# Enka Network Python
EN | [TH](https://github.com/mrwan200/EnkaNetwork.py/blob/master/README_TH.md) | [JA](https://github.com/mrwan200/EnkaNetwork.py/blob/master/README_JA.md)
Library for API wrapper data from site https://enka.network/
# 🏓 Table of content
- [💾 Installation](#installation)
- [✨ Usage](#usage)
- [👀 Example](#example)
- [📗 Class Methods](#class-methods)
- [📥 Response data](#return-data)
- [UID](#uid)
- [Profile](#profile)
- [🚧 Structure](#structure)
- [Player owner](#player-owner)
- [Profile patreon](#profile-patreon)
- [Profile Hoyos](#profile-hoyos)
- [Build(s) info](#avatar-builds-info)
- [Profile info](#profile-info)
- [Player](#player)
- [Namecard](#namecard)
- [Avatar Icon](#avatar-icon)
- [Character preview](#character-preview)
- [Characters](#characters)
- [Icon](#icon)
- [Constellation](#constellation)
- [Skill](#skill)
- [Equipments (Artifact, Weapon)](#equipments-artifact-weapon)
- [Equipments Info](#equipments-info)
- [Equipments Stats](#equipments-stats)
- [FIGHT_PROP Data](#fight_prop-data)
- [Build(s)](#build)
- [🔧 Assets](#assets)
- [Character, constellations, skills, namecards](#assets-character-constellations-skills-namecards)
- [NameTextMapHash](#assets-nametextmaphash)
- [🌎 Languages Supported](#languages-supported)
- [🙋 Support & Question](#support--question)
- [📄 LICENSE](#license)
# Installation
```
pip install enkanetwork.py
```
# Usage
```py
import asyncio
from enkanetwork import EnkaNetworkAPI
client = EnkaNetworkAPI()
async def main():
async with client:
data = await client.fetch_user(843715177)
print("=== Player Info ===")
print(f"Nickname: {data.player.nickname}")
print(f"Level: {data.player.level}")
print(f"Icon: {data.player.avatar.icon.url}")
print(f"Signature: {data.player.signature}")
print(f"Achievement: {data.player.achievement}")
print(f"Abyss floor: {data.player.abyss_floor} - {data.player.abyss_room}")
print(f"Cache timeout: {data.ttl}")
asyncio.run(main())
```
## Preview
```sh
=== Player Info ===
Nickname: mrwan2546
Level: 55
Icon: https://enka.network/ui/UI_AvatarIcon_Kazuha.png
Signature: K A Z U H A M U C H <3
Achievement: 396
Abyss floor: 8 - 3
Cache timeout: 300
```
## Example
Please refer in [example](./example/) folder.
# Class Methods
| Name | Description |
| ----------------------------------- | --------------------------------------------------------------------------------------------------- |
| fetch_user(uid) | Fetch user data (UID) **(Will be depercated soon)** |
| fetch_user_by_uid(uid) | Fetch user data (UID) |
| fetch_user_by_username(profile_id) | Fetch user data (Profile ID) **(For subscriptions in Enka.Network)** |
| fetch_hoyos_by_username(profile_id) | Fetch user hoyo(s) data (Profile ID) **(For subscriptions in Enka.Network)** |
| fetch_builds(profile_id, metaname) | Fetch build data (Profile ID) **(For subscriptions in Enka.Network)** |
| set_language(lang) | Set new language <br> Please refer [Languages Supported](#languages-supported) |
| update_assets() | Update new assets from repo [Enkanetwork.py Data](https://github.com/mrwan200/enkanetwork.py-data/) |
# Return data
## UID
Return type: `EnkaNetworkResponse`
| Wrapper | API | Notes |
| ---------- | -------------- | ------------------------------------------ |
| player | playerInfo | Please refer [Player](#player) |
| characters | avatarInfoList | Please refer [Characters](#characters) |
| profile | - | Please refer [Profile Info](#profile-info) |
| owner | owner | Please refer [Player Owner](#player-owner) |
| ttl | ttl | |
| uid | uid | |
## Profile
Return type: `EnkaNetworkProfileResponse`
| Wrapper | API | Notes |
| -------- | ---------- | --------------------------------------------------- |
| username | playerInfo | Please refer [Player](#player) |
| profile | profile | Please refer in [Profile patreon](#profile-patreon) |
| hoyos | hoyos | Please refer [Profile hoyos](#profile-hoyos) |
