[简体中文](https://github.com/amisadmin/fastapi_amis_admin/blob/master/README.zh.md)
| [English](https://github.com/amisadmin/fastapi_amis_admin)
# Introduction
<h2 align="center">
FastAPI-Amis-Admin
</h2>
<p align="center">
<em>fastapi-amis-admin is a high-performance, efficient and easily extensible FastAPI admin framework.</em><br/>
<em>Inspired by Django-admin, and has as many powerful functions as Django-admin.</em>
</p>
<p align="center">
<a href="https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml" target="_blank">
<img src="https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml/badge.svg" alt="Pytest">
</a>
<a href="https://pypi.org/project/fastapi_amis_admin" target="_blank">
<img src="https://badgen.net/pypi/v/fastapi-amis-admin?color=blue" alt="Package version">
</a>
<a href="https://pepy.tech/project/fastapi-amis-admin" target="_blank">
<img src="https://pepy.tech/badge/fastapi-amis-admin" alt="Downloads">
</a>
<a href="https://gitter.im/amisadmin/fastapi-amis-admin">
<img src="https://badges.gitter.im/amisadmin/fastapi-amis-admin.svg" alt="Chat on Gitter"/>
</a>
</p>
<p align="center">
<a href="https://github.com/amisadmin/fastapi_amis_admin" target="_blank">source code</a>
·
<a href="http://demo.amis.work/admin" target="_blank">online demo</a>
·
<a href="http://docs.gh.amis.work" target="_blank">documentation</a>
·
<a href="http://docs.amis.work" target="_blank">can't open the document?</a>
</p>
------
`fastapi-amis-admin` is a high-performance and efficient framework based on `fastapi` & `amis` with `Python 3.7+`, and
based on standard Python type hints. The original intention of the development is to improve the application ecology and
to quickly generate a visual dashboard for the web application . According to the `Apache2.0` protocol, it is free and
open source . But in order to better operate and maintain this project in the long run, I very much hope to get
everyone's sponsorship and support.
## Features
- **High performance**: Based on [FastAPI](https://fastapi.tiangolo.com/). Enjoy all the benefits.
- **High efficiency**: Perfect code type hints. Higher code reusability.
- **Support asynchronous and synchronous hybrid writing**: `ORM` is based on`SQLModel` & `Sqlalchemy`. Freely customize
database type. Support synchronous and asynchronous mode. Strong scalability.
- **Front-end separation**: The front-end is rendered by `Amis`, the back-end interface is automatically generated
by `fastapi-amis-admin`. The interface is reusable.
- **Strong scalability**: The background page supports `Amis` pages and ordinary `html` pages. Easily customize the
interface freely.
- **Automatic api documentation**: Automatically generate Interface documentation by `FastAPI`. Easily debug and share
interfaces.
## Dependencies
- [FastAPI](https://fastapi.tiangolo.com/): Finish the web part.
- [SQLModel](https://sqlmodel.tiangolo.com/): Finish `ORM` model mapping. Perfectly
combine [SQLAlchemy](https://www.sqlalchemy.org/) with [Pydantic](https://pydantic-docs.helpmanual.io/), and have all
their features .
- [Amis](https://baidu.gitee.io/amis): Finish admin page presentation.
## Composition
`fastapi-amis-admin` consists of three core modules, of which, `amis`, `crud` can be used as separate
modules, `admin` is developed by the former.
- `amis`: Based on the `pydantic` data model building library of `baidu amis`. To generate/parse data rapidly.
- `crud`: Based on `FastAPI` &`Sqlalchemy`. To quickly build Create, Read, Update, Delete common API
interface .
- `admin`: Inspired by `Django-Admin`. Combine `amis` with `crud`. To quickly build Web Admin
dashboard .
## Installation
```bash
pip install fastapi_amis_admin
```
### Note
- After version `fastapi-amis-admin>=0.6.0`, `sqlmodel` is no longer a required dependency library. If you use `sqlmodel`
to create a model, you can install it with the following command.
```bash
pip install fastapi_amis_admin[sqlmodel]
```
## Simple Example
```python
from fastapi import FastAPI
from fastapi_amis_admin.admin.settings import Settings
from fastapi_amis_admin.admin.site import AdminSite
# create FastAPI application
app = FastAPI()
# create AdminSite instance
site = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))
# mount AdminSite instance
site.mount_app(app)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app)
```
## ModelAdmin Example
### Create Model
- Support `SQLModel` model, `SQLAlchemy` model, `SQLAlchemy 2.0` model
- Method 1: Create model through `SQLModel`.
```python
from typing import Optional
from sqlmodel import SQLModel
from fastapi_amis_admin.models.fields import Field
class Base(SQLModel):
pass
# Create an SQLModel, see document for details: https://sqlmodel.tiangolo.com/
class Category(SQLModel, table=True):
id: Optional[int] = Field(default=None, primary_key=True, nullable=False)
name: str = Field(title='CategoryName', max_length=100, unique=True, index=True, nullable=False)
description: str = Field(default='', title='Description', max_length=255)
```
- Method 2: Create model through `SQLAlchemy`.
