# CDK Pipelines for GitHub Workflows

[](https://constructs.dev/packages/cdk-pipelines-github)
> The APIs in this module are experimental and under active development.
> They are subject to non-backward compatible changes or removal in any future version. These are
> not subject to the [Semantic Versioning](https://semver.org/) model and breaking changes will be
> announced in the release notes. This means that while you may use them, you may need to update
> your source code when upgrading to a newer version of this package.
A construct library for painless Continuous Delivery of CDK applications,
deployed via
[GitHub Workflows](https://docs.github.com/en/actions/using-workflows/workflow-syntax-for-github-actions).
The CDK already has a CI/CD solution,
[CDK Pipelines](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.pipelines-readme.html),
which creates an AWS CodePipeline that deploys CDK applications. This module
serves the same surface area, except that it is implemented with GitHub
Workflows.
## Table of Contents
* [CDK Pipelines for GitHub Workflows](#cdk-pipelines-for-github-workflows)
* [Table of Contents](#table-of-contents)
* [Usage](#usage)
* [Initial Setup](#initial-setup)
* [AWS Credentials](#aws-credentials)
* [GitHub Action Role](#github-action-role)
* [`GitHubActionRole` Construct](#githubactionrole-construct)
* [GitHub Secrets](#github-secrets)
* [Runners with Preconfigured Credentials](#runners-with-preconfigured-credentials)
* [Using Docker in the Pipeline](#using-docker-in-the-pipeline)
* [Authenticating to Docker registries](#authenticating-to-docker-registries)
* [Runner Types](#runner-types)
* [GitHub Hosted Runner](#github-hosted-runner)
* [Self Hosted Runner](#self-hosted-runner)
* [Escape Hatches](#escape-hatches)
* [Additional Features](#additional-features)
* [GitHub Action Step](#github-action-step)
* [Configure GitHub Environment](#configure-github-environment)
* [Waves for Parallel Builds](#waves-for-parallel-builds)
* [Manual Approval Step](#manual-approval-step)
* [Pipeline YAML Comments](#pipeline-yaml-comments)
* [Tutorial](#tutorial)
* [Not supported yet](#not-supported-yet)
* [Contributing](#contributing)
* [License](#license)
## Usage
Assuming you have a
[`Stage`](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.Stage.html)
called `MyStage` that includes CDK stacks for your app and you want to deploy it
to two AWS environments (`BETA_ENV` and `PROD_ENV`):
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
aws_creds=AwsCredentials.from_open_id_connect(
git_hub_action_role_arn="arn:aws:iam::<account-id>:role/GitHubActionRole"
)
)
# Build the stages
beta_stage = MyStage(app, "Beta", env=BETA_ENV)
prod_stage = MyStage(app, "Prod", env=PROD_ENV)
# Add the stages for sequential build - earlier stages failing will stop later ones:
pipeline.add_stage(beta_stage)
pipeline.add_stage(prod_stage)
# OR add the stages for parallel building of multiple stages with a Wave:
wave = pipeline.add_wave("Wave")
wave.add_stage(beta_stage)
wave.add_stage(prod_stage)
app.synth()
```
When you run `cdk synth`, a `deploy.yml` workflow will be created under
`.github/workflows` in your repo. This workflow will deploy your application
based on the definition of the pipeline. In the example above, it will deploy
the two stages in sequence, and within each stage, it will deploy all the
stacks according to their dependency order and maximum parallelism. If your app
uses assets, assets will be published to the relevant destination environment.
The `Pipeline` class from `cdk-pipelines-github` is derived from the base CDK
Pipelines class, so most features should be supported out of the box. See the
[CDK Pipelines](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.pipelines-readme.html)
documentation for more details.
To express GitHub-specifc details, such as those outlined in [Additional Features](#additional-features), you have a few options:
* Use a `GitHubStage` instead of `Stage` (or make a `GitHubStage` subclass instead of a `Stage` subclass) - this adds the `GitHubCommonProps` to the `Stage` properties
* With this you can use `pipeline.addStage(myGitHubStage)` or `wave.addStage(myGitHubStage)` and the properties of the
stage will be used
* Using a `Stage` (or subclass thereof) or a `GitHubStage` (or subclass thereof) you can call `pipeline.addStageWithGitHubOptions(stage, stageOptions)` or `wave.addStageWithGitHubOptions(stage, stageOptions)`
* In this case you're providing the same options along with the stage instead of embedded in the stage.
* Note that properties of a `GitHubStage` added with `addStageWithGitHubOptions()` will override the options provided to `addStageWithGitHubOptions()`
**NOTES:**
* Environments must be bootstrapped separately using `cdk bootstrap`. See [CDK
Environment
Bootstrapping](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.pipelines-readme.html#cdk-environment-bootstrapping)
for details.
## Initial Setup
Assuming you have your CDK app checked out on your local machine, here are the suggested steps
to develop your GitHub Workflow.
* Set up AWS Credentials your local environment. It is highly recommended to authenticate via an OpenId
Connect IAM Role. You can set one up using the [`GithubActionRole`](#github-action-role) class provided
in this module. For more information (and alternatives), see [AWS Credentials](#aws-credentials).
* When you've updated your pipeline and are ready to deploy, run `cdk synth`. This creates a workflow file
in `.github/workflows/deploy.yml`.
* When you are ready to test your pipeline, commit your code changes as well as the `deploy.yml` file to
GitHub. GitHub will automatically try to run the workflow found under `.github/workflows/deploy.yml`.
* You will be able to see the result of the run on the `Actions` tab in your repository:
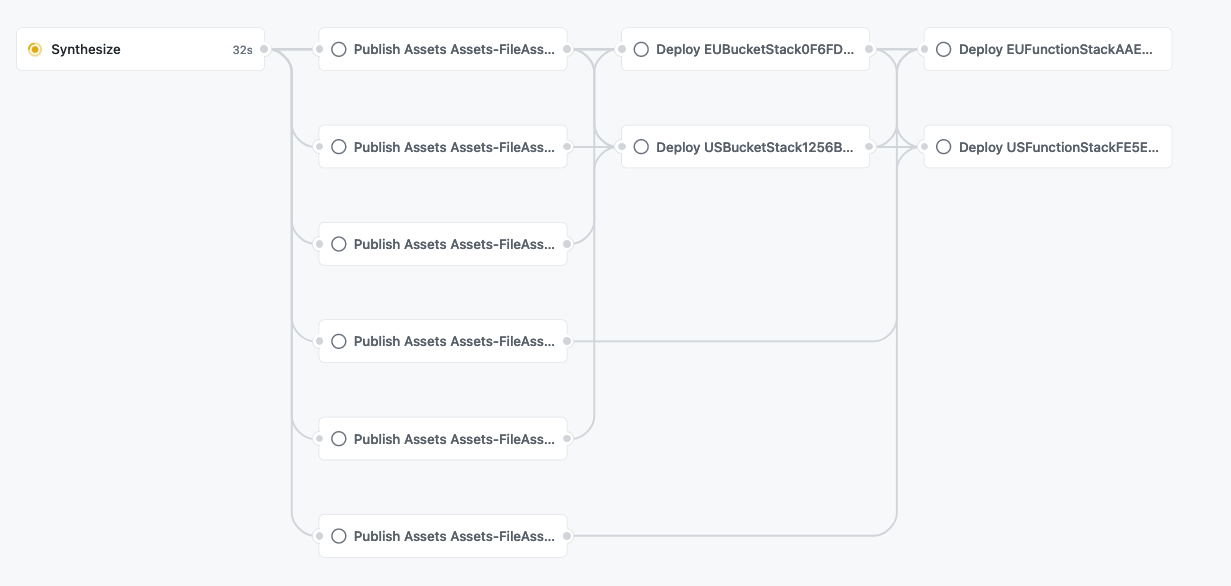
For an in-depth run-through on creating your own GitHub Workflow, see the
[Tutorial](#tutorial) section.