# Structure
## Player owner
| Wrapper | API | Notes |
| -------- | -------- | --------------------------------------------------- |
| hash | hash | |
| username | username | Please refer [Tier](#tier) |
| profile | profile | Please refer in [Profile patreon](#profile-patreon) |
| builds | - | Please refer [Build(s) info](#avatar-builds-info) |
## Profile Patreon
| Wrapper | API | Notes |
| ------------ | ------------ | -------------------------- |
| bio | bio | |
| level | level | Please refer [Tier](#tier) |
| profile | worldLevel | |
| signup_state | signup_state | |
| image_url | image_url | |
## Profile Hoyos
| Wrapper | API | Notes |
| ------------ | ------------ | ------------------------------------------------ |
| uid_public | uid_public | |
| public | public | |
| verified | verified | |
| player_info | player_info | Please refer [Profile Patreon](#profile-patreon) |
| signup_state | signup_state | |
| signup_state | signup_state | |
## Avatar build(s) info
| Wrapper | API | Notes |
| ----------- | ----------- | -------------------------------------- |
| id | id | |
| name | name | |
| avatar_id | avatar_id | |
| avatar_data | avatar_data | Please refer [Characters](#characters) |
| order | order | |
| live | live | |
| settings | settings | |
| public | public | |
## Profile Info
| Wrapper | API | Notes |
| ------- | --- | ------------------------------ |
| uid | - | UID in-game |
| url | - | URL to enter Enka.Network site |
| path | - | Path URL |
## Player
| Wrapper | API | Notes |
| ------------------ | ------------------------ | ---------------------------------------------------- |
| nickname | nickname | Please refer [Namecard](#namecard) |
| signature | signature | |
| world_level | worldLevel | |
| achievement | finishAchievementNum | |
| namecard | namecardId | |
| namecards | showNameCardIdList -> id | Please refer [Namecard](#namecard) |
| abyss_floor | towerFloorIndex | |
| abyss_room | towerLevelIndex | |
| characters_preview | showAvatarInfoList | Please refer [Character Preview](#character-preview) |
| avatar | profilePicture | Please refer [Avatar Icon](#avatar-icon) |
### Avatar icon
| Wrapper | API | Notes |
| ------- | -------- | ------------------------------------ |
| id | avatarId | |
| icon | | Please refer [Icon Data](#icon-data) |
### Namecard
| Wrapper | API | Notes |
| ------- | --- | ------------------------------------------------------------- |
| id | - | Namecard ID |
| name | - | Namecard name |
| icon | - | Namecard icon, Please refer [Icon Data](#icon-data) |
| banner | - | Namecard banner, Please refer [Icon Data](#icon-data) |
| navbar | - | Namecard navbar (Alpha), Please refer [Icon Data](#icon-data) |
### Character preview
| Wrapper | API | Notes |
| ------- | --- | ------------------------------------------------- |
| id | - | Avatar ID |
| name | - | Avatar Name |
| level | - | Avatar Level |
| icon | - | Avatar Icon, Please refer [Icon Data](#icon-data) |
## Characters
| Wrapper | API | Notes |
| ----------------------- | ---------------------- | ------------------------------------------------------ |
| id | avatarId | |
| name | - | Avatar Name |
| element | - | Please refer [Element Type](#element-type) |
| rarity | - | Rarity |
| image | - | Please refer [Icon](#icon) |
| xp | propMap -> 1001 | |
| ascension | propMap -> 1002 | |
| level | propMap -> 4001 | |
| max_level | - | Avatar max level (Like 50/60) |
| friendship_level | fetterInfo.level | |
| equipments | equipList | Please refer [Equipments](#equipments-artifact-weapon) |
| stats | fightPropMap | Please refer [FIGHT_PROP Data](#fight_prop-data) |
| constellations | talentIdList | Please refer [Constellation](#constellation) |
| constellations_unlocked | - | Constellation unlocked |
| skill_data | inherentProudSkillList | |
| skill_id | skillDepotId | |
| skills | - | Please refer [Skill](#skill) |
