# django-pint-field
[](https://djangopackages.org/packages/p/django-pint-field/)
[](https://pypi.org/project/django-pint-field/)
Store, validate, and convert physical quantities in Django using [Pint](https://pint.readthedocs.io/en/stable/).
## Why Django Pint Field?
Django Pint Field enables you to:
- Store quantities (like 1 gram, 3 miles, 8.120391 angstroms) in your Django models
- Edit quantities in forms with automatic unit conversion
- Compare quantities in different units (e.g., compare weights in pounds vs. kilograms)
- Display quantities in user-preferred units while maintaining accurate comparisons
- Perform aggregations and lookups across different units of measurement
The package uses a Postgres composite field to store both the magnitude and units, along with a base unit value for accurate comparisons. This approach ensures that users can work with their preferred units while maintaining data integrity and comparability. For this reason, the project only works with Postgresql databases.
## Requirements
- Python 3.10+
- Django 4.2+
- PostgreSQL database
- Pint 0.23+
## Installation
```bash
pip install django-pint-field
```
Add to your `INSTALLED_APPS`:
```python
INSTALLED_APPS = [
# ...
"django_pint_field",
# ...
]
```
Run migrations:
```bash
python manage.py migrate django_pint_field
```
```{caution}
Failure to run django-pint-field migrations before running migrations for models using PintFields will result in errors. The migration creates a required composite type in your PostgreSQL database.
Previous versions of the package added three composite types to the database. The newest migration modifies the columns with these types to use a single composite type.
```
### Tips for Upgrading from Legacy django-pint-field
```{warning}
If using [django-pgtrigger](https://django-pgtrigger.readthedocs.io/en/latest/commands/) or other packages that depend on it (e.g.: django-pghistory), we highly recommend that you temporarily uninstall all triggers before running the django-pint-field migrations. It is also a good practice to make a backup of your database before running the migration. Users freshly installing `django-pint-field` do not need to worry about this warning.
```
```bash
python manage.py pgtrigger uninstall
```
Then run the migrations:
```bash
python manage.py migrate django_pint_field
```
Reinstall the triggers after the migrations are complete:
```bash
python manage.py pgtrigger install
```
## Quick Start
1. Define your model:
```python
from decimal import Decimal
from django.db import models
from django_pint_field.models import DecimalPintField
class Product(models.Model):
name = models.CharField(max_length=Decimal("100"))
weight = DecimalPintField(
default_unit="gram",
unit_choices=["gram", "kilogram", "pound", "ounce"],
)
```
2. Use it in your code:
```python
from django_pint_field.units import ureg
# Create objects
product = Product.objects.create(
name="Coffee Bag",
weight=ureg.Quantity(Decimal("340"), "gram"),
)
# Query using different units
products = Product.objects.filter(
weight__gte=ureg.Quantity(Decimal("0.5"), "kilogram"),
)
# Access values
print(product.weight) # 3460 gram
print(product.weight.quantity) # 3460 gram (accessing the Pint Quantity object)
# Convert to different units
print(product.weight.quantity.to("kilogram")) # 0.346 kilogram
print(product.weight.kilogram) # 0.346 kilogram
print(product.weight.kilogram__2) # 0.35 kilogram (rounded to 2 decimal places)
```
## Features
### Field Types
- **IntegerPintField**: For whole number quantities
- **DecimalPintField**: For precise decimal quantities
- **BigIntegerPintField**: For large whole number quantities (deprecated, use IntegerPintField instead)
### Form Fields and Widgets
- Built-in form fields with unit conversion
- TabledPintFieldWidget for displaying unit conversion tables
- Customizable validation and unit choices
#### Form Fields
- **IntegerPintFormField**: Used in forms with IntegerPintField and BigIntegerPintField.
- **DecimalPintFormField**: Used in forms with DecimalPintField.
#### Widgets
- **PintFieldWidget**: Default widget for all django pint field types.
- **TabledPintFieldWidget**: Provides a table showing conversion to each of the `unit_choices`.
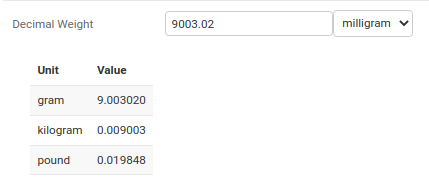
### Django REST Framework Integration
```python
from django_pint_field.rest import DecimalPintRestField
class ProductSerializer(serializers.ModelSerializer):
weight = DecimalPintRestField()
class Meta:
model = Product
fields = ["name", "weight"]
```
```{note}
The package is tested to work with both Django REST Framework and Django Ninja.