## AWS Credentials
There are two ways to supply AWS credentials to the workflow:
* GitHub Action IAM Role (recommended).
* Long-lived AWS Credentials stored in GitHub Secrets.
The GitHub Action IAM Role authenticates via the GitHub OpenID Connect provider
and is recommended, but it requires preparing your AWS account beforehand. This
approach allows your Workflow to exchange short-lived tokens directly from AWS.
With OIDC, benefits include:
* No cloud secrets.
* Authentication and authorization management.
* Rotating credentials.
You can read more
[here](https://docs.github.com/en/actions/deployment/security-hardening-your-deployments/about-security-hardening-with-openid-connect).
### GitHub Action Role
Authenticating via OpenId Connect means you do not need to store long-lived
credentials as GitHub Secrets. With OIDC, you provide a pre-provisioned IAM
role with optional role session name to your GitHub Workflow via the `awsCreds.fromOpenIdConnect` API:
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
aws_creds=AwsCredentials.from_open_id_connect(
git_hub_action_role_arn="arn:aws:iam::<account-id>:role/GitHubActionRole",
role_session_name="optional-role-session-name"
)
)
```
There are two ways to create this IAM role:
* Use the `GitHubActionRole` construct (recommended and described below).
* Manually set up the role ([Guide](https://github.com/cdklabs/cdk-pipelines-github/blob/main/GITHUB_ACTION_ROLE_SETUP.md)).
#### `GitHubActionRole` Construct
Because this construct involves creating an IAM role in your account, it must
be created separate to your GitHub Workflow and deployed via a normal
`cdk deploy` with your local AWS credentials. Upon successful deployment, the
arn of your newly created IAM role will be exposed as a `CfnOutput`.
To utilize this construct, create a separate CDK stack with the following code
and `cdk deploy`:
```python
class MyGitHubActionRole(Stack):
def __init__(self, scope, id, *, description=None, env=None, stackName=None, tags=None, synthesizer=None, terminationProtection=None, analyticsReporting=None, crossRegionReferences=None, permissionsBoundary=None):
super().__init__(scope, id, description=description, env=env, stackName=stackName, tags=tags, synthesizer=synthesizer, terminationProtection=terminationProtection, analyticsReporting=analyticsReporting, crossRegionReferences=crossRegionReferences, permissionsBoundary=permissionsBoundary)
provider = GitHubActionRole(self, "github-action-role",
repos=["myUser/myRepo"]
)
app = App()
MyGitHubActionRole(app, "MyGitHubActionRole")
app.synth()
```
Specifying a `repos` array grants GitHub full access to the specified repositories.
To restrict access to specific git branch, tag, or other
[GitHub OIDC subject claim](https://docs.github.com/en/actions/deployment/security-hardening-your-deployments/about-security-hardening-with-openid-connect#example-subject-claims),
specify a `subjectClaims` array instead of a `repos` array.
```python
class MyGitHubActionRole(Stack):
def __init__(self, scope, id, *, description=None, env=None, stackName=None, tags=None, synthesizer=None, terminationProtection=None, analyticsReporting=None, crossRegionReferences=None, permissionsBoundary=None):
super().__init__(scope, id, description=description, env=env, stackName=stackName, tags=tags, synthesizer=synthesizer, terminationProtection=terminationProtection, analyticsReporting=analyticsReporting, crossRegionReferences=crossRegionReferences, permissionsBoundary=permissionsBoundary)
provider = GitHubActionRole(self, "github-action-role",
subject_claims=["repo:owner/repo1:ref:refs/heads/main", "repo:owner/repo1:environment:prod"
]
)
app = App()
MyGitHubActionRole(app, "MyGitHubActionRole")
app.synth()
```
Note: If you have previously created the GitHub identity provider with url
`https://token.actions.githubusercontent.com`, the above example will fail
because you can only have one such provider defined per account. In this
case, you must provide the already created provider into your `GithubActionRole`
construct via the `provider` property.
> Make sure the audience for the provider is `sts.amazonaws.com` in this case.
```python
class MyGitHubActionRole(Stack):
def __init__(self, scope, id, *, description=None, env=None, stackName=None, tags=None, synthesizer=None, terminationProtection=None, analyticsReporting=None, crossRegionReferences=None, permissionsBoundary=None):
super().__init__(scope, id, description=description, env=env, stackName=stackName, tags=tags, synthesizer=synthesizer, terminationProtection=terminationProtection, analyticsReporting=analyticsReporting, crossRegionReferences=crossRegionReferences, permissionsBoundary=permissionsBoundary)
provider = GitHubActionRole(self, "github-action-role",
repos=["myUser/myRepo"],
provider=GitHubActionRole.existing_git_hub_actions_provider(self)
)
```
### GitHub Secrets
Authenticating via this approach means that you will be manually creating AWS
credentials and duplicating them in GitHub secrets. The workflow expects the
GitHub repository to include secrets with AWS credentials under
`AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY`. You can override these defaults
by supplying the `awsCreds.fromGitHubSecrets` API to the workflow:
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
aws_creds=AwsCredentials.from_git_hub_secrets(
access_key_id="MY_ID", # GitHub will look for the access key id under the secret `MY_ID`
secret_access_key="MY_KEY"
)
)
```
### Runners with Preconfigured Credentials
If your runners provide credentials themselves, you can configure `awsCreds` to
skip passing credentials:
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
aws_creds=AwsCredentials.runner_has_preconfigured_creds()
)
```
### Using Docker in the Pipeline
You can use Docker in GitHub Workflows in a similar fashion to CDK Pipelines.
