Name | fenicsx-beat JSON |
Version |
0.0.1
JSON |
| download |
home_page | None |
Summary | Library to run cardiac EP simulations |
upload_time | 2024-12-02 11:11:26 |
maintainer | None |
docs_url | None |
author | None |
requires_python | >=3.8 |
license | MIT |
keywords |
cardiac
electrophysiology
|
VCS |
 |
bugtrack_url |
|
requirements |
No requirements were recorded.
|
Travis-CI |
No Travis.
|
coveralls test coverage |
No coveralls.
|
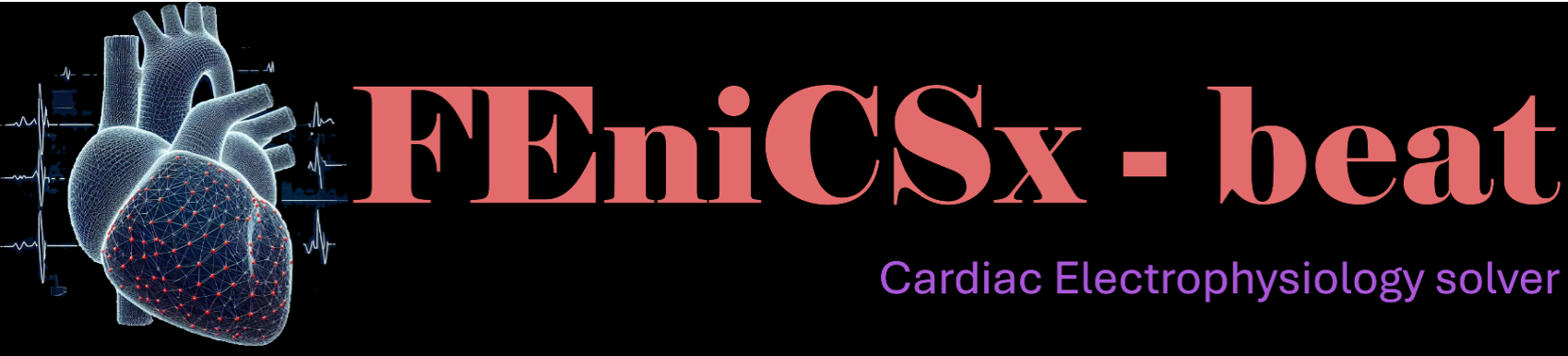
# fenicsx-beat
Cardiac electrophysiology simulator in FEniCSx
- Source code: https://github.com/finsberg/fenicsx-beat
- Documentation: https://finsberg.github.io/fenicsx-beat
## Install
You can install the library with pip
```
python3 -m pip install fenicsx-beat
```
## Getting started
```python
from mpi4py import MPI
import dolfinx
import ufl
import beat
comm = MPI.COMM_WORLD
mesh = dolfinx.mesh.create_unit_square(comm, 10, 10, dolfinx.cpp.mesh.CellType.triangle)
time = dolfinx.fem.Constant(mesh, dolfinx.default_scalar_type(0.0))
# Create stimulus
stim_expr = ufl.conditional(ufl.And(ufl.ge(time, 0.0), ufl.le(time, 0.5)),200.0, 0.0)
stim_marker = 1
cells = dolfinx.mesh.locate_entities(mesh, mesh.topology.dim, lambda x: np.logical_and(x[0] <= 0.5, x[1] <= 0.5))
stim_tags = dolfinx.mesh.meshtags(
mesh,
mesh.topology.dim,
cells,
np.full(len(cells), stim_marker, dtype=np.int32),
)
dx = ufl.Measure("dx", domain=mesh, subdomain_data=stim_tags)
I_s = beat.Stimulus(expr=stim_expr, dZ=dx, marker=stim_marker)
# Create PDE model
pde = beat.MonodomainModel(time=time, mesh=mesh, M=0.01, I_s=I_s, dx=dx)
# Define scheme to solve ODE
def fun(t, states, parameters, dt):
v, s = states
a, b = parameters
values = np.zeros_like(states)
values[0] = v - a * s * dt
values[1] = s + b * v * dt
return values
