<!-- Header -->
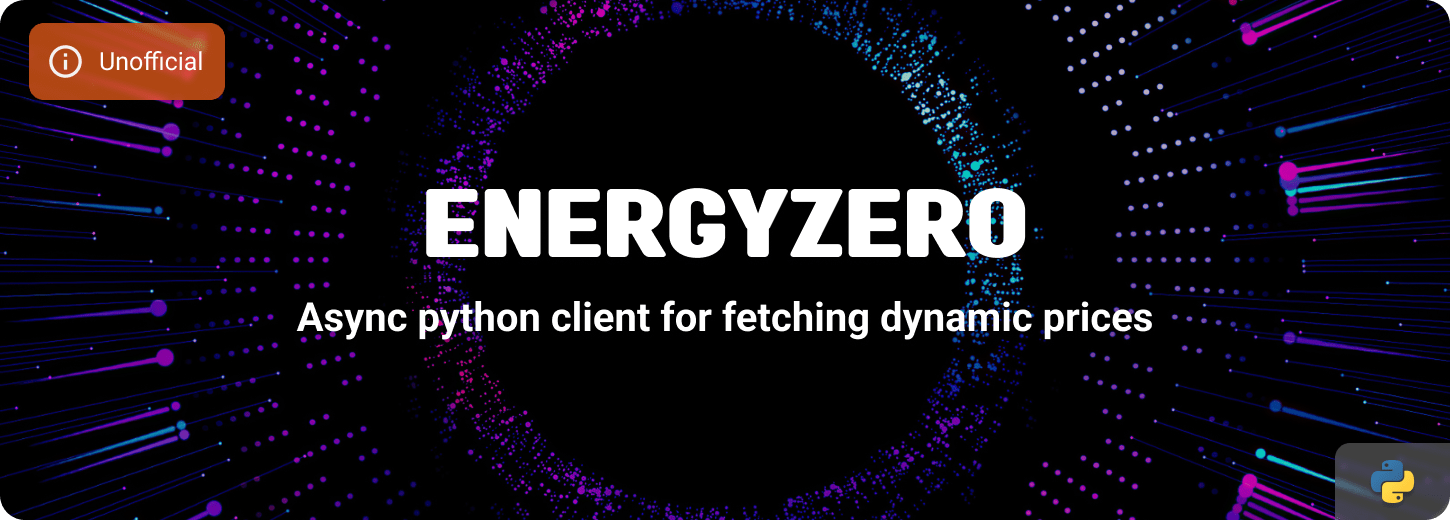
<!-- PROJECT SHIELDS -->
[![GitHub Release][releases-shield]][releases]
[![Python Versions][python-versions-shield]][pypi]
![Project Stage][project-stage-shield]
![Project Maintenance][maintenance-shield]
[![License][license-shield]](LICENSE)
[![GitHub Activity][commits-shield]][commits-url]
[![PyPi Downloads][downloads-shield]][downloads-url]
[![GitHub Last Commit][last-commit-shield]][commits-url]
[![Open in Dev Containers][devcontainer-shield]][devcontainer]
[![Code Quality][code-quality-shield]][code-quality]
[![Build Status][build-shield]][build-url]
[![Typing Status][typing-shield]][typing-url]
[![Maintainability][maintainability-shield]][maintainability-url]
[![Code Coverage][codecov-shield]][codecov-url]
Asynchronous Python client for the EnergyZero API.
## About
A python package with which you can retrieve the dynamic energy/gas prices from [EnergyZero][energyzero] and can therefore also be used for third parties who purchase their energy via EnergyZero, such as:
- [ANWB Energie](https://www.anwb.nl/huis/energie/anwb-energie)
- [Energie van Ons](https://www.energie.vanons.org)
- [GroeneStroomLokaal](https://www.groenestroomlokaal.nl)
- [Mijndomein Energie](https://www.mijndomein.nl/energie)
- [SamSam](https://www.samsam.nu)
- [ZonderGas](https://www.zondergas.nu)
## Installation
```bash
pip install energyzero
```
## Data
**note**: Currently only tested for day/tomorrow prices
You can read the following datasets with this package:
### Electricity prices
The energy prices are different every hour, after 15:00 (more usually already at 14:00) the prices for the next day are published and it is therefore possible to retrieve these data.
