# FastALPR
[](https://github.com/ankandrew/fast-alpr/actions)
[](https://github.com/astral-sh/ruff)
[](https://github.com/pylint-dev/pylint)
[](http://mypy-lang.org/)
[](https://onnx.ai/)
[](https://huggingface.co/spaces/ankandrew/fast-alpr)
[](https://ankandrew.github.io/fast-alpr/)
[](https://www.python.org/)
[](https://github.com/ankandrew/fast-alpr/releases)
[](./LICENSE)
[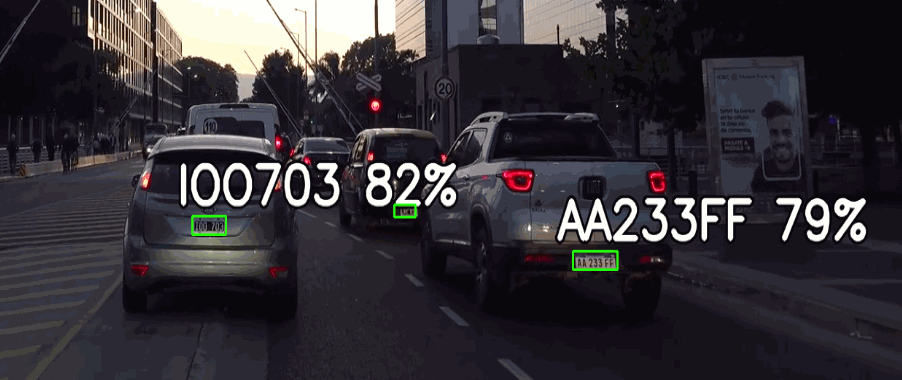](https://youtu.be/-TPJot7-HTs?t=652)
**FastALPR** is a high-performance, customizable Automatic License Plate Recognition (ALPR) system. We offer fast and
efficient ONNX models by default, but you can easily swap in your own models if needed.
For Optical Character Recognition (**OCR**), we use [fast-plate-ocr](https://github.com/ankandrew/fast-plate-ocr) by
default, and for **license plate detection**, we
use [open-image-models](https://github.com/ankandrew/open-image-models). However, you can integrate any OCR or detection
model of your choice.
## 📋 Table of Contents
* [✨ Features](#-features)
* [📦 Installation](#-installation)
* [🚀 Quick Start](#-quick-start)
* [🛠️ Customization and Flexibility](#-customization-and-flexibility)
* [📖 Documentation](#-documentation)
* [🤝 Contributing](#-contributing)
* [🙏 Acknowledgements](#-acknowledgements)
* [📫 Contact](#-contact)
## ✨ Features
- **High Accuracy**: Uses advanced models for precise license plate detection and OCR.
- **Customizable**: Easily switch out detection and OCR models.
- **Easy to Use**: Quick setup with a simple API.
- **Out-of-the-Box Models**: Includes ready-to-use detection and OCR models
- **Fast Performance**: Optimized with ONNX Runtime for speed.
## 📦 Installation
```bash
pip install fast-alpr
```
## 🚀 Quick Start
Here's how to get started with FastALPR:
```python
from fast_alpr import ALPR
# You can also initialize the ALPR with custom plate detection and OCR models.
alpr = ALPR(
detector_model="yolo-v9-t-384-license-plate-end2end",
ocr_model="european-plates-mobile-vit-v2-model",
)
# The "assets/test_image.png" can be found in repo root dit
alpr_results = alpr.predict("assets/test_image.png")
print(alpr_results)
```
Output:
<img alt="ALPR Result" src="https://raw.githubusercontent.com/ankandrew/fast-alpr/5063bd92fdd30f46b330d051468be267d4442c9b/assets/alpr_result.webp"/>
You can also draw the predictions directly on the image:
```python
import cv2
from fast_alpr import ALPR
# Initialize the ALPR
alpr = ALPR(
detector_model="yolo-v9-t-384-license-plate-end2end",
ocr_model="european-plates-mobile-vit-v2-model",
)
# Load the image
image_path = "assets/test_image.png"
frame = cv2.imread(image_path)
# Draw predictions on the image
annotated_frame = alpr.draw_predictions(frame)
# Display the result
cv2.imshow("ALPR Result", annotated_frame)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
Output:
<img alt="ALPR Draw Predictions" src="https://raw.githubusercontent.com/ankandrew/fast-alpr/0a6076dcb8d9084514fe47e8abaaeb77cae45f8e/assets/alpr_draw_predictions.png"/>
## 🛠️ Customization and Flexibility
FastALPR is designed to be flexible. You can customize the detector and OCR models according to your requirements.
