[](https://pypi.python.org/pypi/django-jalali-date)
[](https://pypistats.org/packages/django-jalali-date)
[](https://github.com/a-roomana/django-jalali-date/actions)
[](https://github.com/a-roomana/django-jalali-date)
[](https://pypi.python.org/pypi/django-jalali-date)
# django-jalali-date
Jalali Date support for user interface. Easy conversion of DateTimeField to JalaliDateTimeField within the admin site, view and templates.
## Dependency
To use this module you need to install jdatetime(and of course you need django) and pytz module which you can install it with easy_install or pip
## Version Compatibility
#### Python
- python 3.8 and below is compatible with 0.3.2 and below
- python 3.X and above is compatible with 1.0.0 and above
#### Django
I tested the latest version on some Django versions on python 3.8
- django == 4.2
- django == 3.2.8
- django == 2.2.24
I think it will work properly on other versions as well.
If you plan to use it in Django 1.X, install version 0.3.2
## Install
pip install django-jalali-date
## Usage
#### settings.py
- don't forget to make sure you've also added `jalali_date` to your `INSTALLED_APPS`.
- any global settings for a Django Jalali Date are kept in a single configuration dictionary named `JALALI_DATE_DEFAULTS`
- you can change the default display of dates by override `Strftime`
- you can use your own date picker by override `Static`
```python
INSTALLED_APPS = [
'django_apps',
'jalali_date',
'my_apps',
]
# default settings (optional)
JALALI_DATE_DEFAULTS = {
# if change it to true then all dates of the list_display will convert to the Jalali.
'LIST_DISPLAY_AUTO_CONVERT': False,
'Strftime': {
'date': '%y/%m/%d',
'datetime': '%H:%M:%S _ %y/%m/%d',
},
'Static': {
'js': [
# loading datepicker
'admin/js/django_jalali.min.js',
# OR
# 'admin/jquery.ui.datepicker.jalali/scripts/jquery.ui.core.js',
# 'admin/jquery.ui.datepicker.jalali/scripts/calendar.js',
# 'admin/jquery.ui.datepicker.jalali/scripts/jquery.ui.datepicker-cc.js',
# 'admin/jquery.ui.datepicker.jalali/scripts/jquery.ui.datepicker-cc-fa.js',
# 'admin/js/main.js',
],
'css': {
'all': [
'admin/jquery.ui.datepicker.jalali/themes/base/jquery-ui.min.css',
]
}
},
}
```
(Optional) If you want the names of the dates to be displayed in Farsi, please add the following command to the settings.
If you are on windows:
```python
LANGUAGE_CODE = 'fa'
import locale
locale.setlocale(locale.LC_ALL, "Persian_Iran.UTF-8")
```
If you are on other operating systems:
```python
LANGUAGE_CODE = 'fa'
import locale
locale.setlocale(locale.LC_ALL, "fa_IR.UTF-8")
```
#### views.py
```python
from jalali_date import datetime2jalali, date2jalali
def my_view(request):
jalali_join = datetime2jalali(request.user.date_joined).strftime('%y/%m/%d _ %H:%M:%S')
```
#### forms.py
```python
from django import forms
from jalali_date.fields import JalaliDateField, SplitJalaliDateTimeField
from jalali_date.widgets import AdminJalaliDateWidget, AdminSplitJalaliDateTime
class TestForm(forms.ModelForm):
class Meta:
model = TestModel
fields = ('name', 'date', 'date_time')
def __init__(self, *args, **kwargs):
super(TestForm, self).__init__(*args, **kwargs)
self.fields['date'] = JalaliDateField(label=_('date'), # date format is "yyyy-mm-dd"
widget=AdminJalaliDateWidget # optional, to use default datepicker
)
