# A pure Python module for recording and processing low-level Android input events (Mouse/Keyboard/Touchpad) - no dependencies
## pip install geteventplayback
### Tested against Windows 10 / Python 3.11 / Anaconda
GeteventPlayBack is a powerful Python module that simplifies the capture and
processing of input events from Android devices, including not only touch events but also
mouse and keyboard events. It is designed to work entirely with native Python, making it
a versatile choice for Android input event analysis.
### IMPORTANT: ONLY WORKS ON ROOTED DEVICES/EMULATORS
[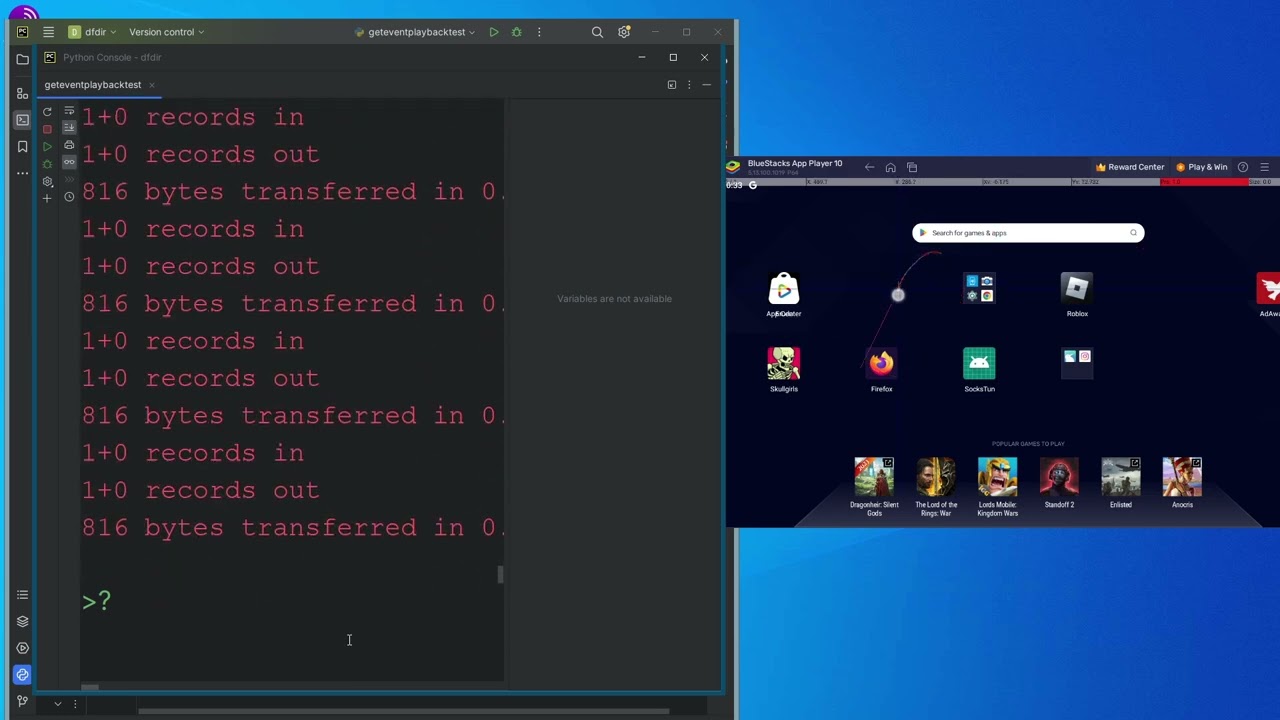](https://www.youtube.com/watch?v=LyGX7rQ-fLY)
[https://www.youtube.com/watch?v=LyGX7rQ-fLY]()
```python
"""
Args:
adb_path (str): The path to the 'adb' tool.
device (str): The device event file to record, e.g., "/dev/input/event3".
device_serial (str): The serial number or address of the Android device.
print_output (bool): Whether to print the 'getevent' output to the console.
tmpfolder_device (str): The temporary folder on the device to store event data.
tempfolder_hdd (str): The temporary folder on the local HDD to store event data.
add_closing_command (bool): Whether to add closing commands to the event replay.
clusterevents (int): The number of events to cluster together for replay, allowing
you to adjust playback speed.
Methods:
- start_recording(): Start recording input events, including mouse and keyboard events,
and customize the playback speed.
- _get_files_and_cmd(unpacked_data): Process the recorded data and generate
commands for event replay.
- _format_binary_data(): Format binary data from 'getevent' output into events.
Attributes:
- alldata (list): The raw 'getevent' output data, stored as bytes.
- FORMAT (str): The format string for parsing binary data.
- chunk_size (int): The size of each event in bytes.
- timestampnow (float): The current timestamp.
