# Django Simple 3rd Party JWT
[](https://github.com/NatLee/Django-Simple-3rd-Party-JWT/actions/workflows/test.yml)
[](https://github.com/NatLee/Django-Simple-3rd-Party-JWT/actions/workflows/release.yml)
<img width="1363" alt="image" src="https://user-images.githubusercontent.com/10178964/222040924-8cb37622-b1ac-4343-bb2f-96e48aabfa87.png">
This is a simple tool for 3rd party login with JWT.
> See Simple Version in another repo -> [JWT 3rd Party Dashboard](https://github.com/NatLee/Django-Simple-3rd-Party-JWT-Dev-Dashboard).
## Installation
```bash
pip install django-simple-third-party-jwt
```
Check it in [Pypi](https://pypi.org/project/django-simple-third-party-jwt/).
## Quick Start
### Backend
1. Add `django_simple_third_party_jwt` to your `INSTALLED_APPS` in `settings.py` like this:
```py
INSTALLED_APPS = [
...
'django_simple_third_party_jwt',
]
```
2. Add APP settings to your `settings.py` like this:
```py
from datetime import timedelta
# -------------- START - CORS Setting --------------
CORS_ALLOW_ALL_ORIGINS = True
CORS_ALLOW_CREDENTIALS = True
CSRF_TRUSTED_ORIGINS = [
"http://*.127.0.0.1",
"http://localhost",
]
# -------------- END - CORS Setting -----------------
# -------------- Start - SimpleJWT Setting --------------
SIMPLE_JWT = {
"ACCESS_TOKEN_LIFETIME": timedelta(minutes=3600),
"REFRESH_TOKEN_LIFETIME": timedelta(days=1),
"ROTATE_REFRESH_TOKENS": False,
"BLACKLIST_AFTER_ROTATION": False,
"UPDATE_LAST_LOGIN": False,
"ALGORITHM": "HS256",
"SIGNING_KEY": SECRET_KEY,
"VERIFYING_KEY": None,
"AUDIENCE": None,
"ISSUER": None,
"JWK_URL": None,
"LEEWAY": 0,
"AUTH_HEADER_TYPES": ("Bearer",),
"AUTH_HEADER_NAME": "HTTP_AUTHORIZATION",
"USER_ID_FIELD": "id",
"USER_ID_CLAIM": "user_id",
"USER_AUTHENTICATION_RULE": "rest_framework_simplejwt.authentication.default_user_authentication_rule",
"AUTH_TOKEN_CLASSES": ("rest_framework_simplejwt.tokens.AccessToken",),
"TOKEN_TYPE_CLAIM": "token_type",
"TOKEN_USER_CLASS": "rest_framework_simplejwt.models.TokenUser",
"JTI_CLAIM": "jti",
"SLIDING_TOKEN_REFRESH_EXP_CLAIM": "refresh_exp",
"SLIDING_TOKEN_LIFETIME": timedelta(minutes=5),
"SLIDING_TOKEN_REFRESH_LIFETIME": timedelta(days=1),
}
# -------------- END - SimpleJWT Setting --------------
# -------------- START - Auth Setting --------------
SECURE_REFERRER_POLICY = "no-referrer-when-downgrade"
# SECURE_CROSS_ORIGIN_OPENER_POLICY = "same-origin-allow-popups"
SECURE_CROSS_ORIGIN_OPENER_POLICY = None
LOGIN_REDIRECT_URL = "/"
VALID_REGISTER_DOMAINS = ["gmail.com", "hotmail.com"] # Only these domains can login.
# API URL Prefix
JWT_3RD_PREFIX = 'api'
# ================== Google Auth ==================
# Add this block if you want to login with Google.
SOCIAL_GOOGLE_CLIENT_ID = "376808175534-d6mefo6b1kqih3grjjose2euree2g3cs.apps.googleusercontent.com"
# ================== END - Google Auth ==================
# ================== Microsoft Auth ==================
# Add this block if you want to login with Microsoft.
# ID
SOCIAL_MICROSOFT_CLIENT_ID = '32346173-22bc-43b2-b6ed-f88f6a76e38c'
# Secret
SOCIAL_MICROSOFT_CLIENT_SECRET = 'K5z8Q~dIXDiFN5qjMjRjIx34cZOJ3Glkrg.dxcG9'
# ================== END - Microsoft Auth ==================
# --------------- END - Auth Setting -----------------
```
> You can regist `SOCIAL_GOOGLE_CLIENT_ID` on Google Cloud Platform.
