# Fritzing Stripboard Generator
Generates Fritzing components matching your particular stripboard.
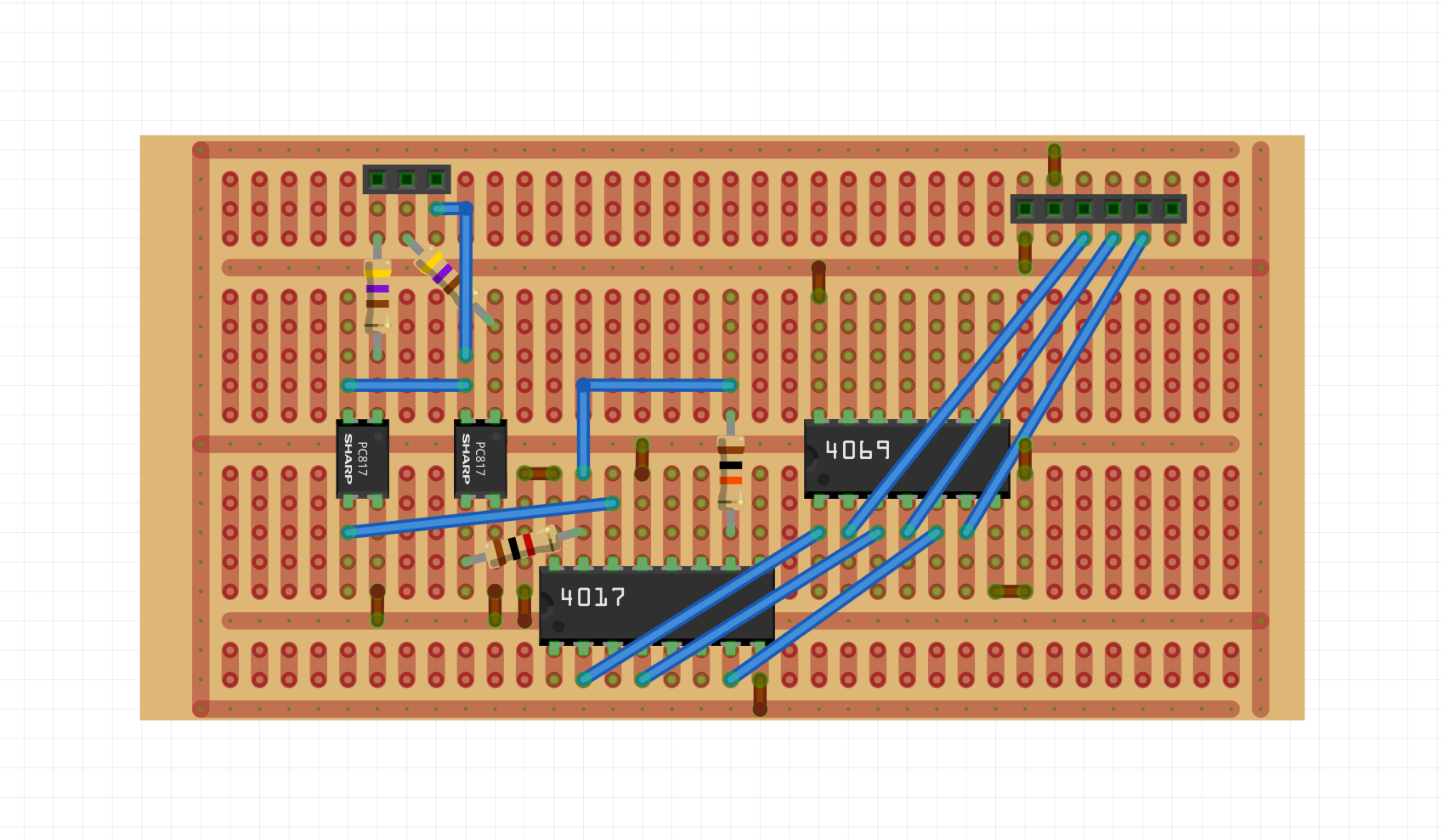
Inspired by Robert P Heller's project having a similar aim: https://github.com/RobertPHeller/fritzing-Stripboards
## Installation
```
pip install fritzing-stripboard
```
You can also install the in-development version with:
```
pip install https://github.com/coddingtonbear/fritzing-stripboard/archive/master.zip
```
## Use
```
fritzing-stripboard /path/to/board.yaml /path/to/output/part.fzpz
```
## Defining Your Board
While digging through my project supplies, I happened across a
