Name | fastapi-analytics JSON |
Version |
1.2.2
JSON |
| download |
home_page | https://github.com/tom-draper/api-analytics |
Summary | Monitoring and analytics for FastAPI applications. |
upload_time | 2024-01-31 19:35:35 |
maintainer | |
docs_url | None |
author | Tom Draper |
requires_python | >=3.6 |
license | MIT License Copyright (c) 2023 Tom Draper Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. |
keywords |
analytics
api
dashboard
fastapi
middleware
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# FastAPI Analytics
A free lightweight API analytics solution, complete with a dashboard.
## Getting Started
### 1. Generate an API key
Head to https://apianalytics.dev/generate to generate your unique API key with a single click. This key is used to monitor your API server and should be stored privately. It's also required in order to view your API analytics dashboard and data.
### 2. Add middleware to your API
Add our lightweight middleware to your API. Almost all processing is handled by our servers so there is minimal impact on the performance of your API.
[](https://pypi.com/project/api-analytics)
```bash
pip install fastapi-analytics
```
```py
import uvicorn
from fastapi import FastAPI
from api_analytics.fastapi import Analytics
app = FastAPI()
app.add_middleware(Analytics, api_key=<API-KEY>) # Add middleware
@app.get('/')
async def root():
return {'message': 'Hello World!'}
if __name__ == "__main__":
uvicorn.run("app:app", reload=True)
```
Custom mapping functions can be provided to override the default behaviour and tailor the retrival of information about each incoming request to your API's environment and usage.
```py
from fastapi import FastAPI
from api_analytics.fastapi import Analytics, Config
config = Config()
config.get_ip_address = lambda request: request.headers.get('X-Forwarded-For', request.client.host)
config.get_user_agent = lambda request: request.headers.get('User-Agent', '')
app = FastAPI()
app.add_middleware(Analytics, api_key=<API-KEY>, config=config) # Add middleware
```
### 3. View your analytics
Your API will now log and store incoming request data on all valid routes. Your logged data can be viewed using two methods:
