# dx
<p align="center">
This package provides convenient formatting and IPython display formatter registration for tabular data and DEX media types.
</p>
<p align="center">
<a href="https://github.com/noteable-io/dx/actions/workflows/ci.yaml">
<img src="https://github.com/noteable-io/dx/actions/workflows/ci.yaml/badge.svg" alt="CI" />
</a>
<a href="https://codecov.io/gh/noteable-io/dx" >
<img src="https://codecov.io/gh/noteable-io/dx/branch/main/graph/badge.svg?token=XGXSTD3GSI" alt="codecov code coverage"/>
</a>
<img alt="PyPI - License" src="https://img.shields.io/pypi/l/dx" />
<img alt="PyPI - Python Version" src="https://img.shields.io/pypi/pyversions/dx" />
<img alt="PyPI" src="https://img.shields.io/pypi/v/dx">
<a href="https://github.com/psf/black"><img alt="Code style: black" src="https://img.shields.io/badge/code%20style-black-000000.svg"></a>
</p>
---------
A Pythonic Data Explorer, open sourced with ❤️ by <a href="https://noteable.io">Noteable</a>, a collaborative notebook platform that enables teams to use and visualize data, together.
## Requirements
Python 3.8+
## Installation
### Poetry
```shell
poetry add dx
```
Then import the package:
```python
import dx
```
### Pip
```shell
pip install dx
```
Then import the package:
```python
import dx
```
## Usage
The `dx` library currently enables DEX media type visualization of pandas `DataFrame` and `Series` objects, as well as numpy `ndarray` objects. This can be handled in two ways:
- explicit `dx.display()` calls
- setting the `display_mode` to update the IPython display formatter for a session
### With `dx.display()`
`dx.display()` will display a single dataset using the DEX media type. It currently supports:
- pandas `DataFrame` objects
```python
import pandas as pd
import random
df = pd.DataFrame({
'random_ints': [random.randint(0, 100) for _ in range(500)],
'random_floats': [random.random() for _ in range(500)],
})
dx.display(df)
```

- tabular data as `dict` or `list` types
```python
dx.display([
[1, 5, 10, 20, 500],