- Current/Next[x] hour electricity market price (float)
- Average electricity price (float)
- Lowest energy price (float)
- Highest energy price (float)
- Time of highest price (datetime)
- Time of lowest price (datetime)
- Percentage of the current price compared to the maximum price
- Number of hours with the current price or lower (int)
### Gas prices
The gas prices do not change per hour, but are fixed for 24 hours. Which means that from 06:00 in the morning the new rate for that day will be used.
- Current/Next[x] hour gas market price (float)
- Average gas price (float)
- Lowest gas price (float)
- Highest gas price (float)
## Example
```python
import asyncio
from datetime import date
from energyzero import EnergyZero, VatOption
async def main() -> None:
"""Show example on fetching the energy prices from EnergyZero."""
async with EnergyZero(vat=VatOption.INCLUDE) as client:
start_date = date(2022, 12, 7)
end_date = date(2022, 12, 7)
energy = await client.energy_prices(start_date, end_date)
gas = await client.gas_prices(start_date, end_date)
if __name__ == "__main__":
asyncio.run(main())
```
### Class Parameters
| Parameter | value Type | Description |
| :-------- | :--------- | :---------- |
| `vat` | enum (default: **VatOption.INCLUDE**) | Include or exclude VAT on class level |
### Function Parameters
| Parameter | value Type | Description |
| :-------- | :--------- | :---------- |
| `start_date` | datetime | The start date of the selected period |
| `end_date` | datetime | The end date of the selected period |
| `interval` | integer (default: **4**) | The interval of data return (**day**, **week**, **month**, **year**) |
| `vat` | enum (default: class value) | Include or exclude VAT (**VatOption.INCLUDE** or **VatOption.EXCLUDE**) |
**Interval**
4: Dag
5: Maand
6: Jaar
9: Week
## Contributing
This is an active open-source project. We are always open to people who want to
use the code or contribute to it.
We've set up a separate document for our
[contribution guidelines](CONTRIBUTING.md).
Thank you for being involved! :heart_eyes:
## Setting up development environment
The simplest way to begin is by utilizing the [Dev Container][devcontainer]
feature of Visual Studio Code or by opening a CodeSpace directly on GitHub.
By clicking the button below you immediately start a Dev Container in Visual Studio Code.
[![Open in Dev Containers][devcontainer-shield]][devcontainer]
This Python project relies on [Poetry][poetry] as its dependency manager,
providing comprehensive management and control over project dependencies.
You need at least:
- Python 3.11+
- [Poetry][poetry-install]
Install all packages, including all development requirements:
```bash
poetry install
```
Poetry creates by default an virtual environment where it installs all
necessary pip packages, to enter or exit the venv run the following commands:
```bash
poetry shell
exit
```
Setup the pre-commit check, you must run this inside the virtual environment:
```bash
pre-commit install
```
*Now you're all set to get started!*
As this repository uses the [pre-commit][pre-commit] framework, all changes
are linted and tested with each commit. You can run all checks and tests
manually, using the following command:
```bash
poetry run pre-commit run --all-files
```
To run just the Python tests:
```bash
poetry run pytest
```
To update the [syrupy](https://github.com/tophat/syrupy) snapshot tests:
```bash
poetry run pytest --snapshot-update
```
## License
MIT License
Copyright (c) 2022-2024 Klaas Schoute
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all
copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
SOFTWARE.
