# Welcome
ezpylog is a minimalistic and easy to use python logger
## Installation
Using pip :
- directly from command line with : `pip install ezpylog`
- manually by [downloading ezpylog](https://github.com/JRodez/ezpylog/releases) and install it with `pip install ezpylog-X.X.tar.gz`
## How to use ?
### Import :
```python
from ezpylog import Logger, LogLevel
```
### Logging Levels :
The level can be as following :
```python
LogLevel.DEBUG
LogLevel.INFO
LogLevel.WARNING
LogLevel.ERROR
LogLevel.CRITICAL
```
### Initialisation :
```python
logger = Logger(name=None,
min_level: int = logging.WARNING,
logfile: str = None,
logfile_level=None,
color_on_console: bool = True)
```
- `name` is the name of the logger. If not set, the name of the module will be used.
- `min_level` is a `LogLevel` enum and filters log messages on the console (ex : `WARNING` will not print `INFO` messages). Default is `WARNING`.
- `context` is the logging context, you can use `"main()"` if you use it in `__main__` for example. Default is `""`.
- `logfile` is the name of your optional log file for `DEBUG`,`INFO` and `DEBUG` messages. Default is `None` (no log file).
- `logfile_level` is a `LogLevel` enum and filters log messages in the log file (ex : `WARNING` will not print `INFO` messages). Default is `WARNING`.
- `color_on_console` is a boolean to enable or disable color on the console. Default is `True`.
### logging :
```python
logger.log(msg)
# or
logger.log(msg, level)
```
with default `level = LogLevel.INFO`.
You can call the loglevel corresonding function too :
```python
logger.debug(msg)
logger.info(msg)
logger.warning(msg)
logger.error(msg)
logger.critical(msg)
```
## Example :
You can find this exemple by calling `Logger.loggerdemo()`
```python
from ezpylog import Logger, LogLevel
a = 1234567
logger = Logger(LogLevel.DEBUG)
logger.log("Debug message", LogLevel.DEBUG, "context")
logger.log("Info message")
logger.log("Warning message", LogLevel.WARNING, "context")
logger.log(f"Error message {a}", LogLevel.ERROR, "context")
logger.log("Critical message", LogLevel.CRITICAL, "context")
logger2 = Logger(LogLevel.WARNING, "__main__")
logger2.log("Debug message", LogLevel.DEBUG, "subcontextA()")
logger2.log("Info message", LogLevel.INFO, "subcontextB()")
logger2.log("Warning message", LogLevel.WARNING, "subcontextA()")
logger2.log(f"Error message {a}", LogLevel.ERROR, "subcontextB()")
logger2.log("Critical message", LogLevel.CRITICAL)
```
prints the following :
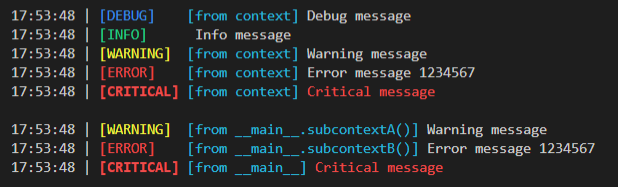
Raw data
{
"_id": null,
"home_page": "https://github.com/JRodez/ezpylog",
"name": "ezpylog",
"maintainer": null,
"docs_url": null,
"requires_python": null,
"maintainer_email": null,
"keywords": "tool print ezpylog logger logging log level debug info warning error critical color terminal cli magnificient colorful beautiful filter filter_by_level filter_by_context filter_by_message",
"author": "J\u00e9r\u00e9mie Rodez",
"author_email": "jeremierodez@outlook.com",
"download_url": "https://files.pythonhosted.org/packages/d0/0d/1440dfc27cea909b523312a7697963caab5bacd7da1a220a7507001ec673/ezpylog-2.2.1.tar.gz",
"platform": null,
"description": "# Welcome\nezpylog is a minimalistic and easy to use python logger\n## Installation\nUsing pip :\n - directly from command line with : `pip install ezpylog` \n - manually by [downloading ezpylog](https://github.com/JRodez/ezpylog/releases) and install it with `pip install ezpylog-X.X.tar.gz`\n\n## How to use ?\n### Import :\n```python\nfrom ezpylog import Logger, LogLevel\n```\n\n### Logging Levels :\nThe level can be as following :\n```python\nLogLevel.DEBUG\nLogLevel.INFO\nLogLevel.WARNING\nLogLevel.ERROR\nLogLevel.CRITICAL\n```\n\n### Initialisation :\n```python\nlogger = Logger(name=None, \n\t\t\t\tmin_level: int = logging.WARNING, \n\t\t\t\tlogfile: str = None, \n\t\t\t\tlogfile_level=None, \n\t\t\t\tcolor_on_console: bool = True)\n```\n- `name` is the name of the logger. If not set, the name of the module will be used.