[1, 2, 3, 4, 5],
[0, 0, 0, 0, 1]
])
```

- `.csv` or `.json` filepaths
```python
df = dx.random_dataframe()
df.to_csv("dx_docs_sample.csv", index=False)
dx.display("dx_docs_sample.csv")
```
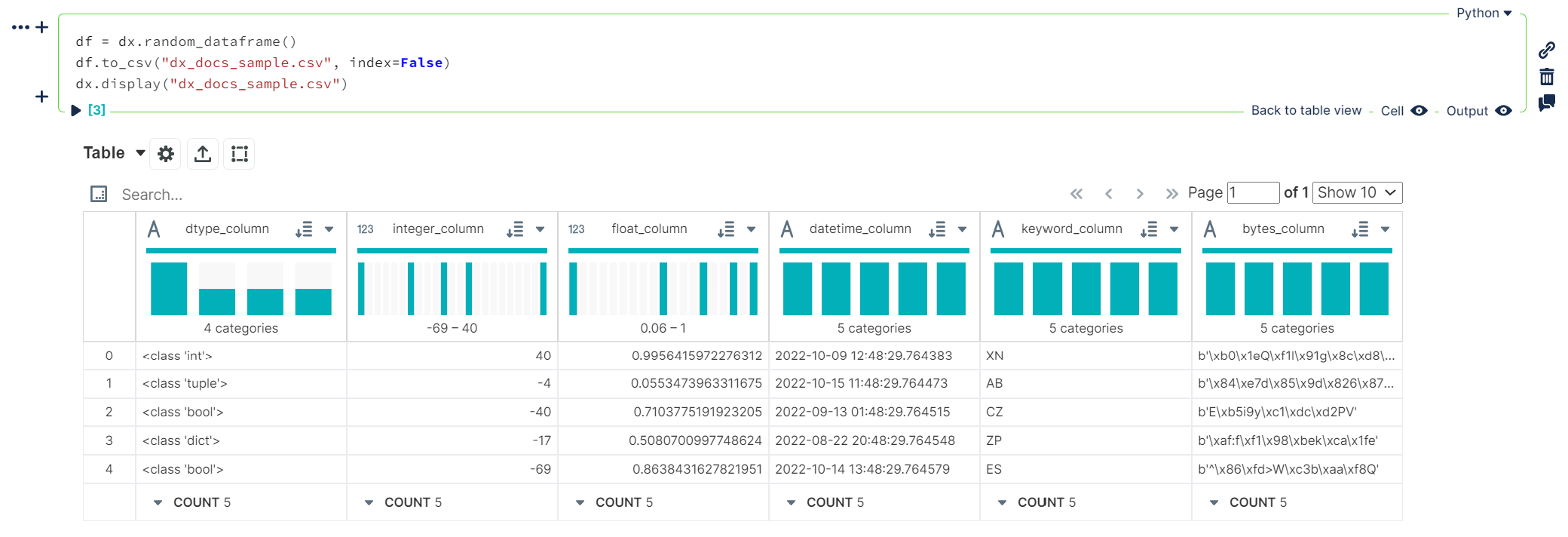
### With `dx.set_display_mode()`
Using either `"simple"` or `"enhanced"` display modes will allow `dx` will update the current `IPython` display formatters to allow DEX media type visualization of pandas `DataFrame` objects for an entire notebook / kernel session instead of the default `DataFrame` display output.
<details>
<summary>Details</summary>
This will adjust pandas options to:
- increasing the number of rows displayed to `50000` from pandas default of `60`
- increasing the number of columns displayed to `50` from pandas default of `20`
- enabling `html.table_schema` (`False` by default in pandas)
This will also handle some basic column cleaning and generate a schema for the `DataFrame` using `pandas.io.json.build_table_schema`. Depending on the display mode, the data will be transformed into either a list of dictionaries or list of lists of columnar values.
- `"simple"` - list of dictionaries
- `"enhanced"` - list of lists
</details>
> **NOTE:**
> Unlike `dx.display()`, this **only** affects pandas DataFrames (or any types set in `settings.RENDERABLE_TYPES`); it does not affect the display of `.csv`/`.json` file data, or `dict`/`list` outputs
- `dx.set_display_mode("simple")`
```python
import dx
import numpy as np
import pandas as pd
# enable DEX display outputs from now on
dx.set_display_mode("simple")
df = pd.read_csv("dx_docs_sample.csv")
df
```
```python
df2 = pd.DataFrame(
[
[1, 5, 10, 20, 500],
[1, 2, 3, np.nan, 5],
[0, 0, 0, np.nan, 1]
],
columns=['a', 'b', 'c', 'd', 'e']
)
df2
```

If, at any point, you want to go back to the default display formatting (vanilla pandas output), use the `"plain"` display mode. This will revert the IPython display format update to its original state and put the pandas options back to their default values.