[Google Colud | API和服務 | 憑證](https://console.cloud.google.com/apis/credentials)
1. Create a new project and create a new OAuth 2.0 Client ID.

2. Add `http://localhost:8000` to `Authorized JavaScript origins` and `Authorized redirect URIs`.

> You can regist `SOCIAL_MICROSOFT_CLIENT_ID` on Microsoft Azure.
[Microsoft Entra 識別碼 | 應用程式註冊](https://portal.azure.com/#view/Microsoft_AAD_IAM/ActiveDirectoryMenuBlade/~/RegisteredApps)
1. Create a new application.

2. Add `http://localhost:8000/api/auth/microsoft/callback` to `Redirect URIs`

3. Get `Client ID` from `Overview` page.

4. Get `Client Secret` from `Certificates & secrets` page.

3. Include the `django_simple_third_party_jwt` URL settings in your project `urls.py` like this:
```py
from django.conf import settings
from django.urls import include
urlpatterns += [
path("api/", include("django_simple_third_party_jwt.urls")),
]
```
You also need to include JWT settings in your `urls.py`.
```py
# --------------- JWT
from rest_framework_simplejwt.views import (
TokenVerifyView, TokenObtainPairView, TokenRefreshView
)
urlpatterns += [
path("api/auth/token", TokenObtainPairView.as_view(), name="token_get"),
path("api/auth/token/refresh", TokenRefreshView.as_view(), name="token_refresh"),
path("api/auth/token/verify", TokenVerifyView.as_view(), name="token_verify"),
]
# ---------------------------------
```
4. Migrate and test on your server.
- Migrate
```bash
python manage.py migrate django_simple_third_party_jwt
```
- Test
```bash
python manage.py runserver
```
### Frontend (Optional)
Here just a demo frontend settings.
#### Google Login
You need to check `{{ social_google_client_id }}` is the same with `Metadata` and your `Html` page.
- Meta
```html
<meta name="google-signin-scope" content="profile email" />
<meta name="google-signin-client_id" content="{{ social_google_client_id }}" />
<script src="https://accounts.google.com/gsi/client" async defer></script>
```
- Html
```html
<li>
<div id="g_id_onload"
data-client_id="{{ social_google_client_id }}"
data-callback="get_jwt_using_google_credential" </div>
<div class="g_id_signin" data-type="standard" data-size="large" data-theme="outline"
data-text="sign_in_with" data-shape="rectangular" data-logo_alignment="left">
</div>
</li>
```
- Javascript
You can try this script to get credential token from Google and verify it with calling our custom 3rd party API.
```html
<script>
function get_jwt_using_google_credential(data) {
const google_token_url = "/api/auth/google/token";
// const google_token_url = "/api/auth/google/token/session"; <------ if you also need login as session, choose this one.
const credential = data.credential;
$.ajax({
method: "POST",
url: google_token_url,
data: { credential: credential },
}).done(function (data) {
const access_token = data.access;
const refresh_token = data.refresh_token;
localStorage.setItem("access", access_token);
localStorage.setItem("refresh", refresh_token);
console.log("Google Login");
$.ajax({
type: "POST",
url: "/api/auth/token/verify",
data: { token: access_token },
headers: {
Authorization: "Bearer" + " " + access_token,
},
success: function (data) {
var json_string = JSON.stringify(data, null, 2);
if (json_string) {
console.log("Token verified successfully!");
}
},
error: function (data) {
var result = "please login " + data.responseText;
console.log(result);
},
});
});
}
</script>
```
#### Microsoft Login
Set `LOGIN_REDIRECT_URL` in `settings.py` and add the following code in your `Html` page.
- Html
```html
<button id="microsoft-login-button" class="btn w-100" onclick="location.href='/api/auth/microsoft/signin';">
<img src="https://upload.wikimedia.org/wikipedia/commons/thumb/4/44/Microsoft_logo.svg/2048px-Microsoft_logo.svg.png" alt="Microsoft logo" style="width: 30px; height: 30px;">
Login with Microsoft
</button>
```
## Example
Check [Example of dashboard](https://github.com/NatLee/Django-Simple-3rd-Party-JWT-Dev-Dashboard).
