# Django TomSelect
A powerful, lightweight Django package for dynamic select inputs with autocomplete, tagging, and more.
[](https://badge.fury.io/py/django-tomselect.png)
[](https://github.com/OmenApps/django-tomselect/blob/main/LICENSE)
Django TomSelect integrates [Tom Select](https://tom-select.js.org/) into your Django projects, providing beautiful and intuitive select inputs with features like:
- **Live Search & Autocomplete**
- Real-time filtering and highlighting as you type
- Server-side search with customizable lookups
- Automatic pagination for large datasets
- Customizable minimum query length
- **Rich UI Options**
- Single and multiple selection modes
- Tabular display with custom columns
- Bootstrap 4/5 theming support
- Clear/remove buttons
- Dropdown headers & footers
- Checkbox options
- Customizable templates
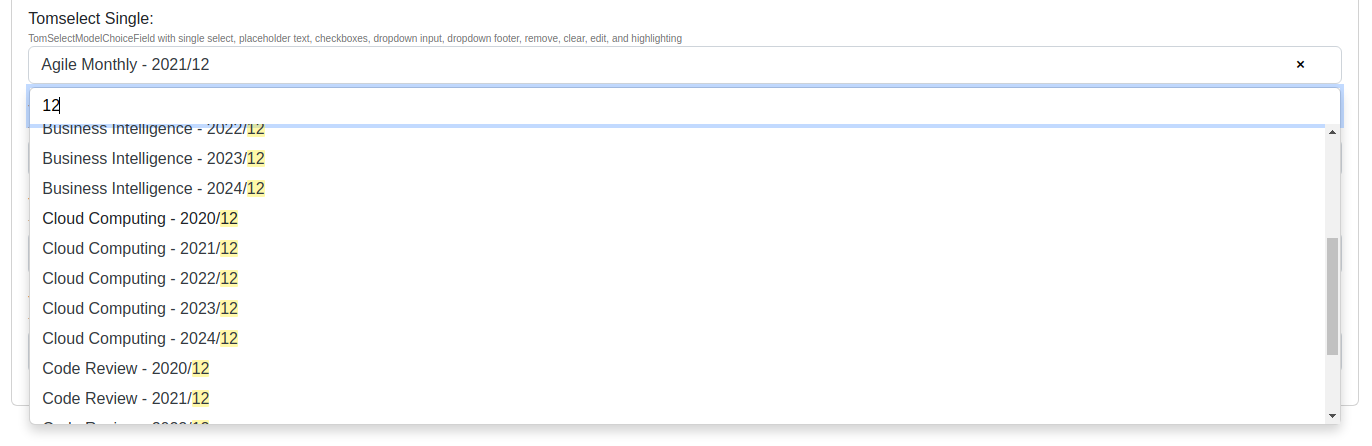
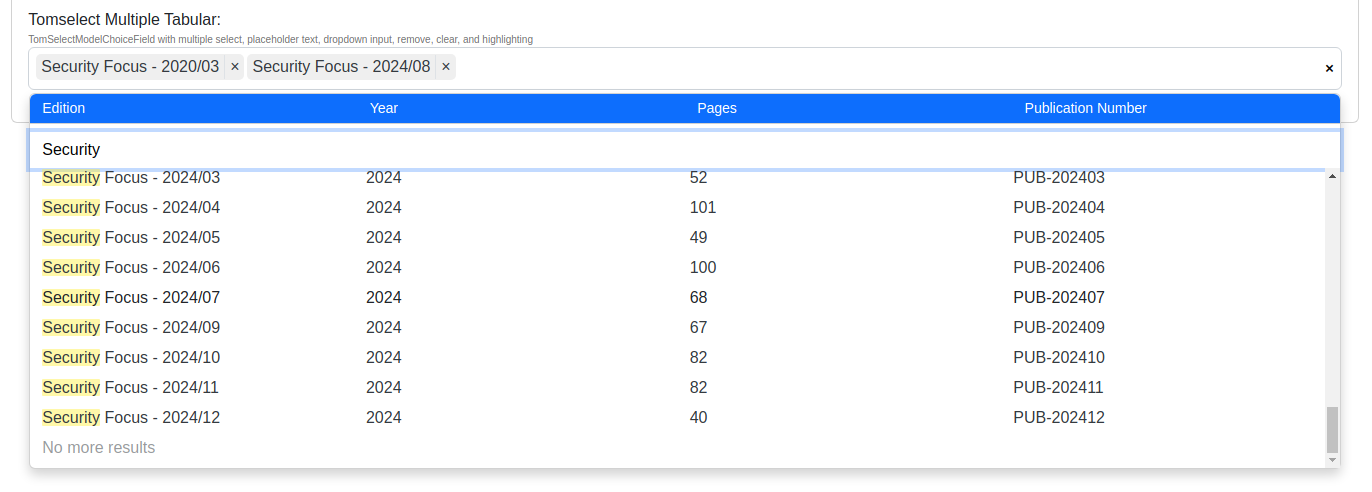
## Quick Start
1. **Install the package:**
```bash
pip install django-tomselect
```
2. **Update settings.py:**
```python
INSTALLED_APPS = [
...
