[](https://pypi.org/project/google-calendar-analytics/)
[](https://pypi.python.org/pypi/google-calendar-analytics)
[](https://pypi.org/project/google-calendar-analytics/)
[](https://pypi.python.org/pypi/google-calendar-analytics)
# Google Calendar Analytics
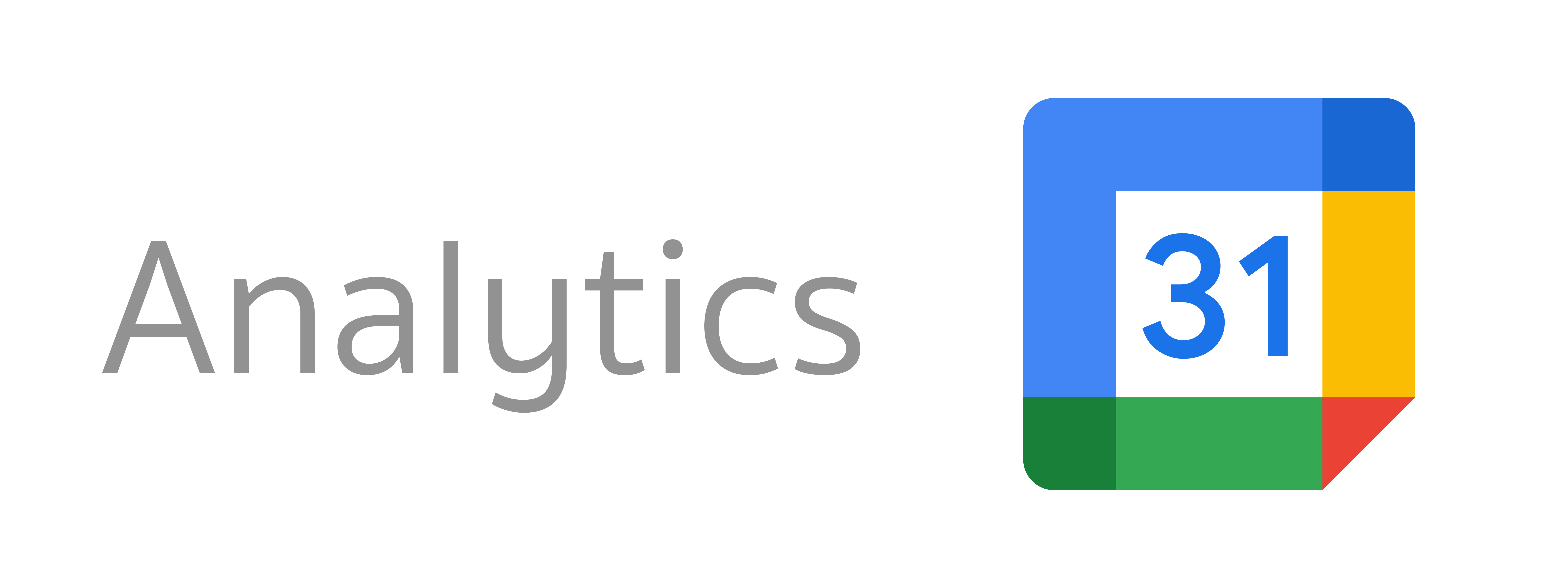
---
- ### [Documentation](https://berupor.github.io/Calendar-Analytics/)
- ### [Source code](https://github.com/Berupor/Calendar-Analytics)
---
This Python program allows you to perform analytics on your Google Calendar events. With this program, you can visualize
the total duration of your events, compare the length of events across different time periods, and gain insights into
which events take up the most time.
## Features
- Async support for faster data retrieval and chart generation
- Extract events from your Google Calendar
- Compute the total duration of events in a specified time range
- Visualize the duration of events in a pie chart, bar chart, line chart and more
- Limit the number of events displayed in the charts
- Wide chart customization. For example, dark mode and transparent background
## Quick Start
To use the Google Calendar Analytics program, first install the dependencies by running the following command:
```bash
pip install google-calendar-analytics
```
You can then import the AnalyzerFacade class and use it to analyze your data:
### How to get credentials from Google?
1. [Google documentation](https://developers.google.com/calendar/api/quickstart/python)
```python
import asyncio
from datetime import datetime
from google.oauth2.credentials import Credentials
from google_calendar_analytics import AnalyzerFacade
# (You can get it from Google OAuth2 in you web app or from link above)
creds = {
"token": "ya29.a0AVvZVsoH4qZcrGK25VwsXspJv-r9K-",
"refresh_token": "1//0hwlhrtalKgeRCgYIARAAGBESNwF-",
"token_uri": "https://oauth2.googleapis.com/token",
"client_id": "395np.apps.googleusercontent.com",
"client_secret": "GOCSPXFqoucE03VRVz",
"scopes": ["https://www.googleapis.com/auth/calendar"],
"expiry": "2023-02-18T15:30:15.674219Z"
}
creds = Credentials.from_authorized_user_info(creds)
```
Once you have created the credentials, you can create an instance of the AnalyzerFacade class and use it to analyze your data:
```python
# Choose time range for analysis
start_time = datetime(2023, 3, 1)
end_time = datetime(2023, 3, 18)
async def main():
async with AnalyzerFacade(creds=creds) as analyzer:
plot = await analyzer.analyze_one(start_time, end_time, event_name="Programming", plot_type="Line")
plot.show()
if __name__ == "__main__":
asyncio.run(main())
```
What's about multiple plots?
