Make your Python output dramatic 🎭
==================================
[](https://pypi.org/project/dramatic/)
[](https://opensource.org/licenses/MIT)
[](https://github.com/treyhunner/dramatic/actions?workflow=test)
[](https://codecov.io/gh/treyhunner/dramatic)
The `dramatic` module includes utilities which cause all text output to display character-by-character (it prints *dramatically*).
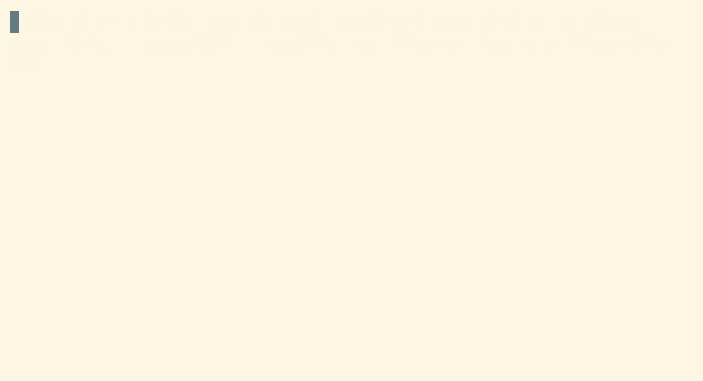
**Note**: This project is based on a [Python Morsels](https://www.pythonmorsels.com) exercise.
If you're working on that exercise right now, please don't look at the source code for this! 😉
<a href="https://www.pythonmorsels.com">
<img src="https://raw.githubusercontent.com/treyhunner/dramatic/main/screenshots/python-morsels-logo.png" alt="an adorable snake taking a bite out of a cookie with the words Python Morsels next to it (Python Morsels logo)" width="250">
</a>
Usage 🥁
-------
The `dramatic` module is available on [PyPI][].
You can install it with `pip`:
```bash
$ python3 -m pip install dramatic
```
There are four primary ways to use the utilities in the `dramatic` module:
1. As a **context manager** that temporarily makes output display dramatically
2. As a **decorator** that temporarily makes output display dramatically
3. Using a `dramatic.start()` function that makes output display dramatically
4. Using a `dramatic.print` function to display specific text dramatically
Dramatic Context Manager 🚪
--------------------------
The `dramatic.output` [context manager][] will temporarily cause all standard output and standard error to display dramatically:
```python
import dramatic
def main():
print("This function prints")
with dramatic.output:
main()
```
To change the printing speed from the default of 75 characters per second to another value (30 characters per second in this case) use the `at_speed` method:
```python
import dramatic
def main():
print("This function prints")
with dramatic.output.at_speed(30):
main()
```
Example context manager usage:
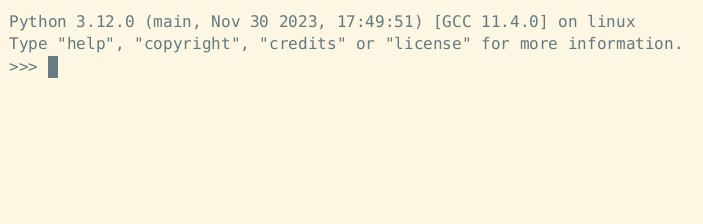
Dramatic Decorator 🎀
--------------------
The `dramatic.output` [decorator][] will cause all standard output and standard error to display dramatically while the decorated function is running:
```python
import dramatic
@dramatic.output
def main():
print("This function prints")
main()
```
The `at_speed` method works as a decorator as well:
```python
import dramatic
@dramatic.output.at_speed(30)
def main():
print("This function prints")
main()
```
Example decorator usage:
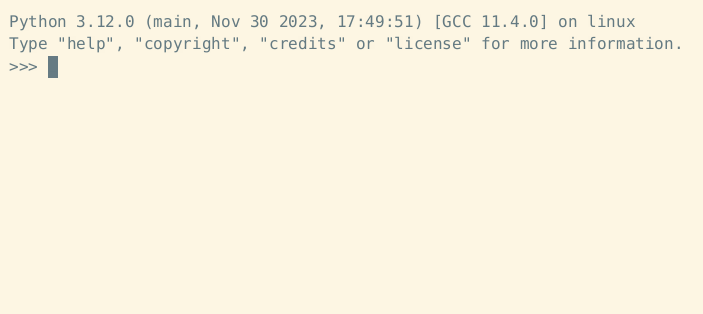
Manually Starting and Stopping 🔧
--------------------------------
Instead of enabling dramatic printing temporarily with a context manager or decorator, the `dramatic.start` function may be used to enable dramatic printing:
```python
import dramatic
def main():
print("This function prints")
dramatic.start()
main()
```
The `speed` keyword argument may be used to change the printing speed (in characters per second):
```python
import dramatic
def main():
print("This function prints")
dramatic.start(speed=30)
main()
```
To make *only* standard output dramatic (but not standard error) pass `stderr=False` to `start`:
```python
import dramatic
def main():
print("This function prints")
dramatic.start(stderr=False)
main()
```
To disable dramatic printing, the `dramatic.stop` function may be used.