# you can added a "class" to this field for use your datepicker!
# self.fields['date'].widget.attrs.update({'class': 'jalali_date-date'})
self.fields['date_time'] = SplitJalaliDateTimeField(label=_('date time'),
widget=AdminSplitJalaliDateTime # required, for decompress DatetimeField to JalaliDateField and JalaliTimeField
)
```
#### template.html
```html
{% load jalali_tags %}
<p>{{ request.user.date_joined|to_jalali:'%y/%m/%d _ %H:%M:%S' }}</p>
<form method="post">{% csrf_token %}
{{ form.as_p }}
<input type="submit">
</form>
<!-- By default, Datepicker using jQuery, you need to set your script after loading jQuery! -->
<!-- loading directly -->
<link rel="stylesheet" href="{% static 'admin/jquery.ui.datepicker.jalali/themes/base/jquery-ui.min.css' %}">
<script src="{% static 'admin/js/django_jalali.min.js' %}"></script>
<!-- OR -->
<!-- loading by form (if used AdminJalaliDateWidget) -->
{{ form.media }}
```
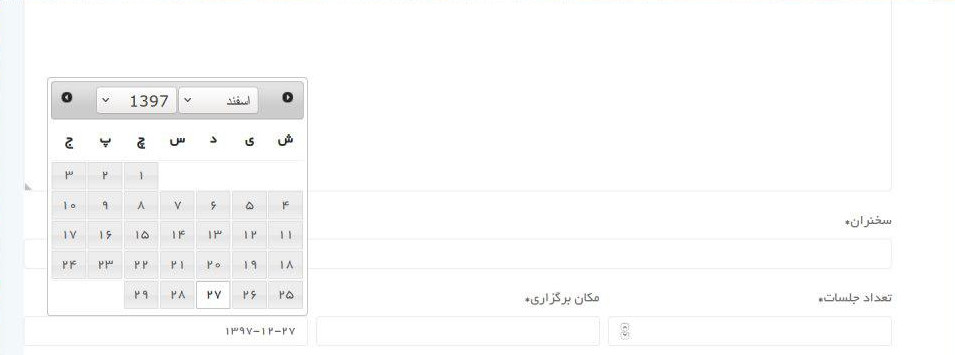
#### admin.py
```python
from django.contrib import admin
from jalali_date import datetime2jalali, date2jalali
from jalali_date.admin import ModelAdminJalaliMixin, StackedInlineJalaliMixin, TabularInlineJalaliMixin
class MyInlines1(TabularInlineJalaliMixin, admin.TabularInline):
model = SecendModel
class MyInlines2(StackedInlineJalaliMixin, admin.StackedInline):
model = ThirdModel
@admin.register(FirstModel)
class FirstModelAdmin(ModelAdminJalaliMixin, admin.ModelAdmin):
#for showing the Jalali date on the list_display, please change the LIST_DISPLAY_AUTO_CONVERT to true or create custom methods. for example:
list_display = ['some_fields', 'get_created_jalali']
inlines = (MyInlines1, MyInlines2, )
raw_id_fields = ('some_fields', )
readonly_fields = ('some_fields', 'date_field',)
# you can override formfield, for example:
formfield_overrides = {
JSONField: {'widget': JSONEditor},
}
@admin.display(description='تاریخ ایجاد', ordering='created')
def get_created_jalali(self, obj):
return datetime2jalali(obj.created).strftime('%a, %d %b %Y %H:%M:%S')
```

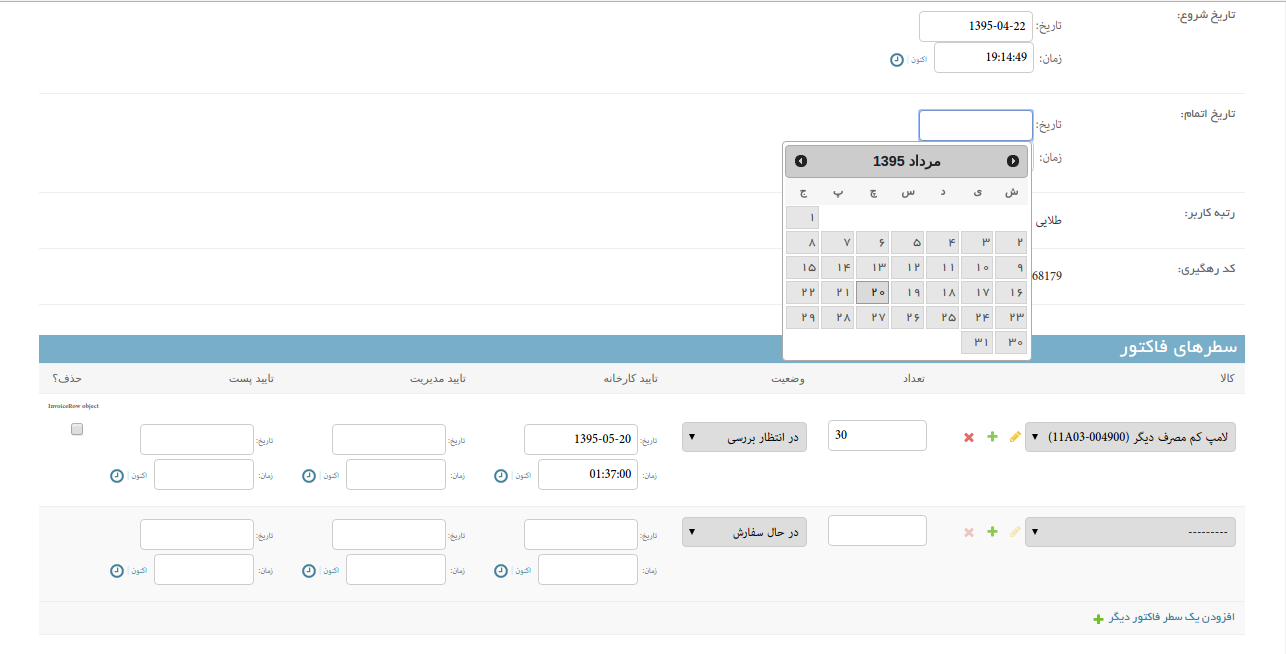