Or you can use the following steps.
### Run example backend
You can see the example in `./example/`
```bash
git clone https://github.com/NatLee/Django-Simple-3rd-Party-JWT
cd Django-Simple-3rd-Party-JWT/example/django_simple_third_party_jwt_example/
pip install -r requirements.txt
python manage.py makemigrations && python manage.py migrate
python manage.py runserver 0.0.0.0:8000
```
If you need superuser, run:
```bash
python manage.py createsuperuser
```
### Visit example frontend
Open browser and visit `localhost:8000`.
There are several url routes available in this example.
```
api/auth/google/ <---- Google Login
api/auth/google/session <------ Google Login with Django Session
api/ auth/microsoft/signin <----- Microsoft Login with Django Session
api/ auth/microsoft/signout <----- Microsoft Logout
api/ auth/microsoft/callback <----- Microsoft Login Callback
api/__hidden_admin/
api/__hidden_dev_dashboard/
api/auth/token [name='token_get']
api/auth/token/refresh [name='token_refresh']
api/auth/token/verify [name='token_verify']
^api/__hidden_swagger(?P<format>\.json|\.yaml)$ [name='schema-json']
^api/__hidden_swagger/$ [name='schema-swagger-ui']
^api/__hidden_redoc/$ [name='schema-redoc']
```
- Dev Dashboard
In the first, visit testing dashboard`http://localhost:8000/api/__hidden_dev_dashboard/`.

And, you can find Google Login in the top right corner like below.
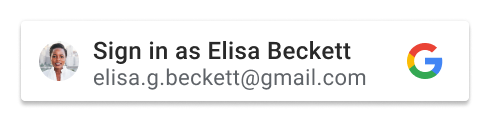
Click it.
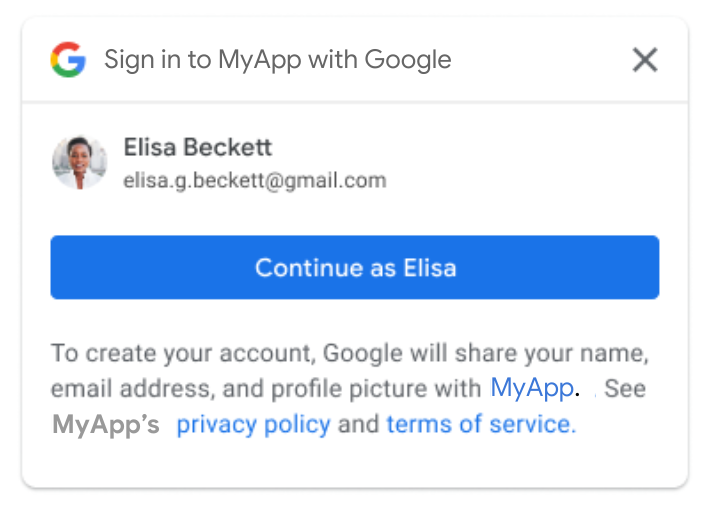
When you login, you will see the following hint.

If you want to filter domains with Google Login, feel free to check `VALID_REGISTER_DOMAINS` in `settings.py`.
Once you login with Google, your account ID will be recorded in the database.
> See more login information in `social_account` table in database.
| id | provider | unique_id | user_id |
| :-: | :------: | :----------------: | :-----: |
| 1 | google | 100056159912345678 | 1 |
- Swagger
Also can see all information of APIs in `http://localhost:8000/api/__hidden_swagger/`.

## More
Check https://developers.google.com/identity/gsi/web/guides/overview with more information of Google Login API.
