# Ensta - Simple Instagram API
[](https://pypi.org/project/ensta)
[]()
[](https://pepy.tech/project/ensta)
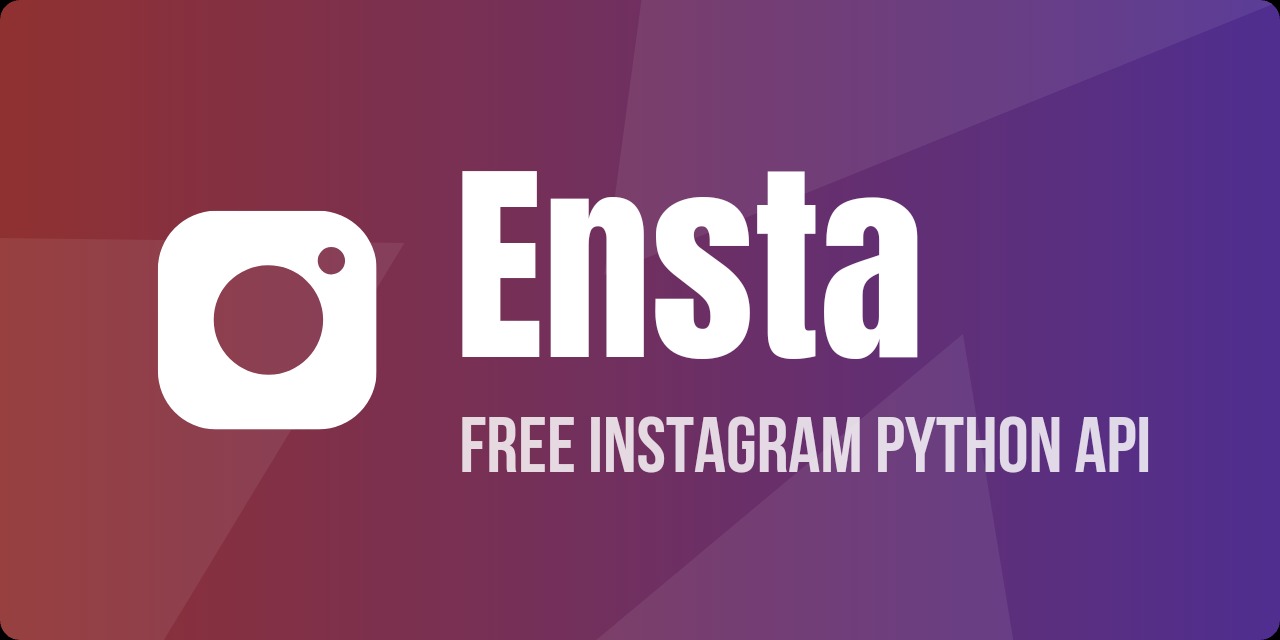
Ensta is a simple, reliable and up-to-date python package for Instagram API.
Both **authenticated** and **anonymous** requests are supported.
[<img style="margin-top: 10px" src="https://raw.githubusercontent.com/diezo/Ensta/master/assets/coffee.svg" width="180"/>](https://buymeacoffee.com/sonii)
<!--
## <img src="https://raw.githubusercontent.com/diezo/Ensta/master/assets/colorful-instagram-icon-vintage-style-art-vector-illustration_836950-30.jpg" width="23"> Account Creator
Download an Instagram [**Account Creator**](https://sonii.gumroad.com/l/account-creator/EARLY20) written in Python.
- Auto-generates **DuckDuckGo Private Email Addresses**.
- Auto-fetches OTP from **ProtonMail Inbox**.
- Auto-updates Profile Picture to an **AI-Generated Human Face**.
- Sets a random **AI-Generated Biography** on account creation.
Creator should only be used for legitimate purposes. [**Discord**](https://discordapp.com/users/1183040947035062382)
-->
## Installation
Read the [**pre-requisites**](https://github.com/diezo/Ensta/wiki/Pre%E2%80%90requisites) here.
pip install ensta
## Example
Fetching profile info by username:
```python
from ensta import Mobile
mobile = Mobile(username, password)
profile = mobile.profile("leomessi")
print(profile.full_name)
print(profile.biography)
print(profile.profile_pic_url)
```
## Features
These features use the **Mobile API**.
<details>
<summary>Using Proxies</summary><br>
When to use a proxy:
- You're being rate limited.