1. Through visualizations and statistics on our dashboard
2. Accessed directly via our data API
You can use the same API key across multiple APIs, but all your data will appear in the same dashboard. We recommend generating a new API key for each additional API server you want analytics for.
#### Dashboard
Head to https://apianalytics.dev/dashboard and paste in your API key to access your dashboard.
Demo: https://apianalytics.dev/dashboard/demo
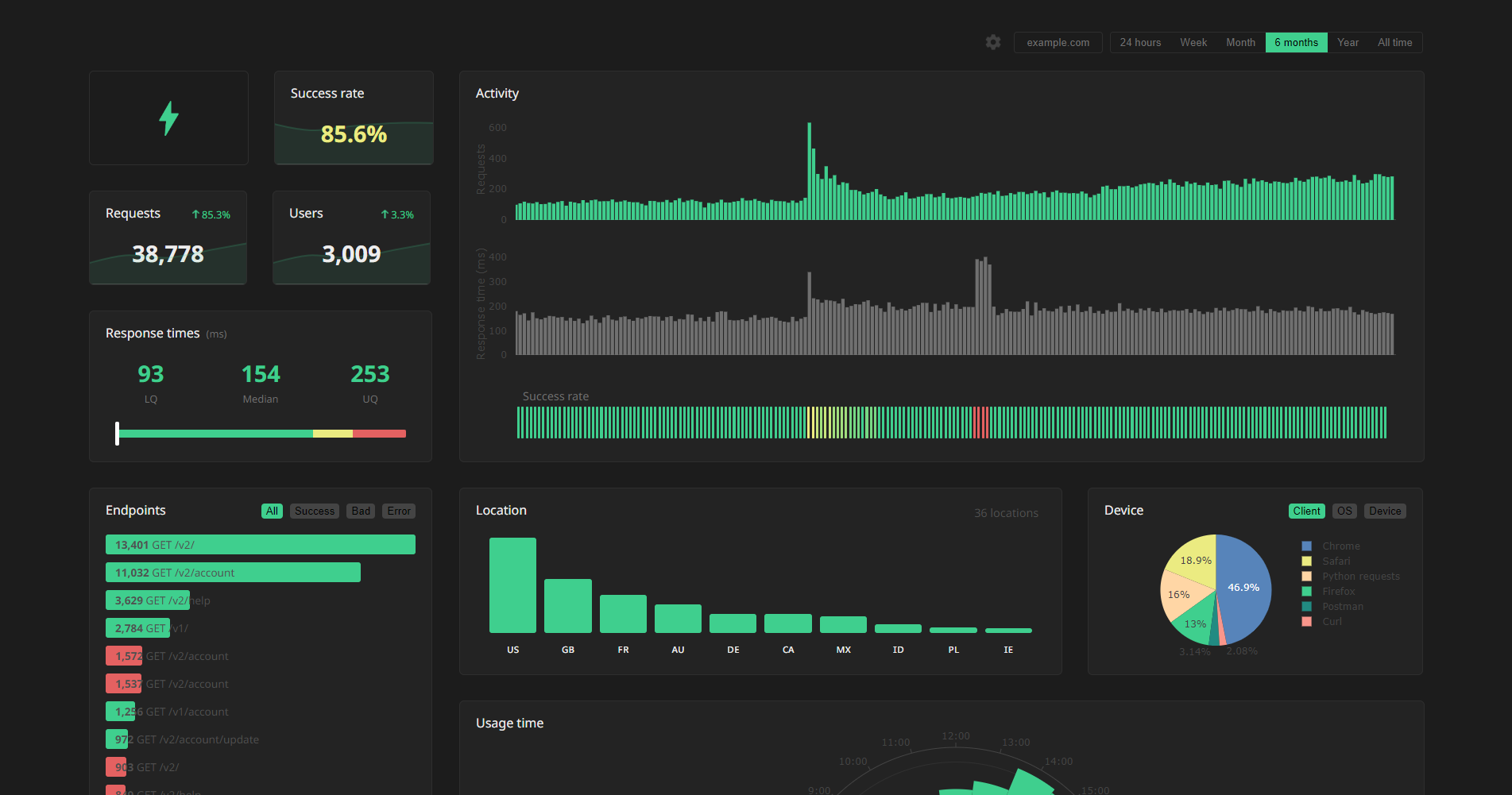
#### Data API
Logged data for all requests can be accessed via our REST API. Simply send a GET request to `https://apianalytics-server.com/api/data` with your API key set as `X-AUTH-TOKEN` in headers.
##### Python
```py
import requests
headers = {
"X-AUTH-TOKEN": <API-KEY>
}
response = requests.get("https://apianalytics-server.com/api/data", headers=headers)
print(response.json())
```
##### Node.js
```js
fetch("https://apianalytics-server.com/api/data", {
headers: { "X-AUTH-TOKEN": <API-KEY> },
})
.then((response) => {
return response.json();
})
.then((data) => {
console.log(data);
});
```
##### cURL
```bash
curl --header "X-AUTH-TOKEN: <API-KEY>" https://apianalytics-server.com/api/data
```
##### Parameters
You can filter your data by providing URL parameters in your request.
- `date` - specifies a particular day the requests occurred on (`YYYY-MM-DD`)
- `dateFrom` - specifies the lower bound of a date range the requests occurred in (`YYYY-MM-DD`)
- `dateTo` - specifies the upper bound of a date range the requests occurred in (`YYYY-MM-DD`)
- `ipAddress` - an IP address string of the client
- `status` - an integer status code of the response
- `location` - a two-character location code of the client
Example:
```bash
curl --header "X-AUTH-TOKEN: <API-KEY>" https://apianalytics-server.com/api/data?dateFrom=2022-01-01&dateTo=2022-06-01&status=200
```
## Monitoring
Active API monitoring can be set up by heading to https://apianalytics.dev/monitoring to enter you API key. Our servers will regularly ping chosen API endpoints to monitor uptime and response time.
