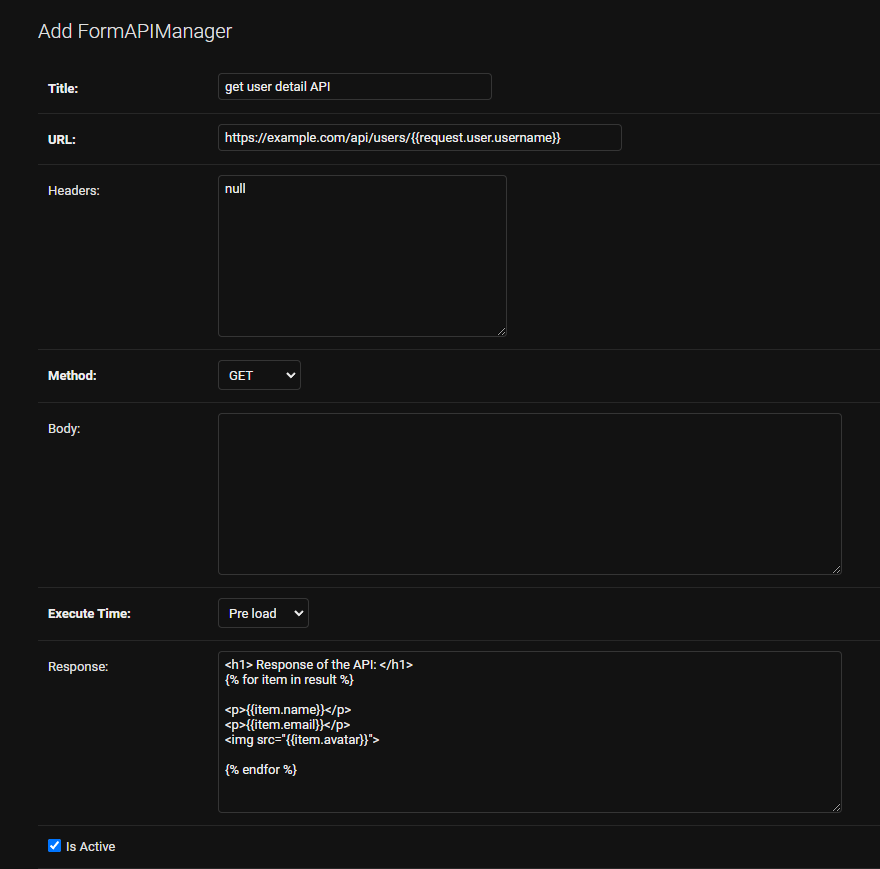\n \n >Note: To show the response use the template tag of `{% render_pre_api form_id api_id %}` inside your html file that the `form` is there.\n\n\n- ### Response\n example:\n - output of the api:\n ```json\n {\n \"result\": [\n {\n \"first_name\": \"john\",\n \"last_name\": \"doe\",\n \"avatar\": \"https://www.example.com/img/john-doe.png\",\n },\n {\n \"first_name\": \"mary\",\n \"last_name\": \"doe\",\n \"avatar\": \"https://www.example.com/img/mary-doe.png\",\n }\n ]\n }\n ```\n\n - in the response field you can do:\n ```html\n <h1> Response of the API: </h1>\n {% for item in result %}\n\n <p>{{item.first_name}}</p>\n <p>{{item.last_name}}</p>\n <img src=\"{{item.avatar}}\">\n\n {% endfor %}\n\n ```\n\n- ### html usage:\n ```html\n <!doctype html>\n <html lang=\"en\">\n <head>\n ...\n </head>\n\n <body>\n {% load django_form_generator %}\n\n {% render_pre_api 1 %} {# it will render all pre apis that are assigned to a form with id of 1 #}\n\n {% render_pre_api 1 6 %} {# it will render the pre api with id of 6 which is assigned to a form with id of 1 #}\n\n\n {% render_post_api 1 %} {# it will render all pre apis that are assigned to a form with id of 1 #}\n\n {% render_post_api 1 7 %} {# it will render the post api with id of 7 which is assigned to a form with id of 1 #}\n\n </body>\n\n </html>\n ```\n\n\n---\n## Extra\n---\n- ### API Manager:\n \n you can access the whole submited data by adding `{{ form_data }}` to your api `body`.\n\n\n- ### Form Style:\n to have multiple styles the html of `template_name_p` (attribute of django.forms.Form) will be replace by our html file. (`{{form.as_p}}`)\n\n there are 3 styles(or actualy styel of rendering fields) for the forms:\n 1. In-line Style[^1].\n\n 2. In-order Style[^2].\n\n 3. Dynamic Style[^3].\n \n [^1]: In every line two fields will be rendered.\n\n [^2]: In every line one field will be rendered.\n\n [^3]: this style will render fields respect to the field position (`inline`, `inorder`, `break`)\n \n\n >Note: If you want to render two fields `inline` and one field `inorder` you should use `break` for the second field \n\n - example:\n - field1 inline\n - field2 break\n - field3 inorder \n\n \n >Note: You can add your custom styles by create new TextChoices object and add it to `settings.py`\n \n - example:\n 1. *myapp/const.py*\n ```python\n from django.db.models import TextChoices\n\n class CustomFormGeneratorStyle(TextChoices):\n MY_STYLE = \"myapp/templates/my_style.html\", 'My Custom Style'\n ...\n\n ```\n 2. *myproject/settings.py*\n ```python\n DJANGO_FORM_GENERATOR = {\n \"FORM_STYLE_CHOICES\": \"myapp.const.CustomFormGeneratorStyle\"\n }\n ```\n\n \n\n- ### URLS:\n ```python\n # to access the form you can call this url:\n \"\"\"GET: http://127.0.0.1:8000/form-generator/form/1/\"\"\"\n #or \n reverse('django_form_generator:form_detail', kwargs={'pk': 1})\n #api:\n reverse('django_form_generator:api_form_detail', kwargs={'pk': 1})\n\n\n # to access the response of the form you can call this url the kwarg_lookup is UUID field\n \"\"\"GET: http://127.0.0.1:8000/form-generator/form-response/xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx/\"\"\"\n #or\n reverse('django_form_generator:form_response', kwargs={'unique_id': 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'})\n #api:\n reverse('django_form_generator:api_form_response', kwargs={'unique_id': 'xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx'})\n\n ```\n\n- ### Settings:\n **below is the default settings for django_form_generator. you can change them by adding it to your `settings.py`**\n ```python\n DJANGO_FORM_GENERATOR = {\n 'FORM_RESPONSE_SAVE': 'django_form_generator.models.save_form_response',\n 