```python
from sqlalchemy import Column, Integer, String
from sqlalchemy.ext.declarative import declarative_base
Base = declarative_base()
# Create an SQLAlchemy model, see document for details: https://docs.sqlalchemy.org/en/14/orm/tutorial.html
class Category(Base):
__tablename__ = 'category'
# Specify the Schema class corresponding to the model. It is recommended to specify it. If omitted, it can be automatically generated.
__pydantic_model__ = CategorySchema
id = Column(Integer, primary_key=True, nullable=False)
name = Column(String(100), unique=True, index=True, nullable=False)
description = Column(String(255), default='')
```
- Method 3: Create model through `SQLAlchemy 2.0`.
```python
from sqlalchemy import String
from sqlalchemy.orm import DeclarativeBase, Mapped, mapped_column
class Base(DeclarativeBase):
pass
# Create an SQLAlchemy 2.0 model, see document for details: https://docs.sqlalchemy.org/en/20/orm/quickstart.html
class Category(Base):
__tablename__ = "category"
# Specify the Schema class corresponding to the model. It is recommended to specify it. If omitted, it can be automatically generated.
__pydantic_model__ = CategorySchema
id: Mapped[int] = mapped_column(primary_key=True, nullable=False)
name: Mapped[str] = mapped_column(String(100), unique=True, index=True, nullable=False)
description: Mapped[str] = mapped_column(String(255), default="")
```
- If you create a model through `sqlalchemy`, it is recommended to create a corresponding pydantic model at the same
time, and set `orm_mode=True`.
```python
from pydantic import BaseModel, Field
class CategorySchema(BaseModel):
id: Optional[int] = Field(default=None, primary_key=True, nullable=False)
name: str = Field(title="CategoryName")
description: str = Field(default="", title="CategoryDescription")
class Config:
orm_mode = True
```
### Register ModelAdmin
```python
from fastapi import FastAPI
from fastapi_amis_admin.admin.settings import Settings
from fastapi_amis_admin.admin.site import AdminSite
from fastapi_amis_admin.admin import admin
# create FastAPI application
app = FastAPI()
# create AdminSite instance
site = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))
# register ModelAdmin
@site.register_admin
class CategoryAdmin(admin.ModelAdmin):
page_schema = 'Category'
# set model
model = Category
# mount AdminSite instance
site.mount_app(app)
# create initial database table
@app.on_event("startup")
async def startup():
await site.db.async_run_sync(Base.metadata.create_all, is_session=False)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app)
```
## FormAdmin Example
```python
from typing import Any
from fastapi import FastAPI
from pydantic import BaseModel
from starlette.requests import Request
from fastapi_amis_admin.amis.components import Form
from fastapi_amis_admin.admin import admin