## Misc tools
### Install & re-install package
* Linux
```bash
bash dev-reinstall.sh
```
* Windows
```powershell
./dev-reinstall.ps1
```
Raw data
{
"_id": null,
"home_page": "https://github.com/NatLee/Django-Simple-3rd-Party-JWT",
"name": "django-simple-third-party-jwt",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "django, jwt, 3rd party login, microsoft login, google login",
"author": "Nat Lee",
"author_email": "natlee.work@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/be/39/513f08beb863893bf16ca4cdba092c24c77e6333783f49aa335f1546c529/django_simple_third_party_jwt-0.3.1.tar.gz",
"platform": null,
"description": "# Django Simple 3rd Party JWT\n\n[](https://github.com/NatLee/Django-Simple-3rd-Party-JWT/actions/workflows/test.yml)\n[](https://github.com/NatLee/Django-Simple-3rd-Party-JWT/actions/workflows/release.yml)\n\n\n<img width=\"1363\" alt=\"image\" src=\"https://user-images.githubusercontent.com/10178964/222040924-8cb37622-b1ac-4343-bb2f-96e48aabfa87.png\">\n\n\nThis is a simple tool for 3rd party login with JWT.\n\n> See Simple Version in another repo -> [JWT 3rd Party Dashboard](https://github.com/NatLee/Django-Simple-3rd-Party-JWT-Dev-Dashboard).\n\n## Installation\n\n```bash\npip install django-simple-third-party-jwt\n```\n\nCheck it in [Pypi](https://pypi.org/project/django-simple-third-party-jwt/).\n\n## Quick Start\n\n### Backend\n\n1. Add `django_simple_third_party_jwt` to your `INSTALLED_APPS` in `settings.py` like this:\n\n```py\nINSTALLED_APPS = [\n...\n'django_simple_third_party_jwt',\n]\n```\n\n2. Add APP settings to your `settings.py` like this:\n\n```py\n\nfrom datetime import timedelta\n\n# -------------- START - CORS Setting --------------\nCORS_ALLOW_ALL_ORIGINS = True\nCORS_ALLOW_CREDENTIALS = True\nCSRF_TRUSTED_ORIGINS = [\n \"http://*.127.0.0.1\",\n \"http://localhost\",\n]\n# -------------- END - CORS Setting -----------------\n\n# -------------- Start - SimpleJWT Setting --------------\nSIMPLE_JWT = {\n \"ACCESS_TOKEN_LIFETIME\": timedelta(minutes=3600),\n \"REFRESH_TOKEN_LIFETIME\": timedelta(days=1),\n \"ROTATE_REFRESH_TOKENS\": False,\n \"BLACKLIST_AFTER_ROTATION\": False,\n \"UPDATE_LAST_LOGIN\": False,\n \"ALGORITHM\": \"HS256\",\n \"SIGNING_KEY\": SECRET_KEY,\n \"VERIFYING_KEY\": None,\n \"AUDIENCE\": None,\n \"ISSUER\": None,\n \"JWK_URL\": None,\n \"LEEWAY\": 0,\n \"AUTH_HEADER_TYPES\": (\"Bearer\",),\n \"AUTH_HEADER_NAME\": \"HTTP_AUTHORIZATION\",\n \"USER_ID_FIELD\": \"id\",\n \"USER_ID_CLAIM\": \"user_id\",\n \"USER_AUTHENTICATION_RULE\": \"rest_framework_simplejwt.authentication.default_user_authentication_rule\",\n \"AUTH_TOKEN_CLASSES\": (\"rest_framework_simplejwt.tokens.AccessToken\",),\n \"TOKEN_TYPE_CLAIM\": \"token_type\",\n \"TOKEN_USER_CLASS\": \"rest_framework_simplejwt.models.TokenUser\",\n \"JTI_CLAIM\": \"jti\",\n \"SLIDING_TOKEN_REFRESH_EXP_CLAIM\": \"refresh_exp\",\n \"SLIDING_TOKEN_LIFETIME\": timedelta(minutes=5),\n \"SLIDING_TOKEN_REFRESH_LIFETIME\": timedelta(days=1),\n}\n# -------------- END - SimpleJWT Setting --------------\n\n# -------------- START - Auth Setting --------------\n\nSECURE_REFERRER_POLICY = \"no-referrer-when-downgrade\"\n# SECURE_CROSS_ORIGIN_OPENER_POLICY = \"same-origin-allow-popups\"\nSECURE_CROSS_ORIGIN_OPENER_POLICY = None\n\nLOGIN_REDIRECT_URL = \"/\"\nVALID_REGISTER_DOMAINS = [\"gmail.com\", \"hotmail.com\"] # Only these domains can login.