# Graphsignal: Observability for AI Stack
[](https://github.com/graphsignal/graphsignal-python/blob/main/LICENSE)
[](https://github.com/graphsignal/graphsignal-python)
Graphsignal is an observability platform for AI agents and LLM-powered applications. It helps developers ensure AI applications run as expected and users have the best experience. With Graphsignal, developers can:
* Trace generations, runs, and sessions with full AI context.
* Score any user interactions and application execution.
* See latency breakdowns and distributions.
* Analyze model API costs for deployments, models, or users.
* Get notified about errors and anomalies.
* Monitor API, compute, and GPU utilization.
[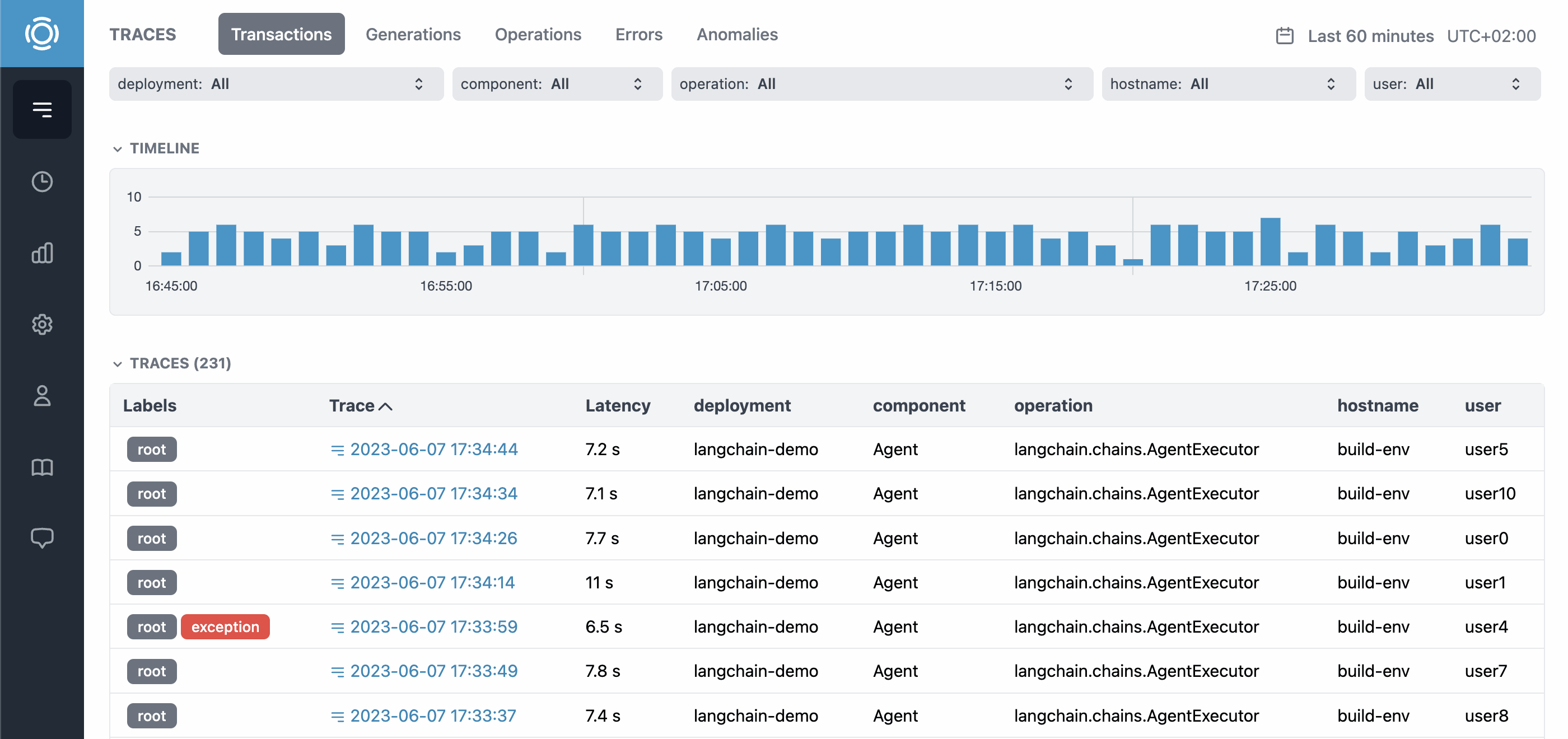](https://graphsignal.com/)
Learn more at [graphsignal.com](https://graphsignal.com).