You can very easily integrate with **Tesseract** OCR to leverage its capabilities:
```python
import re
from statistics import mean
import numpy as np
import pytesseract
from fast_alpr.alpr import ALPR, BaseOCR, OcrResult
class PytesseractOCR(BaseOCR):
def __init__(self) -> None:
"""
Init PytesseractOCR.
"""
def predict(self, cropped_plate: np.ndarray) -> OcrResult | None:
if cropped_plate is None:
return None
# You can change 'eng' to the appropriate language code as needed
data = pytesseract.image_to_data(
cropped_plate,
lang="eng",
config="--oem 3 --psm 6",
output_type=pytesseract.Output.DICT,
)
plate_text = " ".join(data["text"]).strip()
plate_text = re.sub(r"[^A-Za-z0-9]", "", plate_text)
avg_confidence = mean(conf for conf in data["conf"] if conf > 0) / 100.0
return OcrResult(text=plate_text, confidence=avg_confidence)
alpr = ALPR(detector_model="yolo-v9-t-384-license-plate-end2end", ocr=PytesseractOCR())
alpr_results = alpr.predict("assets/test_image.png")
print(alpr_results)
```
> [!TIP]
> See the [docs](https://ankandrew.github.io/fast-alpr/) for more examples!
## 📖 Documentation
Comprehensive documentation is available [here](https://ankandrew.github.io/fast-alpr/), including detailed API
references and additional examples.
## 🤝 Contributing
Contributions to the repo are greatly appreciated. Whether it's bug fixes, feature enhancements, or new models,
your contributions are warmly welcomed.
To start contributing or to begin development, you can follow these steps:
1. Clone repo
```shell
git clone https://github.com/ankandrew/fast-alpr.git
```
2. Install all dependencies using [Poetry](https://python-poetry.org/docs/#installation):
```shell
poetry install --all-extras
```
3. To ensure your changes pass linting and tests before submitting a PR:
```shell
make checks
```
## 🙏 Acknowledgements
- [fast-plate-ocr](https://github.com/ankandrew/fast-plate-ocr) for default **OCR** models.
- [open-image-models](https://github.com/ankandrew/open-image-models) for default plate **detection** models.
## 📫 Contact
For questions or suggestions, feel free to open an issue or reach out through social networks.
Raw data
{
"_id": null,
"home_page": null,
"name": "fast-alpr",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.10",
"maintainer_email": null,
"keywords": "image-processing, computer-vision, deep-learning, object-detection, plate-detection, license-plate-ocr, onnx",
"author": "ankandrew",
"author_email": "61120139+ankandrew@users.noreply.github.com",
"download_url": "https://files.pythonhosted.org/packages/d5/c2/152228f97f640ad3dd4617de8bae3af93eab888ab2104c3d8fefa9a1654c/fast_alpr-0.1.1.tar.gz",
"platform": null,
"description": "# FastALPR\n\n[](https://github.com/ankandrew/fast-alpr/actions)\n[](https://github.com/astral-sh/ruff)\n[](https://github.com/pylint-dev/pylint)\n[](http://mypy-lang.org/)\n[](https://onnx.ai/)\n[](https://huggingface.co/spaces/ankandrew/fast-alpr)\n[](https://ankandrew.github.io/fast-alpr/)\n[](https://www.python.org/)\n[](https://github.com/ankandrew/fast-alpr/releases)\n[](./LICENSE)\n\n[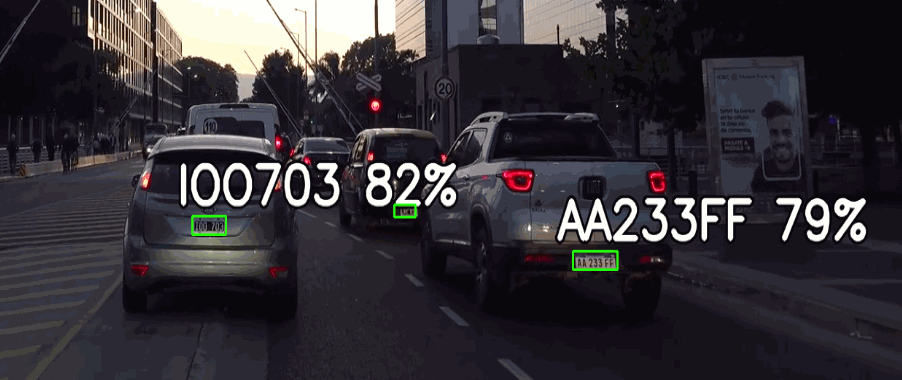](https://youtu.be/-TPJot7-HTs?t=652)\n\n**FastALPR** is a high-performance, customizable Automatic License Plate Recognition (ALPR) system. We offer fast and\nefficient ONNX models by default, but you can easily swap in your own models if needed.