- Ensta is not working because your Home IP is flagged.
- You're deploying Ensta to the cloud. (Instagram blocks requests from IPs of cloud providers, so a proxy must be used)
```python
from ensta import Mobile
mobile = Mobile(
username,
password,
proxy={
"http": "socks5://username:password@host:port",
"https": "socks5://username:password@host:port"
}
)
```
Ensta uses the same proxy settings as the **requests** module.
</details>
<details>
<summary>Username-Password Login</summary><br>
Username is recommended to sign in. However, email can also be used.
```python
from ensta import Mobile
# Recommended
mobile = Mobile(username, password)
# This also works
mobile = Mobile(email, password)
```
</details>
<details>
<summary>Change Profile Picture</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.change_profile_picture("image.jpg")
```
</details>
<details>
<summary>Fetch Profile Information</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
profile = mobile.profile("leomessi")
print(profile.full_name)
print(profile.biography)
print(profile.follower_count)
```
</details>
<details>
<summary>Follow/Unfollow Account</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.follow("leomessi")
mobile.unfollow("leomessi")
```
</details>
<details>
<summary>Change Biography</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.change_biography("New bio here.")
```
</details>
<details>
<summary>Switch to Private/Public Account</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.switch_to_private_account()
mobile.switch_to_public_account()
```
</details>
<details>
<summary>Username to UserID / UserID to Username</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.username_to_userid("leomessi")
mobile.userid_to_username("12345678")
```
</details>
<details>
<summary>Like/Unlike Post</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.like(media_id)
mobile.unlike(media_id)
```
</details>
<details>
<summary>Fetch Followers/Followings</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
followers = mobile.followers("leomessi")
followings = mobile.followings("leomessi")
for user in followers.list:
print(user.full_name)
for user in followings.list:
print(user.full_name)
# Fetching next chunk
followers = mobile.followers(
"leomessi",
next_cursor=followers.next_cursor
)
```
</details>
<details>
<summary>Add Comment to Post</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.comment("Hello", media_id)
```
</details>
<details>
<summary>Upload Photo</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.upload_photo(
upload_id=upload_id,
caption="Hello"
)
```
</details>
<details>
<summary>Upload Sidecar (Multiple Photos)</summary><br>
```python
from ensta import Mobile
from ensta.structures import SidecarChild
mobile = Mobile(username, password)
mobile.upload_sidecar(
children=[
SidecarChild(uploda_id),
SidecarChild(uploda_id),
SidecarChild(uploda_id)
],
caption="Hello"
)
```
</details>
<details>
<summary>Fetch Private Information (Yours)</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
account = mobile.private_info()
print(account.email)
print(account.account_type)
print(account.phone_number)
```
</details>
<details>
<summary>Update Display Name</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.update_display_name("Lionel Messi")
```
</details>
<details>
<summary>Block/Unblock User</summary><br>
```python
from ensta import Mobile
mobile = Mobile(username, password)
mobile.block(123456789) # Use UserID
mobile.unblock(123456789) # Use UserID
```
</details>
<details>
<summary>Upload Story (Photo)</summary>
```python
from ensta import Mobile
mobile = Mobile(username, password)
upload_id = mobile.get_upload_id("image.jpg")
mobile.upload_story(upload_id)
```
</details>
<details>
<summary>Upload Story (Photo) + Link Sticker</summary>
```python
from ensta import Mobile
from ensta.structures import StoryLink
mobile = Mobile(username, password)
upload_id = mobile.get_upload_id("image.jpg")
mobile.upload_story(upload_id, entities=[
StoryLink(title="Google", url="https://google.com")
])
```
</details>
<details>
<summary>Send Message (Text)</summary>
```python
from ensta import Mobile
mobile = Mobile(username, password) # Or use email
direct = mobile.direct()
direct.send_text("Hello", thread_id)
```
</details>
<details>