\n\n# API URL Prefix\nJWT_3RD_PREFIX = 'api'\n\n# ================== Google Auth ==================\n# Add this block if you want to login with Google.\n\nSOCIAL_GOOGLE_CLIENT_ID = \"376808175534-d6mefo6b1kqih3grjjose2euree2g3cs.apps.googleusercontent.com\"\n\n# ================== END - Google Auth ==================\n\n# ================== Microsoft Auth ==================\n# Add this block if you want to login with Microsoft.\n\n# ID\nSOCIAL_MICROSOFT_CLIENT_ID = '32346173-22bc-43b2-b6ed-f88f6a76e38c'\n# Secret\nSOCIAL_MICROSOFT_CLIENT_SECRET = 'K5z8Q~dIXDiFN5qjMjRjIx34cZOJ3Glkrg.dxcG9'\n\n# ================== END - Microsoft Auth ==================\n\n\n# --------------- END - Auth Setting -----------------\n```\n\n> You can regist `SOCIAL_GOOGLE_CLIENT_ID` on Google Cloud Platform.\n\n [Google Colud | API\u548c\u670d\u52d9 | \u6191\u8b49](https://console.cloud.google.com/apis/credentials)\n\n 1. Create a new project and create a new OAuth 2.0 Client ID.\n \n\n 2. Add `http://localhost:8000` to `Authorized JavaScript origins` and `Authorized redirect URIs`.\n \n\n> You can regist `SOCIAL_MICROSOFT_CLIENT_ID` on Microsoft Azure.\n\n[Microsoft Entra \u8b58\u5225\u78bc | \u61c9\u7528\u7a0b\u5f0f\u8a3b\u518a](https://portal.azure.com/#view/Microsoft_AAD_IAM/ActiveDirectoryMenuBlade/~/RegisteredApps)\n\n 1. Create a new application.\n \n 2. Add `http://localhost:8000/api/auth/microsoft/callback` to `Redirect URIs`\n \n 3. Get `Client ID` from `Overview` page.\n \n 4. Get `Client Secret` from `Certificates & secrets` page.\n \n\n3. Include the `django_simple_third_party_jwt` URL settings in your project `urls.py` like this:\n\n```py\nfrom django.conf import settings\nfrom django.urls import include\nurlpatterns += [\n path(\"api/\", include(\"django_simple_third_party_jwt.urls\")),\n]\n```\n\nYou also need to include JWT settings in your `urls.py`.\n\n```py\n# --------------- JWT\nfrom rest_framework_simplejwt.views import (\n TokenVerifyView, TokenObtainPairView, TokenRefreshView\n)\nurlpatterns += [\n path(\"api/auth/token\", TokenObtainPairView.as_view(), name=\"token_get\"),\n path(\"api/auth/token/refresh\", TokenRefreshView.as_view(), name=\"token_refresh\"),\n path(\"api/auth/token/verify\", TokenVerifyView.as_view(), name=\"token_verify\"),\n]\n# ---------------------------------\n```\n\n4. Migrate and test on your server.\n\n- Migrate\n\n```bash\npython manage.py migrate django_simple_third_party_jwt\n```\n\n- Test\n\n```bash\npython manage.py runserver\n```\n\n### Frontend (Optional)\n\nHere just a demo frontend settings.\n\n#### Google Login\n\nYou need to check `{{ social_google_client_id }}` is the same with `Metadata` and your `Html` page.\n\n- Meta\n\n```html\n<meta name=\"google-signin-scope\" content=\"profile email\" />\n<meta name=\"google-signin-client_id\" content=\"{{ social_google_client_id }}\" />\n<script src=\"https://accounts.google.com/gsi/client\" async defer></script>\n```\n\n- Html\n\n```html\n<li>\n <div id=\"g_id_onload\"\n data-client_id=\"{{ social_google_client_id }}\"\n data-callback=\"get_jwt_using_google_credential\" </div>\n <div class=\"g_id_signin\" data-type=\"standard\" data-size=\"large\" data-theme=\"outline\"\n data-text=\"sign_in_with\" data-shape=\"rectangular\" data-logo_alignment=\"left\">\n </div>\n</li>\n```\n\n- Javascript\n\nYou can try this script to get credential token from Google and verify it with calling our custom 3rd party API.