<!-- Optional email alerts when your endpoints are down can be subscribed to. -->
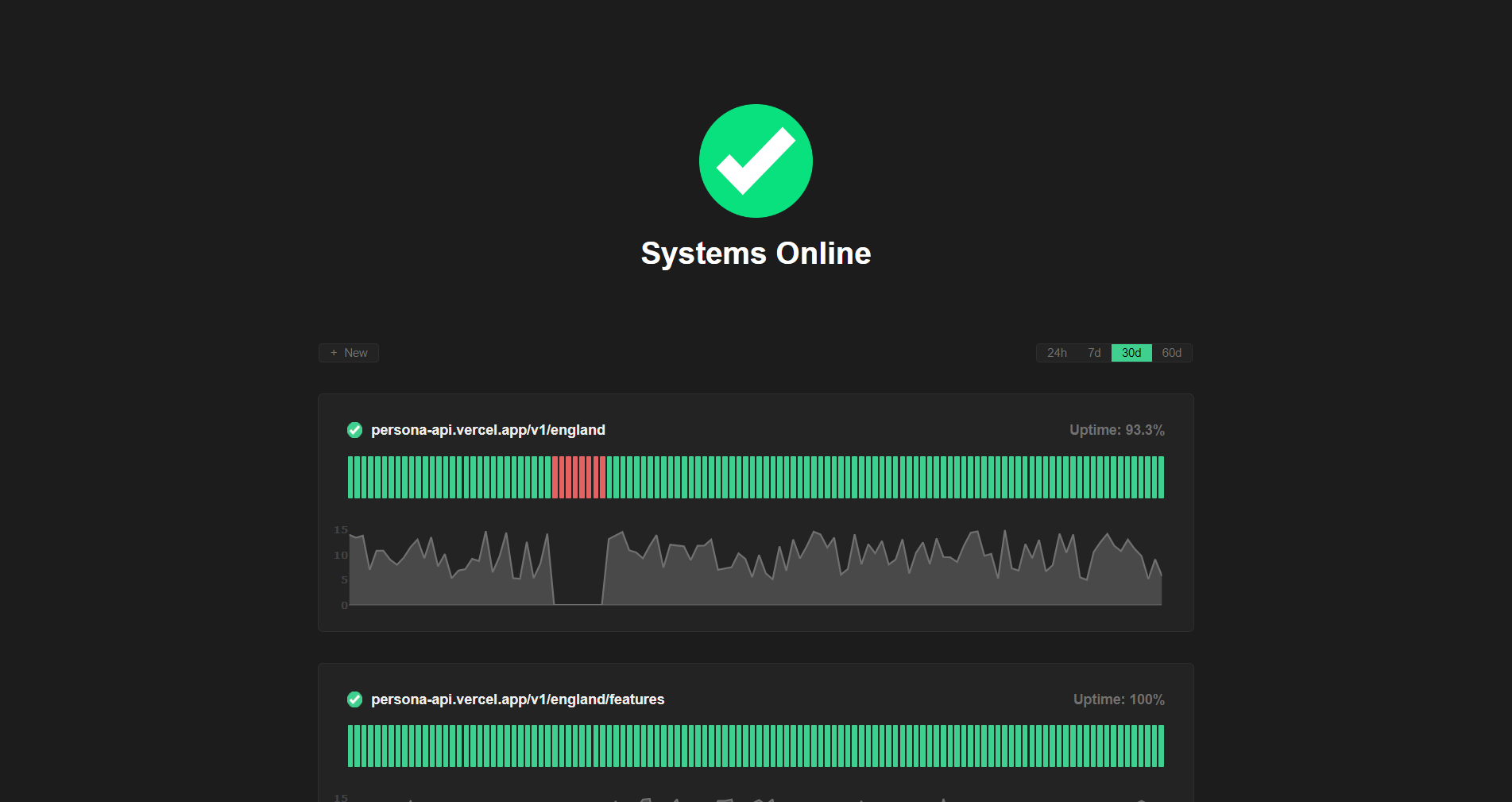
## Data and Security
All data is stored securely in compliance with The EU General Data Protection Regulation (GDPR).
For any given request to your API, data recorded is limited to:
- Path requested by client
- Client IP address
- Client operating system
- Client browser
- Request method (GET, POST, PUT, etc.)
- Time of request
- Status code
- Response time
- API hostname
- API framework (FastAPI, Flask, Express etc.)
Data collected is only ever used to populate your analytics dashboard. All stored data is anonymous, with the API key the only link between you and your logged request data. Should you lose your API key, you will have no method to access your API analytics.
### Data Deletion
At any time you can delete all stored data associated with your API key by going to https://apianalytics.dev/delete and entering your API key.
API keys and their associated logged request data are scheduled to be deleted after 6 months of inactivity.
## Contributions
Contributions, issues and feature requests are welcome.