"""
import subprocess
from geteventplayback import GeteventPlayBack
pla = GeteventPlayBack(
adb_path=r"C:\Android\android-sdk\platform-tools\adb.exe",
device="/dev/input/event4",
device_serial="127.0.0.1:5555",
print_output=True,
tmpfolder_device="/sdcard/event4/",
tempfolder_hdd=rf"C:\sadxxxxxxxxxxxx",
add_closing_command=True,
clusterevents=16,
)
results = pla.start_recording()
subprocess.run(
[pla.adb_path, "-s", pla.device_serial, "shell"],
bufsize=0,
input=results["adbcommand"], # Can be resused
)
```
Raw data
{
"_id": null,
"home_page": "https://github.com/hansalemaos/geteventplayback",
"name": "geteventplayback",
"maintainer": "",
"docs_url": null,
"requires_python": "",
"maintainer_email": "",
"keywords": "Android,getevent,sendevent,record,playback",
"author": "Johannes Fischer",
"author_email": "aulasparticularesdealemaosp@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/1f/88/6d8b9a088e921a35438beb01e0ccf7c19c0efba1a69aaa3e398d7cb57ec0/geteventplayback-0.10.tar.gz",
"platform": null,
"description": "\r\n# A pure Python module for recording and processing low-level Android input events (Mouse/Keyboard/Touchpad) - no dependencies\r\n\r\n## pip install geteventplayback\r\n\r\n### Tested against Windows 10 / Python 3.11 / Anaconda \r\n\r\nGeteventPlayBack is a powerful Python module that simplifies the capture and\r\nprocessing of input events from Android devices, including not only touch events but also\r\nmouse and keyboard events. It is designed to work entirely with native Python, making it\r\na versatile choice for Android input event analysis. \r\n\r\n### IMPORTANT: ONLY WORKS ON ROOTED DEVICES/EMULATORS\r\n\r\n[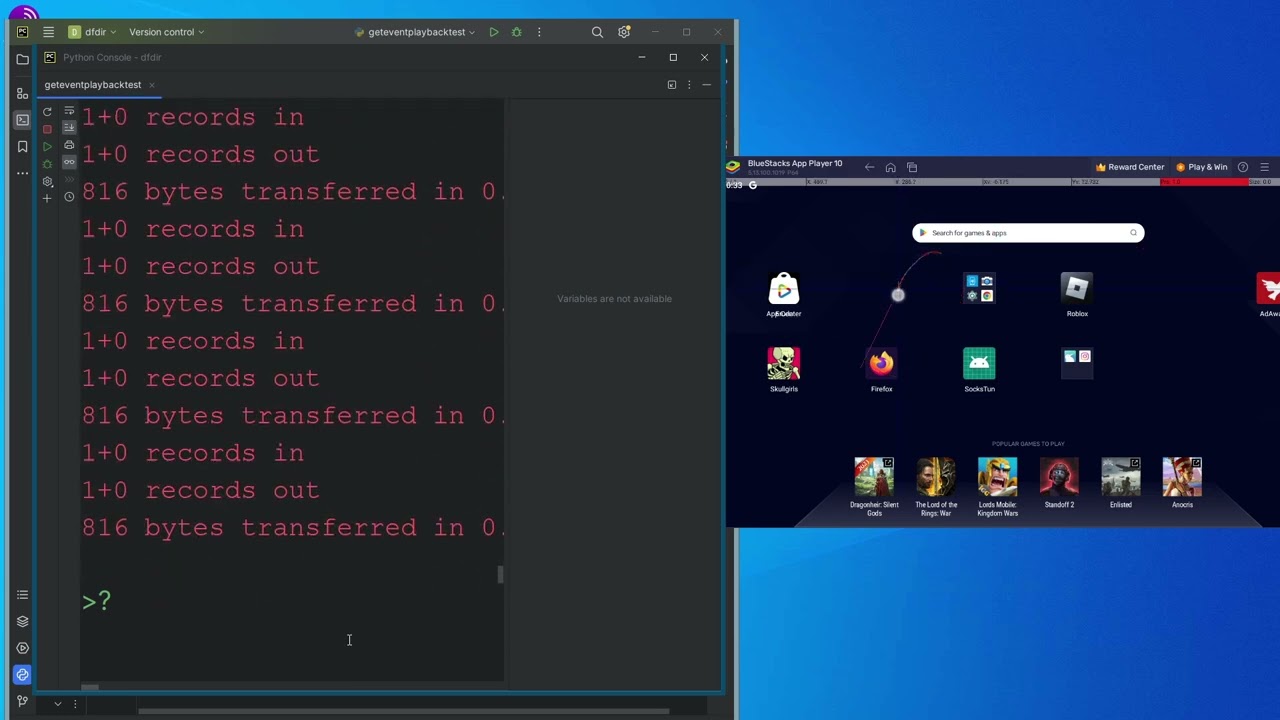](https://www.youtube.com/watch?v=LyGX7rQ-fLY)\r\n[https://www.youtube.com/watch?v=LyGX7rQ-fLY]()\r\n\r\n```python\r\n\r\n\"\"\"\r\nArgs:\r\n\tadb_path (str): The path to the 'adb' tool.\r\n\tdevice (str): The device event file to record, e.g., \"/dev/input/event3\".\r\n\tdevice_serial (str): The serial number or address of the Android device.\r\n\tprint_output (bool): Whether to print the 'getevent' output to the console.\r\n\ttmpfolder_device (str): The temporary folder on the device to store event data.\r\n\ttempfolder_hdd (str): The temporary folder on the local HDD to store event data.\r\n\tadd_closing_command (bool): Whether to add closing commands to the event replay.\r\n\tclusterevents (int): The number of events to cluster together for replay, allowing\r\n\tyou to adjust playback speed.\r\n\r\nMethods:\r\n\t- start_recording(): Start recording input events, including mouse and keyboard events,\r\n\t and customize the playback speed.\r\n\t- _get_files_and_cmd(unpacked_data): Process the recorded data and generate\r\n\t commands for event replay.\r\n\t- _format_binary_data(): Format binary data from 'getevent' output into events.