Name | faust-streaming JSON |
Version |
0.11.2
JSON |
| download |
home_page | None |
Summary | Python Stream Processing. A Faust fork |
upload_time | 2024-08-08 17:53:02 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.8.0 |
license | Copyright (c) 2017-2020, Robinhood Markets, Inc. All rights reserved. Faust is licensed under The BSD License (3 Clause, also known as the new BSD license). The license is an OSI approved Open Source license and is GPL-compatible(1). The license text can also be found here: http://www.opensource.org/licenses/BSD-3-Clause License ======= Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of Robinhood Markets, Inc. nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL Robinhood Markets, Inc. OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. Documentation License ===================== The documentation portion of Faust (the rendered contents of the "docs" directory of a software distribution or checkout) is supplied under the "Creative Commons Attribution-ShareAlike 4.0 International" (CC BY-SA 4.0) License as described by http://creativecommons.org/licenses/by-sa/4.0/ Footnotes ========= (1) A GPL-compatible license makes it possible to combine Faust with other software that is released under the GPL, it does not mean that we're distributing Faust under the GPL license. The BSD license, unlike the GPL, let you distribute a modified version without making your changes open source. |
keywords |
stream
processing
asyncio
distributed
queue
kafka
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
|
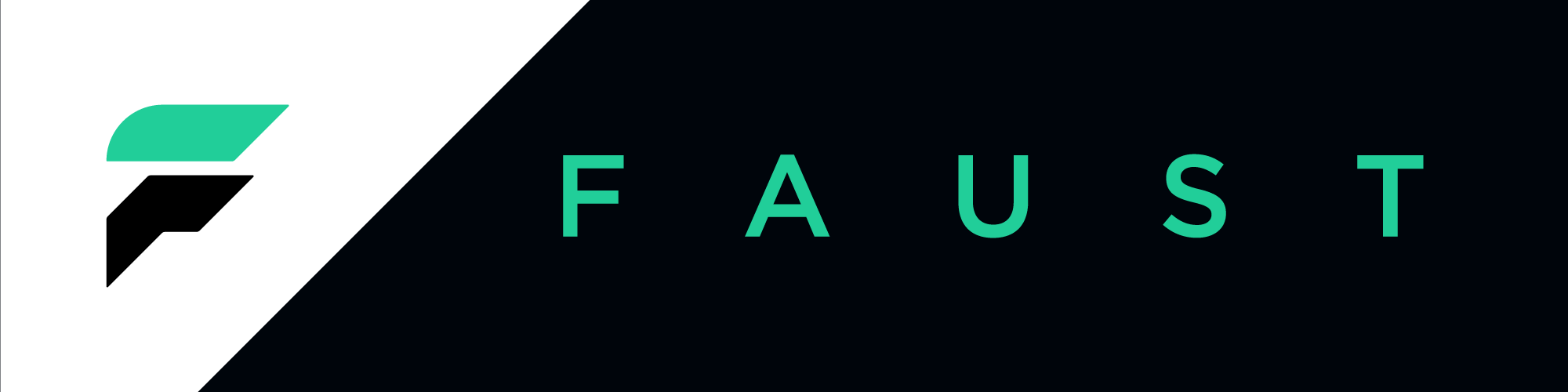
# Python Stream Processing Fork


[](https://codecov.io/gh/faust-streaming/faust)
[](https://join.slack.com/t/fauststreaming/shared_invite/zt-1q1jhq4kh-Q1t~rJgpyuMQ6N38cByE9g)
[](https://github.com/psf/black)



## Installation
`pip install faust-streaming`
## Documentation
- `introduction`: https://faust-streaming.github.io/faust/introduction.html
- `quickstart`: https://faust-streaming.github.io/faust/playbooks/quickstart.html
- `User Guide`: https://faust-streaming.github.io/faust/userguide/index.html
## Why the fork
We have decided to fork the original `Faust` project because there is a critical process of releasing new versions which causes uncertainty in the community. Everybody is welcome to contribute to this `fork`, and you can be added as a maintainer.
We want to:
- Ensure continues release
- Code quality
- Use of latest versions of kafka drivers (for now only [aiokafka](https://github.com/aio-libs/aiokafka))
- Support kafka transactions
- Update the documentation
and more...
## Usage
```python
# Python Streams
# Forever scalable event processing & in-memory durable K/V store;
# as a library w/ asyncio & static typing.
import faust
```
**Faust** is a stream processing library, porting the ideas from
`Kafka Streams` to Python.
It is used at `Robinhood` to build high performance distributed systems
and real-time data pipelines that process billions of events every day.
Faust provides both *stream processing* and *event processing*,
sharing similarity with tools such as `Kafka Streams`, `Apache Spark`, `Storm`, `Samza`, `Flink`,
It does not use a DSL, it's just Python!
This means you can use all your favorite Python libraries
when stream processing: NumPy, PyTorch, Pandas, NLTK, Django,
Flask, SQLAlchemy, ++
Faust requires Python 3.6 or later for the new `async/await`_ syntax,
and variable type annotations.
Here's an example processing a stream of incoming orders:
```python
app = faust.App('myapp', broker='kafka://localhost')
# Models describe how messages are serialized:
# {"account_id": "3fae-...", amount": 3}
class Order(faust.Record):
account_id: str
amount: int
@app.agent(value_type=Order)
async def order(orders):
async for order in orders:
# process infinite stream of orders.
print(f'Order for {order.account_id}: {order.amount}')
```
The Agent decorator defines a "stream processor" that essentially
consumes from a Kafka topic and does something for every event it receives.
The agent is an `async def` function, so can also perform
other operations asynchronously, such as web requests.
This system can persist state, acting like a database.
Tables are named distributed key/value stores you can use
as regular Python dictionaries.
Tables are stored locally on each machine using a super fast
embedded database written in C++, called `RocksDB`.
Tables can also store aggregate counts that are optionally "windowed"
so you can keep track
of "number of clicks from the last day," or
"number of clicks in the last hour." for example. Like `Kafka Streams`,
we support tumbling, hopping and sliding windows of time, and old windows
can be expired to stop data from filling up.
For reliability, we use a Kafka topic as "write-ahead-log".
Whenever a key is changed we publish to the changelog.
Standby nodes consume from this changelog to keep an exact replica
of the data and enables instant recovery should any of the nodes fail.