```python
async def main():
async with AnalyzerFacade(creds=creds) as analyzer:
coroutines = []
coroutines.append(analyzer.analyze_one(start_time, end_time, event_name="Programming", plot_type="Line")
coroutines.append(analyzer.analyze_one(start_time, end_time, event_name="Reading", plot_type="Line"))
coroutines.append(analyzer.analyze_many(start_time, end_time, plot_type="Pie"))
coroutines.append(analyzer.analyze_many(start_time, end_time, plot_type="Bar"))
result = await asyncio.gather(*coroutines)
for plot in result:
plot.show()
```
## Contribution
If you would like to contribute to this project, please feel free to submit a pull request. Some areas where
contributions are particularly welcome include:
- Adding new features
- Improving existing features
- Debugging and fixing bugs
- Adding tests to ensure the program is working as expected
## Analytics example:
| Pie plot | Bar plot |
|:-----------------------------------:|:-----------------------------------:|
| 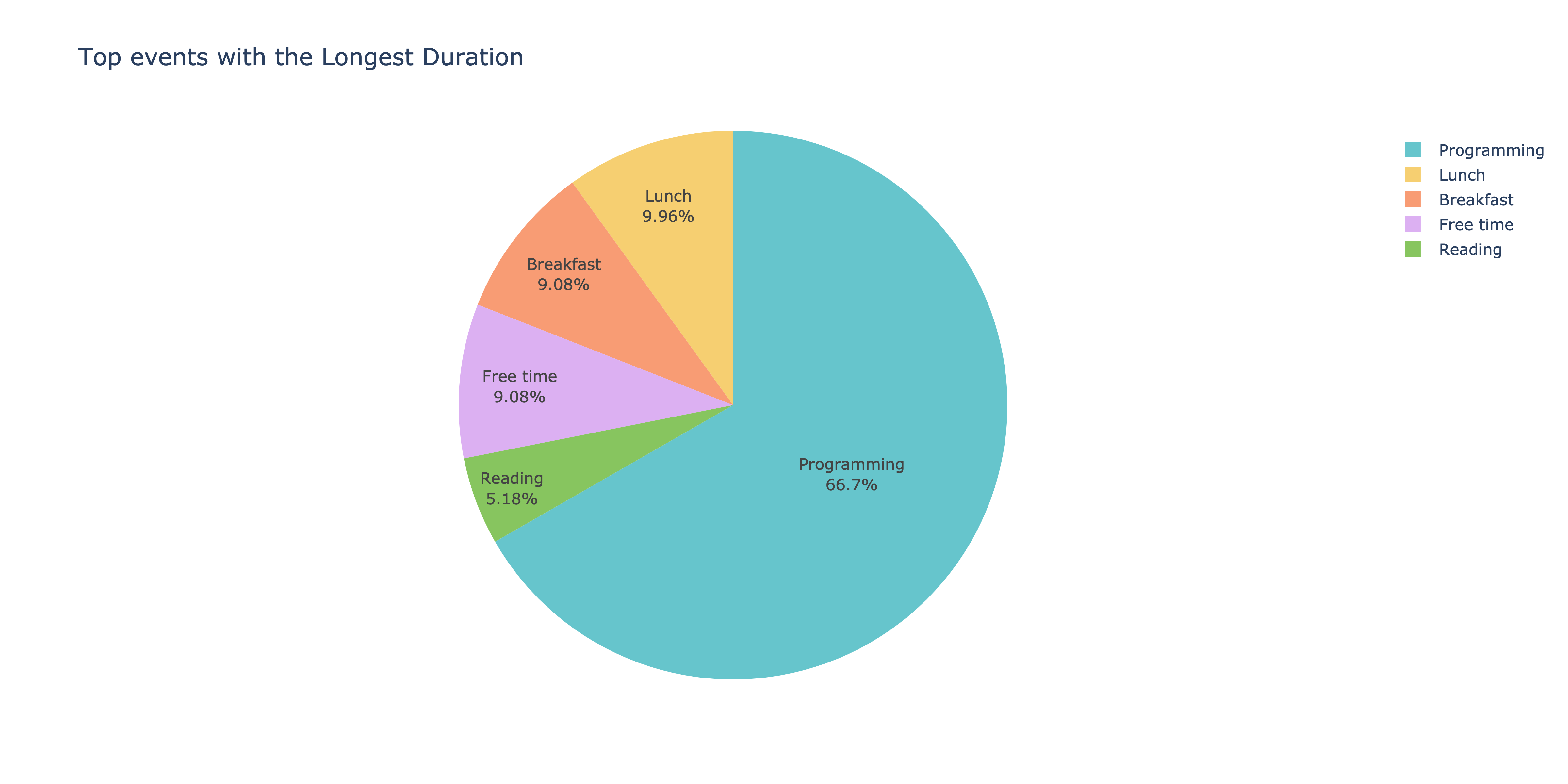 | 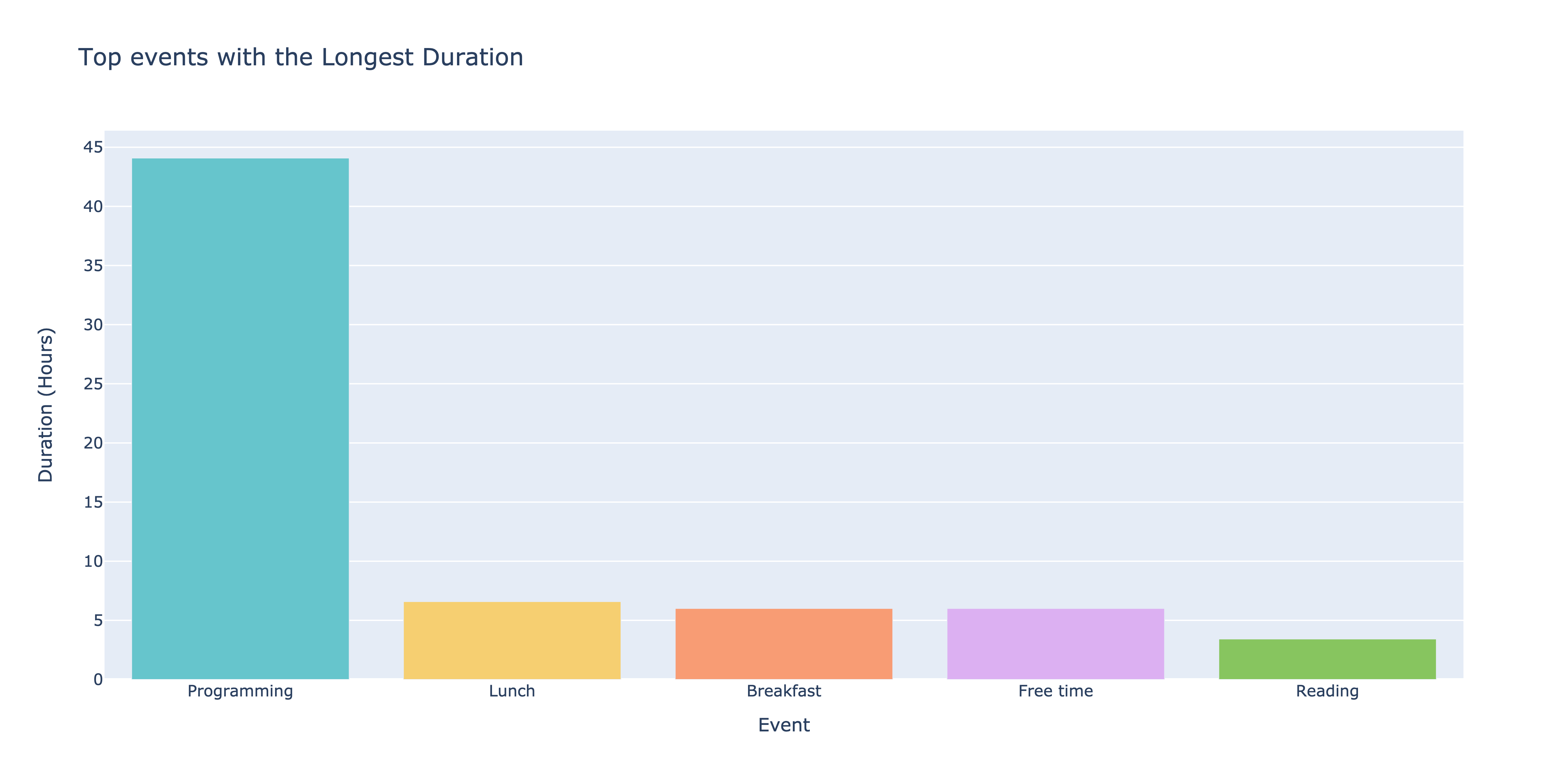 |
| Line plot | Multy line plot |