\r\n\r\nAttributes:\r\n\t- alldata (list): The raw 'getevent' output data, stored as bytes.\r\n\t- FORMAT (str): The format string for parsing binary data.\r\n\t- chunk_size (int): The size of each event in bytes.\r\n\t- timestampnow (float): The current timestamp.\r\n\"\"\"\r\n\t\r\n\r\nimport subprocess\r\n\r\nfrom geteventplayback import GeteventPlayBack\r\n\r\npla = GeteventPlayBack(\r\n adb_path=r\"C:\\Android\\android-sdk\\platform-tools\\adb.exe\",\r\n device=\"/dev/input/event4\",\r\n device_serial=\"127.0.0.1:5555\",\r\n print_output=True,\r\n tmpfolder_device=\"/sdcard/event4/\",\r\n tempfolder_hdd=rf\"C:\\sadxxxxxxxxxxxx\",\r\n add_closing_command=True,\r\n clusterevents=16,\r\n)\r\n\r\nresults = pla.start_recording()\r\n\r\nsubprocess.run(\r\n [pla.adb_path, \"-s\", pla.device_serial, \"shell\"],\r\n bufsize=0,\r\n input=results[\"adbcommand\"], # Can be resused\r\n)\r\n\r\n```\r\n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A pure Python module for recording and processing low-level Android input events (Mouse/Keyboard/Touchpad) - no dependencies",
"version": "0.10",
"project_urls": {
"Homepage": "https://github.com/hansalemaos/geteventplayback"
},
"split_keywords": [
"android",
"getevent",
"sendevent",
"record",
"playback"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "f3c025712d00e3d3d817e717c601382503cfe839a2a3ed0bfc4c46cc079a92b7",
"md5": "19d950ea736875245a0c4b7b5c375171",
"sha256": "27363bc40b25f564645d864a2386690800927fb6abeccfb70d2758eae786a4f7"
},
"downloads": -1,
"filename": "geteventplayback-0.10-py3-none-any.whl",
"has_sig": false,
"md5_digest": "19d950ea736875245a0c4b7b5c375171",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": null,
"size": 8347,
"upload_time": "2023-10-30T13:50:49",
"upload_time_iso_8601": "2023-10-30T13:50:49.619128Z",
"url": "https://files.pythonhosted.org/packages/f3/c0/25712d00e3d3d817e717c601382503cfe839a2a3ed0bfc4c46cc079a92b7/geteventplayback-0.10-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1f886d8b9a088e921a35438beb01e0ccf7c19c0efba1a69aaa3e398d7cb57ec0",
"md5": "5322dfd93d8f9b7b755dd73d12e56b57",
"sha256": "b74d977e9860605348b5caba85ec44000756fba3a28d7d4aa326ee658287dbdb"
},
"downloads": -1,
"filename": "geteventplayback-0.10.tar.gz",
"has_sig": false,
"md5_digest": "5322dfd93d8f9b7b755dd73d12e56b57",
"packagetype": "sdist",
"python_version": "source",
"requires_python": null,
"size": 6665,
"upload_time": "2023-10-30T13:50:51",
"upload_time_iso_8601": "2023-10-30T13:50:51.422269Z",
"url": "https://files.pythonhosted.org/packages/1f/88/6d8b9a088e921a35438beb01e0ccf7c19c0efba1a69aaa3e398d7cb57ec0/geteventplayback-0.10.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-10-30 13:50:51",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "hansalemaos",
"github_project": "geteventplayback",
"travis_ci": false,
"coveralls": false,
"github_actions": false,
"requirements": [],
"lcname": "geteventplayback"
}