Raw data
{
"_id": null,
"home_page": "http://github.com/a-roomana/django-jalali-date",
"name": "django-jalali-date",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3",
"maintainer_email": null,
"keywords": "django jalali date",
"author": "Arman Roomana",
"author_email": "roomana.arman@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/f4/ca/e4393714751e9b1811fdd83e7886d801cbdd4cf8d948e5d091a3ede03e25/django_jalali_date-1.1.3.tar.gz",
"platform": "any",
"description": "\n\n[](https://pypi.python.org/pypi/django-jalali-date)\n[](https://pypistats.org/packages/django-jalali-date)\n[](https://github.com/a-roomana/django-jalali-date/actions)\n[](https://github.com/a-roomana/django-jalali-date)\n\n[](https://pypi.python.org/pypi/django-jalali-date)\n\n# django-jalali-date\n\nJalali Date support for user interface. Easy conversion of DateTimeField to JalaliDateTimeField within the admin site, view and templates.\n\n\n## Dependency\n\nTo use this module you need to install jdatetime(and of course you need django) and pytz module which you can install it with easy_install or pip\n\n\n## Version Compatibility\n#### Python\n- python 3.8 and below is compatible with 0.3.2 and below\n- python 3.X and above is compatible with 1.0.0 and above\n\n#### Django\nI tested the latest version on some Django versions on python 3.8\n- django == 4.2\n- django == 3.2.8\n- django == 2.2.24\n\nI think it will work properly on other versions as well.\n\nIf you plan to use it in Django 1.X, install version 0.3.2\n\n\n\n## Install\n\n pip install django-jalali-date \n\n\n## Usage\n\n#### settings.py\n\n- don't forget to make sure you've also added `jalali_date` to your `INSTALLED_APPS`.\n- any global settings for a Django Jalali Date are kept in a single configuration dictionary named `JALALI_DATE_DEFAULTS`\n - you can change the default display of dates by override `Strftime`\n - you can use your own date picker by override `Static` \n```python\nINSTALLED_APPS = [\n\t'django_apps',\n\t\n\t'jalali_date',\n\t\n\t'my_apps',\n]\n\n# default settings (optional)\nJALALI_DATE_DEFAULTS = {\n # if change it to true then all dates of the list_display will convert to the Jalali.