<summary>Send Message (Picture)</summary>
```python
from ensta import Mobile
mobile = Mobile(username, password) # Or use email
direct = mobile.direct()
media_id = direct.fb_upload_image("image.jpg")
direct.send_photo(media_id, thread_id)
```
</details>
<details>
<summary>Add Biography Link</summary>
```python
from ensta import Mobile
mobile = Mobile(username, password) # Or use email
link_id = mobile.add_bio_link(
url="https://github.com/diezo",
title="Diezo's GitHub"
)
```
</details>
<details>
<summary>Add Multiple Biography Links</summary>
```python
from ensta import Mobile
from ensta.structures import BioLink
mobile = Mobile(username, password) # Or use email
link_ids = mobile.add_bio_links([
BioLink(url="https://example.com", title="Link 1"),
BioLink(url="https://example.com", title="Link 2"),
BioLink(url="https://example.com", title="Link 3")
])
```
</details>
<details>
<summary>Remove Biography Link</summary>
```python
from ensta import Mobile
mobile = Mobile(username, password) # Or use email
mobile.remove_bio_link(link_id)
```
</details>
<details>
<summary>Remove Multiple Biography Links</summary>
```python
from ensta import Mobile
mobile = Mobile(username, password) # Or use email
mobile.remove_bio_links([
link_id_1,
link_id_2,
link_id_3
])
```
</details>
<details>
<summary>Clear All Biography Links</summary>
```python
from ensta import Mobile
mobile = Mobile(username, password) # Or use email
mobile.clear_bio_links()
```
</details>
### Deprecated Features (Web API)
Features still using the **Web API**:
<details>
<summary>Upload Reel</summary><br>
```python
from ensta import Web
host = Web(username, password)
video_id = host.upload_video_for_reel("Video.mp4", thumbnail="Thumbnail.jpg")
host.pub_reel(
video_id,
caption="Enjoying the winter! ⛄"
)
```
</details>
<details>
<summary>Fetch Web Profile Data</summary><br>
```python
from ensta import Web
host = Web(username, password)
profile = host.profile("leomessi")
print(profile.full_name)
print(profile.biography)
print(profile.follower_count)
```
</details>
<details>
<summary>Fetch Someone's Feed</summary><br>
```python
from ensta import Web
host = Web(username, password)
posts = host.posts("leomessi", 100) # Want full list? Set count to '0'
for post in posts:
print(post.caption_text)
print(post.like_count)
```
</details>
<details>
<summary>Fetch Post's Likers</summary><br>
```python
from ensta import Web
host = Web(username, password)
post_id = host.get_post_id("https://www.instagram.com/p/Czr2yLmroCQ/")
likers = host.likers(post_id)
for user in likers.users:
print(user.username)
print(user.profile_picture_url)
```
</details>
They'll be migrated to the **Mobile API** soon.
## Supported Classes
> **Important:**
> The **Web Class** is deprecated and it's features are being migrated to the **Mobile Class**. It'll be removed from Ensta upon completion.
<details>
<br>
<summary><b>Mobile Class</b> (Authenticated)</summary>
Requires login, and has the most features.
```python
from ensta import Mobile
mobile = Mobile(username, password)
profile = mobile.profile("leomessi")
print(profile.full_name)
print(profile.biography)
print(profile.profile_pic_url)
```
</details>
<details>
<br>
<summary><b>Guest Class</b> (Non-Authenticated)</summary>
Doesn't require login, but has limited features.
```python
from ensta import Guest
guest = Guest()
profile = guest.profile("leomessi")
print(profile.biography)
```
</details>
<details>
<br>
<summary><b>Web Class</b> (Authenticated) <i>(Deprecated)</i></summary>
```python
from ensta import Web
host = Web(username, password)
profile = host.profile("leomessi")
print(profile.biography)
```
</details>
## Discord Community
Ask questions, discuss upcoming features and meet other developers.
[<img src="https://i.ibb.co/qdX7F1b/IMG-20240105-115646-modified-modified.png" width="150"/>](https://discord.com/invite/pU4knSwmQe)
## Buy Me A Coffee
Support me in the development of this project.
[<img src="https://raw.githubusercontent.com/diezo/Ensta/master/assets/coffee.svg" width="170"/>](https://buymeacoffee.com/sonii)
## Contributors
[](https://github.com/diezo/ensta/graphs/contributors)
## Disclaimer
This is a third party library and not associated with Instagram. We're strictly against spam. You are liable for all the actions you take.
