[简体中文](https://github.com/Mkadir/fastapi-user-auth/blob/master/README.zh.md)
| [English](https://github.com/Mkadir/fastapi-user-auth)
# Project Introduction
<h2 align="center">
FastAPI-User-Auth
</h2><p align="center">
<em>FastAPI-User-Auth is a simple and powerful FastAPI user authentication and authorization library based on Casbin.</em><br/>
<em>Based on FastAPI-Amis-Admin and provides a freely extensible visual management interface.</em>
</p>
<p align="center">
<a href="https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml" target="_blank">
<img src="https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml/badge.svg" alt="Pytest">
</a>
<a href="https://pypi.org/project/fastapi_user_auth" target="_blank">
<img src="https://badgen.net/pypi/v/fastapi-user-auth?color=blue" alt="Package version">
</a>
<a href="https://pepy.tech/project/fastapi-user-auth" target="_blank">
<img src="https://pepy.tech/badge/fastapi-user-auth" alt="Downloads">
</a>
<a href="https://gitter.im/amisadmin/fastapi-amis-admin">
<img src="https://badges.gitter.im/amisadmin/fastapi-amis-admin.svg" alt="Chat on Gitter"/>
</a>
<a href="https://jq.qq.com/?_wv=1027&k=U4Dv6x8W" target="_blank">
<img src="https://badgen.net/badge/qq%E7%BE%A4/229036692/orange" alt="229036692">
</a>
</p>
<p align="center">
<a href="https://github.com/amisadmin/fastapi_user_auth" target="_blank">Source code</a>
·
<a href="http://user-auth.demo.amis.work/" target="_blank">Online demo</a>
·
<a href="http://docs.amis.work" target="_blank">Documentation</a>
·
<a href="http://docs.gh.amis.work" target="_blank"> can't open the document ?</a>
</p>
------
`FastAPI-User-Auth` is a API based on [FastAPI-Amis-Admin](https://github.com/amisadmin/fastapi_amis_admin)
The application plug-in is deeply integrated with `FastAPI-Amis-Admin` to provide user authentication and authorization.
Casbin-based RBAC permission management supports multiple verification methods, multiple databases, and multiple granularity permission controls.
### Permission types
- **Page Permissions**: Control whether the user can access a certain menu page. If it is not accessible, the menu will not be displayed, and all routes under the page will be inaccessible.
- **Action Permission**: Controls whether the user can perform an action and whether the button is displayed. For example: add, update, delete, etc.
- **Field permissions**: Control whether users can operate a certain field. For example: list display fields, filter fields, add fields, update fields, etc.
- **Data permissions**: Control the range of data that users can operate. For example: they can only operate the data they created, only the data of the last 7 days, etc.
## Installation
```bash
pip install fastapi-user-auth
```
## Simple example
```python
from fastapi import FastAPI
from fastapi_amis_admin.admin.settings import Settings
from fastapi_user_auth.site import AuthAdminSite
from starlette.requests import Request
from sqlmodel import SQLModel
# Create FastAPI application
app = FastAPI()
# Create AdminSite instance
site = AuthAdminSite(settings=Settings(database_url='sqlite:///amisadmin.db?check_same_thread=False'))
auth = site.auth
# Mount the backend management system
site.mount_app(app)
# Create initial database table
@app.on_event("startup")
async def startup():
await site.db.async_run_sync(SQLModel.metadata.create_all, is_session=False)
# Create a default administrator, username: admin, password: admin, please change the password in time!!!
await auth.create_role_user("admin")
#Create the default super administrator, username: root, password: root, please change the password in time!!! await auth.create_role_user("root")
# Run the startup method of the site, load the casbin strategy, etc.
await site.router.startup()
#Add a default casbin rule
if not auth.enforcer.enforce("u:admin", site.unique_id, "page", "page"):
await auth.enforcer.add_policy("u:admin", site.unique_id, "page", "page", "allow")
# Requirements: User must be logged in
@app.get("/auth/get_user")
@auth.requires()
def get_user(request: Request):
return request.user
if __name__ == '__main__':
import uvicorn
uvicorn.run(app)
```
## Authentication methods
### Decorator
- Recommended scenario: Single route. Supports synchronous/asynchronous routing.
```python
# Requirements: User must be logged in
@app.get("/auth/user")
@auth.requires()
def user(request: Request):
return request.user # Current request user object.
# Verify routing: user has admin role
@app.get("/auth/admin_roles")
@auth.requires('admin')
def admin_roles(request: Request):
return request.user
# Requirement: User has VIP role
#Support synchronous/asynchronous routing
@app.get("/auth/vip_roles")
@auth.requires(['vip'])
async def vip_roles(request: Request):
return request.user
# Requirement: User has admin role or vip role
@app.get("/auth/admin_or_vip_roles")
@auth.requires(roles=['admin', 'vip'])
def admin_or_vip_roles(request: Request):
return request.user
```
### Dependencies (recommended)
- Recommended scenarios: single route, route set, FastAPI application.
```python
from fastapi import Depends
from fastapi_user_auth.auth.models import User
# Route parameter dependencies, this method is recommended
@app.get("/auth/admin_roles_depend_1")
def admin_roles(user: User = Depends(auth.get_current_user)):
return user # Current request user object.