|:------------------------------------:|:--------------------------------------------------------------------------------------------------:|
| 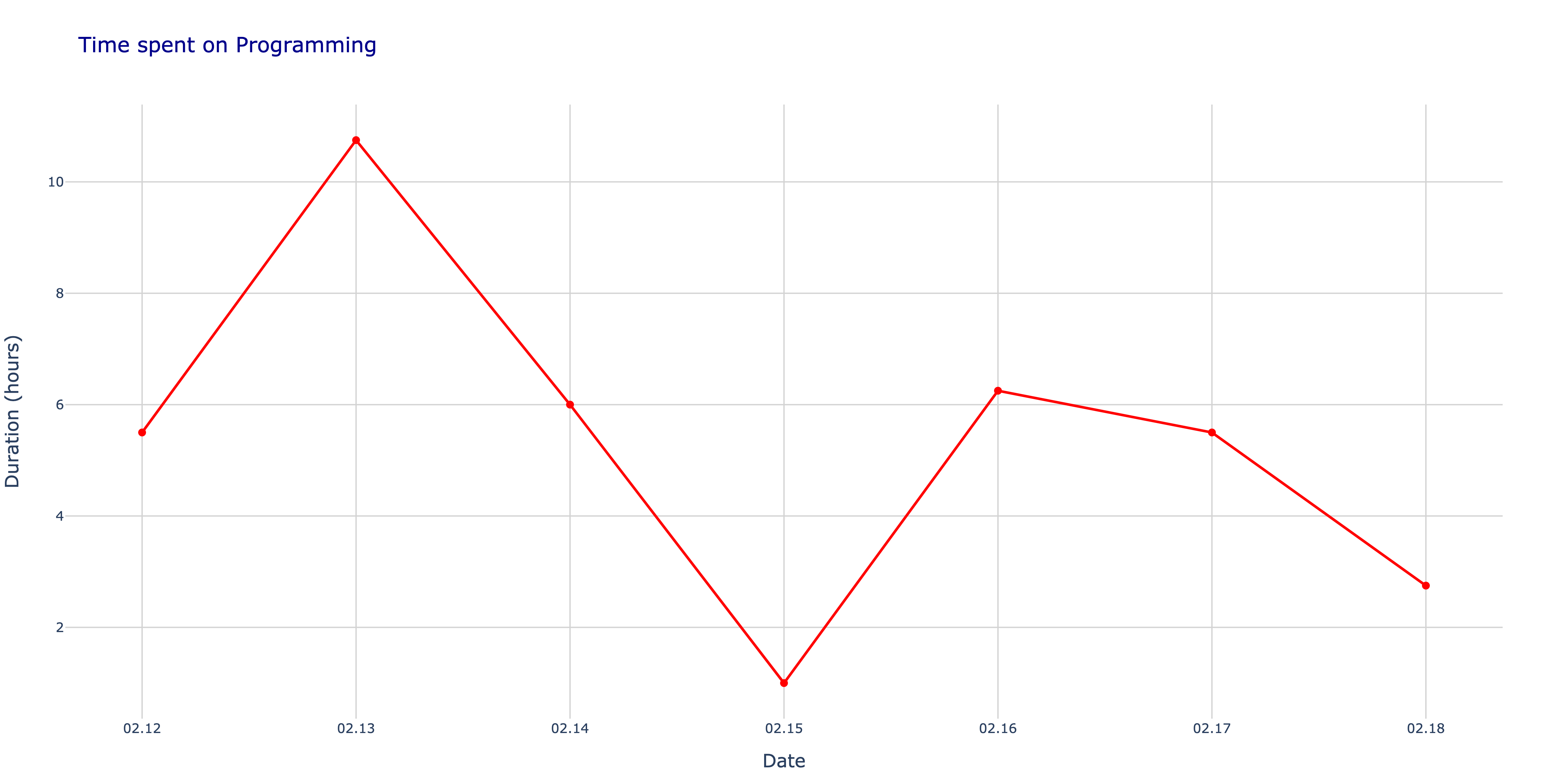 | 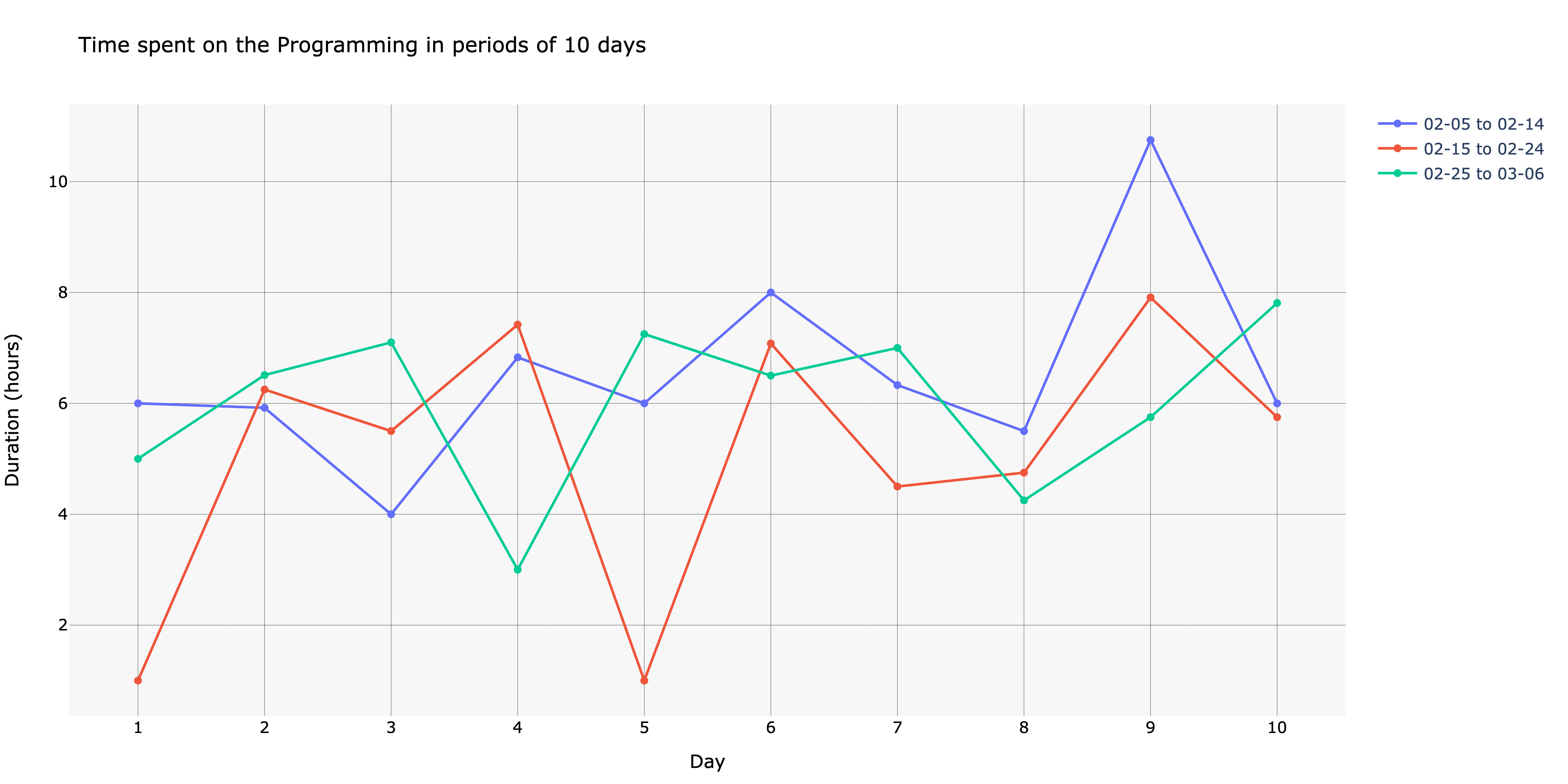 |
Raw data
{
"_id": null,
"home_page": "",
"name": "google-calendar-analytics",
"maintainer": "",
"docs_url": null,
"requires_python": ">=3.11,<4.0",
"maintainer_email": "",
"keywords": "google,calendar,analytics,data analysis,event tracking,attendee tracking",
"author": "Berupor",
"author_email": "evgeniy.zelenoff@gmail.com",
"download_url": "https://files.pythonhosted.org/packages/e4/99/a1c58d95c7103146ff5169c6efd662f3ec006bafb3ff29fc2db698228b08/google_calendar_analytics-0.5.0.tar.gz",
"platform": null,
"description": "[](https://pypi.org/project/google-calendar-analytics/)\n[](https://pypi.python.org/pypi/google-calendar-analytics)\n[](https://pypi.org/project/google-calendar-analytics/)\n[](https://pypi.python.org/pypi/google-calendar-analytics)\n\n# Google Calendar Analytics\n\n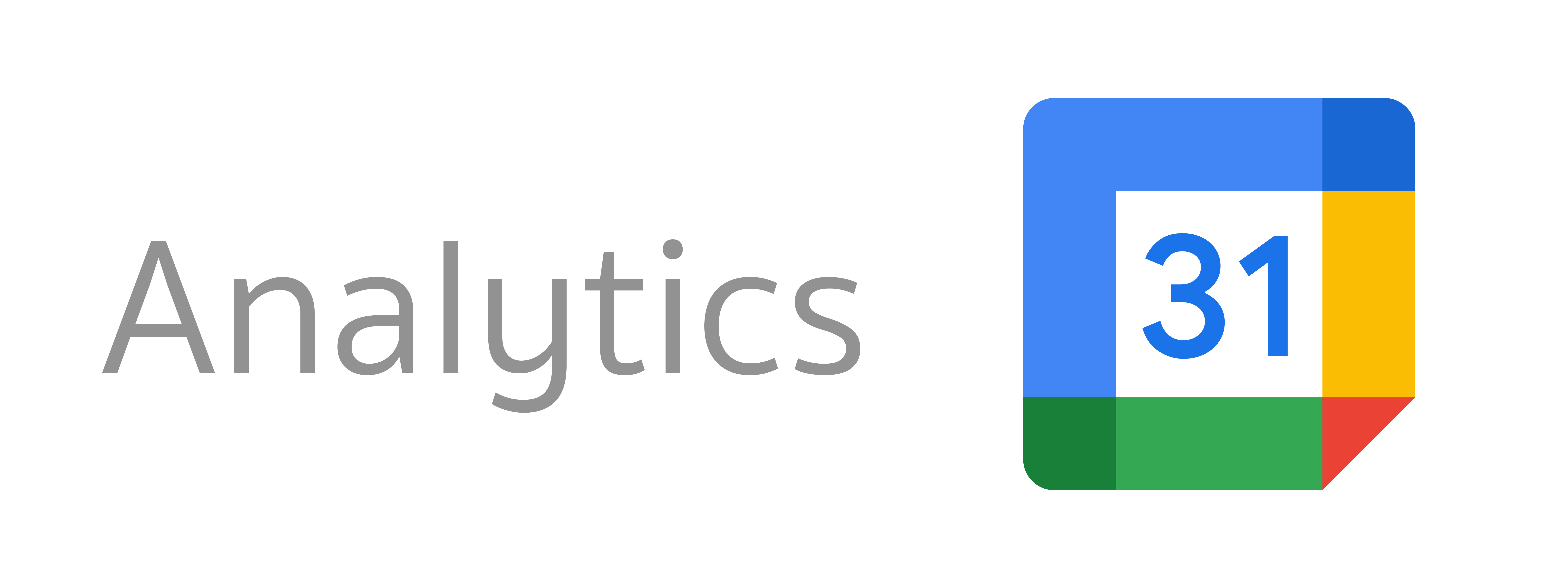\n\n---\n\n- ### [Documentation](https://berupor.github.io/Calendar-Analytics/)\n- ### [Source