from fastapi_amis_admin.admin.settings import Settings
from fastapi_amis_admin.admin.site import AdminSite
from fastapi_amis_admin.crud.schema import BaseApiOut
from fastapi_amis_admin.models.fields import Field
# create FastAPI application
app = FastAPI()
# create AdminSite instance
site = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))
# register FormAdmin
@site.register_admin
class UserLoginFormAdmin(admin.FormAdmin):
page_schema = 'UserLoginForm'
# set form information, optional
form = Form(title='This is a test login form', submitText='login')
# create form schema
class schema(BaseModel):
username: str = Field(..., title='username', min_length=3, max_length=30)
password: str = Field(..., title='password')
# handle form submission data
async def handle(self, request: Request, data: BaseModel, **kwargs) -> BaseApiOut[Any]:
if data.username == 'amisadmin' and data.password == 'amisadmin':
return BaseApiOut(msg='Login successfully!', data={'token': 'xxxxxx'})
return BaseApiOut(status=-1, msg='Incorrect username or password!')
# mount AdminSite instance
site.mount_app(app)
if __name__ == '__main__':
import uvicorn
uvicorn.run(app)
```
## Working with Command
```bash
# Install command line extension
pip install fastapi_amis_admin[cli]
# View help
faa --help
# Initialize a `FastAPI-Amis-Admin` project
faa new project_name --init
# Initialize a `FastAPI-Amis-Admin` application
faa new app_name
# Fast running project
faa run
```
## Preview
- Open `http://127.0.0.1:8000/admin/` in your browser:
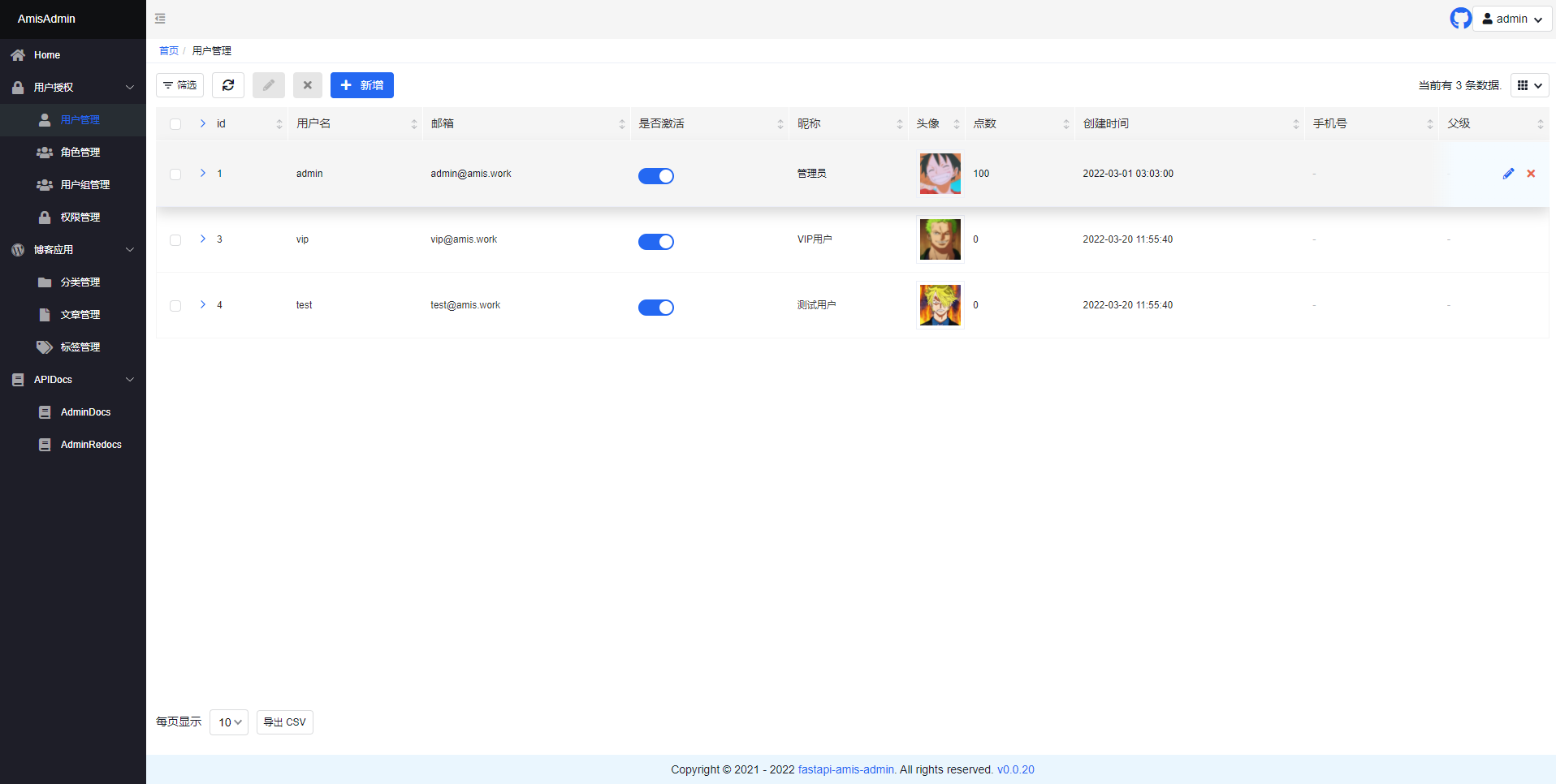
- Open `http://127.0.0.1:8000/admin/docs` in your browser:
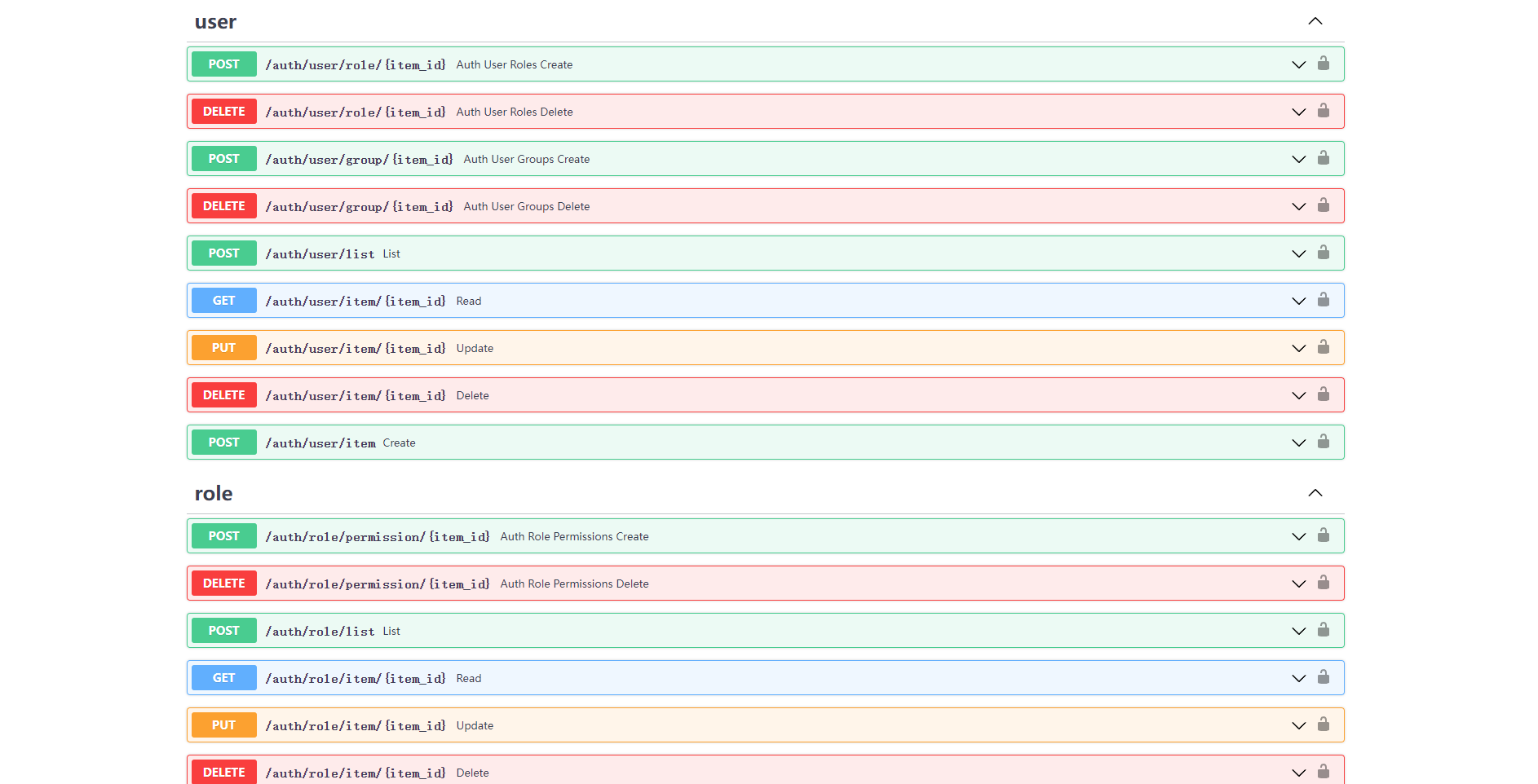
## Project
- [`Amis-Admin-Theme-Editor`](https://github.com/swelcker/amis-admin-theme-editor):Theme-Editor for the fastapi-amis-admin.
Allows to add custom css styles and to apply theme --vars change on the fly.
- [`FastAPI-User-Auth`](https://github.com/amisadmin/fastapi_user_auth): A simple and powerful `FastAPI` user `RBAC`
authentication and authorization library.
- [`FastAPI-Scheduler`](https://github.com/amisadmin/fastapi_scheduler): A simple scheduled task management `FastAPI` extension
based on `APScheduler`.
- [`FastAPI-Config`](https://github.com/amisadmin/fastapi-config): A visual dynamic configuration management extension package
based on `FastAPI-Amis-Admin`.
- [`FastAPI-Amis-Admin-Demo`](https://github.com/amisadmin/fastapi_amis_admin_demo): An example `FastAPI-Amis-Admin` application.
- [`FastAPI-User-Auth-Demo`](https://github.com/amisadmin/fastapi_user_auth_demo): An example `FastAPI-User-Auth` application.
## License
- According to the `Apache2.0` protocol, `fastapi-amis-admin` is free and open source. It can be used for commercial for