For a full discussion on how to use Docker in CDK Pipelines, see
[Using Docker in the Pipeline](https://github.com/aws/aws-cdk/blob/master/packages/@aws-cdk/pipelines/README.md#using-docker-in-the-pipeline).
Just like CDK Pipelines, you may need to authenticate to Docker registries to
avoid being throttled.
#### Authenticating to Docker registries
You can specify credentials to use for authenticating to Docker registries as
part of the Workflow definition. This can be useful if any Docker image assets —
in the pipeline or any of the application stages — require authentication, either
due to being in a different environment (e.g., ECR repo) or to avoid throttling
(e.g., DockerHub).
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
docker_credentials=[
# Authenticate to ECR
DockerCredential.ecr("<account-id>.dkr.ecr.<aws-region>.amazonaws.com"),
# Authenticate to DockerHub
DockerCredential.docker_hub(
# These properties are defaults; feel free to omit
username_key="DOCKERHUB_USERNAME",
personal_access_token_key="DOCKERHUB_TOKEN"
),
# Authenticate to Custom Registries
DockerCredential.custom_registry("custom-registry",
username_key="CUSTOM_USERNAME",
password_key="CUSTOM_PASSWORD"
)
]
)
```
## Runner Types
You can choose to run the workflow in either a GitHub hosted or [self-hosted](https://docs.github.com/en/actions/hosting-your-own-runners/about-self-hosted-runners) runner.
### GitHub Hosted Runner
The default is `Runner.UBUNTU_LATEST`. You can override this as shown below:
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
runner=Runner.WINDOWS_LATEST
)
```
### Self Hosted Runner
The following example shows how to configure the workflow to run on a self-hosted runner. Note that you do not need to pass in `self-hosted` explicitly as a label.
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
runner=Runner.self_hosted(["label1", "label2"])
)
```
## Escape Hatches
You can override the `deploy.yml` workflow file post-synthesis however you like.
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
)
)
deploy_workflow = pipeline.workflow_file
# add `on: workflow_call: {}` to deploy.yml
deploy_workflow.patch(JsonPatch.add("/on/workflow_call", {}))
# remove `on: workflow_dispatch` from deploy.yml
deploy_workflow.patch(JsonPatch.remove("/on/workflow_dispatch"))
```
## Additional Features
Below is a compilation of additional features available for GitHub Workflows.
### GitHub Action Step
If you want to call a GitHub Action in a step, you can utilize the `GitHubActionStep`.
`GitHubActionStep` extends `Step` and can be used anywhere a `Step` type is allowed.
The `jobSteps` array is placed into the pipeline job at the relevant `jobs.<job_id>.steps` as [documented here](https://docs.github.com/en/actions/using-workflows/workflow-syntax-for-github-actions#jobsjob_idsteps).
In this example,
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
)
)
# "Beta" stage with a pre-check that uses code from the repo and an action
stage = MyStage(app, "Beta", env=BETA_ENV)
pipeline.add_stage(stage,
pre=[GitHubActionStep("PreBetaDeployAction",
job_steps=[JobStep(
name="Checkout",
uses="actions/checkout@v3"
), JobStep(
name="pre beta-deploy action",
uses="my-pre-deploy-action@1.0.0"
), JobStep(
name="pre beta-deploy check",
run="npm run preDeployCheck"
)
]
)]
)
app.synth()
```
### Configure GitHub Environment
You can run your GitHub Workflow in select
[GitHub Environments](https://docs.github.com/en/actions/deployment/targeting-different-environments/using-environments-for-deployment).
Via the GitHub UI, you can configure environments with protection rules and secrets, and reference
those environments in your CDK app. A workflow that references an environment must follow any
protection rules for the environment before running or accessing the environment's secrets.
Assuming (just like in the main [example](#usage)) you have a
[`Stage`](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.Stage.html)
called `MyStage` that includes CDK stacks for your app and you want to deploy it
to two AWS environments (`BETA_ENV` and `PROD_ENV`) as well as GitHub Environments
`beta` and `prod`:
```python
from aws_cdk.pipelines import ShellStep
app = App()
pipeline = GitHubWorkflow(app, "Pipeline",
synth=ShellStep("Build",
commands=["yarn install", "yarn build"
]
),
aws_creds=AwsCredentials.from_open_id_connect(
git_hub_action_role_arn="arn:aws:iam::<account-id>:role/GitHubActionRole"
)
)
pipeline.add_stage_with_git_hub_options(Stage(self, "Beta",
env=BETA_ENV
),
git_hub_environment=GitHubEnvironment(name="beta")
)
pipeline.add_stage_with_git_hub_options(MyStage(self, "Prod",
env=PROD_ENV
),
git_hub_environment=GitHubEnvironment(name="prod")
)
app.synth()
```
#### Waves for Parallel Builds
You can add a Wave to a pipeline, where each stage of a wave will build in parallel.
> **Note**: The `pipeline.addWave()` call will return a `Wave` object that is actually a `GitHubWave` object, but
> due to JSII rules the return type of `addWave()` cannot be changed. If you need to use
> `wave.addStageWithGitHubOptions()` then you should call `pipeline.addGitHubWave()` instead, or you can
> use `GitHubStage`s to carry the GitHub properties.
When deploying to multiple accounts or otherwise deploying mostly-unrelated stacks, using waves can be a huge win.
Here's a relatively large (but real) example, **without** a wave:
<img width="1955" alt="without-waves-light-mode" src="https://user-images.githubusercontent.com/386001/217436992-d8e46c23-6295-48ec-b139-add60b1f5a14.png">
You can see how dependencies get chained unnecessarily, where the `cUrl` step should be the final step (a test) for an account:
<img width="1955" alt="without-waves-deps-light-mode" src="https://user-images.githubusercontent.com/386001/217437074-3c86d88e-6be7-4b10-97b1-6b51b100e4d6.png">
Here's the exact same stages deploying the same stacks to the same accounts, but **with** a wave:
<img width="1955" alt="with-waves" src="https://user-images.githubusercontent.com/386001/217437228-72f6c278-7e97-4a88-91fa-089628ea0381.png">
And the dependency chains are reduced to only what is actually needed, with the `cUrl` calls as the final stage for each account:
<img width="1955" alt="deps" src="https://user-images.githubusercontent.com/386001/217437265-1c10cd5f-3c7d-4e3a-af5c-acbdf3acff1b.png">
For additional information and a code example see [here](docs/waves.md).
#### Manual Approval Step
One use case for using GitHub Environments with your CDK Pipeline is to create a
manual approval step for specific environments via Environment protection rules.
From the GitHub UI, you can specify up to 5 required reviewers that must approve
before the deployment can proceed:
<img width="1134" alt="require-reviewers" src="https://user-images.githubusercontent.com/7248260/163494925-627f5ca7-a34e-48fa-bec7-1e4924ab6c0c.png">
For more information and a tutorial for how to set this up, see this
[discussion](https://github.com/cdklabs/cdk-pipelines-github/issues/162).