large collection of stripboards that look like this:
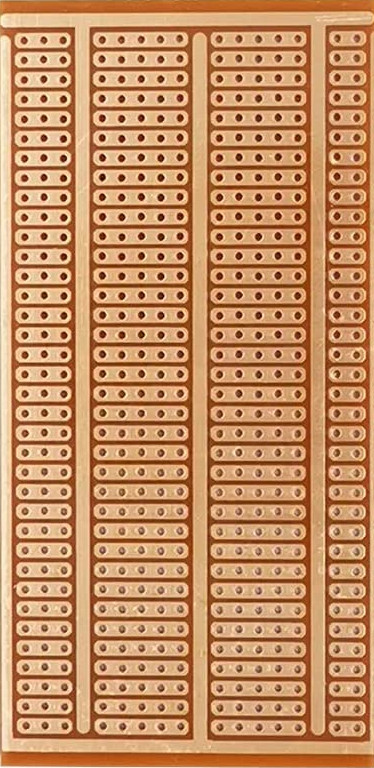
Although there are stripboards available online for Fritzing,
none quite looked like this. Luckily, though, generating a
Fritzing part for this board can be done easily with just a little
bit of yaml:
```yaml
meta:
title: 3-5-5-2 Board with Solid Bus
label: 3-5-5-2
width: 50.5
height: 100.5
board:
- grid:
components:
- shared_bus:
- bus: A1:T1
- bus: E1:E36
- bus: Q1:Q36
- shared_bus:
- bus: A37:T37
- bus: T2:T37
- bus: A2:A37
- bus: K2:K37
- drilled_rows: B2:D36
- drilled_rows: F2:J36