code](https://github.com/Berupor/Calendar-Analytics)\n\n---\nThis Python program allows you to perform analytics on your Google Calendar events. With this program, you can visualize\nthe total duration of your events, compare the length of events across different time periods, and gain insights into\nwhich events take up the most time.\n\n## Features\n\n- Async support for faster data retrieval and chart generation\n- Extract events from your Google Calendar\n- Compute the total duration of events in a specified time range\n- Visualize the duration of events in a pie chart, bar chart, line chart and more\n- Limit the number of events displayed in the charts\n- Wide chart customization. For example, dark mode and transparent background\n\n## Quick Start\n\nTo use the Google Calendar Analytics program, first install the dependencies by running the following command:\n\n```bash\npip install google-calendar-analytics\n```\n\nYou can then import the AnalyzerFacade class and use it to analyze your data:\n\n### How to get credentials from Google?\n\n1. [Google documentation](https://developers.google.com/calendar/api/quickstart/python)\n\n```python\nimport asyncio\nfrom datetime import datetime\n\nfrom google.oauth2.credentials import Credentials\nfrom google_calendar_analytics import AnalyzerFacade\n\n# (You can get it from Google OAuth2 in you web app or from link above)\ncreds = {\n \"token\": \"ya29.a0AVvZVsoH4qZcrGK25VwsXspJv-r9K-\",\n \"refresh_token\": \"1//0hwlhrtalKgeRCgYIARAAGBESNwF-\",\n \"token_uri\": \"https://oauth2.googleapis.com/token\",\n \"client_id\": \"395np.apps.googleusercontent.com\",\n \"client_secret\": \"GOCSPXFqoucE03VRVz\",\n \"scopes\": [\"https://www.googleapis.com/auth/calendar\"],\n \"expiry\": \"2023-02-18T15:30:15.674219Z\"\n}\ncreds = Credentials.from_authorized_user_info(creds)\n```\n\nOnce you have created the credentials, you can create an instance of the AnalyzerFacade class and use it to analyze your data:\n\n```python\n# Choose time range for analysis\nstart_time = datetime(2023, 3, 1)\nend_time = datetime(2023, 3, 18)\n\n\nasync def main():\n async with AnalyzerFacade(creds=creds) as analyzer:\n plot = await analyzer.analyze_one(start_time, end_time, event_name=\"Programming\", plot_type=\"Line\")\n plot.show()\n\nif __name__ == \"__main__\":\n asyncio.run(main())\n```\n\nWhat's about multiple plots?