"django_tomselect"
]
MIDDLEWARE = [
...
"django_tomselect.middleware.TomSelectMiddleware",
...
]
TEMPLATES = [
{
"OPTIONS": {
"context_processors": [
...
"django_tomselect.context_processors.tomselect",
...
],
},
},
]
```
3. **Create an autocomplete view:**
```python
from django_tomselect.autocompletes import AutocompleteModelView
class PersonAutocompleteView(AutocompleteModelView):
model = Person
search_lookups = ["full_name__icontains"]
value_fields = ["id","full_name"]
```
4. **Add URL pattern:**
```python
urlpatterns = [
path("person-autocomplete/", PersonAutocompleteView.as_view(), name="person_autocomplete"),
]
```
5. **Use in your forms:**
```python
from django_tomselect.forms import TomSelectModelChoiceField, TomSelectConfig
class MyForm(forms.Form):
person = TomSelectModelChoiceField(
config = TomSelectConfig(
url="person_autocomplete",
value_field="id",
label_field="full_name",
)
)
```
6. **Include in your template:**
```html
{{ form.media }} {# Adds the required CSS/JS #}
{{ form }}
```
## Other Features
### Advanced Filtering
- Dependent/chained select fields
- Field exclusion support
- Custom search implementations
- Hooks for overriding functionality
### Flexible Configuration
- Support for [Tom Select Plugins](https://tom-select.js.org/plugins/)
- Global settings and per-form-field configuration
- Override any template
### Security
- Built-in permission handling
- including django auth, custom auth, object perms
### Internationalization
- Translation support
- Customizable messages