```
### Supported Lookups
- `exact`
- `gt`, `gte`
- `lt`, `lte`
- `range`
- `isnull`
### Aggregation Support
```python
from django_pint_field.aggregates import PintAvg, PintSum
Product.objects.aggregate(
avg_weight=PintAvg("weight"),
total_weight=PintSum("weight"),
)
```
#### Supported Aggregates
- `PintAvg`
- `PintCount`
- `PintMax`
- `PintMin`
- `PintSum`
- `PintStdDev`
- `PintVariance`
## Advanced Usage
### Custom Units
Create your own unit registry:
```python
from pint import UnitRegistry
custom_ureg = UnitRegistry(non_int_type=Decimal)
custom_ureg.define("custom_unit = [custom]")
# In settings.py
DJANGO_PINT_FIELD_UNIT_REGISTER = custom_ureg
```
### Indexing
Django Pint Field supports creating indexes on the comparator components of Pint fields. Indexes can improve query performance when filtering, ordering, or joining on Pint field values.
#### Single Field Index
```python
from django_pint_field.indexes import PintFieldComparatorIndex
class Package(models.Model):
weight = DecimalPintField("gram")
class Meta:
indexes = [PintFieldComparatorIndex(fields=["weight"])]
```
#### Multi-Field Index
```python
from django_pint_field.indexes import PintFieldComparatorIndex
class Package(models.Model):
weight = DecimalPintField("gram")
volume = DecimalPintField("liter")
class Meta:
indexes = [PintFieldComparatorIndex(fields=["weight", "volume"])]
```
You can also use additional index options, as usual. e.g.:
- `name`: Custom index name
- `condition`: Partial index condition
- `include`: Additional columns to include in the index
- `db_tablespace`: Custom tablespace for the index
### Settings
```python
# settings.py
# Set decimal precision for the entire project
DJANGO_PINT_FIELD_DECIMAL_PRECISION = 40
# Configure custom unit registry
DJANGO_PINT_FIELD_UNIT_REGISTER = custom_ureg
# Set default format for quantity display
DJANGO_PINT_FIELD_DEFAULT_FORMAT = "D" # Options: D, P, ~P, etc.
```
## Credits
Modified from [django-pint](https://github.com/CarliJoy/django-pint) with a focus on composite field storage and enhanced comparison capabilities.
Raw data
{
"_id": null,
"home_page": null,
"name": "django-pint-field",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.10",
"maintainer_email": null,
"keywords": null,
"author": null,
"author_email": "Jack Linke <jacklinke@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/e4/cf/a458b01df94f1c18687f18960805e3258f5b92660051651bff5ea5f8334e/django_pint_field-2025.1.1.tar.gz",
"platform": null,
"description": "# django-pint-field\n\n[](https://djangopackages.org/packages/p/django-pint-field/)\n[](https://pypi.org/project/django-pint-field/)\n\nStore, validate, and convert physical quantities in Django using [Pint](https://pint.readthedocs.io/en/stable/).\n\n## Why Django Pint Field?\n\nDjango Pint Field enables you to:\n\n- Store quantities (like 1 gram, 3 miles, 8.120391 angstroms) in your Django models\n- Edit quantities in forms with automatic unit conversion\n- Compare quantities in different units (e.g., compare weights in pounds vs. kilograms)\n- Display quantities in user-preferred units while maintaining accurate comparisons\n- Perform aggregations and lookups across different units of measurement\n\nThe package uses a Postgres composite field to store both the magnitude and units, along with a base unit value for accurate comparisons. This approach ensures that users can work with their preferred units while maintaining data integrity and comparability. For this reason, the project only works with Postgresql databases.\n\n## Requirements\n\n- Python 3.10+\n- Django 4.2+\n- PostgreSQL database\n- Pint 0.23+\n\n## Installation\n\n```bash\npip install django-pint-field\n```\n\nAdd to your `INSTALLED_APPS`:\n\n```python\nINSTALLED_APPS = [\n # ...\n \"django_pint_field\",\n # ...\n]\n```\n\nRun migrations:\n\n```bash\npython manage.py migrate django_pint_field\n```\n\n```{caution}\nFailure to run django-pint-field migrations before running migrations for models using PintFields will result in errors. The migration creates a required composite type in your PostgreSQL database.