## Install
Install Graphsignal library.
```bash
pip install --upgrade graphsignal
```
## Configure
Configure Graphsignal tracer by specifying your API key directly or via `GRAPHSIGNAL_API_KEY` environment variable.
```python
import graphsignal
graphsignal.configure(api_key='my-api-key', deployment='my-app')
```
To get an API key, sign up for a free account at [graphsignal.com](https://graphsignal.com). The key can then be found in your account's [Settings / API Keys](https://app.graphsignal.com/settings/api-keys) page.
Alternatively, you can add Graphsignal tracer at command line, when running your module or script. Environment variables `GRAPHSIGNAL_API_KEY` and `GRAPHSIGNAL_DEPLOYMENT` must be set.
```bash
python -m graphsignal <script>
```
```bash
python -m graphsignal -m <module>
```
## Integrate
Graphsignal **auto-instruments** and traces libraries and frameworks, such as [OpenAI](https://graphsignal.com/docs/integrations/openai/) and [LangChain](https://graphsignal.com/docs/integrations/langchain/). Traces, errors, and data, such as prompts and completions, are automatically recorded and available for analysis at [app.graphsignal.com](https://app.graphsignal.com/).
Refer to the guides below for detailed information on:
* [Session Tracking](https://graphsignal.com/docs/guides/session-tracking/)
* [User Tracking](https://graphsignal.com/docs/guides/user-tracking/)
* [Manual Tracing](https://graphsignal.com/docs/guides/manual-tracing/)
* [Scores and Feedback](https://graphsignal.com/docs/guides/scores-and-feedback/)
* [Cost and Usage Monitoring](https://graphsignal.com/docs/guides/cost-and-usage-monitoring/)
See [API reference](https://graphsignal.com/docs/reference/python-api/) for full documentation.
Some integration examples are available in [examples](https://github.com/graphsignal/examples) repo.
## Analyze
[Log in](https://app.graphsignal.com/) to Graphsignal to monitor and analyze your application and monitor for issues.
## Overhead
Graphsignal tracer is very lightweight. The overhead per trace is measured to be less than 100 microseconds.
## Security and Privacy
Graphsignal tracer can only open outbound connections to `api.graphsignal.com` and send data, no inbound connections or commands are possible.
Payloads, such as prompts and completions, are recorded by default in case of automatic tracing. To disable, set `record_payloads=False` in `graphsignal.configure`.
## Troubleshooting
To enable debug logging, add `debug_mode=True` to `configure()`. If the debug log doesn't give you any hints on how to fix a problem, please report it to our support team via your account.
In case of connection issues, please make sure outgoing connections to `https://api.graphsignal.com` are allowed.
Raw data
{
"_id": null,
"home_page": "https://graphsignal.com",
"name": "graphsignal",
"maintainer": null,
"docs_url": null,
"requires_python": "<4.0,>=3.8.1",
"maintainer_email": null,