## Example Project
To see Django TomSelect in action, check out the [Example Project](https://github.com/OmenApps/django-tomselect/tree/main/example_project). It demonstrates a variety of use cases, with **15 different implementations** from basic atocompletion to advanced applications, showing how to use django-tomselect's autocomplete fields in a Django project.
Each of the examples is described in [the Example Project docs](https://django-tomselect.readthedocs.io/en/latest/example_app/introduction.html).
Here are a few screenshots from the example project:
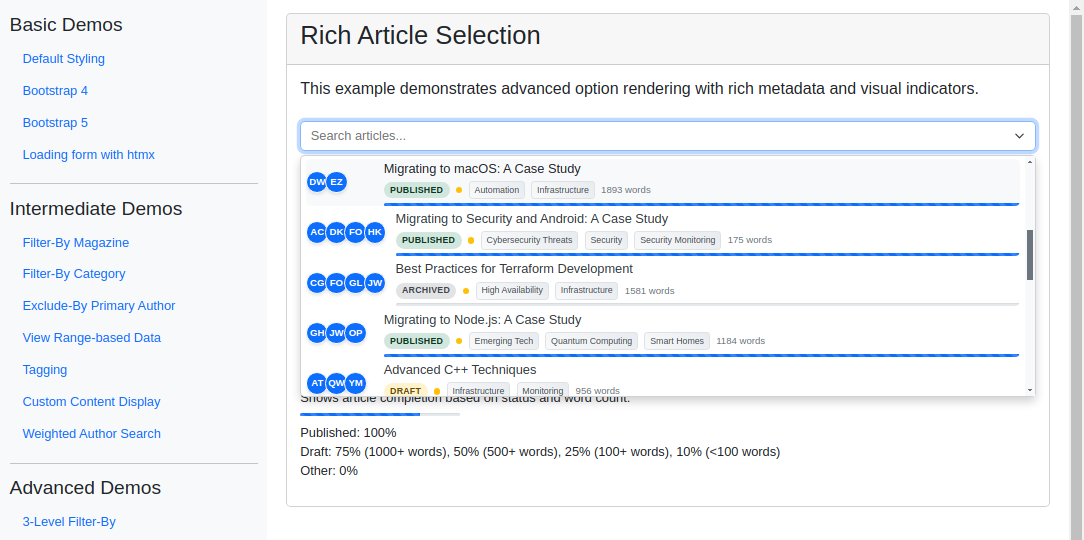
---
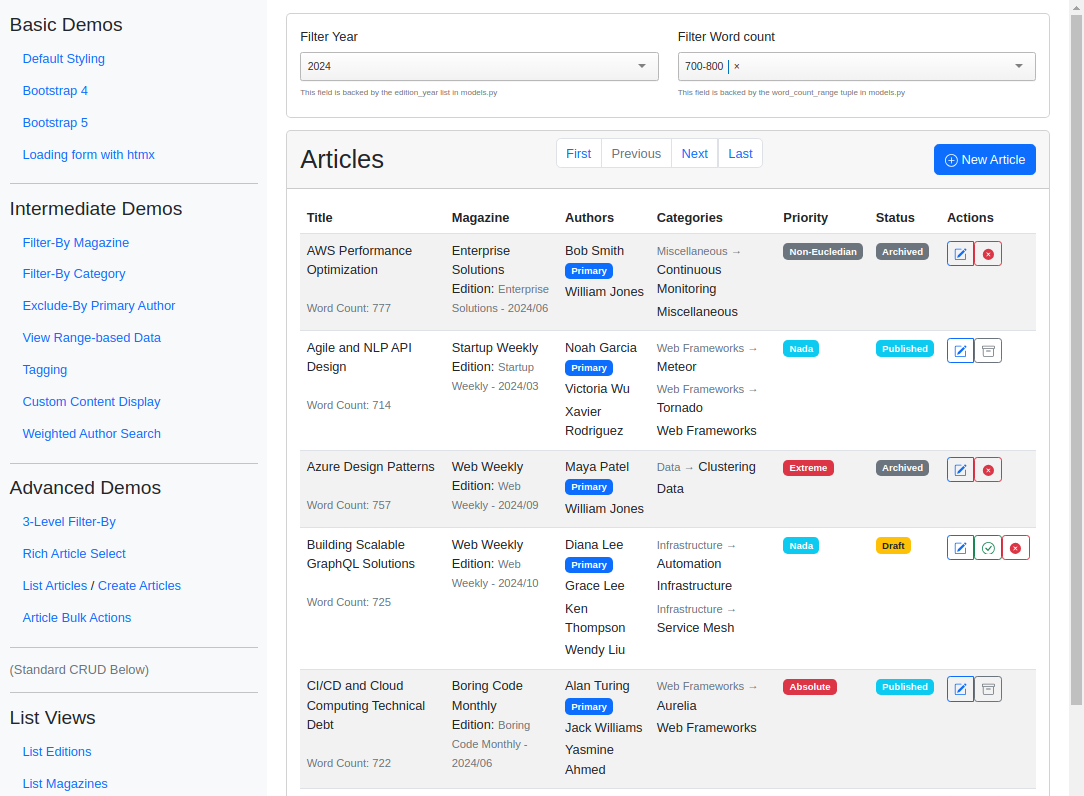
---
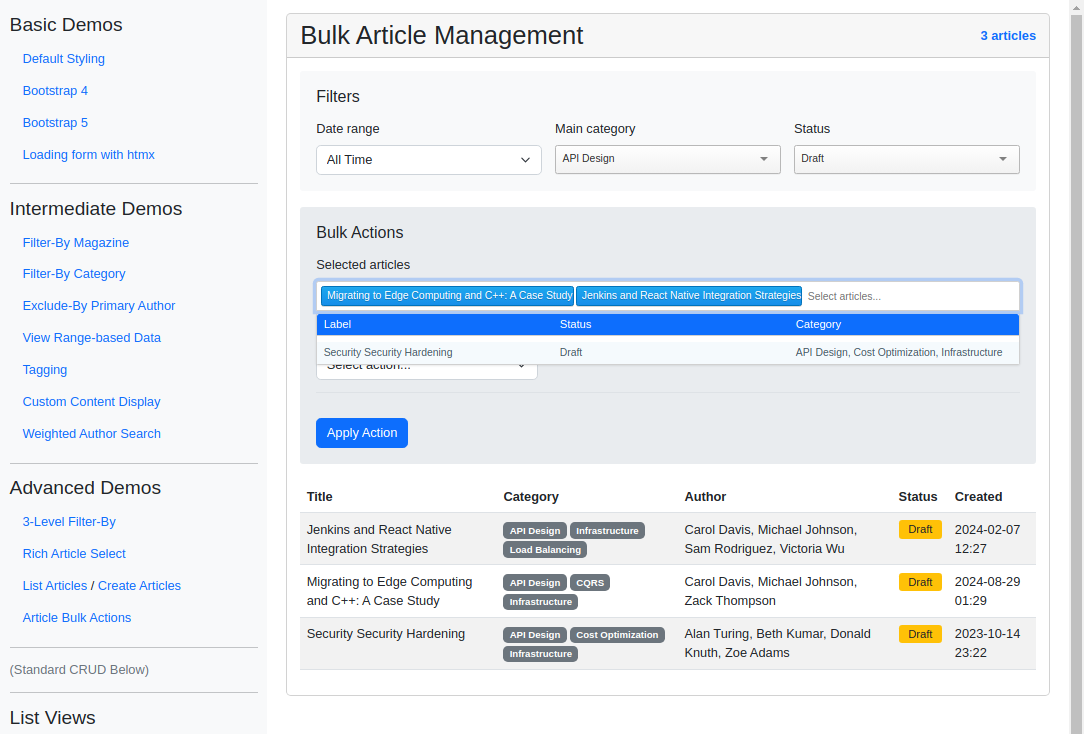
## Documentation
- [Complete Usage Guide](https://django-tomselect.readthedocs.io/en/latest/usage.html)
- [Configuration Reference](https://django-tomselect.readthedocs.io/en/latest/api/config.html)
- [Example Project](https://django-tomselect.readthedocs.io/en/latest/example_app/introduction.html)
## Contributing
Contributions are welcome! Check out our [Contributor Guide](https://github.com/OmenApps/django-tomselect/blob/main/CONTRIBUTING.md) to get started.
## License
This project is licensed under the MIT License - see the [License](https://github.com/OmenApps/django-tomselect/blob/main/LICENSE) file for details.
## Acknowledgments
This package builds on the excellent work of [Philip Becker](https://pypi.org/user/actionb/) in [mizdb-tomselect](https://www.pypi.org/project/mizdb-tomselect/), with a focus on generalization, Django templates, translations, and customization.