To the user a table is just a dictionary, but data is persisted between
restarts and replicated across nodes so on failover other nodes can take over
automatically.
You can count page views by URL:
```python
# data sent to 'clicks' topic sharded by URL key.
# e.g. key="http://example.com" value="1"
click_topic = app.topic('clicks', key_type=str, value_type=int)
# default value for missing URL will be 0 with `default=int`
counts = app.Table('click_counts', default=int)
@app.agent(click_topic)
async def count_click(clicks):
async for url, count in clicks.items():
counts[url] += count
```
The data sent to the Kafka topic is partitioned, which means
the clicks will be sharded by URL in such a way that every count
for the same URL will be delivered to the same Faust worker instance.
Faust supports any type of stream data: bytes, Unicode and serialized
structures, but also comes with "Models" that use modern Python
syntax to describe how keys and values in streams are serialized:
```python
# Order is a json serialized dictionary,
# having these fields:
class Order(faust.Record):
account_id: str
product_id: str
price: float
quantity: float = 1.0
orders_topic = app.topic('orders', key_type=str, value_type=Order)
@app.agent(orders_topic)
async def process_order(orders):
async for order in orders:
# process each order using regular Python
total_price = order.price * order.quantity
await send_order_received_email(order.account_id, order)
```
Faust is statically typed, using the `mypy` type checker,
so you can take advantage of static types when writing applications.
The Faust source code is small, well organized, and serves as a good
resource for learning the implementation of `Kafka Streams`.
**Learn more about Faust in the** `introduction` **introduction page**
to read more about Faust, system requirements, installation instructions,
community resources, and more.
**or go directly to the** `quickstart` **tutorial**
to see Faust in action by programming a streaming application.
**then explore the** `User Guide`
for in-depth information organized by topic.
- `Robinhood`: http://robinhood.com
- `async/await`:https://medium.freecodecamp.org/a-guide-to-asynchronous-programming-in-python-with-asyncio-232e2afa44f6
- `Celery`: http://celeryproject.org
- `Kafka Streams`: https://kafka.apache.org/documentation/streams
- `Apache Spark`: http://spark.apache.org
- `Storm`: http://storm.apache.org
- `Samza`: http://samza.apache.org
- `Flink`: http://flink.apache.org
- `RocksDB`: http://rocksdb.org
- `Aerospike`: https://www.aerospike.com/
- `Apache Kafka`: https://kafka.apache.org
## Local development
1. Clone the project
2. Create a virtualenv: `python3.7 -m venv venv && source venv/bin/activate`
3. Install the requirements: `./scripts/install`
4. Run lint: `./scripts/lint`
5. Run tests: `./scripts/tests`
## Faust key points
### Simple
Faust is extremely easy to use. To get started using other stream processing
solutions you have complicated hello-world projects, and
infrastructure requirements. Faust only requires Kafka,
the rest is just Python, so If you know Python you can already use Faust to do
stream processing, and it can integrate with just about anything.
Here's one of the easier applications you can make::
```python
import faust
class Greeting(faust.Record):
from_name: str
to_name: str
app = faust.App('hello-app', broker='kafka://localhost')
topic = app.topic('hello-topic', value_type=Greeting)
@app.agent(topic)
async def hello(greetings):
async for greeting in greetings:
print(f'Hello from {greeting.from_name} to {greeting.to_name}')
@app.timer(interval=1.0)
async def example_sender(app):
await hello.send(
value=Greeting(from_name='Faust', to_name='you'),
)
if __name__ == '__main__':
app.main()
```
You're probably a bit intimidated by the `async` and `await` keywords,
but you don't have to know how ``asyncio`` works to use
Faust: just mimic the examples, and you'll be fine.
The example application starts two tasks: one is processing a stream,
the other is a background thread sending events to that stream.
In a real-life application, your system will publish
events to Kafka topics that your processors can consume from,
and the background thread is only needed to feed data into our
example.
### Highly Available
Faust is highly available and can survive network problems and server
crashes. In the case of node failure, it can automatically recover,
and tables have standby nodes that will take over.
### Distributed
Start more instances of your application as needed.
### Fast
A single-core Faust worker instance can already process tens of thousands
of events every second, and we are reasonably confident that throughput will
increase once we can support a more optimized Kafka client.
### Flexible
Faust is just Python, and a stream is an infinite asynchronous iterator.
If you know how to use Python, you already know how to use Faust,
and it works with your favorite Python libraries like Django, Flask,
SQLAlchemy, NLTK, NumPy, SciPy, TensorFlow, etc.
## Bundles
Faust also defines a group of ``setuptools`` extensions that can be used
to install Faust and the dependencies for a given feature.
You can specify these in your requirements or on the ``pip``
command-line by using brackets. Separate multiple bundles using the comma:
```sh
pip install "faust-streaming[rocksdb]"
pip install "faust-streaming[rocksdb,uvloop,fast,redis,aerospike]"
```
The following bundles are available:
## Faust with extras
### Stores
#### RocksDB
For using `RocksDB` for storing Faust table state. **Recommended in production.**
`pip install faust-streaming[rocksdb]` (uses RocksDB 6)
`pip install faust-streaming[rocksdict]` (uses RocksDB 8, not backwards compatible with 6)
#### Aerospike
`pip install faust-streaming[aerospike]` for using `Aerospike` for storing Faust table state. **Recommended if supported**
### Aerospike Configuration
Aerospike can be enabled as the state store by specifying
`store="aerospike://"`
By default, all tables backed by Aerospike use `use_partitioner=True` and generate changelog topic events similar
to a state store backed by RocksDB.
The following configuration options should be passed in as keys to the options parameter in [Table](https://faust-streaming.github.io/faust/reference/faust.tables.html)
`namespace` : aerospike namespace
`ttl`: TTL for all KV's in the table
`username`: username to connect to the Aerospike cluster
`password`: password to connect to the Aerospike cluster
`hosts` : the hosts parameter as specified in the [aerospike client](https://www.aerospike.com/apidocs/python/aerospike.html)
`policies`: the different policies for read/write/scans [policies](https://www.aerospike.com/apidocs/python/aerospike.html)
`client`: a dict of `host` and `policies` defined above
### Caching
`faust-streaming[redis]` for using `Redis` as a simple caching backend (Memcached-style).