- Fork it (https://github.com/tom-draper/api-analytics)
- Create your feature branch (`git checkout -b my-new-feature`)
- Commit your changes (`git commit -am 'Add some feature'`)
- Push to the branch (`git push origin my-new-feature`)
- Create a new Pull Request
Raw data
{
"_id": null,
"home_page": "https://github.com/tom-draper/api-analytics",
"name": "fastapi-analytics",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "analytics,api,dashboard,fastapi,middleware",
"author": "Tom Draper",
"author_email": "Tom Draper <tomjdraper1@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/0b/a1/7aba8341c2f7dbd23cf305e4b498c9cd0aec6d83b670aa23937f9ca14b1e/fastapi-analytics-1.2.2.tar.gz",
"platform": null,
"description": "# FastAPI Analytics\r\n\r\nA free lightweight API analytics solution, complete with a dashboard.\r\n\r\n## Getting Started\r\n\r\n### 1. Generate an API key\r\n\r\nHead to https://apianalytics.dev/generate to generate your unique API key with a single click. This key is used to monitor your API server and should be stored privately. It's also required in order to view your API analytics dashboard and data.\r\n\r\n### 2. Add middleware to your API\r\n\r\nAdd our lightweight middleware to your API. Almost all processing is handled by our servers so there is minimal impact on the performance of your API.\r\n\r\n[](https://pypi.com/project/api-analytics)\r\n\r\n```bash\r\npip install fastapi-analytics\r\n```\r\n\r\n```py\r\nimport uvicorn\r\nfrom fastapi import FastAPI\r\nfrom api_analytics.fastapi import Analytics\r\n\r\napp = FastAPI()\r\napp.add_middleware(Analytics, api_key=<API-KEY>) # Add middleware\r\n\r\n@app.get('/')\r\nasync def root():\r\n return {'message': 'Hello World!'}\r\n\r\nif __name__ == \"__main__\":\r\n uvicorn.run(\"app:app\", reload=True)\r\n```\r\n\r\nCustom mapping functions can be provided to override the default behaviour and tailor the retrival of information about each incoming request to your API's environment and usage.