Here's an example [context manager][] that uses both `dramatic.start` and `dramatic.stop`:
```python
import dramatic
class CustomContextManager:
def __enter__(self):
print("Printing will become dramatic now")
dramatic.start()
def __exit__(self):
dramatic.stop()
print("Dramatic printing has stopped")
```
Example `start` and `stop` usage:
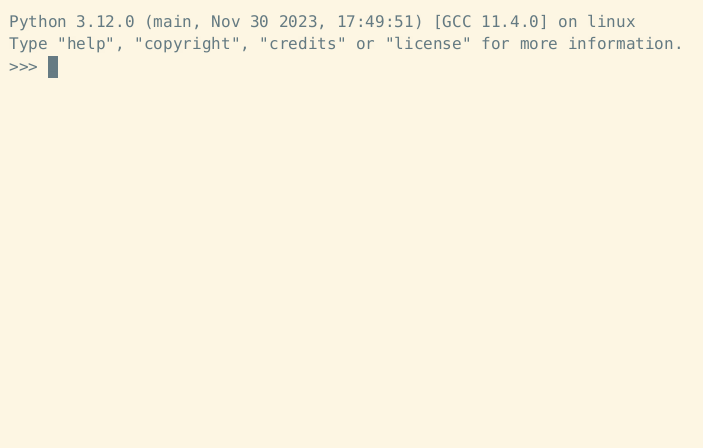
Dramatic Print 🖨️
----------------
The `dramatic.print` function acts just like the built-in `print` function, but it prints dramatically:
```python
import dramatic
dramatic.print("This will print some text dramatically")
```
Dramatic Interpreter ⌨️
----------------------
To start a dramatic [Python REPL][]:
```bash
$ python3 -m dramatic
>>>
```
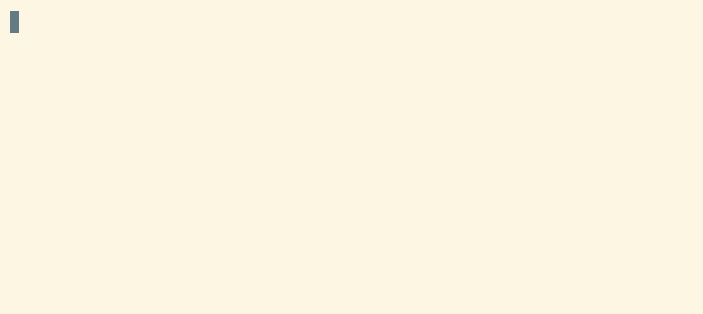
To dramatically run a Python module:
```bash
$ python3 -m dramatic -m this
```
To dramatically run a Python file:
```bash
$ python3 -m dramatic hello_world.py
```
The `dramatic` module also accepts a `--speed` argument to set the characters printed per second.