# Define ODE solver
ode_space = dolfinx.fem.functionspace(mesh, ("P", 1))
parameters = np.array([1.0, 1.0])
init_states = np.array([0.0, 0.0])
ode = beat.odesolver.DolfinODESolver(
v_ode=dolfinx.fem.Function(ode_space),
v_pde=pde.state,
fun=fun,
init_states=states,
parameters=parameters,
num_states=2,
v_index=1,
)
# Combine PDE and ODE solver
solver = beat.MonodomainSplittingSolver(pde=pde, ode=ode)
# Solve
T = 5.0
t = 0.0
i = 0
while t < T:
v = solver.pde.state.x.array
solver.step((t, t + dt))
i += 1
t += dt
```
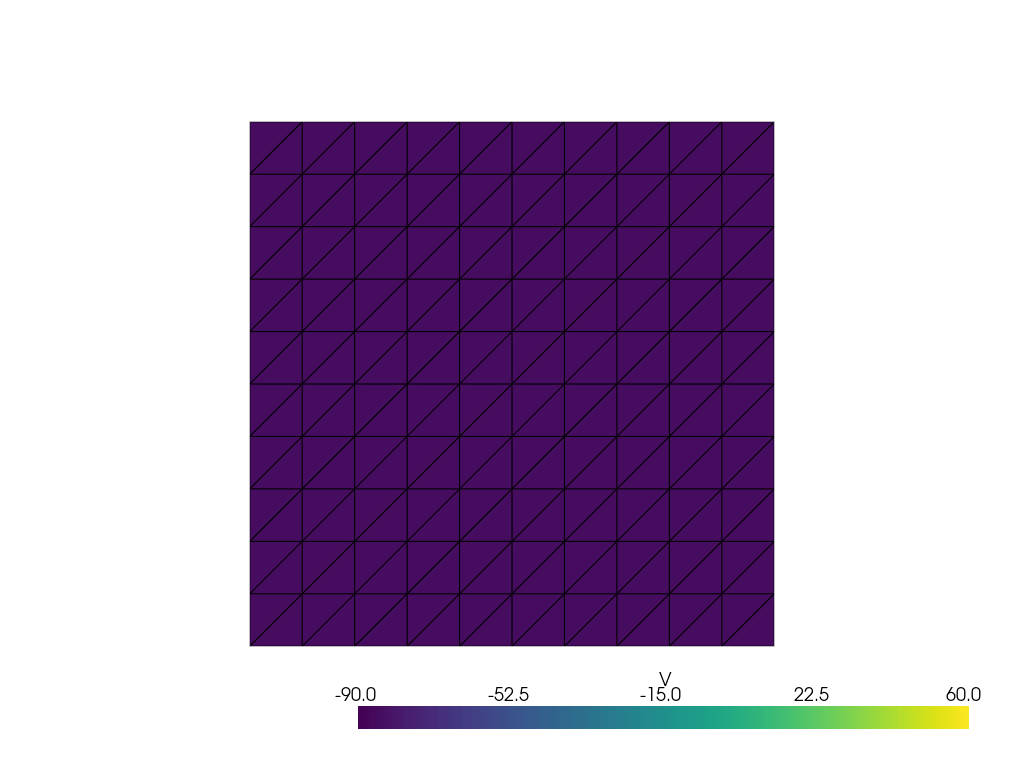
See more examples in the [documentation](https://finsberg.github.io/fenicsx-beat)
## License
MIT
## Need help or having issues
Please submit an [issue](https://github.com/finsberg/fenicsx-beat/issues)
Raw data
{
"_id": null,
"home_page": null,
"name": "fenicsx-beat",
"maintainer": null,
"docs_url": null,
"requires_python": ">=3.8",
"maintainer_email": null,
"keywords": "cardiac, electrophysiology",
"author": null,
"author_email": "Henrik Finsberg <henriknf@simula.no>",
"download_url": "https://files.pythonhosted.org/packages/6c/64/1e5beb66d4941540d29e84c6b0cd09d49beb76d18a10e4aea2c2e9b38de8/fenicsx_beat-0.0.1.tar.gz",
"platform": null,
"description": "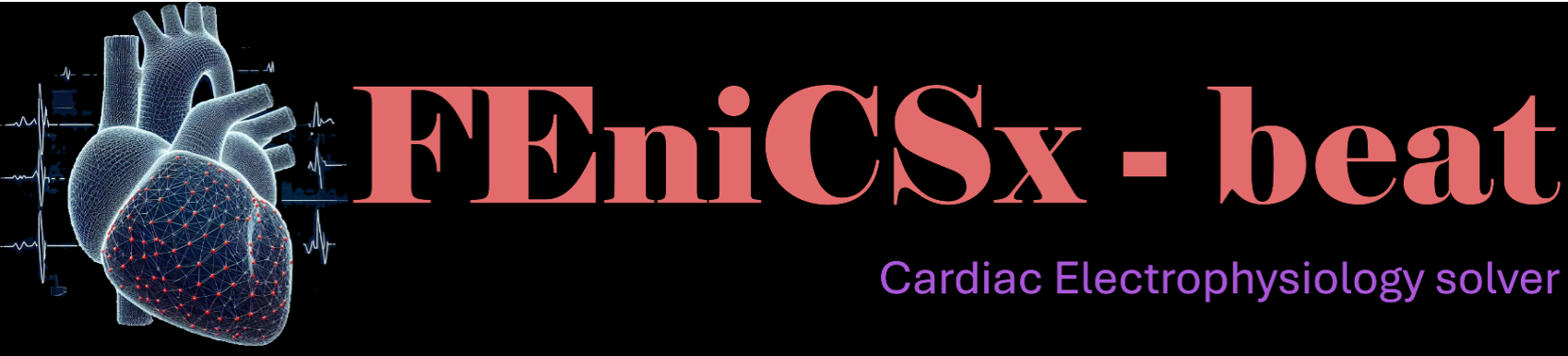\n\n# fenicsx-beat\nCardiac electrophysiology simulator in FEniCSx\n\n- Source code: https://github.com/finsberg/fenicsx-beat\n- Documentation: https://finsberg.github.io/fenicsx-beat\n\n\n## Install\nYou can install the library with pip\n```\npython3 -m pip install fenicsx-beat\n```\n\n\n## Getting started\n\n```python\nfrom mpi4py import MPI\nimport dolfinx\nimport ufl\nimport beat\n\ncomm = MPI.COMM_WORLD\nmesh = dolfinx.mesh.create_unit_square(comm, 10, 10, dolfinx.cpp.mesh.CellType.triangle)\ntime = dolfinx.fem.Constant(mesh, dolfinx.default_scalar_type(0.0))\n# Create