[energyzero]: https://www.energyzero.nl
<!-- MARKDOWN LINKS & IMAGES -->
[build-shield]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/tests.yaml/badge.svg
[build-url]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/tests.yaml
[code-quality-shield]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/codeql.yaml/badge.svg
[code-quality]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/codeql.yaml
[commits-shield]: https://img.shields.io/github/commit-activity/y/klaasnicolaas/python-energyzero.svg
[commits-url]: https://github.com/klaasnicolaas/python-energyzero/commits/main
[codecov-shield]: https://codecov.io/gh/klaasnicolaas/python-energyzero/branch/main/graph/badge.svg?token=29Y5JL4356
[codecov-url]: https://codecov.io/gh/klaasnicolaas/python-energyzero
[devcontainer-shield]: https://img.shields.io/static/v1?label=Dev%20Containers&message=Open&color=blue&logo=visualstudiocode
[devcontainer]: https://vscode.dev/redirect?url=vscode://ms-vscode-remote.remote-containers/cloneInVolume?url=https://github.com/klaasnicolaas/python-energyzero
[downloads-shield]: https://img.shields.io/pypi/dm/energyzero
[downloads-url]: https://pypistats.org/packages/energyzero
[license-shield]: https://img.shields.io/github/license/klaasnicolaas/python-energyzero.svg
[last-commit-shield]: https://img.shields.io/github/last-commit/klaasnicolaas/python-energyzero.svg
[maintenance-shield]: https://img.shields.io/maintenance/yes/2024.svg
[maintainability-shield]: https://api.codeclimate.com/v1/badges/615e7a78f1a6191d4731/maintainability
[maintainability-url]: https://codeclimate.com/github/klaasnicolaas/python-energyzero/maintainability
[project-stage-shield]: https://img.shields.io/badge/project%20stage-production%20ready-brightgreen.svg
[pypi]: https://pypi.org/project/energyzero/
[python-versions-shield]: https://img.shields.io/pypi/pyversions/energyzero
[typing-shield]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/typing.yaml/badge.svg
[typing-url]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/typing.yaml
[releases-shield]: https://img.shields.io/github/release/klaasnicolaas/python-energyzero.svg
[releases]: https://github.com/klaasnicolaas/python-energyzero/releases
[poetry-install]: https://python-poetry.org/docs/#installation
[poetry]: https://python-poetry.org
[pre-commit]: https://pre-commit.com
Raw data
{
"_id": null,
"home_page": "https://github.com/klaasnicolaas/python-energyzero",
"name": "energyzero",
"maintainer": "Klaas Schoute",
"docs_url": null,
"requires_python": "<4.0,>=3.11",
"maintainer_email": "hello@student-techlife.com",
"keywords": "energy, energyzero, gas, prices, api, async, client",
"author": "Klaas Schoute",
"author_email": "hello@student-techlife.com",
"download_url": "https://files.pythonhosted.org/packages/05/d6/18b09cb965df3fe1950ca28760c281bdb279610c292c10129ce94c9a8cd4/energyzero-2.1.1.tar.gz",
"platform": null,
"description": "<!-- Header -->\n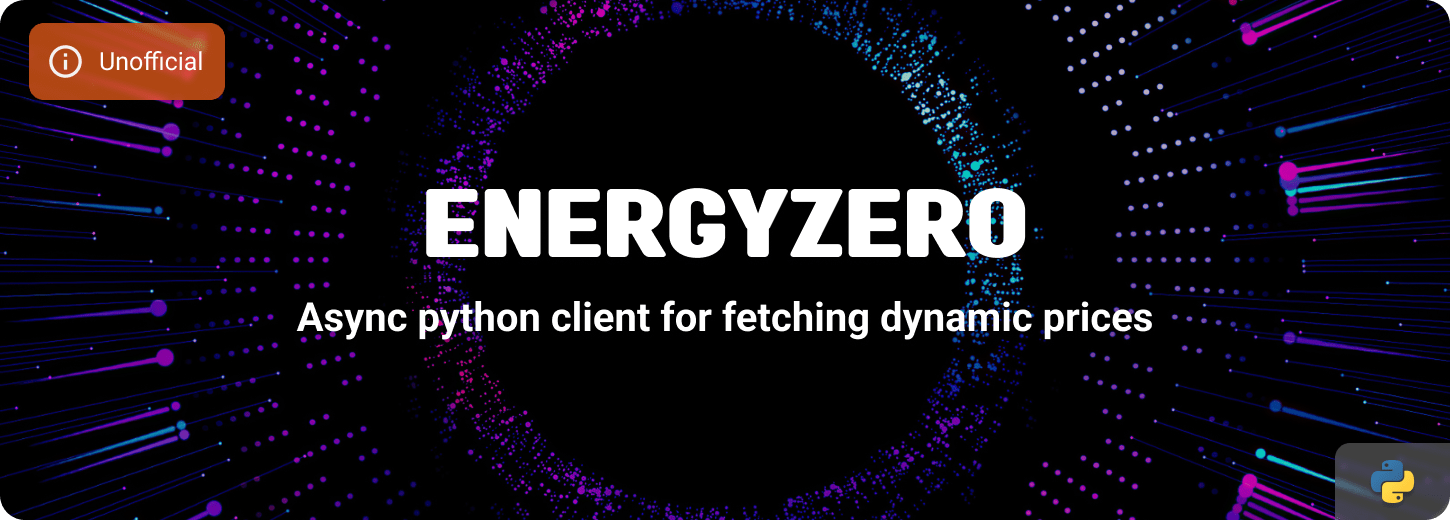\n\n<!-- PROJECT SHIELDS -->\n[![GitHub Release][releases-shield]][releases]\n[![Python Versions][python-versions-shield]][pypi]\n![Project Stage][project-stage-shield]\n![Project Maintenance][maintenance-shield]\n[![License][license-shield]](LICENSE)\n\n[![GitHub Activity][commits-shield]][commits-url]\n[![PyPi Downloads][downloads-shield]][downloads-url]\n[![GitHub Last Commit][last-commit-shield]][commits-url]\n[![Open in Dev Containers][devcontainer-shield]][devcontainer]\n\n[![Code Quality][code-quality-shield]][code-quality]\n[![Build Status][build-shield]][build-url]\n[![Typing Status][typing-shield]][typing-url]\n\n[![Maintainability][maintainability-shield]][maintainability-url]\n[![Code Coverage][codecov-shield]][codecov-url]\n\nAsynchronous Python client for the EnergyZero API.