- `dx.set_display_mode("plain")`
```python
# revert to original pandas display outputs from now on
dx.set_display_mode("plain")
df = pd.read_csv("dx_docs_sample.csv")
df
```
```python
df2 = pd.DataFrame(
[
[1, 5, 10, 20, 500],
[1, 2, 3, np.nan, 5],
[0, 0, 0, np.nan, 1]
],
columns=['a', 'b', 'c', 'd', 'e']
)
df2
```

### Custom Settings
Default settings for `dx` can be found by calling `dx.settings`:

Each can be set using `dx.set_option()`:
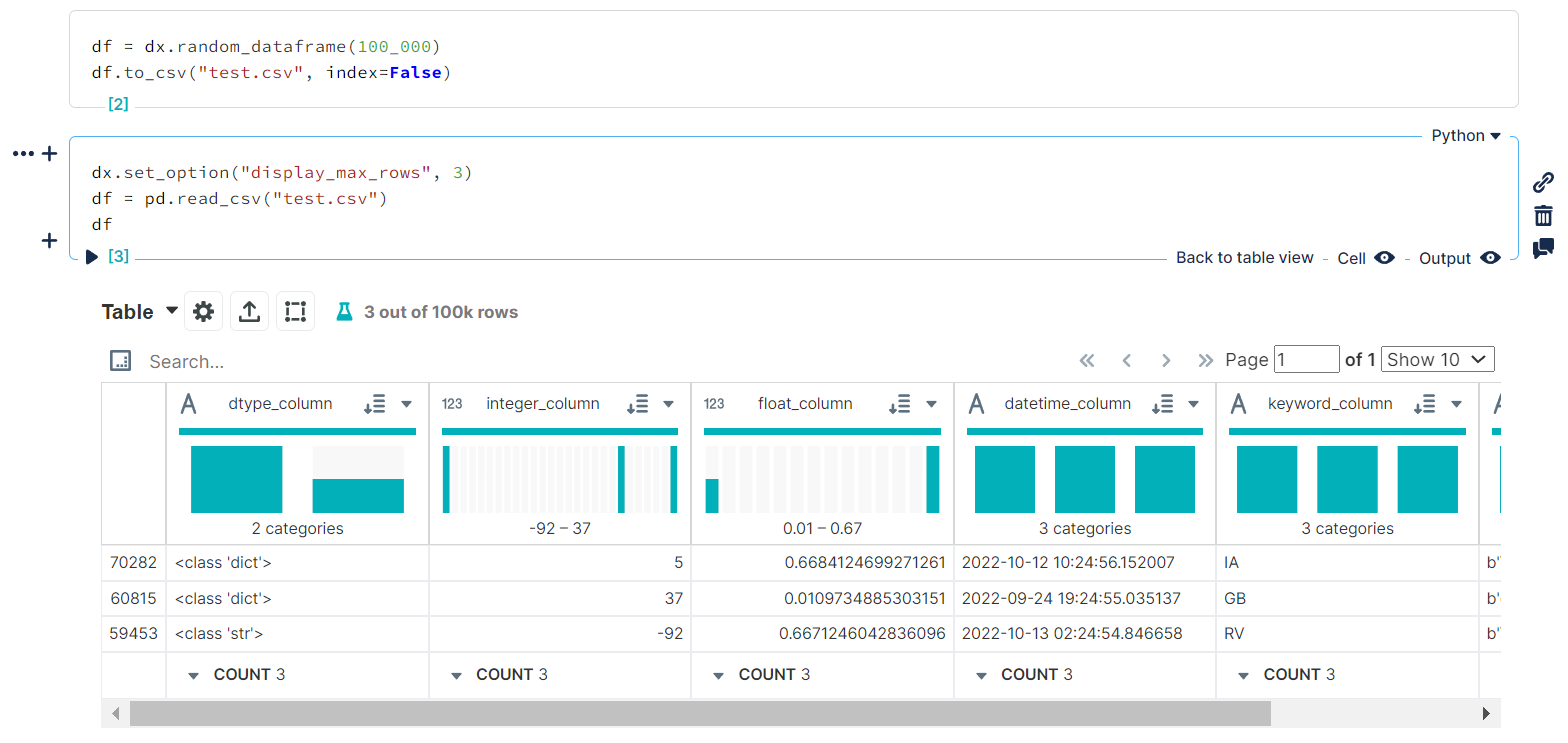
_Setting `DISPLAY_MAX_ROWS` to `3` for the current session_
...or with the `dx.settings_context()` context manager:

_Setting `DISPLAY_MAX_ROWS` to `3` within the current context, leaving options for the rest of the session alone_
### Generating Sample Data
Documentation coming soon!
### Usage Outside of Noteable
If using this package in a notebook environment outside of [Noteable](https://app.noteable.io/), the frontend should support the following media types:
- `application/vnd.dataresource+json` for `"simple"` display mode
- `application/vnd.dex.v1+json` for `"enhanced"` display mode
## Contributing
See [CONTRIBUTING.md](https://github.com/noteable-io/dx/blob/main/CONTRIBUTING.md).
## Code of Conduct
We follow the noteable.io [code of conduct](https://github.com/noteable-io/dx/blob/main/CODE_OF_CONDUCT.md).