- drilled_rows: L2:P36
- drilled_rows: R2:S36
```
The above will generate a board that looks like:
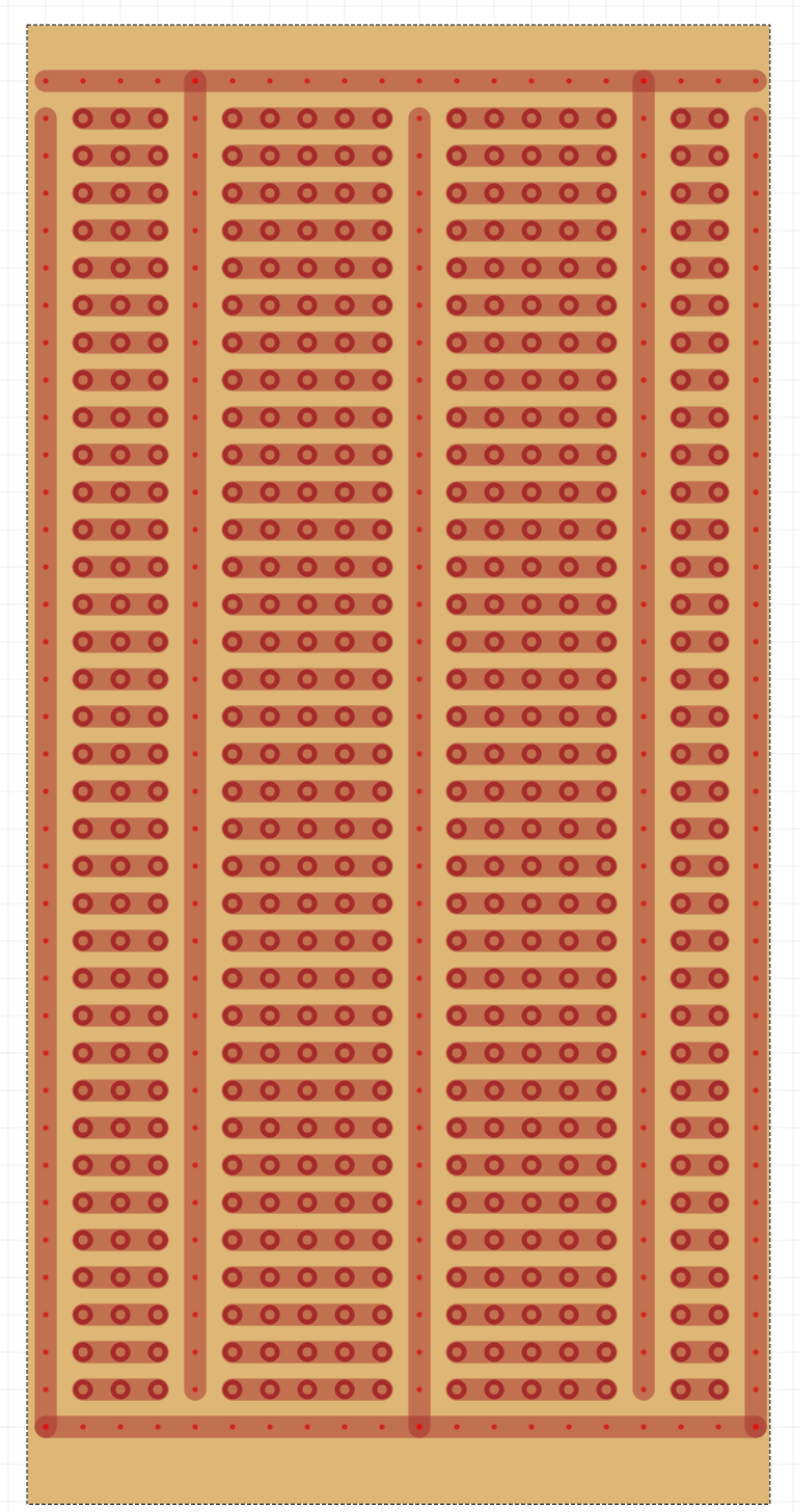
### The Grid
Definitions for boards use excel-like grid square ranges for defining
where new bus (trace) or drilled hole elements should appear:

### Components
#### `bus` or `drilled`
These create a line or a line of drilled holes. For example,
`bus` is used for creating this:
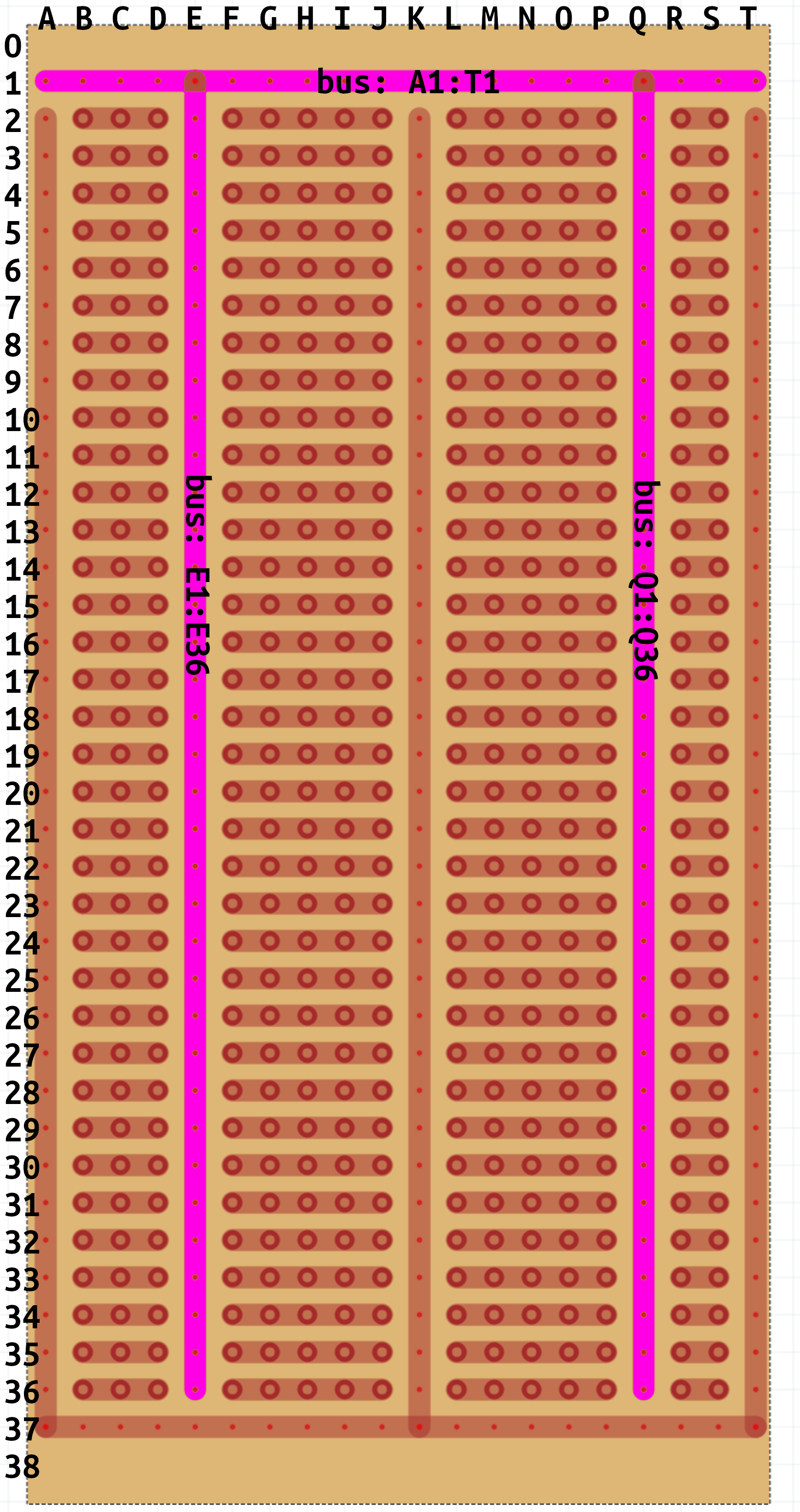
Each `bus` or `drilled` range will be assigned its own "bus"
(a.k.a. "net") unless wrapped by `shared_bus` as you've seen in the
example above.