\n\n```html\n<script>\n function get_jwt_using_google_credential(data) {\n const google_token_url = \"/api/auth/google/token\";\n // const google_token_url = \"/api/auth/google/token/session\"; <------ if you also need login as session, choose this one.\n const credential = data.credential;\n $.ajax({\n method: \"POST\",\n url: google_token_url,\n data: { credential: credential },\n }).done(function (data) {\n const access_token = data.access;\n const refresh_token = data.refresh_token;\n localStorage.setItem(\"access\", access_token);\n localStorage.setItem(\"refresh\", refresh_token);\n console.log(\"Google Login\");\n $.ajax({\n type: \"POST\",\n url: \"/api/auth/token/verify\",\n data: { token: access_token },\n headers: {\n Authorization: \"Bearer\" + \" \" + access_token,\n },\n success: function (data) {\n var json_string = JSON.stringify(data, null, 2);\n if (json_string) {\n console.log(\"Token verified successfully!\");\n }\n },\n error: function (data) {\n var result = \"please login \" + data.responseText;\n console.log(result);\n },\n });\n });\n }\n</script>\n```\n\n#### Microsoft Login\n\nSet `LOGIN_REDIRECT_URL` in `settings.py` and add the following code in your `Html` page.\n\n- Html\n\n```html\n<button id=\"microsoft-login-button\" class=\"btn w-100\" onclick=\"location.href='/api/auth/microsoft/signin';\">\n <img src=\"https://upload.wikimedia.org/wikipedia/commons/thumb/4/44/Microsoft_logo.svg/2048px-Microsoft_logo.svg.png\" alt=\"Microsoft logo\" style=\"width: 30px; height: 30px;\">\n Login with Microsoft\n</button>\n```\n\n\n## Example\n\nCheck [Example of dashboard](https://github.com/NatLee/Django-Simple-3rd-Party-JWT-Dev-Dashboard).\n\nOr you can use the following steps.\n\n### Run example backend\n\nYou can see the example in `./example/`\n\n```bash\ngit clone https://github.com/NatLee/Django-Simple-3rd-Party-JWT\ncd Django-Simple-3rd-Party-JWT/example/django_simple_third_party_jwt_example/\npip install -r requirements.txt\npython manage.py makemigrations && python manage.py migrate\npython manage.py runserver 0.0.0.0:8000\n```\n\nIf you need superuser, run:\n\n```bash\npython manage.py createsuperuser\n```\n\n### Visit example frontend\n\nOpen browser and visit `localhost:8000`.\n\nThere are several url routes available in this example.\n\n```\n\napi/auth/google/ <---- Google Login\napi/auth/google/session <------ Google Login with Django Session\n\napi/ auth/microsoft/signin <----- Microsoft Login with Django Session\napi/ auth/microsoft/signout <----- Microsoft Logout\napi/ auth/microsoft/callback <----- Microsoft Login Callback\n\napi/__hidden_admin/\napi/__hidden_dev_dashboard/\n\napi/auth/token [name='token_get']\napi/auth/token/refresh [name='token_refresh']\napi/auth/token/verify [name='token_verify']\n\n^api/__hidden_swagger(?P<format>\\.json|\\.yaml)$ [name='schema-json']\n^api/__hidden_swagger/$ [name='schema-swagger-ui']\n^api/__hidden_redoc/$ [name='schema-redoc']\n\n```\n\n- Dev Dashboard\n\nIn the first, visit testing dashboard`http://localhost:8000/api/__hidden_dev_dashboard/`.