### Codecs
`faust-streaming[yaml]` for using YAML and the `PyYAML` library in streams.
### Optimization
`faust-streaming[fast]` for installing all the available C speedup extensions to Faust core.
### Sensors
`faust-streaming[datadog]` for using the `Datadog` Faust monitor.
`faust-streaming[statsd]` for using the `Statsd` Faust monitor.
`faust-streaming[prometheus]` for using the `Prometheus` Faust monitor.
### Event Loops
`faust-streaming[uvloop]` for using Faust with `uvloop`.
`faust-streaming[eventlet]` for using Faust with `eventlet`
### Debugging
`faust-streaming[debug]` for using `aiomonitor` to connect and debug a running Faust worker.
`faust-streaming[setproctitle]`when the `setproctitle` module is installed the Faust worker will use it to set a nicer process name in `ps`/`top` listings.vAlso installed with the `fast` and `debug` bundles.
## Downloading and installing from source
Download the latest version of Faust from https://pypi.org/project/faust-streaming/
You can install it by doing:
```sh
$ tar xvfz faust-streaming-0.0.0.tar.gz
$ cd faust-streaming-0.0.0
$ python setup.py build
# python setup.py install
```
The last command must be executed as a privileged user if
you are not currently using a virtualenv.
## Using the development version
### With pip
You can install the latest snapshot of Faust using the following `pip` command:
```sh
pip install https://github.com/faust-streaming/faust/zipball/master#egg=faust
```
## FAQ
### Can I use Faust with Django/Flask/etc
Yes! Use ``eventlet`` as a bridge to integrate with ``asyncio``.
### Using eventlet
This approach works with any blocking Python library that can work with `eventlet`
Using `eventlet` requires you to install the `faust-aioeventlet` module,
and you can install this as a bundle along with Faust:
```sh
pip install -U faust-streaming[eventlet]
```
Then to actually use eventlet as the event loop you have to either
use the `-L <faust --loop>` argument to the `faust` program:
```sh
faust -L eventlet -A myproj worker -l info
```
or add `import mode.loop.eventlet` at the top of your entry point script:
```python
#!/usr/bin/env python3
import mode.loop.eventlet # noqa
```
It's very important this is at the very top of the module,
and that it executes before you import libraries.
### Can I use Faust with Tornado
Yes! Use the `tornado.platform.asyncio` [bridge](http://www.tornadoweb.org/en/stable/asyncio.html)
### Can I use Faust with Twisted
Yes! Use the `asyncio` reactor implementation: https://twistedmatrix.com/documents/current/api/twisted.internet.asyncioreactor.html
### Will you support Python 2.7 or Python 3.5
No. Faust requires Python 3.8 or later, since it heavily uses features that were
introduced in Python 3.6 (`async`, `await`, variable type annotations).
### I get a maximum number of open files exceeded error by RocksDB when running a Faust app locally. How can I fix this
You may need to increase the limit for the maximum number of open files.
On macOS and Linux you can use:
```ulimit -n max_open_files``` to increase the open files limit to max_open_files.
On docker, you can use the --ulimit flag:
```docker run --ulimit nofile=50000:100000 <image-tag>```
where 50000 is the soft limit, and 100000 is the hard limit [See the difference](https://unix.stackexchange.com/a/29579).
### What kafka versions faust supports
Faust supports kafka with version >= 0.10.
## Getting Help
### Slack
For discussions about the usage, development, and future of Faust, please join the `fauststream` Slack.
- https://fauststream.slack.com
- Sign-up: https://join.slack.com/t/fauststreaming/shared_invite/zt-1q1jhq4kh-Q1t~rJgpyuMQ6N38cByE9g
## Resources
### Bug tracker
If you have any suggestions, bug reports, or annoyances please report them
to our issue tracker at https://github.com/faust-streaming/faust/issues/
## License
This software is licensed under the `New BSD License`. See the `LICENSE` file in the top distribution directory for the full license text.
### Contributing
Development of `Faust` happens at [GitHub](https://github.com/faust-streaming/faust)
You're highly encouraged to participate in the development of `Faust`.
### Code of Conduct
Everyone interacting in the project's code bases, issue trackers, chat rooms,
and mailing lists is expected to follow the Faust Code of Conduct.
As contributors and maintainers of these projects, and in the interest of fostering
an open and welcoming community, we pledge to respect all people who contribute
through reporting issues, posting feature requests, updating documentation,
submitting pull requests or patches, and other activities.
We are committed to making participation in these projects a harassment-free
experience for everyone, regardless of level of experience, gender,
gender identity and expression, sexual orientation, disability,
personal appearance, body size, race, ethnicity, age,
religion, or nationality.
Examples of unacceptable behavior by participants include:
- The use of sexualized language or imagery
- Personal attacks
- Trolling or insulting/derogatory comments
- Public or private harassment
- Publishing other's private information, such as physical or electronic addresses, without explicit permission
- Other unethical or unprofessional conduct.
Project maintainers have the right and responsibility to remove, edit, or reject
comments, commits, code, wiki edits, issues, and other contributions that are
not aligned to this Code of Conduct. By adopting this Code of Conduct,
project maintainers commit themselves to fairly and consistently applying
these principles to every aspect of managing this project. Project maintainers
who do not follow or enforce the Code of Conduct may be permanently removed from
the project team.
This code of conduct applies both within project spaces and in public spaces
when an individual is representing the project or its community.
Instances of abusive, harassing, or otherwise unacceptable behavior may be
reported by opening an issue or contacting one or more of the project maintainers.