Raw data
{
"_id": null,
"home_page": "https://github.com/diezo/ensta",
"name": "ensta",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.10",
"maintainer_email": "",
"keywords": "instagram-client,instagram,api-wrapper,instagram-scraper,instagram-api,instagram-sdk,instagram-photos,instagram-api-python,instabot,instagram-stories,instagram-bot,instapy,instagram-downloader,instagram-account,instagram-crawler,instagram-private-api,igtv,instagram-automation,reels,instagram-feed",
"author": "Deepak Soni",
"author_email": "sonniiii@outlook.com",
"download_url": "https://files.pythonhosted.org/packages/07/4a/b58b61734cd41d5277dfcaf79ab7a450a7484390af3d3eb44c6ab82b5882/ensta-5.2.9.tar.gz",
"platform": null,
"description": "# Ensta - Simple Instagram API\n[](https://pypi.org/project/ensta)\n[]()\n[](https://pepy.tech/project/ensta)\n\n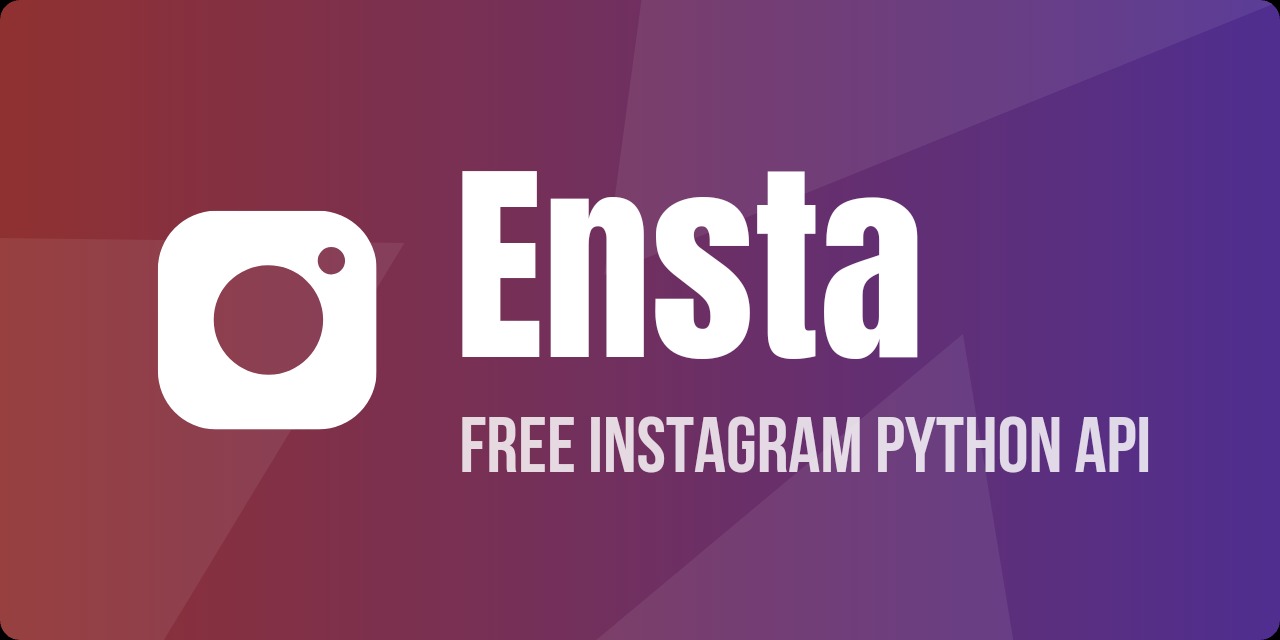\n\nEnsta is a simple, reliable and up-to-date python package for Instagram API.\n\nBoth **authenticated** and **anonymous** requests are supported.\n\n[<img style=\"margin-top: 10px\" src=\"https://raw.githubusercontent.com/diezo/Ensta/master/assets/coffee.svg\" width=\"180\"/>](https://buymeacoffee.com/sonii)\n<!--\n## <img src=\"https://raw.githubusercontent.com/diezo/Ensta/master/assets/colorful-instagram-icon-vintage-style-art-vector-illustration_836950-30.jpg\" width=\"23\"> Account Creator\nDownload an Instagram [**Account Creator**](https://sonii.gumroad.com/l/account-creator/EARLY20) written in Python.\n\n- Auto-generates **DuckDuckGo Private Email Addresses**.\n- Auto-fetches OTP from **ProtonMail Inbox**.\n- Auto-updates Profile Picture to an **AI-Generated Human Face**.\n- Sets a random **AI-Generated Biography** on account creation.\n\nCreator should only be used for legitimate purposes. [**Discord**](https://discordapp.com/users/1183040947035062382)\n-->\n\n## Installation\nRead the [**pre-requisites**](https://github.com/diezo/Ensta/wiki/Pre%E2%80%90requisites) here.\n\n pip install ensta\n\n## Example\nFetching profile info by username:\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nprofile = mobile.profile(\"leomessi\")\n\nprint(profile.full_name)\nprint(profile.biography)\nprint(profile.profile_pic_url)\n```\n\n## Features\nThese features use the **Mobile API**.\n\n<details>\n\n<summary>Using Proxies</summary><br>\n\nWhen to use a proxy:\n- You're being rate limited.\n- Ensta is not working because your Home IP is flagged.\n- You're deploying Ensta to the cloud. (Instagram blocks requests from IPs of cloud providers, so a proxy must be used)\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(\n username,\n password,\n proxy={\n \"http\": \"socks5://username:password@host:port\",\n \"https\": \"socks5://username:password@host:port\"\n }\n)\n```\n\nEnsta uses the same proxy settings as the **requests** module.\n\n</details>\n\n<details>\n\n<summary>Username-Password Login</summary><br>\n\nUsername is recommended to sign in. However, email can also be used.\n\n```python\nfrom ensta import Mobile\n\n# Recommended\nmobile = Mobile(username, password)\n\n# This also works\nmobile = Mobile(email, password)\n```\n\n</details>\n\n<details>\n\n<summary>Change Profile Picture</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.change_profile_picture(\"image.jpg\")\n```\n\n</details>\n\n<details>\n\n<summary>Fetch Profile Information</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nprofile = mobile.profile(\"leomessi\")\n\nprint(profile.full_name)\nprint(profile.biography)\nprint(profile.follower_count)\n```\n\n</details>\n\n<details>\n\n<summary>Follow/Unfollow Account</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.follow(\"leomessi\")\nmobile.unfollow(\"leomessi\")\n```\n\n</details>\n\n<details>\n\n<summary>Change Biography</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.change_biography(\"New bio here.