## LICENSE
See [LICENSE.md](https://github.com/noteable-io/dx/blob/main/LICENSE.md).
-------
<p align="center">Open sourced with ❤️ by <a href="https://noteable.io">Noteable</a> for the community.</p>
<img href="https://pages.noteable.io/private-beta-access" src="https://assets.noteable.io/github/2022-07-29/noteable.png" alt="Boost Data Collaboration with Notebooks">
Raw data
{
"_id": null,
"home_page": "https://app.noteable.io/",
"name": "dx",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.8,<4.0",
"maintainer_email": "",
"keywords": "data,exploration,visualization",
"author": "Dave Shoup",
"author_email": "dave.shoup@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/d2/88/7bd2e955b475d0e15886150fd312553352f4e39ec2c45b1c724acf0f811e/dx-1.3.0.tar.gz",
"platform": null,
"description": "# dx\n\n<p align=\"center\">\nThis package provides convenient formatting and IPython display formatter registration for tabular data and DEX media types.\n</p>\n<p align=\"center\">\n<a href=\"https://github.com/noteable-io/dx/actions/workflows/ci.yaml\">\n <img src=\"https://github.com/noteable-io/dx/actions/workflows/ci.yaml/badge.svg\" alt=\"CI\" />\n</a>\n<a href=\"https://codecov.io/gh/noteable-io/dx\" > \n <img src=\"https://codecov.io/gh/noteable-io/dx/branch/main/graph/badge.svg?token=XGXSTD3GSI\" alt=\"codecov code coverage\"/> \n </a>\n<img alt=\"PyPI - License\" src=\"https://img.shields.io/pypi/l/dx\" />\n<img alt=\"PyPI - Python Version\" src=\"https://img.shields.io/pypi/pyversions/dx\" />\n<img alt=\"PyPI\" src=\"https://img.shields.io/pypi/v/dx\">\n<a href=\"https://github.com/psf/black\"><img alt=\"Code style: black\" src=\"https://img.shields.io/badge/code%20style-black-000000.svg\"></a>\n</p>\n\n---------\n\nA Pythonic Data Explorer, open sourced with \u2764\ufe0f by <a href=\"https://noteable.io\">Noteable</a>, a collaborative notebook platform that enables teams to use and visualize data, together.