stimulus\nstim_expr = ufl.conditional(ufl.And(ufl.ge(time, 0.0), ufl.le(time, 0.5)),200.0, 0.0)\nstim_marker = 1\ncells = dolfinx.mesh.locate_entities(mesh, mesh.topology.dim, lambda x: np.logical_and(x[0] <= 0.5, x[1] <= 0.5))\nstim_tags = dolfinx.mesh.meshtags(\n mesh,\n mesh.topology.dim,\n cells,\n np.full(len(cells), stim_marker, dtype=np.int32),\n)\ndx = ufl.Measure(\"dx\", domain=mesh, subdomain_data=stim_tags)\nI_s = beat.Stimulus(expr=stim_expr, dZ=dx, marker=stim_marker)\n# Create PDE model\npde = beat.MonodomainModel(time=time, mesh=mesh, M=0.01, I_s=I_s, dx=dx)\n# Define scheme to solve ODE\ndef fun(t, states, parameters, dt):\n v, s = states\n a, b = parameters\n values = np.zeros_like(states)\n values[0] = v - a * s * dt\n values[1] = s + b * v * dt\n return values\n# Define ODE solver\node_space = dolfinx.fem.functionspace(mesh, (\"P\", 1))\nparameters = np.array([1.0, 1.0])\ninit_states = np.array([0.0, 0.0])\node = beat.odesolver.DolfinODESolver(\n v_ode=dolfinx.fem.Function(ode_space),\n v_pde=pde.state,\n fun=fun,\n init_states=states,\n parameters=parameters,\n num_states=2,\n v_index=1,\n)\n# Combine PDE and ODE solver\nsolver = beat.MonodomainSplittingSolver(pde=pde, ode=ode)\n# Solve\nT = 5.0\nt = 0.0\ni = 0\nwhile t < T:\n v = solver.pde.state.x.array\n solver.step((t, t + dt))\n i += 1\n t += dt\n```\n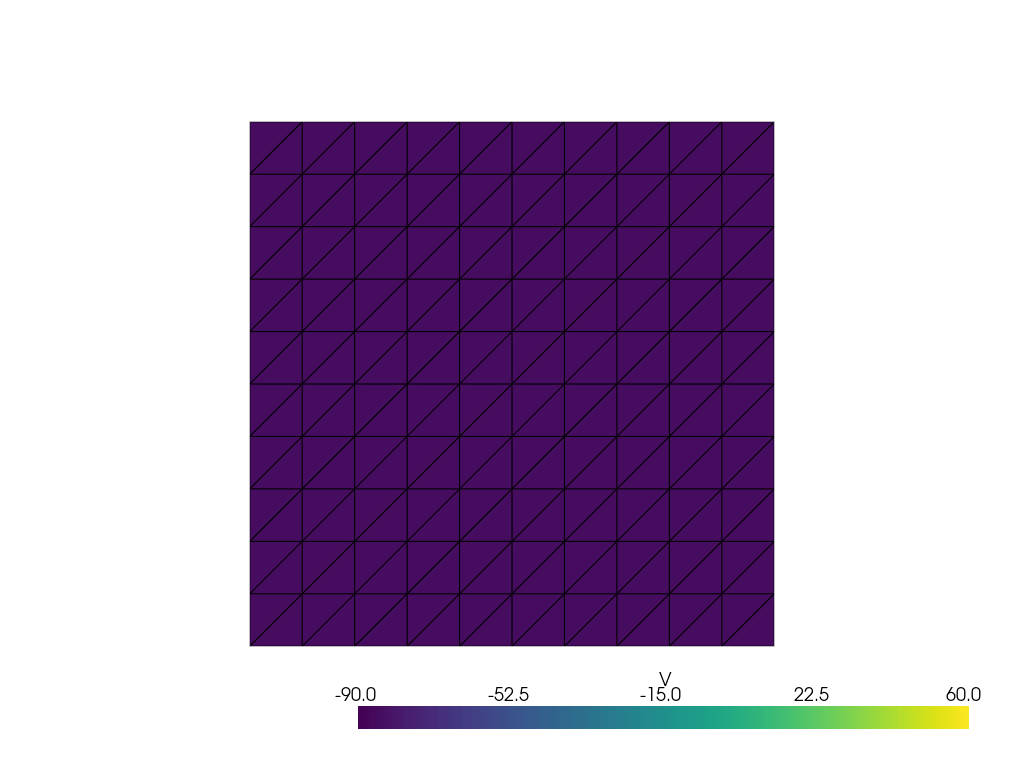\n\nSee more examples in the [documentation](https://finsberg.github.io/fenicsx-beat)\n\n## License\nMIT\n\n## Need help or having issues\nPlease submit an [issue](https://github.com/finsberg/fenicsx-beat/issues)\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Library to run cardiac EP simulations",
"version": "0.0.1",
"project_urls": {
"Documentation": "https://finsberg.github.io/fenicsx-beat",