### Pipeline YAML Comments
An "AUTOMATICALLY GENERATED FILE..." comment will by default be added to the top
of the pipeline YAML. This can be overriden as desired to add additional context
to the pipeline YAML.
```
declare const pipeline: GitHubWorkflow;
pipeline.workflowFile.commentAtTop = `AUTOGENERATED FILE, DO NOT EDIT DIRECTLY!
Deployed stacks from this pipeline:
${STACK_NAMES.map((s)=>`- ${s}\n`)}`;
```
This will generate the normal `deploy.yml` file, but with the additional comments:
```yaml
# AUTOGENERATED FILE, DO NOT EDIT DIRECTLY!
# Deployed stacks from this pipeline:
# - APIStack
# - AuroraStack
name: deploy
on:
push:
branches:
< the rest of the pipeline YAML contents>
```
## Tutorial
You can find an example usage in [test/example-app.ts](./test/example-app.ts)
which includes a simple CDK app and a pipeline.
You can find a repository that uses this example here: [eladb/test-app-cdkpipeline](https://github.com/eladb/test-app-cdkpipeline).
To run the example, clone this repository and install dependencies:
```shell
cd ~/projects # or some other playground space
git clone https://github.com/cdklabs/cdk-pipelines-github
cd cdk-pipelines-github
yarn
```
Now, create a new GitHub repository and clone it as well:
```shell
cd ~/projects
git clone https://github.com/myaccount/my-test-repository
```
You'll need to set up AWS credentials in your environment. Note that this tutorial uses
long-lived GitHub secrets as credentials for simplicity, but it is recommended to set up
a GitHub OIDC role instead.
```shell
export AWS_ACCESS_KEY_ID=xxxx
export AWS_SECRET_ACCESS_KEY=xxxxx
```
Bootstrap your environments:
```shell
export CDK_NEW_BOOTSTRAP=1
npx cdk bootstrap aws://ACCOUNTID/us-east-1
npx cdk bootstrap aws://ACCOUNTID/eu-west-2
```
Now, run the `manual-test.sh` script when your working directory is the new repository:
```shell
cd ~/projects/my-test-repository
~/projects/cdk-piplines/github/test/manual-test.sh
```
This will produce a `cdk.out` directory and a `.github/workflows/deploy.yml` file.
Commit and push these files to your repo and you should see the deployment
workflow in action. Make sure your GitHub repository has `AWS_ACCESS_KEY_ID` and
`AWS_SECRET_ACCESS_KEY` secrets that can access the same account that you
synthesized against.
> In this tutorial, you are supposed to commit `cdk.out` (i.e. the code is pre-synthed).
> Do not do this in your app; you should always synth during the synth step of the GitHub
> workflow. In the example app this is achieved through the `preSynthed: true` option.
> It is for example purposes only and is not something you should do in your app.
>
> ```python
> from aws_cdk.pipelines import ShellStep
>
> pipeline = GitHubWorkflow(App(), "Pipeline",
> synth=ShellStep("Build",
> commands=["echo \"nothing to do (cdk.out is committed)\""]
> ),
> # only the example app should do this. your app should synth in the synth step.
> pre_synthed=True
> )
> ```
## Not supported yet
Most features that exist in CDK Pipelines are supported. However, as the CDK Pipelines
feature are expands, the feature set for GitHub Workflows may lag behind. If you see a
feature that you feel should be supported by GitHub Workflows, please open a GitHub issue
to track it.
## Contributing
See [CONTRIBUTING](CONTRIBUTING.md) for more information.
## License
This project is licensed under the Apache-2.0 License.
Raw data
{
"_id": null,
"home_page": "https://github.com/hojulian/cdk-pipelines-github.git",
"name": "hojulian.cdk-pipelines-github",
"maintainer": "",
"docs_url": null,
"requires_python": "~=3.8",
"maintainer_email": "",
"keywords": "",
"author": "Amazon Web Services<aws-cdk-dev@amazon.com>",
"author_email": "",
"download_url": "https://files.pythonhosted.org/packages/d3/c8/20c8f3e836ffd6d2a996fbca8506da489193cdd963888d2052298ff93756/hojulian.cdk-pipelines-github-0.0.9.tar.gz",
"platform": null,
"description": "# CDK Pipelines for GitHub Workflows\n\n\n\n[](https://constructs.dev/packages/cdk-pipelines-github)\n\n> The APIs in this module are experimental and under active development.\n> They are subject to non-backward compatible changes or removal in any future version. These are\n> not subject to the [Semantic Versioning](https://semver.org/) model and breaking changes will be\n> announced in the release notes. This means that while you may use them, you may need to update\n> your source code when upgrading to a newer version of this package.\n\nA construct library for painless Continuous Delivery of CDK applications,\ndeployed via\n[GitHub Workflows](https://docs.github.com/en/actions/using-workflows/workflow-syntax-for-github-actions).\n\nThe CDK already has a CI/CD solution,\n[CDK Pipelines](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.pipelines-readme.html),\nwhich creates an AWS CodePipeline that deploys CDK applications. This module\nserves the same surface area, except that it is implemented with GitHub\nWorkflows.\n\n## Table of Contents\n\n* [CDK Pipelines for GitHub Workflows](#cdk-pipelines-for-github-workflows)\n\n * [Table of Contents](#table-of-contents)\n * [Usage](#usage)\n * [Initial Setup](#initial-setup)\n * [AWS Credentials](#aws-credentials)\n\n * [GitHub Action Role](#github-action-role)\n\n * [`GitHubActionRole` Construct](#githubactionrole-construct)\n * [GitHub Secrets](#github-secrets)\n * [Runners with Preconfigured Credentials](#runners-with-preconfigured-credentials)\n * [Using Docker in the Pipeline](#using-docker-in-the-pipeline)\n\n * [Authenticating to Docker registries](#authenticating-to-docker-registries)\n * [Runner Types](#runner-types)\n\n * [GitHub Hosted Runner](#github-hosted-runner)\n * [Self Hosted Runner](#self-hosted-runner)\n * [Escape Hatches](#escape-hatches)\n * [Additional Features](#additional-features)\n\n * [GitHub Action Step](#github-action-step)\n * [Configure GitHub Environment](#configure-github-environment)\n\n * [Waves for Parallel Builds](#waves-for-parallel-builds)\n * [Manual Approval Step](#manual-approval-step)\n * [Pipeline YAML Comments](#pipeline-yaml-comments)\n * [Tutorial](#tutorial)\n * [Not supported yet](#not-supported-yet)\n * [Contributing](#contributing)\n * [License](#license)\n\n## Usage\n\nAssuming you have a\n[`Stage`](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.Stage.html)\ncalled `MyStage` that includes CDK stacks for your app and you want to deploy it\nto two AWS environments (`BETA_ENV` and `PROD_ENV`):\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n aws_creds=AwsCredentials.from_open_id_connect(\n git_hub_action_role_arn=\"arn:aws:iam::<account-id>:role/GitHubActionRole\"\n )\n)\n\n# Build the stages\nbeta_stage = MyStage(app, \"Beta\", env=BETA_ENV)\nprod_stage = MyStage(app, \"Prod\", env=PROD_ENV)\n\n# Add the stages for sequential build - earlier stages failing will stop later ones:\npipeline.add_stage(beta_stage)\npipeline.add_stage(prod_stage)\n\n# OR add the stages for parallel building of multiple stages with a Wave:\nwave = pipeline.add_wave(\"Wave\")\nwave.add_stage(beta_stage)\nwave.add_stage(prod_stage)\n\napp.synth()\n```\n\nWhen you run `cdk synth`, a `deploy.yml` workflow will be created under\n`.github/workflows` in your repo. This workflow will deploy your application\nbased on the definition of the pipeline. In the example above, it will deploy\nthe two stages in sequence, and within each stage, it will deploy all the\nstacks according to their dependency order and maximum parallelism. If your app\nuses assets, assets will be published to the relevant destination environment.\n\nThe `Pipeline` class from `cdk-pipelines-github` is derived from the base CDK\nPipelines class, so most features should be supported out of the box. See the\n[CDK Pipelines](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.pipelines-readme.html)\ndocumentation for more details.\n\nTo express GitHub-specifc details, such as those outlined in [Additional Features](#additional-features), you have a few options:\n\n* Use a `GitHubStage` instead of `Stage` (or make a `GitHubStage` subclass instead of a `Stage` subclass) - this adds the `GitHubCommonProps` to the `Stage` properties\n\n * With this you can use `pipeline.addStage(myGitHubStage)` or `wave.addStage(myGitHubStage)` and the properties of the\n stage will be used\n* Using a `Stage` (or subclass thereof) or a `GitHubStage` (or subclass thereof) you can call `pipeline.addStageWithGitHubOptions(stage, stageOptions)` or `wave.addStageWithGitHubOptions(stage, stageOptions)`\n\n * In this case you're providing the same options along with the stage instead of embedded in the stage.\n * Note that properties of a `GitHubStage` added with `addStageWithGitHubOptions()` will override the options provided to `addStageWithGitHubOptions()`\n\n**NOTES:**\n\n* Environments must be bootstrapped separately using `cdk bootstrap`. See [CDK\n Environment\n Bootstrapping](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.pipelines-readme.html#cdk-environment-bootstrapping)\n for details.\n\n## Initial Setup\n\nAssuming you have your CDK app checked out on your local machine, here are the suggested steps\nto develop your GitHub Workflow.\n\n* Set up AWS Credentials your local environment. It is highly recommended to authenticate via an OpenId\n Connect IAM Role. You can set one up using the [`GithubActionRole`](#github-action-role) class provided\n in this module. For more information (and alternatives), see [AWS Credentials](#aws-credentials).\n* When you've updated your pipeline and are ready to deploy, run `cdk synth`. This creates a workflow file\n in `.github/workflows/deploy.yml`.\n* When you are ready to test your pipeline, commit your code changes as well as the `deploy.yml` file to\n GitHub. GitHub will automatically try to run the workflow found under `.github/workflows/deploy.yml`.\n* You will be able to see the result of the run on the `Actions` tab in your repository:\n\n 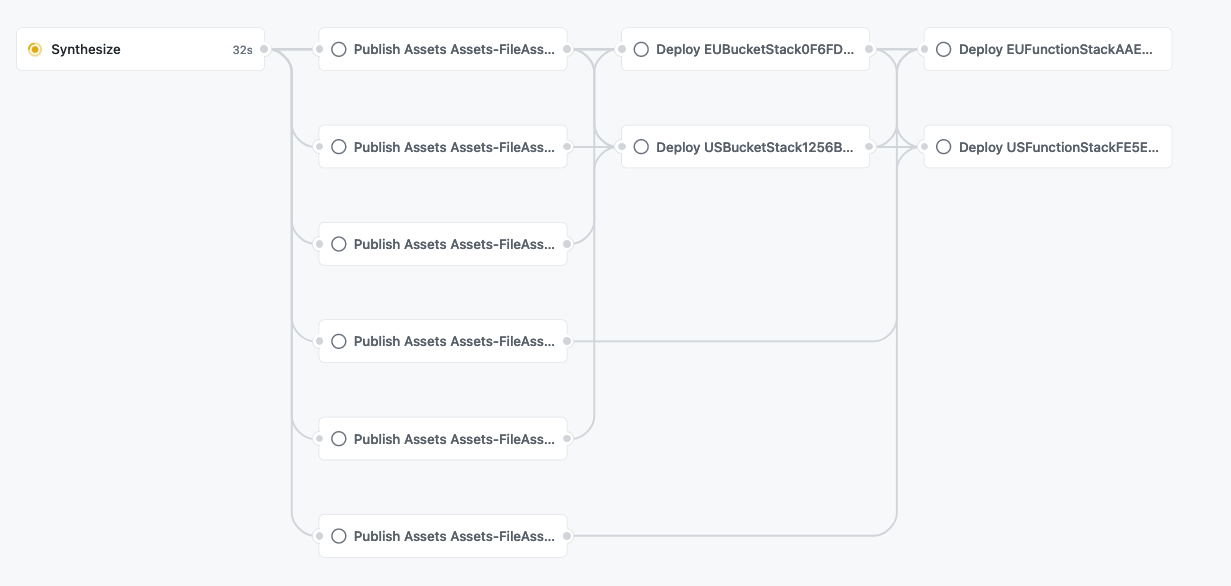\n\nFor an in-depth run-through on creating your own GitHub Workflow, see the\n[Tutorial](#tutorial) section.\n\n## AWS Credentials\n\nThere are two ways to supply AWS credentials to the workflow:\n\n* GitHub Action IAM Role (recommended).\n* Long-lived AWS Credentials stored in GitHub Secrets.\n\nThe GitHub Action IAM Role authenticates via the GitHub OpenID Connect provider\nand is recommended, but it requires preparing your AWS account beforehand. This\napproach allows your Workflow to exchange short-lived tokens directly from AWS.\nWith OIDC, benefits include:\n\n* No cloud secrets.\n* Authentication and authorization management.\n* Rotating credentials.\n\nYou can read more\n[here](https://docs.github.com/en/actions/deployment/security-hardening-your-deployments/about-security-hardening-with-openid-connect).\n\n### GitHub Action Role\n\nAuthenticating via OpenId Connect means you do not need to store long-lived\ncredentials as GitHub Secrets. With OIDC, you provide a pre-provisioned IAM\nrole with optional role session name to your GitHub Workflow via the `awsCreds.fromOpenIdConnect` API:\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n aws_creds=AwsCredentials.from_open_id_connect(\n git_hub_action_role_arn=\"arn:aws:iam::<account-id>:role/GitHubActionRole\",\n role_session_name=\"optional-role-session-name\"\n )\n)\n```\n\nThere are two ways to create this IAM role:\n\n* Use the `GitHubActionRole` construct (recommended and described below).\n* Manually set up the role ([Guide](https://github.com/cdklabs/cdk-pipelines-github/blob/main/GITHUB_ACTION_ROLE_SETUP.md)).