Raw data
{
"_id": null,
"home_page": null,
"name": "django-tomselect",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.10",
"maintainer_email": null,
"keywords": "django, autocomplete, tomselect, widget, forms, views, select, multiselect",
"author": null,
"author_email": "Jack Linke <jacklinke@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/cc/fa/1a5c72620f5c9c521b8885869a051741b547316b273b02c4d0822d7f85f1/django_tomselect-2025.2.1.tar.gz",
"platform": null,
"description": "\n\n# Django TomSelect\n\nA powerful, lightweight Django package for dynamic select inputs with autocomplete, tagging, and more.\n\n[](https://badge.fury.io/py/django-tomselect.png)\n[](https://github.com/OmenApps/django-tomselect/blob/main/LICENSE)\n\nDjango TomSelect integrates [Tom Select](https://tom-select.js.org/) into your Django projects, providing beautiful and intuitive select inputs with features like:\n\n- **Live Search & Autocomplete**\n - Real-time filtering and highlighting as you type\n - Server-side search with customizable lookups\n - Automatic pagination for large datasets\n\t- Customizable minimum query length\n\n- **Rich UI Options**\n - Single and multiple selection modes\n - Tabular display with custom columns\n - Bootstrap 4/5 theming support\n\t- Clear/remove buttons\n\t- Dropdown headers & footers\n\t- Checkbox options\n - Customizable templates\n\n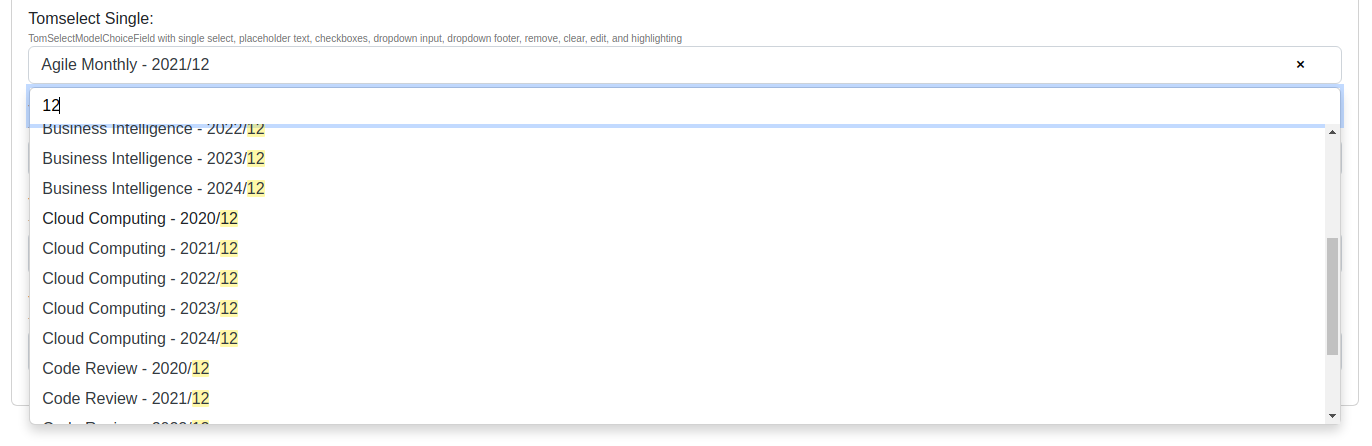\n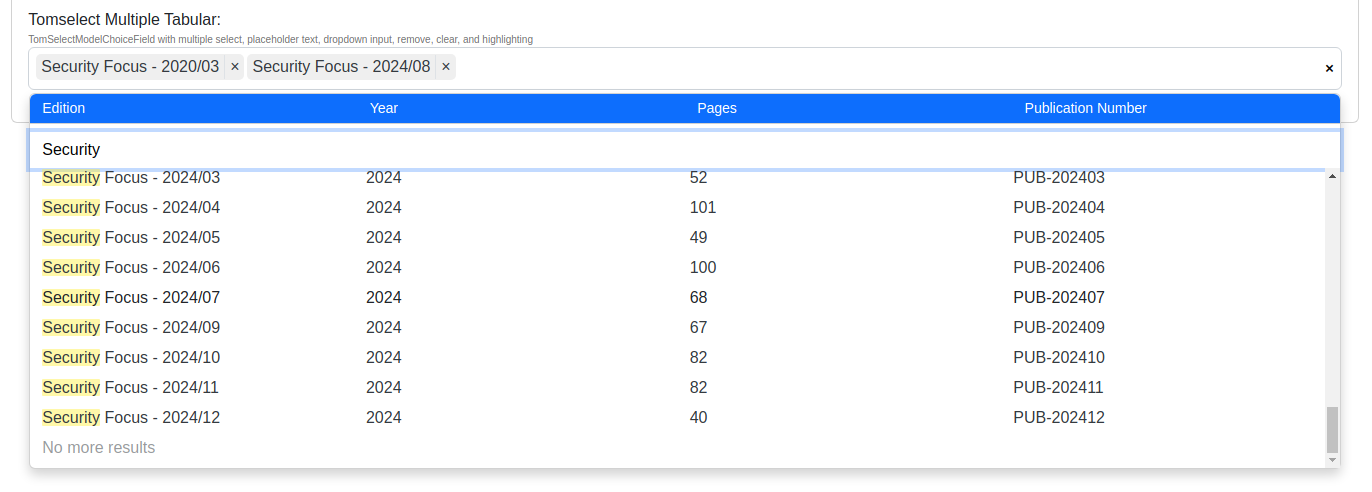\n\n## Quick Start\n\n1. **Install the package:**\n```bash\npip install django-tomselect\n```\n\n2. **Update settings.py:**\n```python\nINSTALLED_APPS = [\n ...