Raw data
{
"_id": null,
"home_page": null,
"name": "faust-streaming",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8.0",
"maintainer_email": "Vikram Patki <vpatki@wayfair.com>, William Barnhart <williambbarnhart@gmail.com>",
"keywords": "stream, processing, asyncio, distributed, queue, kafka",
"author": null,
"author_email": "Ask Solem Hoel <ask@robinhood.com>, Vineet Goel <vineet@robinhood.com>",
"download_url": "https://files.pythonhosted.org/packages/90/1b/cebacd8ff162487555e19d8337bb69d081da034d1fe842bf75752e97ad36/faust-streaming-0.11.2.tar.gz",
"platform": null,
"description": "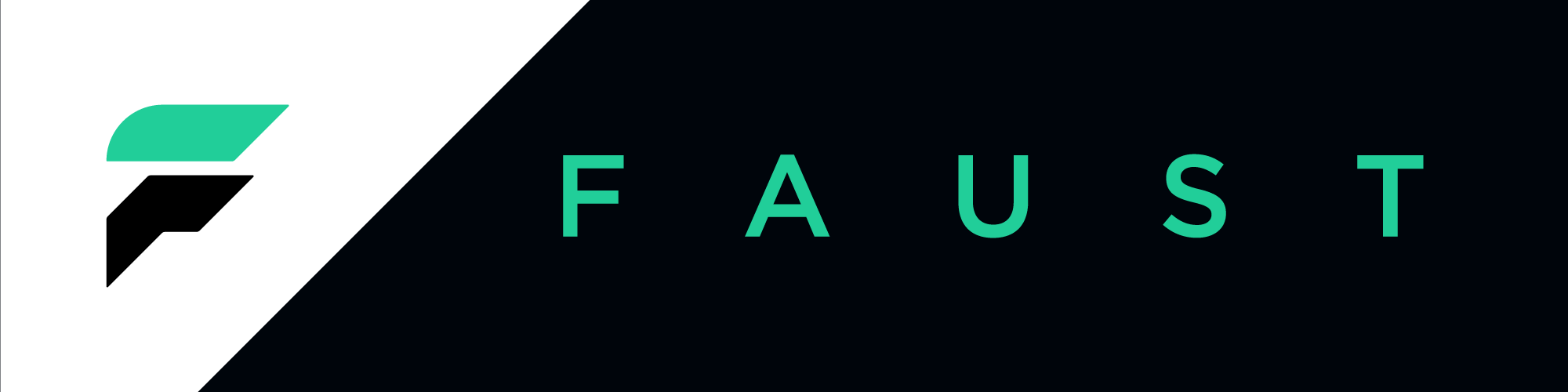\n\n# Python Stream Processing Fork\n\n\n\n[](https://codecov.io/gh/faust-streaming/faust)\n[](https://join.slack.com/t/fauststreaming/shared_invite/zt-1q1jhq4kh-Q1t~rJgpyuMQ6N38cByE9g)\n[](https://github.com/psf/black)\n\n\n\n\n## Installation\n\n`pip install faust-streaming`\n\n## Documentation\n\n- `introduction`: https://faust-streaming.github.io/faust/introduction.html\n- `quickstart`: https://faust-streaming.github.io/faust/playbooks/quickstart.html\n- `User Guide`: https://faust-streaming.github.io/faust/userguide/index.html\n\n## Why the fork\n\nWe have decided to fork the original `Faust` project because there is a critical process of releasing new versions which causes uncertainty in the community. Everybody is welcome to contribute to this `fork`, and you can be added as a maintainer.\n\nWe want to:\n\n- Ensure continues release\n- Code quality\n- Use of latest versions of kafka drivers (for now only [aiokafka](https://github.com/aio-libs/aiokafka))\n- Support kafka transactions\n- Update the documentation\n\nand more...\n\n## Usage\n\n```python\n# Python Streams\n# Forever scalable event processing & in-memory durable K/V store;\n# as a library w/ asyncio & static typing.\nimport faust\n```\n\n**Faust** is a stream processing library, porting the ideas from\n`Kafka Streams` to Python.\n\nIt is used at `Robinhood` to build high performance distributed systems\nand real-time data pipelines that process billions of events every day.\n\nFaust provides both *stream processing* and *event processing*,\nsharing similarity with tools such as `Kafka Streams`, `Apache Spark`, `Storm`, `Samza`, `Flink`,\n\nIt does not use a DSL, it's just Python!\nThis means you can use all your favorite Python libraries\nwhen stream processing: NumPy, PyTorch, Pandas, NLTK, Django,\nFlask, SQLAlchemy, ++\n\nFaust requires Python 3.6 or later for the new `async/await`_ syntax,\nand variable type annotations.\n\nHere's an example processing a stream of incoming orders:\n\n```python\n\napp = faust.App('myapp', broker='kafka://localhost')\n\n# Models describe how messages are serialized:\n# {\"account_id\": \"3fae-...\", amount\": 3}\nclass Order(faust.Record):\n account_id: str\n amount: int\n\n@app.agent(value_type=Order)\nasync def order(orders):\n async for order in orders:\n # process infinite stream of orders.\n print(f'Order for {order.account_id}: {order.amount}')\n```\n\nThe Agent decorator defines a \"stream processor\" that essentially\nconsumes from a Kafka topic and does something for every event it receives.\n\nThe agent is an `async def` function, so can also perform\nother operations asynchronously, such as web requests.\n\nThis system can persist state, acting like a database.\nTables are named distributed key/value stores you can use\nas regular Python dictionaries.\n\nTables are stored locally on each machine using a super fast\nembedded database written in C++, called `RocksDB`.\n\nTables can also store aggregate counts that are optionally \"windowed\"\nso you can keep track\nof \"number of clicks from the last day,\" or\n\"number of clicks in the last hour.\" for example. Like `Kafka Streams`,\nwe support tumbling, hopping and sliding windows of time, and old windows\ncan be expired to stop data from filling up.\n\nFor reliability, we use a Kafka topic as \"write-ahead-log\".\nWhenever a key is changed we publish to the changelog.\nStandby nodes consume from this changelog to keep an exact replica\nof the data and enables instant recovery should any of the nodes fail.\n\nTo the user a table is just a dictionary, but data is persisted between\nrestarts and replicated across nodes so on failover other nodes can take over\nautomatically.\n\nYou can count page views by URL:\n\n```python\n# data sent to 'clicks' topic sharded by URL key.\n# e.g. key=\"http://example.com\" value=\"1\"\nclick_topic = app.topic('clicks', key_type=str, value_type=int)\n\n# default value for missing URL will be 0 with `default=int`\ncounts = app.Table('click_counts', default=int)\n\n@app.agent(click_topic)\nasync def count_click(clicks):\n async for url, count in clicks.items():\n counts[url] += count\n```\n\nThe data sent to the Kafka topic is partitioned, which means\nthe clicks will be sharded by URL in such a way that every count\nfor the same URL will be delivered to the same Faust worker instance.\n\nFaust supports any type of stream data: bytes, Unicode and serialized\nstructures, but also comes with \"Models\" that use modern Python\nsyntax to describe how keys and values in streams are serialized:\n\n```python\n# Order is a json serialized dictionary,\n# having these fields:\n\nclass Order(faust.Record):\n account_id: str\n product_id: str\n price: float\n quantity: float = 1.0\n\norders_topic = app.topic('orders', key_type=str, value_type=Order)\n\n@app.agent(orders_topic)\nasync def process_order(orders):\n async for order in orders:\n # process each order using regular Python\n total_price = order.price * order.quantity\n await send_order_received_email(order.account_id, order)\n```\n\nFaust is statically typed, using the `mypy` type checker,\nso you can take advantage of static types when writing applications.\n\nThe Faust source code is small, well organized, and serves as a good\nresource for learning the implementation of `Kafka Streams`.