\")\n```\n\n</details>\n\n<details>\n\n<summary>Switch to Private/Public Account</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.switch_to_private_account()\nmobile.switch_to_public_account()\n```\n\n</details>\n\n<details>\n\n<summary>Username to UserID / UserID to Username</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.username_to_userid(\"leomessi\")\nmobile.userid_to_username(\"12345678\")\n```\n\n</details>\n\n<details>\n\n<summary>Like/Unlike Post</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.like(media_id)\nmobile.unlike(media_id)\n```\n\n</details>\n\n<details>\n\n<summary>Fetch Followers/Followings</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nfollowers = mobile.followers(\"leomessi\")\nfollowings = mobile.followings(\"leomessi\")\n\nfor user in followers.list:\n print(user.full_name)\n\nfor user in followings.list:\n print(user.full_name)\n\n# Fetching next chunk\nfollowers = mobile.followers(\n \"leomessi\",\n next_cursor=followers.next_cursor\n)\n```\n\n</details>\n\n<details>\n\n<summary>Add Comment to Post</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.comment(\"Hello\", media_id)\n```\n\n</details>\n\n<details>\n\n<summary>Upload Photo</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.upload_photo(\n upload_id=upload_id,\n caption=\"Hello\"\n)\n```\n\n</details>\n\n<details>\n\n<summary>Upload Sidecar (Multiple Photos)</summary><br>\n\n```python\nfrom ensta import Mobile\nfrom ensta.structures import SidecarChild\n\nmobile = Mobile(username, password)\n\nmobile.upload_sidecar(\n children=[\n SidecarChild(uploda_id),\n SidecarChild(uploda_id),\n SidecarChild(uploda_id)\n ],\n caption=\"Hello\"\n)\n```\n\n</details>\n\n<details>\n\n<summary>Fetch Private Information (Yours)</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\naccount = mobile.private_info()\n\nprint(account.email)\nprint(account.account_type)\nprint(account.phone_number)\n```\n\n</details>\n\n<details>\n\n<summary>Update Display Name</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.update_display_name(\"Lionel Messi\")\n```\n\n</details>\n\n<details>\n\n<summary>Block/Unblock User</summary><br>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nmobile.block(123456789) # Use UserID\nmobile.unblock(123456789) # Use UserID\n```\n\n</details>\n\n<details>\n\n<summary>Upload Story (Photo)</summary>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\n\nupload_id = mobile.get_upload_id(\"image.jpg\")\n\nmobile.upload_story(upload_id)\n```\n\n</details>\n\n<details>\n\n<summary>Upload Story (Photo) + Link Sticker</summary>\n\n```python\nfrom ensta import Mobile\nfrom ensta.structures import StoryLink\n\nmobile = Mobile(username, password)\n\nupload_id = mobile.get_upload_id(\"image.jpg\")\n\nmobile.upload_story(upload_id, entities=[\n StoryLink(title=\"Google\", url=\"https://google.com\")\n])\n```\n\n</details>\n\n<details>\n\n<summary>Send Message (Text)</summary>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password) # Or use email\ndirect = mobile.direct()\n\ndirect.send_text(\"Hello\", thread_id)\n```\n\n</details>\n\n<details>\n\n<summary>Send Message (Picture)</summary>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password) # Or use email\ndirect = mobile.direct()\n\nmedia_id = direct.fb_upload_image(\"image.jpg\")\n\ndirect.send_photo(media_id, thread_id)\n```\n\n</details>\n\n<details>\n\n<summary>Add Biography Link</summary>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password) # Or use email\n\nlink_id = mobile.add_bio_link(\n url=\"https://github.com/diezo\",\n title=\"Diezo's GitHub\"\n)\n```\n\n</details>\n\n<details>\n\n<summary>Add Multiple Biography Links</summary>\n\n```python\nfrom ensta import Mobile\nfrom ensta.structures import BioLink\n\nmobile = Mobile(username, password) # Or use email\n\nlink_ids = mobile.add_bio_links([\n BioLink(url=\"https://example.com\", title=\"Link 1\"),\n BioLink(url=\"https://example.com\", title=\"Link 2\"),\n BioLink(url=\"https://example.com\", title=\"Link 3\")\n])\n```\n\n</details>\n\n<details>\n\n<summary>Remove Biography Link</summary>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password) # Or use email\n\nmobile.remove_bio_link(link_id)\n```\n\n</details>\n\n<details>\n\n<summary>Remove Multiple Biography Links</summary>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password) # Or use email\n\nmobile.remove_bio_links([\n link_id_1,\n link_id_2,\n link_id_3\n])\n```\n\n</details>\n\n<details>\n\n<summary>Clear All Biography Links</summary>\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password) # Or use email\n\nmobile.clear_bio_links()\n```\n\n</details>\n\n### Deprecated Features (Web API)\nFeatures still using the **Web API**:\n\n<details>\n\n<summary>Upload Reel</summary><br>\n\n```python\nfrom ensta import Web\n\nhost = Web(username, password)\n\nvideo_id = host.upload_video_for_reel(\"Video.mp4\", thumbnail=\"Thumbnail.jpg\")\n\nhost.pub_reel(\n video_id,\n caption=\"Enjoying the winter! \u26c4\"\n)\n```\n\n</details>\n\n<details>\n\n<summary>Fetch Web Profile Data</summary><br>\n\n```python\nfrom ensta import Web\n\nhost = Web(username, password)\nprofile = host.profile(\"leomessi\")\n\nprint(profile.full_name)\nprint(profile.biography)\nprint(profile.follower_count)\n```\n\n</details>\n\n<details>\n\n<summary>Fetch Someone's Feed</summary><br>\n\n```python\nfrom ensta import Web\n\nhost = Web(username, password)\nposts = host.posts(\"leomessi\", 100) # Want full list? Set count to '0'\n\nfor post in posts:\n print(post.caption_text)\n print(post.like_count) \n```\n\n</details>\n\n<details>\n\n<summary>Fetch Post's Likers</summary><br>\n\n```python\nfrom ensta import Web\n\nhost = Web(username, password)\n\npost_id = host.get_post_id(\"https://www.instagram.com/p/Czr2yLmroCQ/\")\nlikers = host.likers(post_id)\n\nfor user in likers.users:\n print(user.username)\n print(user.profile_picture_url)\n```\n\n</details>\n\nThey'll be migrated to the **Mobile API** soon.\n\n## Supported Classes\n\n> **Important:**\n> The **Web Class** is deprecated and it's features are being migrated to the **Mobile Class**. It'll be removed from Ensta upon completion.\n\n<details>\n\n<br>\n\n<summary><b>Mobile Class</b> (Authenticated)</summary>\n\nRequires login, and has the most features.