\r\n\r\n```py\r\nfrom fastapi import FastAPI\r\nfrom api_analytics.fastapi import Analytics, Config\r\n\r\nconfig = Config()\r\nconfig.get_ip_address = lambda request: request.headers.get('X-Forwarded-For', request.client.host)\r\nconfig.get_user_agent = lambda request: request.headers.get('User-Agent', '')\r\n\r\napp = FastAPI()\r\napp.add_middleware(Analytics, api_key=<API-KEY>, config=config) # Add middleware\r\n```\r\n\r\n### 3. View your analytics\r\n\r\nYour API will now log and store incoming request data on all valid routes. Your logged data can be viewed using two methods:\r\n\r\n1. Through visualizations and statistics on our dashboard\r\n2. Accessed directly via our data API\r\n\r\nYou can use the same API key across multiple APIs, but all your data will appear in the same dashboard. We recommend generating a new API key for each additional API server you want analytics for.\r\n\r\n#### Dashboard\r\n\r\nHead to https://apianalytics.dev/dashboard and paste in your API key to access your dashboard.\r\n\r\nDemo: https://apianalytics.dev/dashboard/demo\r\n\r\n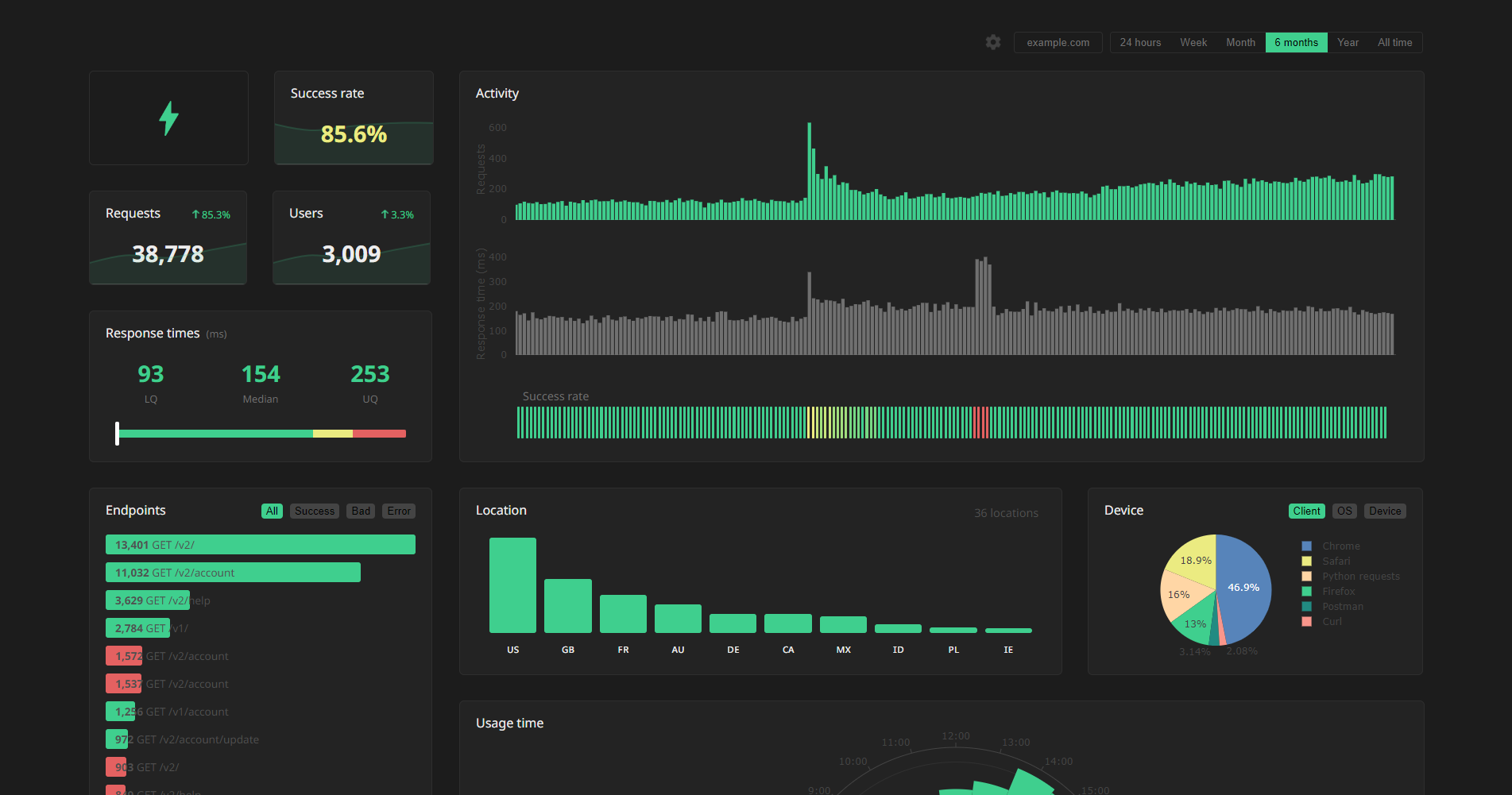\r\n\r\n#### Data API\r\n\r\nLogged data for all requests can be accessed via our REST API. Simply send a GET request to `https://apianalytics-server.com/api/data` with your API key set as `X-AUTH-TOKEN` in headers.\r\n\r\n##### Python\r\n\r\n```py\r\nimport requests\r\n\r\nheaders = {\r\n \"X-AUTH-TOKEN\": <API-KEY>\r\n}\r\n\r\nresponse = requests.get(\"https://apianalytics-server.com/api/data\", headers=headers)\r\nprint(response.json())\r\n```\r\n\r\n##### Node.js\r\n\r\n```js\r\nfetch(\"https://apianalytics-server.com/api/data\", {\r\n headers: { \"X-AUTH-TOKEN\": <API-KEY> },\r\n})\r\n .then((response) => {\r\n return response.json();\r\n })\r\n .then((data) => {\r\n console.log(data);\r\n });\r\n```\r\n\r\n##### cURL\r\n\r\n```bash\r\ncurl --header \"X-AUTH-TOKEN: <API-KEY>\" https://apianalytics-server.com/api/data\r\n```\r\n\r\n##### Parameters\r\n\r\nYou can filter your data by providing URL parameters in your request.