\n\n\n\nAnd, you can find Google Login in the top right corner like below.\n\n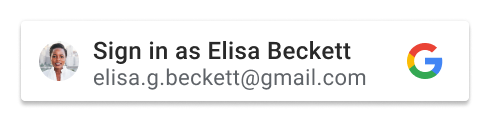\n\nClick it.\n\n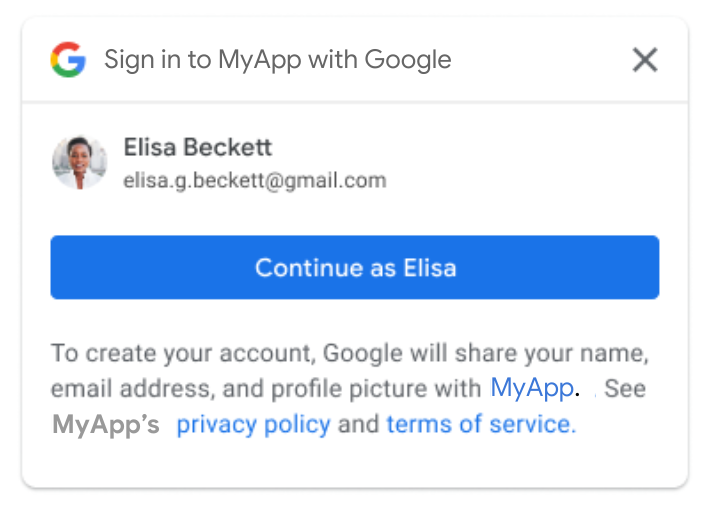\n\nWhen you login, you will see the following hint.\n\n\n\nIf you want to filter domains with Google Login, feel free to check `VALID_REGISTER_DOMAINS` in `settings.py`.\n\nOnce you login with Google, your account ID will be recorded in the database.\n\n> See more login information in `social_account` table in database.\n\n| id | provider | unique_id | user_id |\n| :-: | :------: | :----------------: | :-----: |\n| 1 | google | 100056159912345678 | 1 |\n\n- Swagger\n\nAlso can see all information of APIs in `http://localhost:8000/api/__hidden_swagger/`.\n\n\n\n## More\n\nCheck https://developers.google.com/identity/gsi/web/guides/overview with more information of Google Login API.\n\n## Misc tools\n\n### Install & re-install package\n\n* Linux\n\n```bash\nbash dev-reinstall.sh\n```\n\n* Windows\n\n```powershell\n./dev-reinstall.ps1\n```\n\n",
"bugtrack_url": null,
"license": null,
"summary": "Easy to use JWT with 3rd party login.",
"version": "0.3.1",
"project_urls": {
"Bug Reports": "https://github.com/natlee/Django-Simple-3rd-Party-JWT/issues",
"Documentation": "https://github.com/natlee/Django-Simple-3rd-Party-JWT",
"Homepage": "https://github.com/NatLee/Django-Simple-3rd-Party-JWT",
"Source Code": "https://github.com/natlee/Django-Simple-3rd-Party-JWT"
},
"split_keywords": [
"django",
" jwt",
" 3rd party login",
" microsoft login",
" google login"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "8a8bb912a726ace09332856c3b4fb82ea734b01a002a44cc7ccb7aa3ab27aa32",
"md5": "998ace7ae0c4fbaa65c3c90183b9b9b0",
"sha256": "ef04cdb18439b3ed3c80f7509698b93001e2ee5c9b49526ccea6f7b564a97215"
},
"downloads": -1,
"filename": "django_simple_third_party_jwt-0.3.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "998ace7ae0c4fbaa65c3c90183b9b9b0",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 22249,
"upload_time": "2024-08-06T17:20:27",
"upload_time_iso_8601": "2024-08-06T17:20:27.075106Z",
"url": "https://files.pythonhosted.org/packages/8a/8b/b912a726ace09332856c3b4fb82ea734b01a002a44cc7ccb7aa3ab27aa32/django_simple_third_party_jwt-0.3.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "be39513f08beb863893bf16ca4cdba092c24c77e6333783f49aa335f1546c529",
"md5": "671c87075e61ef2d081c1a6c00bd49bd",
"sha256": "9c48a10a7f8ad9d5ca56782d2d8673276947ac43da2bd36914752c549f1bae4a"
},
"downloads": -1,
"filename": "django_simple_third_party_jwt-0.3.1.tar.gz",
"has_sig": false,
"md5_digest": "671c87075e61ef2d081c1a6c00bd49bd",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 34049,
"upload_time": "2024-08-06T17:20:28",
"upload_time_iso_8601": "2024-08-06T17:20:28.567285Z",
"url": "https://files.pythonhosted.org/packages/be/39/513f08beb863893bf16ca4cdba092c24c77e6333783f49aa335f1546c529/django_simple_third_party_jwt-0.3.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-06 17:20:28",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "NatLee",
"github_project": "Django-Simple-3rd-Party-JWT",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"tox": true,
"lcname": "django-simple-third-party-jwt"
}