\n\n\n## Requirements\n\nPython 3.8+\n\n## Installation\n\n### Poetry\n\n```shell\npoetry add dx\n```\n\nThen import the package:\n\n```python\nimport dx\n```\n\n### Pip\n```shell\npip install dx\n```\n\nThen import the package:\n\n```python\nimport dx\n```\n\n## Usage\n\nThe `dx` library currently enables DEX media type visualization of pandas `DataFrame` and `Series` objects, as well as numpy `ndarray` objects. This can be handled in two ways:\n- explicit `dx.display()` calls\n- setting the `display_mode` to update the IPython display formatter for a session\n\n### With `dx.display()`\n`dx.display()` will display a single dataset using the DEX media type. It currently supports:\n- pandas `DataFrame` objects\n ```python\n import pandas as pd\n import random\n\n df = pd.DataFrame({\n 'random_ints': [random.randint(0, 100) for _ in range(500)],\n 'random_floats': [random.random() for _ in range(500)],\n })\n dx.display(df)\n ```\n \n\n- tabular data as `dict` or `list` types\n ```python\n dx.display([\n [1, 5, 10, 20, 500],\n [1, 2, 3, 4, 5],\n [0, 0, 0, 0, 1]\n ])\n ```\n \n\n- `.csv` or `.json` filepaths \n ```python\n df = dx.random_dataframe()\n df.to_csv(\"dx_docs_sample.csv\", index=False)\n\n dx.display(\"dx_docs_sample.csv\")\n ```\n 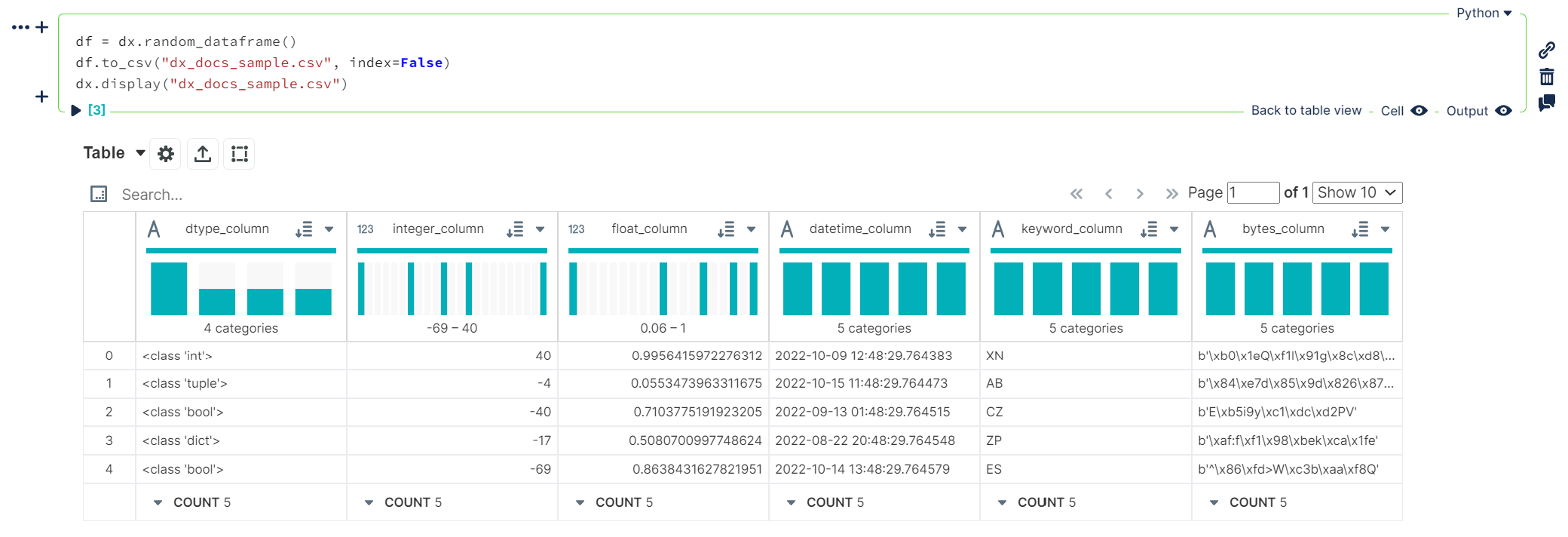\n\n### With `dx.set_display_mode()`\nUsing either `\"simple\"` or `\"enhanced\"` display modes will allow `dx` will update the current `IPython` display formatters to allow DEX media type visualization of pandas `DataFrame` objects for an entire notebook / kernel session instead of the default `DataFrame` display output.\n\n<details>\n<summary>Details</summary>\n\nThis will adjust pandas options to:\n- increasing the number of rows displayed to `50000` from pandas default of `60`\n- increasing the number of columns displayed to `50` from pandas default of `20`\n- enabling `html.table_schema` (`False` by default in pandas)\n\nThis will also handle some basic column cleaning and generate a schema for the `DataFrame` using `pandas.io.json.build_table_schema`. Depending on the display mode, the data will be transformed into either a list of dictionaries or list of lists of columnar values.