In this example we're increasing the speed from 75 characters-per-second to 120:
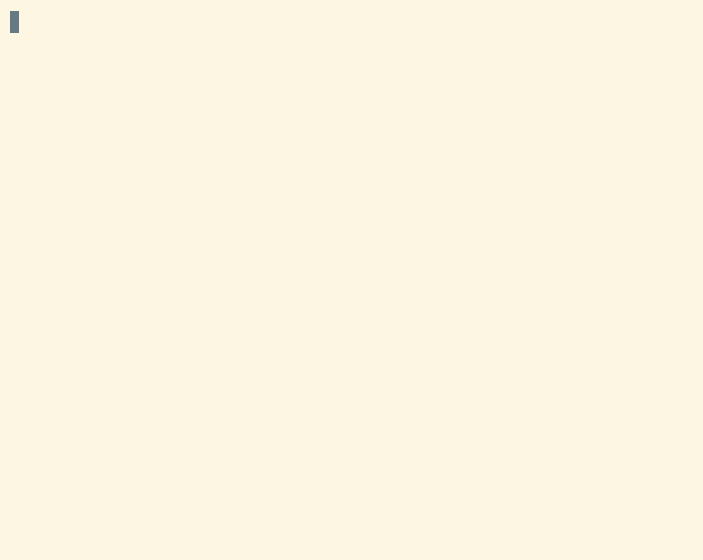
Maximum Drama (Use With Caution ⚠️)
----------------------------------
Want to make your Python interpreter dramatic *by default*?
Run the `dramatic` module as a script with the `--max-drama` argument to modify Python so that *all* your Python programs will print dramatically:
```bash
$ python3 -m dramatic --max-drama
This will cause all Python programs to run dramatically.
Running --min-drama will undo this operation.
Are you sure? [y/N]
```
Example:
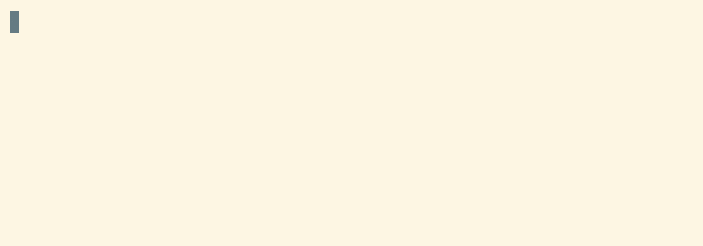
If the drama is too much, run the module again with the argument `--min-drama` to undo:
```bash
$ python3 -m dramatic --min-drama
Deleted file /home/trey/.local/lib/python3.12/site-packages/dramatic.pth
Deleted file /home/trey/.local/lib/python3.12/site-packages/_dramatic.py
No drama.
```
Example:
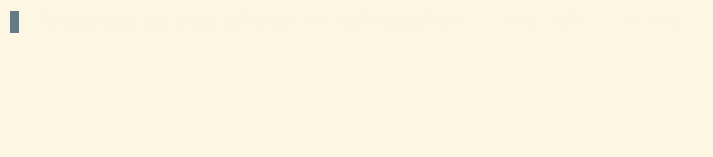
This even works if you don't have the `dramatic` module installed.
Just download [dramatic.py](https://github.com/treyhunner/dramatic/blob/main/dramatic.py) and run it with `--max-drama`!
To disable the drama, you'll need to run `python3 -m _dramatic --min-drama` (note the `_` before `dramatic`).
**Warning**: using `--max-drama` is *probably a bad idea*.
Use with caution.
Other Features ✨
----------------
Other features of note:
- Pressing `Ctrl-C` while text is printing dramatically will cause the remaining text to print immediately.
- Dramatic printing is automatically disabled when the output stream is piped to a file (e.g. `python3 my_script.py > output.txt`)
Credits 💖
---------
This package was inspired by [the **dramatic print** Python Morsels exercise][dramatic print], which was partially inspired by Brandon Rhodes' [adventure][] Python port (which displays its text at 1200 baud).