# Path manipulation decorator dependencies
@app.get("/auth/admin_roles_depend_2", dependencies=[Depends(auth.requires('admin')())])
def admin_roles(request: Request):
return request.user
# Global dependencies
# All requests under the app require the admin role
app = FastAPI(dependencies=[Depends(auth.requires('admin')())])
@app.get("/auth/admin_roles_depend_3")
def admin_roles(request: Request):
return request.user
```
### Middleware
- Recommended scenario: FastAPI application
```python
app = FastAPI()
# Attach `request.auth` and `request.user` objects before each request is processed under the app application
auth.backend.attach_middleware(app)
```
### Call directly
- Recommended scenario: non-routing method
```python
from fastapi_user_auth.auth.models import User
async def get_request_user(request: Request) -> Optional[User]:
# user= await auth.get_current_user(request)
if await auth.requires('admin', response=False)(request):
return request.user
else:
return None
```
## Token Storage Backend
`fastapi-user-auth` supports multiple token storage methods. The default is: `DbTokenStore`, it is recommended to customize it to: `JwtTokenStore`
### JwtTokenStore
- ` pip install fastapi-user-auth[jwt] `
```python
from fastapi_user_auth.auth.backends.jwt import JwtTokenStore
from sqlalchemy_database import Database
from fastapi_user_auth.auth import Auth
from fastapi_amis_admin.admin.site import AuthAdminSite
#Create a sync database engine
db = Database.create(url="sqlite:///amisadmin.db?check_same_thread=False")
# Use `JwtTokenStore` to create an auth object
auth = Auth(
db=db,
token_store=JwtTokenStore(secret_key='09d25e094faa6ca2556c818166b7a9563b93f7099f6f0f4caa6cf63b88e8d3e7')
)
# Pass auth object to AdminSite
site = AuthAdminSite(
settings=Settings(),
db=db,
auth=auth
)
```
### DbTokenStore
```python
# Create an auth object using `DbTokenStore`
from fastapi_user_auth.auth.backends.db import DbTokenStore
auth = Auth(
db=db,
token_store=DbTokenStore(db=db)
)
```
### RedisTokenStore
- `pip install fastapi-user-auth[redis] `
```python
# Use `RedisTokenStore` to create an auth object
from fastapi_user_auth.auth.backends.redis import RedisTokenStore
from redis.asyncio import Redis
auth = Auth(
db=db,
token_store=RedisTokenStore(redis=Redis.from_url('redis://localhost?db=0'))
)
```
## RBAC model
This system adopts the `Casbin RBAC` model and runs a role-based priority strategy.
- Permissions can be assigned to roles or directly to users.
- Users can have multiple roles.
- A role can have multiple sub-roles.
- The permission policy owned by the user has a higher priority than the permission policy of the role it owns.
```mermaid
flowchart LR
User -. m:n .-> Role
User -. m:n .-> CasbinRule
Role -. m:n .-> Role
Role -. m:n .-> CasbinRule
```
## Advanced expansion
### Extend the `User` model
```python
from datetime import date
from fastapi_amis_admin.models.fields import Field
from fastapi_user_auth.auth.models import BaseUser
# Customize the `User` model, inherit `BaseUser`
class MyUser(BaseUser, table = True):
point: float = Field(default = 0, title = 'Point', description = 'User points')
phone: str = Field(None, title = 'Phone', max_length = 15)
parent_id: int = Field(None, title = "Superior", foreign_key = "auth_user.id")
birthday: date = Field(None, title = "date of birth")
location: str = Field(None, title = "Location")
# Use custom `User` model to create auth object
auth = Auth(db = AsyncDatabase(engine), user_model = MyUser)
```
### Extend the `Role` model
```python
from fastapi_amis_admin.models.fields import Field
from fastapi_user_auth.auth.models import Role
# Customize `Role` model, inherit `Role`;
class MyRole(Role, table=True):
icon: str = Field(None, title='图标')
is_active: bool = Field(default=True, title="是否激活")
```
### Customize `User Auth App` default management class
The default management classes can be overridden and replaced through inheritance.
For example: `UserLoginFormAdmin`, `UserRegFormAdmin`, `UserInfoFormAdmin`,
`UserAdmin`,`RoleAdmin`
```python
# Customize the model management class, inherit and override the corresponding default management class
class MyRoleAdmin(admin.ModelAdmin):
page_schema = PageSchema(label='User group management', icon='fa fa-group')
model = MyRole
readonly_fields = ['key']
# Customize user authentication application, inherit and override the default user authentication application
class MyUserAuthApp(UserAuthApp):
RoleAdmin = MyRoleAdmin
# Customize the user management site, inherit and override the default user management site
class MyAuthAdminSite(AuthAdminSite):
UserAuthApp = MyUserAuthApp
# Use the custom `AuthAdminSite` class to create a site object
site = MyAuthAdminSite(settings, auth=auth)
```
## ModelAdmin permission control
### Field permissions
- Inherit the `AutoField ModelAdmin` class to achieve field permission control. By assigning user and role permissions in the background.
- `perm_fields_exclude`: Specify fields that do not require permission control.
```python
from fastapi_user_auth.mixins.admin import AuthFieldModelAdmin
from fastapi_amis_admin.amis import PageSchema
from fastapi_amis_admin.admin import FieldPermEnum
class AuthFieldArticleAdmin(AuthFieldModelAdmin):
page_schema = PageSchema(label="文章管理")
model = Article
# Specify fields that do not need permission control.
perm_fields_exclude = {
FieldPermEnum.CREATE: ["title", "description", "content"],
}
```
### Data permissions
- Inherit the `AuthSelectModelAdmin` class to achieve data permission control. By assigning user and role permissions in the background.
- `select_permisions`: Specify permissions to query data.
```python
from fastapi_user_auth.mixins.admin import AuthSelectModelAdmin
from fastapi_amis_admin.amis import PageSchema
from fastapi_amis_admin.admin import RecentTimeSelectPerm, UserSelectPerm, SimpleSelectPerm
class AuthSelectArticleAdmin(AuthSelectModelAdmin):
page_schema = PageSchema(label="Dataset control article management")
model = Article
select_permissions = [
# Data created in the last 7 days. reverse=True indicates reverse selection, that is, the data within the last 7 days is selected by default.
RecentTimeSelectPerm(name="recent7_create", label="Created in the last 7 days", td=60 * 60 * 24 * 7, reverse=True),
# Data created in the last 30 days
RecentTimeSelectPerm(name="recent30_create", label="Created in the last 30 days", td=60 * 60 * 24 * 30),
#Data updated in the last 3 days
RecentTimeSelectPerm(name="recent3_update", label="Updated in the last 3 days", td=60 * 60 * 24 * 3, time_column="update_time"),
# You can only select the data you created. reverse=True means reverse selection, that is, the data you created is selected by default.