\n\n## About\n\nA python package with which you can retrieve the dynamic energy/gas prices from [EnergyZero][energyzero] and can therefore also be used for third parties who purchase their energy via EnergyZero, such as:\n\n- [ANWB Energie](https://www.anwb.nl/huis/energie/anwb-energie)\n- [Energie van Ons](https://www.energie.vanons.org)\n- [GroeneStroomLokaal](https://www.groenestroomlokaal.nl)\n- [Mijndomein Energie](https://www.mijndomein.nl/energie)\n- [SamSam](https://www.samsam.nu)\n- [ZonderGas](https://www.zondergas.nu)\n\n## Installation\n\n```bash\npip install energyzero\n```\n\n## Data\n\n**note**: Currently only tested for day/tomorrow prices\n\nYou can read the following datasets with this package:\n\n### Electricity prices\n\nThe energy prices are different every hour, after 15:00 (more usually already at 14:00) the prices for the next day are published and it is therefore possible to retrieve these data.\n\n- Current/Next[x] hour electricity market price (float)\n- Average electricity price (float)\n- Lowest energy price (float)\n- Highest energy price (float)\n- Time of highest price (datetime)\n- Time of lowest price (datetime)\n- Percentage of the current price compared to the maximum price\n- Number of hours with the current price or lower (int)\n\n### Gas prices\n\nThe gas prices do not change per hour, but are fixed for 24 hours. Which means that from 06:00 in the morning the new rate for that day will be used.\n\n- Current/Next[x] hour gas market price (float)\n- Average gas price (float)\n- Lowest gas price (float)\n- Highest gas price (float)\n\n## Example\n\n```python\nimport asyncio\n\nfrom datetime import date\nfrom energyzero import EnergyZero, VatOption\n\n\nasync def main() -> None:\n \"\"\"Show example on fetching the energy prices from EnergyZero.\"\"\"\n async with EnergyZero(vat=VatOption.INCLUDE) as client:\n start_date = date(2022, 12, 7)\n end_date = date(2022, 12, 7)\n\n energy = await client.energy_prices(start_date, end_date)\n gas = await client.gas_prices(start_date, end_date)\n\n\nif __name__ == \"__main__\":\n asyncio.run(main())\n```\n\n### Class Parameters\n\n| Parameter | value Type | Description |\n| :-------- | :--------- | :---------- |\n| `vat` | enum (default: **VatOption.INCLUDE**) | Include or exclude VAT on class level |\n\n### Function Parameters\n\n| Parameter | value Type | Description |\n| :-------- | :--------- | :---------- |\n| `start_date` | datetime | The start date of the selected period |\n| `end_date` | datetime | The end date of the selected period |\n| `interval` | integer (default: **4**) | The interval of data return (**day**, **week**, **month**, **year**) |\n| `vat` | enum (default: class value) | Include or exclude VAT (**VatOption.INCLUDE** or **VatOption.EXCLUDE**) |\n\n**Interval**\n4: Dag\n5: Maand\n6: Jaar\n9: Week\n\n## Contributing\n\nThis is an active open-source project. We are always open to people who want to\nuse the code or contribute to it.\n\nWe've set up a separate document for our\n[contribution guidelines](CONTRIBUTING.md).\n\nThank you for being involved! :heart_eyes:\n\n## Setting up development environment\n\nThe simplest way to begin is by utilizing the [Dev Container][devcontainer]\nfeature of Visual Studio Code or by opening a CodeSpace directly on GitHub.