\n\n**Learn more about Faust in the** `introduction` **introduction page**\n to read more about Faust, system requirements, installation instructions,\n community resources, and more.\n\n**or go directly to the** `quickstart` **tutorial**\n to see Faust in action by programming a streaming application.\n\n**then explore the** `User Guide`\n for in-depth information organized by topic.\n\n- `Robinhood`: http://robinhood.com\n- `async/await`:https://medium.freecodecamp.org/a-guide-to-asynchronous-programming-in-python-with-asyncio-232e2afa44f6\n- `Celery`: http://celeryproject.org\n- `Kafka Streams`: https://kafka.apache.org/documentation/streams\n- `Apache Spark`: http://spark.apache.org\n- `Storm`: http://storm.apache.org\n- `Samza`: http://samza.apache.org\n- `Flink`: http://flink.apache.org\n- `RocksDB`: http://rocksdb.org\n- `Aerospike`: https://www.aerospike.com/\n- `Apache Kafka`: https://kafka.apache.org\n\n## Local development\n\n1. Clone the project\n2. Create a virtualenv: `python3.7 -m venv venv && source venv/bin/activate`\n3. Install the requirements: `./scripts/install`\n4. Run lint: `./scripts/lint`\n5. Run tests: `./scripts/tests`\n\n## Faust key points\n\n### Simple\n\nFaust is extremely easy to use. To get started using other stream processing\nsolutions you have complicated hello-world projects, and\ninfrastructure requirements. Faust only requires Kafka,\nthe rest is just Python, so If you know Python you can already use Faust to do\nstream processing, and it can integrate with just about anything.\n\nHere's one of the easier applications you can make::\n\n```python\nimport faust\n\nclass Greeting(faust.Record):\n from_name: str\n to_name: str\n\napp = faust.App('hello-app', broker='kafka://localhost')\ntopic = app.topic('hello-topic', value_type=Greeting)\n\n@app.agent(topic)\nasync def hello(greetings):\n async for greeting in greetings:\n print(f'Hello from {greeting.from_name} to {greeting.to_name}')\n\n@app.timer(interval=1.0)\nasync def example_sender(app):\n await hello.send(\n value=Greeting(from_name='Faust', to_name='you'),\n )\n\nif __name__ == '__main__':\n app.main()\n```\n\nYou're probably a bit intimidated by the `async` and `await` keywords,\nbut you don't have to know how ``asyncio`` works to use\nFaust: just mimic the examples, and you'll be fine.\n\nThe example application starts two tasks: one is processing a stream,\nthe other is a background thread sending events to that stream.\nIn a real-life application, your system will publish\nevents to Kafka topics that your processors can consume from,\nand the background thread is only needed to feed data into our\nexample.\n\n### Highly Available\n\nFaust is highly available and can survive network problems and server\ncrashes. In the case of node failure, it can automatically recover,\nand tables have standby nodes that will take over.\n\n### Distributed\n\nStart more instances of your application as needed.\n\n### Fast\n\nA single-core Faust worker instance can already process tens of thousands\nof events every second, and we are reasonably confident that throughput will\nincrease once we can support a more optimized Kafka client.\n\n### Flexible\n\nFaust is just Python, and a stream is an infinite asynchronous iterator.\nIf you know how to use Python, you already know how to use Faust,\nand it works with your favorite Python libraries like Django, Flask,\nSQLAlchemy, NLTK, NumPy, SciPy, TensorFlow, etc.\n\n## Bundles\n\nFaust also defines a group of ``setuptools`` extensions that can be used\nto install Faust and the dependencies for a given feature.\n\nYou can specify these in your requirements or on the ``pip``\ncommand-line by using brackets. Separate multiple bundles using the comma:\n\n```sh\npip install \"faust-streaming[rocksdb]\"\n\npip install \"faust-streaming[rocksdb,uvloop,fast,redis,aerospike]\"\n```\n\nThe following bundles are available:\n\n## Faust with extras\n\n### Stores\n\n#### RocksDB\n\nFor using `RocksDB` for storing Faust table state. **Recommended in production.**\n\n`pip install faust-streaming[rocksdb]` (uses RocksDB 6)\n\n`pip install faust-streaming[rocksdict]` (uses RocksDB 8, not backwards compatible with 6)\n\n\n#### Aerospike\n\n`pip install faust-streaming[aerospike]` for using `Aerospike` for storing Faust table state. **Recommended if supported**\n\n### Aerospike Configuration\nAerospike can be enabled as the state store by specifying\n`store=\"aerospike://\"`\n\nBy default, all tables backed by Aerospike use `use_partitioner=True` and generate changelog topic events similar\nto a state store backed by RocksDB.\nThe following configuration options should be passed in as keys to the options parameter in [Table](https://faust-streaming.github.io/faust/reference/faust.tables.html)\n`namespace` : aerospike namespace\n\n`ttl`: TTL for all KV's in the table\n\n`username`: username to connect to the Aerospike cluster\n\n`password`: password to connect to the Aerospike cluster\n\n`hosts` : the hosts parameter as specified in the [aerospike client](https://www.aerospike.com/apidocs/python/aerospike.html)\n\n`policies`: the different policies for read/write/scans [policies](https://www.aerospike.com/apidocs/python/aerospike.html)\n\n`client`: a dict of `host` and `policies` defined above\n\n### Caching\n\n`faust-streaming[redis]` for using `Redis` as a simple caching backend (Memcached-style).\n\n### Codecs\n\n`faust-streaming[yaml]` for using YAML and the `PyYAML` library in streams.\n\n### Optimization\n\n`faust-streaming[fast]` for installing all the available C speedup extensions to Faust core.\n\n### Sensors\n\n`faust-streaming[datadog]` for using the `Datadog` Faust monitor.\n\n`faust-streaming[statsd]` for using the `Statsd` Faust monitor.\n\n`faust-streaming[prometheus]` for using the `Prometheus` Faust monitor.