UserSelectPerm(name="self_create", label="Create yourself", user_column="user_id", reverse=True),
# # You can only select the data you updated
# UserSelectPerm(name="self_update", label="Update yourself", user_column="update_by"),
# Only published data can be selected
SimpleSelectPerm(name="published", label="Published", column="is_published", values=[True]),
# Only data with status [1,2,3] can be selected
SimpleSelectPerm(name="status_1_2_3", label="Status is 1_2_3", column="status", values=[1, 2, 3]),
]
```
## Interface preview
- Open `http://127.0.0.1:8000/admin/auth/form/login` in your browser:
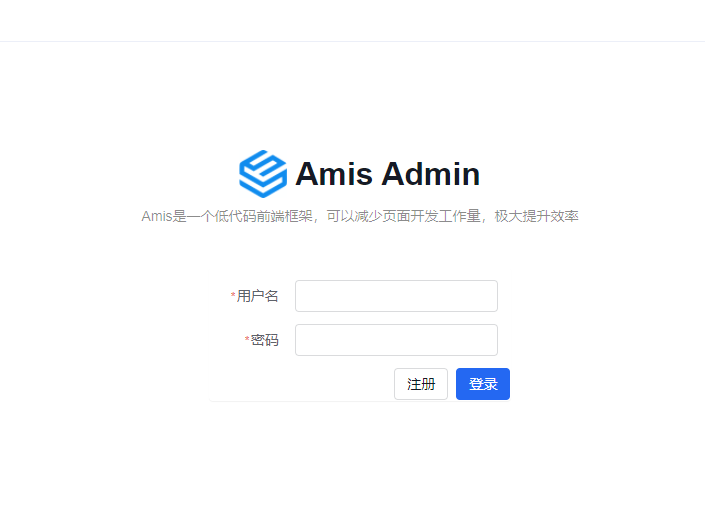
- Open `http://127.0.0.1:8000/admin/` in your browser:
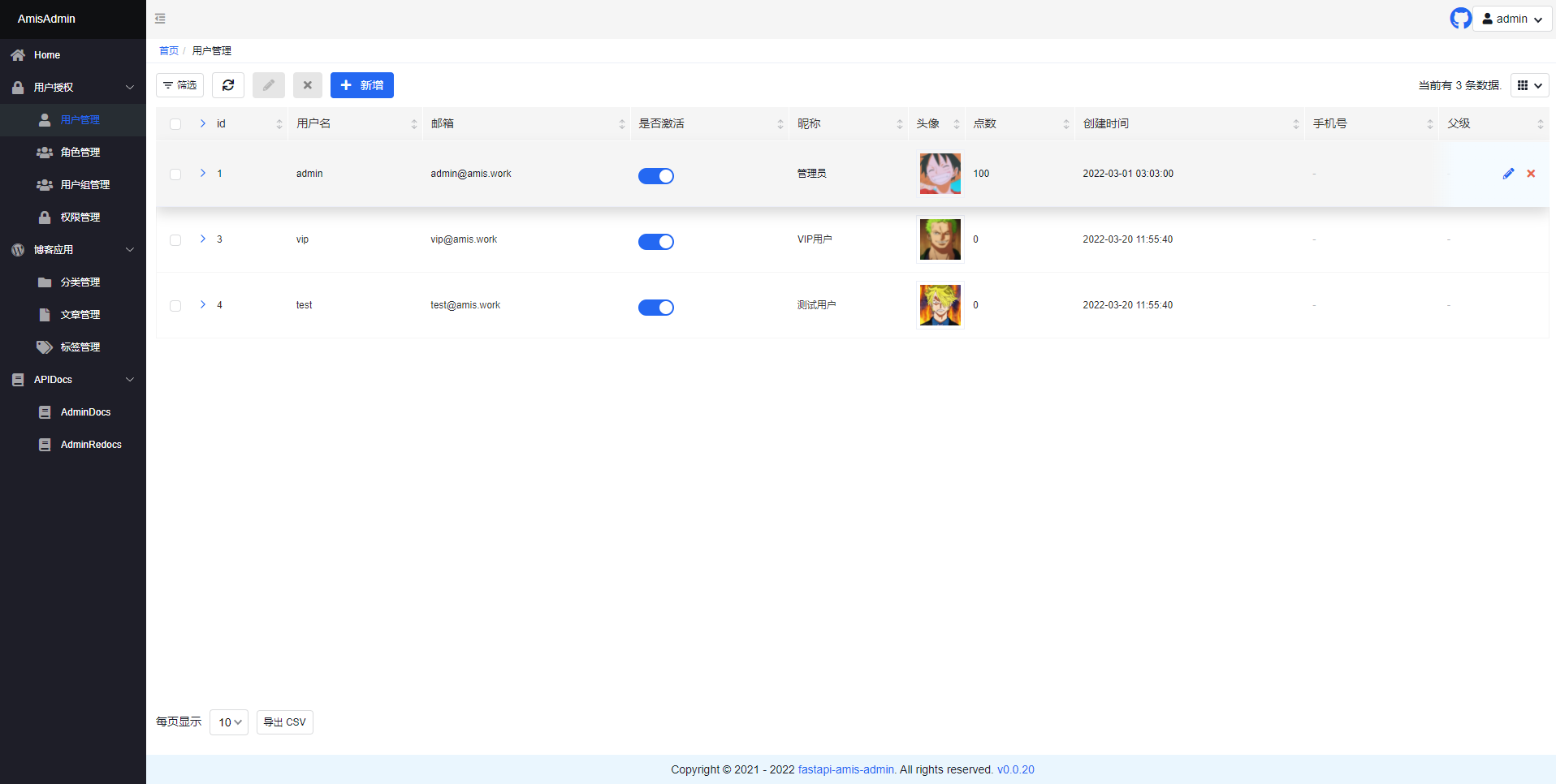
- Open `http://127.0.0.1:8000/admin/docs` in your browser:
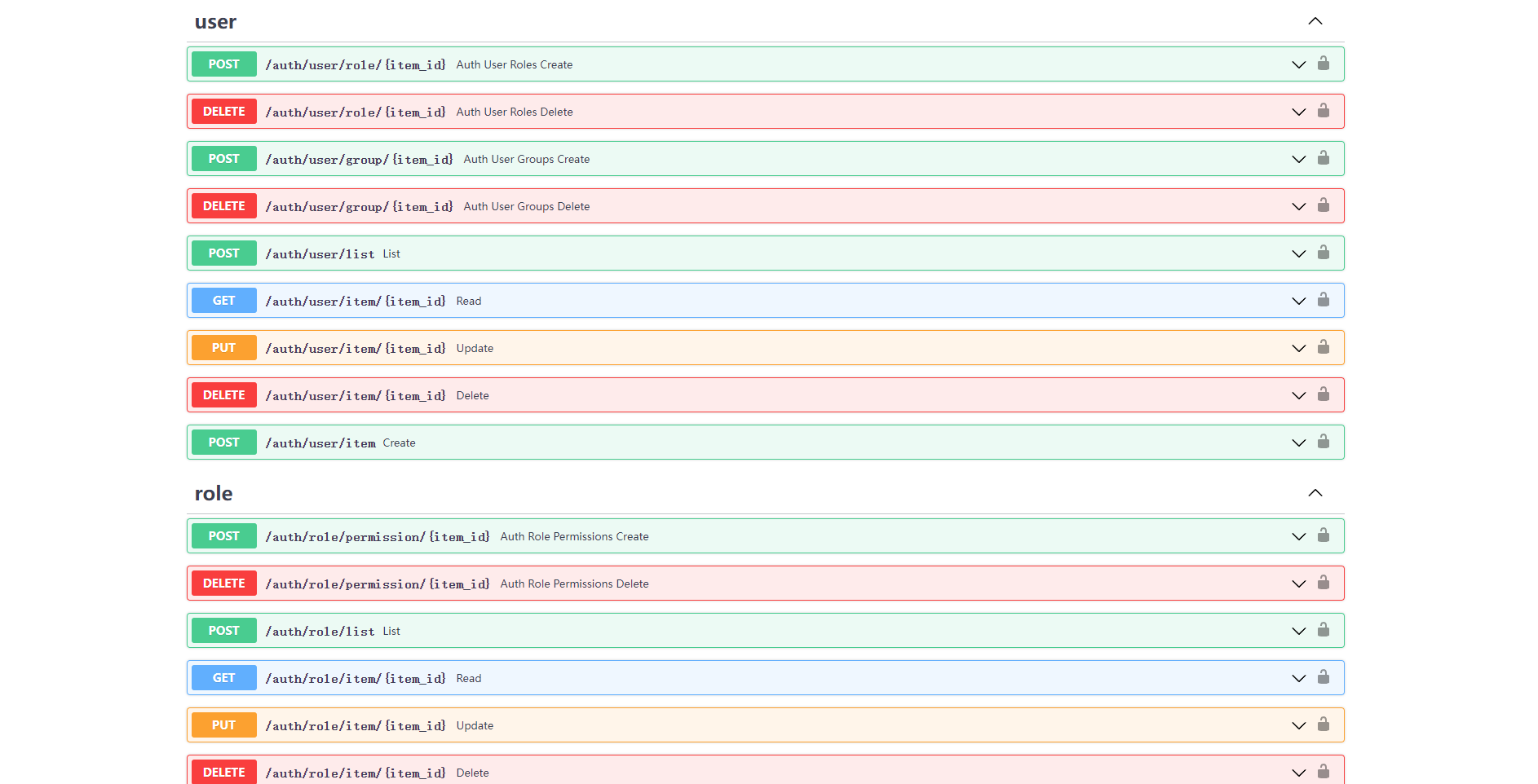
## License Agreement
- `fastapi-amis-admin` is open source and free to use based on `Apache2.0` and can be used for commercial purposes for free, but please clearly display the copyright information about FastAPI-Amis-Admin in the display interface.
## Thanks
Thanks to the following developers for their contributions to FastAPI-User-Auth:
<a href="https://github.com/amisadmin/fastapi_user_auth/graphs/contributors">
<img src="https://contrib.rocks/image?repo=amisadmin/fastapi_user_auth" alt=""/>
</a>
Raw data
{
"_id": null,
"home_page": null,
"name": "fastapi_user_auth",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": "Atomi <1456417373@qq.com>",
"keywords": "fastapi, fastapi-user-auth, fastapi-amis-admin, fastapi-auth, fastapi-users, fastapi-jwt-auth, sqlmodel",
"author": null,
"author_email": "Atomi <1456417373@qq.com>",
"download_url": "https://files.pythonhosted.org/packages/fb/fd/376d6bf9b52e46be8f55df7a82d7c71de6296c6a6a17fa0b5f8364465689/fastapi_user_auth-0.7.3.tar.gz",
"platform": null,
"description": "[\u7b80\u4f53\u4e2d\u6587](https://github.com/Mkadir/fastapi-user-auth/blob/master/README.zh.md)\n| [English](https://github.com/Mkadir/fastapi-user-auth)\n# Project Introduction\n<h2 align=\"center\">\n FastAPI-User-Auth\n</h2><p align=\"center\">\n <em>FastAPI-User-Auth is a simple and powerful FastAPI user authentication and authorization library based on Casbin.</em><br/>\n <em>Based on FastAPI-Amis-Admin and provides a freely extensible visual management interface.</em>\n</p>\n<p align=\"center\">\n <a href=\"https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml\" target=\"_blank\">\n <img src=\"https://github.com/amisadmin/fastapi_amis_admin/actions/workflows/pytest.yml/badge.svg\" alt=\"Pytest\">\n </a>\n <a href=\"https://pypi.org/project/fastapi_user_auth\" target=\"_blank\">\n <img src=\"https://badgen.net/pypi/v/fastapi-user-auth?color=blue\" alt=\"Package version\">\n </a>\n <a href=\"https://pepy.tech/project/fastapi-user-auth\" target=\"_blank\">\n <img src=\"https://pepy.tech/badge/fastapi-user-auth\" alt=\"Downloads\">\n </a>\n <a href=\"https://gitter.im/amisadmin/fastapi-amis-admin\">\n <img src=\"https://badges.gitter.im/amisadmin/fastapi-amis-admin.svg\" alt=\"Chat on Gitter\"/>\n </a>\n <a href=\"https://jq.qq.com/?