free, but please clearly display copyright information about `FastAPI-Amis-Admin` on the display interface.
Raw data
{
"_id": null,
"home_page": null,
"name": "fastapi_amis_admin",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "Atomi <1456417373@qq.com>",
"keywords": "fastapi, fastapi-admin, fastapi-amis-admin, django-admin, sqlmodel, sqlalchemy",
"author": null,
"author_email": "Atomi <1456417373@qq.com>",
"download_url": "https://files.pythonhosted.org/packages/f6/ef/9920511d02c5c1dbac288744fd1c7447b6fa8a204fc864029f0ad1a1afb8/fastapi_amis_admin-0.7.2.tar.gz",
"platform": null,
"description": "[\u7b80\u4f53\u4e2d\u6587](https://github.com/amisadmin/fastapi_amis_admin/blob/master/README.zh.md)\n| [English](https://github.com/amisadmin/fastapi_amis_admin)\n\n# Introduction\n\n<h2 align=\"center\">\n FastAPI-Amis-Admin\n</h2>\n<p align=\"center\">\n <em>fastapi-amis-admin is a high-performance, efficient and easily extensible FastAPI admin framework.</em><br/>\n <em>Inspired by Django-admin, and has as many powerful functions as Django-admin.</em>\n</p>\n<p align=\"center\">\n <a href=\"https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml\" target=\"_blank\">\n <img src=\"https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml/badge.svg\" alt=\"Pytest\">\n </a>\n <a href=\"https://pypi.org/project/fastapi_amis_admin\" target=\"_blank\">\n <img src=\"https://badgen.net/pypi/v/fastapi-amis-admin?color=blue\" alt=\"Package version\">\n </a>\n <a href=\"https://pepy.tech/project/fastapi-amis-admin\" target=\"_blank\">\n <img src=\"https://pepy.tech/badge/fastapi-amis-admin\" alt=\"Downloads\">\n </a>\n <a href=\"https://gitter.im/amisadmin/fastapi-amis-admin\">\n <img src=\"https://badges.gitter.im/amisadmin/fastapi-amis-admin.svg\" alt=\"Chat on Gitter\"/>\n </a>\n</p>\n<p align=\"center\">\n <a href=\"https://github.com/amisadmin/fastapi_amis_admin\" target=\"_blank\">source code</a>\n \u00b7\n <a href=\"http://demo.amis.work/admin\" target=\"_blank\">online demo</a>\n \u00b7\n <a href=\"http://docs.gh.amis.work\" target=\"_blank\">documentation</a>\n \u00b7\n <a href=\"http://docs.amis.work\" target=\"_blank\">can't open the document?</a>\n</p>\n\n\n------\n\n`fastapi-amis-admin` is a high-performance and efficient framework based on `fastapi` & `amis` with `Python 3.7+`, and\nbased on standard Python type hints. The original intention of the development is to improve the application ecology and\nto quickly generate a visual dashboard for the web application . According to the `Apache2.0` protocol, it is free and\nopen source . But in order to better operate and maintain this project in the long run, I very much hope to get\neveryone's sponsorship and support.\n\n## Features\n\n- **High performance**: Based on [FastAPI](https://fastapi.tiangolo.com/). Enjoy all the benefits.