"keywords": "LLM observability, LLM analytics, Agent observability, Agent analytics",
"author": "Graphsignal, Inc.",
"author_email": "devops@graphsignal.com",
"download_url": "https://files.pythonhosted.org/packages/1c/12/983d641124d75b8df4ae685ed00ad0105390dc13d3ba43cdc85aafa6bb83/graphsignal-0.15.6.tar.gz",
"platform": null,
"description": "# Graphsignal: Observability for AI Stack\n\n[](https://github.com/graphsignal/graphsignal-python/blob/main/LICENSE)\n[](https://github.com/graphsignal/graphsignal-python)\n\n\nGraphsignal is an observability platform for AI agents and LLM-powered applications. It helps developers ensure AI applications run as expected and users have the best experience. With Graphsignal, developers can:\n\n* Trace generations, runs, and sessions with full AI context.\n* Score any user interactions and application execution.\n* See latency breakdowns and distributions.\n* Analyze model API costs for deployments, models, or users.\n* Get notified about errors and anomalies.\n* Monitor API, compute, and GPU utilization.\n\n[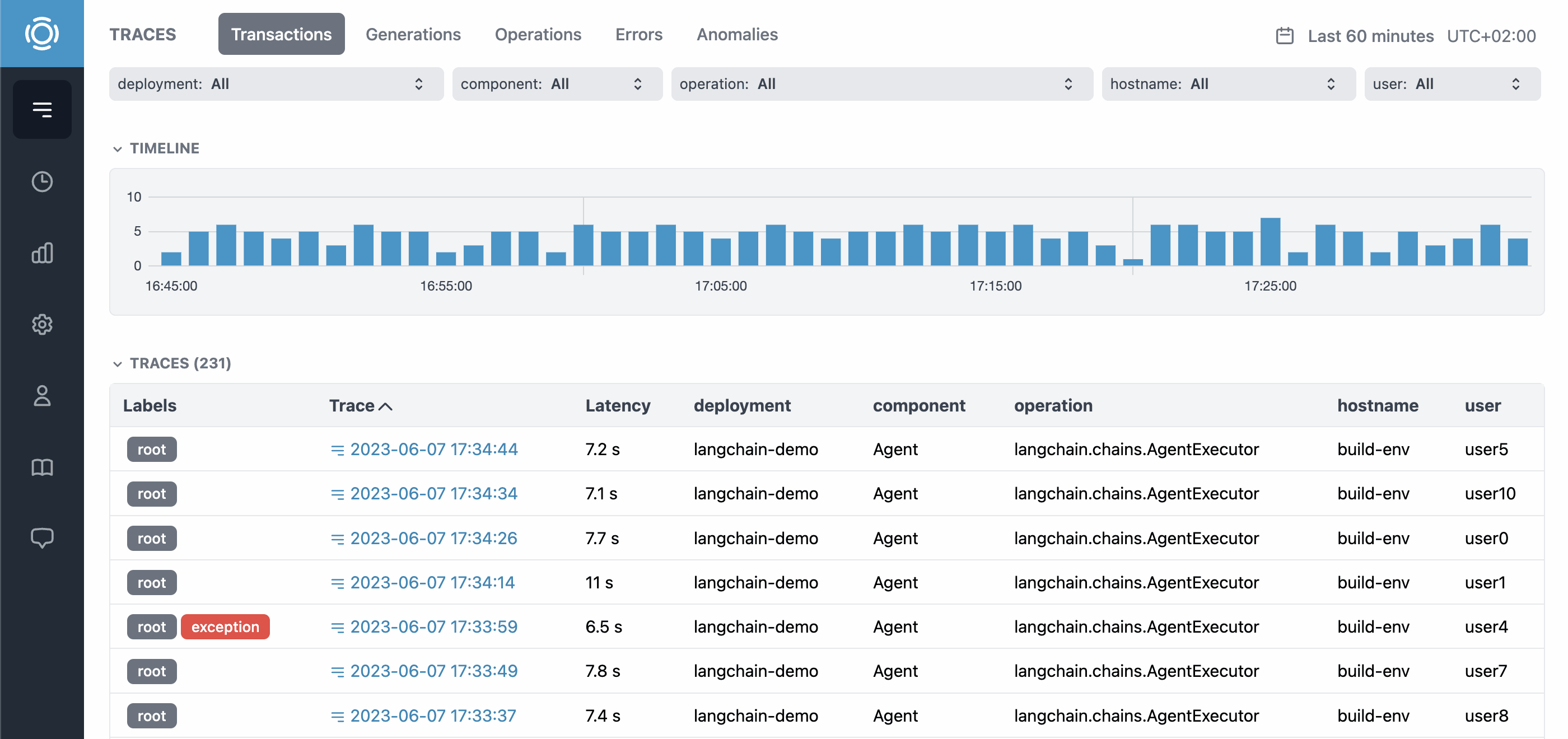](https://graphsignal.com/)\n\nLearn more at [graphsignal.com](https://graphsignal.com).\n\n\n## Install\n\nInstall Graphsignal library.\n\n```bash\npip install --upgrade graphsignal\n```\n\n\n## Configure\n\nConfigure Graphsignal tracer by specifying your API key directly or via `GRAPHSIGNAL_API_KEY` environment variable.\n\n```python\nimport graphsignal\n\ngraphsignal.configure(api_key='my-api-key', deployment='my-app')\n```\n\nTo get an API key, sign up for a free account at [graphsignal.com](https://graphsignal.com). The key can then be found in your account's [Settings / API Keys](https://app.graphsignal.com/settings/api-keys) page.\n\nAlternatively, you can add Graphsignal tracer at command line, when running your module or script. Environment variables `GRAPHSIGNAL_API_KEY` and `GRAPHSIGNAL_DEPLOYMENT` must be set.\n\n```bash\npython -m graphsignal <script>\n```\n\n```bash\npython -m graphsignal -m <module>\n```\n\n\n## Integrate\n\nGraphsignal **auto-instruments** and traces libraries and frameworks, such as [OpenAI](https://graphsignal.com/docs/integrations/openai/) and [LangChain](https://graphsignal.com/docs/integrations/langchain/). Traces, errors, and data, such as prompts and completions, are automatically recorded and available for analysis at [app.graphsignal.com](https://app.graphsignal.com/).\n\nRefer to the guides below for detailed information on:\n\n* [Session Tracking](https://graphsignal.com/docs/guides/session-tracking/)\n* [User Tracking](https://graphsignal.com/docs/guides/user-tracking/)\n* [Manual Tracing](https://graphsignal.com/docs/guides/manual-tracing/)\n* [Scores and Feedback](https://graphsignal.com/docs/guides/scores-and-feedback/)\n* [Cost and Usage Monitoring](https://graphsignal.com/docs/guides/cost-and-usage-monitoring/)\n\nSee [API reference](https://graphsignal.com/docs/reference/python-api/) for full documentation.