Name | fastqueue-lib JSON |
Version |
0.0.17
JSON |
| download |
home_page | |
Summary | Fast single ended queue library for python |
upload_time | 2023-10-22 05:32:21 |
maintainer | |
docs_url | None |
author | |
requires_python | >=3.7 |
license | |
keywords |
queue
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
# fastqueue
[](https://github.com/MatthewAndreTaylor/fastqueue/actions)
[](https://github.com/MatthewAndreTaylor/fastqueue/)
[](https://github.com/MatthewAndreTaylor/fastqueue/blob/master/LICENSE)\
[](https://pypi.org/project/fastqueue-lib/)
Single-ended fast queues built in C tuned for Python.
## Requirements
- `python 3.7+`
## Installation
To install fastqueue, using pip:
```bash
pip install fastqueue-lib
```
## Quickstart
For general use cases `fastqueue.Queue()` objects are tuned to perform well.
The enqueue and dequeue methods perform well over a large sequence of arbitrary operations.
`fastqueue.Queue()` supports many standard sequence methods similar to lists.
`fastqueue.Queue()` minimizes memory usage but maintains the fast queue speeds.
```py
>>> from fastqueue import Queue
>>> queue = Queue()
>>> queue.extend(['🚒', '🛴'])
>>> queue[0]
'🚒'
>>> '🛴' in queue
True
>>> queue.enqueue('🚅')
>>> queue.enqueue('🚗')
>>> queue[-1]
'🚗'
>>> [queue.dequeue() for _ in range(len(queue)) ]
['🚒', '🛴', '🚅', '🚗']
```
For more specialized cases `fastqueue.QueueC()` objects are tuned to perform well.
The interface for `fastqueue.QueueC()` is identical to `fastqueue.Queue()`.
The enqueue and dequeue methods perform similarly well over a large sequence of arbitrary operations.
`fastqueue.QueueC()` handles memory differently by doubling the capacity when full.
This increases the complexity but maintains fast amortized cost.
The benefit of this approach is even faster `__getitem__` and `__setitem__` speeds