\n- **High efficiency**: Perfect code type hints. Higher code reusability.\n- **Support asynchronous and synchronous hybrid writing**: `ORM` is based on`SQLModel` & `Sqlalchemy`. Freely customize\n database type. Support synchronous and asynchronous mode. Strong scalability.\n- **Front-end separation**: The front-end is rendered by `Amis`, the back-end interface is automatically generated\n by `fastapi-amis-admin`. The interface is reusable.\n- **Strong scalability**: The background page supports `Amis` pages and ordinary `html` pages. Easily customize the\n interface freely.\n- **Automatic api documentation**: Automatically generate Interface documentation by `FastAPI`. Easily debug and share\n interfaces.\n\n## Dependencies\n\n- [FastAPI](https://fastapi.tiangolo.com/): Finish the web part.\n- [SQLModel](https://sqlmodel.tiangolo.com/): Finish `ORM` model mapping. Perfectly\n combine [SQLAlchemy](https://www.sqlalchemy.org/) with [Pydantic](https://pydantic-docs.helpmanual.io/), and have all\n their features .\n- [Amis](https://baidu.gitee.io/amis): Finish admin page presentation.\n\n## Composition\n\n`fastapi-amis-admin` consists of three core modules, of which, `amis`, `crud` can be used as separate\nmodules, `admin` is developed by the former.\n\n- `amis`: Based on the `pydantic` data model building library of `baidu amis`. To generate/parse data rapidly.\n- `crud`: Based on `FastAPI` &`Sqlalchemy`. To quickly build Create, Read, Update, Delete common API\n interface .\n- `admin`: Inspired by `Django-Admin`. Combine `amis` with `crud`. To quickly build Web Admin\n dashboard .\n\n## Installation\n\n```bash\npip install fastapi_amis_admin\n```\n\n### Note\n\n- After version `fastapi-amis-admin>=0.6.0`, `sqlmodel` is no longer a required dependency library. If you use `sqlmodel`\n to create a model, you can install it with the following command.\n\n```bash\npip install fastapi_amis_admin[sqlmodel]\n```\n\n## Simple Example\n\n```python\nfrom fastapi import FastAPI\nfrom fastapi_amis_admin.admin.settings import Settings\nfrom fastapi_amis_admin.admin.site import AdminSite\n\n# create FastAPI application\napp = FastAPI()\n\n# create AdminSite instance\nsite = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))\n\n# mount AdminSite instance\nsite.mount_app(app)\n\nif __name__ == '__main__':\n import uvicorn\n\n uvicorn.run(app)\n```\n\n## ModelAdmin Example\n\n### Create Model\n\n- Support `SQLModel` model, `SQLAlchemy` model, `SQLAlchemy 2.0` model\n- Method 1: Create model through `SQLModel`.