\r\n\r\n- `date` - specifies a particular day the requests occurred on (`YYYY-MM-DD`)\r\n- `dateFrom` - specifies the lower bound of a date range the requests occurred in (`YYYY-MM-DD`)\r\n- `dateTo` - specifies the upper bound of a date range the requests occurred in (`YYYY-MM-DD`)\r\n- `ipAddress` - an IP address string of the client\r\n- `status` - an integer status code of the response\r\n- `location` - a two-character location code of the client\r\n\r\nExample:\r\n\r\n```bash\r\ncurl --header \"X-AUTH-TOKEN: <API-KEY>\" https://apianalytics-server.com/api/data?dateFrom=2022-01-01&dateTo=2022-06-01&status=200\r\n```\r\n\r\n## Monitoring\r\n\r\nActive API monitoring can be set up by heading to https://apianalytics.dev/monitoring to enter you API key. Our servers will regularly ping chosen API endpoints to monitor uptime and response time. \r\n<!-- Optional email alerts when your endpoints are down can be subscribed to. -->\r\n\r\n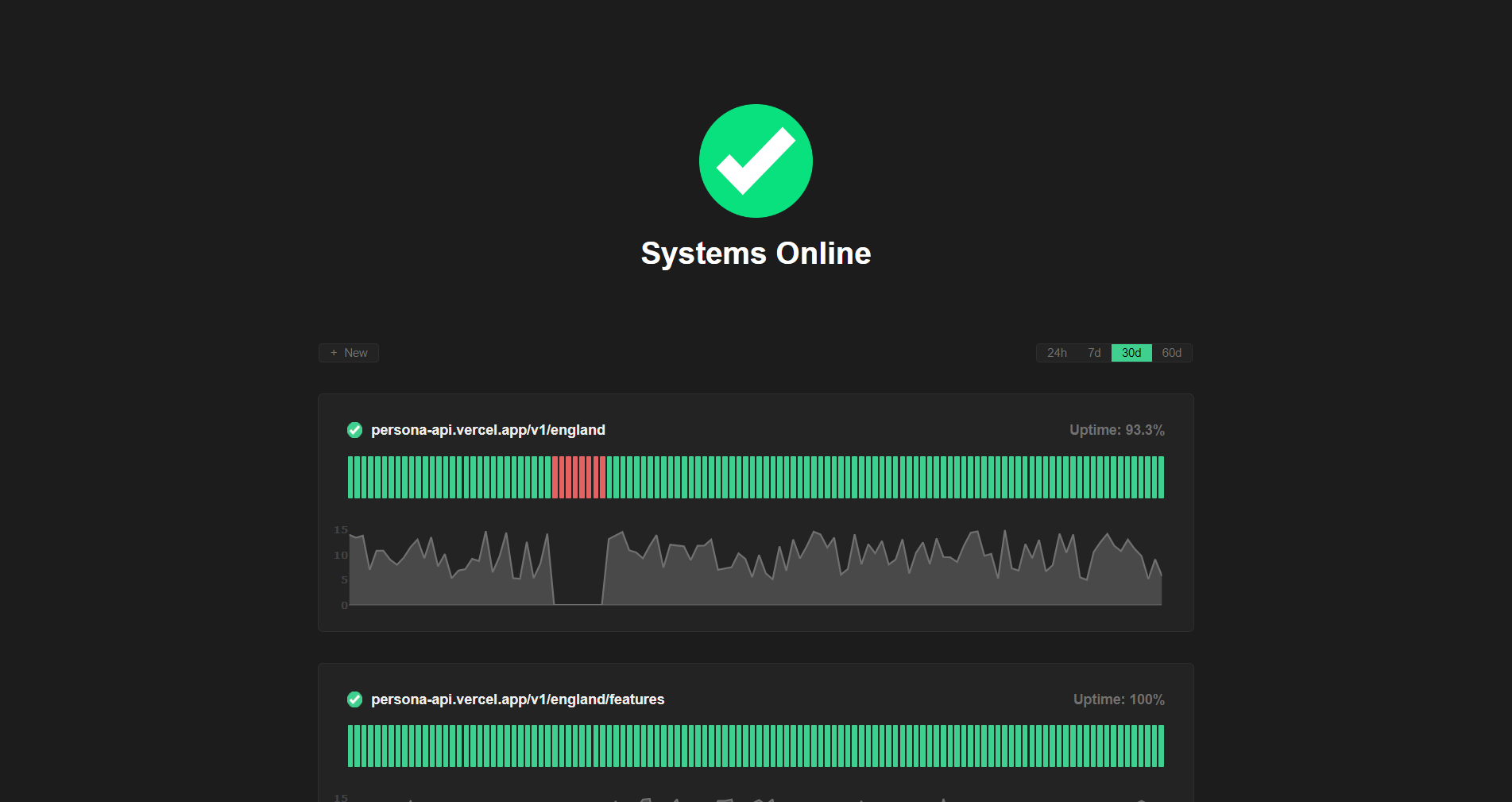\r\n\r\n## Data and Security\r\n\r\nAll data is stored securely in compliance with The EU General Data Protection Regulation (GDPR).\r\n\r\nFor any given request to your API, data recorded is limited to:\r\n - Path requested by client\r\n - Client IP address\r\n - Client operating system\r\n - Client browser\r\n - Request method (GET, POST, PUT, etc.)\r\n - Time of request\r\n - Status code\r\n - Response time\r\n - API hostname\r\n - API framework (FastAPI, Flask, Express etc.)\r\n\r\nData collected is only ever used to populate your analytics dashboard. All stored data is anonymous, with the API key the only link between you and your logged request data. Should you lose your API key, you will have no method to access your API analytics.\r\n\r\n### Data Deletion\r\n\r\nAt any time you can delete all stored data associated with your API key by going to https://apianalytics.dev/delete and entering your API key.\r\n\r\nAPI keys and their associated logged request data are scheduled to be deleted after 6 months of inactivity.\r\n\r\n## Contributions\r\n\r\nContributions, issues and feature requests are welcome.\r\n\r\n- Fork it (https://github.com/tom-draper/api-analytics)\r\n- Create your feature branch (`git checkout -b my-new-feature`)\r\n- Commit your changes (`git commit -am 'Add some feature'`)\r\n- Push to the branch (`git push origin my-new-feature`)\r\n- Create a new Pull Request\r\n",
"bugtrack_url": null,