```py
>>> from fastqueue import QueueC
>>> queue_c = QueueC()
>>> queue_c.extend(['🚒', '🛴'])
>>> queue_c[0]
'🚒'
>>> '🛴' in queue_c
True
>>> queue_c.enqueue('🚅')
>>> queue_c.enqueue('🚗')
>>> queue_c[-1]
'🚗'
>>> [queue_c.dequeue() for _ in range(len(queue_c)) ]
['🚒', '🛴', '🚅', '🚗']
```
Another alternative is `fastqueue.LockQueue()` which supports all queue operations.
`fastqueue.LockQueue()` is built as a thread-safe alternative to the other queue types.
## Example Benchmarks
### Queue operations
Ubuntu


Windows
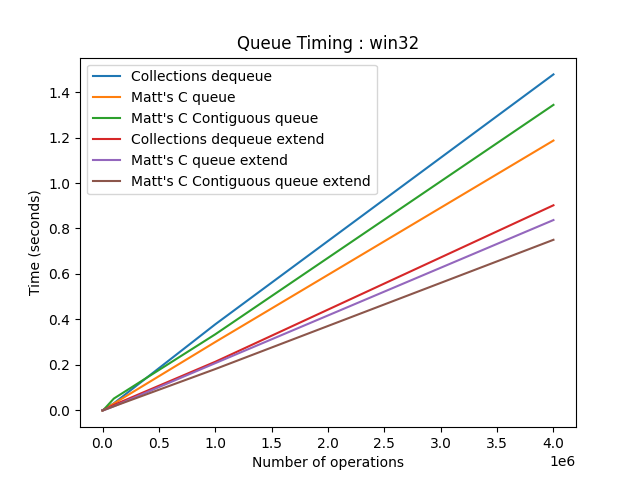
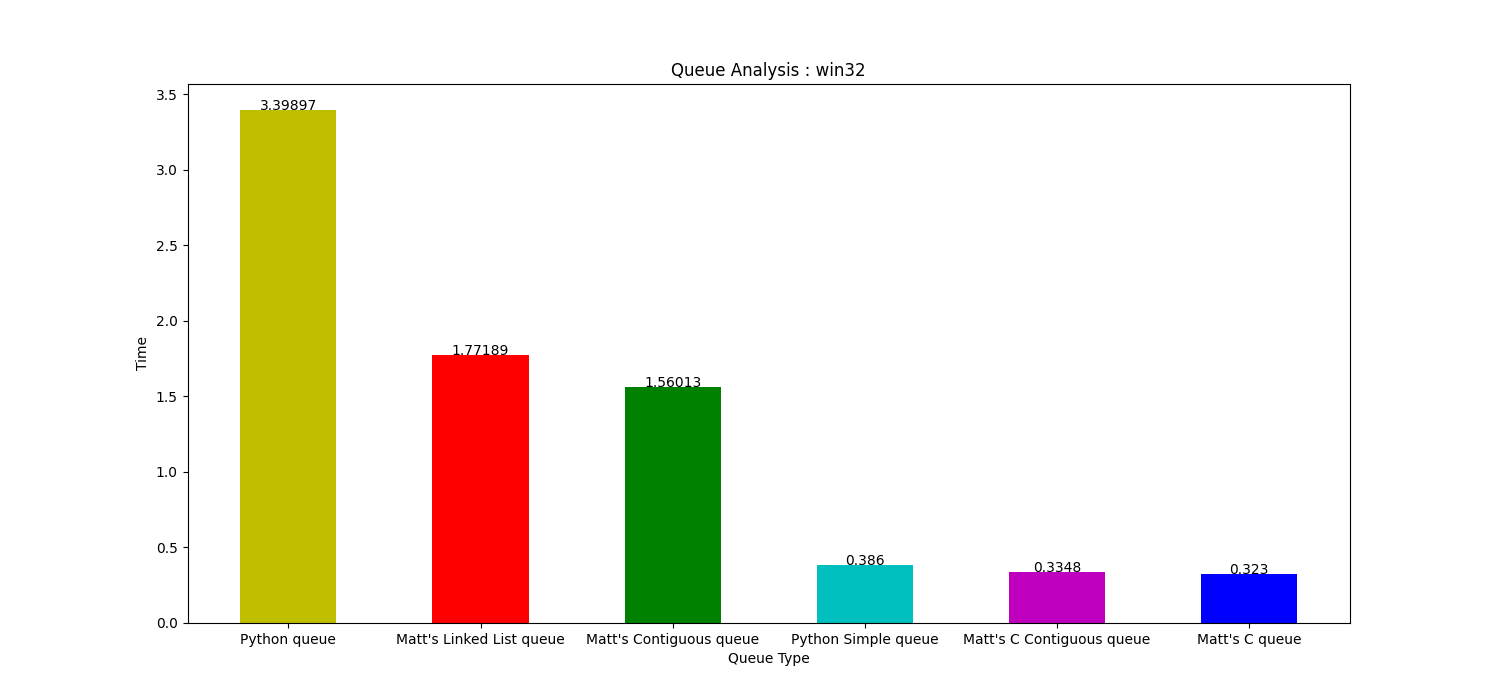
### Iteration
Ubuntu


Windows


Raw data
{
"_id": null,
"home_page": "",
"name": "fastqueue-lib",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.7",
"maintainer_email": "",
"keywords": "Queue",
"author": "",
"author_email": "Matthew Taylor <matthew.taylor.andre@gmail.com>",
"download_url": "https://files.pythonhosted.org/packages/80/e2/ec12040e38ba3f8d9797957d384b5953a390d16a1c114e6bc246dbc972ab/fastqueue-lib-0.0.17.tar.gz",
"platform": null,
"description": "# fastqueue\n\n[](https://github.com/MatthewAndreTaylor/fastqueue/actions)\n[](https://github.com/MatthewAndreTaylor/fastqueue/)\n[](https://github.com/MatthewAndreTaylor/fastqueue/blob/master/LICENSE)\\\n[](https://pypi.org/project/fastqueue-lib/)\n\n\nSingle-ended fast queues built in C tuned for Python.\n\n## Requirements\n\n- `python 3.7+`\n\n## Installation\n\nTo install fastqueue, using pip:\n\n```bash\npip install fastqueue-lib\n```\n\n## Quickstart\n\nFor general use cases `fastqueue.Queue()` objects are tuned to perform well.\nThe enqueue and dequeue methods perform well over a large sequence of arbitrary operations.\n`fastqueue.Queue()` supports many standard sequence methods similar to lists.\n`fastqueue.Queue()` minimizes memory usage but maintains the fast queue speeds.