\n\n### Event Loops\n\n`faust-streaming[uvloop]` for using Faust with `uvloop`.\n\n`faust-streaming[eventlet]` for using Faust with `eventlet`\n\n### Debugging\n\n`faust-streaming[debug]` for using `aiomonitor` to connect and debug a running Faust worker.\n\n`faust-streaming[setproctitle]`when the `setproctitle` module is installed the Faust worker will use it to set a nicer process name in `ps`/`top` listings.vAlso installed with the `fast` and `debug` bundles.\n\n## Downloading and installing from source\n\nDownload the latest version of Faust from https://pypi.org/project/faust-streaming/\n\nYou can install it by doing:\n\n```sh\n$ tar xvfz faust-streaming-0.0.0.tar.gz\n$ cd faust-streaming-0.0.0\n$ python setup.py build\n# python setup.py install\n```\n\nThe last command must be executed as a privileged user if\nyou are not currently using a virtualenv.\n\n## Using the development version\n\n### With pip\n\nYou can install the latest snapshot of Faust using the following `pip` command:\n\n```sh\npip install https://github.com/faust-streaming/faust/zipball/master#egg=faust\n```\n\n## FAQ\n\n### Can I use Faust with Django/Flask/etc\n\nYes! Use ``eventlet`` as a bridge to integrate with ``asyncio``.\n\n### Using eventlet\n\nThis approach works with any blocking Python library that can work with `eventlet`\n\nUsing `eventlet` requires you to install the `faust-aioeventlet` module,\nand you can install this as a bundle along with Faust:\n\n```sh\npip install -U faust-streaming[eventlet]\n```\n\nThen to actually use eventlet as the event loop you have to either\nuse the `-L <faust --loop>` argument to the `faust` program:\n\n```sh\nfaust -L eventlet -A myproj worker -l info\n```\n\nor add `import mode.loop.eventlet` at the top of your entry point script:\n\n```python\n#!/usr/bin/env python3\nimport mode.loop.eventlet # noqa\n```\n\nIt's very important this is at the very top of the module,\nand that it executes before you import libraries.\n\n### Can I use Faust with Tornado\n\nYes! Use the `tornado.platform.asyncio` [bridge](http://www.tornadoweb.org/en/stable/asyncio.html)\n\n### Can I use Faust with Twisted\n\nYes! Use the `asyncio` reactor implementation: https://twistedmatrix.com/documents/current/api/twisted.internet.asyncioreactor.html\n\n### Will you support Python 2.7 or Python 3.5\n\nNo. Faust requires Python 3.8 or later, since it heavily uses features that were\nintroduced in Python 3.6 (`async`, `await`, variable type annotations).\n\n### I get a maximum number of open files exceeded error by RocksDB when running a Faust app locally. How can I fix this\n\nYou may need to increase the limit for the maximum number of open files.\nOn macOS and Linux you can use:\n\n```ulimit -n max_open_files``` to increase the open files limit to max_open_files.\n\nOn docker, you can use the --ulimit flag:\n\n```docker run --ulimit nofile=50000:100000 <image-tag>```\nwhere 50000 is the soft limit, and 100000 is the hard limit [See the difference](https://unix.stackexchange.com/a/29579).\n\n### What kafka versions faust supports\n\nFaust supports kafka with version >= 0.10.\n\n## Getting Help\n\n### Slack\n\nFor discussions about the usage, development, and future of Faust, please join the `fauststream` Slack.\n\n- https://fauststream.slack.com\n- Sign-up: https://join.slack.com/t/fauststreaming/shared_invite/zt-1q1jhq4kh-Q1t~rJgpyuMQ6N38cByE9g\n\n## Resources\n\n### Bug tracker\n\nIf you have any suggestions, bug reports, or annoyances please report them\nto our issue tracker at https://github.com/faust-streaming/faust/issues/\n\n## License\n\nThis software is licensed under the `New BSD License`. See the `LICENSE` file in the top distribution directory for the full license text.\n\n### Contributing\n\nDevelopment of `Faust` happens at [GitHub](https://github.com/faust-streaming/faust)\n\nYou're highly encouraged to participate in the development of `Faust`.\n\n### Code of Conduct\n\nEveryone interacting in the project's code bases, issue trackers, chat rooms,\nand mailing lists is expected to follow the Faust Code of Conduct.\n\nAs contributors and maintainers of these projects, and in the interest of fostering\nan open and welcoming community, we pledge to respect all people who contribute\nthrough reporting issues, posting feature requests, updating documentation,\nsubmitting pull requests or patches, and other activities.\n\nWe are committed to making participation in these projects a harassment-free\nexperience for everyone, regardless of level of experience, gender,\ngender identity and expression, sexual orientation, disability,\npersonal appearance, body size, race, ethnicity, age,\nreligion, or nationality.\n\nExamples of unacceptable behavior by participants include:\n\n- The use of sexualized language or imagery\n- Personal attacks\n- Trolling or insulting/derogatory comments\n- Public or private harassment\n- Publishing other's private information, such as physical or electronic addresses, without explicit permission\n- Other unethical or unprofessional conduct.\n\nProject maintainers have the right and responsibility to remove, edit, or reject\ncomments, commits, code, wiki edits, issues, and other contributions that are\nnot aligned to this Code of Conduct. By adopting this Code of Conduct,\nproject maintainers commit themselves to fairly and consistently applying\nthese principles to every aspect of managing this project. Project maintainers\nwho do not follow or enforce the Code of Conduct may be permanently removed from\nthe project team.\n\nThis code of conduct applies both within project spaces and in public spaces\nwhen an individual is representing the project or its community.\n\nInstances of abusive, harassing, or otherwise unacceptable behavior may be\nreported by opening an issue or contacting one or more of the project maintainers.\n",
"bugtrack_url": null,