#### `drilled_rows` or `drilled_columns`
These create an array of rows or columns. For example,
`drilled_rows` is used for creating this:
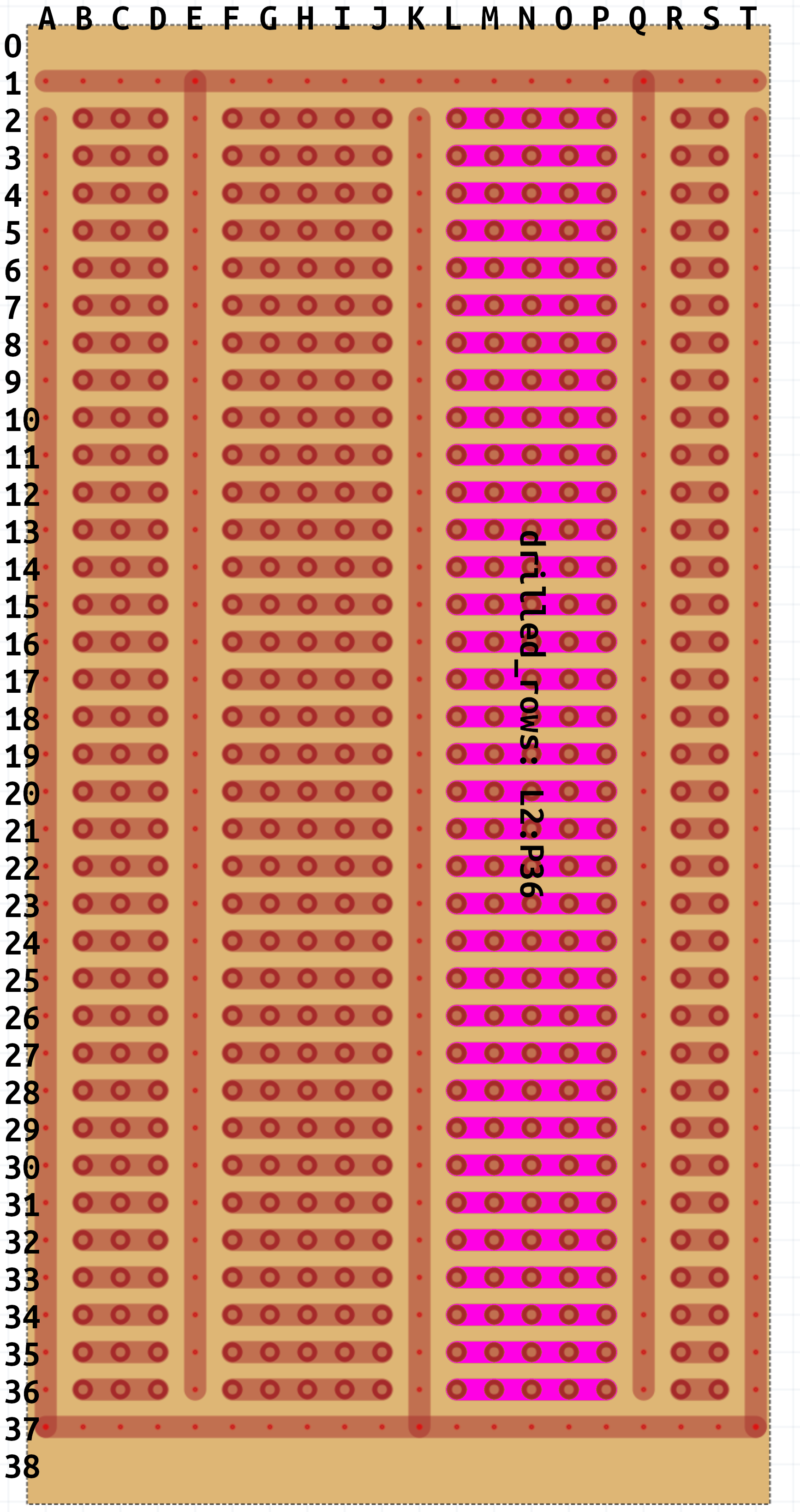
Each row or column of the range will be assigned its own "bus"
(a.k.a. "net") unless wrapped by `shared_bus`.
#### `shared_bus`
`bus` and `drilled` ranges by default each get their own bus. If your
stripboard has a more complex layout (like in the example), you can
use this 'component' for causing all components below it to share
the same bus.
### Metadata
See [BoardMetadata](https://github.com/coddingtonbear/fritzing-stripboard/blob/main/src/fritzing_stripboard/types.py#L12) for a full list of properties.
Raw data
{
"_id": null,
"home_page": "https://github.com/coddingtonbear/fritzing-stripboard",
"name": "fritzing-stripboard",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.6",
"maintainer_email": "",
"keywords": "",
"author": "Adam Coddington",
"author_email": "me@adamcoddington.net",
"download_url": "https://files.pythonhosted.org/packages/95/c3/ae34e0b39922fd7edb9f7d819b05e0cb1bb32ec315bf4f7afc8bcda0345a/fritzing-stripboard-1.0.0.tar.gz",