[pypi]: https://pypi.org/project/dramatic/
[context manager]: https://www.pythonmorsels.com/what-is-a-context-manager/
[decorator]: https://www.pythonmorsels.com/what-is-a-decorator/
[python repl]: https://www.pythonmorsels.com/using-the-python-repl/
[dramatic print]: https://www.pythonmorsels.com/exercises/57338fa2ecc342e3bad18afdbf12aacd/
[adventure]: https://pypi.org/project/adventure/
Raw data
{
"_id": null,
"home_page": null,
"name": "dramatic",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "Python Morsels, TTY, modem, teletype, terminal, typewriter",
"author": "Trey Hunner",
"author_email": null,
"download_url": "https://files.pythonhosted.org/packages/0a/f0/ccdb4a063882cc3896bf96791e8b988e99b9416c4ddb015e5d130f3f27a5/dramatic-0.4.1.post1.tar.gz",
"platform": null,
"description": "Make your Python output dramatic \ud83c\udfad\n==================================\n\n[](https://pypi.org/project/dramatic/)\n[](https://opensource.org/licenses/MIT)\n[](https://github.com/treyhunner/dramatic/actions?workflow=test)\n[](https://codecov.io/gh/treyhunner/dramatic)\n\nThe `dramatic` module includes utilities which cause all text output to display character-by-character (it prints *dramatically*).\n\n\n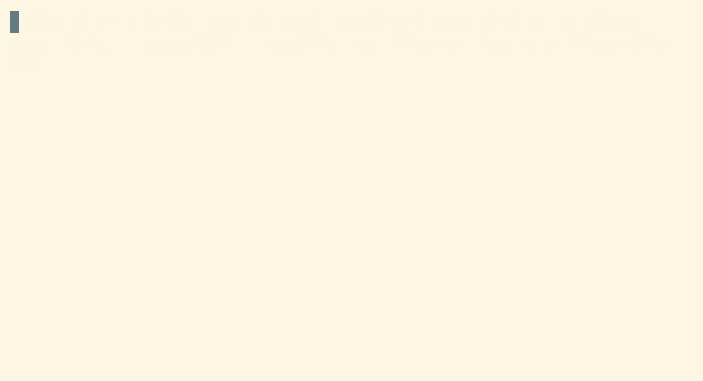\n\n**Note**: This project is based on a [Python Morsels](https://www.pythonmorsels.com) exercise.\nIf you're working on that exercise right now, please don't look at the source code for this! \ud83d\ude09\n\n<a href=\"https://www.pythonmorsels.com\">\n <img src=\"https://raw.githubusercontent.com/treyhunner/dramatic/main/screenshots/python-morsels-logo.png\" alt=\"an adorable snake taking a bite out of a cookie with the words Python Morsels next to it (Python Morsels logo)\" width=\"250\">\n</a>\n\n\nUsage \ud83e\udd41\n-------\n\nThe `dramatic` module is available on [PyPI][].\nYou can install it with `pip`:\n\n```bash\n$ python3 -m pip install dramatic\n```\n\nThere are four primary ways to use the utilities in the `dramatic` module:\n\n1. As a **context manager** that temporarily makes output display dramatically\n2. As a **decorator** that temporarily makes output display dramatically\n3. Using a `dramatic.start()` function that makes output display dramatically\n4. Using a `dramatic.print` function to display specific text dramatically\n\n\nDramatic Context Manager \ud83d\udeaa\n--------------------------\n\nThe `dramatic.output` [context manager][] will temporarily cause all standard output and standard error