\n\n```python\nfrom typing import Optional\nfrom sqlmodel import SQLModel\nfrom fastapi_amis_admin.models.fields import Field\n\n\nclass Base(SQLModel):\n pass\n\n\n# Create an SQLModel, see document for details: https://sqlmodel.tiangolo.com/\nclass Category(SQLModel, table=True):\n id: Optional[int] = Field(default=None, primary_key=True, nullable=False)\n name: str = Field(title='CategoryName', max_length=100, unique=True, index=True, nullable=False)\n description: str = Field(default='', title='Description', max_length=255)\n\n```\n\n- Method 2: Create model through `SQLAlchemy`.\n\n```python\nfrom sqlalchemy import Column, Integer, String\nfrom sqlalchemy.ext.declarative import declarative_base\n\nBase = declarative_base()\n\n\n# Create an SQLAlchemy model, see document for details: https://docs.sqlalchemy.org/en/14/orm/tutorial.html\nclass Category(Base):\n __tablename__ = 'category'\n # Specify the Schema class corresponding to the model. It is recommended to specify it. If omitted, it can be automatically generated.\n __pydantic_model__ = CategorySchema\n\n id = Column(Integer, primary_key=True, nullable=False)\n name = Column(String(100), unique=True, index=True, nullable=False)\n description = Column(String(255), default='')\n```\n\n- Method 3: Create model through `SQLAlchemy 2.0`.\n\n```python\nfrom sqlalchemy import String\nfrom sqlalchemy.orm import DeclarativeBase, Mapped, mapped_column\n\n\nclass Base(DeclarativeBase):\n pass\n\n\n# Create an SQLAlchemy 2.0 model, see document for details: https://docs.sqlalchemy.org/en/20/orm/quickstart.html\nclass Category(Base):\n __tablename__ = \"category\"\n # Specify the Schema class corresponding to the model. It is recommended to specify it. If omitted, it can be automatically generated.\n __pydantic_model__ = CategorySchema\n\n id: Mapped[int] = mapped_column(primary_key=True, nullable=False)\n name: Mapped[str] = mapped_column(String(100), unique=True, index=True, nullable=False)\n description: Mapped[str] = mapped_column(String(255), default=\"\")\n```\n\n- If you create a model through `sqlalchemy`, it is recommended to create a corresponding pydantic model at the same\n time, and set `orm_mode=True`.\n\n```python\nfrom pydantic import BaseModel, Field\n\n\nclass CategorySchema(BaseModel):\n id: Optional[int] = Field(default=None, primary_key=True, nullable=False)\n name: str = Field(title=\"CategoryName\")\n description: str = Field(default=\"\", title=\"CategoryDescription\")\n\n class Config:\n orm_mode = True\n```\n\n### Register ModelAdmin\n\n```python\nfrom fastapi import FastAPI\nfrom fastapi_amis_admin.admin.settings import Settings\nfrom fastapi_amis_admin.admin.site import AdminSite\nfrom fastapi_amis_admin.admin import admin\n\n# create FastAPI application\napp = FastAPI()\n\n# create AdminSite instance\nsite = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))\n\n\n# register ModelAdmin\n@site.register_admin\nclass CategoryAdmin(admin.ModelAdmin):\n page_schema = 'Category'\n # set model\n model = Category\n\n\n# mount AdminSite