"license": "MIT License Copyright (c) 2023 Tom Draper Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the \"Software\"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions: The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software. THE SOFTWARE IS PROVIDED \"AS IS\", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.",
"summary": "Monitoring and analytics for FastAPI applications.",
"version": "1.2.2",
"project_urls": {
"Homepage": "https://github.com/tom-draper/api-analytics",
"repository": "https://github.com/tom-draper/api-analytics"
},
"split_keywords": [
"analytics",
"api",
"dashboard",
"fastapi",
"middleware"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "f61d331c810d8395b1e4728c28e11226cc47baee157297c59c99e2b7246d4892",
"md5": "9f8579eea3a02479b2780e126326794e",
"sha256": "720ffa6d8eb63662831d4cd040083e09a4c6516dac4f9037c2d1c5c2aa3d7777"
},
"downloads": -1,
"filename": "fastapi_analytics-1.2.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "9f8579eea3a02479b2780e126326794e",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.6",
"size": 7338,
"upload_time": "2024-01-31T19:35:33",
"upload_time_iso_8601": "2024-01-31T19:35:33.463894Z",
"url": "https://files.pythonhosted.org/packages/f6/1d/331c810d8395b1e4728c28e11226cc47baee157297c59c99e2b7246d4892/fastapi_analytics-1.2.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "0ba17aba8341c2f7dbd23cf305e4b498c9cd0aec6d83b670aa23937f9ca14b1e",
"md5": "54f432950702e82885c73c366ec7fc98",
"sha256": "25e32dcaa5488a1d8c866b58711715bfc688cdf88e480786ef28afcd62ad39c3"
},
"downloads": -1,
"filename": "fastapi-analytics-1.2.2.tar.gz",
"has_sig": false,
"md5_digest": "54f432950702e82885c73c366ec7fc98",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 6570,
"upload_time": "2024-01-31T19:35:35",
"upload_time_iso_8601": "2024-01-31T19:35:35.178124Z",
"url": "https://files.pythonhosted.org/packages/0b/a1/7aba8341c2f7dbd23cf305e4b498c9cd0aec6d83b670aa23937f9ca14b1e/fastapi-analytics-1.2.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-01-31 19:35:35",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "tom-draper",
"github_project": "api-analytics",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"lcname": "fastapi-analytics"
}