"platform": null,
"description": "# Fritzing Stripboard Generator\n\nGenerates Fritzing components matching your particular stripboard.\n\n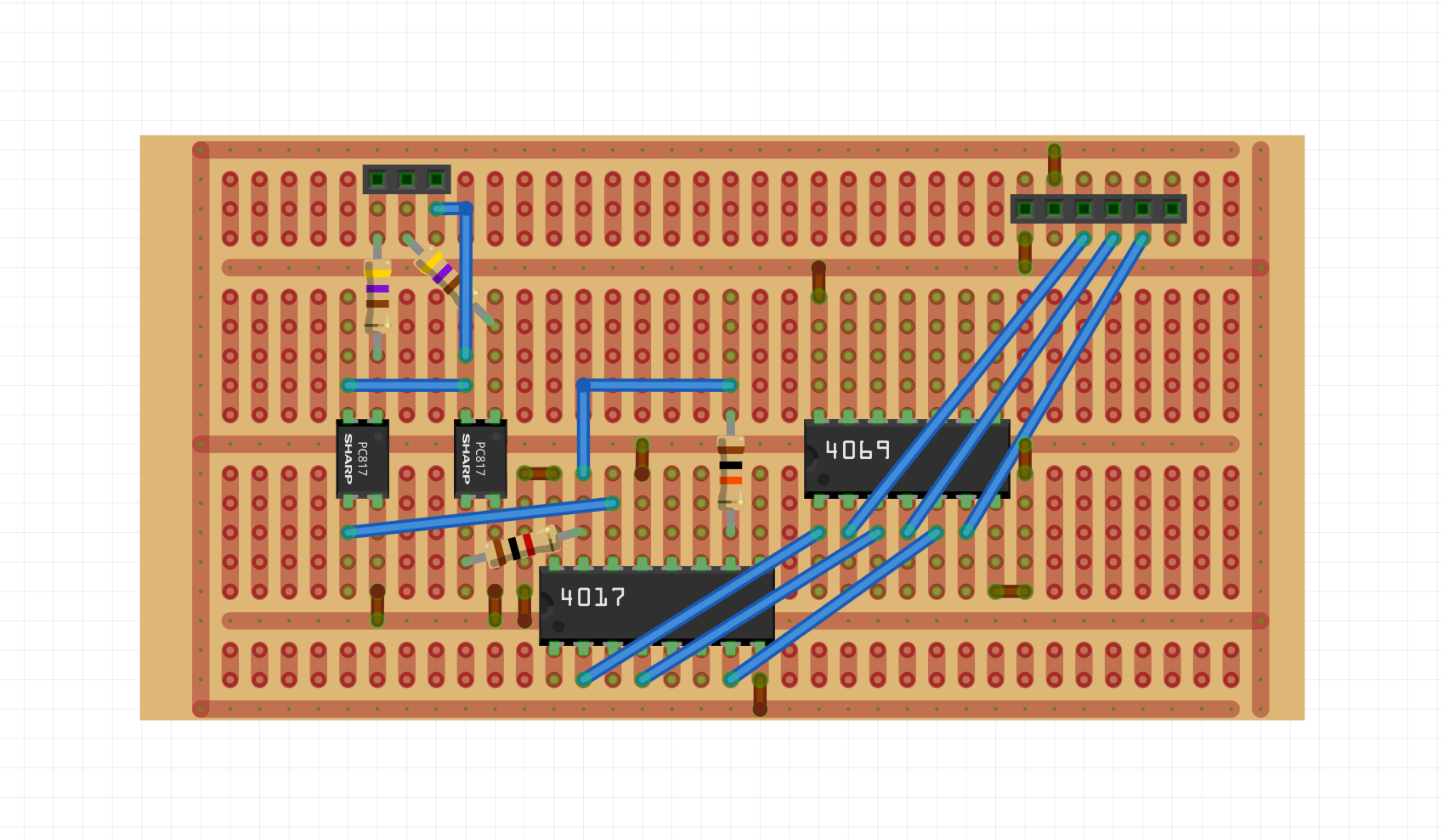\n\nInspired by Robert P Heller's project having a similar aim: https://github.com/RobertPHeller/fritzing-Stripboards\n\n## Installation\n\n```\npip install fritzing-stripboard\n```\n\nYou can also install the in-development version with:\n\n```\n\npip install https://github.com/coddingtonbear/fritzing-stripboard/archive/master.zip\n\n```\n\n## Use\n\n```\nfritzing-stripboard /path/to/board.yaml /path/to/output/part.fzpz\n```\n\n## Defining Your Board\n\nWhile digging through my project supplies, I happened across a\nlarge collection of stripboards that look like this:\n\n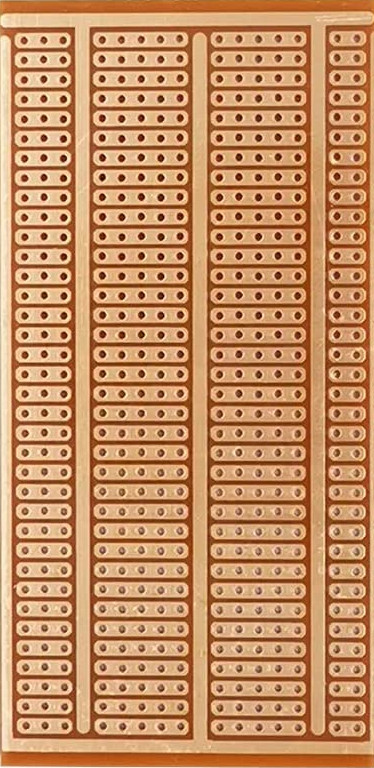\n\nAlthough there are stripboards available online for Fritzing,\nnone quite looked like this. Luckily, though, generating a \nFritzing part for this board can be done easily with just a little\nbit of yaml:\n\n\n```yaml\nmeta:\n title: 3-5-5-2 Board with Solid Bus\n label: 3-5-5-2\nwidth: 50.5\nheight: 100.5\nboard:\n - grid:\n components:\n - shared_bus:\n - bus: A1:T1\n - bus: E1:E36\n - bus: Q1:Q36\n - shared_bus:\n - bus: A37:T37\n - bus: T2:T37\n - bus: A2:A37\n - bus: K2:K37\n - drilled_rows: B2:D36\n - drilled_rows: F2:J36\n - drilled_rows: L2:P36\n - drilled_rows: R2:S36\n\n```\n\nThe above will generate a board that looks like:\n\n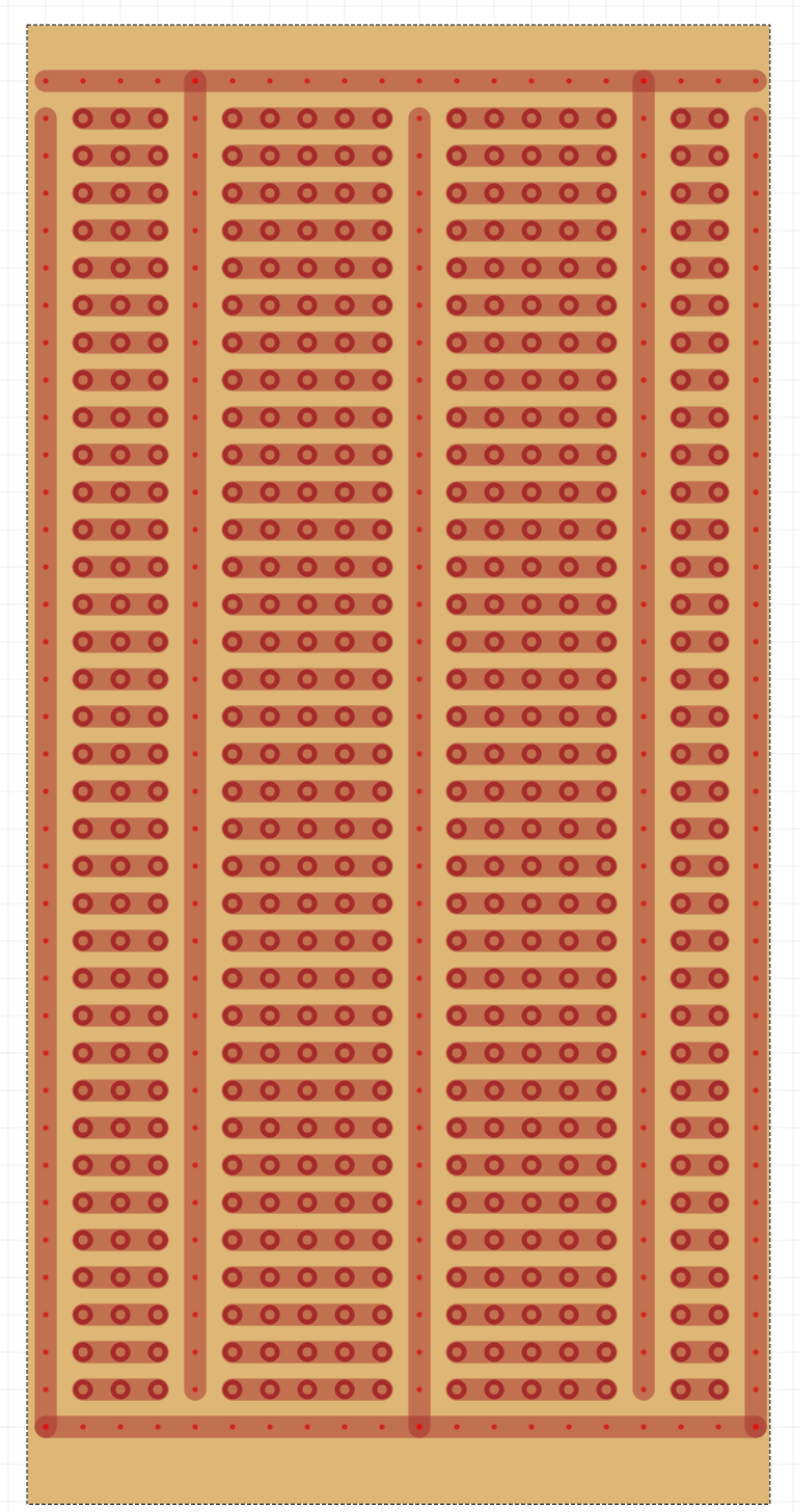\n\n### The Grid\n\nDefinitions for boards use excel-like grid square ranges for defining\nwhere new bus (trace) or drilled hole elements should appear:\n\n\n\n\n### Components\n\n#### `bus` or `drilled`\n\nThese create a line or a line of drilled holes. For example,\n`bus` is used for creating this:\n\n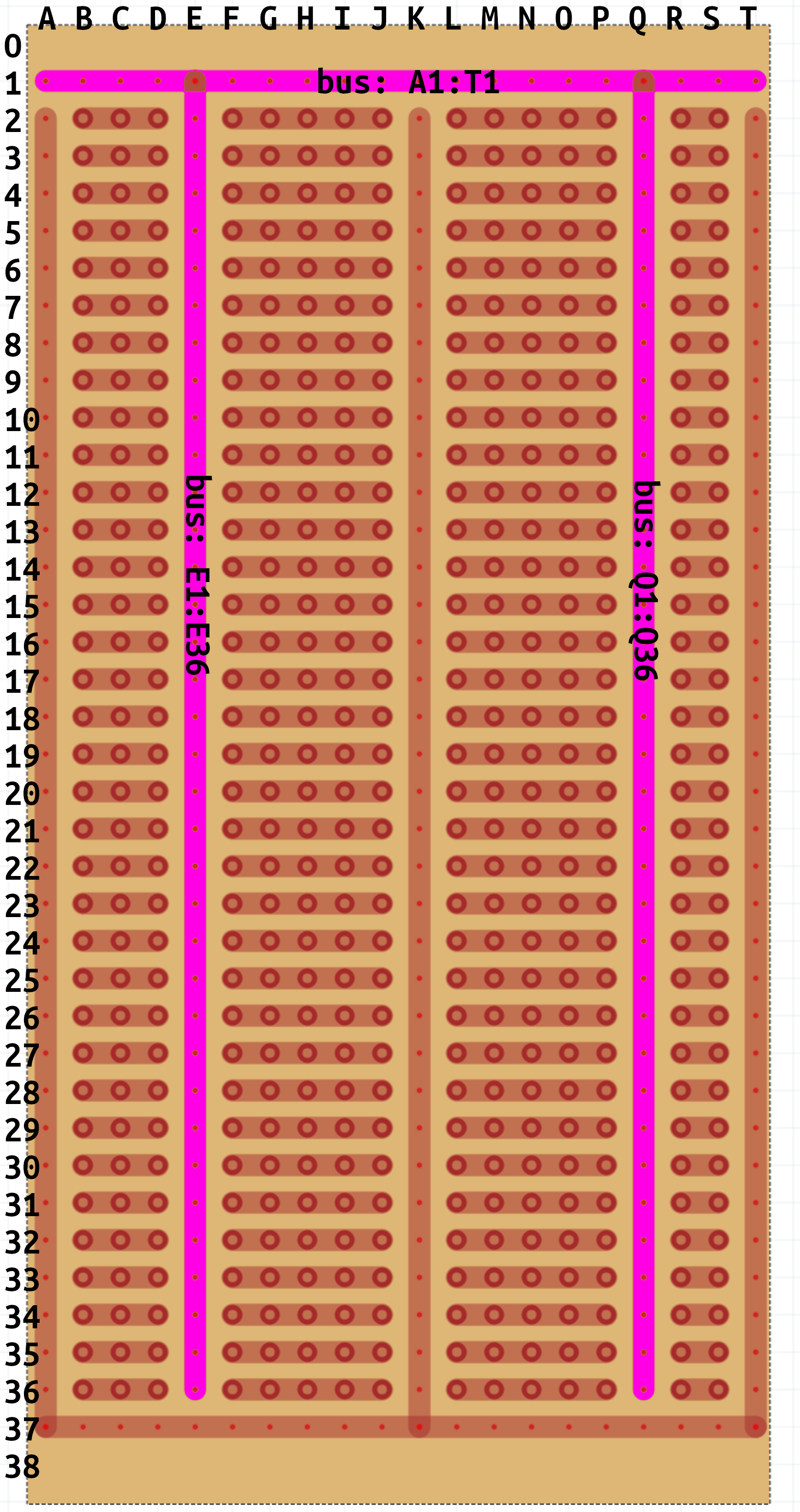\n\nEach `bus` or `drilled` range will be assigned its own \"bus\"\n(a.k.a. \"net\") unless wrapped by `shared_bus` as you've seen in the\nexample above.\n\n#### `drilled_rows` or `drilled_columns`\n\nThese create an array of rows or columns. For example, \n`drilled_rows` is used for creating this:\n\n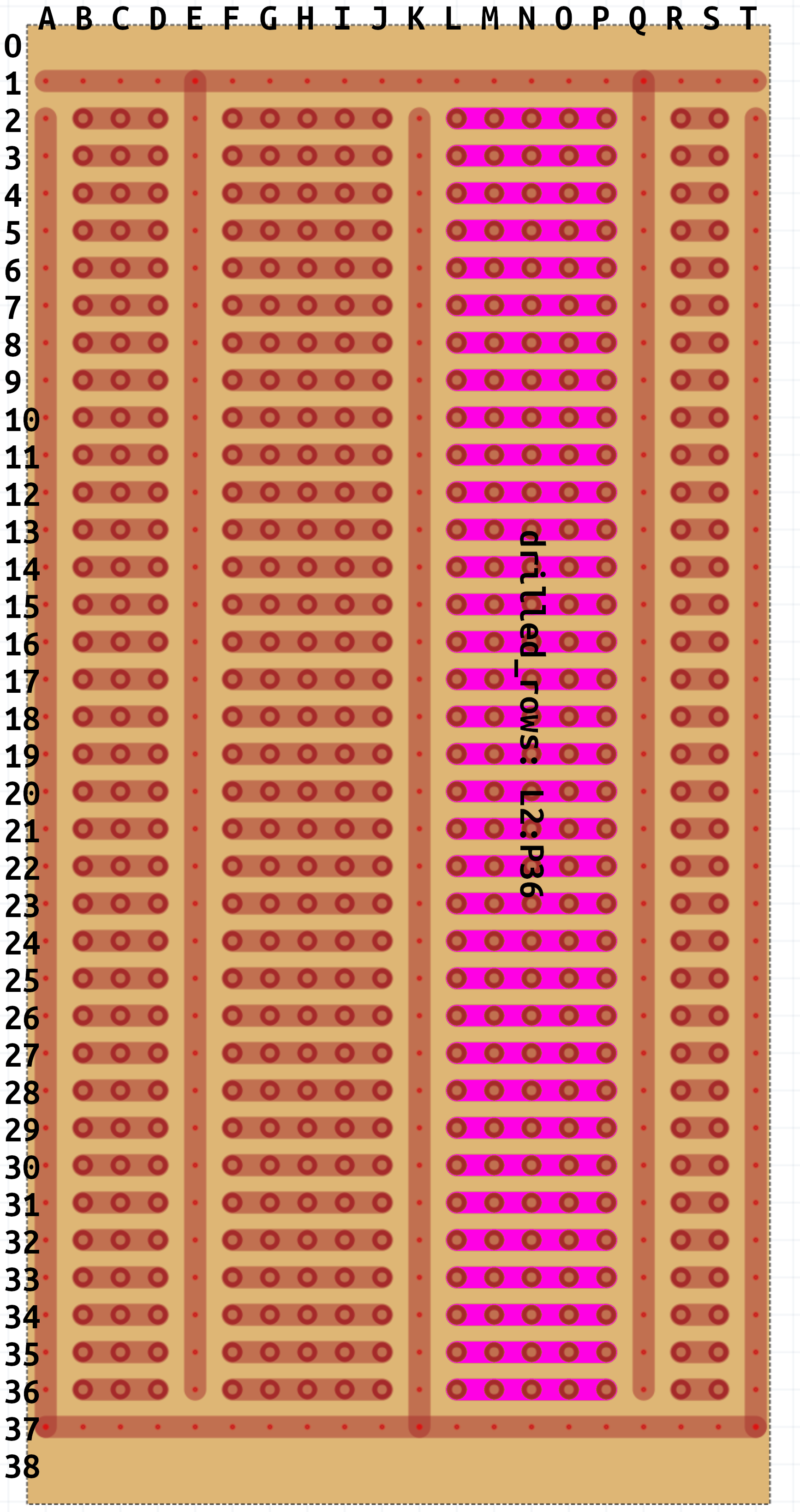\n\nEach row or column of the range will be assigned its own \"bus\"\n(a.k.a. \"net\") unless wrapped by `shared_bus`.