'FORM_EVALUATIONS': {'form_data': '{{form_data}}'},\n 'FORM_GENERATOR_FORM': 'django_form_generator.forms.FormGeneratorForm',\n 'FORM_RESPONSE_FORM': 'django_form_generator.forms.FormGeneratorResponseForm',\n 'FORM_STYLE_CHOICES': 'django_form_generator.const.FormStyle',\n 'FORM_MANAGER': 'django_form_generator.managers.FormManager',\n 'FORM_GENERATOR_SERIALIZER': 'django_form_generator.api.serializers.FormGeneratorSerializer',\n 'FORM_RESPONSE_SERIALIZER': 'django_form_generator.api.serializers.FormGeneratorResponseSerializer',\n }\n ```\n\n---\n## Attention\n---\n**To have nice styled fields you can add crispy-form to your `settings.py`**\n\n ```python\n CRISPY_ALLOWED_TEMPLATE_PACKS = 'bootstrap5'\n CRISPY_TEMPLATE_PACK = 'bootstrap5'\n ```\n\n**To access google recaptcha you should add these to your `settings.py`**\n\n>Note: you can have public & private keys by registring your domain on [Google recaptcha admin console](https://www.google.com/recaptcha/admin/create)\n\n ```python\n RECAPTCHA_PUBLIC_KEY = 'public_key'\n RECAPTCHA_PRIVATE_KEY = 'private_key'\n # ===== FOR DRF =========\n DRF_RECAPTCHA_SECRET_KEY = \"YOUR SECRET KEY\"\n ```\n\n\n>Note: If you don't want to use google-recaptcha(`django-recaptcah`)\nyou should add below code to your `settings.py`\n\n ```python\n SILENCED_SYSTEM_CHECKS = ['captcha.recaptcha_test_key_error']\n ```\n\n---\n## Dependency Packages\n---\n1. [HTMX](https://htmx.org/docs/)\n2. [CRISPY-FORMS (crispy-bootstrap5)](https://github.com/django-crispy-forms/crispy-bootstrap5)\n3. [REQUESTS](https://requests.readthedocs.io/en/latest/)\n4. [DJANGO-RECAPTCHA](https://pypi.org/project/django-recaptcha/)\n5. [DJANGO-TEMPUS-DOMINUS](https://github.com/FlipperPA/django-tempus-dominus)\n6. [DJANGO-REST-FRAMEWORK](https://www.django-rest-framework.org/)\n\n---\n## License\n---\n[MIT](https://choosealicense.com/licenses/mit/)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "An app to create any form in django admin panel.",
"version": "0.0.55",
"project_urls": {
"Homepage": "https://github.com/m3h-D/Form-Generator/tree/main"
},
"split_keywords": [
"django",
"form",
"generator",
"form_generator",
"django_form_generator"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "7b126742774b964ef2d69551e1b54baf783d064def368658a50921c67a52fe7b",
"md5": "7aba67d7b1b90dc3596687bb9e6efbd0",
"sha256": "7ae8960e0551eb3fb03c06574289f00c9570ce74230a5b2d3bff9f1484361245"
},
"downloads": -1,
"filename": "django_form_generator-0.0.55-py3-none-any.whl",
"has_sig": false,
"md5_digest": "7aba67d7b1b90dc3596687bb9e6efbd0",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 145665,
"upload_time": "2023-05-23T17:47:09",
"upload_time_iso_8601": "2023-05-23T17:47:09.917854Z",
"url": "https://files.pythonhosted.org/packages/7b/12/6742774b964ef2d69551e1b54baf783d064def368658a50921c67a52fe7b/django_form_generator-0.0.55-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "8c5efd5e72fd0817f48169bb973c1678fb347899b98f4831aaa68e238638d09f",
"md5": "00092c243da1cf931ca0b8d05a38597a",
"sha256": "83cb19ac8c91d432d29c19e34377744cfc872df45d1e0ac55766c61a5a9cfc19"
},
"downloads": -1,
"filename": "django_form_generator-0.0.55.tar.gz",
"has_sig": false,
"md5_digest": "00092c243da1cf931ca0b8d05a38597a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 137268,
"upload_time": "2023-05-23T17:47:14",
"upload_time_iso_8601": "2023-05-23T17:47:14.179791Z",
"url": "https://files.pythonhosted.org/packages/8c/5e/fd5e72fd0817f48169bb973c1678fb347899b98f4831aaa68e238638d09f/django_form_generator-0.0.55.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-23 17:47:14",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "m3h-D",
"github_project": "Form-Generator",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "django-form-generator"
}