_wv=1027&k=U4Dv6x8W\" target=\"_blank\">\n <img src=\"https://badgen.net/badge/qq%E7%BE%A4/229036692/orange\" alt=\"229036692\">\n </a>\n</p>\n<p align=\"center\">\n <a href=\"https://github.com/amisadmin/fastapi_user_auth\" target=\"_blank\">Source code</a>\n \u00b7\n <a href=\"http://user-auth.demo.amis.work/\" target=\"_blank\">Online demo</a>\n \u00b7\n <a href=\"http://docs.amis.work\" target=\"_blank\">Documentation</a>\n \u00b7\n <a href=\"http://docs.gh.amis.work\" target=\"_blank\"> can't open the document \uff1f</a>\n</p>\n\n------\n`FastAPI-User-Auth` is a API based on [FastAPI-Amis-Admin](https://github.com/amisadmin/fastapi_amis_admin)\nThe application plug-in is deeply integrated with `FastAPI-Amis-Admin` to provide user authentication and authorization.\nCasbin-based RBAC permission management supports multiple verification methods, multiple databases, and multiple granularity permission controls.\n\n### Permission types\n- **Page Permissions**: Control whether the user can access a certain menu page. If it is not accessible, the menu will not be displayed, and all routes under the page will be inaccessible.\n- **Action Permission**: Controls whether the user can perform an action and whether the button is displayed. For example: add, update, delete, etc.\n- **Field permissions**: Control whether users can operate a certain field. For example: list display fields, filter fields, add fields, update fields, etc.\n- **Data permissions**: Control the range of data that users can operate. For example: they can only operate the data they created, only the data of the last 7 days, etc.\n\n\n## Installation\n```bash\npip install fastapi-user-auth\n```\n## Simple example\n```python\nfrom fastapi import FastAPI\nfrom fastapi_amis_admin.admin.settings import Settings\nfrom fastapi_user_auth.site import AuthAdminSite\nfrom starlette.requests import Request\nfrom sqlmodel import SQLModel\n\n# Create FastAPI application\napp = FastAPI()\n\n# Create AdminSite instance\nsite = AuthAdminSite(settings=Settings(database_url='sqlite:///amisadmin.db?check_same_thread=False'))\nauth = site.auth\n# Mount the backend management system\nsite.mount_app(app)\n\n\n# Create initial database table\n@app.on_event(\"startup\")\nasync def startup():\n await site.db.async_run_sync(SQLModel.metadata.create_all, is_session=False)\n # Create a default administrator, username: admin, password: admin, please change the password in time!!!\n await auth.create_role_user(\"admin\")\n #Create the default super administrator, username: root, password: root, please change the password in time!!! await auth.create_role_user(\"root\")\n # Run the startup method of the site, load the casbin strategy, etc.