\n\n#### `GitHubActionRole` Construct\n\nBecause this construct involves creating an IAM role in your account, it must\nbe created separate to your GitHub Workflow and deployed via a normal\n`cdk deploy` with your local AWS credentials. Upon successful deployment, the\narn of your newly created IAM role will be exposed as a `CfnOutput`.\n\nTo utilize this construct, create a separate CDK stack with the following code\nand `cdk deploy`:\n\n```python\nclass MyGitHubActionRole(Stack):\n def __init__(self, scope, id, *, description=None, env=None, stackName=None, tags=None, synthesizer=None, terminationProtection=None, analyticsReporting=None, crossRegionReferences=None, permissionsBoundary=None):\n super().__init__(scope, id, description=description, env=env, stackName=stackName, tags=tags, synthesizer=synthesizer, terminationProtection=terminationProtection, analyticsReporting=analyticsReporting, crossRegionReferences=crossRegionReferences, permissionsBoundary=permissionsBoundary)\n\n provider = GitHubActionRole(self, \"github-action-role\",\n repos=[\"myUser/myRepo\"]\n )\n\napp = App()\nMyGitHubActionRole(app, \"MyGitHubActionRole\")\napp.synth()\n```\n\nSpecifying a `repos` array grants GitHub full access to the specified repositories.\nTo restrict access to specific git branch, tag, or other\n[GitHub OIDC subject claim](https://docs.github.com/en/actions/deployment/security-hardening-your-deployments/about-security-hardening-with-openid-connect#example-subject-claims),\nspecify a `subjectClaims` array instead of a `repos` array.\n\n```python\nclass MyGitHubActionRole(Stack):\n def __init__(self, scope, id, *, description=None, env=None, stackName=None, tags=None, synthesizer=None, terminationProtection=None, analyticsReporting=None, crossRegionReferences=None, permissionsBoundary=None):\n super().__init__(scope, id, description=description, env=env, stackName=stackName, tags=tags, synthesizer=synthesizer, terminationProtection=terminationProtection, analyticsReporting=analyticsReporting, crossRegionReferences=crossRegionReferences, permissionsBoundary=permissionsBoundary)\n\n provider = GitHubActionRole(self, \"github-action-role\",\n subject_claims=[\"repo:owner/repo1:ref:refs/heads/main\", \"repo:owner/repo1:environment:prod\"\n ]\n )\n\napp = App()\nMyGitHubActionRole(app, \"MyGitHubActionRole\")\napp.synth()\n```\n\nNote: If you have previously created the GitHub identity provider with url\n`https://token.actions.githubusercontent.com`, the above example will fail\nbecause you can only have one such provider defined per account. In this\ncase, you must provide the already created provider into your `GithubActionRole`\nconstruct via the `provider` property.\n\n> Make sure the audience for the provider is `sts.amazonaws.com` in this case.\n\n```python\nclass MyGitHubActionRole(Stack):\n def __init__(self, scope, id, *, description=None, env=None, stackName=None, tags=None, synthesizer=None, terminationProtection=None, analyticsReporting=None, crossRegionReferences=None, permissionsBoundary=None):\n super().__init__(scope, id, description=description, env=env, stackName=stackName, tags=tags, synthesizer=synthesizer, terminationProtection=terminationProtection, analyticsReporting=analyticsReporting, crossRegionReferences=crossRegionReferences, permissionsBoundary=permissionsBoundary)\n\n provider = GitHubActionRole(self, \"github-action-role\",\n repos=[\"myUser/myRepo\"],\n provider=GitHubActionRole.existing_git_hub_actions_provider(self)\n )\n```\n\n### GitHub Secrets\n\nAuthenticating via this approach means that you will be manually creating AWS\ncredentials and duplicating them in GitHub secrets. The workflow expects the\nGitHub repository to include secrets with AWS credentials under\n`AWS_ACCESS_KEY_ID` and `AWS_SECRET_ACCESS_KEY`. You can override these defaults\nby supplying the `awsCreds.fromGitHubSecrets` API to the workflow:\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n aws_creds=AwsCredentials.from_git_hub_secrets(\n access_key_id=\"MY_ID\", # GitHub will look for the access key id under the secret `MY_ID`\n secret_access_key=\"MY_KEY\"\n )\n)\n```\n\n### Runners with Preconfigured Credentials\n\nIf your runners provide credentials themselves, you can configure `awsCreds` to\nskip passing credentials:\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n aws_creds=AwsCredentials.runner_has_preconfigured_creds()\n)\n```\n\n### Using Docker in the Pipeline\n\nYou can use Docker in GitHub Workflows in a similar fashion to CDK Pipelines.\nFor a full discussion on how to use Docker in CDK Pipelines, see\n[Using Docker in the Pipeline](https://github.com/aws/aws-cdk/blob/master/packages/@aws-cdk/pipelines/README.md#using-docker-in-the-pipeline).\n\nJust like CDK Pipelines, you may need to authenticate to Docker registries to\navoid being throttled.\n\n#### Authenticating to Docker registries\n\nYou can specify credentials to use for authenticating to Docker registries as\npart of the Workflow definition. This can be useful if any Docker image assets \u2014\nin the pipeline or any of the application stages \u2014 require authentication, either\ndue to being in a different environment (e.g., ECR repo) or to avoid throttling\n(e.g., DockerHub).\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n docker_credentials=[\n # Authenticate to ECR\n DockerCredential.ecr(\"<account-id>.dkr.ecr.<aws-region>.amazonaws.com\"),\n\n # Authenticate to DockerHub\n DockerCredential.docker_hub(\n # These properties are defaults; feel free to omit\n username_key=\"DOCKERHUB_USERNAME\",\n personal_access_token_key=\"DOCKERHUB_TOKEN\"\n ),\n\n # Authenticate to Custom Registries\n DockerCredential.custom_registry(\"custom-registry\",\n username_key=\"CUSTOM_USERNAME\",\n password_key=\"CUSTOM_PASSWORD\"\n )\n ]\n)\n```\n\n## Runner Types\n\nYou can choose to run the workflow in either a GitHub hosted or [self-hosted](https://docs.github.com/en/actions/hosting-your-own-runners/about-self-hosted-runners) runner.