# 🎷 FuncTown 🎷
[](https://pypi.python.org/pypi/functown)
[](https://pypistats.org/packages/functown)

[](https://pypi.org/project/functown/)
[](https://github.com/psf/black)
`FuncTown` is a python library that is designed to make your life with Azure Functions
easier.
The core features of `FuncTown` are:
* **Error handling** - automatically handle errors and return a response to the user
* **Debugging** - Set debug flags that automatically return logs and traces as part of
error responses from your function
* **JWT token validation** - automatically validate JWT tokens and provide the user
information
* **Request argument parsing** - automatically parse arguments from the `HttpRequest`
and provide them to your function
* **Metrics** - Handle connections to Application Insights and gives you easy to use
metrics objects
* **Logging, Tracing & Events** - Log your functions data directly into Application
Insights
For detailed features see the [docs](docs/overview.md).
## Getting Started
You can install `FuncTown` using `pip`:
```bash
pip install functown
```
> Note that some dependencies are hidden behind sub-packages (e.g. `functown[jwt]` for
> JWT token validation).
Almost all functionality of `FuncTown` is provided through decorators.
If you want to add error handling to your function:
```python
from logging import Logger
from functown import ErrorHandler
@ErrorHandler(debug=True, enable_logger=True)
def main(req: func.HttpRequest, logger: Logger, **kwargs) -> func.HttpResponse:
logger.info('Python HTTP trigger function processed a request.')
# ...
# exception will be caught and handled by the decorator (returning a 500)
raise ValueError("something went wrong")
return func.HttpResponse("success", status_code=200)
```
> Note: Decorators might pass down additional arguments to your function,
> so it is generally a good idea to modify your function signature to accept these
> arguments (see [docs](docs/overview.md) for more information) and add a `**kwargs`
> to the end.
Decorators are also stackable, so we could parse function arguments and handle a JWT
Token in the same function:
```python
from functown import ArgsHandler, RequestArgHandler, AuthHandler
from functown.auth import Token
@ArgsHandler()
@AuthHandler(scopes=["user.read"])
def main(
req: func.HttpRequest, args: RequestArgHandler, token: Token
) -> func.HttpResponse:
# retrieve some arguments
data = args.get_body_query("data_name", required=True, allowed=["foo", "bar"])
switch = args.get_body("bool_name", map_fct='bool')
file = args.get_file('file_name', required=True)
# check the user id
user_id = token.user_id
# ...
```
This would also directly fail with a `400` message if there is no token provided,
or the token does not contain the required scopes.
Be sure to check out the [docs](docs/overview.md) for a full overview of all
decorators.
If you want to test it on your own Azure Subscription, you can check out the
[example guide](docs/dev-guide.md#setting-up-the-function-app) in the dev section of the
docs.
🎷 Welcome to FuncTown! 🎷
## Note
‼️ If you find this library helpful or have suggestions please let me know.
Also any contributions are welcome! ‼️
[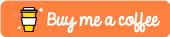](https://www.buymeacoffee.com/felixnext)
Raw data
{
"_id": null,
"home_page": "https://github.com/felixnext/python-functown",
"name": "functown",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "azure functions",
"author": "Felix Geilert",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/dd/eb/70907b77ee5b1a1938a2a501819e57dce55744868e48bca34d789fc65727/functown-2.2.0.tar.gz",
"platform": null,
"description": "# \ud83c\udfb7 FuncTown \ud83c\udfb7\n\n[](https://pypi.python.org/pypi/functown)\n[](https://pypistats.org/packages/functown)\n\n[](https://pypi.org/project/functown/)\n[](https://github.com/psf/black)\n\n`FuncTown` is a python library that is designed to make your life with Azure Functions\neasier.\n\nThe core features of `FuncTown` are:\n\n* **Error handling** - automatically handle errors and return a response to the user\n* **Debugging** - Set debug flags that automatically return logs and traces as part of\nerror responses from your function\n* **JWT token validation** - automatically validate JWT tokens and provide the user\ninformation\n* **Request argument parsing** - automatically parse arguments from the `HttpRequest`\nand provide them to your function\n* **Metrics** - Handle connections to Application Insights and gives you easy to use\nmetrics objects\n* **Logging, Tracing & Events** - Log your functions data directly into Application\nInsights\n\nFor detailed features see the [docs](docs/overview.md).