\n await site.router.startup()\n #Add a default casbin rule\n if not auth.enforcer.enforce(\"u:admin\", site.unique_id, \"page\", \"page\"):\n await auth.enforcer.add_policy(\"u:admin\", site.unique_id, \"page\", \"page\", \"allow\")\n\n\n# Requirements: User must be logged in\n@app.get(\"/auth/get_user\")\n@auth.requires()\ndef get_user(request: Request):\n return request.user\n\n\nif __name__ == '__main__':\n import uvicorn\n\n uvicorn.run(app)\n\n```\n\n## Authentication methods\n### Decorator\n- Recommended scenario: Single route. Supports synchronous/asynchronous routing.\n```python\n# Requirements: User must be logged in\n@app.get(\"/auth/user\")\n@auth.requires()\ndef user(request: Request):\n return request.user # Current request user object.\n\n\n# Verify routing: user has admin role\n@app.get(\"/auth/admin_roles\")\n@auth.requires('admin')\ndef admin_roles(request: Request):\n return request.user\n\n\n# Requirement: User has VIP role\n#Support synchronous/asynchronous routing\n@app.get(\"/auth/vip_roles\")\n@auth.requires(['vip'])\nasync def vip_roles(request: Request):\n return request.user\n\n\n# Requirement: User has admin role or vip role\n@app.get(\"/auth/admin_or_vip_roles\")\n@auth.requires(roles=['admin', 'vip'])\ndef admin_or_vip_roles(request: Request):\n return request.user\n```\n### Dependencies (recommended)\n- Recommended scenarios: single route, route set, FastAPI application.\n\n```python\nfrom fastapi import Depends\nfrom fastapi_user_auth.auth.models import User\n\n\n# Route parameter dependencies, this method is recommended\n@app.get(\"/auth/admin_roles_depend_1\")\ndef admin_roles(user: User = Depends(auth.get_current_user)):\n return user # Current request user object.\n\n\n# Path manipulation decorator dependencies\n@app.get(\"/auth/admin_roles_depend_2\", dependencies=[Depends(auth.requires('admin')())])\ndef admin_roles(request: Request):\n return request.user\n\n\n# Global dependencies\n# All requests under the app require the admin role\napp = FastAPI(dependencies=[Depends(auth.requires('admin')())])\n\n\n@app.get(\"/auth/admin_roles_depend_3\")\ndef admin_roles(request: Request):\n return request.user\n\n```\n### Middleware\n- Recommended scenario: FastAPI application\n```python\napp = FastAPI()\n# Attach `request.auth` and `request.user` objects before each request is processed under the app application\nauth.backend.attach_middleware(app)\n```\n\n### Call directly\n\n- Recommended scenario: non-routing method\n```python\nfrom fastapi_user_auth.auth.models import User\n\n\nasync def get_request_user(request: Request) -> Optional[User]:\n # user= await auth.get_current_user(request)\n if await auth.requires('admin', response=False)(request):\n return request.user\n else:\n return None\n```\n\n## Token Storage Backend\n`fastapi-user-auth` supports multiple token storage methods. The default is: `DbTokenStore`, it is recommended to customize it to: `JwtTokenStore`\n\n### JwtTokenStore\n- ` pip install fastapi-user-auth[jwt] `\n\n```python\nfrom fastapi_user_auth.auth.backends.jwt import JwtTokenStore\nfrom sqlalchemy_database import Database\nfrom fastapi_user_auth.auth import Auth\nfrom fastapi_amis_admin.admin.site import AuthAdminSite\n\n#Create a sync database engine\ndb = Database.create(url=\"sqlite:///amisadmin.db?check_same_thread=False\")\n\n# Use `JwtTokenStore` to create an auth object\nauth = Auth(\n db=db,\n token_store=JwtTokenStore(secret_key='09d25e094faa6ca2556c818166b7a9563b93f7099f6f0f4caa6cf63b88e8d3e7')\n)\n\n# Pass auth object to AdminSite\nsite = AuthAdminSite(\n settings=Settings(),\n db=db,\n auth=auth\n)\n\n```\n\n### DbTokenStore\n```python\n# Create an auth object using `DbTokenStore`\nfrom fastapi_user_auth.auth.backends.db import DbTokenStore\n\nauth = Auth(\n db=db,\n token_store=DbTokenStore(db=db)\n)\n```\n### RedisTokenStore\n\n- `pip install fastapi-user-auth[redis] `\n\n```python\n# Use `RedisTokenStore` to create an auth object\nfrom fastapi_user_auth.auth.backends.redis import RedisTokenStore\nfrom redis.asyncio import Redis\n\nauth = Auth(\n db=db,\n token_store=RedisTokenStore(redis=Redis.from_url('redis://localhost?db=0'))\n)\n```\n## RBAC model\nThis system adopts the `Casbin RBAC` model and runs a role-based priority strategy.\n- Permissions can be assigned to roles or directly to users.\n- Users can have multiple roles.\n- A role can have multiple sub-roles.\n- The permission policy owned by the user has a higher priority than the permission policy of the role it owns.\n\n```mermaid\nflowchart LR\n User -. m:n .-> Role\n User -. m:n .-> CasbinRule\n Role -. m:n .-> Role\n Role -. m:n .-> CasbinRule \n```\n\n## Advanced expansion\n### Extend the `User` model\n\n```python\nfrom datetime import date\n\nfrom fastapi_amis_admin.models.fields import Field\nfrom fastapi_user_auth.auth.models import BaseUser\n\n# Customize the `User` model, inherit `BaseUser`\nclass MyUser(BaseUser, table = True):\n point: float = Field(default = 0, title = 'Point', description = 'User points')\n phone: str = Field(None, title = 'Phone', max_length = 15)\n parent_id: int = Field(None, title = \"Superior\", foreign_key = \"auth_user.id\")\n birthday: date = Field(None, title = \"date of birth\")\n location: str = Field(None, title = \"Location\")\n\n# Use custom `User` model to create auth object\nauth = Auth(db = AsyncDatabase(engine), user_model = MyUser)\n```\n\n### Extend the `Role` model\n\n```python\nfrom fastapi_amis_admin.models.fields import Field\nfrom fastapi_user_auth.auth.models import Role\n\n\n# Customize `Role` model, inherit `Role`;\nclass MyRole(Role, table=True):\n icon: str = Field(None, title='\u56fe\u6807')\n is_active: bool = Field(default=True, title=\"\u662f\u5426\u6fc0\u6d3b\")\n\n```\n\n### Customize `User Auth App` default management class\n\nThe default management classes can be overridden and replaced through inheritance.\nFor example: `UserLoginFormAdmin`, `UserRegFormAdmin`, `UserInfoFormAdmin`,\n`UserAdmin`,`RoleAdmin`\n\n```python\n# Customize the model management class, inherit and override the corresponding default management class\nclass MyRoleAdmin(admin.ModelAdmin):\n page_schema = PageSchema(label='User group management', icon='fa fa-group')\n model = MyRole\n readonly_fields = ['key']\n\n\n# Customize user authentication application, inherit and override the default user authentication