\n- `min_level` is a `LogLevel` enum and filters log messages on the console (ex : `WARNING` will not print `INFO` messages). Default is `WARNING`.\n- `context` is the logging context, you can use `\"main()\"` if you use it in `__main__` for example. Default is `\"\"`.\n- `logfile` is the name of your optional log file for `DEBUG`,`INFO` and `DEBUG` messages. Default is `None` (no log file).\n- `logfile_level` is a `LogLevel` enum and filters log messages in the log file (ex : `WARNING` will not print `INFO` messages). Default is `WARNING`.\n- `color_on_console` is a boolean to enable or disable color on the console. Default is `True`.\n\n### logging : \n```python\nlogger.log(msg)\n# or\nlogger.log(msg, level)\n```\nwith default `level = LogLevel.INFO`.\n\nYou can call the loglevel corresonding function too :\n```python\nlogger.debug(msg)\nlogger.info(msg)\nlogger.warning(msg)\nlogger.error(msg)\nlogger.critical(msg)\n```\n\n## Example :\nYou can find this exemple by calling `Logger.loggerdemo()`\n\n```python\nfrom ezpylog import Logger, LogLevel\n\na = 1234567\n\nlogger = Logger(LogLevel.DEBUG)\nlogger.log(\"Debug message\", LogLevel.DEBUG, \"context\")\nlogger.log(\"Info message\")\nlogger.log(\"Warning message\", LogLevel.WARNING, \"context\")\nlogger.log(f\"Error message {a}\", LogLevel.ERROR, \"context\")\nlogger.log(\"Critical message\", LogLevel.CRITICAL, \"context\")\n\nlogger2 = Logger(LogLevel.WARNING, \"__main__\")\nlogger2.log(\"Debug message\", LogLevel.DEBUG, \"subcontextA()\")\nlogger2.log(\"Info message\", LogLevel.INFO, \"subcontextB()\")\nlogger2.log(\"Warning message\", LogLevel.WARNING, \"subcontextA()\")\nlogger2.log(f\"Error message {a}\", LogLevel.ERROR, \"subcontextB()\")\nlogger2.log(\"Critical message\", LogLevel.CRITICAL)\n```\t\n\nprints the following : \n\n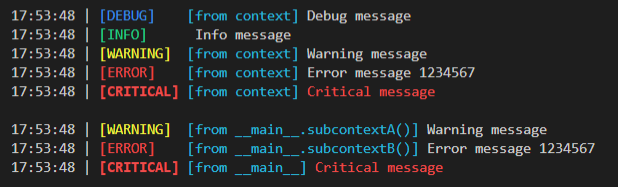\n",
"bugtrack_url": null,
"license": "GPLv3",
"summary": "A nice-looking, minimalist and easy-to-use Python library for logging your Python programs.",
"version": "2.2.1",
"project_urls": {
"Homepage": "https://github.com/JRodez/ezpylog"
},
"split_keywords": [
"tool",
"print",
"ezpylog",
"logger",
"logging",
"log",
"level",
"debug",
"info",
"warning",
"error",
"critical",
"color",
"terminal",
"cli",
"magnificient",
"colorful",
"beautiful",
"filter",
"filter_by_level",
"filter_by_context",
"filter_by_message"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "78942e3c6eacf7ebfaa19bd473fd55b9bf317e0e801bb06657b4e65d227295c0",
"md5": "082469693d9dbfe656af7022c1fff398",
"sha256": "10c7d8b53b9f9d947f109cc6e87413ae2bff3d1100758a681d1b0c2f6f3a76fe"
},
"downloads": -1,
"filename": "ezpylog-2.2.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "082469693d9dbfe656af7022c1fff398",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 19149,
"upload_time": "2024-08-29T11:46:17",
"upload_time_iso_8601": "2024-08-29T11:46:17.356751Z",
"url": "https://files.pythonhosted.org/packages/78/94/2e3c6eacf7ebfaa19bd473fd55b9bf317e0e801bb06657b4e65d227295c0/ezpylog-2.2.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d00d1440dfc27cea909b523312a7697963caab5bacd7da1a220a7507001ec673",
"md5": "4f3bf7d97b4ed085ce09baa811b80e13",
"sha256": "992b55ad9277a5b80876ea17516c30b9027c6b2b15cc1d6e3e3c1ac344f53bf4"
},
"downloads": -1,
"filename": "ezpylog-2.2.1.tar.gz",
"has_sig": false,
"md5_digest": "4f3bf7d97b4ed085ce09baa811b80e13",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 20310,
"upload_time": "2024-08-29T11:46:19",
"upload_time_iso_8601": "2024-08-29T11:46:19.071589Z",
"url": "https://files.pythonhosted.org/packages/d0/0d/1440dfc27cea909b523312a7697963caab5bacd7da1a220a7507001ec673/ezpylog-2.2.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-29 11:46:19",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "JRodez",
"github_project": "ezpylog",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "ezpylog"
}