\n```python\nasync def main():\n async with AnalyzerFacade(creds=creds) as analyzer:\n coroutines = []\n \n coroutines.append(analyzer.analyze_one(start_time, end_time, event_name=\"Programming\", plot_type=\"Line\")\n coroutines.append(analyzer.analyze_one(start_time, end_time, event_name=\"Reading\", plot_type=\"Line\"))\n coroutines.append(analyzer.analyze_many(start_time, end_time, plot_type=\"Pie\"))\n coroutines.append(analyzer.analyze_many(start_time, end_time, plot_type=\"Bar\"))\n \n result = await asyncio.gather(*coroutines)\n for plot in result:\n plot.show()\n```\n\n## Contribution\n\nIf you would like to contribute to this project, please feel free to submit a pull request. Some areas where\ncontributions are particularly welcome include:\n\n- Adding new features\n- Improving existing features\n- Debugging and fixing bugs\n- Adding tests to ensure the program is working as expected\n\n## Analytics example:\n\n| Pie plot | Bar plot |\n|:-----------------------------------:|:-----------------------------------:|\n| 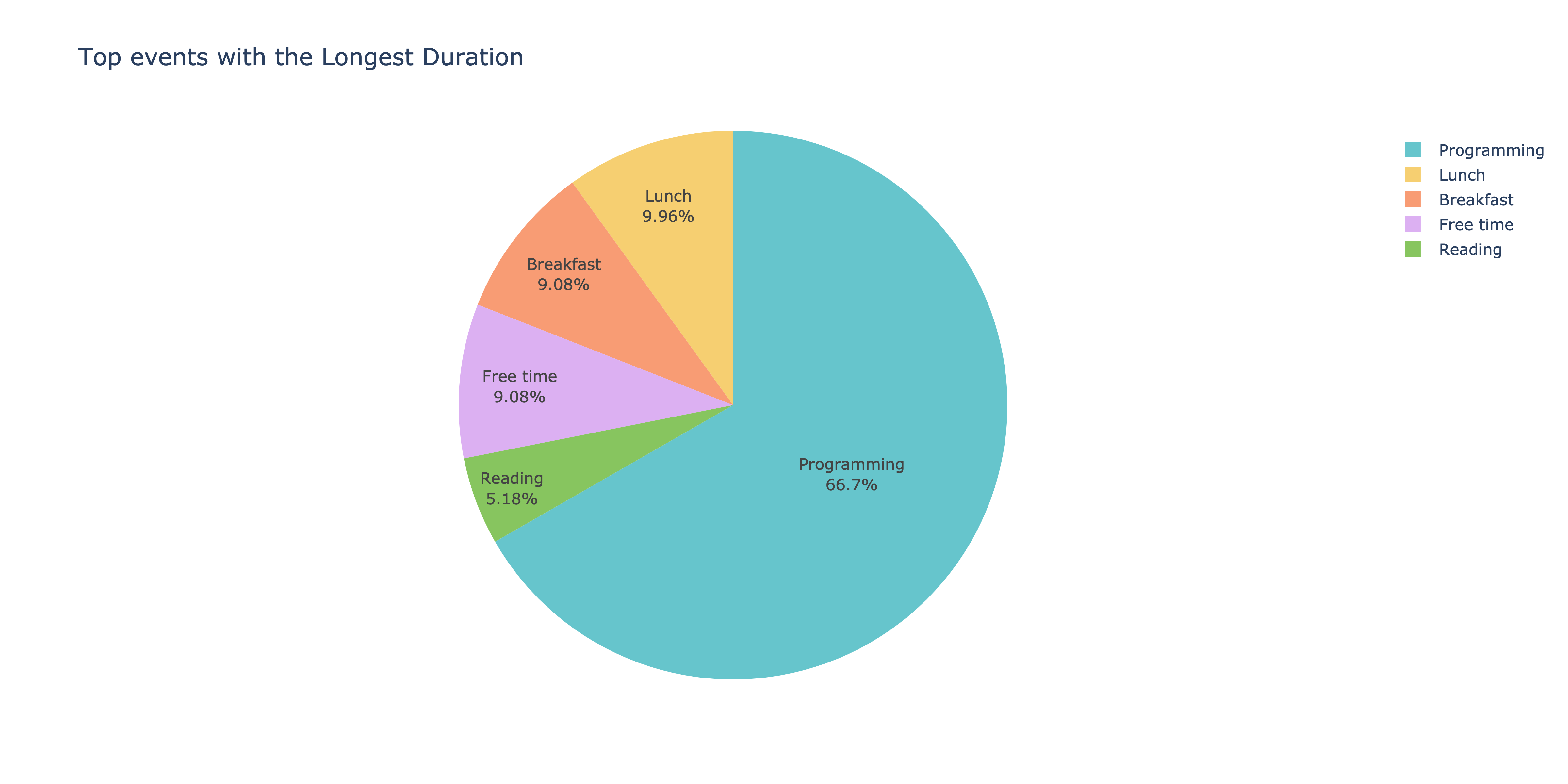 | 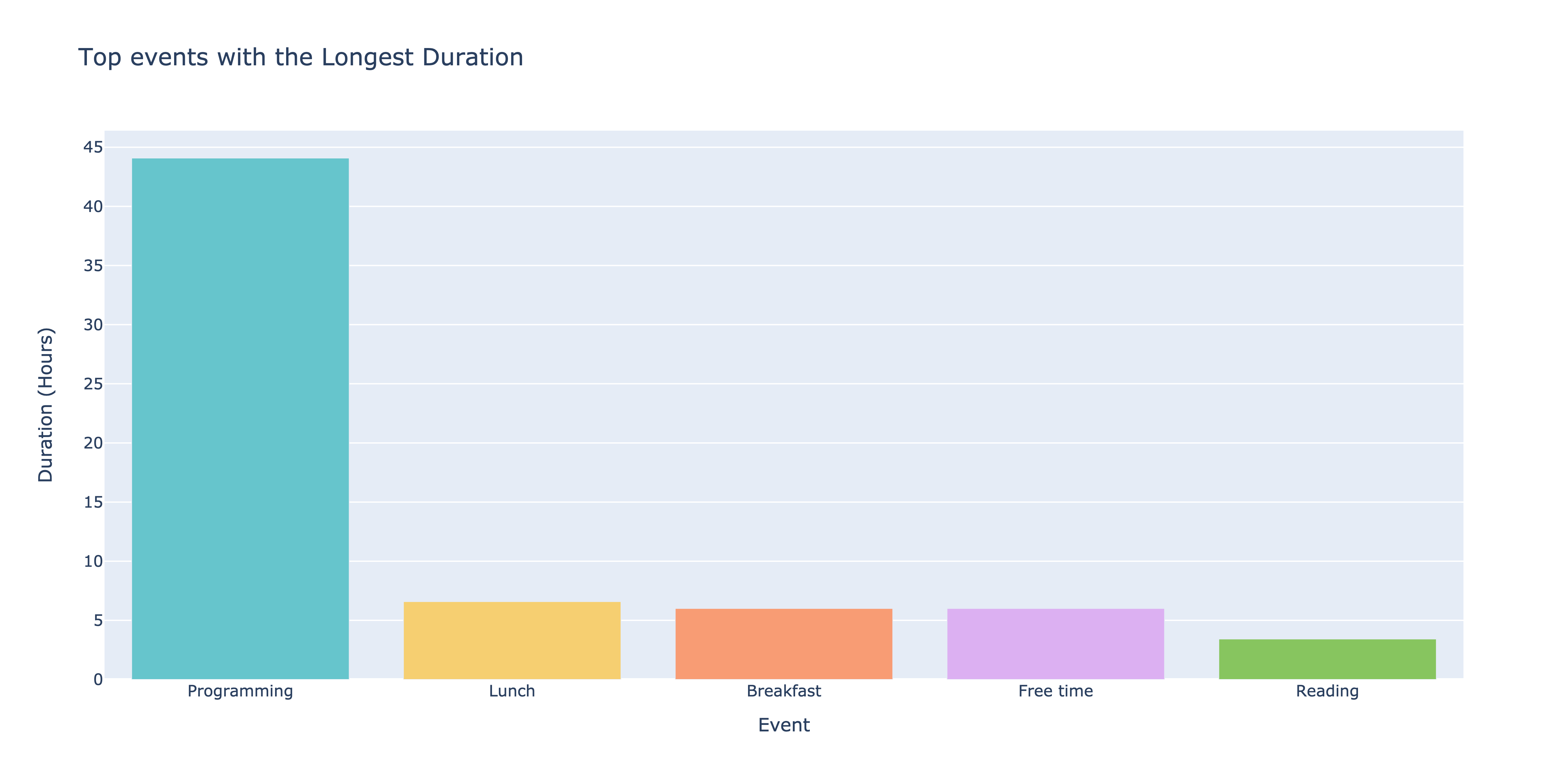 |\n\n| Line plot | Multy line plot | \n|:------------------------------------:|:--------------------------------------------------------------------------------------------------:|\n| 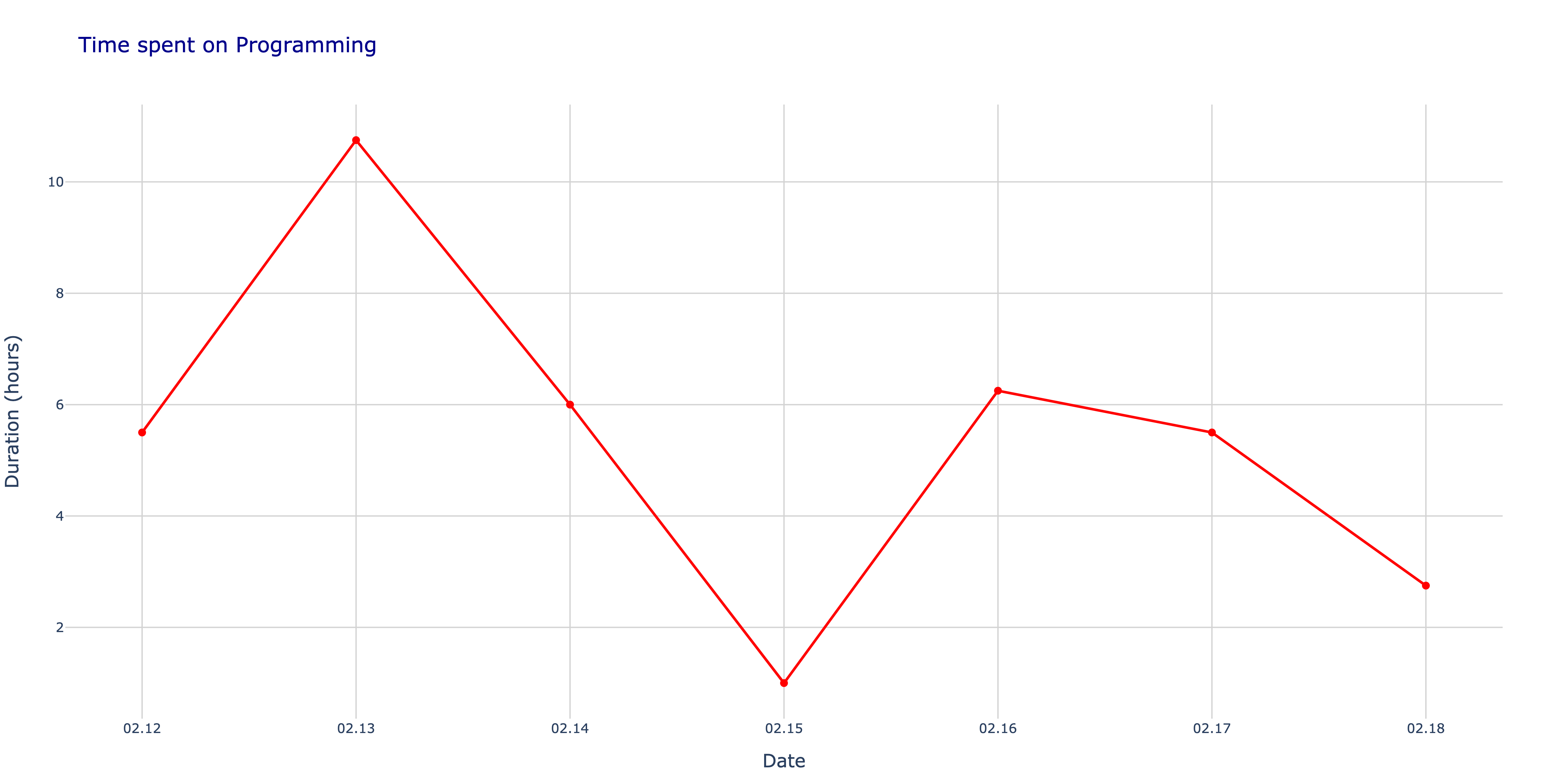 | 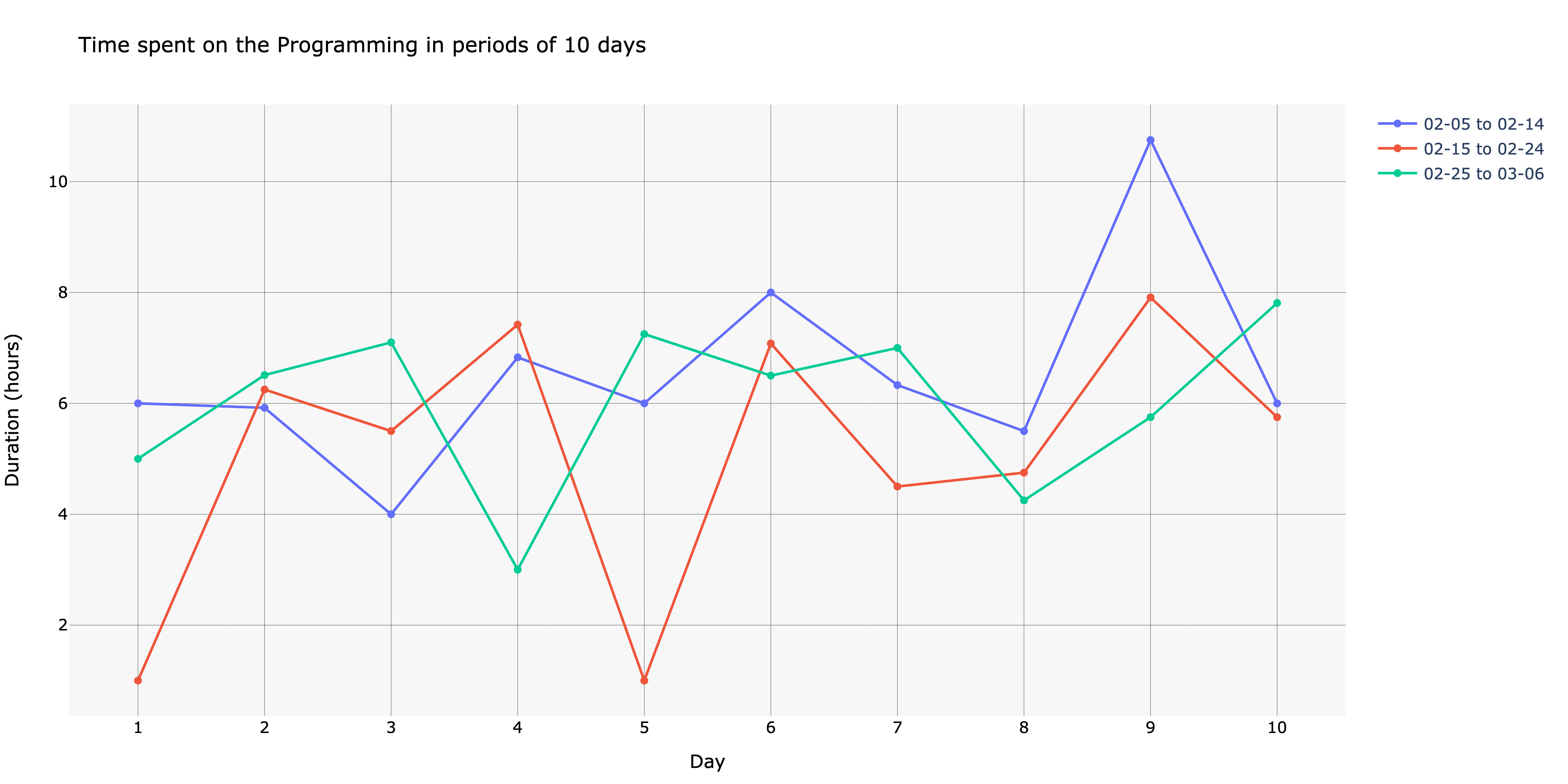 |\n \n",
"bugtrack_url": null,
"license": "MIT",
"summary": "A Python library for analyzing Google Calendar data.",
"version": "0.5.0",
"project_urls": null,
"split_keywords": [
"google",
"calendar",
"analytics",
"data analysis",
"event tracking",
"attendee tracking"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "00a38e1d97c63d081abf70deb04642b4906f87c3005250c38bf1a1e8c05fed6a",
"md5": "1251684fc870aaf69d4db8c7fb9a6b10",
"sha256": "0b59b7807abd7b20a4fc8e5f00eb4f29aa63d2aca532d5da213dee0a646d3b3c"
},
"downloads": -1,
"filename": "google_calendar_analytics-0.5.0-py3-none-any.whl",
"has_sig": false,
"md5_digest": "1251684fc870aaf69d4db8c7fb9a6b10",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": ">=3.11,<4.0",
"size": 16418,