\n\n### GitHub Hosted Runner\n\nThe default is `Runner.UBUNTU_LATEST`. You can override this as shown below:\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n runner=Runner.WINDOWS_LATEST\n)\n```\n\n### Self Hosted Runner\n\nThe following example shows how to configure the workflow to run on a self-hosted runner. Note that you do not need to pass in `self-hosted` explicitly as a label.\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n runner=Runner.self_hosted([\"label1\", \"label2\"])\n)\n```\n\n## Escape Hatches\n\nYou can override the `deploy.yml` workflow file post-synthesis however you like.\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n )\n)\n\ndeploy_workflow = pipeline.workflow_file\n# add `on: workflow_call: {}` to deploy.yml\ndeploy_workflow.patch(JsonPatch.add(\"/on/workflow_call\", {}))\n# remove `on: workflow_dispatch` from deploy.yml\ndeploy_workflow.patch(JsonPatch.remove(\"/on/workflow_dispatch\"))\n```\n\n## Additional Features\n\nBelow is a compilation of additional features available for GitHub Workflows.\n\n### GitHub Action Step\n\nIf you want to call a GitHub Action in a step, you can utilize the `GitHubActionStep`.\n`GitHubActionStep` extends `Step` and can be used anywhere a `Step` type is allowed.\n\nThe `jobSteps` array is placed into the pipeline job at the relevant `jobs.<job_id>.steps` as [documented here](https://docs.github.com/en/actions/using-workflows/workflow-syntax-for-github-actions#jobsjob_idsteps).\n\nIn this example,\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n )\n)\n\n# \"Beta\" stage with a pre-check that uses code from the repo and an action\nstage = MyStage(app, \"Beta\", env=BETA_ENV)\npipeline.add_stage(stage,\n pre=[GitHubActionStep(\"PreBetaDeployAction\",\n job_steps=[JobStep(\n name=\"Checkout\",\n uses=\"actions/checkout@v3\"\n ), JobStep(\n name=\"pre beta-deploy action\",\n uses=\"my-pre-deploy-action@1.0.0\"\n ), JobStep(\n name=\"pre beta-deploy check\",\n run=\"npm run preDeployCheck\"\n )\n ]\n )]\n)\n\napp.synth()\n```\n\n### Configure GitHub Environment\n\nYou can run your GitHub Workflow in select\n[GitHub Environments](https://docs.github.com/en/actions/deployment/targeting-different-environments/using-environments-for-deployment).\nVia the GitHub UI, you can configure environments with protection rules and secrets, and reference\nthose environments in your CDK app. A workflow that references an environment must follow any\nprotection rules for the environment before running or accessing the environment's secrets.\n\nAssuming (just like in the main [example](#usage)) you have a\n[`Stage`](https://docs.aws.amazon.com/cdk/api/v2/docs/aws-cdk-lib.Stage.html)\ncalled `MyStage` that includes CDK stacks for your app and you want to deploy it\nto two AWS environments (`BETA_ENV` and `PROD_ENV`) as well as GitHub Environments\n`beta` and `prod`:\n\n```python\nfrom aws_cdk.pipelines import ShellStep\n\n\napp = App()\n\npipeline = GitHubWorkflow(app, \"Pipeline\",\n synth=ShellStep(\"Build\",\n commands=[\"yarn install\", \"yarn build\"\n ]\n ),\n aws_creds=AwsCredentials.from_open_id_connect(\n git_hub_action_role_arn=\"arn:aws:iam::<account-id>:role/GitHubActionRole\"\n )\n)\n\npipeline.add_stage_with_git_hub_options(Stage(self, \"Beta\",\n env=BETA_ENV\n),\n git_hub_environment=GitHubEnvironment(name=\"beta\")\n)\npipeline.add_stage_with_git_hub_options(MyStage(self, \"Prod\",\n env=PROD_ENV\n),\n git_hub_environment=GitHubEnvironment(name=\"prod\")\n)\n\napp.synth()\n```\n\n#### Waves for Parallel Builds\n\nYou can add a Wave to a pipeline, where each stage of a wave will build in parallel.\n\n> **Note**: The `pipeline.addWave()` call will return a `Wave` object that is actually a `GitHubWave` object, but\n> due to JSII rules the return type of `addWave()` cannot be changed. If you need to use\n> `wave.addStageWithGitHubOptions()` then you should call `pipeline.addGitHubWave()` instead, or you can\n> use `GitHubStage`s to carry the GitHub properties.\n\nWhen deploying to multiple accounts or otherwise deploying mostly-unrelated stacks, using waves can be a huge win.\n\nHere's a relatively large (but real) example, **without** a wave:\n\n<img width=\"1955\" alt=\"without-waves-light-mode\" src=\"https://user-images.githubusercontent.com/386001/217436992-d8e46c23-6295-48ec-b139-add60b1f5a14.png\">\n\nYou can see how dependencies get chained unnecessarily, where the `cUrl` step should be the final step (a test) for an account:\n\n<img width=\"1955\" alt=\"without-waves-deps-light-mode\" src=\"https://user-images.githubusercontent.com/386001/217437074-3c86d88e-6be7-4b10-97b1-6b51b100e4d6.png\">\n\nHere's the exact same stages deploying the same stacks to the same accounts, but **with** a wave:\n\n<img width=\"1955\" alt=\"with-waves\" src=\"https://user-images.githubusercontent.com/386001/217437228-72f6c278-7e97-4a88-91fa-089628ea0381.png\">\n\nAnd the dependency chains are reduced to only what is actually needed, with the `cUrl` calls as the final stage for each account:\n\n<img width=\"1955\" alt=\"deps\" src=\"https://user-images.githubusercontent.com/386001/217437265-1c10cd5f-3c7d-4e3a-af5c-acbdf3acff1b.png\">\n\nFor additional information and a code example see [here](docs/waves.md).\n\n#### Manual Approval Step\n\nOne use case for using GitHub Environments with your CDK Pipeline is to create a\nmanual approval step for specific environments via Environment protection rules.\nFrom the GitHub UI, you can specify up to 5 required reviewers that must approve\nbefore the deployment can proceed:\n\n<img width=\"1134\" alt=\"require-reviewers\" src=\"https://user-images.githubusercontent.com/7248260/163494925-627f5ca7-a34e-48fa-bec7-1e4924ab6c0c.png\">\n\nFor more information and a tutorial for how to set this up, see this\n[discussion](https://github.com/cdklabs/cdk-pipelines-github/issues/162).