\n\nPrevious versions of the package added three composite types to the database. The newest migration modifies the columns with these types to use a single composite type.\n```\n\n### Tips for Upgrading from Legacy django-pint-field\n\n```{warning}\nIf using [django-pgtrigger](https://django-pgtrigger.readthedocs.io/en/latest/commands/) or other packages that depend on it (e.g.: django-pghistory), we highly recommend that you temporarily uninstall all triggers before running the django-pint-field migrations. It is also a good practice to make a backup of your database before running the migration. Users freshly installing `django-pint-field` do not need to worry about this warning.\n```\n\n```bash\npython manage.py pgtrigger uninstall\n```\n\nThen run the migrations:\n\n```bash\npython manage.py migrate django_pint_field\n```\n\nReinstall the triggers after the migrations are complete:\n\n```bash\npython manage.py pgtrigger install\n```\n\n## Quick Start\n\n1. Define your model:\n\n```python\nfrom decimal import Decimal\nfrom django.db import models\nfrom django_pint_field.models import DecimalPintField\n\n\nclass Product(models.Model):\n name = models.CharField(max_length=Decimal(\"100\"))\n weight = DecimalPintField(\n default_unit=\"gram\",\n unit_choices=[\"gram\", \"kilogram\", \"pound\", \"ounce\"],\n )\n```\n\n2. Use it in your code:\n\n```python\nfrom django_pint_field.units import ureg\n\n# Create objects\nproduct = Product.objects.create(\n name=\"Coffee Bag\",\n weight=ureg.Quantity(Decimal(\"340\"), \"gram\"),\n)\n\n# Query using different units\nproducts = Product.objects.filter(\n weight__gte=ureg.Quantity(Decimal(\"0.5\"), \"kilogram\"),\n)\n\n# Access values\nprint(product.weight) # 3460 gram\nprint(product.weight.quantity) # 3460 gram (accessing the Pint Quantity object)\n# Convert to different units\nprint(product.weight.quantity.to(\"kilogram\")) # 0.346 kilogram\nprint(product.weight.kilogram) # 0.346 kilogram\nprint(product.weight.kilogram__2) # 0.35 kilogram (rounded to 2 decimal places)\n```\n\n## Features\n\n### Field Types\n\n- **IntegerPintField**: For whole number quantities\n- **DecimalPintField**: For precise decimal quantities\n- **BigIntegerPintField**: For large whole number quantities (deprecated, use IntegerPintField instead)\n\n### Form Fields and Widgets\n\n- Built-in form fields with unit conversion\n- TabledPintFieldWidget for displaying unit conversion tables\n- Customizable validation and unit choices\n\n#### Form Fields\n\n- **IntegerPintFormField**: Used in forms with IntegerPintField and BigIntegerPintField.\n- **DecimalPintFormField**: Used in forms with DecimalPintField.\n\n#### Widgets\n\n- **PintFieldWidget**: Default widget for all django pint field types.\n- **TabledPintFieldWidget**: Provides a table showing conversion to each of the `unit_choices`.\n\n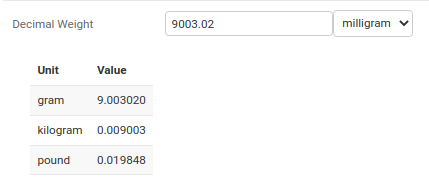\n\n### Django REST Framework Integration\n\n```python\nfrom django_pint_field.rest import DecimalPintRestField\n\n\nclass ProductSerializer(serializers.ModelSerializer):\n weight = DecimalPintRestField()\n\n class Meta:\n model = Product\n fields = [\"name\", \"weight\"]\n```\n\n```{note}\nThe package is tested to work with both Django REST Framework and Django Ninja.\n```\n\n### Supported Lookups\n\n- `exact`\n- `gt`, `gte`\n- `lt`, `lte`\n- `range`\n- `isnull`\n\n### Aggregation Support\n\n```python\nfrom django_pint_field.aggregates import PintAvg, PintSum\n\nProduct.objects.aggregate(\n avg_weight=PintAvg(\"weight\"),\n total_weight=PintSum(\"weight\"),\n)\n```\n\n#### Supported Aggregates\n\n- `PintAvg`\n- `PintCount`\n- `PintMax`\n- `PintMin`\n- `PintSum`\n- `PintStdDev`\n- `PintVariance`\n\n## Advanced Usage\n\n### Custom Units\n\nCreate your own unit registry:\n\n```python\nfrom pint import UnitRegistry\n\ncustom_ureg = UnitRegistry(non_int_type=Decimal)\ncustom_ureg.define(\"custom_unit = [custom]\")\n\n# In settings.py\nDJANGO_PINT_FIELD_UNIT_REGISTER = custom_ureg\n```\n\n### Indexing\n\nDjango Pint Field supports creating indexes on the comparator components of Pint fields. Indexes can improve query performance when filtering, ordering, or joining on Pint field values.