"upload_time": "2023-05-22T03:17:20",
"upload_time_iso_8601": "2023-05-22T03:17:20.428915Z",
"url": "https://files.pythonhosted.org/packages/00/a3/8e1d97c63d081abf70deb04642b4906f87c3005250c38bf1a1e8c05fed6a/google_calendar_analytics-0.5.0-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "e499a1c58d95c7103146ff5169c6efd662f3ec006bafb3ff29fc2db698228b08",
"md5": "f8697301d027dd830ea38c4f7a14113b",
"sha256": "6aea6ae0a4db2d952e6cf5513a827117f60ffbe212a1acdf2245829b4427286a"
},
"downloads": -1,
"filename": "google_calendar_analytics-0.5.0.tar.gz",
"has_sig": false,
"md5_digest": "f8697301d027dd830ea38c4f7a14113b",
"packagetype": "sdist",
"python_version": "source",
"requires_python": ">=3.11,<4.0",
"size": 13861,
"upload_time": "2023-05-22T03:17:22",
"upload_time_iso_8601": "2023-05-22T03:17:22.233205Z",
"url": "https://files.pythonhosted.org/packages/e4/99/a1c58d95c7103146ff5169c6efd662f3ec006bafb3ff29fc2db698228b08/google_calendar_analytics-0.5.0.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2023-05-22 03:17:22",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "google-calendar-analytics"
}