\n\nSome integration examples are available in [examples](https://github.com/graphsignal/examples) repo.\n\n\n## Analyze\n\n[Log in](https://app.graphsignal.com/) to Graphsignal to monitor and analyze your application and monitor for issues.\n\n\n## Overhead\n\nGraphsignal tracer is very lightweight. The overhead per trace is measured to be less than 100 microseconds.\n\n\n## Security and Privacy\n\nGraphsignal tracer can only open outbound connections to `api.graphsignal.com` and send data, no inbound connections or commands are possible.\n\nPayloads, such as prompts and completions, are recorded by default in case of automatic tracing. To disable, set `record_payloads=False` in `graphsignal.configure`.\n\n\n## Troubleshooting\n\nTo enable debug logging, add `debug_mode=True` to `configure()`. If the debug log doesn't give you any hints on how to fix a problem, please report it to our support team via your account.\n\nIn case of connection issues, please make sure outgoing connections to `https://api.graphsignal.com` are allowed.\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "Graphsignal Tracer for Python",
"version": "0.15.6",
"project_urls": {
"Homepage": "https://graphsignal.com",
"Repository": "https://graphsignal.com"
},
"split_keywords": [
"llm observability",
" llm analytics",
" agent observability",
" agent analytics"
],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "ba7b3aeb7820717687717457a2f474a42bfc0986ae03d2a02d35d22a30ea0dd7",
"md5": "7ae97fc7cb98ea571e8cb4cd92735f37",
"sha256": "2679f7fc8ea78487b1920fbec227a2d2dce2ce0408b896bf0427e19344433594"
},
"downloads": -1,
"filename": "graphsignal-0.15.6-py3-none-any.whl",
"has_sig": false,
"md5_digest": "7ae97fc7cb98ea571e8cb4cd92735f37",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "<4.0,>=3.8.1",
"size": 105407,
"upload_time": "2024-08-16T09:39:22",
"upload_time_iso_8601": "2024-08-16T09:39:22.081074Z",
"url": "https://files.pythonhosted.org/packages/ba/7b/3aeb7820717687717457a2f474a42bfc0986ae03d2a02d35d22a30ea0dd7/graphsignal-0.15.6-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "1c12983d641124d75b8df4ae685ed00ad0105390dc13d3ba43cdc85aafa6bb83",
"md5": "7f1f9ac3cad78411eebed56d4f597d20",
"sha256": "6bfca2aed53c1343b5d9955741d5cfbfe084560f287c3caaa1819202905b21f8"
},
"downloads": -1,
"filename": "graphsignal-0.15.6.tar.gz",
"has_sig": false,
"md5_digest": "7f1f9ac3cad78411eebed56d4f597d20",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "<4.0,>=3.8.1",
"size": 74617,
"upload_time": "2024-08-16T09:39:23",
"upload_time_iso_8601": "2024-08-16T09:39:23.682851Z",
"url": "https://files.pythonhosted.org/packages/1c/12/983d641124d75b8df4ae685ed00ad0105390dc13d3ba43cdc85aafa6bb83/graphsignal-0.15.6.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-08-16 09:39:23",
"github": false,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"lcname": "graphsignal"
}