"Homepage": "https://finsberg.github.io/fenicsx-beat",
"Source": "https://github.com/finsberg/fenicsx-beat",
"Tracker": "https://github.com/finsberg/fenicsx-beat/issues"
},
"split_keywords": [
"cardiac",
" electrophysiology"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "6b82a27900391518bec590623998f50ae43e51f48033e9af302cc126c301a502",
"md5": "e9ce47631bf985db7d7ece3e047922ff",
"sha256": "ed674948426bfaa07a1f04834ddd65b50eb572b174d1fdf0e01f37377e59a35a"
},
"downloads": -1,
"filename": "fenicsx_beat-0.0.1-py3-none-any.whl",
"has_sig": false,
"md5_digest": "e9ce47631bf985db7d7ece3e047922ff",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.8",
"size": 19765,
"upload_time": "2024-12-02T11:11:23",
"upload_time_iso_8601": "2024-12-02T11:11:23.894390Z",
"url": "https://files.pythonhosted.org/packages/6b/82/a27900391518bec590623998f50ae43e51f48033e9af302cc126c301a502/fenicsx_beat-0.0.1-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "6c641e5beb66d4941540d29e84c6b0cd09d49beb76d18a10e4aea2c2e9b38de8",
"md5": "baee0716203c4fef3bbbe3c489f963cc",
"sha256": "a2a0591bdfba10f5bdb7770716a42943868071667f4bb978cfb3bb763474d73e"
},
"downloads": -1,
"filename": "fenicsx_beat-0.0.1.tar.gz",
"has_sig": false,
"md5_digest": "baee0716203c4fef3bbbe3c489f963cc",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.8",
"size": 21731,
"upload_time": "2024-12-02T11:11:26",
"upload_time_iso_8601": "2024-12-02T11:11:26.062732Z",
"url": "https://files.pythonhosted.org/packages/6c/64/1e5beb66d4941540d29e84c6b0cd09d49beb76d18a10e4aea2c2e9b38de8/fenicsx_beat-0.0.1.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-12-02 11:11:26",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "finsberg",
"github_project": "fenicsx-beat",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "fenicsx-beat"
}