\n\n## Getting Started\n\nYou can install `FuncTown` using `pip`:\n\n```bash\npip install functown\n```\n\n> Note that some dependencies are hidden behind sub-packages (e.g. `functown[jwt]` for\n> JWT token validation).\n\nAlmost all functionality of `FuncTown` is provided through decorators.\nIf you want to add error handling to your function:\n\n```python\nfrom logging import Logger\nfrom functown import ErrorHandler\n\n@ErrorHandler(debug=True, enable_logger=True)\ndef main(req: func.HttpRequest, logger: Logger, **kwargs) -> func.HttpResponse:\n logger.info('Python HTTP trigger function processed a request.')\n\n # ...\n # exception will be caught and handled by the decorator (returning a 500)\n raise ValueError(\"something went wrong\")\n\n return func.HttpResponse(\"success\", status_code=200)\n```\n\n> Note: Decorators might pass down additional arguments to your function,\n> so it is generally a good idea to modify your function signature to accept these\n> arguments (see [docs](docs/overview.md) for more information) and add a `**kwargs`\n> to the end.\n\nDecorators are also stackable, so we could parse function arguments and handle a JWT\nToken in the same function:\n\n```python\nfrom functown import ArgsHandler, RequestArgHandler, AuthHandler\nfrom functown.auth import Token\n\n@ArgsHandler()\n@AuthHandler(scopes=[\"user.read\"])\ndef main(\n req: func.HttpRequest, args: RequestArgHandler, token: Token\n) -> func.HttpResponse:\n # retrieve some arguments\n data = args.get_body_query(\"data_name\", required=True, allowed=[\"foo\", \"bar\"])\n switch = args.get_body(\"bool_name\", map_fct='bool')\n file = args.get_file('file_name', required=True)\n\n # check the user id\n user_id = token.user_id\n\n # ...\n```\n\nThis would also directly fail with a `400` message if there is no token provided,\nor the token does not contain the required scopes.\n\nBe sure to check out the [docs](docs/overview.md) for a full overview of all\ndecorators.\n\nIf you want to test it on your own Azure Subscription, you can check out the\n[example guide](docs/dev-guide.md#setting-up-the-function-app) in the dev section of the\ndocs.\n\n\ud83c\udfb7 Welcome to FuncTown! \ud83c\udfb7\n\n## Note\n\n\u203c\ufe0f If you find this library helpful or have suggestions please let me know.\nAlso any contributions are welcome! \u203c\ufe0f\n\n[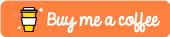](https://www.buymeacoffee.com/felixnext)\n",
"bugtrack_url": null,
"license": "MIT License",
"summary": "Various Helper Tools to make working with azure functions easier",
"version": "2.2.0",
"split_keywords": [
"azure",
"functions"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "a2ca6a70f22d437125e9dd745d916a135e7bc4e224cd80ed46326402c3247c5f",
"md5": "4aec22e82a32736a7510ed8ed062d362",
"sha256": "30d9f2bb93115536135e4dc6a48d95265f9944a74e91a3737c24fe514746bd15"
},
"downloads": -1,
"filename": "functown-2.2.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "4aec22e82a32736a7510ed8ed062d362",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 41495,
"upload_time": "2023-03-13T07:33:31",
"upload_time_iso_8601": "2023-03-13T07:33:31.017022Z",
"url": "https://files.pythonhosted.org/packages/a2/ca/6a70f22d437125e9dd745d916a135e7bc4e224cd80ed46326402c3247c5f/functown-2.2.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "ddeb70907b77ee5b1a1938a2a501819e57dce55744868e48bca34d789fc65727",
"md5": "cb86fb84ccbc980785c9aa4d37fca5e3",
"sha256": "919b205f24dbe24ae6acdd59c57ce5dab43f80a1349b7f2856d5fb5fdb929402"
},
"downloads": -1,
"filename": "functown-2.2.0.tar.gz",
"has_sig": false,
"md5_digest": "cb86fb84ccbc980785c9aa4d37fca5e3",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 30807,
"upload_time": "2023-03-13T07:33:32",
"upload_time_iso_8601": "2023-03-13T07:33:32.178690Z",
"url": "https://files.pythonhosted.org/packages/dd/eb/70907b77ee5b1a1938a2a501819e57dce55744868e48bca34d789fc65727/functown-2.2.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-03-13 07:33:32",
"github": true,
"gitlab": false,
"bitbucket": false,
"github_user": "felixnext",
"github_project": "python-functown",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"requirements": [],
"lcname": "functown"
}