\n 'LIST_DISPLAY_AUTO_CONVERT': False,\n 'Strftime': {\n 'date': '%y/%m/%d',\n 'datetime': '%H:%M:%S _ %y/%m/%d',\n },\n 'Static': {\n 'js': [\n # loading datepicker\n 'admin/js/django_jalali.min.js',\n # OR\n # 'admin/jquery.ui.datepicker.jalali/scripts/jquery.ui.core.js',\n # 'admin/jquery.ui.datepicker.jalali/scripts/calendar.js',\n # 'admin/jquery.ui.datepicker.jalali/scripts/jquery.ui.datepicker-cc.js',\n # 'admin/jquery.ui.datepicker.jalali/scripts/jquery.ui.datepicker-cc-fa.js',\n # 'admin/js/main.js',\n ],\n 'css': {\n 'all': [\n 'admin/jquery.ui.datepicker.jalali/themes/base/jquery-ui.min.css',\n ]\n }\n },\n}\n```\n\n(Optional) If you want the names of the dates to be displayed in Farsi, please add the following command to the settings.\n\nIf you are on windows:\n```python\nLANGUAGE_CODE = 'fa'\n\nimport locale\nlocale.setlocale(locale.LC_ALL, \"Persian_Iran.UTF-8\")\n```\nIf you are on other operating systems:\n```python\nLANGUAGE_CODE = 'fa'\n\nimport locale\nlocale.setlocale(locale.LC_ALL, \"fa_IR.UTF-8\")\n```\n\n\n#### views.py\n```python\nfrom jalali_date import datetime2jalali, date2jalali\n\ndef my_view(request):\n\tjalali_join = datetime2jalali(request.user.date_joined).strftime('%y/%m/%d _ %H:%M:%S')\n```\n#### forms.py\n```python\nfrom django import forms\nfrom jalali_date.fields import JalaliDateField, SplitJalaliDateTimeField\nfrom jalali_date.widgets import AdminJalaliDateWidget, AdminSplitJalaliDateTime\n\n\nclass TestForm(forms.ModelForm):\n class Meta:\n model = TestModel\n fields = ('name', 'date', 'date_time')\n\n def __init__(self, *args, **kwargs):\n super(TestForm, self).__init__(*args, **kwargs)\n self.fields['date'] = JalaliDateField(label=_('date'), # date format is \"yyyy-mm-dd\"\n widget=AdminJalaliDateWidget # optional, to use default datepicker\n )\n\n # you can added a \"class\" to this field for use your datepicker!