to display dramatically:\n\n```python\nimport dramatic\n\ndef main():\n print(\"This function prints\")\n\nwith dramatic.output:\n main()\n```\n\nTo change the printing speed from the default of 75 characters per second to another value (30 characters per second in this case) use the `at_speed` method:\n\n\n```python\nimport dramatic\n\ndef main():\n print(\"This function prints\")\n\nwith dramatic.output.at_speed(30):\n main()\n```\n\nExample context manager usage:\n\n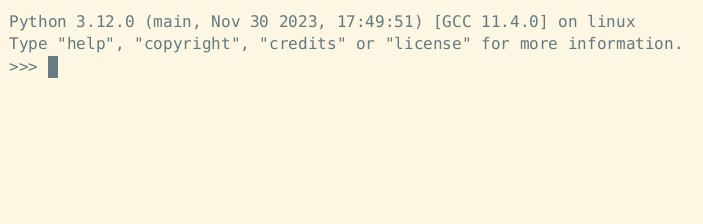\n\n\nDramatic Decorator \ud83c\udf80\n--------------------\n\nThe `dramatic.output` [decorator][] will cause all standard output and standard error to display dramatically while the decorated function is running:\n\n```python\nimport dramatic\n\n@dramatic.output\ndef main():\n print(\"This function prints\")\n\nmain()\n```\n\nThe `at_speed` method works as a decorator as well:\n\n\n```python\nimport dramatic\n\n@dramatic.output.at_speed(30)\ndef main():\n print(\"This function prints\")\n\nmain()\n```\n\nExample decorator usage:\n\n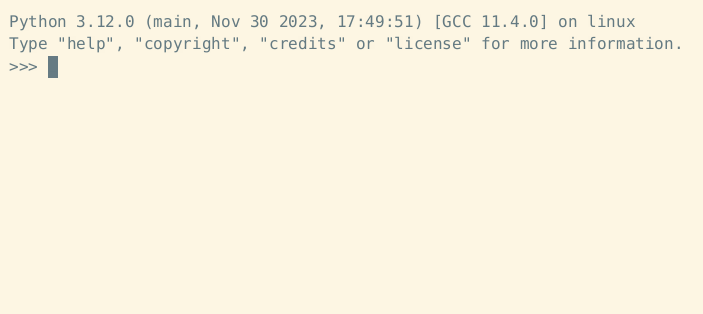\n\n\nManually Starting and Stopping \ud83d\udd27\n--------------------------------\n\nInstead of enabling dramatic printing temporarily with a context manager or decorator, the `dramatic.start` function may be used to enable dramatic printing:\n\n```python\nimport dramatic\n\ndef main():\n print(\"This function prints\")\n\ndramatic.start()\nmain()\n```\n\nThe `speed` keyword argument may be used to change the printing speed (in characters per second):\n\n```python\nimport dramatic\n\ndef main():\n print(\"This function prints\")\n\ndramatic.start(speed=30)\nmain()\n```\n\nTo make *only* standard output dramatic (but not standard error) pass `stderr=False` to `start`:\n\n```python\nimport dramatic\n\ndef main():\n print(\"This function prints\")\n\ndramatic.start(stderr=False)\nmain()\n```\n\nTo disable dramatic printing, the `dramatic.stop` function may be used.\nHere's an example [context manager][] that uses both `dramatic.start` and `dramatic.stop`:\n\n```python\nimport dramatic\n\n\nclass CustomContextManager:\n def __enter__(self):\n print(\"Printing will become dramatic now\")\n dramatic.start()\n def __exit__(self):\n dramatic.stop()\n print(\"Dramatic printing has stopped\")\n```\n\nExample `start` and `stop` usage:\n\n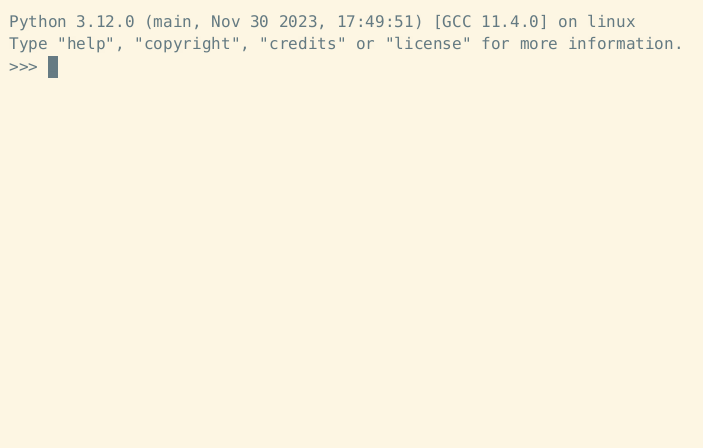\n\n\nDramatic Print \ud83d\udda8\ufe0f\n----------------\n\nThe `dramatic.print` function acts just like the