\n\n#### Single Field Index\n\n```python\nfrom django_pint_field.indexes import PintFieldComparatorIndex\n\n\nclass Package(models.Model):\n weight = DecimalPintField(\"gram\")\n\n class Meta:\n indexes = [PintFieldComparatorIndex(fields=[\"weight\"])]\n```\n\n#### Multi-Field Index\n\n```python\nfrom django_pint_field.indexes import PintFieldComparatorIndex\n\n\nclass Package(models.Model):\n weight = DecimalPintField(\"gram\")\n volume = DecimalPintField(\"liter\")\n\n class Meta:\n indexes = [PintFieldComparatorIndex(fields=[\"weight\", \"volume\"])]\n```\n\nYou can also use additional index options, as usual. e.g.:\n\n- `name`: Custom index name\n- `condition`: Partial index condition\n- `include`: Additional columns to include in the index\n- `db_tablespace`: Custom tablespace for the index\n\n### Settings\n\n```python\n# settings.py\n\n# Set decimal precision for the entire project\nDJANGO_PINT_FIELD_DECIMAL_PRECISION = 40\n\n# Configure custom unit registry\nDJANGO_PINT_FIELD_UNIT_REGISTER = custom_ureg\n\n# Set default format for quantity display\nDJANGO_PINT_FIELD_DEFAULT_FORMAT = \"D\" # Options: D, P, ~P, etc.\n```\n\n## Credits\n\nModified from [django-pint](https://github.com/CarliJoy/django-pint) with a focus on composite field storage and enhanced comparison capabilities.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Unit Conversion fields for Django and Postgres",
"version": "2025.1.1",
"project_urls": {
"Changelog": "https://github.com/OmenApps/django-pint-field/releases",
"Documentation": "https://django-pint-field.readthedocs.io/en/latest/",
"Homepage": "https://github.com/OmenApps/django-pint-field",
"Repository": "https://github.com/OmenApps/django-pint-field"
},
"split_keywords": [],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "c6d19f60455d41c9374c6ee594dff0c3c1673ff184a4fe80c688c660e10a51c2",
"md5": "00cd61cdf748b9814be012a1b0687d3a",
"sha256": "fc95f939571d17e86772ae4802f0de7c3c3a7f4227cca12da71a555dadb4606c"
},
"downloads": -1,
"filename": "django_pint_field-2025.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "00cd61cdf748b9814be012a1b0687d3a",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.10",
"size": 40563,
"upload_time": "2025-01-17T06:56:14",
"upload_time_iso_8601": "2025-01-17T06:56:14.084956Z",
"url": "https://files.pythonhosted.org/packages/c6/d1/9f60455d41c9374c6ee594dff0c3c1673ff184a4fe80c688c660e10a51c2/django_pint_field-2025.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "e4cfa458b01df94f1c18687f18960805e3258f5b92660051651bff5ea5f8334e",
"md5": "c4066854bff9ee34542aedcceddec589",
"sha256": "4fbfd130ffc0c7ab246ea166eebbe930e0c1a6c3c8d9cd0470e6f73a3a1f30d5"
},
"downloads": -1,
"filename": "django_pint_field-2025.1.1.tar.gz",
"has_sig": false,
"md5_digest": "c4066854bff9ee34542aedcceddec589",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.10",
"size": 36786,
"upload_time": "2025-01-17T06:56:16",
"upload_time_iso_8601": "2025-01-17T06:56:16.194677Z",
"url": "https://files.pythonhosted.org/packages/e4/cf/a458b01df94f1c18687f18960805e3258f5b92660051651bff5ea5f8334e/django_pint_field-2025.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-01-17 06:56:16",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "OmenApps",
"github_project": "django-pint-field",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "appdirs",
"specs": [
[
"==",
"1.4.4"
]
]
},
{
"name": "asgiref",
"specs": [
[
"==",
"3.8.1"
]
]
},
{
"name": "django",
"specs": [
[
"==",
"5.1.3"
]
]
},
{
"name": "flexcache",
"specs": [
[
"==",
"0.3"
]
]
},
{
"name": "flexparser",
"specs": [
[
"==",
"0.3.1"
]
]
},
{
"name": "pint",
"specs": [
[
"==",
"0.24.3"
]
]
},
{
"name": "sqlparse",
"specs": [
[
"==",
"0.5.1"
]
]
},
{
"name": "typing-extensions",
"specs": [
[
"==",
"4.12.2"
]
]
}
],
"lcname": "django-pint-field"
}