"license": "Copyright (c) 2017-2020, Robinhood Markets, Inc. All rights reserved. Faust is licensed under The BSD License (3 Clause, also known as the new BSD license). The license is an OSI approved Open Source license and is GPL-compatible(1). The license text can also be found here: http://www.opensource.org/licenses/BSD-3-Clause License ======= Redistribution and use in source and binary forms, with or without modification, are permitted provided that the following conditions are met: * Redistributions of source code must retain the above copyright notice, this list of conditions and the following disclaimer. * Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the following disclaimer in the documentation and/or other materials provided with the distribution. * Neither the name of Robinhood Markets, Inc. nor the names of its contributors may be used to endorse or promote products derived from this software without specific prior written permission. THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS \"AS IS\" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL Robinhood Markets, Inc. OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. Documentation License ===================== The documentation portion of Faust (the rendered contents of the \"docs\" directory of a software distribution or checkout) is supplied under the \"Creative Commons Attribution-ShareAlike 4.0 International\" (CC BY-SA 4.0) License as described by http://creativecommons.org/licenses/by-sa/4.0/ Footnotes ========= (1) A GPL-compatible license makes it possible to combine Faust with other software that is released under the GPL, it does not mean that we're distributing Faust under the GPL license. The BSD license, unlike the GPL, let you distribute a modified version without making your changes open source. ",
"summary": "Python Stream Processing. A Faust fork",
"version": "0.11.2",
"project_urls": {
"Changes": "https://github.com/faust-streaming/faust/releases",
"Documentation": "https://faust-streaming.github.io/faust/",
"Source": "https://github.com/faust-streaming/faust"
},
"split_keywords": [
"stream",
" processing",
" asyncio",
" distributed",
" queue",
" kafka"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "3fd3115147290a029ddd131777d5dd29c394e83c2c8b0cd207e8dae3b5d575fc",
"md5": "e0c207be0a0d7e5b062507bfe9bd5cac",
"sha256": "3e6414ddd489c0dbc847b5384735aec0ce3ec972a4a7b037e8683ae321fcfd0b"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp310-cp310-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "e0c207be0a0d7e5b062507bfe9bd5cac",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8.0",
"size": 517928,
"upload_time": "2024-08-08T17:52:31",
"upload_time_iso_8601": "2024-08-08T17:52:31.680865Z",
"url": "https://files.pythonhosted.org/packages/3f/d3/115147290a029ddd131777d5dd29c394e83c2c8b0cd207e8dae3b5d575fc/faust_streaming-0.11.2-cp310-cp310-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ec35604db5a13e4cba8e91d3b37bdf93fb90594212c718ebd8acf3f907af9540",
"md5": "fed032ca52a39311d3171405706a61b6",
"sha256": "f6cd7240091273bcec4a7f2ade86c44f85703375cf5bae13f10a23a7342326c4"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp310-cp310-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "fed032ca52a39311d3171405706a61b6",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8.0",
"size": 514762,
"upload_time": "2024-08-08T17:52:35",
"upload_time_iso_8601": "2024-08-08T17:52:35.098981Z",
"url": "https://files.pythonhosted.org/packages/ec/35/604db5a13e4cba8e91d3b37bdf93fb90594212c718ebd8acf3f907af9540/faust_streaming-0.11.2-cp310-cp310-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "532b4df002a8152741ac9eedf461b57ab1bb32e5c8f0ad38f4258b3349f6e036",
"md5": "8a0bd35da486cec0238a7c51525c5465",
"sha256": "516b2bb71391aa7861f455f4a86c9c65b9283d67cd2311fbc51bf0ed6f2410b0"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp310-cp310-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "8a0bd35da486cec0238a7c51525c5465",
"packagetype": "bdist_wheel",
"python_version": "cp310",
"requires_python": ">=3.8.0",
"size": 1160595,
"upload_time": "2024-08-08T17:52:37",
"upload_time_iso_8601": "2024-08-08T17:52:37.777831Z",
"url": "https://files.pythonhosted.org/packages/53/2b/4df002a8152741ac9eedf461b57ab1bb32e5c8f0ad38f4258b3349f6e036/faust_streaming-0.11.2-cp310-cp310-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6b6ef865957cb8423c4310f710a377cde251cc78263f1070ca297d5a9700517a",
"md5": "4296e4461f644bff01abbbc447ffac6d",
"sha256": "2d7ca4be1f4c2bc041f9372a5cd81e8e093f13af7e01dc4640a4c81360bbe17d"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp311-cp311-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "4296e4461f644bff01abbbc447ffac6d",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8.0",
"size": 518202,
"upload_time": "2024-08-08T17:52:40",
"upload_time_iso_8601": "2024-08-08T17:52:40.338808Z",
"url": "https://files.pythonhosted.org/packages/6b/6e/f865957cb8423c4310f710a377cde251cc78263f1070ca297d5a9700517a/faust_streaming-0.11.2-cp311-cp311-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "b0aaf0414070e88e17b3054b127bc161c69679cb55bb80cac3fb4b62407a891e",
"md5": "359e3d5530813efdb8207c9aef0b2c48",
"sha256": "6e66a7c0302c0d946dc8b60cf660efd5eb8ec3774e8185f0dd18ea8e260e9c5d"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp311-cp311-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "359e3d5530813efdb8207c9aef0b2c48",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8.0",
"size": 514779,
"upload_time": "2024-08-08T17:52:42",
"upload_time_iso_8601": "2024-08-08T17:52:42.827389Z",
"url": "https://files.pythonhosted.org/packages/b0/aa/f0414070e88e17b3054b127bc161c69679cb55bb80cac3fb4b62407a891e/faust_streaming-0.11.2-cp311-cp311-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "85e6c8596b989b7ec7d3b02218c6812c04a3e8064a970faf9d1cea5d9202206b",
"md5": "77ddbe128902894f4a691191b6c99466",