\n\n```py\n>>> from fastqueue import Queue\n>>> queue = Queue()\n>>> queue.extend(['\ud83d\ude92', '\ud83d\udef4'])\n>>> queue[0]\n'\ud83d\ude92'\n>>> '\ud83d\udef4' in queue\nTrue\n>>> queue.enqueue('\ud83d\ude85')\n>>> queue.enqueue('\ud83d\ude97')\n>>> queue[-1]\n'\ud83d\ude97'\n>>> [queue.dequeue() for _ in range(len(queue)) ]\n['\ud83d\ude92', '\ud83d\udef4', '\ud83d\ude85', '\ud83d\ude97']\n```\n\n\nFor more specialized cases `fastqueue.QueueC()` objects are tuned to perform well.\nThe interface for `fastqueue.QueueC()` is identical to `fastqueue.Queue()`.\nThe enqueue and dequeue methods perform similarly well over a large sequence of arbitrary operations.\n`fastqueue.QueueC()` handles memory differently by doubling the capacity when full.\nThis increases the complexity but maintains fast amortized cost.\nThe benefit of this approach is even faster `__getitem__` and `__setitem__` speeds\n\n```py\n>>> from fastqueue import QueueC\n\n>>> queue_c = QueueC()\n>>> queue_c.extend(['\ud83d\ude92', '\ud83d\udef4'])\n>>> queue_c[0]\n'\ud83d\ude92'\n>>> '\ud83d\udef4' in queue_c\nTrue\n>>> queue_c.enqueue('\ud83d\ude85')\n>>> queue_c.enqueue('\ud83d\ude97')\n>>> queue_c[-1]\n'\ud83d\ude97'\n>>> [queue_c.dequeue() for _ in range(len(queue_c)) ]\n['\ud83d\ude92', '\ud83d\udef4', '\ud83d\ude85', '\ud83d\ude97']\n```\n\nAnother alternative is `fastqueue.LockQueue()` which supports all queue operations.\n`fastqueue.LockQueue()` is built as a thread-safe alternative to the other queue types.\n\n## Example Benchmarks\n\n### Queue operations\n\nUbuntu\n\n\n\n\nWindows\n\n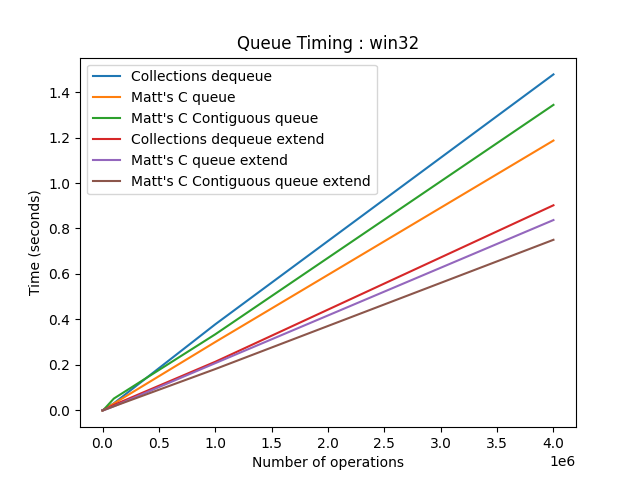\n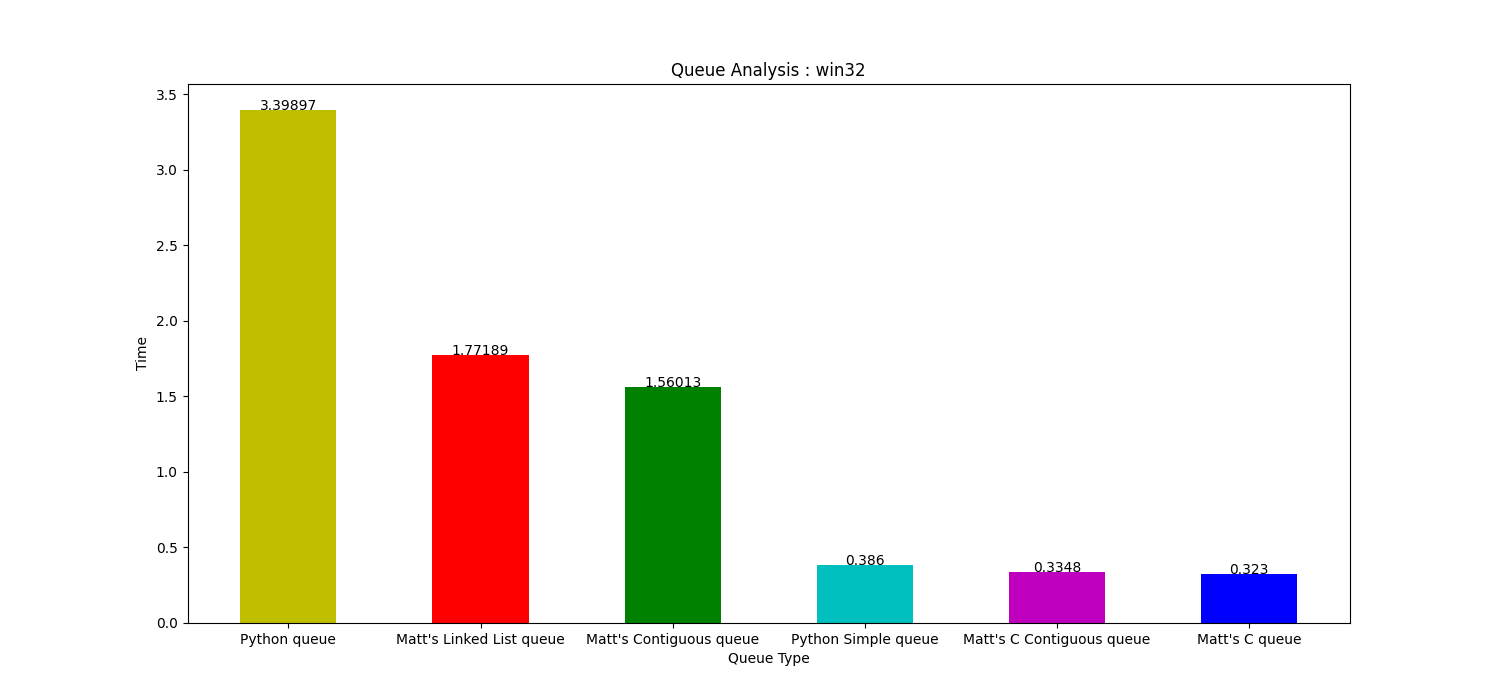\n\n### Iteration\n\n\nUbuntu\n\n\n\n\nWindows\n\n\n\n\n",
"bugtrack_url": null,
"license": "",
"summary": "Fast single ended queue library for python",