\n\n```python\nfrom ensta import Mobile\n\nmobile = Mobile(username, password)\nprofile = mobile.profile(\"leomessi\")\n\nprint(profile.full_name)\nprint(profile.biography)\nprint(profile.profile_pic_url)\n```\n\n</details>\n\n<details>\n\n<br>\n\n<summary><b>Guest Class</b> (Non-Authenticated)</summary>\n\nDoesn't require login, but has limited features.\n\n```python\nfrom ensta import Guest\n\nguest = Guest()\nprofile = guest.profile(\"leomessi\")\n\nprint(profile.biography)\n```\n\n</details>\n\n<details>\n\n<br>\n\n<summary><b>Web Class</b> (Authenticated) <i>(Deprecated)</i></summary>\n\n```python\nfrom ensta import Web\n\nhost = Web(username, password)\nprofile = host.profile(\"leomessi\")\n\nprint(profile.biography)\n```\n\n</details>\n\n## Discord Community\nAsk questions, discuss upcoming features and meet other developers.\n\n[<img src=\"https://i.ibb.co/qdX7F1b/IMG-20240105-115646-modified-modified.png\" width=\"150\"/>](https://discord.com/invite/pU4knSwmQe)\n\n## Buy Me A Coffee\nSupport me in the development of this project.\n\n[<img src=\"https://raw.githubusercontent.com/diezo/Ensta/master/assets/coffee.svg\" width=\"170\"/>](https://buymeacoffee.com/sonii)\n\n## Contributors\n[](https://github.com/diezo/ensta/graphs/contributors)\n\n## Disclaimer\nThis is a third party library and not associated with Instagram. We're strictly against spam. You are liable for all the actions you take.\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "\ud83d\udd25 Fastest & Simplest Python Package For Instagram Automation",
"version": "5.2.9",
"project_urls": {
"Download": "https://github.com/diezo/ensta/archive/refs/tags/v5.2.9.tar.gz",
"Homepage": "https://github.com/diezo/ensta"
},
"split_keywords": [
"instagram-client",
"instagram",
"api-wrapper",
"instagram-scraper",
"instagram-api",
"instagram-sdk",
"instagram-photos",
"instagram-api-python",
"instabot",
"instagram-stories",
"instagram-bot",
"instapy",
"instagram-downloader",
"instagram-account",
"instagram-crawler",
"instagram-private-api",
"igtv",
"instagram-automation",
"reels",
"instagram-feed"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "fae3ed01c25fad00eeeab8e359553cdb7a775d0c618524bed4395308ba3c15f2",
"md5": "e3b5b5ca4bac9179c03c68d7eaf7f8cc",
"sha256": "a5a50eae8a330d9842f2ad9abeb1d3674c1460bafc3f55cb52011397e9e4fa1a"
},
"downloads": -1,
"filename": "ensta-5.2.9-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e3b5b5ca4bac9179c03c68d7eaf7f8cc",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.10",
"size": 66140,
"upload_time": "2024-03-12T14:44:12",
"upload_time_iso_8601": "2024-03-12T14:44:12.618386Z",
"url": "https://files.pythonhosted.org/packages/fa/e3/ed01c25fad00eeeab8e359553cdb7a775d0c618524bed4395308ba3c15f2/ensta-5.2.9-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "074ab58b61734cd41d5277dfcaf79ab7a450a7484390af3d3eb44c6ab82b5882",
"md5": "ca23f91403ea273b9169c0066380c415",
"sha256": "313a1987e757a03889c1d1fa789d76b6c10d3857f0b3e763dd4ec686cdf9fe73"
},
"downloads": -1,
"filename": "ensta-5.2.9.tar.gz",
"has_sig": false,
"md5_digest": "ca23f91403ea273b9169c0066380c415",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.10",
"size": 49369,
"upload_time": "2024-03-12T14:44:14",
"upload_time_iso_8601": "2024-03-12T14:44:14.911885Z",
"url": "https://files.pythonhosted.org/packages/07/4a/b58b61734cd41d5277dfcaf79ab7a450a7484390af3d3eb44c6ab82b5882/ensta-5.2.9.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-12 14:44:14",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "diezo",
"github_project": "ensta",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "ensta"
}