### Icon
| Wrapper | API | Notes |
| ------- | --- | -------------------------------------------------------- |
| icon | - | Avatar icon, Please refer [Icon Data](#icon-data) |
| side | - | Avatar side icon, Please refer [Icon Data](#icon-data) |
| banner | - | Avatar wish banner, Please refer [Icon Data](#icon-data) |
### Constellation
| Wrapper | API | Notes |
| -------- | --- | -------------------------- |
| id | - | Constellation ID |
| name | - | Constellation Name |
| icon | - | Constellation Icon (URL) |
| unlocked | - | Constellation has unlocked |
### Skill
| Wrapper | API | Notes |
| ---------- | --- | ----------------------- |
| id | - | Skill ID |
| name | - | Skill Name |
| icon | - | Skill Icon (URL) |
| level | - | Skill Level |
| is_boosted | - | Skill level has boosted |
## Equipments (Artifact, Weapon)
| Wrapper | API | Notes |
| ---------- | ----------------------------------- | ------------------------------------------------ |
| id | itemId | |
| level | reliquary -> level, weapon -> level |
| type | - | Type of equipment (Artifact or Weapon) |
| refinement | weapon -> affixMap | |
| ascension | weapon -> promoteLevel | |
| detail | flat | Please refer [Equipments Info](#equipments-info) |
### Equipments Info
| Wrapper | API | Notes |
| ------------- | ----------------------------------- | -------------------------------------------------- |
| name | - | Equipment Name (Artifact name or Weapon name) |
| icon | icon | Please refer [Icon Data](#icon-data) |
| artifact_type | - | Please refer [Artifact Type](#artifact-type) |
| rarity | rankLevel | |
| mainstats | reliquaryMainstat, weaponStats -> 0 | Please refer [Equipments Stats](#equipments-stats) |
| substats | reliquarySubstats, weaponStats -> 1 | Please refer [Equipments Stats](#equipments-stats) |
### Equipments Stats
| Wrapper | API | Notes |
| ------- | ------- | ------------------------------ |
| prop_id | prop_id | |
| type | - | Value type (NUMBER or PERCENT) |
| name | - | Name of FIGHT_PROP |
| value | value | |
## FIGHT_PROP Data
In FIGHT_PROP data. You can get the value from 4 methods.
| Choice | Example | Output |
|------------------|---------------------------|----------------------------|
| Get raw value | stats.FIGHT_PROP_HP.value | 15552.306640625 |
| Get rounded value| stats.FIGHT_PROP_ATTACK.to_rounded() | 344 |
| Get percentage | stats.FIGHT_PROP_FIRE_ADD_HURT.to_percentage() | 61.5 |
| Get percentage and symbol | stats.FIGHT_PROP_FIRE_ADD_HURT.to_percentage_symbol() | 61.5% |
## Build
In this `Builds` It's not pretty data. You can use this method to get data. Or if you want get full, You can use `raw` argument
| Choice | Example | Output |
|------------------|---------------------------|----------------------------|
| Get avatar ID list | builds.get_avatar_list() | [10000021,10000037,10000025, ...] |
| Get character build | builds.get_character(10000021) | List of [Build info](#avatar-builds-info) |
| Get build info by avatar id | builds.get_character(10000021, 11111111) | [Build info](#avatar-builds-info) |
# Icon Data
In icon data. You can get the value from 2 methods.
| Choice | Example | Output |
|------------------|---------------------------|--------------------------------|
| Get filename | icon.filename | UI_AvatarIcon_Kazuha_Card.png |
| Get URL | icon.url | https://enka.network/ui/UI_AvatarIcon_Kazuha_Card.png |
## Artifact Type
| Key | Value |
| ------- | -------------- |
| Flower | EQUIP_BRACER |
| Feather | EQUIP_NECKLACE |
| Sands | EQUIP_SHOES |
| Goblet | EQUIP_RING |
| Circlet | EQUIP_DRESS |
## Element Type
| Key | Value |
| ------- | -------- |
| Cryo | Ice |
| Hydro | Water |
| Anemo | Wind |
| Pyro | Fire |
| Geo | Rock |
| Electro | Electric |
# Assets
## Assets character, constellations, skills, namecards
You can use avatarId to get the character, constellations, skills, namecards from assets.