\n\n#### `shared_bus`\n\n`bus` and `drilled` ranges by default each get their own bus. If your\nstripboard has a more complex layout (like in the example), you can\nuse this 'component' for causing all components below it to share\nthe same bus.\n\n### Metadata\n\nSee [BoardMetadata](https://github.com/coddingtonbear/fritzing-stripboard/blob/main/src/fritzing_stripboard/types.py#L12) for a full list of properties.\n\n\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "Generate Fritzing components that match your stripboard",
"version": "1.0.0",
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "956c6f4a88eee873da02ae4be9173ea6beb7dba210141039e760da7dc05e57b2",
"md5": "1fd44a19995488bea786fed3ad4bf4fb",
"sha256": "03fbd6030312128cec209b25939f8fe594fcd4434294d77dbfa20bf5d93a6091"
},
"downloads": -1,
"filename": "fritzing_stripboard-1.0.0-py2.py3-none-any.whl",
"has_sig": false,
"md5_digest": "1fd44a19995488bea786fed3ad4bf4fb",
"packagetype": "bdist_wheel",
"python_version": "py2.py3",
"requires_python": ">=3.6",
"size": 9377,
"upload_time": "2023-04-20T05:36:09",
"upload_time_iso_8601": "2023-04-20T05:36:09.483254Z",
"url": "https://files.pythonhosted.org/packages/95/6c/6f4a88eee873da02ae4be9173ea6beb7dba210141039e760da7dc05e57b2/fritzing_stripboard-1.0.0-py2.py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "95c3ae34e0b39922fd7edb9f7d819b05e0cb1bb32ec315bf4f7afc8bcda0345a",
"md5": "2b0183de800acbe28d3f21369d9abd13",
"sha256": "43ec5e2710ea2a3c50d2a969d783be80cb470e478a07b9dc1096944c2f167b6f"
},
"downloads": -1,
"filename": "fritzing-stripboard-1.0.0.tar.gz",
"has_sig": false,
"md5_digest": "2b0183de800acbe28d3f21369d9abd13",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.6",
"size": 66035,
"upload_time": "2023-04-20T05:36:11",
"upload_time_iso_8601": "2023-04-20T05:36:11.060968Z",
"url": "https://files.pythonhosted.org/packages/95/c3/ae34e0b39922fd7edb9f7d819b05e0cb1bb32ec315bf4f7afc8bcda0345a/fritzing-stripboard-1.0.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-04-20 05:36:11",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "coddingtonbear",
"github_project": "fritzing-stripboard",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "fritzing-stripboard"
}