\n- `\"simple\"` - list of dictionaries\n- `\"enhanced\"` - list of lists\n</details>\n\n> **NOTE:**\n> Unlike `dx.display()`, this **only** affects pandas DataFrames (or any types set in `settings.RENDERABLE_TYPES`); it does not affect the display of `.csv`/`.json` file data, or `dict`/`list` outputs\n\n\n- `dx.set_display_mode(\"simple\")`\n \n ```python\n import dx\n import numpy as np\n import pandas as pd\n\n # enable DEX display outputs from now on\n dx.set_display_mode(\"simple\")\n\n df = pd.read_csv(\"dx_docs_sample.csv\")\n df\n ```\n ```python\n df2 = pd.DataFrame(\n [\n [1, 5, 10, 20, 500],\n [1, 2, 3, np.nan, 5],\n [0, 0, 0, np.nan, 1]\n ],\n columns=['a', 'b', 'c', 'd', 'e']\n )\n df2\n ```\n \n\nIf, at any point, you want to go back to the default display formatting (vanilla pandas output), use the `\"plain\"` display mode. This will revert the IPython display format update to its original state and put the pandas options back to their default values.\n\n- `dx.set_display_mode(\"plain\")`\n ```python\n # revert to original pandas display outputs from now on\n dx.set_display_mode(\"plain\")\n\n df = pd.read_csv(\"dx_docs_sample.csv\")\n df\n ```\n ```python\n df2 = pd.DataFrame(\n [\n [1, 5, 10, 20, 500],\n [1, 2, 3, np.nan, 5],\n [0, 0, 0, np.nan, 1]\n ],\n columns=['a', 'b', 'c', 'd', 'e']\n )\n df2\n ```\n \n\n\n### Custom Settings\nDefault settings for `dx` can be found by calling `dx.settings`:\n\n\nEach can be set using `dx.set_option()`:\n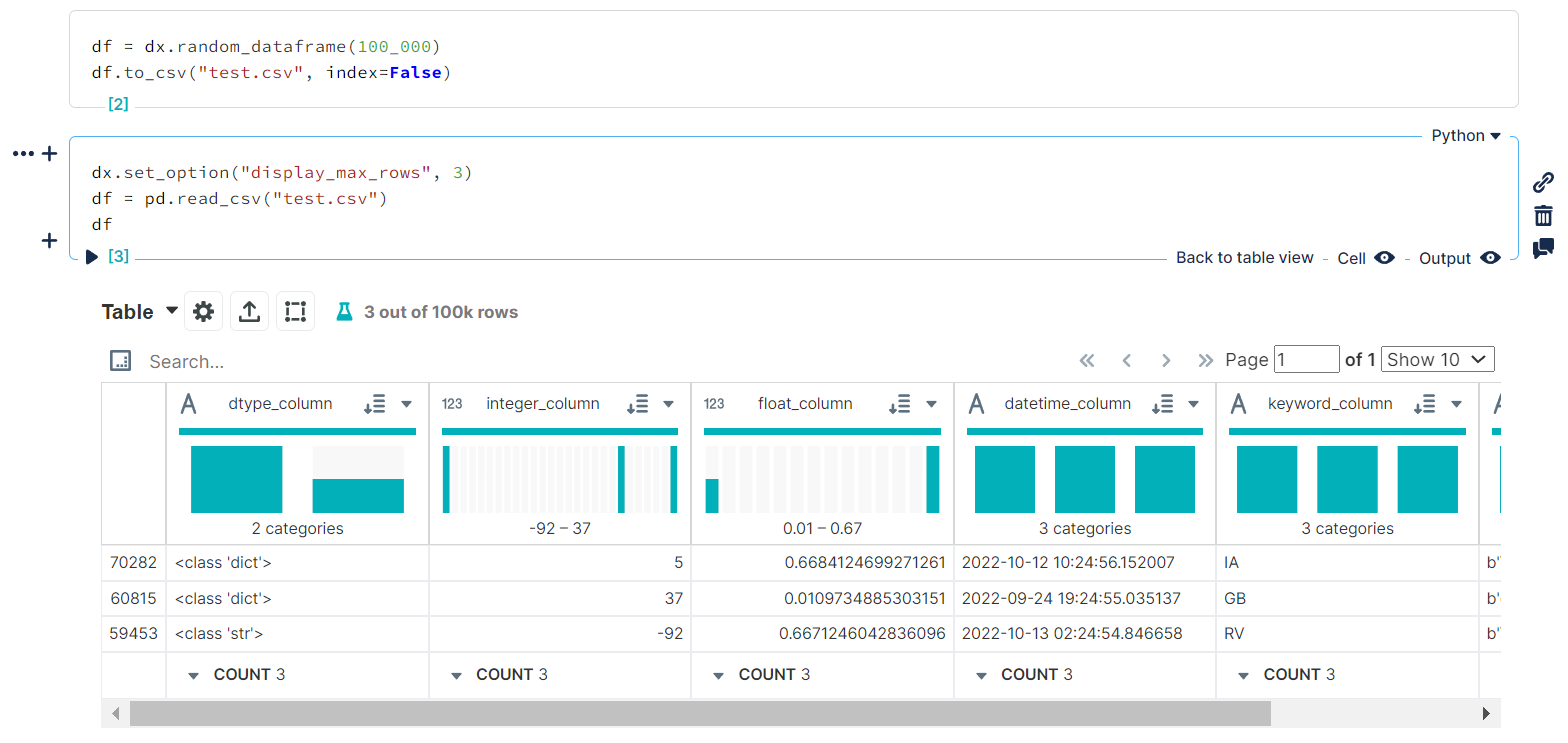\n_Setting `DISPLAY_MAX_ROWS` to `3` for the current session_\n\n...or with the `dx.settings_context()` context manager:\n\n_Setting `DISPLAY_MAX_ROWS` to `3` within the current context, leaving options for the rest of the session alone_\n\n### Generating Sample Data\nDocumentation coming soon!\n\n### Usage Outside of Noteable \nIf using this package in a notebook environment outside of [Noteable](https://app.noteable.io/), the frontend should support the following media types:\n- `application/vnd.dataresource+json` for `\"simple\"` display mode\n- `application/vnd.dex.v1+json` for `\"enhanced\"` display mode\n## Contributing\n\nSee [CONTRIBUTING.md](https://github.com/noteable-io/dx/blob/main/CONTRIBUTING.md).