"sha256": "e7815721b912cf50aeee495702341439608f850e6e06a79111ce6d83b151ae53"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp311-cp311-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "77ddbe128902894f4a691191b6c99466",
"packagetype": "bdist_wheel",
"python_version": "cp311",
"requires_python": ">=3.8.0",
"size": 1220504,
"upload_time": "2024-08-08T17:52:45",
"upload_time_iso_8601": "2024-08-08T17:52:45.844452Z",
"url": "https://files.pythonhosted.org/packages/85/e6/c8596b989b7ec7d3b02218c6812c04a3e8064a970faf9d1cea5d9202206b/faust_streaming-0.11.2-cp311-cp311-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "dbed4461ed7215e8455af9be7df29746f7a67af196cd1d86fce8f52b4542705e",
"md5": "611709074258a2a8de66d2585a13f1cd",
"sha256": "d5eaea086f99cfda4aa6f91ef1ec3fe38af77beb266e787d0215b1e779ece90a"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp38-cp38-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "611709074258a2a8de66d2585a13f1cd",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8.0",
"size": 519926,
"upload_time": "2024-08-08T17:52:47",
"upload_time_iso_8601": "2024-08-08T17:52:47.782900Z",
"url": "https://files.pythonhosted.org/packages/db/ed/4461ed7215e8455af9be7df29746f7a67af196cd1d86fce8f52b4542705e/faust_streaming-0.11.2-cp38-cp38-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "44ebba51d0931344f59253464d8e5d5fef220a16d06254aadcaae65622f319e0",
"md5": "4d3eaa8db1f8b66fb06feb816dddce71",
"sha256": "07edd4861cb63915a19e0ba9174b748449437ff6b4f440b3eb56aabec9d07e3f"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp38-cp38-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "4d3eaa8db1f8b66fb06feb816dddce71",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8.0",
"size": 516541,
"upload_time": "2024-08-08T17:52:50",
"upload_time_iso_8601": "2024-08-08T17:52:50.540222Z",
"url": "https://files.pythonhosted.org/packages/44/eb/ba51d0931344f59253464d8e5d5fef220a16d06254aadcaae65622f319e0/faust_streaming-0.11.2-cp38-cp38-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "cb4ccfef05e21c6cf74ca34b6caa0a90c1e02312ac0e6bbf484c57f03dc8ac68",
"md5": "fa6006ab6395439b955ca8dda338be44",
"sha256": "0e1125dd2bab37b8600f22753ac9dacd046247fcc7eae25e49ecc57e9dfafe93"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp38-cp38-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "fa6006ab6395439b955ca8dda338be44",
"packagetype": "bdist_wheel",
"python_version": "cp38",
"requires_python": ">=3.8.0",
"size": 1205251,
"upload_time": "2024-08-08T17:52:53",
"upload_time_iso_8601": "2024-08-08T17:52:53.048225Z",
"url": "https://files.pythonhosted.org/packages/cb/4c/cfef05e21c6cf74ca34b6caa0a90c1e02312ac0e6bbf484c57f03dc8ac68/faust_streaming-0.11.2-cp38-cp38-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "31bbd31a1152ded1c27f80a70d0cfa47c110896c388889a59703db075ff1a648",
"md5": "34187e45c824d48ae4b824273241c49d",
"sha256": "d08f444a700e4989624ba98a077f112f27cb02aff8b04a36b423c373d099c61c"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp39-cp39-macosx_10_9_x86_64.whl",
"has_sig": false,
"md5_digest": "34187e45c824d48ae4b824273241c49d",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8.0",
"size": 518649,
"upload_time": "2024-08-08T17:52:55",
"upload_time_iso_8601": "2024-08-08T17:52:55.461856Z",
"url": "https://files.pythonhosted.org/packages/31/bb/d31a1152ded1c27f80a70d0cfa47c110896c388889a59703db075ff1a648/faust_streaming-0.11.2-cp39-cp39-macosx_10_9_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "7cd740e2ac0afec8b1bbd950334dd3d5948f9e66525532ffb0821df019efd08a",
"md5": "83f805df0ed0d9e2ef99cfe22d937bf6",
"sha256": "e65f0d3df8c090e024625ba52fafd668da76dc3186b9e68d8315e69e3d62f543"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp39-cp39-macosx_11_0_arm64.whl",
"has_sig": false,
"md5_digest": "83f805df0ed0d9e2ef99cfe22d937bf6",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8.0",
"size": 515510,
"upload_time": "2024-08-08T17:52:57",
"upload_time_iso_8601": "2024-08-08T17:52:57.602125Z",
"url": "https://files.pythonhosted.org/packages/7c/d7/40e2ac0afec8b1bbd950334dd3d5948f9e66525532ffb0821df019efd08a/faust_streaming-0.11.2-cp39-cp39-macosx_11_0_arm64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ddeb5e3dd907e58456cdb5a98a7a176e305f47fdd56f6e5fa48ca46ebb3dec48",
"md5": "9375e9d185fdff3852ce687d82296512",
"sha256": "133ac68be8034663fdef71eca0198cef2e60550240f576d6e67a96c60fbf22fb"
},
"downloads": -1,
"filename": "faust_streaming-0.11.2-cp39-cp39-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"has_sig": false,
"md5_digest": "9375e9d185fdff3852ce687d82296512",
"packagetype": "bdist_wheel",
"python_version": "cp39",
"requires_python": ">=3.8.0",
"size": 1164676,
"upload_time": "2024-08-08T17:52:59",
"upload_time_iso_8601": "2024-08-08T17:52:59.995874Z",
"url": "https://files.pythonhosted.org/packages/dd/eb/5e3dd907e58456cdb5a98a7a176e305f47fdd56f6e5fa48ca46ebb3dec48/faust_streaming-0.11.2-cp39-cp39-manylinux_2_5_x86_64.manylinux1_x86_64.manylinux_2_17_x86_64.manylinux2014_x86_64.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "901bcebacd8ff162487555e19d8337bb69d081da034d1fe842bf75752e97ad36",
"md5": "83b2aa2f2ff8de5930695b6df4778b6d",
"sha256": "b5ad07033ef91a31c271ae2b854034e40701b11e135dcb5136d3eae66330e017"
},
"downloads": -1,
"filename": "faust-streaming-0.11.2.tar.gz",
"has_sig": false,
"md5_digest": "83b2aa2f2ff8de5930695b6df4778b6d",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8.0",
"size": 759476,
"upload_time": "2024-08-08T17:53:02",
"upload_time_iso_8601": "2024-08-08T17:53:02.006905Z",
"url": "https://files.pythonhosted.org/packages/90/1b/cebacd8ff162487555e19d8337bb69d081da034d1fe842bf75752e97ad36/faust-streaming-0.11.2.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-08 17:53:02",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "faust-streaming",
"github_project": "faust",
"travis_ci": false,
"coveralls": true,
"github_actions": true,
"tox": true,
"lcname": "faust-streaming"
}