built-in `print` function, but it prints dramatically:\n\n```python\nimport dramatic\ndramatic.print(\"This will print some text dramatically\")\n```\n\n\nDramatic Interpreter \u2328\ufe0f\n----------------------\n\nTo start a dramatic [Python REPL][]:\n\n```bash\n$ python3 -m dramatic\n>>>\n```\n\n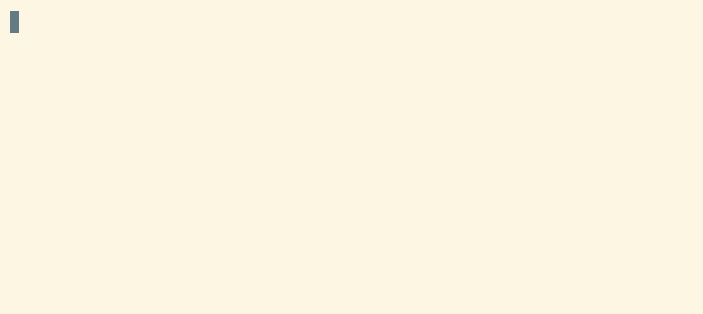\n\nTo dramatically run a Python module:\n\n```bash\n$ python3 -m dramatic -m this\n```\n\nTo dramatically run a Python file:\n\n```bash\n$ python3 -m dramatic hello_world.py\n```\n\nThe `dramatic` module also accepts a `--speed` argument to set the characters printed per second.\nIn this example we're increasing the speed from 75 characters-per-second to 120:\n\n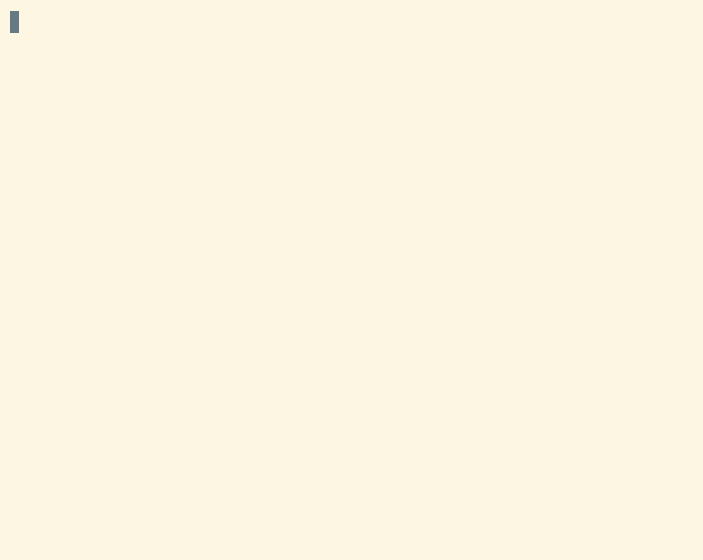\n\n\nMaximum Drama (Use With Caution \u26a0\ufe0f)\n----------------------------------\n\nWant to make your Python interpreter dramatic *by default*?\n\nRun the `dramatic` module as a script with the `--max-drama` argument to modify Python so that *all* your Python programs will print dramatically:\n\n```bash\n$ python3 -m dramatic --max-drama\nThis will cause all Python programs to run dramatically.\nRunning --min-drama will undo this operation.\nAre you sure? [y/N]\n```\n\nExample:\n\n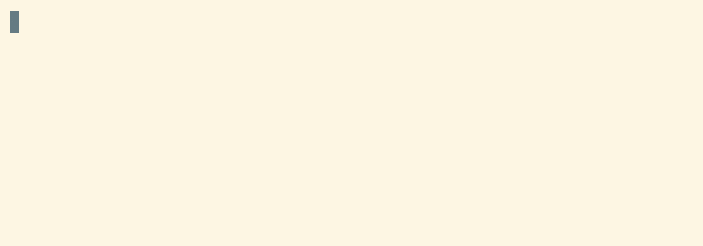\n\nIf the drama is too much, run the module again with the argument `--min-drama` to undo:\n\n```bash\n$ python3 -m dramatic --min-drama\nDeleted file /home/trey/.local/lib/python3.12/site-packages/dramatic.pth\nDeleted file /home/trey/.local/lib/python3.12/site-packages/_dramatic.py\nNo drama.\n```\n\nExample:\n\n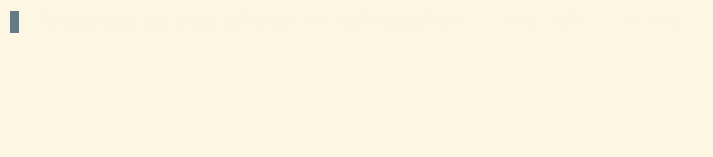\n\nThis even works if you don't have the `dramatic` module installed.\nJust download [dramatic.py](https://github.com/treyhunner/dramatic/blob/main/dramatic.py) and run it with `--max-drama`!\nTo disable the drama, you'll need to run `python3 -m _dramatic --min-drama` (note the `_` before `dramatic`).\n\n**Warning**: using `--max-drama` is *probably a bad idea*.\nUse with caution.\n\n\nOther Features \u2728\n----------------\n\nOther features of note:\n\n- Pressing `Ctrl-C` while text is printing dramatically will cause the remaining text to print immediately.\n- Dramatic printing is automatically disabled when the output stream is piped to a file (e.g. `python3 my_script.py > output.txt`)\n\n\nCredits \ud83d\udc96\n---------\n\nThis package was inspired by [the **dramatic print** Python Morsels exercise][dramatic print], which was partially inspired by Brandon Rhodes' [adventure][] Python port (which displays its text at 1200 baud).