```py
import asyncio
from enkanetwork import Assets
assets = Assets()
async def main():
# Character
assets.character(10000046)
# Constellations
assets.constellations(2081199193)
# Skills
assets.constellations(10462)
# Namecards
assets.namecards(210059)
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
```
## Assets NameTextMapHash
The `NameTextMapHash` is a hash map that contains the name text of the assets. You can get `NameTextMapHash` from `hash_id` like this:
```py
import asyncio
from enkanetwork import Assets
assets = Assets(lang="en") # Set languege before get name (Ex. English)
async def main():
print(assets.get_hash_map(1940919994)) # Hu tao
# OR you can get FIGHT_PROP name
print(assets.get_hash_map("FIGHT_PROP_BASE_ATTACK")) # Base ATK
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
```
## Languages Supported
| Languege | Code |
| ---------- | ---- |
| English | en |
| русский | ru |
| Tiếng Việt | vi |
| ไทย | th |
| português | pt |
| 한국어 | kr |
| 日本語 | jp |
| 中文 | zh |
| Indonesian | id |
| français | fr |
| español | es |
| deutsch | de |
| Taiwan | cht |
| Chinese | chs |
If you want full docs for the API, visit [EnkaNetwork API Docs](https://github.com/EnkaNetwork/API-docs)
## Support & Question
If you need support or some question about EnkaNetwokt.py. You can feel free contact to me in [Enka.network discord server](https://discord.gg/G3m7CWkssY) in [𝖯𝖸┃enkanetwork․py](https://discord.com/channels/840335525621268520/1046281445049647104) channel and mention (Ping) to **@M-307** for support and help
# LICENSE
[MIT License](./LICENSE)
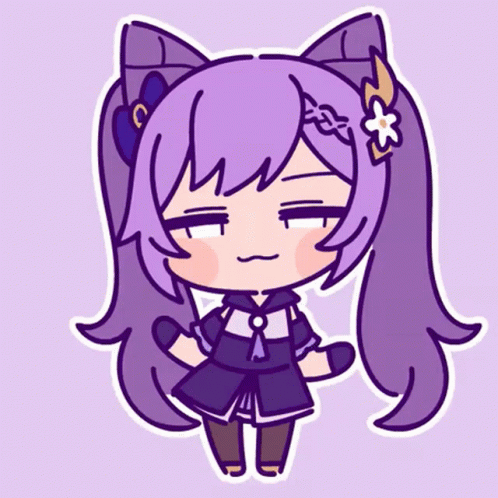
[Picture by KKOMDASTRO](https://twitter.com/KKOMDASTRO)
Raw data
{
"_id": null,
"home_page": "https://github.com/mrwan200/EnkaNetwork.py",
"name": "enkanetwork.py",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "enkanetwork.py,enkanetwork,enka.network,genshinapi",
"author": "M-307",
"author_email": "me@m307.dev",
"download_url": "https://files.pythonhosted.org/packages/84/fc/188d49959475ea255d5f1d26aad13647b81cde0cfa731f0ff6e3bca35a93/enkanetwork.py-1.4.5.tar.gz",
"platform": null,
"description": "# Enka Network Python\n\nEN | [TH](https://github.com/mrwan200/EnkaNetwork.py/blob/master/README_TH.md) | [JA](https://github.com/mrwan200/EnkaNetwork.py/blob/master/README_JA.md)\n\nLibrary for API wrapper data from site https://enka.network/\n\n# \ud83c\udfd3 Table of content\n\n- [\ud83d\udcbe Installation](#installation)\n- [\u2728 Usage](#usage)\n- [\ud83d\udc40 Example](#example)\n- [\ud83d\udcd7 Class Methods](#class-methods)\n- [\ud83d\udce5 Response data](#return-data)\n - [UID](#uid)\n - [Profile](#profile)\n- [\ud83d\udea7 Structure](#structure)\n - [Player owner](#player-owner)\n - [Profile patreon](#profile-patreon)\n - [Profile Hoyos](#profile-hoyos)\n - [Build(s) info](#avatar-builds-info)\n - [Profile info](#profile-info)\n - [Player](#player)\n - [Namecard](#namecard)\n - [Avatar Icon](#avatar-icon)\n - [Character preview](#character-preview)\n - [Characters](#characters)\n - [Icon](#icon)\n - [Constellation](#constellation)\n - [Skill](#skill)\n - [Equipments (Artifact, Weapon)](#equipments-artifact-weapon)\n - [Equipments Info](#equipments-info)\n - [Equipments Stats](#equipments-stats)\n - [FIGHT_PROP Data](#fight_prop-data)\n - [Build(s)](#build)\n- [\ud83d\udd27 Assets](#assets)\n - [Character, constellations, skills, namecards](#assets-character-constellations-skills-namecards)\n - [NameTextMapHash](#assets-nametextmaphash)\n- [\ud83c\udf0e