instance\nsite.mount_app(app)\n\n\n# create initial database table\n@app.on_event(\"startup\")\nasync def startup():\n await site.db.async_run_sync(Base.metadata.create_all, is_session=False)\n\n\nif __name__ == '__main__':\n import uvicorn\n\n uvicorn.run(app)\n```\n\n## FormAdmin Example\n\n```python\nfrom typing import Any\nfrom fastapi import FastAPI\nfrom pydantic import BaseModel\nfrom starlette.requests import Request\nfrom fastapi_amis_admin.amis.components import Form\nfrom fastapi_amis_admin.admin import admin\nfrom fastapi_amis_admin.admin.settings import Settings\nfrom fastapi_amis_admin.admin.site import AdminSite\nfrom fastapi_amis_admin.crud.schema import BaseApiOut\nfrom fastapi_amis_admin.models.fields import Field\n\n# create FastAPI application\napp = FastAPI()\n\n# create AdminSite instance\nsite = AdminSite(settings=Settings(database_url_async='sqlite+aiosqlite:///amisadmin.db'))\n\n\n# register FormAdmin\n@site.register_admin\nclass UserLoginFormAdmin(admin.FormAdmin):\n page_schema = 'UserLoginForm'\n # set form information, optional\n form = Form(title='This is a test login form', submitText='login')\n\n # create form schema\n class schema(BaseModel):\n username: str = Field(..., title='username', min_length=3, max_length=30)\n password: str = Field(..., title='password')\n\n # handle form submission data\n async def handle(self, request: Request, data: BaseModel, **kwargs) -> BaseApiOut[Any]:\n if data.username == 'amisadmin' and data.password == 'amisadmin':\n return BaseApiOut(msg='Login successfully!', data={'token': 'xxxxxx'})\n return BaseApiOut(status=-1, msg='Incorrect username or password!')\n\n\n# mount AdminSite instance\nsite.mount_app(app)\n\nif __name__ == '__main__':\n import uvicorn\n\n uvicorn.run(app)\n```\n\n## Working with Command\n\n```bash\n# Install command line extension\npip install fastapi_amis_admin[cli]\n\n# View help\nfaa --help\n\n# Initialize a `FastAPI-Amis-Admin` project\nfaa new project_name --init\n\n# Initialize a `FastAPI-Amis-Admin` application\nfaa new app_name\n\n# Fast running project\nfaa run\n```\n\n## Preview\n\n- Open `http://127.0.0.1:8000/admin/` in your browser:\n\n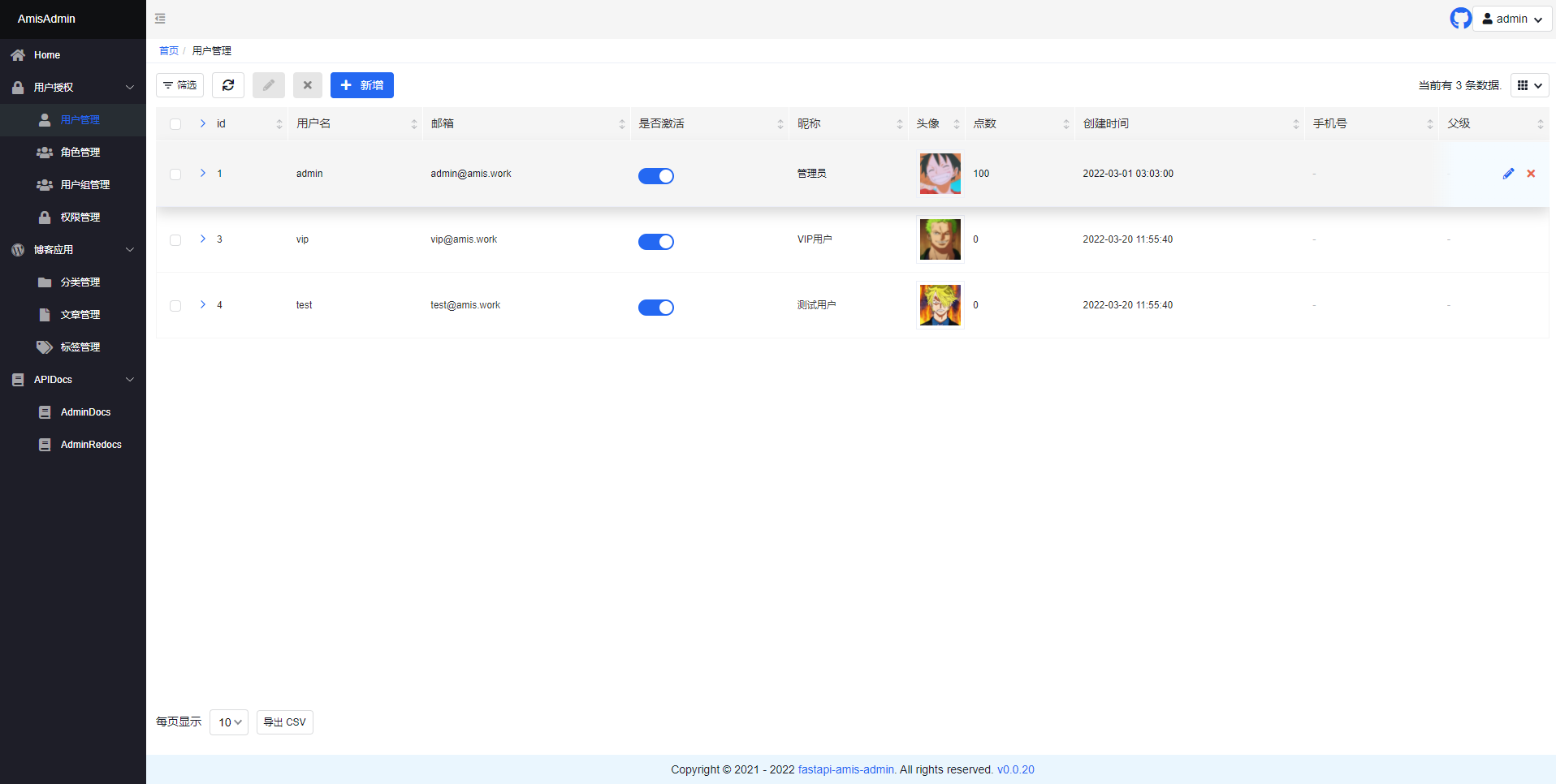\n\n- Open `http://127.0.0.1:8000/admin/docs` in your browser:\n\n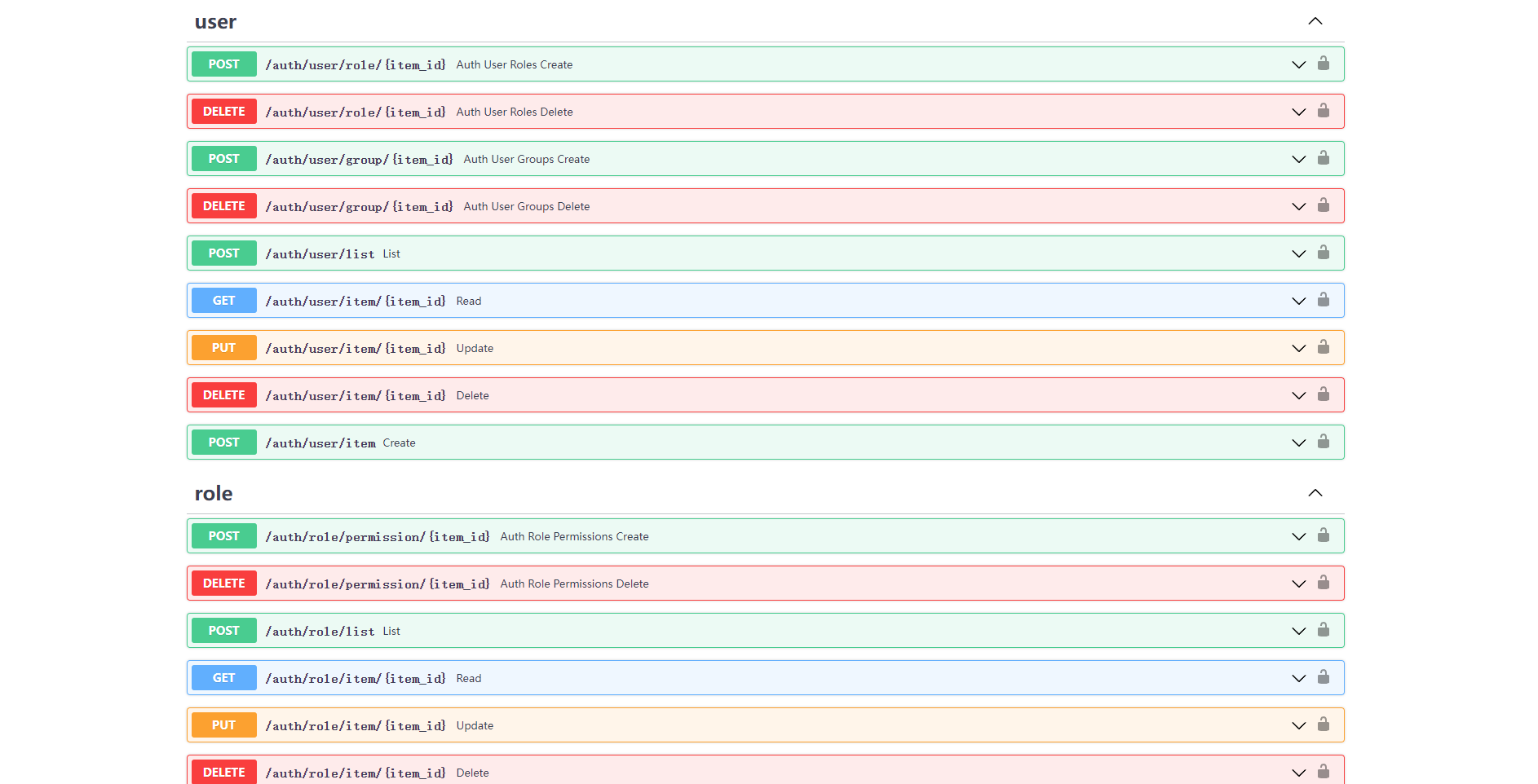\n\n## Project\n\n- [`Amis-Admin-Theme-Editor`](https://github.com/swelcker/amis-admin-theme-editor):Theme-Editor for the fastapi-amis-admin.\n Allows to add custom css styles and to apply theme --vars change on the fly.\n- [`FastAPI-User-Auth`](https://github.com/amisadmin/fastapi_user_auth): A simple and powerful `FastAPI` user `RBAC`\n authentication and authorization library.\n- [`FastAPI-Scheduler`](https://github.com/amisadmin/fastapi_scheduler): A simple scheduled task management `FastAPI` extension\n based on `APScheduler`.