"version": "0.0.17",
"project_urls": {
"Homepage": "https://github.com/MatthewAndreTaylor/fastqueue"
},
"split_keywords": [
"queue"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "bd5befa58fb7d507190d33bdccde2564c073b54ca85df6236bb45a661ed5e0e5",
"md5": "13d15c699a1b09789bf51e632c4f5484",
"sha256": "9ece9f1a61c9d42a8e4c88aac389b2092e82c5feeb37cdec0888f709cec835ee"
},
"downloads": -1,
"filename": "fastqueue_lib-0.0.17-cp39-cp39-manylinux_2_5_x86_64.manylinux1_x86_64.whl",
"has_sig": false,
"md5_digest": "13d15c699a1b09789bf51e632c4f5484",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.7",
"size": 63172,
"upload_time": "2023-10-22T05:32:20",
"upload_time_iso_8601": "2023-10-22T05:32:20.177311Z",
"url": "https://files.pythonhosted.org/packages/bd/5b/efa58fb7d507190d33bdccde2564c073b54ca85df6236bb45a661ed5e0e5/fastqueue_lib-0.0.17-cp39-cp39-manylinux_2_5_x86_64.manylinux1_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "80e2ec12040e38ba3f8d9797957d384b5953a390d16a1c114e6bc246dbc972ab",
"md5": "72dbda0f79abb2f627b777d7e28bdc16",
"sha256": "e0911d698f8b10f3f7f6d1101d54b59b37a085b12ad8da793703a303bb4f308f"
},
"downloads": -1,
"filename": "fastqueue-lib-0.0.17.tar.gz",
"has_sig": false,
"md5_digest": "72dbda0f79abb2f627b777d7e28bdc16",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.7",
"size": 11845,
"upload_time": "2023-10-22T05:32:21",
"upload_time_iso_8601": "2023-10-22T05:32:21.862327Z",
"url": "https://files.pythonhosted.org/packages/80/e2/ec12040e38ba3f8d9797957d384b5953a390d16a1c114e6bc246dbc972ab/fastqueue-lib-0.0.17.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-22 05:32:21",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "MatthewAndreTaylor",
"github_project": "fastqueue",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"tox": true,
"lcname": "fastqueue-lib"
}