Languages Supported](#languages-supported)\n- [\ud83d\ude4b Support & Question](#support--question)\n- [\ud83d\udcc4 LICENSE](#license)\n\n# Installation\n\n```\npip install enkanetwork.py\n```\n\n# Usage\n\n```py\nimport asyncio\n\nfrom enkanetwork import EnkaNetworkAPI\n\nclient = EnkaNetworkAPI()\n\nasync def main():\n async with client:\n data = await client.fetch_user(843715177)\n print(\"=== Player Info ===\")\n print(f\"Nickname: {data.player.nickname}\")\n print(f\"Level: {data.player.level}\")\n print(f\"Icon: {data.player.avatar.icon.url}\")\n print(f\"Signature: {data.player.signature}\")\n print(f\"Achievement: {data.player.achievement}\")\n print(f\"Abyss floor: {data.player.abyss_floor} - {data.player.abyss_room}\")\n print(f\"Cache timeout: {data.ttl}\")\n\nasyncio.run(main())\n```\n\n## Preview\n\n```sh\n=== Player Info ===\nNickname: mrwan2546\nLevel: 55\nIcon: https://enka.network/ui/UI_AvatarIcon_Kazuha.png\nSignature: K A Z U H A M U C H <3\nAchievement: 396\nAbyss floor: 8 - 3\nCache timeout: 300\n```\n\n## Example\n\nPlease refer in [example](./example/) folder.\n\n# Class Methods\n\n| Name | Description |\n| ----------------------------------- | --------------------------------------------------------------------------------------------------- |\n| fetch_user(uid) | Fetch user data (UID) **(Will be depercated soon)** |\n| fetch_user_by_uid(uid) | Fetch user data (UID) |\n| fetch_user_by_username(profile_id) | Fetch user data (Profile ID) **(For subscriptions in Enka.Network)** |\n| fetch_hoyos_by_username(profile_id) | Fetch user hoyo(s) data (Profile ID) **(For subscriptions in Enka.Network)** |\n| fetch_builds(profile_id, metaname) | Fetch build data (Profile ID) **(For subscriptions in Enka.Network)** |\n| set_language(lang) | Set new language <br> Please refer [Languages Supported](#languages-supported) |\n| update_assets() | Update new assets from repo [Enkanetwork.py Data](https://github.com/mrwan200/enkanetwork.py-data/) |\n\n# Return data\n\n## UID\n\nReturn type: `EnkaNetworkResponse`\n| Wrapper | API | Notes |\n| ---------- | -------------- | ------------------------------------------ |\n| player | playerInfo | Please refer [Player](#player) |\n| characters | avatarInfoList | Please refer [Characters](#characters) |\n| profile | - | Please refer [Profile Info](#profile-info) |\n| owner | owner | Please refer [Player Owner](#player-owner) |\n| ttl | ttl | |\n| uid | uid | |\n\n## Profile\n\nReturn type: `EnkaNetworkProfileResponse`\n| Wrapper | API | Notes |\n| -------- | ---------- | --------------------------------------------------- |\n| username | playerInfo | Please refer [Player](#player) |\n| profile | profile | Please refer in [Profile patreon](#profile-patreon) |\n| hoyos | hoyos | Please refer [Profile hoyos](#profile-hoyos) |\n\n# Structure\n\n## Player owner\n\n| Wrapper | API | Notes |\n| -------- | -------- | --------------------------------------------------- |\n| hash | hash | |\n| username | username | Please refer [Tier](#tier) |\n| profile | profile | Please refer in [Profile patreon](#profile-patreon) |\n| builds | - | Please refer [Build(s) info](#avatar-builds-info) |\n\n## Profile Patreon\n\n| Wrapper | API | Notes |\n| ------------ | ------------ | -------------------------- |\n| bio | bio | |\n| level | level | Please refer [Tier](#tier) |\n| profile | worldLevel | |\n| signup_state | signup_state | |\n| image_url | image_url | |\n\n## Profile Hoyos\n\n| Wrapper | API | Notes |\n| ------------ | ------------ | ------------------------------------------------ |\n| uid_public | uid_public | |\n| public | public | |\n| verified | verified | |\n| player_info | player_info | Please refer [Profile Patreon](#profile-patreon) |\n| signup_state | signup_state | |\n| signup_state | signup_state | |\n\n## Avatar build(s) info\n\n| Wrapper | API | Notes |\n| ----------- | ----------- | -------------------------------------- |\n| id | id | |\n| name | name | |\n| avatar_id | avatar_id | |\n| avatar_data | avatar_data | Please refer [Characters](#characters) |\n| order | order | |\n| live | live | |\n| settings | settings | |\n| public | public | |\n\n## Profile Info\n\n| Wrapper | API | Notes |\n| ------- | --- | ------------------------------ |\n| uid | - | UID in-game |\n| url | - | URL to enter Enka.Network site |\n| path | - | Path URL |\n\n## Player\n\n| Wrapper | API | Notes |\n| ------------------ | ------------------------ | ---------------------------------------------------- |\n| nickname | nickname | Please refer [Namecard](#namecard) |\n| signature | signature | |\n| world_level | worldLevel | |\n| achievement | finishAchievementNum | |\n| namecard | namecardId | |\n| namecards | showNameCardIdList -> id | Please refer [Namecard](#namecard) |\n| abyss_floor | towerFloorIndex | |\n| abyss_room | towerLevelIndex | |\n| characters_preview | showAvatarInfoList | Please refer [Character Preview](#character-preview) |\n| avatar | profilePicture | Please refer [Avatar Icon](#avatar-icon) |\n\n### Avatar icon\n\n| Wrapper | API | Notes |\n| ------- | -------- | ------------------------------------ |\n| id | avatarId | |\n| icon | | Please refer [Icon Data](#icon-data) |\n\n### Namecard\n\n| Wrapper | API | Notes |\n| ------- | --- | ------------------------------------------------------------- |\n| id | - | Namecard ID |\n| name | - | Namecard name |\n| icon | - | Namecard icon, Please refer [Icon Data](#icon-data) |\n| banner | - | Namecard banner, Please refer [Icon Data](#icon-data) |\n| navbar | - | Namecard navbar (Alpha), Please refer [Icon Data](#icon-data) |\n\n### Character preview\n\n| Wrapper | API | Notes |\n| ------- | --- | ------------------------------------------------- |\n| id | - | Avatar ID |\n| name | - | Avatar Name |\n| level | - | Avatar Level |\n| icon | - | Avatar Icon, Please refer [Icon Data](#icon-data) |\n\n## Characters\n\n| Wrapper | API | Notes |\n| ----------------------- | ---------------------- | ------------------------------------------------------ |\n| id | avatarId | |\n| name | - | Avatar Name |\n| element | - | Please refer [Element Type](#element-type) |\n| rarity | - | Rarity |\n| image | - | Please refer [Icon](#icon) |\n| xp | propMap -> 1001 | |\n| ascension | propMap -> 1002 | |\n| level | propMap -> 4001 | |\n| max_level | - | Avatar max level (Like 50/60) |\n| friendship_level | fetterInfo.level | |\n| equipments | equipList | Please refer [Equipments](#equipments-artifact-weapon) |\n| stats | fightPropMap | Please refer [FIGHT_PROP Data](#fight_prop-data) |\n| constellations | talentIdList | Please refer [Constellation](#constellation) |\n| constellations_unlocked | - | Constellation unlocked |\n| skill_data | inherentProudSkillList | |\n| skill_id | skillDepotId | |\n| skills | - | Please refer [Skill](#skill) |\n\n### Icon\n\n| Wrapper | API | Notes |\n| ------- | --- | -------------------------------------------------------- |\n| icon | - | Avatar icon, Please refer [Icon