\n \"django_tomselect\"\n]\n\nMIDDLEWARE = [\n ...\n \"django_tomselect.middleware.TomSelectMiddleware\",\n ...\n]\n\nTEMPLATES = [\n {\n \"OPTIONS\": {\n \"context_processors\": [\n ...\n \"django_tomselect.context_processors.tomselect\",\n ...\n ],\n },\n },\n]\n```\n\n3. **Create an autocomplete view:**\n```python\nfrom django_tomselect.autocompletes import AutocompleteModelView\n\nclass PersonAutocompleteView(AutocompleteModelView):\n model = Person\n search_lookups = [\"full_name__icontains\"]\n value_fields = [\"id\",\"full_name\"]\n```\n\n4. **Add URL pattern:**\n```python\nurlpatterns = [\n path(\"person-autocomplete/\", PersonAutocompleteView.as_view(), name=\"person_autocomplete\"),\n]\n```\n\n5. **Use in your forms:**\n```python\nfrom django_tomselect.forms import TomSelectModelChoiceField, TomSelectConfig\n\nclass MyForm(forms.Form):\n person = TomSelectModelChoiceField(\n config = TomSelectConfig(\n url=\"person_autocomplete\",\n value_field=\"id\",\n label_field=\"full_name\",\n )\n )\n```\n\n6. **Include in your template:**\n```html\n{{ form.media }} {# Adds the required CSS/JS #}\n\n{{ form }}\n```\n\n## Other Features\n\n### Advanced Filtering\n- Dependent/chained select fields\n- Field exclusion support\n- Custom search implementations\n- Hooks for overriding functionality\n\n### Flexible Configuration\n- Support for [Tom Select