\n # self.fields['date'].widget.attrs.update({'class': 'jalali_date-date'})\n\n self.fields['date_time'] = SplitJalaliDateTimeField(label=_('date time'), \n widget=AdminSplitJalaliDateTime # required, for decompress DatetimeField to JalaliDateField and JalaliTimeField\n )\n```\n\n#### template.html\n```html \n{% load jalali_tags %}\n\n<p>{{ request.user.date_joined|to_jalali:'%y/%m/%d _ %H:%M:%S' }}</p>\n\n<form method=\"post\">{% csrf_token %}\n {{ form.as_p }}\n <input type=\"submit\">\n</form>\n\n<!-- By default, Datepicker using jQuery, you need to set your script after loading jQuery! -->\n\t<!-- loading directly -->\n\t\t<link rel=\"stylesheet\" href=\"{% static 'admin/jquery.ui.datepicker.jalali/themes/base/jquery-ui.min.css' %}\">\n\t\t<script src=\"{% static 'admin/js/django_jalali.min.js' %}\"></script>\n\t<!-- OR -->\n\t<!-- loading by form (if used AdminJalaliDateWidget) -->\n\t\t{{ form.media }}\n```\n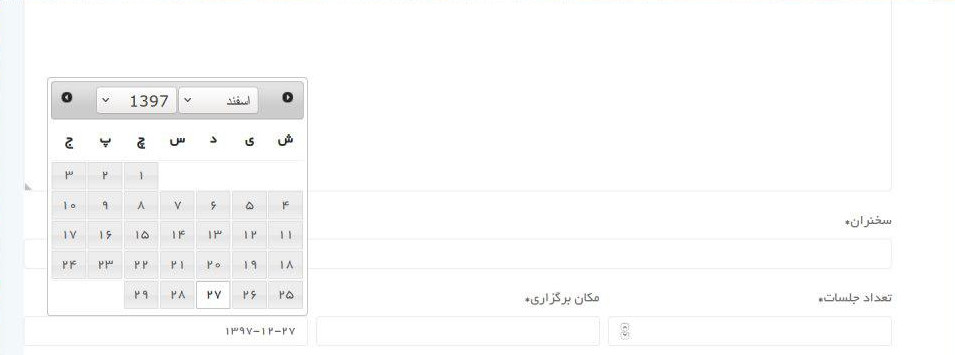\n\n#### admin.py\n```python\nfrom django.contrib import admin\nfrom jalali_date import datetime2jalali, date2jalali\nfrom jalali_date.admin import ModelAdminJalaliMixin, StackedInlineJalaliMixin, TabularInlineJalaliMixin\t\n \nclass MyInlines1(TabularInlineJalaliMixin, admin.TabularInline):\n\tmodel = SecendModel\n\nclass MyInlines2(StackedInlineJalaliMixin, admin.StackedInline):\n\tmodel = ThirdModel\n\t\n@admin.register(FirstModel)\nclass FirstModelAdmin(ModelAdminJalaliMixin, admin.ModelAdmin):\n\t#for showing the Jalali date on the list_display, please change the LIST_DISPLAY_AUTO_CONVERT to true or create custom methods. for example:\n list_display = ['some_fields', 'get_created_jalali']\n\t\n\tinlines = (MyInlines1, MyInlines2, )\n\traw_id_fields = ('some_fields', )\n\treadonly_fields = ('some_fields', 'date_field',)\n\t# you can override formfield, for example:\n\tformfield_overrides = {\n\t JSONField: {'widget': JSONEditor},\n\t}\n\t\n\t@admin.display(description='\u062a\u0627\u0631\u06cc\u062e \u0627\u06cc\u062c\u0627\u062f', ordering='created')\n\tdef get_created_jalali(self, obj):\n\t\treturn datetime2jalali(obj.created).strftime('%a, %d %b %Y %H:%M:%S')\n```\n\n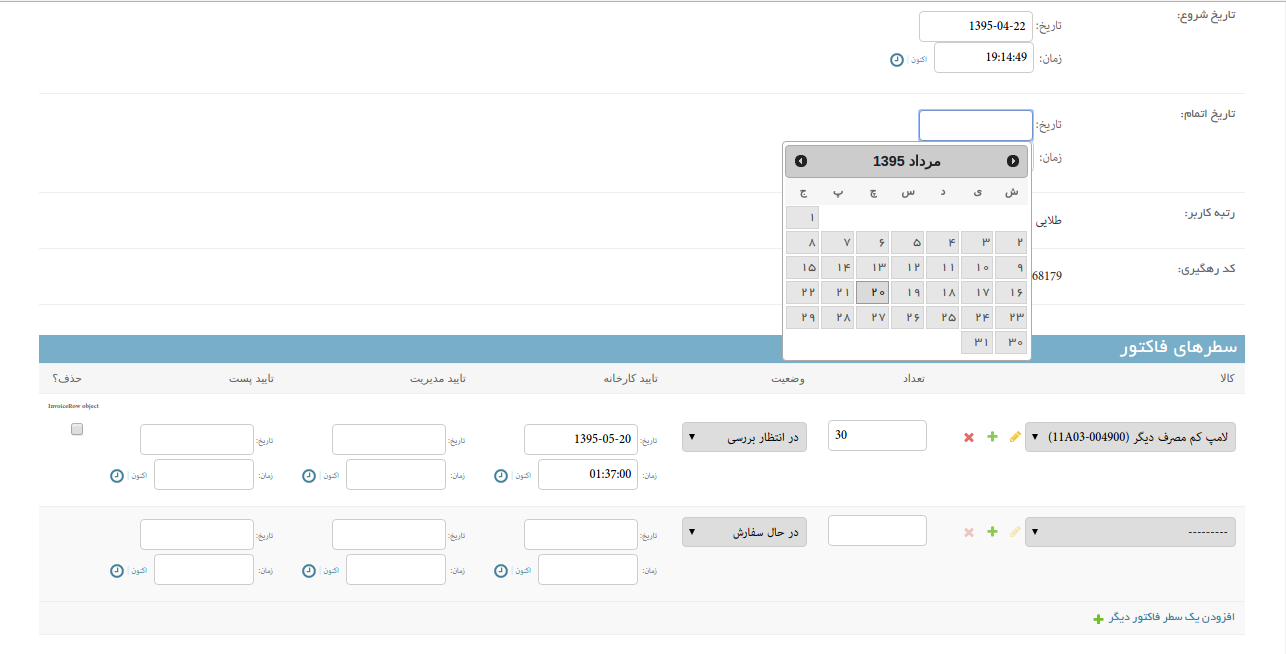\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Jalali Date support for user interface. Easy conversion of DateTimeFiled to JalaliDateTimeField within the admin site, views, forms and templates.",