\n\n## Code of Conduct\n\nWe follow the noteable.io [code of conduct](https://github.com/noteable-io/dx/blob/main/CODE_OF_CONDUCT.md).\n\n## LICENSE\n\nSee [LICENSE.md](https://github.com/noteable-io/dx/blob/main/LICENSE.md).\n\n-------\n\n<p align=\"center\">Open sourced with \u2764\ufe0f by <a href=\"https://noteable.io\">Noteable</a> for the community.</p>\n\n<img href=\"https://pages.noteable.io/private-beta-access\" src=\"https://assets.noteable.io/github/2022-07-29/noteable.png\" alt=\"Boost Data Collaboration with Notebooks\">",
"bugtrack_url": null,
"license": "MIT",
"summary": "Python wrapper for Data Explorer",
"version": "1.3.0",
"split_keywords": [
"data",
"exploration",
"visualization"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "eb8f3494fbb2b6ae3691ac28edba3030186c7d54159a8128ad2ae128126856ef",
"md5": "ef01db2379a514111018b806d9973688",
"sha256": "55b7c9c8381d24500afd013f80634f363dfc250a71853c313430ccc4af120e59"
},
"downloads": -1,
"filename": "dx-1.3.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "ef01db2379a514111018b806d9973688",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8,<4.0",
"size": 65807,
"upload_time": "2023-03-31T12:33:25",
"upload_time_iso_8601": "2023-03-31T12:33:25.603810Z",
"url": "https://files.pythonhosted.org/packages/eb/8f/3494fbb2b6ae3691ac28edba3030186c7d54159a8128ad2ae128126856ef/dx-1.3.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d2887bd2e955b475d0e15886150fd312553352f4e39ec2c45b1c724acf0f811e",
"md5": "ad9a643295eb78faea2a55a82ac7952a",
"sha256": "8d8c7f7eac20569f031d00f37eed9dc712cd96cae57a4f09b67c5d11b36d598a"
},
"downloads": -1,
"filename": "dx-1.3.0.tar.gz",
"has_sig": false,
"md5_digest": "ad9a643295eb78faea2a55a82ac7952a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8,<4.0",
"size": 51369,
"upload_time": "2023-03-31T12:33:27",
"upload_time_iso_8601": "2023-03-31T12:33:27.390086Z",
"url": "https://files.pythonhosted.org/packages/d2/88/7bd2e955b475d0e15886150fd312553352f4e39ec2c45b1c724acf0f811e/dx-1.3.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-03-31 12:33:27",
"github": false,
"gitlab": false,
"bitbucket": false,
"lcname": "dx"
}