Plugins](https://tom-select.js.org/plugins/)\n- Global settings and per-form-field configuration\n- Override any template\n\n### Security\n- Built-in permission handling\n\t- including django auth, custom auth, object perms\n\n### Internationalization\n- Translation support\n- Customizable messages\n\n## Example Project\n\nTo see Django TomSelect in action, check out the [Example Project](https://github.com/OmenApps/django-tomselect/tree/main/example_project). It demonstrates a variety of use cases, with **15 different implementations** from basic atocompletion to advanced applications, showing how to use django-tomselect's autocomplete fields in a Django project.\n\nEach of the examples is described in [the Example Project docs](https://django-tomselect.readthedocs.io/en/latest/example_app/introduction.html).\n\nHere are a few screenshots from the example project:\n\n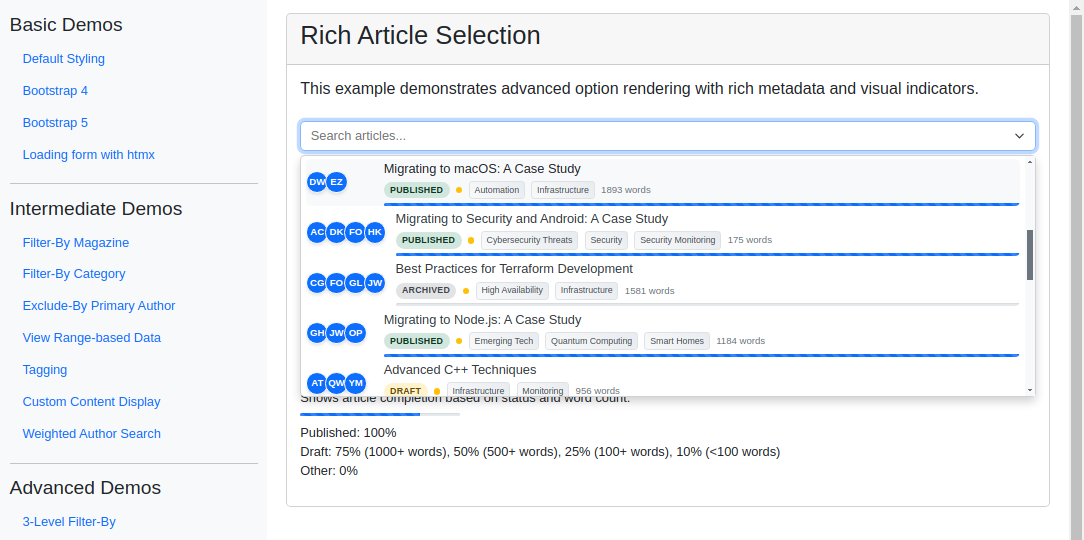\n\n---\n\n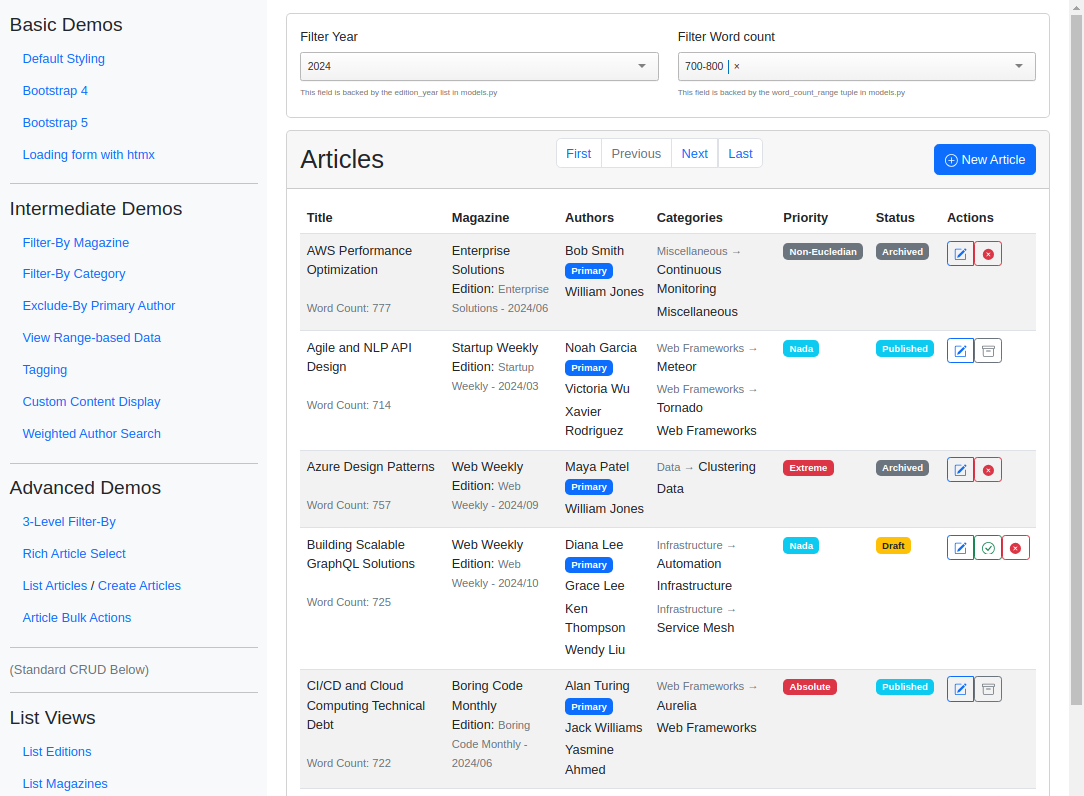\n\n---\n\n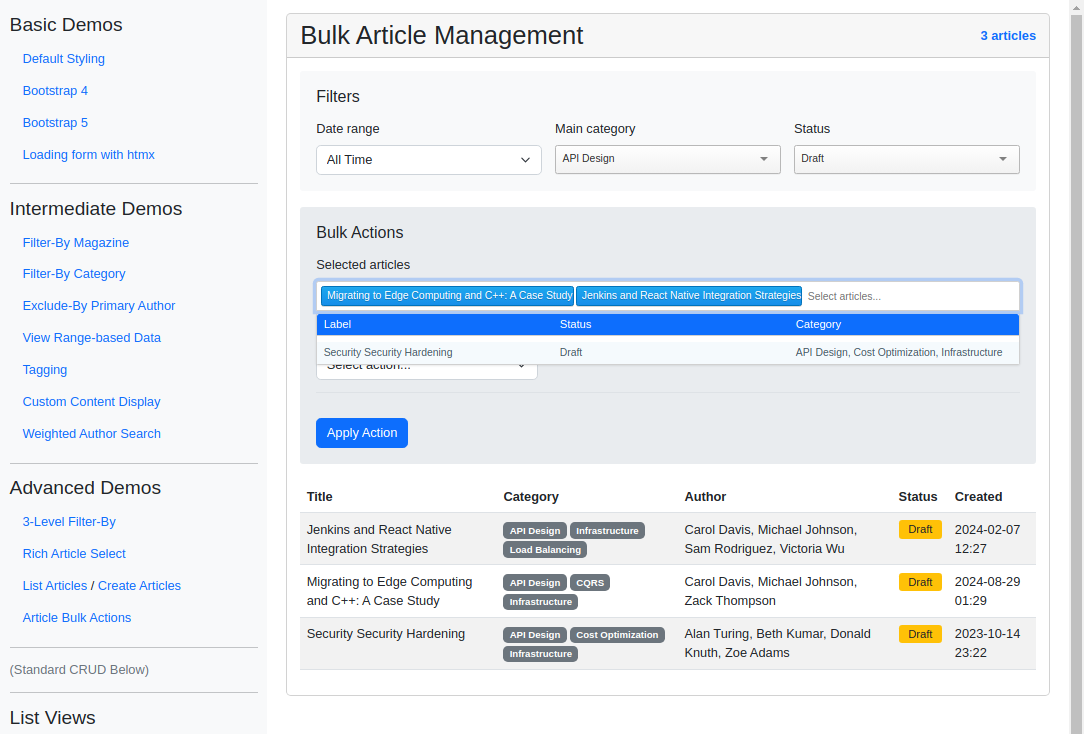\n\n## Documentation\n\n- [Complete Usage Guide](https://django-tomselect.readthedocs.io/en/latest/usage.html)\n- [Configuration Reference](https://django-tomselect.readthedocs.io/en/latest/api/config.html)\n- [Example Project](https://django-tomselect.readthedocs.io/en/latest/example_app/introduction.html)\n\n## Contributing\n\nContributions are welcome! Check out our [Contributor Guide](https://github.com/OmenApps/django-tomselect/blob/main/CONTRIBUTING.md) to get started.