\n- [`FastAPI-Config`](https://github.com/amisadmin/fastapi-config): A visual dynamic configuration management extension package\n based on `FastAPI-Amis-Admin`.\n- [`FastAPI-Amis-Admin-Demo`](https://github.com/amisadmin/fastapi_amis_admin_demo): An example `FastAPI-Amis-Admin` application.\n- [`FastAPI-User-Auth-Demo`](https://github.com/amisadmin/fastapi_user_auth_demo): An example `FastAPI-User-Auth` application.\n\n## License\n\n- According to the `Apache2.0` protocol, `fastapi-amis-admin` is free and open source. It can be used for commercial for\n free, but please clearly display copyright information about `FastAPI-Amis-Admin` on the display interface.\n\n",
"bugtrack_url": null,
"license": null,
"summary": "FastAPI-Amis-Admin is a high-performance, efficient and easily extensible FastAPI admin framework. Inspired by Django-admin, and has as many powerful functions as Django-admin. ",
"version": "0.7.2",
"project_urls": {
"Documentation": "http://docs.amis.work/",
"Source": "https://github.com/amisadmin/fastapi_amis_admin"
},
"split_keywords": [
"fastapi",
" fastapi-admin",
" fastapi-amis-admin",
" django-admin",
" sqlmodel",
" sqlalchemy"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "ff30fe3481db9a5c217f935904befa38534a22c8f7b685db3334aa5435f8b001",
"md5": "124e93a2ab941d51ac638e7fe399e9d1",
"sha256": "919840af27dcd831d5dadbd1a57ad61c2dda5dd8ae2880cd4e03e23c0401f48c"
},
"downloads": -1,
"filename": "fastapi_amis_admin-0.7.2-py3-none-any.whl",
"has_sig": false,
"md5_digest": "124e93a2ab941d51ac638e7fe399e9d1",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 114946,
"upload_time": "2024-03-31T06:42:43",
"upload_time_iso_8601": "2024-03-31T06:42:43.609453Z",
"url": "https://files.pythonhosted.org/packages/ff/30/fe3481db9a5c217f935904befa38534a22c8f7b685db3334aa5435f8b001/fastapi_amis_admin-0.7.2-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "f6ef9920511d02c5c1dbac288744fd1c7447b6fa8a204fc864029f0ad1a1afb8",
"md5": "9f870ff64d837bee3f22ea00f1c43a13",
"sha256": "e80d93acbb2a4840ef597f901204d8f43029541724333924f34b9468aa644582"
},
"downloads": -1,
"filename": "fastapi_amis_admin-0.7.2.tar.gz",
"has_sig": false,
"md5_digest": "9f870ff64d837bee3f22ea00f1c43a13",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 471184,
"upload_time": "2024-03-31T06:42:45",
"upload_time_iso_8601": "2024-03-31T06:42:45.657986Z",
"url": "https://files.pythonhosted.org/packages/f6/ef/9920511d02c5c1dbac288744fd1c7447b6fa8a204fc864029f0ad1a1afb8/fastapi_amis_admin-0.7.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-31 06:42:45",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "amisadmin",
"github_project": "fastapi_amis_admin",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "fastapi_amis_admin"
}