"version": "1.1.3",
"project_urls": {
"Download": "https://pypi.python.org/pypi/django-jalali-date/",
"Homepage": "http://github.com/a-roomana/django-jalali-date"
},
"split_keywords": [
"django",
"jalali",
"date"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6d59359b205ee4a694b6654ea9af0f9e795b8454f649be8d0087c35d10b05443",
"md5": "9369cd3c9ba45f5b8476cf9f9361217d",
"sha256": "9610c0f24c6ce49b5f0d47863c7be1b7bfa12935664ca89a420690fd641a1f3a"
},
"downloads": -1,
"filename": "django_jalali_date-1.1.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "9369cd3c9ba45f5b8476cf9f9361217d",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3",
"size": 296807,
"upload_time": "2024-06-14T19:13:56",
"upload_time_iso_8601": "2024-06-14T19:13:56.513518Z",
"url": "https://files.pythonhosted.org/packages/6d/59/359b205ee4a694b6654ea9af0f9e795b8454f649be8d0087c35d10b05443/django_jalali_date-1.1.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "f4cae4393714751e9b1811fdd83e7886d801cbdd4cf8d948e5d091a3ede03e25",
"md5": "57d9dcdf47d5dd553d11e51c62627425",
"sha256": "7b0639e3594f34bc4458f5d371e3c072fda06bccacc5f68c751df3ce3d111ed3"
},
"downloads": -1,
"filename": "django_jalali_date-1.1.3.tar.gz",
"has_sig": false,
"md5_digest": "57d9dcdf47d5dd553d11e51c62627425",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3",
"size": 281019,
"upload_time": "2024-06-14T19:13:59",
"upload_time_iso_8601": "2024-06-14T19:13:59.893511Z",
"url": "https://files.pythonhosted.org/packages/f4/ca/e4393714751e9b1811fdd83e7886d801cbdd4cf8d948e5d091a3ede03e25/django_jalali_date-1.1.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-14 19:13:59",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "a-roomana",
"github_project": "django-jalali-date",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "django-jalali-date"
}