\n\n## License\n\nThis project is licensed under the MIT License - see the [License](https://github.com/OmenApps/django-tomselect/blob/main/LICENSE) file for details.\n\n## Acknowledgments\n\nThis package builds on the excellent work of [Philip Becker](https://pypi.org/user/actionb/) in [mizdb-tomselect](https://www.pypi.org/project/mizdb-tomselect/), with a focus on generalization, Django templates, translations, and customization.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Django autocomplete widgets and views using Tom Select",
"version": "2025.2.1",
"project_urls": {
"Changelog": "https://github.com/OmenApps/django-tomselect/releases",
"Documentation": "https://django-tomselect.readthedocs.io/en/latest/",
"Issues": "https://github.com/OmenApps/django-tomselect/issues",
"Source": "https://github.com/OmenApps/django-tomselect"
},
"split_keywords": [
"django",
" autocomplete",
" tomselect",
" widget",
" forms",
" views",
" select",
" multiselect"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "873c010269ba972b6aa6557264ec68ef0eeb6d6c1505e0a2d9cf746bca416bea",
"md5": "2110b95dd7fe7dd2cf415dbf8f3aa3cc",
"sha256": "874fc2d5817a7b4fbdb18b84e344af856281de6f1f5da1881452b4e604ad0892"
},
"downloads": -1,
"filename": "django_tomselect-2025.2.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2110b95dd7fe7dd2cf415dbf8f3aa3cc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.10",
"size": 221113,
"upload_time": "2025-02-16T04:54:52",
"upload_time_iso_8601": "2025-02-16T04:54:52.983719Z",