\n\nFor Optical Character Recognition (**OCR**), we use [fast-plate-ocr](https://github.com/ankandrew/fast-plate-ocr) by\ndefault, and for **license plate detection**, we\nuse [open-image-models](https://github.com/ankandrew/open-image-models). However, you can integrate any OCR or detection\nmodel of your choice.\n\n## \ud83d\udccb Table of Contents\n\n* [\u2728 Features](#-features)\n* [\ud83d\udce6 Installation](#-installation)\n* [\ud83d\ude80 Quick Start](#-quick-start)\n* [\ud83d\udee0\ufe0f Customization and Flexibility](#-customization-and-flexibility)\n* [\ud83d\udcd6 Documentation](#-documentation)\n* [\ud83e\udd1d Contributing](#-contributing)\n* [\ud83d\ude4f Acknowledgements](#-acknowledgements)\n* [\ud83d\udceb Contact](#-contact)\n\n## \u2728 Features\n\n- **High Accuracy**: Uses advanced models for precise license plate detection and OCR.\n- **Customizable**: Easily switch out detection and OCR models.\n- **Easy to Use**: Quick setup with a simple API.\n- **Out-of-the-Box Models**: Includes ready-to-use detection and OCR models\n- **Fast Performance**: Optimized with ONNX Runtime for speed.\n\n## \ud83d\udce6 Installation\n\n```bash\npip install fast-alpr\n```\n\n## \ud83d\ude80 Quick Start\n\nHere's how to get started with FastALPR:\n\n```python\nfrom fast_alpr import ALPR\n\n# You can also initialize the ALPR with custom plate detection and OCR models.\nalpr = ALPR(\n detector_model=\"yolo-v9-t-384-license-plate-end2end\",\n ocr_model=\"european-plates-mobile-vit-v2-model\",\n)\n\n# The \"assets/test_image.png\" can be found in repo root dit\nalpr_results = alpr.predict(\"assets/test_image.png\")\nprint(alpr_results)\n```\n\nOutput:\n\n<img alt=\"ALPR Result\" src=\"https://raw.githubusercontent.com/ankandrew/fast-alpr/5063bd92fdd30f46b330d051468be267d4442c9b/assets/alpr_result.webp\"/>\n\nYou can also draw the predictions directly on the image:\n\n```python\nimport cv2\n\nfrom fast_alpr import ALPR\n\n# Initialize the ALPR\nalpr = ALPR(\n detector_model=\"yolo-v9-t-384-license-plate-end2end\",\n ocr_model=\"european-plates-mobile-vit-v2-model\",\n)\n\n# Load the image\nimage_path = \"assets/test_image.png\"\nframe = cv2.imread(image_path)\n\n# Draw predictions on the image\nannotated_frame = alpr.draw_predictions(frame)\n\n# Display the result\ncv2.imshow(\"ALPR Result\", annotated_frame)\ncv2.waitKey(0)\ncv2.destroyAllWindows()\n```\n\nOutput:\n\n<img alt=\"ALPR Draw Predictions\" src=\"https://raw.githubusercontent.com/ankandrew/fast-alpr/0a6076dcb8d9084514fe47e8abaaeb77cae45f8e/assets/alpr_draw_predictions.png\"/>\n\n## \ud83d\udee0\ufe0f Customization and Flexibility\n\nFastALPR is designed to be flexible. You can customize the detector and OCR models according to your requirements.\nYou can very easily integrate with **Tesseract** OCR to leverage its capabilities:\n\n```python\nimport re\nfrom statistics import mean\n\nimport numpy as np\nimport pytesseract\n\nfrom fast_alpr.alpr import ALPR, BaseOCR, OcrResult\n\n\nclass PytesseractOCR(BaseOCR):\n def __init__(self) -> None:\n \"\"\"\n Init PytesseractOCR.