Data](#icon-data) |\n| side | - | Avatar side icon, Please refer [Icon Data](#icon-data) |\n| banner | - | Avatar wish banner, Please refer [Icon Data](#icon-data) |\n\n### Constellation\n\n| Wrapper | API | Notes |\n| -------- | --- | -------------------------- |\n| id | - | Constellation ID |\n| name | - | Constellation Name |\n| icon | - | Constellation Icon (URL) |\n| unlocked | - | Constellation has unlocked |\n\n### Skill\n\n| Wrapper | API | Notes |\n| ---------- | --- | ----------------------- |\n| id | - | Skill ID |\n| name | - | Skill Name |\n| icon | - | Skill Icon (URL) |\n| level | - | Skill Level |\n| is_boosted | - | Skill level has boosted |\n\n## Equipments (Artifact, Weapon)\n\n| Wrapper | API | Notes |\n| ---------- | ----------------------------------- | ------------------------------------------------ |\n| id | itemId | |\n| level | reliquary -> level, weapon -> level |\n| type | - | Type of equipment (Artifact or Weapon) |\n| refinement | weapon -> affixMap | |\n| ascension | weapon -> promoteLevel | |\n| detail | flat | Please refer [Equipments Info](#equipments-info) |\n\n### Equipments Info\n\n| Wrapper | API | Notes |\n| ------------- | ----------------------------------- | -------------------------------------------------- |\n| name | - | Equipment Name (Artifact name or Weapon name) |\n| icon | icon | Please refer [Icon Data](#icon-data) |\n| artifact_type | - | Please refer [Artifact Type](#artifact-type) |\n| rarity | rankLevel | |\n| mainstats | reliquaryMainstat, weaponStats -> 0 | Please refer [Equipments Stats](#equipments-stats) |\n| substats | reliquarySubstats, weaponStats -> 1 | Please refer [Equipments Stats](#equipments-stats) |\n\n### Equipments Stats\n\n| Wrapper | API | Notes |\n| ------- | ------- | ------------------------------ |\n| prop_id | prop_id | |\n| type | - | Value type (NUMBER or PERCENT) |\n| name | - | Name of FIGHT_PROP |\n| value | value | |\n\n## FIGHT_PROP Data\n\nIn FIGHT_PROP data. You can get the value from 4 methods.\n| Choice | Example | Output |\n|------------------|---------------------------|----------------------------|\n| Get raw value | stats.FIGHT_PROP_HP.value | 15552.306640625 |\n| Get rounded value| stats.FIGHT_PROP_ATTACK.to_rounded() | 344 |\n| Get percentage | stats.FIGHT_PROP_FIRE_ADD_HURT.to_percentage() | 61.5 |\n| Get percentage and symbol | stats.FIGHT_PROP_FIRE_ADD_HURT.to_percentage_symbol() | 61.5% |\n\n## Build\n\nIn this `Builds` It's not pretty data. You can use this method to get data. Or if you want get full, You can use `raw` argument\n| Choice | Example | Output |\n|------------------|---------------------------|----------------------------|\n| Get avatar ID list | builds.get_avatar_list() | [10000021,10000037,10000025, ...] |\n| Get character build | builds.get_character(10000021) | List of [Build info](#avatar-builds-info) |\n| Get build info by avatar id | builds.get_character(10000021, 11111111) | [Build info](#avatar-builds-info) |\n\n# Icon Data\n\nIn icon data. You can get the value from 2 methods.\n| Choice | Example | Output |\n|------------------|---------------------------|--------------------------------|\n| Get filename | icon.filename | UI_AvatarIcon_Kazuha_Card.png |\n| Get URL | icon.url | https://enka.network/ui/UI_AvatarIcon_Kazuha_Card.png |\n\n## Artifact Type\n\n| Key | Value |\n| ------- | -------------- |\n| Flower | EQUIP_BRACER |\n| Feather | EQUIP_NECKLACE |\n| Sands | EQUIP_SHOES |\n| Goblet | EQUIP_RING |\n| Circlet | EQUIP_DRESS |\n\n## Element Type\n\n| Key | Value |\n| ------- | -------- |\n| Cryo | Ice |\n| Hydro | Water |\n| Anemo | Wind |\n| Pyro | Fire |\n| Geo | Rock |\n| Electro | Electric |\n\n# Assets\n\n## Assets character, constellations, skills, namecards\n\nYou can use avatarId to get the character, constellations, skills, namecards from assets.