\n\n### Pipeline YAML Comments\n\nAn \"AUTOMATICALLY GENERATED FILE...\" comment will by default be added to the top\nof the pipeline YAML. This can be overriden as desired to add additional context\nto the pipeline YAML.\n\n```\ndeclare const pipeline: GitHubWorkflow;\n\npipeline.workflowFile.commentAtTop = `AUTOGENERATED FILE, DO NOT EDIT DIRECTLY!\n\nDeployed stacks from this pipeline:\n${STACK_NAMES.map((s)=>`- ${s}\\n`)}`;\n```\n\nThis will generate the normal `deploy.yml` file, but with the additional comments:\n\n```yaml\n# AUTOGENERATED FILE, DO NOT EDIT DIRECTLY!\n\n# Deployed stacks from this pipeline:\n# - APIStack\n# - AuroraStack\n\nname: deploy\non:\n push:\n branches:\n< the rest of the pipeline YAML contents>\n```\n\n## Tutorial\n\nYou can find an example usage in [test/example-app.ts](./test/example-app.ts)\nwhich includes a simple CDK app and a pipeline.\n\nYou can find a repository that uses this example here: [eladb/test-app-cdkpipeline](https://github.com/eladb/test-app-cdkpipeline).\n\nTo run the example, clone this repository and install dependencies:\n\n```shell\ncd ~/projects # or some other playground space\ngit clone https://github.com/cdklabs/cdk-pipelines-github\ncd cdk-pipelines-github\nyarn\n```\n\nNow, create a new GitHub repository and clone it as well:\n\n```shell\ncd ~/projects\ngit clone https://github.com/myaccount/my-test-repository\n```\n\nYou'll need to set up AWS credentials in your environment. Note that this tutorial uses\nlong-lived GitHub secrets as credentials for simplicity, but it is recommended to set up\na GitHub OIDC role instead.\n\n```shell\nexport AWS_ACCESS_KEY_ID=xxxx\nexport AWS_SECRET_ACCESS_KEY=xxxxx\n```\n\nBootstrap your environments:\n\n```shell\nexport CDK_NEW_BOOTSTRAP=1\nnpx cdk bootstrap aws://ACCOUNTID/us-east-1\nnpx cdk bootstrap aws://ACCOUNTID/eu-west-2\n```\n\nNow, run the `manual-test.sh` script when your working directory is the new repository:\n\n```shell\ncd ~/projects/my-test-repository\n~/projects/cdk-piplines/github/test/manual-test.sh\n```\n\nThis will produce a `cdk.out` directory and a `.github/workflows/deploy.yml` file.\n\nCommit and push these files to your repo and you should see the deployment\nworkflow in action. Make sure your GitHub repository has `AWS_ACCESS_KEY_ID` and\n`AWS_SECRET_ACCESS_KEY` secrets that can access the same account that you\nsynthesized against.\n\n> In this tutorial, you are supposed to commit `cdk.out` (i.e. the code is pre-synthed).\n> Do not do this in your app; you should always synth during the synth step of the GitHub\n> workflow. In the example app this is achieved through the `preSynthed: true` option.\n> It is for example purposes only and is not something you should do in your app.\n>\n> ```python\n> from aws_cdk.pipelines import ShellStep\n>\n> pipeline = GitHubWorkflow(App(), \"Pipeline\",\n> synth=ShellStep(\"Build\",\n> commands=[\"echo \\\"nothing to do (cdk.out is committed)\\\"\"]\n> ),\n> # only the example app should do this. your app should synth in the synth step.\n> pre_synthed=True\n> )\n> ```\n\n## Not supported yet\n\nMost features that exist in CDK Pipelines are supported. However, as the CDK Pipelines\nfeature are expands, the feature set for GitHub Workflows may lag behind. If you see a\nfeature that you feel should be supported by GitHub Workflows, please open a GitHub issue\nto track it.\n\n## Contributing\n\nSee [CONTRIBUTING](CONTRIBUTING.md) for more information.\n\n## License\n\nThis project is licensed under the Apache-2.0 License.\n",
"bugtrack_url": null,
"license": "Apache-2.0",
"summary": "GitHub Workflows support for CDK Pipelines",
"version": "0.0.9",
"project_urls": {
"Homepage": "https://github.com/hojulian/cdk-pipelines-github.git",
"Source": "https://github.com/hojulian/cdk-pipelines-github.git"
},
"split_keywords": [],
"urls": [
{
"comment_text": "",
"digests": {
"blake2b_256": "45bdc2d248e78c8181b1115e4ee0695988417ba2f41685e93cba44b467729f05",
"md5": "76ba954fc5add58fd8732a00cadfad65",
"sha256": "8e4c9026e636bfa3a325d70088339b0ff4d1feaf692dc80b8cce672999cfde81"
},
"downloads": -1,
"filename": "hojulian.cdk_pipelines_github-0.0.9-py3-none-any.whl",
"has_sig": false,
"md5_digest": "76ba954fc5add58fd8732a00cadfad65",
"packagetype": "bdist_wheel",
"python_version": "py3",
"requires_python": "~=3.8",
"size": 427473,
"upload_time": "2024-03-06T04:58:59",
"upload_time_iso_8601": "2024-03-06T04:58:59.270206Z",
"url": "https://files.pythonhosted.org/packages/45/bd/c2d248e78c8181b1115e4ee0695988417ba2f41685e93cba44b467729f05/hojulian.cdk_pipelines_github-0.0.9-py3-none-any.whl",
"yanked": false,
"yanked_reason": null
},
{
"comment_text": "",
"digests": {
"blake2b_256": "d3c820c8f3e836ffd6d2a996fbca8506da489193cdd963888d2052298ff93756",
"md5": "e77ad685382f99466e53b6e208a2fbdd",
"sha256": "53adb4de015c264a82a3cb5af2e320ae7a15891265ee9736903317d4390ea3c1"
},
"downloads": -1,
"filename": "hojulian.cdk-pipelines-github-0.0.9.tar.gz",
"has_sig": false,
"md5_digest": "e77ad685382f99466e53b6e208a2fbdd",
"packagetype": "sdist",
"python_version": "source",
"requires_python": "~=3.8",
"size": 436280,
"upload_time": "2024-03-06T04:59:01",
"upload_time_iso_8601": "2024-03-06T04:59:01.521839Z",
"url": "https://files.pythonhosted.org/packages/d3/c8/20c8f3e836ffd6d2a996fbca8506da489193cdd963888d2052298ff93756/hojulian.cdk-pipelines-github-0.0.9.tar.gz",
"yanked": false,
"yanked_reason": null
}
],
"upload_time": "2024-03-06 04:59:01",
"github": true,
"gitlab": false,
"bitbucket": false,
"codeberg": false,
"github_user": "hojulian",
"github_project": "cdk-pipelines-github",
"travis_ci": false,
"coveralls": false,
"github_actions": true,
"lcname": "hojulian.cdk-pipelines-github"
}