\n\n\n[pypi]: https://pypi.org/project/dramatic/\n[context manager]: https://www.pythonmorsels.com/what-is-a-context-manager/\n[decorator]: https://www.pythonmorsels.com/what-is-a-decorator/\n[python repl]: https://www.pythonmorsels.com/using-the-python-repl/\n[dramatic print]: https://www.pythonmorsels.com/exercises/57338fa2ecc342e3bad18afdbf12aacd/\n[adventure]: https://pypi.org/project/adventure/\n",
"bugtrack_url": null,
"license": null,
"summary": "Display all Python process output character-by-character",
"version": "0.4.1.post1",
"project_urls": {
"Documentation": "https://github.com/treyhunner/dramatic#readme",
"Issues": "https://github.com/treyhunner/dramatic/issues",
"Source": "https://github.com/treyhunner/dramatic"
},
"split_keywords": [
"python morsels",
" tty",
" modem",
" teletype",
" terminal",
" typewriter"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "6028ec1da6c7f83bb2a5896352b43d7fc9ab8070cf331cb4818c19278c41cffa",
"md5": "2b2a4ee59044264c1ae35a3971cf6708",
"sha256": "171caabeb333665f72de873ef7964c0297b4e5abd907d430614dd01b180fa434"
},
"downloads": -1,
"filename": "dramatic-0.4.1.post1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "2b2a4ee59044264c1ae35a3971cf6708",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 7281,
"upload_time": "2024-04-01T07:03:45",
"upload_time_iso_8601": "2024-04-01T07:03:45.198849Z",
"url": "https://files.pythonhosted.org/packages/60/28/ec1da6c7f83bb2a5896352b43d7fc9ab8070cf331cb4818c19278c41cffa/dramatic-0.4.1.post1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "0af0ccdb4a063882cc3896bf96791e8b988e99b9416c4ddb015e5d130f3f27a5",
"md5": "846da597b84af24f9b510ff957a17912",
"sha256": "97a2aea21280ac6bd81b015a6f509ad2d742da503e7b3ff1fd62e5e1c21bac31"
},
"downloads": -1,
"filename": "dramatic-0.4.1.post1.tar.gz",
"has_sig": false,
"md5_digest": "846da597b84af24f9b510ff957a17912",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 728370,
"upload_time": "2024-04-01T07:03:47",
"upload_time_iso_8601": "2024-04-01T07:03:47.005027Z",
"url": "https://files.pythonhosted.org/packages/0a/f0/ccdb4a063882cc3896bf96791e8b988e99b9416c4ddb015e5d130f3f27a5/dramatic-0.4.1.post1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-04-01 07:03:47",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "treyhunner",
"github_project": "dramatic#readme",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "dramatic"
}