\nBy clicking the button below you immediately start a Dev Container in Visual Studio Code.\n\n[![Open in Dev Containers][devcontainer-shield]][devcontainer]\n\nThis Python project relies on [Poetry][poetry] as its dependency manager,\nproviding comprehensive management and control over project dependencies.\n\nYou need at least:\n\n- Python 3.11+\n- [Poetry][poetry-install]\n\nInstall all packages, including all development requirements:\n\n```bash\npoetry install\n```\n\nPoetry creates by default an virtual environment where it installs all\nnecessary pip packages, to enter or exit the venv run the following commands:\n\n```bash\npoetry shell\nexit\n```\n\nSetup the pre-commit check, you must run this inside the virtual environment:\n\n```bash\npre-commit install\n```\n\n*Now you're all set to get started!*\n\nAs this repository uses the [pre-commit][pre-commit] framework, all changes\nare linted and tested with each commit. You can run all checks and tests\nmanually, using the following command:\n\n```bash\npoetry run pre-commit run --all-files\n```\n\nTo run just the Python tests:\n\n```bash\npoetry run pytest\n```\n\nTo update the [syrupy](https://github.com/tophat/syrupy) snapshot tests:\n\n```bash\npoetry run pytest --snapshot-update\n```\n\n## License\n\nMIT License\n\nCopyright (c) 2022-2024 Klaas Schoute\n\nPermission is hereby granted, free of charge, to any person obtaining a copy\nof this software and associated documentation files (the \"Software\"), to deal\nin the Software without restriction, including without limitation the rights\nto use, copy, modify, merge, publish, distribute, sublicense, and/or sell\ncopies of the Software, and to permit persons to whom the Software is\nfurnished to do so, subject to the following conditions:\n\nThe above copyright notice and this permission notice shall be included in all\ncopies or substantial portions of the Software.\n\nTHE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR\nIMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,\nFITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE\nAUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER\nLIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,\nOUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE\nSOFTWARE.\n\n[energyzero]: https://www.energyzero.nl\n\n<!-- MARKDOWN LINKS & IMAGES -->\n[build-shield]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/tests.yaml/badge.svg\n[build-url]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/tests.yaml\n[code-quality-shield]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/codeql.yaml/badge.svg\n[code-quality]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/codeql.yaml\n[commits-shield]: https://img.shields.io/github/commit-activity/y/klaasnicolaas/python-energyzero.svg\n[commits-url]: https://github.com/klaasnicolaas/python-energyzero/commits/main\n[codecov-shield]: https://codecov.io/gh/klaasnicolaas/python-energyzero/branch/main/graph/badge.svg?token=29Y5JL4356\n[codecov-url]: https://codecov.io/gh/klaasnicolaas/python-energyzero\n[devcontainer-shield]: https://img.shields.io/static/v1?label=Dev%20Containers&message=Open&color=blue&logo=visualstudiocode\n[devcontainer]: https://vscode.dev/redirect?url=vscode://ms-vscode-remote.remote-containers/cloneInVolume?url=https://github.com/klaasnicolaas/python-energyzero\n[downloads-shield]: https://img.shields.io/pypi/dm/energyzero\n[downloads-url]: https://pypistats.org/packages/energyzero\n[license-shield]: https://img.shields.io/github/license/klaasnicolaas/python-energyzero.svg\n[last-commit-shield]: https://img.shields.io/github/last-commit/klaasnicolaas/python-energyzero.svg\n[maintenance-shield]: https://img.shields.io/maintenance/yes/2024.svg\n[maintainability-shield]: https://api.codeclimate.com/v1/badges/615e7a78f1a6191d4731/maintainability\n[maintainability-url]: https://codeclimate.com/github/klaasnicolaas/python-energyzero/maintainability\n[project-stage-shield]: https://img.shields.io/badge/project%20stage-production%20ready-brightgreen.svg\n[pypi]: https://pypi.org/project/energyzero/\n[python-versions-shield]: https://img.shields.io/pypi/pyversions/energyzero\n[typing-shield]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/typing.yaml/badge.svg\n[typing-url]: https://github.com/klaasnicolaas/python-energyzero/actions/workflows/typing.yaml\n[releases-shield]: https://img.shields.io/github/release/klaasnicolaas/python-energyzero.svg\n[releases]: https://github.com/klaasnicolaas/python-energyzero/releases\n\n[poetry-install]: https://python-poetry.org/docs/#installation\n[poetry]: https://python-poetry.org\n[pre-commit]: https://pre-commit.com\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Asynchronous Python client providing energy/gas prices from EnergyZero",
"version": "2.1.1",
"project_urls": {
"Bug Tracker": "https://github.com/klaasnicolaas/python-energyzero/issues",
"Changelog": "https://github.com/klaasnicolaas/python-energyzero/releases",
"Documentation": "https://github.com/klaasnicolaas/python-energyzero",
"Homepage": "https://github.com/klaasnicolaas/python-energyzero",
"Repository": "https://github.com/klaasnicolaas/python-energyzero"
},
"split_keywords": [
"energy",
" energyzero",
" gas",
" prices",
" api",
" async",
" client"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "aa2521529ffef995658b6018caff7676fbd1751c88545f402ebfd0e752c3560d",
"md5": "53b1d5b0abfbb2728fa6cef6094abeaf",
"sha256": "cd9207b2bb9ff80958b94007723b41923654f9f71afa0057cb03383a4d5d7659"
},
"downloads": -1,
"filename": "energyzero-2.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "53b1d5b0abfbb2728fa6cef6094abeaf",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.11",
"size": 10211,
"upload_time": "2024-06-28T19:45:47",
"upload_time_iso_8601": "2024-06-28T19:45:47.817485Z",
"url": "https://files.pythonhosted.org/packages/aa/25/21529ffef995658b6018caff7676fbd1751c88545f402ebfd0e752c3560d/energyzero-2.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "05d618b09cb965df3fe1950ca28760c281bdb279610c292c10129ce94c9a8cd4",
"md5": "858a51b177e22bf5fa8a6b8e49a34576",
"sha256": "9f7c8f330c3bed67a52e6112dbaa48b179d03d98f08fb26ad5c183bd7da54efd"
},
"downloads": -1,
"filename": "energyzero-2.1.1.tar.gz",
"has_sig": false,
"md5_digest": "858a51b177e22bf5fa8a6b8e49a34576",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.11",
"size": 12336,
"upload_time": "2024-06-28T19:45:49",
"upload_time_iso_8601": "2024-06-28T19:45:49.085080Z",
"url": "https://files.pythonhosted.org/packages/05/d6/18b09cb965df3fe1950ca28760c281bdb279610c292c10129ce94c9a8cd4/energyzero-2.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-06-28 19:45:49",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "klaasnicolaas",
"github_project": "python-energyzero",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "energyzero"
}