application\nclass MyUserAuthApp(UserAuthApp):\n RoleAdmin = MyRoleAdmin\n\n\n# Customize the user management site, inherit and override the default user management site\nclass MyAuthAdminSite(AuthAdminSite):\n UserAuthApp = MyUserAuthApp\n\n\n# Use the custom `AuthAdminSite` class to create a site object\nsite = MyAuthAdminSite(settings, auth=auth)\n```\n\n## ModelAdmin permission control\n\n### Field permissions\n\n- Inherit the `AutoField ModelAdmin` class to achieve field permission control. By assigning user and role permissions in the background.\n\n- `perm_fields_exclude`: Specify fields that do not require permission control.\n\n```python\nfrom fastapi_user_auth.mixins.admin import AuthFieldModelAdmin\nfrom fastapi_amis_admin.amis import PageSchema\nfrom fastapi_amis_admin.admin import FieldPermEnum\n\nclass AuthFieldArticleAdmin(AuthFieldModelAdmin):\n page_schema = PageSchema(label=\"\u6587\u7ae0\u7ba1\u7406\")\n model = Article\n # Specify fields that do not need permission control. \n perm_fields_exclude = {\n FieldPermEnum.CREATE: [\"title\", \"description\", \"content\"],\n }\n```\n\n### Data permissions\n\n- Inherit the `AuthSelectModelAdmin` class to achieve data permission control. By assigning user and role permissions in the background.\n- `select_permisions`: Specify permissions to query data.\n\n```python\nfrom fastapi_user_auth.mixins.admin import AuthSelectModelAdmin\nfrom fastapi_amis_admin.amis import PageSchema\nfrom fastapi_amis_admin.admin import RecentTimeSelectPerm, UserSelectPerm, SimpleSelectPerm\n\n\nclass AuthSelectArticleAdmin(AuthSelectModelAdmin):\n page_schema = PageSchema(label=\"Dataset control article management\")\n model = Article\n select_permissions = [\n # Data created in the last 7 days. reverse=True indicates reverse selection, that is, the data within the last 7 days is selected by default.\n RecentTimeSelectPerm(name=\"recent7_create\", label=\"Created in the last 7 days\", td=60 * 60 * 24 * 7, reverse=True),\n # Data created in the last 30 days\n RecentTimeSelectPerm(name=\"recent30_create\", label=\"Created in the last 30 days\", td=60 * 60 * 24 * 30),\n #Data updated in the last 3 days\n RecentTimeSelectPerm(name=\"recent3_update\", label=\"Updated in the last 3 days\", td=60 * 60 * 24 * 3, time_column=\"update_time\"),\n # You can only select the data you created. reverse=True means reverse selection, that is, the data you created is selected by default.\n \n UserSelectPerm(name=\"self_create\", label=\"Create yourself\", user_column=\"user_id\", reverse=True),\n # # You can only select the data you updated\n # UserSelectPerm(name=\"self_update\", label=\"Update yourself\", user_column=\"update_by\"),\n # Only published data can be selected\n SimpleSelectPerm(name=\"published\", label=\"Published\", column=\"is_published\", values=[True]),\n # Only data with status [1,2,3] can be selected\n SimpleSelectPerm(name=\"status_1_2_3\", label=\"Status is 1_2_3\", column=\"status\", values=[1, 2, 3]),\n ]\n```\n\n## Interface preview\n\n- Open `http://127.0.0.1:8000/admin/auth/form/login` in your browser:\n\n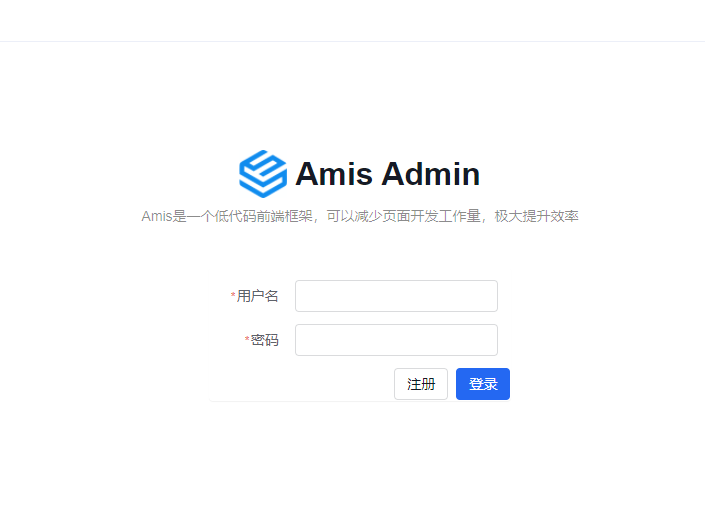\n\n- Open `http://127.0.0.1:8000/admin/` in your browser:\n\n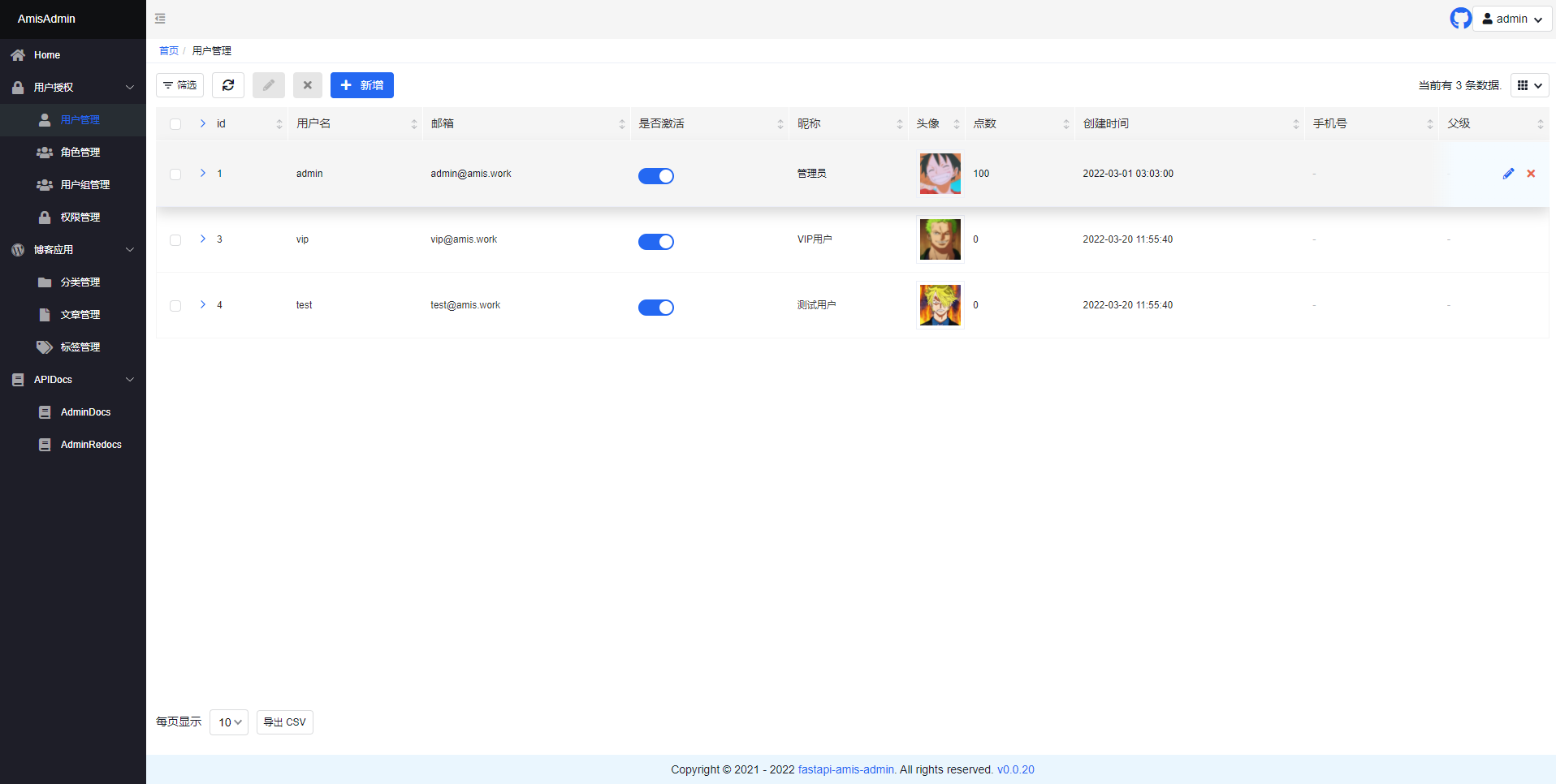\n\n- Open `http://127.0.0.1:8000/admin/docs` in your browser:\n\n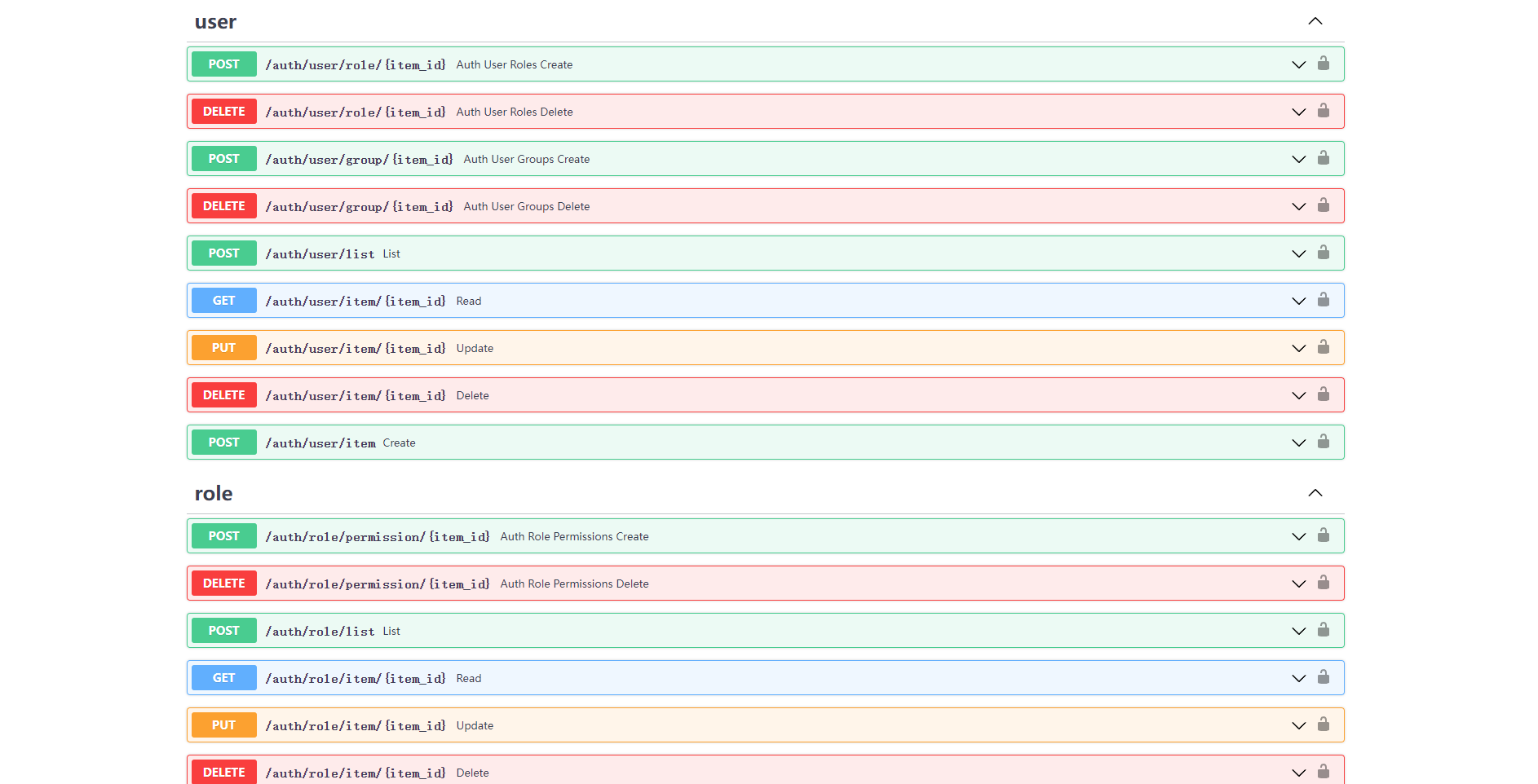\n\n## License Agreement\n\n- `fastapi-amis-admin` is open source and free to use based on `Apache2.0` and can be used for commercial purposes for free, but please clearly display the copyright information about FastAPI-Amis-Admin in the display interface.\n## Thanks\n\nThanks to the following developers for their contributions to FastAPI-User-Auth:\n\n<a href=\"https://github.com/amisadmin/fastapi_user_auth/graphs/contributors\">\n <img src=\"https://contrib.rocks/image?repo=amisadmin/fastapi_user_auth\" alt=\"\"/>\n</a>",
"bugtrack_url": null,
"license": null,
"summary": "FastAPI-User-Auth is a simple and powerful FastAPI user RBAC authentication and authorization library. Based on FastAPI-Amis-Admin and provides a freely extensible visual management interface.",
"version": "0.7.3",
"project_urls": {
"Documentation": "http://docs.amis.work/",
"FastAPI-Amis-Admin": "https://github.com/amisadmin/fastapi_amis_admin",
"Source": "https://github.com/amisadmin/fastapi_user_auth"
},
"split_keywords": [
"fastapi",
" fastapi-user-auth",
" fastapi-amis-admin",
" fastapi-auth",
" fastapi-users",
" fastapi-jwt-auth",
" sqlmodel"
],
"urls": [
{
"comment_text": null,
"digests": {
"blake2b_256": "ca12196e417c90503dcb3a0cd0778427e8ea71dbbc8b56072e7dbee01a98b9cf",
"md5": "78ff31a60bc5995be91c072166efadb6",
"sha256": "4080462be01c069a5db767b75fbed7afca902c8af08d267c04598301a83b6f61"
},
"downloads": -1,
"filename": "fastapi_user_auth-0.7.3-py3-none-any.whl",
"has_sig": false,
"md5_digest": "78ff31a60bc5995be91c072166efadb6",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 47287,
"upload_time": "2024-03-31T06:51:23",
"upload_time_iso_8601": "2024-03-31T06:51:23.867027Z",
"url": "https://files.pythonhosted.org/packages/ca/12/196e417c90503dcb3a0cd0778427e8ea71dbbc8b56072e7dbee01a98b9cf/fastapi_user_auth-0.7.3-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": null,
"digests": {
"blake2b_256": "fbfd376d6bf9b52e46be8f55df7a82d7c71de6296c6a6a17fa0b5f8364465689",
"md5": "1f1deb90079ac344756d1d8b192e6000",
"sha256": "7d6f35b4eaf469927d3bd1d39232fb0594c28d8296e838eb031325ddadc49c3c"
},
"downloads": -1,
"filename": "fastapi_user_auth-0.7.3.tar.gz",
"has_sig": false,
"md5_digest": "1f1deb90079ac344756d1d8b192e6000",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 91091,
"upload_time": "2024-03-31T06:51:26",
"upload_time_iso_8601": "2024-03-31T06:51:26.085991Z",
"url": "https://files.pythonhosted.org/packages/fb/fd/376d6bf9b52e46be8f55df7a82d7c71de6296c6a6a17fa0b5f8364465689/fastapi_user_auth-0.7.3.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-31 06:51:26",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "amisadmin",
"github_project": "fastapi_amis_admin",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "fastapi_user_auth"
}