\n \"\"\"\n\n def predict(self, cropped_plate: np.ndarray) -> OcrResult | None:\n if cropped_plate is None:\n return None\n # You can change 'eng' to the appropriate language code as needed\n data = pytesseract.image_to_data(\n cropped_plate,\n lang=\"eng\",\n config=\"--oem 3 --psm 6\",\n output_type=pytesseract.Output.DICT,\n )\n plate_text = \" \".join(data[\"text\"]).strip()\n plate_text = re.sub(r\"[^A-Za-z0-9]\", \"\", plate_text)\n avg_confidence = mean(conf for conf in data[\"conf\"] if conf > 0) / 100.0\n return OcrResult(text=plate_text, confidence=avg_confidence)\n\n\nalpr = ALPR(detector_model=\"yolo-v9-t-384-license-plate-end2end\", ocr=PytesseractOCR())\n\nalpr_results = alpr.predict(\"assets/test_image.png\")\nprint(alpr_results)\n```\n\n> [!TIP]\n> See the [docs](https://ankandrew.github.io/fast-alpr/) for more examples!\n\n## \ud83d\udcd6 Documentation\n\nComprehensive documentation is available [here](https://ankandrew.github.io/fast-alpr/), including detailed API\nreferences and additional examples.\n\n## \ud83e\udd1d Contributing\n\nContributions to the repo are greatly appreciated. Whether it's bug fixes, feature enhancements, or new models,\nyour contributions are warmly welcomed.\n\nTo start contributing or to begin development, you can follow these steps:\n\n1. Clone repo\n ```shell\n git clone https://github.com/ankandrew/fast-alpr.git\n ```\n2. Install all dependencies using [Poetry](https://python-poetry.org/docs/#installation):\n ```shell\n poetry install --all-extras\n ```\n3. To ensure your changes pass linting and tests before submitting a PR:\n ```shell\n make checks\n ```\n\n## \ud83d\ude4f Acknowledgements\n\n- [fast-plate-ocr](https://github.com/ankandrew/fast-plate-ocr) for default **OCR** models.\n- [open-image-models](https://github.com/ankandrew/open-image-models) for default plate **detection** models.\n\n## \ud83d\udceb Contact\n\nFor questions or suggestions, feel free to open an issue or reach out through social networks.\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Fast Automatic License Plate Recognition.",
"version": "0.1.1",
"project_urls": null,
"split_keywords": [
"image-processing",
" computer-vision",
" deep-learning",
" object-detection",
" plate-detection",
" license-plate-ocr",
" onnx"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "f792bf34980b3021aa6fea19a95fe173fc26c8d76cfed852a5a88a60959e5d29",
"md5": "f36721b2c55ab4b7f221b2b097a6ceab",
"sha256": "462fd84c6a3e803e31f6c6a25ab8d1106dc76e53ed7934c6ce60c2e7bce64671"
},
"downloads": -1,
"filename": "fast_alpr-0.1.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "f36721b2c55ab4b7f221b2b097a6ceab",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.10",
"size": 10199,
"upload_time": "2024-11-12T03:30:51",
"upload_time_iso_8601": "2024-11-12T03:30:51.692326Z",
"url": "https://files.pythonhosted.org/packages/f7/92/bf34980b3021aa6fea19a95fe173fc26c8d76cfed852a5a88a60959e5d29/fast_alpr-0.1.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d5c2152228f97f640ad3dd4617de8bae3af93eab888ab2104c3d8fefa9a1654c",
"md5": "0eb67ec694f4bae231c2a3599824e6a5",
"sha256": "febf1ee6500e1b5043288e18a7dc87ca0a0b50435387cc397c5d84ec631392fc"
},
"downloads": -1,
"filename": "fast_alpr-0.1.1.tar.gz",
"has_sig": false,
"md5_digest": "0eb67ec694f4bae231c2a3599824e6a5",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.10",
"size": 10475,
"upload_time": "2024-11-12T03:30:53",
"upload_time_iso_8601": "2024-11-12T03:30:53.455687Z",
"url": "https://files.pythonhosted.org/packages/d5/c2/152228f97f640ad3dd4617de8bae3af93eab888ab2104c3d8fefa9a1654c/fast_alpr-0.1.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-11-12 03:30:53",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "fast-alpr"
}