"url": "https://files.pythonhosted.org/packages/87/3c/010269ba972b6aa6557264ec68ef0eeb6d6c1505e0a2d9cf746bca416bea/django_tomselect-2025.2.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "ccfa1a5c72620f5c9c521b8885869a051741b547316b273b02c4d0822d7f85f1",
"md5": "ab343aba15c28522ef643dbda78b7704",
"sha256": "daabdf09fd1b75df97815ba5dad4b92a0b2e7d3f15aeb10e3d992ece8cbf9515"
},
"downloads": -1,
"filename": "django_tomselect-2025.2.1.tar.gz",
"has_sig": false,
"md5_digest": "ab343aba15c28522ef643dbda78b7704",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.10",
"size": 192551,
"upload_time": "2025-02-16T04:54:54",
"upload_time_iso_8601": "2025-02-16T04:54:54.642035Z",
"url": "https://files.pythonhosted.org/packages/cc/fa/1a5c72620f5c9c521b8885869a051741b547316b273b02c4d0822d7f85f1/django_tomselect-2025.2.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2025-02-16 04:54:54",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "OmenApps",
"github_project": "django-tomselect",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [
{
"name": "asgiref",
"specs": [
[
"==",
"3.8.1"
]
]
},
{
"name": "django",
"specs": [
[
"==",
"5.1.2"
]
]
},
{
"name": "django-htmx",
"specs": [
[
"==",
"1.21.0"
]
]
},
{
"name": "iniconfig",
"specs": [
[
"==",
"2.0.0"
]
]
},
{
"name": "packaging",
"specs": [
[
"==",
"24.2"
]
]
},
{
"name": "pluggy",
"specs": [
[
"==",
"1.5.0"
]
]
},
{
"name": "pytest",
"specs": [
[
"==",
"8.3.4"
]
]
},
{
"name": "pytest-asyncio",
"specs": [
[
"==",
"0.25.0"
]
]
},
{
"name": "sqlparse",
"specs": [
[
"==",
"0.5.1"
]
]
}
],
"lcname": "django-tomselect"
}