\n\n```py\nimport asyncio\n\nfrom enkanetwork import Assets\n\nassets = Assets()\n\nasync def main():\n # Character\n assets.character(10000046)\n # Constellations\n assets.constellations(2081199193)\n # Skills\n assets.constellations(10462)\n # Namecards\n assets.namecards(210059)\n\nloop = asyncio.get_event_loop()\nloop.run_until_complete(main())\n```\n\n## Assets NameTextMapHash\n\nThe `NameTextMapHash` is a hash map that contains the name text of the assets. You can get `NameTextMapHash` from `hash_id` like this:\n\n```py\nimport asyncio\n\nfrom enkanetwork import Assets\n\nassets = Assets(lang=\"en\") # Set languege before get name (Ex. English)\n\nasync def main():\n print(assets.get_hash_map(1940919994)) # Hu tao\n # OR you can get FIGHT_PROP name\n print(assets.get_hash_map(\"FIGHT_PROP_BASE_ATTACK\")) # Base ATK\n\nloop = asyncio.get_event_loop()\nloop.run_until_complete(main())\n```\n\n## Languages Supported\n\n| Languege | Code |\n| ---------- | ---- |\n| English | en |\n| \u0440\u0443\u0441\u0441\u043a\u0438\u0439 | ru |\n| Ti\u1ebfng Vi\u1ec7t | vi |\n| \u0e44\u0e17\u0e22 | th |\n| portugu\u00eas | pt |\n| \ud55c\uad6d\uc5b4 | kr |\n| \u65e5\u672c\u8a9e | jp |\n| \u4e2d\u6587 | zh |\n| Indonesian | id |\n| fran\u00e7ais | fr |\n| espa\u00f1ol | es |\n| deutsch | de |\n| Taiwan | cht |\n| Chinese | chs |\n\nIf you want full docs for the API, visit [EnkaNetwork API Docs](https://github.com/EnkaNetwork/API-docs)\n\n## Support & Question\n\nIf you need support or some question about EnkaNetwokt.py. You can feel free contact to me in [Enka.network discord server](https://discord.gg/G3m7CWkssY) in [\ud835\uddaf\ud835\uddb8\u2503enkanetwork\u2024py](https://discord.com/channels/840335525621268520/1046281445049647104) channel and mention (Ping) to **@M-307** for support and help\n\n# LICENSE\n\n[MIT License](./LICENSE)\n\n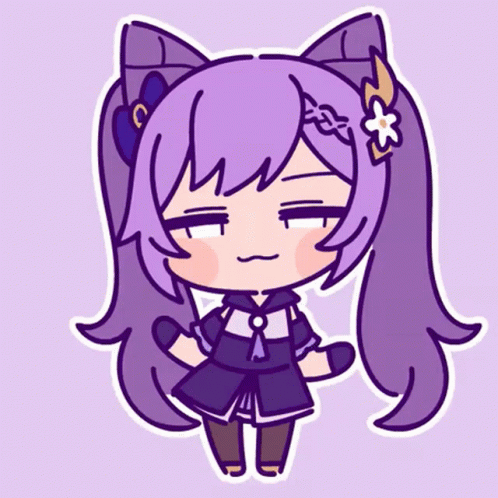\n\n[Picture by KKOMDASTRO](https://twitter.com/KKOMDASTRO)\n\n\n",
"bugtrack_url": null,
"license": "",
"summary": "Library for fetching JSON data from site https://enka.network/",
"version": "1.4.5",
"project_urls": {
"Homepage": "https://github.com/mrwan200/EnkaNetwork.py"
},
"split_keywords": [
"enkanetwork.py",
"enkanetwork",
"enka.network",
"genshinapi"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "84fc188d49959475ea255d5f1d26aad13647b81cde0cfa731f0ff6e3bca35a93",
"md5": "260b5f07caf2ba5404d34bd91f2c588a",
"sha256": "bdc99679bec55017bcf951f636e31793e8cf1fdb040315a430adedb890c0a34d"
},
"downloads": -1,
"filename": "enkanetwork.py-1.4.5.tar.gz",
"has_sig": false,
"md5_digest": "260b5f07caf2ba5404d34bd91f2c588a",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 423002,
"upload_time": "2023-08-30T08:25:44",
"upload_time_iso_8601": "2023-08-30T08:25:44.627733Z",
"url": "https://files.pythonhosted.org/packages/84/fc/188d49959475ea255d5f1d26aad13647b81cde0cfa731f0ff6e3bca35a93/enkanetwork.py-1.4.5.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-08-30 08:25:44",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "mrwan200",
"github_project": "EnkaNetwork.py",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "enkanetwork.py"
}